#raw code
Explore tagged Tumblr posts
Text
#Playstation7 Security backend FireWall Dynamic Encryption, NFT integration CG’s and Online Store, Game download, installation and run processes.

Creating a comprehensive backend system for a console that integrates security, encryption, store functionality, NFT integration, and blockchain encoding is an extensive task, but here’s a detailed outline and code implementation for these components:

1. Security and Firewall System with Dynamic Encryption
The security system will need robust firewalls and periodic encryption mechanisms that update dynamically every 5 minutes and every 30th of a second.
1.1 Encryption Structure (Python-based) with Time-Based Swapping
We’ll use the cryptography library in Python for encryption, and random for generating random encryption keys, which will change periodically.
Encryption Swapping Code:
import os
import time
import random
from cryptography.fernet import Fernet
class SecuritySystem:
def __init__(self):
self.current_key = self.generate_key()
self.cipher_suite = Fernet(self.current_key)
def generate_key(self):
return Fernet.generate_key()
def update_key(self):
self.current_key = self.generate_key()
self.cipher_suite = Fernet(self.current_key)
print(f"Encryption key updated: {self.current_key}")
def encrypt_data(self, data):
encrypted = self.cipher_suite.encrypt(data.encode())
return encrypted
def decrypt_data(self, encrypted_data):
return self.cipher_suite.decrypt(encrypted_data).decode()
# Swapping encryption every 5 minutes and 30th of a second
def encryption_swapper(security_system):
while True:
security_system.update_key()
time.sleep(random.choice([5 * 60, 1 / 30])) # 5 minutes or 30th of a second
if __name__ == "__main__":
security = SecuritySystem()
# Simulate swapping
encryption_swapper(security)
1.2 Firewall Setup (Using UFW for Linux-based OS)
The console could utilize a basic firewall rule set using UFW (Uncomplicated Firewall) on Linux:
# Set up UFW firewall for the console backend
sudo ufw default deny incoming
sudo ufw default allow outgoing
# Allow only specific ports (e.g., for the store and NFT transactions)
sudo ufw allow 8080 # Store interface
sudo ufw allow 443 # HTTPS for secure transactions
sudo ufw enable
This basic rule ensures that no incoming traffic is accepted except for essential services like the store or NFT transfers.
2. Store Functionality: Download, Installation, and Game Demos
The store will handle downloads, installations, and demo launches. The backend will manage game storage, DLC handling, and digital wallet integration for NFTs.

2.1 Download System and Installation Process (Python)
This code handles the process of downloading a game, installing it, and launching a demo.
Store Backend (Python + MySQL for Game Listings):
import mysql.connector
import os
import requests
class GameStore:
def __init__(self):
self.db = self.connect_db()
def connect_db(self):
return mysql.connector.connect(
host="localhost",
user="admin",
password="password",
database="game_store"
)
def fetch_games(self):
cursor = self.db.cursor()
cursor.execute("SELECT * FROM games")
return cursor.fetchall()
def download_game(self, game_url, game_id):
print(f"Downloading game {game_id} from {game_url}...")
response = requests.get(game_url)
with open(f"downloads/{game_id}.zip", "wb") as file:
file.write(response.content)
print(f"Game {game_id} downloaded.")
def install_game(self, game_id):
print(f"Installing game {game_id}...")
os.system(f"unzip downloads/{game_id}.zip -d installed_games/{game_id}")
print(f"Game {game_id} installed.")
def launch_demo(self, game_id):
print(f"Launching demo for game {game_id}...")
os.system(f"installed_games/{game_id}/demo.exe")
# Example usage
store = GameStore()
games = store.fetch_games()
# Simulate downloading, installing, and launching a demo

store.download_game("http://game-download-url.com/game.zip", 1)
store.install_game(1)
store.launch_demo(1)
2.2 Subsections for Games, DLC, and NFTs
This section of the store manages where games, DLCs, and NFTs are stored.
