#Java UI
Explore tagged Tumblr posts
Text
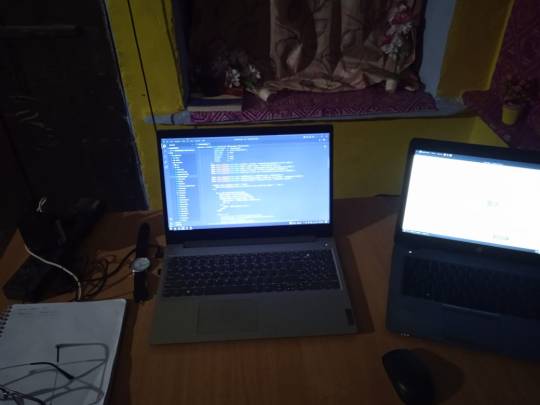
Technology talent sourcing
In a world where diversity and inclusion are more important than ever, it is crucial for companies to prioritize workplace equality. As we look ahead to 2024, supporting women in the workforce is not only a moral imperative but also a strategic business decision. In this blog post, we will explore what companies need to know about creating an environment that empowers and champions women in the workplace. From closing the gender pay gap to fostering inclusive leadership opportunities, let’s dive into how organizations can truly support and uplift all employees for success in the years to come.
#IT staffing Tech recruitment#IT job placement#Technology staffing agency#IT talent acquisition#Tech staffing solutions#IT staffing services#IT job agency#Technology talent sourcing#IT staffing firm#Tech workforce solutions#IT staffing consultancy#Technology recruitment agency#IT staffing specialists#Tech employment agency#Contract hiring#Permanent Hiring#Fulltime Hiring#Contract to Hire#Corp to Corp#C2H#Bigdata Hiring#Cloud Serviecs Hiring#Google GCP Hiring#Web Services hiring#Technology Hiring#IAM hiring#Identity access management#AWS Hiring#Java Backened#Java UI
0 notes
Text
JavaScript Fundamentals
I have recently completed a course that extensively covered the foundational principles of JavaScript, and I'm here to provide you with a concise overview. This post will enable you to grasp the fundamental concepts without the need to enroll in the course.
Prerequisites: Fundamental HTML Comprehension
Before delving into JavaScript, it is imperative to possess a basic understanding of HTML. Knowledge of CSS, while beneficial, is not mandatory, as it primarily pertains to the visual aspects of web pages.
Manipulating HTML Text with JavaScript
When it comes to modifying text using JavaScript, the innerHTML function is the go-to tool. Let's break down the process step by step:
Initiate the process by selecting the HTML element whose text you intend to modify. This selection can be accomplished by employing various DOM (Document Object Model) element selection methods offered by JavaScript ( I'll talk about them in a second )
Optionally, you can store the selected element in a variable (we'll get into variables shortly).
Employ the innerHTML function to substitute the existing text with your desired content.
Element Selection: IDs or Classes
You have the opportunity to enhance your element selection by assigning either an ID or a class:
Assigning an ID:
To uniquely identify an element, the .getElementById() function is your go-to choice. Here's an example in HTML and JavaScript:
HTML:
<button id="btnSearch">Search</button>
JavaScript:
document.getElementById("btnSearch").innerHTML = "Not working";
This code snippet will alter the text within the button from "Search" to "Not working."
Assigning a Class:
For broader selections of elements, you can assign a class and use the .querySelector() function. Keep in mind that this method can select multiple elements, in contrast to .getElementById(), which typically focuses on a single element and is more commonly used.
Variables
Let's keep it simple: What's a variable? Well, think of it as a container where you can put different things—these things could be numbers, words, characters, or even true/false values. These various types of stuff that you can store in a variable are called DATA TYPES.
Now, some programming languages are pretty strict about mentioning these data types. Take C and C++, for instance; they're what we call "Typed" languages, and they really care about knowing the data type.
But here's where JavaScript stands out: When you create a variable in JavaScript, you don't have to specify its data type or anything like that. JavaScript is pretty laid-back when it comes to data types.
So, how do you make a variable in JavaScript?
There are three main keywords you need to know: var, let, and const.
But if you're just starting out, here's what you need to know :
const: Use this when you want your variable to stay the same, not change. It's like a constant, as the name suggests.
var and let: These are the ones you use when you're planning to change the value stored in the variable as your program runs.
Note that var is rarely used nowadays
Check this out:
let Variable1 = 3; var Variable2 = "This is a string"; const Variable3 = true;
Notice how we can store all sorts of stuff without worrying about declaring their types in JavaScript. It's one of the reasons JavaScript is a popular choice for beginners.
Arrays
Arrays are a basically just a group of variables stored in one container ( A container is what ? a variable , So an array is also just a variable ) , now again since JavaScript is easy with datatypes it is not considered an error to store variables of different datatypeslet
for example :
myArray = [1 , 2, 4 , "Name"];
Objects in JavaScript
Objects play a significant role, especially in the world of OOP : object-oriented programming (which we'll talk about in another post). For now, let's focus on understanding what objects are and how they mirror real-world objects.
In our everyday world, objects possess characteristics or properties. Take a car, for instance; it boasts attributes like its color, speed rate, and make.
So, how do we represent a car in JavaScript? A regular variable won't quite cut it, and neither will an array. The answer lies in using an object.
const Car = { color: "red", speedRate: "200km", make: "Range Rover" };
In this example, we've encapsulated the car's properties within an object called Car. This structure is not only intuitive but also aligns with how real-world objects are conceptualized and represented in JavaScript.
