#most things can handle basic code projects but
Explore tagged Tumblr posts
Text
I need a side hustle
#I need a fucking computer#I’m sick of using my phone all the time#I can replace the battery to my laptop but it’s SO expensive#most things can handle basic code projects but#some other stuff I’ll be doing could end up on the heavier side
2 notes
·
View notes
Text
Edgaring time!
Tutorial on how to make your own responsive Edgar :D I will try to explain it in really basic terms, like you’ve never touched a puter (which if you’re making this… I’m sure you’ve touched plenty of computers amirite??? EL APLAUSO SEÑOOOREEES).
If you have some experience I tried to highlight the most important things so you won’t have to read everything, this is literally building a website but easier.
I will only show how to make him move like this:
Disclaimer: I’m a yapper.
Choosing an engine First of all you’ll need something that will allow you to display a responsive background, I used LivelyWallpaper since it’s free and open-source (we love open-source).
Choosing an IDE Next is having any IDE to make some silly code! (Unless you can rawdog code… Which would be honestly impressive and you need to slide in my DMs and we will make out) I use Visual Studio!!!
So now that we have those two things we just need to set up the structure we will use.
Project structure
We will now create our project, which I will call “Edgar”, we will include some things inside as follows:
Edgar
img (folder that will contain images) - thumbnail.png (I literally just have a png of his face :]) - [some svgs…]
face.js (script that will make him interactive)
index.html (script that structures his face!)
LivelyInfo,json (script that LivelyWallpaper uses to display your new wallpaper)
style.css (script we will use to paint him!)
All of those scripts are just literally like a “.txt” file but instead of “.txt” we use “.js”, “.html”, etc… You know? We just write stuff and tell the puter it’s in “.{language}”, nothing fancy.
index.html
Basically the way you build his silly little face! Here’s the code:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Face!</title> <link rel = "stylesheet" type = "text/css" href = "style.css"> </head> <body> <div class="area"> <div class="face"> <div class="eyes"> <div class="eyeR"></div> <div class="eyeL"></div> </div> <div class="mouth"></div> </div> </div> <script src="face.js"></script> </body> </html>
Ok so now some of you will be thinking “Why would you use eyeR and eyeL? Just use eye!“ and you’d be right but I’m a dummy who couldn’t handle making two different instances of the same object and altering it… It’s scary but if you can do it, please please please teach me ;0;!!!
Area comes in handy to the caress function we will implement in the next module (script)! It encapsulates face.
Face just contains the elements inside, trust me it made sense but i can’t remember why…
Eyes contains each different eye, probably here because I wanted to reuse code and it did not work out and when I kept going I was too scared to restructure it.
EyeR/EyeL are the eyes! We will paint them in the “.css”.
Mouth, like the eyeR/eyeL, will be used in the “.css”.
face.js
Here I will only show how to make it so he feels you mouse on top of him! Too ashamed of how I coded the kisses… Believe me, it’s not pretty at all and so sooo repetitive…
// ######################### // ## CONSTANTS ## // ######################### const area = document.querySelector('.area'); const face = document.querySelector('.face'); const mouth = document.querySelector('.mouth'); const eyeL = document.querySelector('.eyeL'); const eyeR = document.querySelector('.eyeR'); // ######################### // ## CARESS HIM ## // ######################### // When the mouse enters the area the face will follow the mouse area.addEventListener('mousemove', (event) => { const rect = area.getBoundingClientRect(); const x = event.clientX - rect.left; const y = event.clientY - rect.top; face.style.left = `${x}px`; face.style.top = `${y}px`; }); // When the mouse leaves the area the face will return to the original position area.addEventListener('mouseout', () => { face.style.left = '50%'; face.style.top = '50%'; });
God bless my past self for explaining it so well, but tbf it’s really simple,,
style.css
body { padding: 0; margin: 0; background: #c9c368; overflow: hidden; } .area { width: 55vh; height: 55vh; position: absolute; top: 50%; left: 50%; transform: translate(-50%,-50%); background: transparent; display: flex; } .face { width: 55vh; height: 55vh; position: absolute; top: 50%; left: 50%; transform: translate(-50%,-50%); background: transparent; display: flex; justify-content: center; align-items: center; transition: 0.5s ease-out; } .mouth { width: 75vh; height: 70vh; position: absolute; bottom: 5vh; background: transparent; border-radius: 100%; border: 1vh solid #000; border-color: transparent transparent black transparent; pointer-events: none; animation: mouth-sad 3s 420s forwards step-end; } .face:hover .mouth { animation: mouth-happy 0.5s forwards; } .eyes { position: relative; bottom: 27%; display: flex; } .eyes .eyeR { position: relative; width: 13vh; height: 13vh; display: block; background: black; margin-right: 11vh; border-radius: 50%; transition: 1s ease } .face:hover .eyeR { transform: translateY(10vh); border-radius: 20px 100% 20px 100%; } .eyes .eyeL { position: relative; width: 13vh; height: 13vh; display: block; background: black; margin-left: 11vh; border-radius: 50%; transition: 1s ease; } .face:hover .eyeL { transform: translateY(10vh); border-radius: 100% 20px 100% 20px; } @keyframes mouth-happy { 0% { background-color: transparent; height: 70vh; width: 75vh; } 100% { border-radius: 0 0 25% 25%; transform: translateY(-10vh); } } @keyframes mouth-sad { 12.5%{ height: 35vh; width: 67vh; } 25% { height: 10vh; width: 60vh; } 37.5% { width: 53vh; border-radius: 0%; border-bottom-color: black; } 50% { width: 60vh; height: 10vh; transform: translateY(11vh); border-radius: 100%; border-color: black transparent transparent transparent; } 62.5% { width: 64vh; height: 20vh; transform: translateY(21vh); } 75% { width: 69vh; height: 40vh; transform: translateY(41vh); } 87.5% { width: 75vh; height: 70vh; transform: translateY(71vh); } 100% { width: 77vh; height: 90vh; border-color: black transparent transparent transparent; transform: translateY(91vh); } }
I didn’t show it but this also makes it so if you don’t pay attention to him he will get sad (mouth-sad, tried to make it as accurate to the movie as possible, that’s why it’s choppy!)
The .hover is what makes him go like a creature when you hover over him, if you want to change it just… Change it! If you’d rather him always have the same expression, delete it!
Anyway, lots of easy stuff, lots of code that I didn’t reuse and I probably should’ve (the eyes!!! Can someone please tell me a way I can just… Mirror the other or something…? There must be a way!!!) So now this is when we do a thinking exercise in which you think about me as like someone who is kind of dumb and take some pity on me.
LivelyInfo.json
{ "AppVersion": "1.0.0.0", "Title": "Edgar", "Thumbnail": "img/thumbnail.png", "Preview": "thumbnail.png", "Desc": "It's me!.", "Author": "Champagne?", "License": "", "Type": 1, "FileName": "index.html" }
Easy stuff!!!
Conclusion
This could've been a project on git but i'm not ready and we're already finished. I'm curious about how this will be seen on mobile and PC,,, i'm not one to post here.
Sorry if I rambled too much or if i didn't explain something good enough! If you have any doubts please don't hesitate to ask.
And if you add any functionality to my code or see improvements please please please tell me, or make your own post!
88 notes
·
View notes
Note
komaedas have you tried straw.page?
(i hope you don't mind if i make a big ollllle webdev post off this!)
i have never tried straw.page but it looks similar to carrd and other WYSIWYG editors (which is unappealing to me, since i know html/css/js and want full control of the code. and can't hide secrets in code comments.....)
my 2 cents as a web designer is if you're looking to learn web design or host long-term web projects, WYSIWYG editors suck doodooass. you don't learn the basics of coding, someone else does it for you! however, if you're just looking to quickly host images, links to your other social medias, write text entries/blogposts, WYSIWYG can be nice.
toyhouse, tumblr, deviantart, a lot of sites implement WYSIWYG for their post editors as well, but then you can run into issues relying on their main site features for things like the search system, user profiles, comments, etc. but it can be nice to just login to your account and host your information in one place, especially on a platform that's geared towards that specific type of information. (toyhouse is a better example of this, since you have a lot of control of how your profile/character pages look, even without a premium account) carrd can be nice if you just want to say "here's where to find me on other sites," for example. but sometimes you want a full website!