class GameContentManager:
def __init__(self):
self.games_folder = "installed_games/"
self.dlc_folder = "dlcs/"
self.nft_folder = "nfts/"
def store_game(self, game_id):
os.makedirs(f"{self.games_folder}/{game_id}", exist_ok=True)
def store_dlc(self, game_id, dlc_id):
os.makedirs(f"{self.dlc_folder}/{game_id}/{dlc_id}", exist_ok=True)
def store_nft(self, nft_data, nft_id):
with open(f"{self.nft_folder}/{nft_id}.nft", "wb") as nft_file:
nft_file.write(nft_data)
# Example usage
manager = GameContentManager()
manager.store_game(1)
manager.store_dlc(1, "dlc_1")
manager.store_nft(b"NFT content", "nft_1")
3. NFT Integration and Blockchain Encoding
We’ll use blockchain to handle NFT transactions, storing them securely in a blockchain ledger.
3.1 NFT Blockchain Encoding (Python)
This script simulates a blockchain where each block stores an NFT.
import hashlib
import time
class Block:
def __init__(self, index, timestamp, data, previous_hash=''):
self.index = index
self.timestamp = timestamp
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
block_string = f"{self.index}{self.timestamp}{self.data}{self.previous_hash}"
return hashlib.sha256(block_string.encode()).hexdigest()
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block(0, time.time(), "Genesis Block", "0")
def get_latest_block(self):
return self.chain[-1]
def add_block(self, new_data):
previous_block = self.get_latest_block()
new_block = Block(len(self.chain), time.time(), new_data, previous_block.hash)
self.chain.append(new_block)
def print_blockchain(self):
for block in self.chain:
print(f"Block {block.index} - Data: {block.data} - Hash: {block.hash}")
# Adding NFTs to the blockchain
nft_blockchain = Blockchain()
nft_blockchain.add_block("NFT1: Digital Sword")
nft_blockchain.add_block("NFT2: Magic Shield")
nft_blockchain.print_blockchain()
3.2 NFT Wallet Transfer Integration (Python)
This script will transfer NFTs into wallets or digital blockchain systems.
class NFTWallet:
def __init__(self):
self.wallet = {}
def add_nft(self, nft_id, nft_data):
self.wallet[nft_id] = nft_data
print(f"Added NFT {nft_id} to wallet.")
def transfer_nft(self, nft_id, recipient_wallet):
if nft_id in self.wallet:
recipient_wallet.add_nft(nft_id, self.wallet[nft_id])
del self.wallet[nft_id]
print(f"Transferred NFT {nft_id} to recipient.")
# Example usage
user_wallet = NFTWallet()
user_wallet.add_nft("nft_1", "Digital Art Piece 1")
recipient_wallet = NFTWallet()
user_wallet.transfer_nft("nft_1", recipient_wallet)
4. CG (Computer Graphics) Storage for Cutscenes and Artwork
4.1 Storing and Retrieving CG Assets
This system stores CG assets (cutscenes, artwork, etc.) for later viewing and reminiscing.
class CGStorage:
def __init__(self):
self.cg_folder = "cg_assets/"
os.makedirs(self.cg_folder, exist_ok=True)
def store_cg(self, cg_id, cg_data):
with open(f"{self.cg_folder}/{cg_id}.mp4", "wb") as cg_file:
cg_file.write(cg_data)
print(f"CG {cg_id} stored.")
def retrieve_cg(self, cg_id):
with open(f"{self.cg_folder}/{cg_id}.mp4", "rb") as cg_file:
return cg_file.read()
# Example usage
cg_storage = CGStorage()
cg_storage.store_cg("cutscene_1", b"CG video data")
cg_data = cg_storage.retrieve_cg("cutscene_1")
Summary of the System:

1. Security: Dynamic encryption swaps with regular firewall management.
2. Store: Handles game downloads, installations, and demo launches, including storage for games, DLC, and NFTs.
3. NFT Integration: A blockchain-based system for NFT encoding and wallet transfers.
4. CG Storage: Storing and retrieving game cutscenes and artwork for later viewing.
This framework is scalable and can be extended as the console ecosystem grows. Each component works independently but can be integrated into a larger gaming console backend system.