Variable Scope
There are three variable scopes : global scope, local scope, and function scope. Let's break it down in plain terms.
Global Scope: Think of global scope as the wild west of variables. When you declare a variable here, it's like planting a flag that says, "I'm available everywhere in the code!" No need for any special enclosures or curly braces.
Local Scope: Picture local scope as a cozy room with its own rules. When you create a variable inside a pair of curly braces, like this:
//Not here { const Variable1 = true; //Variable1 can only be used here } //Neither here
Variable1 becomes a room-bound secret. You can't use it anywhere else in the code
Function Scope: When you declare a variable inside a function (don't worry, we'll cover functions soon), it's a member of an exclusive group. This means you can only name-drop it within that function. .
So, variable scope is all about where you place your variables and where they're allowed to be used.
Adding in user input
To capture user input in JavaScript, you can use various methods and techniques depending on the context, such as web forms, text fields, or command-line interfaces.We’ll only talk for now about HTML forms
HTML Forms:
You can create HTML forms using the <;form> element and capture user input using various input elements like text fields, radio buttons, checkboxes, and more.
JavaScript can then be used to access and process the user's input.
Functions in JavaScript
Think of a function as a helpful individual with a specific task. Whenever you need that task performed in your code, you simply call upon this capable "person" to get the job done.
Declaring a Function: Declaring a function is straightforward. You define it like this:
function functionName() { // The code that defines what the function does goes here }
Then, when you need the function to carry out its task, you call it by name:
functionName();
Using Functions in HTML: Functions are often used in HTML to handle events. But what exactly is an event? It's when a user interacts with something on a web page, like clicking a button, following a link, or interacting with an image.
Event Handling: JavaScript helps us determine what should happen when a user interacts with elements on a webpage. Here's how you might use it:
HTML:
<button onclick="FunctionName()" id="btnEvent">Click me</button>
JavaScript:
function FunctionName() { var toHandle = document.getElementById("btnEvent"); // Once I've identified my button, I can specify how to handle the click event here }
In this example, when the user clicks the "Click me" button, the JavaScript function FunctionName() is called, and you can specify how to handle that event within the function.
Arrow functions : is a type of functions that was introduced in ES6, you can read more about it in the link below
If Statements
These simple constructs come into play in your code, no matter how advanced your projects become.
If Statements Demystified: Let's break it down. "If" is precisely what it sounds like: if something holds true, then do something. You define a condition within parentheses, and if that condition evaluates to true, the code enclosed in curly braces executes.
If statements are your go-to tool for handling various scenarios, including error management, addressing specific cases, and more.
Writing an If Statement:
if (Variable === "help") { console.log("Send help"); // The console.log() function outputs information to the console }
In this example, if the condition inside the parentheses (in this case, checking if the Variable is equal to "help") is true, the code within the curly braces gets executed.
Else and Else If Statements
Else: When the "if" condition is not met, the "else" part kicks in. It serves as a safety net, ensuring your program doesn't break and allowing you to specify what should happen in such cases.
Else If: Now, what if you need to check for a particular condition within a series of possibilities? That's where "else if" steps in. It allows you to examine and handle specific cases that require unique treatment.
Styling Elements with JavaScript
This is the beginner-friendly approach to changing the style of elements in JavaScript. It involves selecting an element using its ID or class, then making use of the .style.property method to set the desired styling property.
Example:
Let's say you have an HTML button with the ID "myButton," and you want to change its background color to red using JavaScript. Here's how you can do it:
HTML: <button id="myButton">Click me</button>
JavaScript:
// Select the button element by its ID const buttonElement = document.getElementById("myButton"); // Change the background color property buttonElement.style.backgroundColor = "red";
In this example, we first select the button element by its ID using document.getElementById("myButton"). Then, we use .style.backgroundColor to set the background color property of the button to "red." This straightforward approach allows you to dynamically change the style of HTML elements using JavaScript.
#studyblr#code#codeblr#css#html#javascript#java development company#python#study#progblr#programming#studying#comp sci#web design#web developers#web development#website design#ui ux design#reactjs#webdev#website#tech
400 notes
·
View notes
Text
youtube
#online courses#coding#graphic designing#web design#ict skills#india#hindi#gujarati#english#www.ictskills.in#online training#live training#full stack course#digital marketing#ui ux design#backend#online#live courses#courses#education#computer science#engineering#java#python#php#dot net development company#spring mvc#javascript#Youtube
2 notes
·
View notes
Text
25 Udemy Paid Courses for Free with Certification (Only for Limited Time)
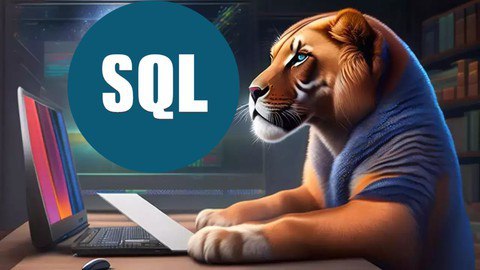
2023 Complete SQL Bootcamp from Zero to Hero in SQL
Become an expert in SQL by learning through concept & Hands-on coding :)
What you'll learn
Use SQL to query a database Be comfortable putting SQL on their resume Replicate real-world situations and query reports Use SQL to perform data analysis Learn to perform GROUP BY statements Model real-world data and generate reports using SQL Learn Oracle SQL by Professionally Designed Content Step by Step! Solve any SQL-related Problems by Yourself Creating Analytical Solutions! Write, Read and Analyze Any SQL Queries Easily and Learn How to Play with Data! Become a Job-Ready SQL Developer by Learning All the Skills You will Need! Write complex SQL statements to query the database and gain critical insight on data Transition from the Very Basics to a Point Where You can Effortlessly Work with Large SQL Queries Learn Advanced Querying Techniques Understand the difference between the INNER JOIN, LEFT/RIGHT OUTER JOIN, and FULL OUTER JOIN Complete SQL statements that use aggregate functions Using joins, return columns from multiple tables in the same query
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Python Programming Complete Beginners Course Bootcamp 2023
2023 Complete Python Bootcamp || Python Beginners to advanced || Python Master Class || Mega Course
What you'll learn
Basics in Python programming Control structures, Containers, Functions & Modules OOPS in Python How python is used in the Space Sciences Working with lists in python Working with strings in python Application of Python in Mars Rovers sent by NASA
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Learn PHP and MySQL for Web Application and Web Development
Unlock the Power of PHP and MySQL: Level Up Your Web Development Skills Today
What you'll learn
Use of PHP Function Use of PHP Variables Use of MySql Use of Database
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
T-Shirt Design for Beginner to Advanced with Adobe Photoshop
Unleash Your Creativity: Master T-Shirt Design from Beginner to Advanced with Adobe Photoshop
What you'll learn
Function of Adobe Photoshop Tools of Adobe Photoshop T-Shirt Design Fundamentals T-Shirt Design Projects
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Complete Data Science BootCamp
Learn about Data Science, Machine Learning and Deep Learning and build 5 different projects.
