#UI/UX design and development company
Explore tagged Tumblr posts
Text
Top 5 Benefits of UI/UX Design and Development Services
Your business app or website’s user interface and user experience (UI/UX) are critical to its success. Understanding the significance of UI and UX for your website or app is the first step towards developing appealing, functional, and engaging business solutions. When designing parts, you must adhere to the best practices. And if you want unrivalled design services, you should work with a reputable software solutions company like InstaIT
#UI UX Designer and Developer#UI/UX design and development company#mobile app ui ux designers#UI UX Development Services#ui ux design and development services#UI and UX Design Services#mobile app design service#ux ui design company#ui/ux design and development services#ui ux design and development company
0 notes
Text
issuu
UI/UX design and development services are necessary for any company that wants to grow online. Businesses can improve conversion rates by using UI UX Design and Development Services to enhance the user experience. Businesses may boost the possibility of conversions by making it simpler for users to accomplish tasks and achieve their goals.
#UI/UX Design and Development Company#UI UX Development Services#ui ux design and development services#UI and UX Design Services#UI UX Designer and Developer
0 notes
Text
JavaScript Fundamentals
I have recently completed a course that extensively covered the foundational principles of JavaScript, and I'm here to provide you with a concise overview. This post will enable you to grasp the fundamental concepts without the need to enroll in the course.
Prerequisites: Fundamental HTML Comprehension
Before delving into JavaScript, it is imperative to possess a basic understanding of HTML. Knowledge of CSS, while beneficial, is not mandatory, as it primarily pertains to the visual aspects of web pages.
Manipulating HTML Text with JavaScript
When it comes to modifying text using JavaScript, the innerHTML function is the go-to tool. Let's break down the process step by step:
Initiate the process by selecting the HTML element whose text you intend to modify. This selection can be accomplished by employing various DOM (Document Object Model) element selection methods offered by JavaScript ( I'll talk about them in a second )
Optionally, you can store the selected element in a variable (we'll get into variables shortly).
Employ the innerHTML function to substitute the existing text with your desired content.
Element Selection: IDs or Classes
You have the opportunity to enhance your element selection by assigning either an ID or a class:
Assigning an ID:
To uniquely identify an element, the .getElementById() function is your go-to choice. Here's an example in HTML and JavaScript:
HTML:
<button id="btnSearch">Search</button>
JavaScript:
document.getElementById("btnSearch").innerHTML = "Not working";
This code snippet will alter the text within the button from "Search" to "Not working."
Assigning a Class:
For broader selections of elements, you can assign a class and use the .querySelector() function. Keep in mind that this method can select multiple elements, in contrast to .getElementById(), which typically focuses on a single element and is more commonly used.
Variables
Let's keep it simple: What's a variable? Well, think of it as a container where you can put different things—these things could be numbers, words, characters, or even true/false values. These various types of stuff that you can store in a variable are called DATA TYPES.
Now, some programming languages are pretty strict about mentioning these data types. Take C and C++, for instance; they're what we call "Typed" languages, and they really care about knowing the data type.
But here's where JavaScript stands out: When you create a variable in JavaScript, you don't have to specify its data type or anything like that. JavaScript is pretty laid-back when it comes to data types.
So, how do you make a variable in JavaScript?
There are three main keywords you need to know: var, let, and const.
But if you're just starting out, here's what you need to know :
const: Use this when you want your variable to stay the same, not change. It's like a constant, as the name suggests.
var and let: These are the ones you use when you're planning to change the value stored in the variable as your program runs.
Note that var is rarely used nowadays
Check this out:
let Variable1 = 3; var Variable2 = "This is a string"; const Variable3 = true;
Notice how we can store all sorts of stuff without worrying about declaring their types in JavaScript. It's one of the reasons JavaScript is a popular choice for beginners.
Arrays
Arrays are a basically just a group of variables stored in one container ( A container is what ? a variable , So an array is also just a variable ) , now again since JavaScript is easy with datatypes it is not considered an error to store variables of different datatypeslet
for example :
myArray = [1 , 2, 4 , "Name"];
Objects in JavaScript
Objects play a significant role, especially in the world of OOP : object-oriented programming (which we'll talk about in another post). For now, let's focus on understanding what objects are and how they mirror real-world objects.