#playstation7#ps7#deardearestbrands#digitalconsole#framework#python#soundcloud#celestiallink#raw code#rawscript#blockchain#NFTs#Security#Frontend#backend#encryption processes
1 note
·
View note
Text
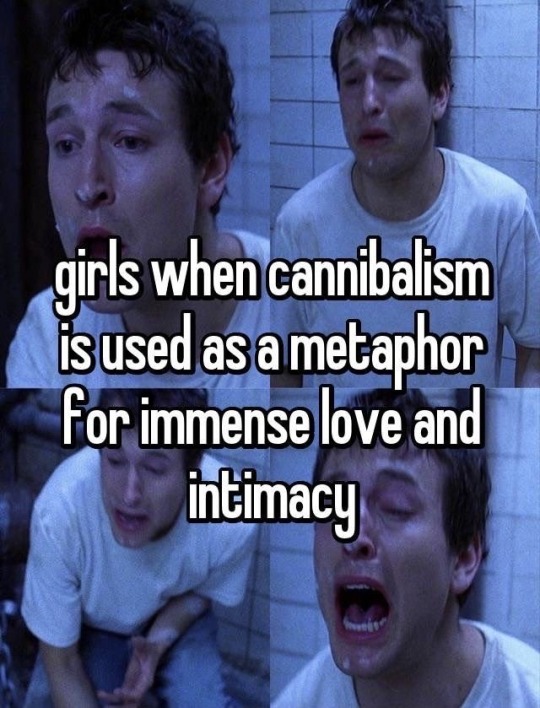
#mothercain#ethel cain#bones and all#raw 2016#yellowjackets#girlblog#girlhood#girlblogging#female hysteria#this is a girlblog#gaslight gatekeep girlboss#mother cain#ethel cain core#female manipulator#coquette girl#manic pixie dream girl#ethel cain coded#preachers daughter#religious trauma#this is what makes us girls#girlblogger#girl boss gaslight gatekeep#just girly posts#girl blogger
14K notes
·
View notes
Text
CHYNA & EDDIE GUERRERO RAW IS WAR (Aug 17, 2000)
(cred to FullWithDivas for the videos!)
Bonus:
#chyna#wwe chyna#eddie guerrero#wwf#wwe#wweedit#wrestlingedit#wrestling gifs#attitude era#wwe raw#wwe smackdown#raw is war#pro wrestling#latino heat#flashing lights#cw flashing#this is so cute like omgggg#eddie letting chyna put a rose in his hair while locking eyes with her omggggggg no one doing it like em#and this is so mortica/gomez coded! their whole dynamic is!#and then chyna kissing him on the back of his head like - me and who please!?!?! me and who when?!?!
413 notes
·
View notes
Text
I support Seth Rollins when he's right, and I support Seth Rollins when he's wrong but most of all I support Seth Rollins when he's being just a Lil bit ✨cunty✨
199 notes
·
View notes
Text
RESULTS ARE IN 👍
i posted this poll as a joke but i got a little miffed at the makoto character assassination in the notes/ask box so now im giving my reasoning of why i think this fight is a hard 50/50 and not just an easy makoto win:
(btw, this isnt out of anger. im genuinely having a lot of fun ranting about this silly topic because i love these two clowns)
Rage: the biggest pro-makoto argument people had was that he was filled with pent up rage, which... okay, but i actually don't think it's that simple. more than anything, makoto is frustrated with himself. not his original, and, hell, not even yomi. the only reason he started beefing with either of them is because both were putting kanai ward in danger trying to reveal the homunculus info. otherwise, his original is a non-issue and yomi is nothing more than a nuisance. in the game, when makoto's carefully concealed emotions begin to trickle out, he is far from angry. he's sad, helpless, and broken. he's close to crumbling under the pressure of his own tower of lies, and he's not the contained rage beast yall think he is 😭 makoto has the conviction to fight with his all, but i genuinely don't think he's going sicko mode when angry.