What you'll learn
Learn about Libraries like Pandas and Numpy which are heavily used in Data Science. Build Impactful visualizations and charts using Matplotlib and Seaborn. Learn about Machine Learning LifeCycle and different ML algorithms and their implementation in sklearn. Learn about Deep Learning and Neural Networks with TensorFlow and Keras Build 5 complete projects based on the concepts covered in the course.
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Essentials User Experience Design Adobe XD UI UX Design
Learn UI Design, User Interface, User Experience design, UX design & Web Design
What you'll learn
How to become a UX designer Become a UI designer Full website design All the techniques used by UX professionals
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Build a Custom E-Commerce Site in React + JavaScript Basics
Build a Fully Customized E-Commerce Site with Product Categories, Shopping Cart, and Checkout Page in React.
What you'll learn
Introduction to the Document Object Model (DOM) The Foundations of JavaScript JavaScript Arithmetic Operations Working with Arrays, Functions, and Loops in JavaScript JavaScript Variables, Events, and Objects JavaScript Hands-On - Build a Photo Gallery and Background Color Changer Foundations of React How to Scaffold an Existing React Project Introduction to JSON Server Styling an E-Commerce Store in React and Building out the Shop Categories Introduction to Fetch API and React Router The concept of "Context" in React Building a Search Feature in React Validating Forms in React
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Complete Bootstrap & React Bootcamp with Hands-On Projects
Learn to Build Responsive, Interactive Web Apps using Bootstrap and React.
What you'll learn
Learn the Bootstrap Grid System Learn to work with Bootstrap Three Column Layouts Learn to Build Bootstrap Navigation Components Learn to Style Images using Bootstrap Build Advanced, Responsive Menus using Bootstrap Build Stunning Layouts using Bootstrap Themes Learn the Foundations of React Work with JSX, and Functional Components in React Build a Calculator in React Learn the React State Hook Debug React Projects Learn to Style React Components Build a Single and Multi-Player Connect-4 Clone with AI Learn React Lifecycle Events Learn React Conditional Rendering Build a Fully Custom E-Commerce Site in React Learn the Foundations of JSON Server Work with React Router
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Build an Amazon Affiliate E-Commerce Store from Scratch
Earn Passive Income by Building an Amazon Affiliate E-Commerce Store using WordPress, WooCommerce, WooZone, & Elementor
What you'll learn
Registering a Domain Name & Setting up Hosting Installing WordPress CMS on Your Hosting Account Navigating the WordPress Interface The Advantages of WordPress Securing a WordPress Installation with an SSL Certificate Installing Custom Themes for WordPress Installing WooCommerce, Elementor, & WooZone Plugins Creating an Amazon Affiliate Account Importing Products from Amazon to an E-Commerce Store using WooZone Plugin Building a Customized Shop with Menu's, Headers, Branding, & Sidebars Building WordPress Pages, such as Blogs, About Pages, and Contact Us Forms Customizing Product Pages on a WordPress Power E-Commerce Site Generating Traffic and Sales for Your Newly Published Amazon Affiliate Store
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
The Complete Beginner Course to Optimizing ChatGPT for Work
Learn how to make the most of ChatGPT's capabilities in efficiently aiding you with your tasks.