---------------------------------------
neocities hosting
currently, i host my website on neocities, but i would say the web2.0sphere has sucked some doodooass right now and i'm fiending for something better than it. it's a static web host, e.g. you can upload text, image, audio, and client-side (mostly javascript and css) files, and html pages. for the past few years, neocities' servers have gotten slower and slower and had total blackouts with no notices about why it's happening... and i'm realizing they host a lot of crypto sites that have crypto miners that eat up a ton of server resources. i don't think they're doing anything to limit bot or crypto mining activity and regular users are taking a hit.
↑ page 1 on neocitie's most viewed sites we find this site. this site has a crypto miner on it, just so i'm not making up claims without proof here. there is also a very populated #crypto tag on neocities (has porn in it tho so be warned...).
---------------------------------------
dynamic/server-side web hosting
$5/mo for neocities premium seems cheap until you realize... The Beautiful World of Server-side Web Hosting!
client-side AKA static web hosting (neocities, geocities) means you can upload images, audio, video, and other files that do not interact with the server where the website is hosted, like html, css, and javascript. the user reading your webpage does not send any information to the server like a username, password, their favourite colour, etc. - any variables handled by scripts like javascript will be forgotten when the page is reloaded, since there's no way to save it to the web server. server-side AKA dynamic web hosting can utilize any script like php, ruby, python, or perl, and has an SQL database to store variables like the aforementioned that would have previously had nowhere to be stored.
there are many places in 2024 you can host a website for free, including: infinityfree (i use this for my test websites :B has tons of subdomains to choose from) [unlimited sites, 5gb/unlimited storage], googiehost [1 site, 1gb/1mb storage], freehostia [5 sites/1 database, 250mb storage], freehosting [1 site, 10gb/unlimited storage]
if you want more features like extra websites, more storage, a dedicated e-mail, PHP configuration, etc, you can look into paying a lil shmoney for web hosting: there's hostinger (this is my promocode so i get. shmoney. if you. um. 🗿🗿🗿) [$2.40-3.99+/mo, 100 sites/300 databases, 100gb storage, 25k visits/mo], a2hosting [$1.75-12.99+/mo, 1 site/5 databases, 10gb/1gb storage], and cloudways [$10-11+/mo, 25gb/1gb]. i'm seeing people say to stay away from godaddy and hostgator. before you purchase a plan, look up coupons, too! (i usually renew my plan ahead of time when hostinger runs good sales/coupons LOL)
here's a big webhost comparison chart from r/HostingHostel circa jan 2024.
---------------------------------------
domain names
most of the free website hosts will give you a subdomain like yoursite.has-a-cool-website-69.org, and usually paid hosts expect you to bring your own domain name. i got my domain on namecheap (enticing registration prices, mid renewal prices), there's also porkbun, cloudflare, namesilo, and amazon route 53. don't use godaddy or squarespace. make sure you double check the promo price vs. the actual renewal price and don't get charged $120/mo when you thought it was $4/mo during a promo, certain TLDs (endings like .com, .org, .cool, etc) cost more and have a base price (.car costs $2,300?!?). look up coupons before you purchase these as well!
namecheap and porkbun offer something called "handshake domains," DO NOT BUY THESE. 🤣🤣🤣 they're usually cheaper and offer more appealing, hyper-specific endings like .iloveu, .8888, .catgirl, .dookie, .gethigh, .♥, .❣, and .✟. I WISH WE COULD HAVE THEM but they're literally unusable. in order to access a page using a handshake domain, you need to download a handshake resolver. every time the user connects to the site, they have to provide proof of work. aside from it being incredibly wasteful, you LITERALLY cannot just type in the URL and go to your own website, you need to download a handshake resolver, meaning everyday internet users cannot access your site.
---------------------------------------
hosting a static site on a dynamic webhost
you can host a static (html/css/js only) website on a dynamic web server without having to learn PHP and SQL! if you're coming from somewhere like neocities, the only thing you need to do is configure your website's properties. your hosting service will probably have tutorials to follow for this, and possibly already did some steps for you. you need to point the nameserver to your domain, install an SSL certificate, and connect to your site using FTP for future uploads. FTP is a faster, alternative way to upload files to your website instead of your webhost's file upload system; programs like WinSCP or FileZilla can upload using FTP for you.
if you wanna learn PHP and SQL and really get into webdev, i wrote a forum post at Mysidia Adoptables here, tho it's sorted geared at the mysidia script library itself (Mysidia Adoptables is a free virtual pet site script, tiny community. go check it out!)
---------------------------------------
file storage & backups
a problem i have run into a lot in my past like, 20 years of internet usage (/OLD) is that a site that is free, has a small community, and maybe sounds too good/cheap to be true, has a higher chance of going under. sometimes this happens to bigger sites like tinypic, photobucket, and imageshack, but for every site like that, there's like a million of baby sites that died with people's files. host your files/websites on a well-known site, or at least back it up and expect it to go under!
i used to host my images on something called "imgjoe" during the tinypic/imageshack era, it lasted about 3 years, and i lost everything hosted on there. more recently, komaedalovemail had its webpages hosted here on tumblr, and tumblr changed its UI so custom pages don't allow javascript, which prevented any new pages from being edited/added. another test site i made a couple years ago on hostinger's site called 000webhost went under/became a part of hostinger's paid-only plans, so i had to look very quickly for a new host or i'd lose my test site.
if you're broke like me, looking into physical file storage can be expensive. anything related to computers has gone through baaaaad inflation due to crypto, which again, I Freaquing Hate, and is killing mother nature. STOP MINING CRYPTO this is gonna be you in 1 year
...um i digress. ANYWAYS, you can archive your websites, which'll save your static assets on The Internet Archive (which could use your lovely donations right now btw), and/or archive.today (also taking donations). having a webhost service with lots of storage and automatic backups can be nice if you're worried about file loss or corruption, or just don't have enough storage on your computer at home!
if you're buying physical storage, be it hard drive, solid state drive, USB stick, whatever... get an actual brand like Western Digital or Seagate and don't fall for those cheap ones on Amazon that claim to have 8,000GB for $40 or you're going to spend 13 days in windows command prompt trying to repair the disk and thenthe power is gong to go out in your shit ass neighvborhood and you have to run it tagain and then Windows 10 tryes to update and itresets the /chkdsk agin while you're awayfrom town nad you're goig to start crytypting and kts just hnot going tot br the same aever agai nikt jus not ggiog to be the saeme
---------------------------------------
further webhosting options
there are other Advanced options when it comes to web hosting. for example, you can physically own and run your own webserver, e.g. with a computer or a raspberry pi. r/selfhosted might be a good place if you're looking into that!
if you know or are learning PHP, SQL, and other server-side languages, you can host a webserver on your computer using something like XAMPP (Apache, MariaDB, PHP, & Perl) with minimal storage space (the latest version takes up a little under 1gb on my computer rn). then, you can test your website without needing an internet connection or worrying about finding a hosting plan that can support your project until you've set everything up!
there's also many PHP frameworks which can be useful for beginners and wizards of the web alike. WordPress is one which you're no doubt familiar with for creating blog posts, and Bluehost is a decent hosting service tailored to WordPress specifically. there's full frameworks like Laravel, CakePHP, and Slim, which will usually handle security, user authentication, web routing, and database interactions that you can build off of. Laravel in particular is noob-friendly imo, and is used by a large populace, and it has many tutorials, example sites built with it, and specific app frameworks.
---------------------------------------
addendum: storing sensitive data
if you decide to host a server-side website, you'll most likely have a login/out functionality (user authentication), and have to store things like usernames, passwords, and e-mails. PLEASE don't launch your website until you're sure your site security is up to snuff!
when trying to check if your data is hackable... It's time to get into the Mind of a Hacker. OWASP has some good cheat sheets that list some of the bigger security concerns and how to mitigate them as a site owner, and you can look up filtered security issues on the Exploit Database.
this is kind of its own topic if you're coding a PHP website from scratch; most frameworks securely store sensitive data for you already. if you're writing your own PHP framework, refer to php.net's security articles and this guide on writing an .htaccess file.
---------------------------------------
but. i be on that phone... :(
ok one thing i see about straw.page that seems nice is that it advertises the ability to make webpages from your phone. WYSIWYG editors in general are more capable of this. i only started looking into this yesterday, but there ARE source code editor apps for mobile devices! if you have a webhosting plan, you can download/upload assets/code from your phone and whatnot and code on the go. i downloaded Runecode for iphone. it might suck ass to keep typing those brackets.... we'll see..... but sometimes you're stuck in the car and you're like damn i wanna code my site GRRRR I WANNA CODE MY SITE!!!


↑ code written in Runecode, then uploaded to Hostinger. Runecode didn't tell me i forgot a semicolon but Hostinger did... i guess you can code from your webhost's file uploader on mobile but i don't trust them since they tend not to autosave or prompt you before closing, and if the wifi dies idk what happens to your code.