yuma, on the other hand, is ROYALLY PISSED at makoto. this is the chapter 5 bathhouse!! makoto just put him in zombie hell and spent the last couple hours taunting yuma with the corpses of his friends! yuma may be a doormat but he's literally shown himself capable of snapping in anger before! if anyone here is falling into a rage-filled frenzy, it is not makoto
Stamina + Strength: all im saying is that one of these characters spends most of his time doing legwork, running from cops, and doing QTEs with a small level of physical competence, and the other one takes baths while plotting in his fancy penthouse all day
Strategy: makoto 100% has the edge in this one. he still has number one's memories, and yuma is also a little stupid. i can see makoto fighting using trickery. sand-in-the-eyes level stuff, yknow? i dont think number one himself was actually trained in close combat though. he's a shut-in who is always on the run from criminal syndicates, so i'd imagine most of his skillset lies in how to stay out of fights rather than win them. still, im sure makoto can come up with a few ways to put himself at an advantage, as long as he can take/avoid enough hits.
Patheticness: this is a joke stat but i feel like people forget that makoto is JUST as pathetic as yuma is. he's just better at hiding it.
Power of Friendship: also a joke stat i think it has no actual bearing on this fight lmao. though yuma may be motivated by the "deaths" of his friends.
that being said, i still think makoto could win! i actually fully expected these poll results! but i also think it depends on whether or not yuma's raw determination beats out makoto's trickiness... and even then, they're both coming out of this looking utterly beaten and bruised. anyway if you got this far, thanks for reading my manifesto on this shit lmao
#rain code#raincode#mdarc#master detective archives: rain code#yuma kokohead#makoto kagutsuchi#rain code spoilers#mdarc spoilers#master detective archives: rain code spoilers#btw in a perfect world they have this raw and visceral fistfight but in the end they're roughly making out on the bathhouse floor#biggie's rain code ramblings
82 notes
·
View notes
Text
I love you horrible gut wrenching queer media I love you narrative doom and damnation I love you bleakness and bloodiness I love you tragedy as a tool of union I love horrible depressing unhappy eviscerating queerness I love that we are so full of horror and terror and bloodshed and guilt and tragedy and pain and yet we are all still so full of love and joy and bliss and happiness in ourselves and we are all still beautiful in each others eyes I love u adam and lawrence from saw 2004
#cal.txt#queer media#queer coding#sawposting#chainshipping#saw 2004#saw 1#lawrence gordon#adam stanheight#lawrence x adam#toxic yaoi#doomed yaoi#this is what tragic gay media means to me#heartstopper is literally palatable queerness I won’t back down from that I want . raw and diseased gay people#thisbis so bad but I’m so fivkinf emotional and wrecked right now#queer studies I guess#I’d not fiuvking know ignore my dimb ass
311 notes
·
View notes
Text
IV who always gets onto his partner when they skip meals, his heart aches at the thought of his love not taking proper care of themself. He's the kind to whip up a bowl of buttered noodles or a little quesadilla at a moment's notice when he hears their tummy grumble.
IV who comes home from a long time away, desperate to get his hands on his partner again, missing the feeling of their skin against his. He drops his bags at the door and is on them in seconds, his lips meeting theirs in the hungriest kiss, pulling them flush against his body, hands wandering to every inch of exposed skin he can reach. His tongue just barely slips through their lips when they pull back to whisper, a mischievous smile on their face.
"Have you eaten anything yet today?" they whispered, knowing he had a bad habit of not eating on travel days.