What you'll learn
Learn how to harness ChatGPT's functionalities to efficiently assist you in various tasks, maximizing productivity and effectiveness. Delve into the captivating fusion of product development and SEO, discovering effective strategies to identify challenges, create innovative tools, and expertly Understand how ChatGPT is a technological leap, akin to the impact of iconic tools like Photoshop and Excel, and how it can revolutionize work methodologies thr Showcase your learning by creating a transformative project, optimizing your approach to work by identifying tasks that can be streamlined with artificial intel
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
AWS, JavaScript, React | Deploy Web Apps on the Cloud
Cloud Computing | Linux Foundations | LAMP Stack | DBMS | Apache | NGINX | AWS IAM | Amazon EC2 | JavaScript | React
What you'll learn
Foundations of Cloud Computing on AWS and Linode Cloud Computing Service Models (IaaS, PaaS, SaaS) Deploying and Configuring a Virtual Instance on Linode and AWS Secure Remote Administration for Virtual Instances using SSH Working with SSH Key Pair Authentication The Foundations of Linux (Maintenance, Directory Commands, User Accounts, Filesystem) The Foundations of Web Servers (NGINX vs Apache) Foundations of Databases (SQL vs NoSQL), Database Transaction Standards (ACID vs CAP) Key Terminology for Full Stack Development and Cloud Administration Installing and Configuring LAMP Stack on Ubuntu (Linux, Apache, MariaDB, PHP) Server Security Foundations (Network vs Hosted Firewalls). Horizontal and Vertical Scaling of a virtual instance on Linode using NodeBalancers Creating Manual and Automated Server Images and Backups on Linode Understanding the Cloud Computing Phenomenon as Applicable to AWS The Characteristics of Cloud Computing as Applicable to AWS Cloud Deployment Models (Private, Community, Hybrid, VPC) Foundations of AWS (Registration, Global vs Regional Services, Billing Alerts, MFA) AWS Identity and Access Management (Mechanics, Users, Groups, Policies, Roles) Amazon Elastic Compute Cloud (EC2) - (AMIs, EC2 Users, Deployment, Elastic IP, Security Groups, Remote Admin) Foundations of the Document Object Model (DOM) Manipulating the DOM Foundations of JavaScript Coding (Variables, Objects, Functions, Loops, Arrays, Events) Foundations of ReactJS (Code Pen, JSX, Components, Props, Events, State Hook, Debugging) Intermediate React (Passing Props, Destrcuting, Styling, Key Property, AI, Conditional Rendering, Deployment) Building a Fully Customized E-Commerce Site in React Intermediate React Concepts (JSON Server, Fetch API, React Router, Styled Components, Refactoring, UseContext Hook, UseReducer, Form Validation)
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Run Multiple Sites on a Cloud Server: AWS & Digital Ocean
Server Deployment | Apache Configuration | MySQL | PHP | Virtual Hosts | NS Records | DNS | AWS Foundations | EC2
What you'll learn
A solid understanding of the fundamentals of remote server deployment and configuration, including network configuration and security. The ability to install and configure the LAMP stack, including the Apache web server, MySQL database server, and PHP scripting language. Expertise in hosting multiple domains on one virtual server, including setting up virtual hosts and managing domain names. Proficiency in virtual host file configuration, including creating and configuring virtual host files and understanding various directives and parameters. Mastery in DNS zone file configuration, including creating and managing DNS zone files and understanding various record types and their uses. A thorough understanding of AWS foundations, including the AWS global infrastructure, key AWS services, and features. A deep understanding of Amazon Elastic Compute Cloud (EC2) foundations, including creating and managing instances, configuring security groups, and networking. The ability to troubleshoot common issues related to remote server deployment, LAMP stack installation and configuration, virtual host file configuration, and D An understanding of best practices for remote server deployment and configuration, including security considerations and optimization for performance. Practical experience in working with remote servers and cloud-based solutions through hands-on labs and exercises. The ability to apply the knowledge gained from the course to real-world scenarios and challenges faced in the field of web hosting and cloud computing. A competitive edge in the job market, with the ability to pursue career opportunities in web hosting and cloud computing.
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Cloud-Powered Web App Development with AWS and PHP
AWS Foundations | IAM | Amazon EC2 | Load Balancing | Auto-Scaling Groups | Route 53 | PHP | MySQL | App Deployment
What you'll learn
Understanding of cloud computing and Amazon Web Services (AWS) Proficiency in creating and configuring AWS accounts and environments Knowledge of AWS pricing and billing models Mastery of Identity and Access Management (IAM) policies and permissions Ability to launch and configure Elastic Compute Cloud (EC2) instances Familiarity with security groups, key pairs, and Elastic IP addresses Competency in using AWS storage services, such as Elastic Block Store (EBS) and Simple Storage Service (S3) Expertise in creating and using Elastic Load Balancers (ELB) and Auto Scaling Groups (ASG) for load balancing and scaling web applications Knowledge of DNS management using Route 53 Proficiency in PHP programming language fundamentals Ability to interact with databases using PHP and execute SQL queries Understanding of PHP security best practices, including SQL injection prevention and user authentication Ability to design and implement a database schema for a web application Mastery of PHP scripting to interact with a database and implement user authentication using sessions and cookies Competency in creating a simple blog interface using HTML and CSS and protecting the blog content using PHP authentication. Students will gain practical experience in creating and deploying a member-only blog with user authentication using PHP and MySQL on AWS.