---------------------------------------
ANYWAYS! HAPPY WEBSITE BUILDING~! HOPE THIS HELPS~!~!~!
-Mod 12 @eeyes
54 notes
·
View notes
Note
Juno, out of curiosity, what does an accountant DO? What does it mean to be one? Because I know there's math involved. I've heard it's very boring. But I don't know anything else and I'm curious because you're very good at putting things to words.
Okay first of all, I cannot express just how excited I got when I first saw this message. There is nothing I love more than talking about things I know about, and usually when my career is mentioned I don't get questions so much as immediate "Oh, bless you" and "I could never"s. Which- totally fair! For some people, accounting would be boring as all hell! But for a multitude of reasons, I adore it.
There are multiple types of accounting. The type most people tend to be more familiar with is that done by CPAs- CPAs, or Certified Public Accountants, are those that have done the lengthy and expensive process to be certified to handle other peoples' tax documents and submit taxes in their name, amongst other things. Yawn, taxes, right? Well, the thing with that is that there's a lot of little loopholes that tax accountants have to remain familiar with, because saving their clients a little more here or getting a little more back there can really add up, and can do a lot for people who, say, have enough money to afford to hire someone to do their taxes but not necessarily enough to be going hog wild with. Public accountants can work for large firms or by themselves, and also do things like preparing financial statements for businesses, auditing businesses to ensure all of their financial transactions are true and accurately reported to shareholders and clients, and consulting on how finances can be managed to maximize revenue (money in - money out = revenue, in very simple terms).
The type of accounting I do is private accounting! That basically just means that I work for a company in their in-house accounting/finance department. Private accounting tends to get split up into several different areas. My company has Payroll, Accounts Receivable, and Accounts Payable.
Payroll handles everyone's paychecks, PTO, ensuring the correct amount of taxes are withheld from individuals per their desires, and so on. Accounts Receivable handles money flow into the company- so when our company sells the product/service, our Accounts Receivable people are the ones who review the work, create the invoices, send the invoices to the clients, remind clients about overdue invoices, receive incoming payments via ACH (Automatic Clearing House- direct bank-to-bank deposits), Wire (Usually used for international transactions), or Check, and prepare statements that show how much revenue we are expected to gain in a period of time, or have gained in a period of time. This requires a lot of interfacing with clients and project managers.
My department is Accounts Payable. Accounts Payable does basically the other side of the coin from what Accounts Receivable does. We work mostly with vendors and our purchasing/receiving departments. We receive invoices from people and companies that have sold us products/services we need in order to make our own products/perform our services, enter them into our ERP (Enterprise Resource Planning, a system that integrates the departments in a company together- there are many different ERPs, and most people simply refer to their ERP as "the system" when talking internally to other employees of the same company that they work at, because saying the name of the system is redundant) using a set of codes that automatically places the costs into appropriate groups to be referenced for later financial reports, and run the payment processing to ensure that the vendors are being paid.
To break that down because I know that was a lot of words, here's some things I do in my day-to-day at work:
- Reconciliations, making sure two different statements match up: the most common one is Credit Card reconciliations, ensuring that there are appropriately coded entries in the system that match the payments made on our credit line in our bank.
- Invoice entry: this is basic data entry, for the most part. This can have two different forms, though
- Purchase Order Invoice entry: Invoices that are matched both to the service/product provided from the vendor and the purchase order created by our Purchasing/Receiving department. We ensure that the item, the quantity, and the price all match between our records, the purchase order, and the invoice, before we enter this.
- Hard Coded Invoice entry: Invoices that we enter manually due to there being no Purchase Order for them. This is often recurring services, like cleaning or repairs, that may happen too often or have prices vary too much for Purchase Orders to be practical.
- Cleaning up old purchase orders: sometimes Purchase Orders are put in the system and then never fulfilled. Because this shows on financial statements as being a long-standing open commitment, it looks bad, so we have to periodically research these and find out if the vendor simply didn't send us the invoice, if the order was cancelled, or if something else is going on.
- Forensics! This is my personal favorite part of the job, where someone has massively borked something that is affecting my work, and so I go dig into it, sometimes going back as four or five years in records to find the origin point of the first mistake, and untangling the threads of what happened following that mistake to get us to where we are today. There's an entire field called Forensic Accounting that is basically just doing This but for other companies (it's a subset of auditing, and often is done via the IRS) and that's my dream position to be totally honest. I loooove the dopamine hit i get with solving the mystery and getting praised for doing so faster than anyone else has even begun to realize the problem to start with.
- Balancing Credits/Debits: This is more of a Main Accountant role thing, but the long and short of it is that every business has Assets, Liabilities, and Equity. Liabilities and Equity are what we put into the company/what we owe, and assets are what we have received/what we are owed. Anything that increases Assets or lowers Liabilities or Equity is a Debit. Anything that decreases Assets or raises Liabilities or Equity is a Credit. Every monetary change we process has to include an equal Debit and Credit. This is its own whole lecture, so if you wanna know more about double-entry accounting, let me know, but it's yawnsville for most people.
- Actually cutting checks or initiating bank payments to vendors for amounts we owe them.
- Vendor communication: I'm on the phones and email a lot with vendors who are wondering where their payment is, or why something was short-paid, or if I can change some of their info in our system, and so on and so on. Every job is customer service, unfortunately. I don't love it, but I do a lot less of it in private accounting than I would have to do in public accounting.
- Spreadsheets: I make so many spreadsheets I am a goddamn Excel wizard. I love spreadsheets. This isn't necessarily accounting-specific though, most people in Finance jobs love spreadsheets, or at least use them to make their lives easier. I make them just for fun, because I'm a giant fucking nerd who finds that kind of thing enjoyable lol. So if you ever need a spreadsheet made for anything, hit me up.
As for math, that's a pretty common misconception. While there is math, it is very rarely more complicated than "I paid $3 of the $8 I owe, now I owe $5" for me. There are some formulas you learn in school (Business Administration with a focus in Accounting is what I studied), but they're also pretty standard and rarely include more than like... basic algebra. Which. Thanks @ god because I flunked so hard out of pre-calc in college. I could not have done accounting if it really were all that math heavy.
Aaaand yeah! That's all I've got off the top of my head- if you have any more questions about it, do let me know, I'm happy to ramble on for hours, but I'm cutting it here so I don't start meandering on without direction lol.
33 notes
·
View notes
Text
My Jay, Nya, Pixal, Zane, Kai, and Lloyd Headcanons about their expertise in STEM
I was struck by inspiration and I just had to write it down. It doesn't help that I'm taking a programming class and have a bunch of STEM friends and family, so I was given plenty of ideas
Please excuse any spelling and grammar mistakes. I forgot to switch my keyboard from Portuguese to english
Jay
He is good with circuits and hardware
If he had the funds and motivstion, he would have been an electrical engineer, or at least become certified as an electrician
He practically already has the experience since he always tinkered with circuits and wires as a kid
His parents would always have him fixing the toaster, fridge, TV, basically any electrical appliance at home
He never had once touched a computer program until he came across his first computer at the junkyard
After tinkering with it and booting it up, he began to mess around and trid his best to understand the programs in it
He understood a little, but he wasn't a pro at it until Pixal came along (she taught him about different programming languages and the fundamentals, and he found out he's not into programming as much as he is with messing with circuits)
He does know mechanics and car stuff thanks to his dad, but he's more interested in wires and circuits
Nya
Mechanic
She definitely has a certification as a mechanic
Thanks to Kai, she had the time to study for a technical education
She also knows enough about electrical engineering to make all the cool cars and mechs
She didn't know very much about programming but she was very interested in it
She went to the library and read outdated books on programming (the local library near their village isn't the most well funded)
Hey, at last she grasped the fundamentals even though she learned an ancient language that most programmers don't use or learn anymore
When Pixal joined the team and started to teach her about programming, she was ecstatic
Now all her inventions could be more modern!