Furrowing his brow, he shakes his head. It had been a long day of driving and he'd been so excited to see them the thought hadn't even crossed his mind. When he shook his head, they slip from his grasp, causing him to whine at the loss of their familiar warmth in his arms.
"No play until you've eaten something," they tease with a wink, tapping their index finger against his nose gently. They disappear into the kitchen, leaving him alone in the entryway of their home. He groans loudly, knowing that they're making him pay for every well-meaning message about their eating habits.
He's grinding his teeth while he watches them whisk through the kitchen, his eyes raking over their figure as they're gathering the necessary ingredients for their signature meal. It wasn't anything complicated, but it tasted like them and it tasted like love, and despite the much less innocent thoughts racing through his mind, he couldn't help but feel a sense of peace he hadn't felt in weeks as the familiar smell wafted towards his nose as he sat at the kitchen table watching them work.
They've never seen him eat so fast, shoveling every last bite into his mouth before he practically throws his dishes into the sink, scooping them off their chair. A surprised squeal echoes through the kitchen as he planted them onto the table, standing between their legs with his hands on their face.
"Happy now?" to which they only nod. He wasted no time in crashing his lips back against theirs, his tongue slipping into their mouth to offer the faintest taste of the delicious meal they'd just made for him. A token of appreciation for the care they showed to him, a silent thank you for looking out for him.
But he was about to make and even more delicious meal out of them.
#high key this is also very iii x iv coded. like obv most of the time when iv's gone iii would be with him (tour) but like still#housewife iv is and always will be alive and well here on tumblr dot com slash politemagic#this is only edited for spelling errors she is raw and off the dome#not writing the whole thing because tbh. i think i'd die if i tried to write kitchen sex with iv. i think i would actually fucking pass awa#so use your imagination + feel free to add on if you so desire <3#i have a thing about the kitchen is it obvious?#sleep token fanfiction#sleep token x reader#sleep token iv x reader#em's stcu
59 notes
·
View notes
Text
#Playstation7 #framework #BasicArchitecture #RawCode #RawScript #Opensource #DigitalConsole
To build a new gaming console’s digital framework from the ground up, you would need to integrate several programming languages and technologies to manage different aspects of the system. Below is an outline of the code and language choices required for various parts of the framework, focusing on languages like C++, Python, JavaScript, CSS, MySQL, and Perl for different functionalities.
1. System Architecture Design (Low-level)
• Language: C/C++, Assembly
• Purpose: To program the low-level system components such as CPU, GPU, and memory management.
• Example Code (C++) – Low-Level Hardware Interaction:
#include <iostream>
int main() {
// Initialize hardware (simplified example)
std::cout << "Initializing CPU...\n";
// Set up memory management
std::cout << "Allocating memory for GPU...\n";
// Example: Allocating memory for gaming graphics
int* graphicsMemory = new int[1024]; // Allocate 1KB for demo purposes
std::cout << "Memory allocated for GPU graphics rendering.\n";
// Simulate starting the game engine
std::cout << "Starting game engine...\n";
delete[] graphicsMemory; // Clean up
return 0;
}
2. Operating System Development
• Languages: C, C++, Python (for utilities)
• Purpose: Developing the kernel and OS for hardware abstraction and user-space processes.
• Kernel Code Example (C) – Implementing a simple syscall:
#include <stdio.h>
#include <unistd.h>
int main() {
// Example of invoking a custom system call
syscall(0); // System call 0 - usually reserved for read in UNIX-like systems
printf("System call executed\n");
return 0;
}
3. Software Development Kit (SDK)
• Languages: C++, Python (for tooling), Vulkan or DirectX (for graphics APIs)
• Purpose: Provide libraries and tools for developers to create games.