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
CSS, Bootstrap, JavaScript And PHP Stack Complete Course
CSS, Bootstrap And JavaScript And PHP Complete Frontend and Backend Course
What you'll learn
Introduction to Frontend and Backend technologies Introduction to CSS, Bootstrap And JavaScript concepts, PHP Programming Language Practically Getting Started With CSS Styles, CSS 2D Transform, CSS 3D Transform Bootstrap Crash course with bootstrap concepts Bootstrap Grid system,Forms, Badges And Alerts Getting Started With Javascript Variables,Values and Data Types, Operators and Operands Write JavaScript scripts and Gain knowledge in regard to general javaScript programming concepts PHP Section Introduction to PHP, Various Operator types , PHP Arrays, PHP Conditional statements Getting Started with PHP Function Statements And PHP Decision Making PHP 7 concepts PHP CSPRNG And PHP Scalar Declaration
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Learn HTML - For Beginners
Lean how to create web pages using HTML
What you'll learn
How to Code in HTML Structure of an HTML Page Text Formatting in HTML Embedding Videos Creating Links Anchor Tags Tables & Nested Tables Building Forms Embedding Iframes Inserting Images
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Learn Bootstrap - For Beginners
Learn to create mobile-responsive web pages using Bootstrap
What you'll learn
Bootstrap Page Structure Bootstrap Grid System Bootstrap Layouts Bootstrap Typography Styling Images Bootstrap Tables, Buttons, Badges, & Progress Bars Bootstrap Pagination Bootstrap Panels Bootstrap Menus & Navigation Bars Bootstrap Carousel & Modals Bootstrap Scrollspy Bootstrap Themes
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
JavaScript, Bootstrap, & PHP - Certification for Beginners
A Comprehensive Guide for Beginners interested in learning JavaScript, Bootstrap, & PHP
What you'll learn
Master Client-Side and Server-Side Interactivity using JavaScript, Bootstrap, & PHP Learn to create mobile responsive webpages using Bootstrap Learn to create client and server-side validated input forms Learn to interact with a MySQL Database using PHP
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Linode: Build and Deploy Responsive Websites on the Cloud
Cloud Computing | IaaS | Linux Foundations | Apache + DBMS | LAMP Stack | Server Security | Backups | HTML | CSS
What you'll learn
Understand the fundamental concepts and benefits of Cloud Computing and its service models. Learn how to create, configure, and manage virtual servers in the cloud using Linode. Understand the basic concepts of Linux operating system, including file system structure, command-line interface, and basic Linux commands. Learn how to manage users and permissions, configure network settings, and use package managers in Linux. Learn about the basic concepts of web servers, including Apache and Nginx, and databases such as MySQL and MariaDB. Learn how to install and configure web servers and databases on Linux servers. Learn how to install and configure LAMP stack to set up a web server and database for hosting dynamic websites and web applications. Understand server security concepts such as firewalls, access control, and SSL certificates. Learn how to secure servers using firewalls, manage user access, and configure SSL certificates for secure communication. Learn how to scale servers to handle increasing traffic and load. Learn about load balancing, clustering, and auto-scaling techniques. Learn how to create and manage server images. Understand the basic structure and syntax of HTML, including tags, attributes, and elements. Understand how to apply CSS styles to HTML elements, create layouts, and use CSS frameworks.
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
PHP & MySQL - Certification Course for Beginners
Learn to Build Database Driven Web Applications using PHP & MySQL
What you'll learn
PHP Variables, Syntax, Variable Scope, Keywords Echo vs. Print and Data Output PHP Strings, Constants, Operators PHP Conditional Statements PHP Elseif, Switch, Statements PHP Loops - While, For PHP Functions PHP Arrays, Multidimensional Arrays, Sorting Arrays Working with Forms - Post vs. Get PHP Server Side - Form Validation Creating MySQL Databases Database Administration with PhpMyAdmin Administering Database Users, and Defining User Roles SQL Statements - Select, Where, And, Or, Insert, Get Last ID MySQL Prepared Statements and Multiple Record Insertion PHP Isset MySQL - Updating Records
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Linode: Deploy Scalable React Web Apps on the Cloud
Cloud Computing | IaaS | Server Configuration | Linux Foundations | Database Servers | LAMP Stack | Server Security
What you'll learn
Introduction to Cloud Computing Cloud Computing Service Models (IaaS, PaaS, SaaS) Cloud Server Deployment and Configuration (TFA, SSH) Linux Foundations (File System, Commands, User Accounts) Web Server Foundations (NGINX vs Apache, SQL vs NoSQL, Key Terms) LAMP Stack Installation and Configuration (Linux, Apache, MariaDB, PHP) Server Security (Software & Hardware Firewall Configuration) Server Scaling (Vertical vs Horizontal Scaling, IP Swaps, Load Balancers) React Foundations (Setup) Building a Calculator in React (Code Pen, JSX, Components, Props, Events, State Hook) Building a Connect-4 Clone in React (Passing Arguments, Styling, Callbacks, Key Property) Building an E-Commerce Site in React (JSON Server, Fetch API, Refactoring)
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Internet and Web Development Fundamentals
Learn how the Internet Works and Setup a Testing & Production Web Server
What you'll learn
How the Internet Works Internet Protocols (HTTP, HTTPS, SMTP) The Web Development Process Planning a Web Application Types of Web Hosting (Shared, Dedicated, VPS, Cloud) Domain Name Registration and Administration Nameserver Configuration Deploying a Testing Server using WAMP & MAMP Deploying a Production Server on Linode, Digital Ocean, or AWS Executing Server Commands through a Command Console Server Configuration on Ubuntu Remote Desktop Connection and VNC SSH Server Authentication FTP Client Installation FTP Uploading
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Linode: Web Server and Database Foundations
Cloud Computing | Instance Deployment and Config | Apache | NGINX | Database Management Systems (DBMS)
What you'll learn
Introduction to Cloud Computing (Cloud Service Models) Navigating the Linode Cloud Interface Remote Administration using PuTTY, Terminal, SSH Foundations of Web Servers (Apache vs. NGINX) SQL vs NoSQL Databases Database Transaction Standards (ACID vs. CAP Theorem) Key Terms relevant to Cloud Computing, Web Servers, and Database Systems
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Java Training Complete Course 2022
Learn Java Programming language with Java Complete Training Course 2022 for Beginners
What you'll learn
You will learn how to write a complete Java program that takes user input, processes and outputs the results You will learn OOPS concepts in Java You will learn java concepts such as console output, Java Variables and Data Types, Java Operators And more You will be able to use Java for Selenium in testing and development
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Learn To Create AI Assistant (JARVIS) With Python
How To Create AI Assistant (JARVIS) With Python Like the One from Marvel's Iron Man Movie
What you'll learn
how to create an personalized artificial intelligence assistant how to create JARVIS AI how to create ai assistant
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
Keyword Research, Free Backlinks, Improve SEO -Long Tail Pro
LongTailPro is the keyword research service we at Coursenvy use for ALL our clients! In this course, find SEO keywords,
What you'll learn
Learn everything Long Tail Pro has to offer from A to Z! Optimize keywords in your page/post titles, meta descriptions, social media bios, article content, and more! Create content that caters to the NEW Search Engine Algorithms and find endless keywords to rank for in ALL the search engines! Learn how to use ALL of the top-rated Keyword Research software online! Master analyzing your COMPETITIONS Keywords! Get High-Quality Backlinks that will ACTUALLY Help your Page Rank!