Pixal
She is just like her dad, so robotics and computer science are her thing
She does know a bit about everything since she has an entire database in her head to glean info from
Still, programming is her favorite thing and the thing she considers herself the best at
She leaves vehicle maintenance to Nya and any circuitry to Jay
When Jay, Nya, or Zane aren't around, she handles all the things they handle even if she doesn't enjoy it as much as programming. Someone has to be there to take care of all their tech
Whenever there's a bug she can't seem to fix, she explains her code to Zane to help herself think
Zane listens and i happy to be helping his gf <3
When Zane isn't there, she talks to Nya, her bestie, instead
When neither are there, she talks to Jay
Jay only listens since he's not very experienced with programming, but he does try to help from time to time
Zane
Zane doesn't consider himself to be an expert in any tech related field
Like yeah, he knows basically everything and can do it all, but he's not very interested in them
He will fix cars or fix a line of code, but only when it's necessary or if he's asked to
Unlike the others, he doesn't spend his free time inventing or tinkering with anything
He's mostly meditating or hanging out in nature
He likes plants, like, A LOT
Plants, cooking, and animals are his expertise
He also knows a lot about herbs and tea, so he's basically the team's medic
Pixal is his medical assistant just like he is her programming assistant <3
Zane and Cole go out on walks and hikes a lot, and they drag Kai along just for fun
Kai
He knows a bit about mechanic since he sometimes helped Nya with her homework and projects
Him and Nya know exactly what they're talking about when Jya asks him to bring her the thingamabob
Kai had to teach Jay what the hell a thingamabob and a doohickey is so Jay could actually help Nya
In return, Kai learned some of Jay's weird names for tools like the thing and thingamajig
If things are really dire (like none of the team's inventors and Zane are with them) then Kai will try to fix a vehicle
50% chance of failure, 49% chance of success
The 1%? Who knows
It explodes
Lloyd
Like Kai, he knows a bit about mechanics, if not more because he spent a lot of time with Nya when he was younger and when nobody knew he was the green ninja
He has more success fixing vehicles than Kai, so Kai is always the last resort
Lloyd also knows about plants and herbs since he read about them after Wu was gone
Someone had to take care of all the plants for Wu and Zane after the merge happened
Lloyd also knows a bit of programming and electrical engineering, though it's not very useful
He could tell you what a variable is but he cannot write a program to save his life
He can tell you what XOR does, but he can't build a circuit
Due to his interest in video games, he's been trying to learn programming, but due to everything that happened in his life, he hasn't learned much
He's still trying, and that's what matters
#ninjago#lego ninjago#lego#nya smith#kai smith#lloyd montgomery garmadon#lloyd garmadon#pixal#zane julien#ninjago pixal#pixal ninjago#ninjago headcanons#headcanons#jay walker#ninjago kai#ninjago nya#ninjago jay#ninjago lloyd#ninjago zane#janitors headcanons
37 notes
·
View notes
Note
I do not get how Sun can be blamed for Eclipse, there’s so many reasons why not
Sun AND Moon had no idea he was there, even Moon erroneously thought he just left some inert code in there that wouldn’t turn into something, but Sun had no idea because Moon never told him and Eclipse didn’t say anything right up until after July 16th
They blaming Sun’s bad thoughts is some of the most victim-blaming nonsense I’ve ever heard, this is the kind of belief people with OCD rail against because EVERYBODY has bad thoughts and/or intrusive thoughts, especially if they’re as abused and neglected as Sun is, the only thing that matters is if you act on them and Sun never did, he’s not evil for just having mean thoughts, it’s an excuse
Some argue Eclipse was upset with Sun for not standing up for himself and that’s why but that’s also nonsense, this is about as idiotic as Moon abusing Sun to “make him have a spine”, that’s NOT how you do it that’s basic abuse 101, if Eclipse was really seeing Sun’s true thoughts on his mistreatment and really cared he wouldn’t have made Sun’s life twice as awful
And then there’s Solar, living proof every choice Eclipse made didn’t have to happen, he could’ve chosen to be better at any point and all the bad was his choice, Solar proves he could’ve approached this all differently and got what he wanted sooner, he didn’t have to make himself everyone’s enemy, or at least Sun’s enemy as Sun even tried to plead with him even AFTER July 16th(which says something because they didn’t know about Bloodmoon, they thought Eclipse did it, so Sun trying to make nice with and beg the presence that killed the children he was closest too is a lot, even if it’s partially out of fear he had every right to hate Eclipse but he instead tried making peace)
All of the arguments people give for blaming Sun for Eclipse really irk me as it is victim-blaming, the one who’s far more responsible is Moon because he made Eclipse in the first place, but instead of just focusing on the perpetrator Eclipse just had to lash out at Sun instead
You're absolutely right, dear anon! Thank you for sharing your thoughts with me ^^
I needed this more than you could think
My theory or take on this is that despite Eclipse hearing Sun's most deep hidden thoughts he didn't understand Sun..
I mean I think that Sun's inner thoughts were intrusive thoughts and thoughts that he's bad.. so I think that this is where Eclipse's words came from.. that Sun isn't better than them and he's just lying.. and he tried to show that to Moon..
But despite that Sun didn't stand up for himself.. despite Eclipse being sure that Sun would be capable of doing so and more.. Sun not only didn't stand up for himself but also he stayed with Moon and believed in him..
Hence Eclipse's words that he hated how Sun was handling himself..
Eclipse doesn't understand Sun.. he doesn't know that Sun's inner thoughts - from what I've gathered - are just his delusional perception of himself with also intrusive and impulsive thoughts and paranoia..
None of the things that happened to Eclipse were Sun's fault.. and yeah Eclipse should've focus more on Moon for leaving him behind abandoning him in Sun's head.. and for being abusive towards Sun as Eclipse could only watch..
Same was with Moon at the beginning.. instead of being angry at FazCo. he had to lash out at Sun who was nice and tried to help him..
Sun was really unfairly treated in life.. he got the worst hand..
And Eclipse was too bitter to care.. but I think that he still felt something.. because he was shocked by Sun's outbursts.. because he doesn't understand Sun..
And I think that he realized that Sun wasn't at fault like previous versions of him thought.. but I agree with goodolddumbbanana that Eclipse doesn't like to admit that he's wrong hence why he acts like the Sun from eaps is actually just like our Sun was.. even if that's not true.. but I think that he's just projecting to not have to face the truth fully.. because then he had to admit that he was wrong and he had to apologize for what he's done to Sun.. (even though it wasn't exactly him but he treats it like that)
But you're absolutely right that fans are awful for victim blaming Sun for Eclipse's situation.. because none of this was his fault.. even a tiniest bit..
Sun doesn't deserve such treatment especially when we see how much he tries to be better and do good and be kind.. despite everything..
Thank you once again, dear anon ^^
#anon#dear anon#anon ask#ask answered#sun and moon show#sams#sams sun#sams moon#sams eclipse#eaps eclipse#tw abuse#tw delusion
24 notes
·
View notes
Note
Hewwo could I pleasd possibly get C F K L and T for my robot king Franky? I love him he's so underrated! Let him hit cause he's silly coded man!
I completely agree, Franky deserves so much more love than he gets! Honestly, he would probably be the Straw Hat I would hang out with the most besides my man Sanji.
Cruelty: How would they treat their darling once abducted? Would they mock them?
Right off the bat, he's treating you like you're a part of the family and as if you've been here for way longer than you really have. He's "recalling" stories of you together that never happened and there's nothing you can do or say to get him to admit that they aren't real.
For as lax and carefree as he acts, he's very sharp and pays close attention to you, making sure that you're never alone and easily thwarting any and all escape attempts. He never reacts with anger, he just casually redirects you and acts as if nothing happened. He has a sort of 'you'll attract more flies with honey than vinegar' approach to his darling. He's sure that if he keeps showing how nice he is, you'll realize how good this is for you and accept him. There's no mocking from him for that reason. He makes himself very hard to hate.
Fight: How would they feel if their darling fought back?
Given how strong he is and the fact that he's made of metal, it's highly unlikely that you'll be strong enough to even make him flinch. He takes you fighting back the same way he handles escape attempts. He brushes it off and redirects. He'll give you something to break or rip apart as a better means of getting out your anger. It would be a really nice gesture if he wasn't holding you against your will.
Kisses: How do they act around or with their darling?
He's your personal hype man. You can't even pour yourself a glass of water without him striking a pose and holding up a sigh with a "10" on it for your technique. It's ridiculous and excessive, but he won't stop. The man follows you around everywhere while hyping you up to literally anyone he sees. He doesn't understand that it might be more than a little embarrassing to have some pantsless man stalking you while calling attention to you constantly, he just sees this as him boosting your confidence.
In private, more quiet moments, he loves to have you around him while he's working on his most recent project. He's fine with you just doing your own thing, but he's going to try his damnedest to rope you into working on his project with him, even if you're just handing him tools. He'll talk you through what he's doing because he loves teaching people new skills, and he hopes you'll take an interest in this.
Love letters: How would they go about courting or approaching their darling?