In our everyday world, objects possess characteristics or properties. Take a car, for instance; it boasts attributes like its color, speed rate, and make.
So, how do we represent a car in JavaScript? A regular variable won't quite cut it, and neither will an array. The answer lies in using an object.
const Car = { color: "red", speedRate: "200km", make: "Range Rover" };
In this example, we've encapsulated the car's properties within an object called Car. This structure is not only intuitive but also aligns with how real-world objects are conceptualized and represented in JavaScript.
Variable Scope
There are three variable scopes : global scope, local scope, and function scope. Let's break it down in plain terms.
Global Scope: Think of global scope as the wild west of variables. When you declare a variable here, it's like planting a flag that says, "I'm available everywhere in the code!" No need for any special enclosures or curly braces.
Local Scope: Picture local scope as a cozy room with its own rules. When you create a variable inside a pair of curly braces, like this:
//Not here { const Variable1 = true; //Variable1 can only be used here } //Neither here
Variable1 becomes a room-bound secret. You can't use it anywhere else in the code
Function Scope: When you declare a variable inside a function (don't worry, we'll cover functions soon), it's a member of an exclusive group. This means you can only name-drop it within that function. .
So, variable scope is all about where you place your variables and where they're allowed to be used.
Adding in user input
To capture user input in JavaScript, you can use various methods and techniques depending on the context, such as web forms, text fields, or command-line interfaces.We’ll only talk for now about HTML forms
HTML Forms:
You can create HTML forms using the <;form> element and capture user input using various input elements like text fields, radio buttons, checkboxes, and more.
JavaScript can then be used to access and process the user's input.
Functions in JavaScript
Think of a function as a helpful individual with a specific task. Whenever you need that task performed in your code, you simply call upon this capable "person" to get the job done.
Declaring a Function: Declaring a function is straightforward. You define it like this:
function functionName() { // The code that defines what the function does goes here }
Then, when you need the function to carry out its task, you call it by name:
functionName();
Using Functions in HTML: Functions are often used in HTML to handle events. But what exactly is an event? It's when a user interacts with something on a web page, like clicking a button, following a link, or interacting with an image.
Event Handling: JavaScript helps us determine what should happen when a user interacts with elements on a webpage. Here's how you might use it:
HTML:
<button onclick="FunctionName()" id="btnEvent">Click me</button>
JavaScript:
function FunctionName() { var toHandle = document.getElementById("btnEvent"); // Once I've identified my button, I can specify how to handle the click event here }
In this example, when the user clicks the "Click me" button, the JavaScript function FunctionName() is called, and you can specify how to handle that event within the function.
Arrow functions : is a type of functions that was introduced in ES6, you can read more about it in the link below
If Statements
These simple constructs come into play in your code, no matter how advanced your projects become.
If Statements Demystified: Let's break it down. "If" is precisely what it sounds like: if something holds true, then do something. You define a condition within parentheses, and if that condition evaluates to true, the code enclosed in curly braces executes.
If statements are your go-to tool for handling various scenarios, including error management, addressing specific cases, and more.
Writing an If Statement:
if (Variable === "help") { console.log("Send help"); // The console.log() function outputs information to the console }
In this example, if the condition inside the parentheses (in this case, checking if the Variable is equal to "help") is true, the code within the curly braces gets executed.
Else and Else If Statements
Else: When the "if" condition is not met, the "else" part kicks in. It serves as a safety net, ensuring your program doesn't break and allowing you to specify what should happen in such cases.
Else If: Now, what if you need to check for a particular condition within a series of possibilities? That's where "else if" steps in. It allows you to examine and handle specific cases that require unique treatment.
Styling Elements with JavaScript
This is the beginner-friendly approach to changing the style of elements in JavaScript. It involves selecting an element using its ID or class, then making use of the .style.property method to set the desired styling property.
Example:
Let's say you have an HTML button with the ID "myButton," and you want to change its background color to red using JavaScript. Here's how you can do it:
HTML: <button id="myButton">Click me</button>
JavaScript:
// Select the button element by its ID const buttonElement = document.getElementById("myButton"); // Change the background color property buttonElement.style.backgroundColor = "red";
In this example, we first select the button element by its ID using document.getElementById("myButton"). Then, we use .style.backgroundColor to set the background color property of the button to "red." This straightforward approach allows you to dynamically change the style of HTML elements using JavaScript.
