#NLTK
Explore tagged Tumblr posts
Text
python iterative monte carlo search for text generation using nltk
You are playing a game and you want to win. But you don't know what move to make next, because you don't know what the other player will do. So, you decide to try different moves randomly and see what happens. You repeat this process again and again, each time learning from the result of the move you made. This is called iterative Monte Carlo search. It's like making random moves in a game and learning from the outcome each time until you find the best move to win.
Iterative Monte Carlo search is a technique used in AI to explore a large space of possible solutions to find the best ones. It can be applied to semantic synonym finding by randomly selecting synonyms, generating sentences, and analyzing their context to refine the selection.
# an iterative monte carlo search example using nltk # https://pythonprogrammingsnippets.tumblr.com import random from nltk.corpus import wordnet # Define a function to get the synonyms of a word using wordnet def get_synonyms(word): synonyms = [] for syn in wordnet.synsets(word): for l in syn.lemmas(): if '_' not in l.name(): synonyms.append(l.name()) return list(set(synonyms)) # Define a function to get a random variant of a word def get_random_variant(word): synonyms = get_synonyms(word) if len(synonyms) == 0: return word else: return random.choice(synonyms) # Define a function to get the score of a candidate sentence def get_score(candidate): return len(candidate) # Define a function to perform one iteration of the monte carlo search def monte_carlo_search(candidate): variants = [get_random_variant(word) for word in candidate.split()] max_candidate = ' '.join(variants) max_score = get_score(max_candidate) for i in range(100): variants = [get_random_variant(word) for word in candidate.split()] candidate = ' '.join(variants) score = get_score(candidate) if score > max_score: max_score = score max_candidate = candidate return max_candidate initial_candidate = "This is an example sentence." # Perform 10 iterations of the monte carlo search for i in range(10): initial_candidate = monte_carlo_search(initial_candidate) print(initial_candidate)
output:
This manufacture Associate_in_Nursing theoretical_account sentence. This fabricate Associate_in_Nursing theoretical_account sentence. This construct Associate_in_Nursing theoretical_account sentence. This cathode-ray_oscilloscope Associate_in_Nursing counteract sentence. This collapse Associate_in_Nursing computed_axial_tomography sentence. This waste_one's_time Associate_in_Nursing gossip sentence. This magnetic_inclination Associate_in_Nursing temptingness sentence. This magnetic_inclination Associate_in_Nursing conjure sentence. This magnetic_inclination Associate_in_Nursing controversy sentence. This inclination Associate_in_Nursing magnetic_inclination sentence.
#python#nltk#iterative monte carlo search#monte carlo search#monte carlo#search#text generation#generative text#text#generation#text prediction#synonyms#synonym#semantics#semantic#language#language model#ai#iterative#iteration#artificial intelligence#sentence rewriting#sentence rewrite#story generation#deep learning#learning#educational#snippet#code#source code
2 notes
·
View notes
Text
El arte de escribir bien (y algunas estadísticas al respecto)
El arte de escribir bien (y algunas estadísticas al respecto)
Desde hace un tiempo, muy a menudo me sorprendo a mí mismo maravillado frente a un texto. Pero no porque la historia que cuenta sea impresionante o me esté dando información súper interesante. A medida que mi lista de lecturas ha ido creciendo, también lo ha hecho mi gozo al encontrar pasajes particularmente bien escritos. Además, durante los últimos años he invertido muchas horas intentando…
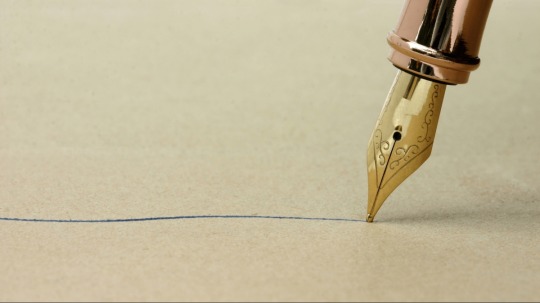
View On WordPress
#Escritura#Flow#Lenguaje#Libros#Macro#Natural Language Processing Toolkit#NLTK#Python#Ritmo#Texto#Visual Basic#Word
2 notes
·
View notes
Text
Resource punkt_tab not found - for NLTK (NLP)
Over the past few years, I see that quite a few folks are STILL getting this error in Jupyter Notebook. And it means, again, a lot of troubleshooting on my part. The instructor for the course I am taking (one of several Gen AI courses) did not get that error or they have an environment set up for that specific .ipynb file. And as such, they did not comment on it. After I did that last…
View On WordPress
0 notes
Text
El concepto de los diccionarios de sentimientos y cómo son fundamentales en el análisis de sentimientos.