Enroll Now 👇👇👇👇👇👇👇 https://www.book-somahar.com/2023/10/25-udemy-paid-courses-for-free-with.html
#udemy#free course#paid course for free#design#development#ux ui#xd#figma#web development#python#javascript#php#java#cloud
2 notes
·
View notes
Text
How to install bootstrap in React.js? Installing Bootstrap in React.js: A Step-by-Step Guide.
Bootstrap is a popular CSS framework that provides a plethora of pre-designed components and styles to help developers create responsive and visually appealing web applications. Integrating Bootstrap with React.js can enhance your project's user interface and save you valuable development time. In this tutorial, we'll walk you through the process of installing Bootstrap in a React.js application.
#reactjs#bootstrap#javascript#developer#web development#web developers#web design#website#html#code#codeblr#coding#pythor p chumsworth#responsivedesign#tumblr ui#ui ux design#java development company#developers#software#python#sql
2 notes
·
View notes
Text
Is Hyderabad a good place to learn AWS and DevOps from the best institute?
Hyderabad is a good location to train in AWS and DevOps from leading training institutes providing industry-oriented training, live projects, and placement assistance.
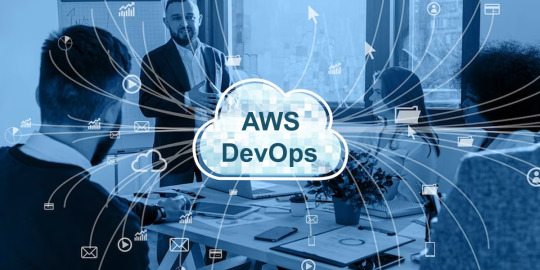
The city provides extensive courses with actual project exposure, qualified faculty, and effective placement assistance.
Be it a fresher or an IT professional, studying AWS and DevOps in Hyderabad provides real-world skills and certification orientation that makes it the ultimate place to launch a successful career in cloud and DevOps.
1 note
·
View note
Text
UI/UX Design Mastery Course in Madurai – Elevate Your Design Skills
The UI/UX Design Mastery Course in Madurai is an ideal program for aspiring designers and tech enthusiasts eager to break into the world of user interface and user experience design. Tailored for beginners as well as professionals looking to enhance their skills, this course offers a perfect blend of theoretical knowledge and hands-on experience.
Participants will learn the fundamentals of design thinking, user research, wireframing, prototyping, and usability testing. The curriculum also covers industry-leading tools like Figma, Adobe XD, and Sketch, ensuring learners gain practical expertise. The course emphasizes real-world projects and case studies to help students build an impressive design portfolio.
Guided by experienced mentors and industry experts, students receive personalized feedback and career guidance throughout the program. Whether you aim to work with top tech companies, start your freelance design journey, or build your own product, this course equips you with the skills to deliver intuitive and engaging digital experiences.
Located in Madurai, this program offers in-person and hybrid learning options, making it accessible and flexible for learners from different backgrounds. With a focus on creativity, innovation, and user-centric design, the UI/UX Design Mastery Course is your gateway to a rewarding career in the dynamic field of digital design.
Enroll today to unlock the power of design and transform your ideas into user-friendly interfaces that make a lasting impact.
#Software Courses: Software Courses#Animation Courses#IT Training#Data science and Data Analytics#Full stack development#Software Testing#C#C++#Java#Dotnet#Python#Networking and Cloud#Web development. Animation Courses: Graphic Designing#UI UX Design#2D & 3D Animation#Game Designing#Visual Effects#Digital Marketing.
0 notes
Text
KINFOMEDIA delivers creative design, web & app development, digital marketing, and tech solutions to help businesses grow online.
#web design#website development#graphic design#ui/ux design#digital marketing#search engine optimization#social media marketing#emailmarketing#app development#.net#php#javascript#java
1 note
·
View note
Text
🎓 From Learner to Leader: Your Journey Starts Here!
The tech industry is evolving fast, and companies are constantly hunting for skilled Full Stack Developers who can handle both front-end and back-end with ease. Want to be one of them?
💻 Join our Placement Assistance Program in Full Stack JAVA & .NET and get ready to turn your passion into a profession.
📅 Start Date: 9th June 2025 🕚 Time: 11:00 AM IST 🏫 Mode: Online / Classroom 🔗 Register Now: https://tr.ee/7MlU6p
This isn’t just a course — it’s your launchpad. Learn:
✅ Core & Advanced Java ✅ .NET Framework & Technologies ✅ Frontend tools (HTML, CSS, JavaScript) ✅ Real-time projects with ReactJS and Node.js ✅ Interview prep, resume building & mock interviews

👨🏫 Taught by industry experts, this program is designed to guide freshers and career-switchers step-by-step, helping you gain the skills and confidence needed to ace interviews and thrive on the job.