#studyblr#code#codeblr#css#html#javascript#java development company#python#study#progblr#programming#studying#comp sci#web design#web developers#web development#website design#ui ux design#reactjs#webdev#website#tech
386 notes
·
View notes
Text
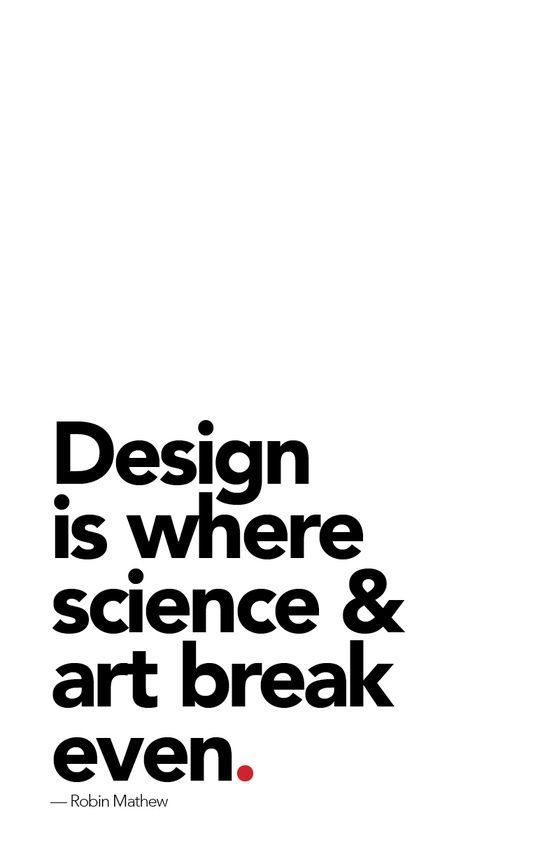
Design is the point where two seemingly opposite worlds meet
the structured precision of science and the boundless creativity of art.
Think about it
great design isn’t just about making things look good;
it’s about function as much as it’s about form.
It’s the science of understanding how people think, behave, and interact with the world. It's psychology, mathematics, and ergonomics shaping every line, color, and space.
At the same time, design is also an art.
It’s the creativity that brings a unique voice and personality to a project, transforming the ordinary into something that resonates on an emotional level.
When we create, we're not just drawing or choosing colors.
We're solving problems, anticipating needs, and crafting experiences.
Design is at its best when it serves a purpose when it’s more than
decoration and becomes a tool for communication, connection, and transformation.
So, here’s to the beautiful synergy of science and art in design.
It’s what makes our work meaningful, impactful, and endlessly inspiring. 🌌✨
Need a designer to level up your business
Inbox me now
in Fiverr :https://www.fiverr.com/s/GzXzwLe
#100 days of productivity#batman#19th century#35mm#911 abc#academia#aespa#ace attorney#adidas#adventure#100 likes#mob psycho 100#fairy tail 100 years quest#the 100#100 posts#gucci#mine#design#ui ux design#ui ux company#ui ux development services#ui ux agency#ui ux development company#creative logo#logodesigner#logo design#logotype#logo#graphicdesigner#billford
6 notes
·
View notes
Text
Curbcut Effect
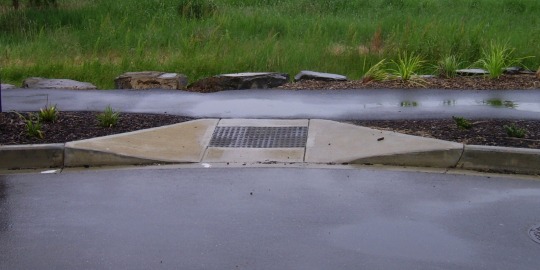
(src. wikipedia/ Michael3 https://en.wikipedia.org/wiki/Curb_cut#/media/File:Pram_Ramp.jpg )
I learned about curbcuts. Curbcuts are those litle ramps, made especially as accessibility features to help people bound to wheelchairs to get on stairs.
But what's even just as interesting: Curbcut Effect is the name of the phenomenon, when suddenly not just wheelchairs profit from this little feature, but every parent with a stroller or people having to transport heavy stuff.
So this applies to everything where you consider accessibiity for handicapped citizens that then would also be of use for everyone else.