¿Qué son los diccionarios de sentimientos y cómo funcionan? Imagina un diccionario, pero en lugar de definir palabras, clasifica las palabras según la emoción que expresan. Estos son los diccionarios de sentimientos. Son como una especie de “tesauro emocional” que asigna a cada palabra una puntuación que indica si es positiva, negativa o neutral. ¿Cómo funcionan? Lexicón: Contienen una extensa…
#alicante#análisis de sentimientos#anotación manual#aprendizaje automático#comunidad valenciana#contexto#corpus#diccionarios de sentimientos#empresas locales.#F1-score#gobierno#Google Cloud Natural Language API#herramientas de análisis de sentimientos#IBM Watson#inteligencia artificial#intensidad#MonkeyLearn#NLTK#polaridad#precisión#procesamiento del lenguaje natural#RapidMiner#recall#redes neuronales#spaCy#turismo
0 notes
Text
In this tutorial, we will explore how to perform sentiment analysis using Python with three popular libraries — NLTK, TextBlob, and VADER.
#machine learning#data science#python#sentiment analysis#natural language processing#NLTK#TextBlob#VADER#tutorial#medium#medium writers#artificial intelligence#ai#data analysis#data scientist#data analytics#computer science
1 note
·
View note
Text
Not me out here writing a program to log into my AO3 account and perform a natural language sentiment analysis of the comments in my inbox to identify trolls without having to read their garbage...
#trolls begone#that's what i get for daring to write fic with darker themes...#this was a fun lil project tho#here's the stack i used:#python3#nltk + vader sentiment analyzer#beautifulsoup4 to grok html#python-requests to wrangle cookies
3 notes
·
View notes
Text
#nlp libraries#natural language processing libraries#python libraries#nodejs nlp libraries#python and libraries#javascript nlp libraries#best nlp libraries for nodejs#nlp libraries for java script#best nlp libraries for javascript#nlp libraries for nodejs and javascript#nltk library#python library#pattern library#python best gui library#python library re#python library requests#python library list#python library pandas#python best plotting library
0 notes
Text
in what situation is the word "oh" a proper noun
#🍯 talks#using nltk for my project and skimming through to fix some mistakes#and it keeps tagging oh as nnp
1 note
·
View note
Text
Can't believe I had to miss my morphology lecture because comp sci has no concept of timetables
#it's so sad#i literally only took it as a minor to get a leg up on python for nltk#and i'm doing intensive french with it as well so i'm swamped and i'm only two weeks in#bro i just wanna do linguistics :(
0 notes
Text

Want to make NLP tasks a breeze? Explore how NLTK streamlines text analysis in Python, making it easier to extract valuable insights from your data. Discover more https://bit.ly/487hj9L
0 notes
Text
part of speech tagging? oh man sorry i though u meant piece of shit tagging
0 notes
Text
python keyword extraction using nltk wordnet
import re # include wordnet.morphy from nltk.corpus import wordnet # https://pythonprogrammingsnippets.tumblr.com/ def get_non_plural(word): # return the non-plural form of a word # if word is not empty if word != "": # get the non-plural form non_plural = wordnet.morphy(word, wordnet.NOUN) # if non_plural is not empty if non_plural != None: # return the non-plural form # print(word, "->", non_plural) return non_plural # if word is empty or non_plural is empty return word def get_root_word(word): # return the root word of a word # if word is not empty if word != "": word = get_non_plural(word) # get the root word root_word = wordnet.morphy(word) # if root_word is not empty if root_word != None: # return the root word # print(word, "->", root_word) word = root_word # if word is empty or root_word is empty return word def process_keywords(keywords): ret_k = [] for k in keywords: # replace all characters that are not letters, spaces, or apostrophes with a space k = re.sub(r"[^a-zA-Z' ]", " ", k) # if there is more than one whitespace in a row, replace it # with a