• Example SDK Code (Vulkan API with C++):
#include <vulkan/vulkan.h>
VkInstance instance;
void initVulkan() {
VkApplicationInfo appInfo = {};
appInfo.sType = VK_STRUCTURE_TYPE_APPLICATION_INFO;
appInfo.pApplicationName = "GameApp";
appInfo.applicationVersion = VK_MAKE_VERSION(1, 0, 0);
appInfo.pEngineName = "GameEngine";
appInfo.engineVersion = VK_MAKE_VERSION(1, 0, 0);
appInfo.apiVersion = VK_API_VERSION_1_0;
VkInstanceCreateInfo createInfo = {};
createInfo.sType = VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO;
createInfo.pApplicationInfo = &appInfo;
vkCreateInstance(&createInfo, nullptr, &instance);
std::cout << "Vulkan SDK Initialized\n";
}
4. User Interface (UI) Development
• Languages: JavaScript, HTML, CSS (for UI), Python (backend)
• Purpose: Front-end interface design for the user experience and dashboard.
• Example UI Code (HTML/CSS/JavaScript):
<!DOCTYPE html>
<html>
<head>
<title>Console Dashboard</title>
<style>
body { font-family: Arial, sans-serif; background-color: #282c34; color: white; }
.menu { display: flex; justify-content: center; margin-top: 50px; }
.menu button { padding: 15px 30px; margin: 10px; background-color: #61dafb; border: none; cursor: pointer; }
</style>
</head>
<body>
<div class="menu">
<button onclick="startGame()">Start Game</button>
<button onclick="openStore()">Store</button>
</div>
<script>
function startGame() {
alert("Starting Game...");
}
function openStore() {
alert("Opening Store...");
}
</script>
</body>
</html>
5. Digital Store Integration
• Languages: Python (backend), MySQL (database), JavaScript (frontend)
• Purpose: A backend system for purchasing and managing digital game licenses.
• Example Backend Code (Python with MySQL):
import mysql.connector
def connect_db():
db = mysql.connector.connect(
host="localhost",
user="admin",
password="password",
database="game_store"
)
return db
def fetch_games():
db = connect_db()
cursor = db.cursor()
cursor.execute("SELECT * FROM games")
games = cursor.fetchall()
for game in games:
print(f"Game ID: {game[0]}, Name: {game[1]}, Price: {game[2]}")
db.close()
fetch_games()
6. Security Framework Implementation
• Languages: C++, Python, Perl (for system scripts)
• Purpose: Ensure data integrity, authentication, and encryption.
• Example Code (Python – Encrypting User Data):
from cryptography.fernet import Fernet
# Generate a key for encryption
key = Fernet.generate_key()
cipher_suite = Fernet(key)
# Encrypt sensitive user information (e.g., account password)
password = b"SuperSecretPassword"
encrypted_password = cipher_suite.encrypt(password)
print(f"Encrypted Password: {encrypted_password}")
# Decrypting the password
decrypted_password = cipher_suite.decrypt(encrypted_password)
print(f"Decrypted Password: {decrypted_password}")
7. Testing and Quality Assurance
• Languages: Python (for automated tests), Jest (for JavaScript testing)
• Purpose: Unit testing, integration testing, and debugging.
• Example Unit Test (Python using unittest):
import unittest
def add(a, b):
return a + b
class TestGameFramework(unittest.TestCase):
def test_add(self):
self.assertEqual(add(10, 20), 30)
if __name__ == '__main__':
unittest.main()
8. Order of Implementation
• Phase 1: Focus on core system architecture (low-level C/C++) and OS development.
• Phase 2: Develop SDK and start building the basic framework for UI and input management.
• Phase 3: Work on backend systems like the digital store and integrate with front-end UI.
• Phase 4: Begin rigorous testing and debugging using automated test suites.
This comprehensive approach gives you a detailed idea of how each component can be built using a combination of these languages and technologies. Each section is modular, allowing teams to work on different aspects of the console simultaneously.