#ux#usability#design#user design#ux research#ui ux design#ui ux company#ui ux development services#curbcut
6 notes
·
View notes
Text
New project for Sunny Highlight!
Excited to present a fresh and light design for the Sunny Highlight brand!
Low-calorie drinks, natural ingredients, and vibrant flavors — all reflected in every element of the project. Stay tuned and discover the joy of natural refreshment!
Behance: wonixdel
#ui ux company#ui ux design#ui ux development services#ui/ux development company#web design#uidesign#drinks#shop#ui ux agency
3 notes
·
View notes
Text
Crypto trading mobile app
Designing a Crypto Trading Mobile App involves a balance of usability, security, and aesthetic appeal, tailored to meet the needs of a fast-paced, data-driven audience. Below is an overview of key components and considerations to craft a seamless and user-centric experience for crypto traders.
Key Elements of a Crypto Trading Mobile App Design
1. Intuitive Onboarding
First Impressions: The onboarding process should be simple, guiding users smoothly from downloading the app to making their first trade.
Account Creation: Offer multiple sign-up options (email, phone number, Google/Apple login) and include KYC (Know Your Customer) verification seamlessly.
Interactive Tutorials: For new traders, provide interactive walkthroughs to explain key features like trading pairs, order placement, and wallet setup.
2. Dashboard & Home Screen
Clean Layout: Display an overview of the user's portfolio, including current balances, market trends, and quick access to popular trading pairs.
Market Overview: Real-time market data should be clearly visible. Include options for users to view coin performance, historical charts, and news snippets.
Customization: Let users customize their dashboard by adding favorite assets or widgets like price alerts, trading volumes, and news feeds.
3. Trading Interface
Simple vs. Advanced Modes: Provide two versions of the trading interface. A simple mode for beginners with basic buy/sell options, and an advanced mode with tools like limit orders, stop losses, and technical indicators.
Charting Tools: Integrate interactive, real-time charts powered by TradingView or similar APIs, allowing users to analyze market movements with tools like candlestick patterns, RSI, and moving averages.
Order Placement: Streamline the process of placing market, limit, and stop orders. Use clear buttons and a concise form layout to minimize errors.
Real-Time Data: Update market prices, balances, and order statuses in real-time. Include a status bar that shows successful or pending trades.
4. Wallet & Portfolio Management
Asset Overview: Provide an easy-to-read portfolio page where users can view all their holdings, including balances, performance (gains/losses), and allocation percentages.
Multi-Currency Support: Display a comprehensive list of supported cryptocurrencies. Enable users to transfer between wallets, send/receive assets, and generate QR codes for transactions.
Transaction History: Offer a detailed transaction history, including dates, amounts, and transaction IDs for transparency and record-keeping.
5. Security Features
Biometric Authentication: Use fingerprint, facial recognition, or PIN codes for secure logins and transaction confirmations.
Two-Factor Authentication (2FA): Strong security protocols like 2FA with Google Authenticator or SMS verification should be mandatory for withdrawals and sensitive actions.
Push Notifications for Security Alerts: Keep users informed about logins from new devices, suspicious activities, or price movements via push notifications.
6. User-Friendly Navigation
Bottom Navigation Bar: Include key sections like Home, Markets, Wallet, Trade, and Settings. The icons should be simple, recognizable, and easily accessible with one hand.
Search Bar: A prominent search feature to quickly locate specific coins, trading pairs, or help topics.
7. Analytics & Insights
Market Trends: Display comprehensive analytics including top gainers, losers, and market sentiment indicators.
Push Alerts for Price Movements: Offer customizable price alert notifications to help users react quickly to market changes.
Educational Content: Include sections with tips on technical analysis, crypto market basics, or new coin listings.
8. Social and Community Features
Live Chat: Provide a feature for users to chat with customer support or engage with other traders in a community setting.
News Feed: Integrate crypto news from trusted sources to keep users updated with the latest market-moving events.
9. Light and Dark Mode
Themes: Offer both light and dark mode to cater to users who trade at different times of day. The dark mode is especially important for night traders to reduce eye strain.
10. Settings and Customization
Personalization Options: Allow users to choose preferred currencies, set trading limits, and configure alerts based on their personal preferences.
Language and Regional Settings: Provide multilingual support and regional settings for global users.