single whitespace k = re.sub(r"\s+", " ", k) # remove leading and trailing whitespace k = k.strip() k = k.lower() # if k has more than one word, split it into words and add each word # back to keywords if " " in k: ret_k.append(k) # we still want the original keyword k = k.split(" ") for k2 in k: #if not is_adjective(k2): ret_k.append(get_root_word(k2)) ret_k.append(k2.strip()) else: # if not is_adjective(k): ret_k.append(get_root_word(k)) ret_k.append(k.strip()) # unique ret_k = list(set(ret_k)) # remove empty strings ret_k = [k for k in ret_k if k != ""] # remove all words that are less than 3 characters ret_k = [k for k in ret_k if len(k) >= 3] # remove words like 'and', 'or', 'the', etc. ret_k = [k for k in ret_k if k not in ["and", "or", "the", "a", "an", "of", "to", "in", "on", "at", "for", "with", "from", "by", "as", "into", "like", "through", "after", "over", "between", "out", "against", "during", "without", "before", "under", "around", "among", "throughout", "despite", "towards", "upon", "concerning", "of", "to", "in", "on", "at", "for", "with", "from", "by", "as", "into", "like", "through", "after", "over", "between", "out", "against", "during", "without", "before", "under", "around", "among", "throughout", "despite", "towards", "upon", "concerning", "this", "that", "these", "those", "is", "are", "was", "were", "be", "been", "being", "have", "has", "had", "having", "do", "does", "did", "doing", "will", "would", "shall", "should", "can", "could", "may", "might", "must", "ought", "i", "me", "my", "mine", "we", "us", "our", "ours", "you", "your", "yours", "he", "him", "his", "she", "her", "hers", "it", "its", "they", "them", "their", "theirs", "what", "which", "who", "whom", "whose", "this", "that", "these", "those", "myself", "yourself", "himself", "herself", "itself", "ourselves", "yourselves", "themselves", "whoever", "whatever", "whomever", "whichever", "whichever" ]] return ret_k def extract_keywords(paragraph): if " " in paragraph: return paragraph.split(" ") return [paragraph]
example usage:
the_string = "Jims House of Judo and Karate is a martial arts school in the heart of downtown San Francisco. We offer classes in Judo, Karate, and Jiu Jitsu. We also offer private lessons and group classes. We have a great staff of instructors who are all black belts. We have been in business for over 20 years. We are located at 123 Main Street." keywords = process_keywords(extract_keywords(the_string)) print(keywords)
output:
# output: ['jims', 'instructors', 'class', 'lesson', 'all', 'school', 'san', 'martial', 'classes', 'karate', 'great', 'lessons', 'downtown', 'private', 'arts', 'also', 'locate', 'belts', 'business', 'judo', 'years', 'located', 'main', 'street', 'jitsu', 'house', 'offer', 'staff', 'group', 'heart', 'instructor', 'belt', 'black', 'francisco', 'jiu']
#python#keyword extraction#keywords#keyword#extraction#natural language processing#natural language#nltk#natural language toolkit#wordnet#morphy#keyword creation#seo#keyword maker#keywording#depluralization#plurals#pluralize#filtering#language processing#text processing#data processing#data#text#paragraph#regex#geek#nerd#nerdy#geeky
1 note
·
View note
Text
Using NLP for Advanced Business Analytics Strategies
Introduction:
In this age of digital transformation, businesses generate a tremendous amount of data daily. While it is easier to analyze structured data like sales figures or the number of people visiting a website, unstructured data, such as messages posted by customers on social media, emails, or reviews, contains huge potential for insights. That's where Natural Language Processing—a subset of AI—comes into play.
What is Natural Language Processing?
NLP is a subdomain of AI that focuses on how computers interact with humans through language. Its primary objective is that machines interpret, understand, and create responses similar to human beings in written or verbal communication. Some examples of the application of NLP include sentiment analysis, chatbots, text summarization, and predictive modeling.