[Pixel Art/Sprites/Buildinodels/VictoriaSecretPixelArtKit #Playstation7 #DearDearestBrands]


#victoriasecretrunway#runwaybattle#victoria secret fashion#victoria secert model#victoriasecret#victoria secret#VictoriaSecretRunwayBattle#capcom victoriasecret#capcom videogames#playstation7#ps7#deardearestbrands#capcom#digitalconsole#python#script#rawscript#raw code#framework#VictoriaSecretVideoGame#deardearestbrandswordpress
0 notes
Text
you fuckers are really hating on travis when hes literally a he/him lesbian, also the wilderness is literally a metaphor for girlhood do you really think it wouldve let him survive if he wasnt at least a little girl coded ?
#a bunch of ppl have said this in more smarter ways but i feel like the yj fandom needs to be reminded of this every once in a while#also hes just genuinely a fascinating character?? like he saved his girlfriend only to have to eat his brother#he ate his brothers heart RAW#literally that scene is so insane and underrated#like yall went crazy about shauna eating jackies ear (which fair because it is gen insane) but not over travis eating his brothers heart ra#(which equally as insane)#any ways sorry for the rant my tags are always so long 💀#i am gonna make a more analysis type post on this once i rewatch the show#yellowjackets#travis martinez#travnat#(its not my fav ship but its still toxic yuri so i have no choice but to stan)#javi martinez#🐝#also i get not wanting to see any men in this show about women but guys please he’s literally just a girl as well#hate on jeff or waltor or kevyn or the weird cop dude just leave travis alone 🙏🙏😭#also not girl coded in the way some men are. hes girl coded in the more literal sense#gender fluid travnat my beloved they are so t4t <33
71 notes
·
View notes
Text
CHYNA & EDDIE GUERRERO WWF (2000)
"People have asked me about Eddie Guerrero many times. I would like to keep my memories of him to myself. However, for this event, let me say that Eddie was a talented performer & a very good friend. He always made me feel special in the ring. R.I.P. to the great Eddie Guerrero. I love you and miss you, from your “Mamacita” " - Chyna
(huge cred to FullWithDivas for the videos!)
#chyna#wwe chyna#eddie guerrero#wwf#wwe#wweedit#wrestlingedit#wrestling gifs#attitude era#wwe raw#wwe smackdown#raw is war#pro wrestling#latino heat#flashing lights#cw flashing#that 2nd and 4th gif is so gomez/mortica coded like wow#but eddie i get it I GET IT#love finding all these gems#and seeing chyna mention how much eddie meant to her is so nice to hear!#miss them so much
253 notes
·
View notes
Text
theres no way my babygirls (terror twins) got beat up by those rats (the flopment day) and then so cinematically and artistically made one of the most (sadly) badass shots in wwe

#shit is lookin like a renaissance painting HELLO#i might draw my terror twins soon with the flopment day dead and bleeding around them 🤑#thatd be MORE renaissance painting coded#wwe#wwe lb#wwe monday night raw#wwe raw#monday night raw#terror twins#the terror twins#rhea ripley#damian priest#the judgement day#the flopment day#LMAO
21 notes
·
View notes
Text
The craziest scene in Hannibal is just a normal day for French veterinarians.
(From Consider This by Chuck Palahniuk)
17 notes
·
View notes
Text
It’s funny to me that the writers of fallout most definitely intended for the audience to attach father daughter vibes to Lucy and Cooper, but have seemingly ended up with most ppl shipping them
I blame the fact that they did not make Lucy an actual child and instead introduced us to her with her shameless and enthusiastic interest in sex
Like
Her first character trait you gave us is that she’s bored and lonely
The dad stuff is new. The “my dad is missing” is not an intrinsic aspect of her history, it’s an entirely new plot point that she’s honestly not approaching with any real sense of terror.
Her first real human thing were shown is that she’s ACHING to be married, to find her person, to connect and build a life.
COOPER has built in family trauma issues. His first scene is LITTERED with family complexes. We’re left wondering if his daughter made it.