Very boldly. He abruptly inserts himself into your life while acting like you're old friends and is immediately trying to recruit you into his family/the Straw Hats. He'll be right there for every part of your daily routine while trying to help you or cheering you on with a guitar riff as you do basic tasks. It's all very confusing and overwhelming for you.
Being the handyman that he is, he'll invite himself into your home and try to upgrade everything you have as a means of endearing himself to you. He ignores silly questions like "Who are you?!" and "Why are you in my house?!" while he shows off the new functions he added to your miscellaneous household appliances. Afterwards, he invites you (read: drags you) to a cookout with him and his family/crew. You probably won't be going home after it.
Tears: How do they feel about seeing their darling scream, cry, and/or isolate themselves?
He really doesn't like it when you cry. It's one of the rare moments where he drops his goofy confidence and looks genuinely unsure of what to do. He wants to see you happy and thriving. Seeing you cry hurts him and makes him reassess his approach with you. Unfortunately, he never thinks that letting you go is what you need, so his new techniques usually boil down to more one on one time doing different things that might improve your mood.
Isolating yourself is pointless. He'll just scoop you up and carry you back out where everyone else is while lightly scolding you because "everyone missed you and was worried".
#cyborg franky#franky the cyborg#franky x reader#yandere one piece#one piece x reader#one piece x you#one piece x y/n#yandere alphabet#yandere#i'm sorry if this felt platonic i swear i wrote this with romantic intentions#one piece#reader insert#x reader
51 notes
·
View notes
Note
I'm very sorry. Six months ago you commented on a Gordon Ramsey accessibility post that your job to help make websites accessible. How do I get that job? I would love that so much. I'm sure you've answered this before but I can't find it.
I, too, am sure I've answered this before, but I can't remember where and I'm not about to brave the Tumblr search feature to go looking, so you and @the0dd0ne get a twofer.
Hi, I'm not a bot, and I was wondering if I could ask you a weird career question? I saw your addition on that "Accessibility Nightmares" post where you mentioned it's your actual job to email websites about their lack of accessibility and what they need to do to make it accessible, and can I ask how you got into that? I got injured on the job and need to make a huge career change, and that type of work has always been really interesting to me, but I don't even know where to start to get into it! Also feel free to ignore this lol I know it's out of left field.
(This is actually the third question I've got on this, so no, not that out of left field.)
So the first thing to understand is that it's actually pretty hard to get into digital accessibility because there just aren't that many companies doing it. As far as I know from company meetings there aren't that many schools teaching it as a part of their core web development curriculum. It's just not that common to think about it as part of web development. Which is vastly irritating.
I started mucking around with the web when there was first a web to muck around on, but when the pandemic hit and my Mom suggested (in a hilarious twist of circumstances) that I go to one of those Learn to Code boot camps to get a certificate that said I actually knew my shit so I could get a job in web development. A number of these boot camps also have job placement programs and pipeline agreements with certain companies. and in a nutshell that's how I got into it. The company sent my boot camp a letter saying "we need N warm bodies" and they sent the company a list of names, I got interviewed, I got hired as a contractor, and after a couple years of good work for them I got invited to interview for a permanent position, which I got.
These days due to the state of the everything, there are probably 10-50 programmers for every open development position, depending on language and job type and company. It's a rough field out there and I got very, very lucky in my timing. But if you want to try it, the boot camp to job pipeline is probably your best bet. Ask the boot camp recruiters if they have connections to accessibility firms. If they don't, you can always try asking if they have connections to web development/site packaging firms and then check if the firms have an accessibility department. Tell the recruiter up front what you're looking to work in, and keep in mind that the recruiter's job is to convince you to give the boot camp your money. (Mine was $12k USD.)
For resources to study in the meantime, there's the A11y Project which has discussions, videos, articles, posts, etc about digital accessibility, a lot of good information. You can also look at the resources for the CPACC exam, I don't recommend taking it unless you have a few hundred USD to burn but you can definitely study up on the Body of Knowledge, which is a free PDF to download. And there is, in fact, an accessibility job board, although I don't have any experience with applying for any of these jobs cold.
The languages I use most in my job are HTML and jQuery, and I passively use (meaning I read and interpret but don't actually program in) JavaScript and CSS. This is mainly because we work with client sites and there's only so much of the client code we can touch; if there's a problem in the client code we can't touch we have to write it up and tell them to fix it. If you end up in house for some large brand you may end up working in more web development languages, but a lot of accessibility can be handled by basic HTML attributes called ARIA attributes (and roles) and there's the documentation on that. Another tool to have is your soft skills: communication, specificity of language, writing up good descriptions of what code does what so you can explain exactly what needs to be fixed where and why. You might also want to look at documentation on what makes good alt text, where it's needed, what kind of labels are standard, etc. I think you can find that in the A11y Project pages, but honestly I just learned it on the job working with senior developers.
It's a hard time to get into software development at all, let alone a niche field like web accessibility. But Europe is about to have a digital accessibility law come into effect in July of next year (that encompasses more than just the web, that's just my area of expertise) and the US is making slow but steady strides in requiring digital accessibility as well, so there are jobs out there and there might be companies hiring to capitalize on the need. There will definitely always be companies putting off conforming to regulations until the last possible minute, and then needing services and specialists. So study up, practice, and good luck!
22 notes
·
View notes
Text
Combinatorics of n-Dimensional Chess
So my current project of n-dimensional chess has involved perhaps, some of the most interesting combinatorics I've ever handled. Let's talk about them! It's a perfect showcase of what I think are the 3 most important parts of math 1.> Start with simple, easy examples 2.> Break large problems into smaller parts 3.> Shift your perspective and abstract problems in a way that they are easier to deal with, while retaining a way to shift back once you've solved it to reapply what you've done. So, let's first start with the problem. Here's a piece in 5D chess known as a unicorn. It can move along any triagonal, which means it has to pick 3 out of the 5 dimensions available. in 4D time travel chess, it actually gets to move in 6 dimensional space, but it was originally introduced in space chess, a 3D variant. My point here is that n-dimensional chess means that we have to be able to list these kinds of moves for a lot of different dimensionalities, and hard coding them quite simply isnt an option. So, we turn to combinatorics!
We'll start with point 1.> by starting with our easiest example. Let's take a look at a bishop's movement in 2D space first to get a good baseline. The directions a bishop can travel along in 2D space are as follows
Now I purposely arranged this in a way that if we replace negative numbers with 0, we get
And hey! That's just counting in binary! Which makes sense, because we only have two choices, positive and negative. Once we move up to 3D space, we can actually re-use this tactic as well. Let's list all the possible combinations of directions in 3D space first, without worrying about the sign. This is what I mean in part 2.> by breaking complex problems into smaller ones, in this case breaking the movement into directions and signs.
Basically, now we can take each one of these 3 possibilities and just apply our same trick by ignoring whichever part the 0 is in. And this works for triagonals and quadragonals in higher dimensional space as well. This makes iterating through signs the easier part Of course, that means the other half is going to be the hard part. Now, with diagonals it isn't too bad. Lets look at diagonals in 5D space. Get ready because this one is a bit more of a jump
Basically what I'm doing here is I'm starting with each 1 as far left as possible, and sliding them over repeatably. not the best explanation, but its doesn't matter because this method doesn't extend well to triagonals very well (choosing 3 directions at once). Instead, we take a shift of perspective, demonstrating point 3.> Instead of representing this as a series of 1's and zeros, we simply represent the *position* of each 1. For example
We have a 1 in the 0th and 3rd locations, so we can simply represent this as just (0, 3). Suddenly, instead of trying to figure out how many ways there are to arrange 1's and 0's, we are asking how many ways can we choose 2 numbers out of 0-4. Now, there is a slight catch in that (0, 3) and (3, 0) are the same thing, so when counting them order doesn't matter, making this a simple combination problem. However we don't just want to count them, we also want to iterate over them. Lets try to generate all possible triagaonals within 7D space. That means we want to choose 3 numbers ranging from 0-6. We start with our easiest one
In order to avoid repeats (no pun intended) we maintain a strict order that is always increasing. Each number should always be larger than the previous one. To iterate through new combinations, we simply increase the last number until we can't anymore.
Now at this point, we can't increase the last number any more, so we increase the second to last and start over
And since the number after 2 has to be larger than 2, the smallest number we can roll over to is 3. From here we continue. Basically, it turns this complicated problem into a type of counting game. Just like with regular numbers, we increase the last one until it can't go anymore, then we increase the next one over and restart, just instead of restarting to 0, we restart to the smallest allowed number that keeps things in order.