Visual Design Considerations
Modern, Minimalist Design: A clean, minimal UI is essential for avoiding clutter, especially when dealing with complex data like market trends and charts.
Color Scheme: Use a professional color palette with accents for call-to-action buttons. Green and red are typically used for indicating gains and losses, respectively.
Animations & Micro-interactions: Subtle animations can enhance the experience by providing feedback on button presses or transitions between screens. However, keep these minimal to avoid slowing down performance.
Conclusion
Designing a crypto trading mobile app requires focusing on accessibility, performance, and security. By blending these elements with a modern, intuitive interface and robust features, your app can empower users to navigate the fast-paced world of crypto trading with confidence and ease.
#uxbridge#uxuidesign#ui ux development services#ux design services#ux research#ux tools#ui ux agency#ux#uxinspiration#ui ux development company#crypto#blockchain#defi#ethereum#altcoin#fintech
2 notes
·
View notes
Text
#web design#website#web design india#website design#website design india#web designers#ui ux design#ux#design#uidesign#ui ux development services#ui ux company#ecommerce#ecommerce website development#ecommerce website design#ecommerce website developer in india#ecommerce web development company#ecommerce website builder
3 notes
·
View notes
Text
The Step-by-Step Design Process
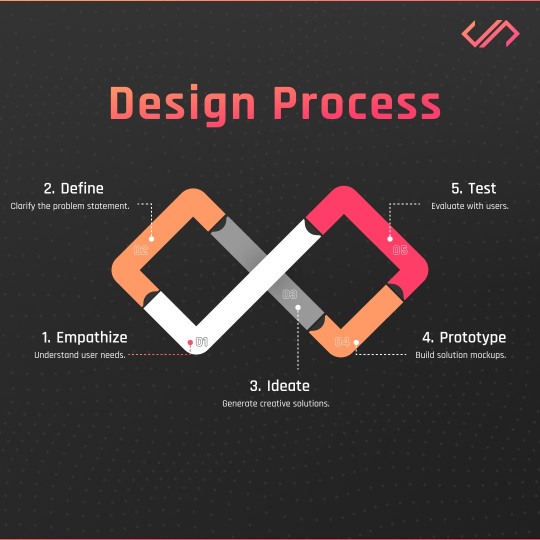
Explore how a design process bridges creativity and functionality. Each step is crafted to ensure that projects not only look great but also perform flawlessly, achieving desired outcomes.
Get in Touch: 📧 [email protected]
👥Follow Us On:
Facebook: www.facebook.com/jhavtech
Instagram: www.instagram.com/jhavtechstudios
LinkedIn: www.linkedin.com/company/jhavtech-studios
Twitter: https://bit.ly/3WoJFWM
#mobileapps#technology#app developers#webdesign#mobile apps#app development#artificial intelligence#software development#jhavtechstudios#graphic design#design#mobile app design#ui ux design#web design company#creative#steps
3 notes
·
View notes
Text
Website, Application Development Services to accelerate your business growth.
SpryBit is an IT consultation and solution provider which is home to some of the best skilled technical professionals in the industry.
#Web Design Services#Web Design & Development Company#Web App Development#Mobile App Devleopment Company#Mobile App Design#Magento Ecommerce Development Company#UI UX Design Company#Microsoft Technologies Development Compnay
2 notes
·
View notes
Text
Businesses can improve conversion rates by using UI UX Design and Development Services to enhance the user experience. Businesses may boost the possibility of conversions by making it simpler for users to accomplish tasks and achieve their goals.
#UI/UX Design and Development Company#UI UX Development Services#ui ux design and development services#UI and UX Design Services#UI UX Designer and Developer
0 notes
Text
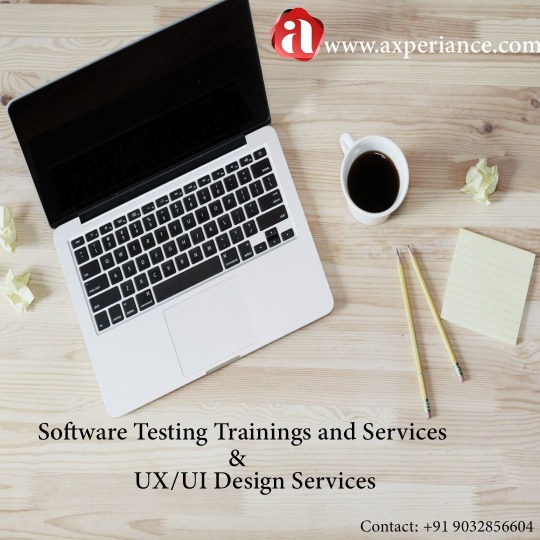
" Level up your skills with our Software Testing Training and Services, paired with stunning UI UX Designs! "
https://axperiance.com/
#software testing#software#ui ux design#ui ux development company#online class#information technology#services#softwarecompany#softwarecourses#ui ux design services#ui ux designs
3 notes
·
View notes
Text
ppl complaining about the new legally blonde prequel coming out didn't seem to get the movie cause elle WAS interesting and smart from the get go????