💼 Placement Support? Absolutely! We walk with you till your offer letter is in hand. Your success is our goal.
🌟 Don't wait for opportunities. Create them.
🔍 Want to explore more career-focused courses? Visit: https://linktr.ee/ITcoursesFreeDemos
🧭 Let Naresh i Technologies be your guide to a brighter tech future. ✍️ Register Today and start building your dream career!
#java course#javaprogramming#coding#fullstackjava#learnfromhome#full stack training#full stack developer#online training#ui ux design#offline#placementassistance
0 notes
Text
Software Training Institute in Hyderabad | Digitalwin academics
Best IT Trainings" is a large online education portal serving global digital enthusiasts. With a wide selection of workshops, courses, and tools, it's a one-stop shop for anyone who wants to succeed in the digital world. One of its most regarded programs is Digital Win Academy, a cutting-edge platform well-known for its interactive learning environments and expert-led classes. Flexible scheduling, practical assignments, and ongoing assistance help learners stay current with emerging trends and technologies. "Best IT Trainings" is a large online learning platform dedicated to people who love technology worldwide. For anyone seeking success in the world of technology, this one-stop shop offers an extensive range of workshops, courses, and resources. Digital Win Academy, a cutting-edge platform renowned for its interactive learning settings and expert-led classes, is one of its most highly rated programs. Learners may keep up with recent developments in technology with the help of flexible scheduling, practical assignments, and continuing support.
0 notes
Text
#internship#python#java#ui ux design#artificial intelligence#data science#data science course#digital marketing
1 note
·
View note
Text
Beginner to Pro: Top UI/UX Design Tricks You Need to Know

Introduction
UI/UX design plays a crucial role in crafting user-friendly digital experiences. Whether you're starting your journey in UI/UX or aiming to enhance your skills, mastering the right tricks can set you apart. In this blog, we'll explore essential UI/UX design tips that can help you transition from a beginner to a pro.
1. Understand Your Users
Before you start designing, it's essential to know your users. Research their preferences, behavior, and pain points. Conduct user testing and surveys to gather insights. A strong understanding of user needs leads to a more intuitive design.
2. Keep It Simple and Intuitive
A cluttered interface confuses users. Stick to minimal design principles by using whitespace effectively and ensuring that navigation is easy. A well-structured UI makes interactions smooth, improving user satisfaction.
3. Master Typography and Color Theory
Typography and color are powerful tools in UI/UX design. Use fonts that are readable and align with the brand personality. Colors should be strategically chosen to evoke emotions and improve usability. Contrast is key for accessibility.
4. Mobile-First Approach
With a significant number of users accessing websites and applications through mobile devices, designing with a mobile-first approach is essential. Ensure that the interface is responsive and adapts seamlessly across different screen sizes.
5. Focus on Microinteractions
Microinteractions, such as button animations, hover effects, and subtle transitions, enhance user experience by making interactions feel engaging and natural. They provide feedback and guide users through the interface effortlessly.
6. Prioritize Loading Speed
Slow-loading websites and applications drive users away. Optimize images, use compressed files, and implement caching techniques to improve performance. A fast-loading UI keeps users engaged and enhances usability.
7. Utilize UI/UX Design Tools
Leverage powerful design tools like Figma, Adobe XD, and Sketch to create wireframes and prototypes. These tools allow designers to visualize ideas and collaborate efficiently.
8. Stay Updated with UI/UX Trends
UI/UX is an ever-evolving field, and keeping up with trends is vital. Follow industry experts, take up courses, and experiment with new design patterns to stay ahead of the competition.
9. Get Certified and Build a Portfolio
Enrolling in a UI UX design certification in Yamuna Vihar or UX UI design training in Yamuna Vihar helps solidify your expertise. A strong portfolio showcasing your work can significantly boost your career prospects.
10. Learn Web Development Basics
A solid understanding of Web Designing Training in Yamuna Vihar or Web Development Training Institute in Yamuna Vihar can complement your UI/UX skills. Knowing HTML, CSS, and JavaScript helps designers create functional prototypes and work efficiently with developers.
Conclusion
UI/UX design is an exciting and dynamic field that requires continuous learning and creativity. By implementing these strategies, you can refine your skills and deliver exceptional user experiences. If you're looking to enhance your expertise, consider enrolling in a UI and UX design course in Yamuna Vihar or Full Stack Web Development Training in Uttam Nagar to gain hands-on knowledge and industry exposure.
Start your journey today and transform into a professional UI/UX designer. Visit Us.
Suggested Links
Oracle Database Administration
MY SQL Training
PHP Development
#ui ux design#html training#css tips#java programming language#full stack development#web design#web development
0 notes
Text
Software Development: Life Cycle Phases
Software Development Service or what is known as Software Development Life Cycle is process of this Phases: Planning, Analysis, Design, Implementation, Testing Deployment and Maintenance by a software developer. There are many types of Software Development like Web Development, Mobile App Development and Computer Software Development. In addition to this popular Programming Languages: Python, JavaScript, Java, C++ and Swift. without forgetting the essential Development Tools from Visual Studio Code, GitHub or Chrome DevTools. That are the best laptop for software development:
MacBook Pro 14-inch (M2 Pro/Max)
Dell XPS 15 (9520)
Lenovo ThinkPad X1 Carbon Gen 10
Acer Swift 3
ASUS ROG Zephyrus G14
Microsoft Surface Laptop 5
Custom Software Development is a dynamic field that continues to evolve with new technologies and Artificial Intelligence.