And at this point, we now have to increase our first number. I'll go ahead and finish this up despite it being pretty long, so that we can make sure we have all the possibilities
Now if we do some quick calculations for how many combinations we have of 3 numbers out of 7 total we get 3 choose 7 = 7! / ( 3! * (7-3)! ) = 7! / ( 3! * 4! ) = (7 * 6 * 5) / (3 * 2 * 1) = (7 * 5) = 35
Which is exactly how much we counted to! There are a few reasons we know there aren't any repeats but this has gotten long enough, and I can't quite articulate them off the top of my head. From here we convert back into 1's and 0's, then we count up in binary for each one of them to get the sign permutations. To wrap things up, I'll finish up by demonstrating all those sign permutations for one of these direction combinations. As stated in point 3.> we can translate back from our abstraction back to the original problem, so we can apply the smaller part that is generating the sign permutations.
There we go. A little bit of a mess, but that's it! And that's how I generate arbitrary m-agonals in arbitrary n-dimensional space for my chess engine. Finally, here's a picture of a queen's movement allowed to move along any agonal up to heptagonals (seven!) on a 7D board generated in a precursor to my current iteration of nDimensional chess
Y'know, not that anyone would ever play that lol. Here's a bishop on the same board, which is (somewhat) more... manageable lol.
And that's all I got! this math right here is the bread and butter of what makes n-dimensional chess possible! and is potentially one of my favorite problems I've worked on.
#mathblr#math#programming#combinatorics#chess#chessboard#5D Chess#gamedev#progblr#codeblr#coding#mathematics
125 notes
·
View notes
Text
Lyoko Warriors Run A D&D Game
I wrote something about this for Code: July and said I'd expand on it later And Then I Didn't so
Aelita: After much deliberation, was chosen to be the DM given her skillset making her good at handling both mechanics and storytelling (though the others occasionally try their hands in one-shots). Was incredibly nervous at first, but naturally settled into the role and thrives in it. Makes a lot of homebrew and gets crazy into setting development. Is very careful about the players' mental states and tries not to mirror any actual event they've been through too closely in her stories, though she can't help projecting a little bit (Mister Puck shows up as an NPC wherever she can fit him, she focuses a lot of plots around family crises and the importance of bonds between people, always puts emphasis on hope and happy endings, even if they're difficult to reach). Can be absolutely cruel in regard to making enemy encounters strategically difficult, but only because she knows the others will somehow make it through. Has a DMPC elf druid who acts as a healer (and shamelessly flirts with Jeremie's character)
Jeremie: Was also considered for the DM role, but declined--he admitted that while he'd read the rulebook before and would do great with mechanics, he'd make a poor storyteller. It's also kind of cathartic for him to be with the party of fantasy action heroes for once. He made a human wizard initially as a poorly-hidden, powered-up self-insert, focusing on spells that would mechanically and strategically benefit the party, but as he gets into the story the character comes into its own and he branches out with spells he just thinks are cool. The designated Only One Who Remembers What Happened Last Session, and usually the first to see through Aelita's battle gimmicks, though whether or not he tells the others about them immediately is a toss-up.
Odd: Made a nonbinary dwarf bard before most of the group knew what nonbinary was (mid-2000s, not a well known thing despite two of them being trans). Takes up the healer role when Aelita can't juggle her PC. Often has to be gently nudged out of the spotlight and reminded he isn't playing the only main character, but Aelita can always rely on him to take her hooks and drag the others along with him, or force the rest of the party to actually talk to each other. Basically that one really talkative, enthusiastic player in the group who always fills any awkward silences.
Ulrich: Initially wasn't too interested in this whole thing and was just joining to hang out with his friends. Has a dragonborn fighter who eventually multiclasses into monk. Also started off as very much a self-insert character, and Ulrich is occasionally a bit of a difficult player because he has trouble with his character being wrong and screwing things up. Eventually, though, he starts to loosen up, sometimes rivaling Odd as the spotlight-stealer because of his character's dedication to their team and "awesome dragon powers"
Yumi: Originally tried to make a healer until Aelita sussed out she didn't actually want to play support and was only doing it because she thought she had to; after some nudging, she made a human rogue assassin instead. She still winds up being the party's dedicated problem-solver and only sane man, the one who'll actually try the doorknob to see if it's locked before Jeremie's character makes a dangerous attempt to teleport past it or Ulrich's character tries to kick it in. The most quiet person at the table, but when she does speak up, it's clear she's put a lot of thought into her character's backstory, how she behaves, and her relationships and opinions on others. Always describes her attacks in battle, and encourages Ulrich into eventually doing the same.
William: Was invited by Aelita to mend his relationships with the rest of the group, and is the silently-acknowledged exception to the "don't play anything too close to Lyoko" rule since he's still working through some crap and this is the only way he'll actually open up to the others. As such, has a tiefling warlock afraid of his own powers and patron that's difficult to integrate into the group at first because of William's own reluctance and being one of those infamous brooding edgy loner characters. Eventually, William shows the character has a very notable soft side and gets him in somewhat good graces with the others. Is incredibly dramatic and clearly using this as an acting outlet, but is also very good at said acting and tends to get the rest of the group swept up in his energy.
38 notes
·
View notes
Text
Wrap030-ATX First Code
This is a big step forward — Wrap030-ATX, my microATX form factor 68030-based homebrew computer, is running code from ROM. Externally, all it's doing is blinking an LED, but that LED is software-controlled, with a sizable delay loop between blinks to make it something that is human-visible.
Getting to this point took quite a bit of work after the free run tests. Nearly all of the logic on this project is in CPLDs. Of note here is the primary bus controller, which handles access timing, bus cycle termination, and a settings register.
For the computer to run code, it has to be able to read from ROM. Reading from ROM requires the bus controller to decode the CPU address, assert the ROM's Chip Enable (CE#) and Output Enable (OE#) signals, wait the appropriate length of time for the ROM to output stable data on the bus, and then assert the appropriate bus cycle termination signal for an 8-bit peripheral (DSACK0#).
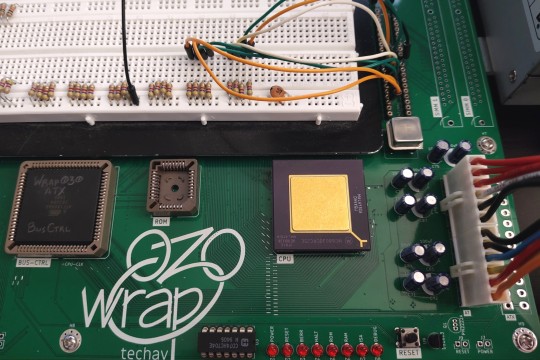
Once I had the minimal functionality for ROM access cycles, I was able to repeat the free run test, but this time with only the to 8 bits of the data bus (D[31:24]) pulled low.
Once I confirmed the ROM access cycle logic was working, I added the bus controller register access cycle logic. The bus controller has a single settings register that will control the Debug LED, startup ROM overlay, and ATX soft power. The CPU will need to be able to write to this register, and reading from it is helpful as well.
The bus controller logic is fully synchronous and managed by a state machine, so all that was needed to add the settings register was a couple new states for the state machine — one for read and one for write.
Put all that together, and we have a computer that can run the most basic of programs, with just a single LED for output.
The next thing I need to get working is a serial port. Everything that comes after this point will be a lot easier if I can output helpful debugging messages over serial.
#homebrew computing#motorola#mc68030#motorola 68k#verilog#cpld#motorola 68030#assembly programming#wrap030 atx#retro computing
26 notes
·
View notes
Note
Hi! I've been following Snacko for a long while! Your gamedev journey is inspiring
I've been thinking over very carefully how I should plan the jump to fulltime dev myself. When did you decide you were ready to take the leap of faith, and how much of the game was done at that point? How have you been supporting yourself regarding funds during development since then?
first, thank you so much! i'm glad my girldisaster journey was at least helpful for somebody out there!