NLP is important in business analytics because it enables organizations to unlock actionable insights from unstructured data, enhancing decision-making and efficiency.
Applications of NLP in Business Analytics:
1- Customer Sentiment Analysis
There is a high requirement to understand the emotions of customers so that proper services and products can be provided. NLP allows analyzing customer reviews, feedback, and social media comments to determine whether customers are satisfied, neutral, or dissatisfied. For instance, text classification allows the concerned e-commerce websites to enhance their user experience of the service by the emotions of the customers.
2- Text Classification for Better Insights
Most businesses usually handle extensive data, ranging from emails and tickets to a variety of documents. NLP, in this aspect, classifies texts to categorize your data for more convenient management automatically. Companies can easily make their analysis a little easier for processing customer support tickets or survey responses.
3- Predictive Analytics
Textual data integrates NLP with predictive modelling to predict the future based on the integration of such data. For example, sales emails or queries from customers within a given period can help in identifying patterns of buying.
4- Chatbots and Virtual Assistants
Customer support is being revolutionized with the help of NLP-based chatbots and virtual assistants. They can provide answers, resolve problems, and even offer customer suggestions for a more seamless experience while reducing costs involved in the operation.
5- Market Research and Competitive Analysis
NLP helps scan through social media, news articles, and forums for industry trends and competitors' strategies. This helps evaluate market positioning and what to expect from your customers.
Why Businesses Need NLP for Analytics:
It captures the data in numbers. So, in present times, if 80% of data comes out unstructured, this thing is very highly missed from a perspective point. NLP filled in the gap by helping companies process and analyze unstructured data in record time.
For a professional who wants to get deep into this integration, a Business Analytics Course in Hyderabad can prove to be knowledge and skill-gaining for the appropriate utilization of NLP tools.
Advantages of NLP in Business Analytics:
Superior Decision Making: Decisions made based on customer sentiment and trend analysis through NLP can go in alignment with the market.
Better Customer Experience: Sentiment insights can gauge customer needs, and therefore, business enterprises may develop better services.
Better Operational Efficiency: Tasks that could get automated might also comprise ticket categorization or chat assistance to save time, along with an overall amount of effort done as human input
Immediate Insights: By processing data in real-time, NLP helps business enterprises react promptly to changes in customer behavior or any market trend.
Tools and Technologies for NLP in Business Analytics:
Python Libraries: NLTK, spaCy, and TextBlob are used the most for NLP tasks.
Google Natural Language AI: It is a cloud-based NLP tool that analyzes text.
IBM Watson: Offers NLP capabilities that assist in sentiment analysis, keyword extraction, and many more.
Microsoft Azure Text Analytics: A suite of NLP tools for business.
Adding these tools to your arsenal can be a game-changer in business analytics. For those interested, a Business Analytics Course in Hyderabad can teach these tools and provide hands-on training.
Challenges in Implementing NLP:
Data Quality Issues: The output from NLP is prone to poor-quality or biased data.
Language Nuances: Capturing idioms and language along with culture are very tough for the NLP model.
Heavy Computing Requirement: The NLP models take an extensive time period for training along with requiring more computational resources.
Constant Updates: Updating in NLP is highly continuous. Languages are always evolving, and models require periodic updates to keep pace.
Even though these problems seem to dominate, the benefit of NLP outweighs its drawbacks when appropriately implemented.
NLP in the Future of Business Analytics:
Future NLP trends are going to involve developments like deep learning and transformer models, e.g., GPT, and BERT. Deep learning and transformer models are increasing the efficiency and accuracy of NLP. Adopting NLP will give business houses a competitive edge because they'll be equipped with better analytics capabilities to derive decisions from unstructured data.
This would set ambitious analysts and professionals ahead in their curve because it teaches how the latest NLP techniques could be integrated into one's workflow from a Business Analytics Course in Hyderabad.
Conclusion:
Business data analysis is being transformed with the integration of natural language processing. Deep insights are extracted from unstructured sources, from the sentiment analysis of customers to predictive analytics, all falling within the domain of NLP business analytics applications. Businesses will have better customer insight and an ability to outcompete their competition with improved decision-making skills as they implement this technology and evolve.