"i have a 4.0...." "but in fashion merchandising"
girlie's got a business related degree from the beginning, even if it's a "frivolous" thing
i think u rlly missed the main idea?
#personal#omg no i want the FIT MPS in global fashion manageneny#management*** im rlly grumpy cuz like 3 yrs ago it was an MBA but now its an MPS ;^;#which i wanna try to shmooze my way into having my company pay for#(or hop to someone else who WILL pay cuz at work i built basically the same automation system VS has lmao#like my coworker who used to be at VS was like 'u made this....huh this is what VS contracted out')#BUUUUUUUUUUUUT like i was one of the top students in my class for my art school and ran a student org#and when i told ppl i wanted to do either animation or apparel with my graphic design degree ppl were sooo nasty#i mean like PROFESSORS and advisors /#:/#so i feel for the character lmao?????? cuz i know for a fact i earn more than my archi and a couple of my ui/ux friends who#like...arent at faangs so.........and im happier than them :)#like ive been on a product development design team as one of two artists for 2.5 years now#and like i have to be in the FASHION MERCHANDISING meetings#and....theyre business meetings about sourcing and costing and meeting minimums to make profits#its not cutesy clothing its....spreadsheets#and my art automation system is also based off of a spreadsheet :)
2 notes
·
View notes
Text
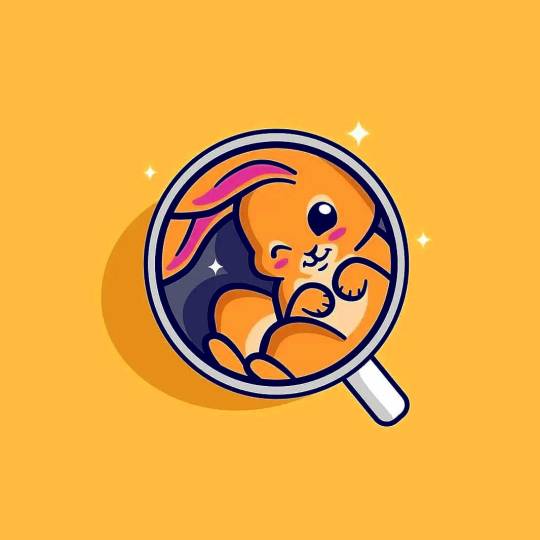
"Bunny in a coffee cup" logo design ♡♡♡
◇ Why it is important to have a professional logo for your business?
◇ Are you sure that clients understand your visuals?
◇ Are you sending the right message to them?
Get your FREE design consultation at:
#bunny#cutepets#cute animals#rabbit#coffee cup#coffetime#logo#business#illustration#entrepreneur#artists on tumblr#creative#startup#identity#visuals#ui ux development company#ui ux design#consulting services#califorina#newyork#los angeles
21 notes
·
View notes
Text
Website Design for Savory: Aesthetics and Functionality of Kitchen Accessories
https://www.behance.net/wonixdel
3 notes
·
View notes
Text
Mohali's emergence as a hub for software companies is a testament to the city's dynamic business environment and the continuous efforts of these companies to drive technological innovation. The top 10 software companies in Mohali have not only contributed to the local economy but have also made a mark on the global stage, showcasing the city as a thriving IT destination. As Mohali continues to grow and evolve, these companies will likely play a crucial role in shaping the future of the technology industry in the region.
#graphic design#marketing#branding#web development#ecommerce#technology#web design#mobile app development#mobile application development#web application development#logo design#software development#android#app development companies#android app developers#ui ux company#mobile app developer company#mobile app company
4 notes
·
View notes