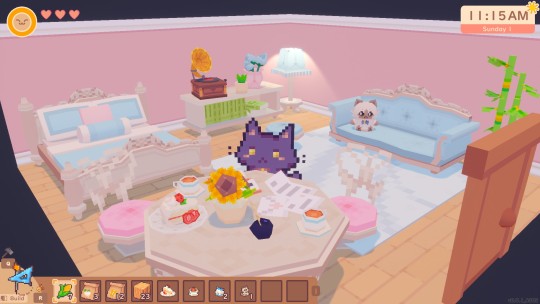
this is gonna be a bit long so i'll just put a break here;;
when did we go fulltime?
about a year after working on the project purely for fun
we were going to release it as a tiny game for any amount of money just to say we released something
well that didn't happen, obviously lol
when we realized people were legitimately interested in it and got publisher meetings, we decided to scale it up
but then found out it's way too difficult (mentally) to work on a game while working full-time
we had some money saved up, and i just finished college. what better time to absolutely ruin our lives give fulltime dev a try?
our plan was: if our savings dip below about 70% of the total saved, we quit
luckily, we never had to worry about hitting that point because we finalized with a publisher soon after (but we no longer have any savings now so ? lol)
if you don't think you'll need outside help it will be easier on your wallet, but remember that means more time on your end taken away to do things you will be less comfortable with
how much of the game was done at that point?
not a lot. it had basic features: farming, walking, talking to people, placing objects, fishing, etc.
this is what the game looked like around that time (2019 summer/fall)
the problem? they were all not that great or they didn't scale well. since then, the game has evolved and changed a lot that it's almost unrecognizable from a systems standpoint
best examples is schedule system to handle NPC actions, dialogue trees, and cutscenes
i would say only maybe 10% code/asset-wise, but 90% of the concepts and plans were already in place
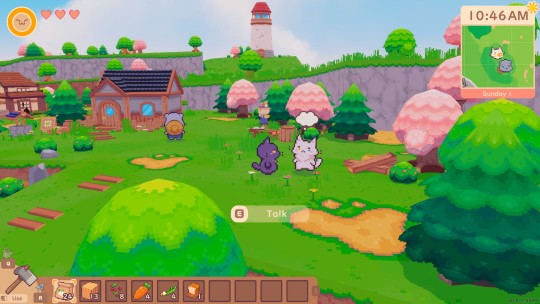
this is what the game looks like now!
how have we been supporting ourselves?
barely hahahaha.
we got a set amount from our publisher each month. this can vary depending on your contract or if you decide to forgo a publisher
most of it goes towards paying for contract work (vfx, sfx, music, additional programming, etc.)
another portion gets sucked into admin (yearly accountant, lawyer, business operating expenses like licenses)
the rest goes into our living expenses. mortgage, bills, etc.
patreon was anywhere from $300-$600 at our peak, but some of that went back into merch for the higher tiers, so we can just say $400 avg
we got a $10k USD grant from epic games (megagrant, free to apply) in october 2019
our kickstarter was around $40k USD. most of it went to paying composer! (it's worth it trust me)
now that our publisher funding has ended (it's been...5 years wow), we have some personal debt as i made the choice to keep some business cash on hand just in case
paying our contractors > paying ourselves
8 notes
·
View notes
Text
Project RBH Devlog 0016
Everyone.
After two whole weeks of banging my head against this problem, I’ve done it.
I’ve finally made it so that status effects can also change an enemy’s stats.
Obviously, what you’re seeing in the GIF isn’t what will actually happen when you light an enemy on fire in any public builds, but I needed something that was immediately visually clear what was happening for demonstration purposes. Thus, the super-fast quick-shotting shotgunner zipping around. This is also my test level where I run all of my dark and diabolical experiments, so the walls are invisible because I never saw a point to making them visible.
(The walls in this game are actually separate from the wall tileset, which is a very common means of handling collision in 2D games. They’re the same walls as the ones in the dungeons, but without the code automatically filling in the wall tiles where the wall collision objects are.)
With this I can really make status effects change up some things about how enemies function while under the influence of whatever you shot them full of. Remember kids, don’t do fire.
This is, unfortunately, the only thing I was able to accomplish last week, but hey, now that it’s working I won’t ever need to touch it again! Mostly!
Right now it can only affect four stats, so if I really want variation, I’ll need to add more to that, and it’s also not exactly plug-and-play; I need to reference the changed variables in the enemy code. For example, right now, if I had a different enemy than the shotgun enemy, they wouldn’t have any of the stat changes when I set them on fire because I was only experimenting with the shotgun enemy. Adding in that code is super easy, especially since I can just copy what I already did for the shotgun enemy, but it is something that I will have to remember in every single enemy I make for the rest of the game.
The four stats I can currently change are, Fire Rate, Damage, Defense, and Movement Speed. You already saw what happens when I dramatically increase the Fire Rate and Movement Speed back at the top of this article.
Defense is quite useful since I can theoretically give enemies armor that way, though I’ll also need to change a few things about how I initialize my variables to make that happen as right now I set them all to a default value without being able to change them when I create an enemy.
There’s also a minor concern with Damage. Unlike the enemies, the Player Character doesn’t have a float value for health, but an integer. In laymen’s terms, the Player HP can only ever be a whole number. If you pay attention to the enemy health number I have for debugging up in that GIF, you’ll see that by contrast, it can take 0.5 damage. That’s not really a problem. What is a problem is that most enemies deal exactly 1 damage, so any reduction of that damage means they deal no damage. Something else I’ll have to figure something out for.
But honestly that can all come later. This week, my plan is to finally fix the problem with what I call the ‘grenade’ mechanic because I still haven’t found a better name for it; the mechanic where you can get a power-up that spawns something when your bullet fades.
Most of that should be simple; turn it from an object reference into an array, plug all the things I want to spawn into the array, run a for loop for the length of the array (meaning once per item in the list) and spawn it. It’s basically the same code I use to make status effects work.
In other game design news, Matt Colville has finally started the Designing The Game youtube series he’s been teasing for a while now. If you aren’t familiar, Matt Colville is a game designer who’s worked on a lot of titles both physical and digital and has a long-running series called Running The Game where he gives advice for being the Dungeon Master in TTRPGs. Most of this advice is system agnostic, but largely focused on Dungeons and Dragons. His company, MCDM, also announced back at the start of the year when Wizards of the Coast announced the new bullshit OGL for Dungeons and Dragons that they were working on their own flagship TTRPG which wouldn’t have any of the legal restriction nonsense that WotC was pushing. Designing The Game is his series where he discusses the work the company has done on this game. The first episode is surprisingly relevant to this specific DevLog series, as it’s a discussion of the benefits of preproduction documentation versus rapid iterative prototyping.
That’s all I’ve got for this week, hopefully next week I’ll be reporting back that I’ve fixed the grenade system, allowing for easy synergies down the line.
Until next Devlog!
-DeusVerve
Special thanks to my Tier 3 Patrons, Haelerin and Christos Kempf!
Support me on Patreon
14 notes
·
View notes
Text
More Inform Basics (#2)
Last time, I talked about getting a project ready. We're still doing that, but I need to take a minute to talk about output. There aren't a lot of options for configuring the appearance of text output in default Inform.
If this seems surprising, remember that the original prototypes for parser games, Adventure and Zork, were written to be experienced via mainframe terminals. Later iterations upon this model used virtual machines that prioritized portability over system-specific features. There were tons of micros in the 80s, and each did things their own way. This method made it possible to port games to many different systems.
The bottom line is that most features related to appearance (font, colors, etc.) are not in the "story" at all*; they're handled by what is called the "interpreter." The story is the actual game file. An interpreter executes the game. The story is platform-agnostic; the interpreter is system-specific. In an interpreter application, a player can change some formatting settings themselves. In a browser-based interpreter, the author can mess with CSS if they are in a position to publish the game somewhere, since web browsers don't have settings specific to parser games.
[*Technically, there are ways to set colors in an Inform game, but the method doesn't work with all interpreters. Unless you publish a web-playable version, you can't control which interpreters players use.]
Whew, TL;DR: vanilla Inform's DNA is descended from a mainframe terminal model. Why is this important? Well, there are still some things under your control as an author, and it's best to do this in a simple, readable way.
Almost everything the player sees is coded with a "say" construction. If we want to print some text just as the game begins (before the player's first turn), we could just:
when play begins: say "Thank you for playing my game!".
[note: I couldn’t find a way to insert a tab, so I used five spaces instead. tabs are important in Inform 7!]
Let's say we wanted to say something after the player examines a lamp:
after examining the lamp: say "I think we did the right thing, choosing to examine that lamp."
Trying to tie everything together: we have a condition that gets set at compile time (test vs. production). We talked about this last time:
PROD is a truth state that varies.
Next time, we'll try using the PROD condition while tweaking output. It's pretty straightforward!