For those interested in specializing in this field, the Business Analytics Course in Hyderabad will equip them with sufficient expertise to emerge as great performers in analytics led by NLP.
0 notes
Text
How To Create A Chatbot Using Python : A Comprehensive Guide

Introduction
Chatbots are commonplace in our digital world changing the way we interact with business and technology. From customer support queries to informal conversations chatbots are being increasingly incorporated into our everyday life. Python thanks to its extensive library and framework libraries is the most popular programming language to build these intelligent chatbots.
This thorough guide will go deeper into the intricate details of chatbot development with Python and will explore the most important considerations advanced techniques, as well as the best practices for creating highly engaging and efficient chatbots.
1-Defining Objectives and Target Audience
Before launching chatbot development it is essential to have a clear knowledge of the primary goals and the intended audience is essential.
Crystal-Clear Objectives:
Definition of the Scope Define the scope chatbot's primary role. Are they designed to offer 24/7 support for customers or respond to frequently-asked questions (FAQs) or schedule appointments, give specific product recommendations or simply engage in chat?
Identification of the Key Performance Indicators (KPIs) Decide the metrics for success. Are you looking to cut down on customer support ticketing or boost lead generation, increase the satisfaction of customers, or boost the brand's engagement? Setting clear KPIs will help guide your design process and help facilitate efficient evaluation.
Understanding Your Audience:
Demographics and psychographics: Perform extensive research to determine the demographics of your audience (age or place of residence job) as well as psychographics (interests and values, behavior,).
Communication preferences: Determine what your intended audience prefers to communicate. They may be more at ease using text-based communication or voice commands or a mixture of both?
Tone and Language: Adjust the chatbot's tone and language to be able to connect with your targeted people. A formal and professional tone may be appropriate to a bank however a more casual and relaxed tone may be more appropriate for a social media site.
2-Architecting the Conversational Experience: Choosing the Right Approach
The choice of the architecture affects the chatbot's capabilities as well as its complexity.
Rule-Based Systems:
Pros: Very easy to set up, it is ideal for dealing with simple interaction and questions.
Cons: Lack of flexibility, unable to adjust to new inputs from users or complex conversations.
An example: A chatbot that can answer frequently asked questions on the company's policies.
Machine Learning-Powered Chatbots:
Pros: Learn by observing user conversations, be able to adapt to changing circumstances and offer more natural conversations.
Cons: Requires a lot of computation and training information.
Example: A client service chatbot that is able to learn from previous interactions to give more precise and useful responses.
Deep Learning-Based Chatbots:
Pros: Utilize sophisticated deep-learning models (e.g., Recurrent Neural Networks, Transformers) for sophisticated language comprehension and generation.
Cons: They require a large amount of computation power and huge databases to ensure optimal performance.
Examples: A chatbot which can write creatively and poetry writing or even translate different languages.
Hybrid Approaches:
The pros: Combining the advantages of machine learning and rule-based techniques, allowing a combination of control and scalability.
Cons: It can be more complicated to create and implement.
Example Chatbots that use rules-based logic to begin interactions before transferring to machine learning to answer more complicated queries.
3-Leveraging the Power of Python Libraries
Python's extensive ecosystem provides an array of tools to help chatbot developers.
NLTK (Natural Natural Language Toolkit) The NLTK (Natural Language Toolkit) is a complete library of NLP tasks, such as stemming, tokenization speech tagging, part-of-speech tagging entity recognition, as well as sentiment analysis.
spaCy is a fast and effective NLP library praised for its precision and user-friendliness.
Rasa: A free-of-cost framework for creating conversational AI that provides tools for managing dialogue machines learning models and integration with different channels.
Dialogflow is a powerful platform to build dialogic interfaces that provide an easy-to-use interface, built-in integrations, as well as access to the latest NLP capabilities.
ChatterBot ChatterBot is a simple and user-friendly library for the creation of machine learning and rule-based chatbots that are ideal for novices.
Transformers (Hugging Face): A high-tech library to work with transformer models. It offers the most cutting-edge features like sophisticated language comprehension and the generation.