Lucy? Lucy watched her dad get kidnapped and then happy go luckied her way on a search and rescue mission. She fully expected for that to be successful, and then resume her mission to find a partner for life. Her first trauma wasn’t even him getting ���napped. It was her husband betraying her and attempting to kill her.
The other meaningful story she has is another romance. She’s not exactly trudging through her narrative with this bleak “give me my father” outlook. Again, that’s cooper.
Her whole arc is becoming the honourable sheriff that Cooper gave up being. She’s literally WEARING HIS COLOURS.
Like. There was no chance in hell that when that lost to the darkness outlaw encountered a flash from the past do gooder and paused his murder spree to take her in, with humour, not aching loss, that anyone was gonna do anything but ship them.
I love a good father daughter thing - I adore them. This? This is so not that.
#doesn’t help that my romance manic sis immediate reaction was OOOH LOVE INTEREST#like you just can’t beat a good enemies to lovers#even if he is QUITE the enemy#like they’re whole thing is so intertwined it’s like crazy#they could’ve made her 17 and it might’ve translated#but she was raw dogging a guy she JUST MET on a table#her first thematic look was bloodied bride#her second the hardened final girl#where she looked like a cowboy#come ON#where’s the daughter coding of that#they didn’t even bond over the family stuff#fallout#fallout prime#spoilers fallout#lucy maclean
23 notes
·
View notes
Text
WIP of my Exclamation!2080 wiki
Yeah I finished three character pages today, translated them and still have so much work to do! Haven't shared with you all my hard work sooo... look what I've done already!
Homepage, characters page, the timeline table, locations page (with interactive map I'm still working on), gallery (with tabs! and other content), Ideas and Concepts page (for uncategorized things like side-story ideas, episodic characters, cool items and other stuff)
For now I've got Abe, Cleo and Gandhi pages on my wiki, but there will be more soon! I hope. Here's the preview of how the character page looks:
You can check it all on my Wiki! And it's RU version, если вы говорите по-русски, как я :D
ruwiki gets updates earlier obviously!
BEFORE YOU VISIT, please turn on "desktop site" or whatever it's called in your browser, if you're on mobile. Mobile wikifandom SUCKS, and it's hard to customize, so I decided to not spend my time and energy on it. Thanks! HTML and CSS rules!
ALSOOOOO for english speakers... If you find any errors in my text, you're free to fix them, since it's not my first language and I'm not sure if i'm good at it 😭
ok enjoy!! Hope you find my AU interesting, I'm working REEEEEALLLYYY hard on it as you see
#clone high#clone high au#custom wiki#exclamation!2080#wiki fandom#yeah look how good i am at web design#haha jk#it's so raw atm but i'll fill it with more information i swear#but at least the site looks good and i had fun writing the code (i cried and had meltdowns)
14 notes
·
View notes
Text
im getting trump ads under gay porn on twitter and its so fucking funny 😭 im looking at boothill porn i can promise you im not intrested in whatever you have to say
#tw politics#sorry for the politics/trump post but it was SO funny#im watvhing a man get rawed and theres an ad underneath like“🦅USE CODE USAFREEDOME45 TO GET 45% OFF THIS $400 SHIRT WITH TRUMPS FACE ON IT”#nsft#mdni#mdni 18+#prettypupp thoughts
7 notes
·
View notes
Text
KÄÄRIJÄ TAROT READING
the stuff I do for you guys I swear
I haven't coded shit since 2008 and I had to call my ex bf so he can help me fix my shit javascript lol ANYWAY
@darkerthanblack-666 made those beautiful tarot cards and I wanted to do readings with them, but printing cards is expensive, so I coded a page that does a classical three cards draw for you
(my ex wasn't able to fix the duplicate glitch either so if you get two times the same card just refresh the page lol)
HERE IT IS HAVE FUN
#käärijä#i spent too long on that shit code#too tired to do actual css in it sorry for the raw state
81 notes
·
View notes