12 notes
·
View notes
Note
hey there! a couple years ago, you made a custom version of simler90's and Midge's turn on replacements mod. i've been using Midge's mod for a while, but some of the turn ons dont fit nicely in my gameplay, and there are others from yours and simler90's mod that would fit better. basically, what i want to ask, is how did you do it? and how hard was it?
i have no experience with coding or mod-making, so im scared of screwing things up, but turn ons are quite the important feature in my gameplay and not having a replacement mod that fits my needs has been frustrating. i dont want to make any new turn ons, i just want a different mash-up of the turn ons already made, preferably using Midge's version as a base. from what i've seen making a mod like this can be quite complicated, but i would still like to try, i just dont even know where to start
so, i know this might be too much to ask, but if you remember how you went about making your version of the mod, would you mind making a detailed tutorial on it? if im only using turn ons already made, could i get away with only copy and pasting the code? even then, how would one go about doing that?
Hey!
Well, this is just a personal opinion, but I don't think making turn on/off replacements is very hard (especially if you're not making any new ones). It's a big chunk of code in one BHAV which might be scary, but after getting past the initial shock the actual code related to most TOs is just a few lines per each.
In addition to the BHAV some TOs are handled in the LUA script, but since Lazy Duchess re-wrote that in a sensible way, it's not very hard to understand either.
It might be a complex project to take if you don't have experience in modding, though. But if you have motivation to learn how to read the code, it's not impossible to do.
To your other question, I'm sorry but even if I'd do a tutorial like that (I won't) it would only help you so much, as there isn't one repeatable pattern for how to replace each TO. What you need to do depends on the TO you're replacing and what you're replacing it with.
However, I once offered advice to anon who wanted to do the same thing as you. You'll find answers to some of your questions and might even get started by checking these: 1, 2, 3. (There's also an explanation about replacing the icons here, but that's the last problem for you to solve.)
If after reading all of that you feel like it's something you're willing to learn, feel free to ask any additional questions. The more specific they are, the better I can answer. I hope this helps at all!
10 notes
·
View notes
Text
histdir
So I've started a stupid-simple shell/REPL history mechanism that's more friendly to Syncthing-style cloud sync than a history file (like basically every shell and REPL do now) or a SQLite database (which is probably appropriate, and it's what Atuin does while almost single-handedly dragging CLI history UX into the 21st century):
You have a history directory.
Every history entry gets its own file.
The file name of a history entry is a hash of that history entry.
The contents of a history entry file is the history entry itself.
So that's the simple core concept around which I'm building the rest. If you just want a searchable, syncable record of everything you ever executed, well there you go. This was the smallest MVP, and I implemented that last night - a little shell script to actually create the histdir entries (entry either passed as an argument or read on stdin if there's no entry argument), and some Elisp code in my Emacs to replace Eshell's built-in history file save and load. Naturally my loaded history stopped remembering order of commands reliably, as expected, which would've been a deal-breaker problem in the long term. But the fact that it instantly plugged into Syncthing with no issues was downright blissful.
(I hate to throw shade on Atuin... Atuin is the best project in the space, I recommend checking it out, and it significantly inspired the featureset and UX of my current setup. But it's important for understanding the design choices of histdir: Atuin has multiple issues related to syncing - histdir will never have any sync issues. And that's part of what made it so blissful. I added the folder to Syncthing - no separate account, no separate keys, nothing I must never lose. In most ways, Atuin's design choice of a SQLite database is just better. That's real, proper engineering. Serious software developers all know that this is exactly the kind of thing where a database is better than a bunch of files. But one benefit you get from this file-oriented granularity is that if you just design the naming scheme right, history entries never collide/conflict in the same file. So we get robust sync, even with concurrent use, on multiple devices - basically for free, or at least amortized with the setup effort for whatever solution you're using to sync your other files (none of which could handle updates from two different devices to a single SQLite database). Deleting a history entry in histdir is an "rm"/"unlink" - in Atuin it's a whole clever engineering puzzle.)
So onto preserving order. In principle, the modification time of these files is enough for ordering: the OS already records when they were last written to, so if you sort on that, you preserve history order. I was initially going to go with this, but: it's moderately inconvenient in some programming languages, it can only handle a 1-to-1 mapping (one last-modified timestamp) even though many uses of history might prefer an n-to-1 (an entry for every time the command was called), and it requires worrying about questions like "does {sync,copy,restore-from-backup,this-programmatic-manipulation-I-quickly-scripted} preserve the timestamp correctly?"
So tonight I did what any self-respecting drank-too-much-UNIX-philosophy-coolaid developer would do: more files. In particular:
Each call of a history entry gets its own file.
The file name of a call is a timestamp.
The contents of a call file is the hash of the history entry file.
The hash is mainly serving the purpose of being a deterministic, realistically-will-never-collide-with-another-history-entry (literally other causes of collision like hackers getting into your box and overwriting your memory are certain and inevitable by comparison) identifier - in a proper database, this would just be the primary key of a table, or some internal pointer.
The timestamp files allow a simple lexical sort, which is a default provided by most languages, most libraries, and built in by default in almost everything that lists/iterates a directory. That's what I do in my latest Elisp code in my Emacs: directory-files does a lexical sort by default - it's not pretty from an algorithmic efficiency standpoint, but it makes the simplest implementation super simple. Of course, you could get reasonably more efficient if you really wanted to.
I went with the hash as contents, rather than using hardlinks or symlinks, because of programmatic introspection simplicity and portability. I'm not entirely sure if the programmatic introspection benefits are actually worth anything in practice. The biggest portability case against symlinks/hardlinks/etc is Windows (technically can do symlinks, but it's a privileged operation unless you go fiddle with OS settings), Android (can't do hardlinks at all, and symlinks can't exist in shared storage), and if you ever want to have your histdir on something like a USB stick or whatever.
Depending on the size of the hash, given that the typical lengths of history entries might be rather short, it might be better for deduplication and storage to just drop the hash files entirely, and leave only the timestamp files. But it's not necessarily so clear-cut.
Sure, the average shell command is probably shorter by a wide margin than a good hash. The stuff I type into something like a Node or Python REPL will trend a little longer than the shell commands. But now what about, say, URLs? That's also history, it's not even that different conceptually from shell/REPL history, and I haven't yet ruled out it making sense for me to reuse histdir for that.
And moreover, conceptually they achieve different goals. The entry files are things that have been in your history (and that you've decided to keep). They're more of a toolbox or repertoire - when you do a fuzzy search on history to re-run a command, duplicates just get in the way. Meanwhile, call files are a "here's what I did", more of a log than a toolbox.
And obviously this whole histdir thing is very expandable - you could have other files containing metadata. Some metadata might be the kind of thing we'd want to associate with a command run (exit status, error output, relevant state like working directory or environment variables, and so on), but other stuff might make more sense for commands themselves (for example: this command is only useful/valid on [list of hosts], so don't use it in auto-complete and fuzzy search anywhere else).
So... I think it makes sense to have history entries and calls to those entries "normalized" into their own separate files like that. But it might be overkill in practice, and the value might not materialize in practice, so that's more in the TBD I guess.
So that's where I'm at now. A very expandable template, but for now I've just replicated basic shell/REPL history, in an a very high-overhead way. A big win is great history sync almost for free, without a lot of the technical downsides or complexity (and with a little effort to set up inotify/etc watches on a histdir, I can have newly sync'ed entries go directly into my running shells/REPLs... I mean, within Emacs at least, where that kind of across-the-board malleability is accessible with a reasonably low amount of effort). Another big win is that in principle, it should be really easy to build on existing stuff in almost any language to do anything I might want to do. And the biggest win is that I can now compose those other wins with every REPL I use, so long as I can either wrap that REPL a little bit (that's how I'll start, with Emacs' comint mode), or patch the common libraries like readline to do histdir, or just write some code to translate between a traditional history file and my histdir approach.
At every step of the way, I've optimized first and foremost for easiest-to-implement and most-accessible-to-work-with decision. So far I don't regret it, and I think it'll help a lot with iteratively trying different things, and with all sorts of integration and composition that I haven't even thought of yet. But I'll undoubtedly start seeing problems as my histdirs grow - it's just a question of how soon and how bad, and if it'll be tractable to fix without totally abandoning the approach. But it's also possible that we're just at the point where personal computers and phones are powerful enough, and OS and FS optimizations are advanced enough, that the overhead will never be perceptible to me for as long as I live - after all, its history for an interface with a live human.
So... happy so far. It seems promising. Tentatively speaking, I have a better daily-driver shell history UX than I've ever had, because I now have great reliable and fast history sync across my devices, without regressions to my shell history UX (and that's saying something, since I was already very happy with zsh's vi mode, and then I was even more happy with Eshell+Eat+Consult+Evil), but I've only just implemented it and given it basic testing. And I remain very optimistic that I could trivially layer this onto basically any other REPL with minimal effort thanks to Emacs' comint mode.
3 notes
·
View notes