4-Designing the Conversational Flow: Mapping User Journeys
Personas and User Stories Create user stories that are detailed to comprehend the user's needs and expectations. Personas for users are created to reflect different user segments and their traits.
Intents and Entities: Identify intentions (user desires, e.g., "order food," "check weather") and entities (specific details, e.g., "pizza," "location") to be able to understand user input.
Dialog Flowcharts: See flow of conversation using flowcharts and state diagrams to show various conversation routes as well as user inputs, chatbot replies and other decisions.
Contextual Understanding: Use mechanisms to keep the context of conversations. For instance when a user inquires about a specific item the chatbot must be able to recall that product during subsequent interactions.
5-Building and Training the Chatbot Model
Data Collection and Preparation Collect high-quality training data which could include transcripts of conversations, customer service tickets, or other publicly accessible datasets. Clean and prepreprocess the data by eliminating noise processing punctuation, handling, and converting text into lowercase.
Learning and Model Selection: Select the most appropriate models for learning by machine (e.g. the Support Vector Machines, Naive Bayes, Recurrent Neural Networks) Based on the chatbot's complexity and the data available. Train the model using the data you have prepared using methods like supervised learning, unsupervised learning or reinforcement learning.
Hyperparameter Tuning: Fine-tune the model parameters to increase the performance and accuracy. Test different hyperparameters to determine the optimal combination for your particular use situation.
Assessment and testing: assess the performance of your chatbot using measures like precision, accuracy recall, F1-score and satisfaction of users. Conduct extensive testing using different scenarios and inputs from users to find and fix any issues.
6-Integration and Deployment
Platform Selection: Select the best platform to run the chatbot. This could be an app for messaging, a website (e.g., Facebook Messenger, WhatsApp), voice assistant (e.g., Amazon Alexa, Google Assistant), or a custom-built application.
API Integration: Use APIs that are specific to your platform to seamlessly integrate chatbots and manage interactions efficiently.
Hosting and deployment: Move the chatbot in the right environment, for example, cloud platforms (e.g., AWS, Azure, Google Cloud) or servers on premises.
Scalability and Maintenance: Make sure the chatbot is able to handle growing users and keep high availability. Create the system to allow for simple maintenance and upgrades.
7-Continuous Improvement and Monitoring
User Feedback Collection: Collect feedback from your users via surveys or in-app feedback mechanisms or through social media channels.
Performance Monitoring: Keep track of the most important metrics, such as customer engagement, conversation success rates, as well as customer satisfaction to pinpoint areas for improvement.
Regular Maintenance and Updates Chatbots should be updated regularly with fresh data, enhance its communication capabilities and tackle any issues that arise.
A/B Testing: Perform A/B testing to play around with different conversation flow patterns as well as features, responses, and features to improve the user experience.
Key Considerations for Advanced Chatbot Development:
Personalization: Customize the chatbot's responses according to user preferences and previous interactions.
Multilingual Support: Set chatbots to understand and respond to messages in a variety of languages in order to reach a wider population.
Accessibility: Ensure that the chatbot is accessible to people who have disabilities (e.g. screen readers or the keyboard).
Data Security and Privacy Take effective security measures to safeguard personal information of users and to comply with the relevant privacy laws.
Emotional Intelligence: Provide your chatbot with capacity to recognize and respond to emotions of the user like anger or frustration. anger.
Proactive Engagement: Set chatbots to interact with users, for example by giving personalized suggestions or anticipating user requirements.
8-Chatbot employing the OpenAI API Python
Utilizing the potential of OpenAI's API in your Python chatbot will allow you to make important advancements in conversational AI. Through integrating models like GPT to your chatbot, you can accomplish:
More human-like and more coherent responses.
Improved language comprehension and capability to generate.
Increased capacity to manage complicated and complex conversations.
How Xcelore Can Help You ?
Xcelore, a leading AI development company , specializes in creating cutting-edge AI-powered solutions. Our expertise encompasses:
Custom Chatbot Development: We tailor chatbot solutions to your specific business needs, ensuring they align with your brand and achieve your desired outcomes.