Find more information HERE !!
#technology#software development#web developers#ui ux design#javascript#java#html css#coding#artificial intelligence
0 notes
Text
JavaScript’s native Array and Object methods
Think you know JavaScript? Find out how native features can handle Objects and Arrays that simplify coding. No libraries necessary!
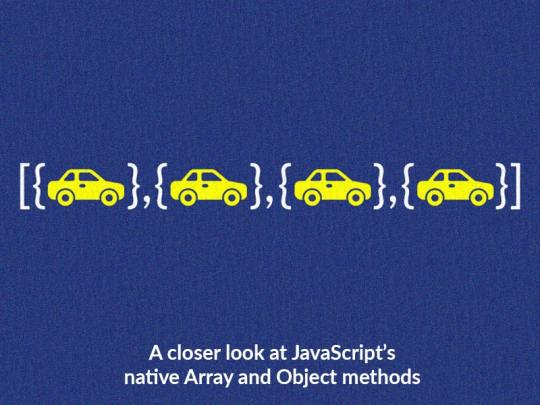
0 notes
Text
Building a Strong Foundation: Why a Java Full Stack Developer Course is a Smart Investment
In the ever-evolving world of technology, staying ahead of the curve is essential for career advancement. One of the most effective ways to secure a robust foundation in the tech industry is by enrolling in a Java Full Stack Developer Course in Pune. This course offers a comprehensive skill set that is highly valued in today’s job market. Here’s why investing in this course can be a game-changer for your career.
1. Comprehensive Skill Set
A Java Full Stack Developer Course in Pune provides a well-rounded education in both front-end and back-end technologies. By mastering Java, along with essential tools and frameworks, you gain the skills needed to develop and manage complex web applications. This course typically covers technologies such as HTML, CSS, JavaScript, and various Java frameworks, offering you a complete toolkit for full-stack development.
2. High Demand for Full Stack Developers
Full stack developers are in high demand due to their versatility and ability to handle both client-side and server-side development. Completing a Java Full Stack Developer Course positions you as a well-rounded professional capable of taking on diverse roles within tech teams. This demand translates into better job opportunities and competitive salaries.
3. Integration with Other Courses
A Java Full Stack Developer Course complements other technical courses such as a Software Testing Course in Pune and a UI/UX Design Course in Pune. Understanding the full stack of development enhances your ability to collaborate effectively with software testers and UI/UX designers. For instance, knowing how software is built allows you to better understand how it should be tested and how design elements should be integrated.
4. Versatility and Career Growth
Investing in a Java Full Stack Developer Course offers unparalleled career versatility. The skills acquired can be applied to various roles within the technology sector, including web development, software engineering, and application development. Additionally, it opens doors to further specialization and advanced roles in areas such as software architecture and project management.
5. Practical Experience and Industry-Relevant Training
A key advantage of enrolling in a Java Full Stack Developer Course in Pune is the practical experience it provides. Most courses offer hands-on projects and real-world scenarios that equip you with the skills needed to tackle real industry challenges. This practical approach ensures that you are job-ready upon completion of the course.
6. Networking and Industry Connections
Courses often provide opportunities to network with industry professionals and peers. Building these connections can lead to job opportunities, mentorship, and collaborations. In a city like Pune, known for its vibrant tech scene, these networking opportunities can be particularly valuable.
Complementing Your Skills
While a Java Full Stack Developer Course is incredibly beneficial, combining it with other courses can further enhance your career prospects. For example, a Software Testing Course in Pune will add a crucial dimension to your skill set, enabling you to ensure the quality and functionality of the applications you develop. Similarly, a UI/UX Design Course in Pune will help you understand user-centered design principles, making your applications more intuitive and engaging.
Conclusion
Investing in a Java Full Stack Developer Course in Pune is a strategic move for anyone looking to build a solid foundation in the tech industry. The comprehensive skill set, high demand for full stack developers, and the ability to integrate with other technical courses make it a smart investment for career growth. By enhancing your expertise with courses in software testing and UI/UX design, you position yourself as a well-rounded professional ready to tackle the challenges of modern technology.
Whether you are just starting your tech career or looking to upgrade your skills, a Java Full Stack Developer Course provides the tools and knowledge needed to succeed in today’s competitive job market.
#Java Full Stack Developer Course in Pune#software testing course in pune#ui ux design course in pune
0 notes
Text

At Tandem Informatics, we are dedicated to empowering the next generation of tech professionals through cutting-edge training and hands-on experience. Our courses cover essential programming languages, frameworks, and industry best practices to ensure you are job-ready. With our top-tier corporate-level training, we have established a benchmark for placing students in leading companies. We specialize in equipping individuals with the skills and certifications needed to thrive in the dynamic world of technology. Imagine becoming a Cisco Certified Network Associate (CCNA), a Microsoft Certified Technology Specialist (MCTS), or an Oracle Certified Professional Java Programmer (OCPJP). The possibilities are endless! Our expert trainers also offer comprehensive courses in areas such as 3D Animation, Visual Effects, UI/UX Designing, and much more.
#Software Courses: Software Courses#Animation Courses#IT Training#Data science and Data Analytics#Full stack development#Software Testing#C#C++#Java#Dotnet#Python#Networking and Cloud#Web development. Animation Courses: Graphic Designing#UI UX Design#2D & 3D Animation#Game Designing#Visual Effects#Digital Marketing.
0 notes