AI development services: Our team of skilled AI engineers leverages advanced AI technologies, including machine learning, deep learning, and NLP, to build intelligent and sophisticated chatbots.
Chatbot using OpenAI API Python: We seamlessly integrate OpenAI's powerful language models into your Python chatbot, unlocking advanced conversational capabilities and enhancing user experiences.
By partnering with Xcelore, you gain access to:
Industry-leading expertise: Our team of AI/ML experts possesses deep knowledge and experience in chatbot development.
Customized solutions: We tailor our approach to your unique requirements and business objectives.
Cutting-edge technologies: We leverage the latest AI advancements to deliver state-of-the-art chatbot solutions.
Proven track record: We have a successful history of delivering high-quality AI solutions to clients across various industries.
Conclusion
The development of a successful chatbot requires an array of factors that include carefully planned planning, a thorough knowledge of NLP techniques, as well as an emphasis on user-centric design. Through leveraging the potential that is Python and its extensive libraries developers can build sophisticated and interactive chatbots that transform customer interactions improve user experiences and help drive the growth of businesses.
Ready to embark on your chatbot development journey? Explore the resources and libraries mentioned in this blog post, or consider engaging professional chatbot development services to bring your vision to life.I hope this blog post provides a helpful overview of how to create a chatbot using Python. Feel free to ask if you have any further questions.
0 notes
Text
How to Build AI Agents: A Step-by-Step Guide
In recent years, AI agents have emerged as powerful tools that are revolutionizing industries, improving customer experiences, and enhancing automation across various domains. AI agents are autonomous systems that use artificial intelligence to perform tasks, make decisions, and interact with users without requiring direct human intervention. Whether you're looking to build an AI agent for your business, develop a personal assistant, or create a chatbot, this step-by-step guide will walk you through the process of building an AI agent from scratch.

Step 1: Define the Purpose of Your AI Agent
Before diving into the development of your AI agent development, the first and most crucial step is to define its purpose. What problem will your AI agent solve? What specific tasks will it perform, and what kind of interactions will it have with users?
An AI agent can serve many purposes, such as:
Customer support agents: Helping users resolve issues or answer questions.
Personal assistants: Managing schedules, setting reminders, and performing tasks.
Recommendation systems: Suggesting products, services, or content based on user preferences.
Data analysis agents: Analyzing large datasets and providing insights.
Defining your AI agent's purpose helps determine the tools, technologies, and algorithms you need to employ in the development process.
Step 2: Choose the Right AI Technologies
There are several AI technologies and techniques you can use to develop an AI agent, and selecting the right one depends on the type of tasks your agent will perform. Here are the most commonly used AI technologies in AI agent development:
a. Natural Language Processing (NLP)
NLP is essential for AI agents that need to understand and process human language. Whether you're building a chatbot, a voice assistant, or any system that requires language understanding, NLP algorithms allow your agent to interpret text and spoken input. Key NLP techniques include:
Text classification: Identifying the intent behind user input.
Named entity recognition: Extracting specific data points (e.g., names, dates).
Sentiment analysis: Understanding the emotion or sentiment behind a user's words.
Popular NLP libraries include spaCy, NLTK, and Transformers.
b. Machine Learning (ML)
Machine learning is crucial for enabling AI agents to learn from experience and improve over time. There are several approaches to machine learning that can be used to train your agent:
Supervised learning: Training the agent with labeled data to predict outcomes.
Unsupervised learning: Identifying patterns or clusters within data without labeled examples.
Reinforcement learning: Teaching the agent to make decisions based on rewards and punishments.
Popular machine learning frameworks include TensorFlow, PyTorch, and scikit-learn.
c. Deep Learning
Deep learning is a subset of machine learning that focuses on neural networks with many layers. It is particularly useful for tasks such as image recognition, speech recognition, and complex decision-making. For example, deep learning can be used in AI agents that require advanced pattern recognition or the ability to process large volumes of unstructured data.
Popular deep learning frameworks include Keras, TensorFlow, and PyTorch.
Step 3: Design Your AI Agent’s Architecture
Once you've defined the purpose and selected the AI technologies, the next step is to design the architecture of your AI agent. The architecture defines how your agent will process information, interact with users, and make decisions. Here’s a breakdown of the key components to consider in your agent’s architecture:
a. Input Layer
The input layer receives data from users, which could come in the form of text, voice, or other formats. If you're building a chatbot, the input might be text entered by the user. For voice assistants, you might need to convert speech to text using speech recognition algorithms.
b. Processing Layer
The processing layer is where the magic happens. This layer uses AI algorithms such as NLP or machine learning models to understand the input and determine the appropriate response. Depending on the complexity of your agent, this layer may include:
Intent recognition: Identifying what the user wants to do (e.g., ask for the weather, set a reminder).
Response generation: Crafting a suitable response based on the recognized intent.
Learning mechanism: Continuously improving the agent’s responses and capabilities through training.
c. Output Layer
The output layer is where the agent communicates its response to the user. This could be in the form of text (e.g., a chatbot's reply) or speech (e.g., a voice assistant's spoken response).
Step 4: Develop the Core Features of Your AI Agent
Now that you've designed the architecture, it’s time to start building the core features of your AI agent. Below are the essential features you need to develop for your agent:
a. Natural Language Understanding (NLU)
The NLU component allows your AI agent to understand and interpret human language. This is where NLP algorithms come into play. NLU involves:
Intent recognition: Understanding the user’s intent, such as asking for the weather or making a purchase.
Entity extraction: Extracting relevant information, like names, dates, or locations, from the input text.
Libraries like spaCy and Dialogflow are excellent for implementing NLU.
b. Dialog Management
The dialog management system helps your AI agent manage ongoing conversations with users. It determines how the agent should respond based on context, previous user interactions, and available knowledge. This component ensures that your agent provides relevant and coherent answers.
c. Decision Making
For more advanced AI agents, you need to integrate decision-making capabilities. This could involve implementing a rule-based system, using machine learning models for decision support, or even employing reinforcement learning to improve decision-making over time.
Step 5: Train Your AI Agent
Training is a critical step in building an AI agent. The more data your agent has to learn from, the better it can understand and respond to user requests. Here’s how you can approach training:
Gather Data: Collect data that matches the type of interactions your agent will have. This might include text or voice conversations, domain-specific information, and feedback from users.
Label Data: If you're using supervised learning, label the data to help the agent learn how to identify intents and entities.
Train Models: Use machine learning or deep learning techniques to train the agent on your data. This will involve splitting your data into training, validation, and test sets.
You can also use pre-trained models (e.g., GPT-3, BERT) and fine-tune them to suit your specific use case.
Step 6: Test and Deploy Your AI Agent
Once the AI agent is trained, it's essential to test it in real-world scenarios. During testing, you can assess the agent’s performance, identify areas for improvement, and ensure that it meets user expectations.
Beta testing: Allow real users to interact with the agent and provide feedback.
Iterative improvements: Use the feedback to make adjustments to the agent’s performance, understanding, and responses.
Once you're satisfied with the testing phase, you can deploy your AI agent on your preferred platform, whether it’s a website, mobile app, or virtual assistant.
Step 7: Monitor and Improve
Building an AI agent is not a one-time process. After deployment, you need to continuously monitor the agent’s performance and make improvements. Collect feedback, track user interactions, and update your models periodically to ensure the agent stays accurate and relevant.
Conclusion
Building an AI agent can be an exciting and rewarding process, but it requires a combination of technical expertise, careful planning, and iterative improvements. By following this step-by-step guide, you’ll be well on your way to creating an intelligent and functional AI agent that can enhance automation, improve customer service, and deliver better user experiences. Remember that AI agent development is an ongoing process, and the more data you provide, the smarter and more efficient your agent will become.
0 notes
Text
"Hands-On Text Preprocessing with NLTK and spaCy for NLP Applications"
Introduction Hands-On Text Preprocessing with NLTK and spaCy for NLP Applications is a crucial step in Natural Language Processing (NLP) that involves cleaning, normalizing, and transforming raw text data into a format that can be used for analysis, modeling, and machine learning tasks. In this tutorial, we will cover the technical aspects of text preprocessing using two popular NLP libraries:…
0 notes