#SOLIDPrinciples
Explore tagged Tumblr posts
Text
https://www.thefullstack.co.in/software-design-patterns/
#SoftwareDesign#DesignPatterns#ProgrammingTutorial#CodeArchitecture#SoftwareEngineering#CodingTips#LearnToCode#DevCommunity#CleanCode#OOP (Object-Oriented Programming)#SOLIDPrinciples
0 notes
Text
0 notes
Text
Factory Method Pattern
Problem: In game development, you often need to create various types of characters with different attributes and behaviors. For example, warriors may have different characteristics and attack behaviors compared to mages. The problem is how to create these diverse character types in a structured and maintainable manner without tightly coupling the code to specific character…
View On WordPress
0 notes
Text
SOLID Principle C#
What is SOLID Principle?
'In object-oriented computer programming, SOLID is a mnemonic acronym for five design principles intended to make software designs more understandable, flexible and maintainable.'
SOLID is an acronym for the following.
S: Single Responsibility Principle (SRP)
O: Open-closed Principle (OCP)
L: Liskov substitution Principle (LSP)
I: Interface Segregation Principle (ISP)
D: Dependency Inversion Principle (DIP)
S: Single Responsibility Principle (SRP)
SRP says, "Every software module should have only one reason to change.".
Any class must have one and only one reason to change. In other words, a class must only have a single responsibility. This principle is simple, but not always easy to follow
Example
class Customer {
public function createCustomer(Request $request)
{ // Create customer
}
}
class Order {
public function submitOrder (Request $request)
{ // Submit Orders
}
}
O: Open/Closed Principle
The Open/closed Principle says, "A software module/class is open for extension and closed for modification."
Objects or entities must be open for extension but closed for modification. AÂ class must be easily extensible without requiring modification of the class itself. The goal of this principle is to help create well-encapsulated, highly cohesive systems.Â
Example
interface ISavingAccount {
public function CalculateInterest();
}
class RegularSavingAccount implements ISavingAccount {
public function CalculateInterest()
{
//Calculate interest for regular saving account
}
}
class SalarySavingAccount implements ISavingAccount {
public function CalculateInterest()
{
//Calculate interest for regular saving account
}
}
L: Liskov Substitution Principle
The Liskov Substitution Principle (LSP) states, "you should be able to use any derived class instead of a parent class and have it behave in the same manner without modification.".Â
Derived types must be completely substitutable for their base types. New derived classes must just extend without replacing the functionality of old classes.
Example
class Bird{
// Methods related to birds
}
class FlyingBirds extends Bird
{
public function Fly()
{
Return “I can Fly”;
}
}
class Ostrich extends Bird
{
// Methods related to birds
}
I: Interface Segregation Principle (ISP)
The Interface Segregation Principle states "that clients should not be forced to implement interfaces they don't use. Instead of one fat interface, many small interfaces are preferred based on groups of methods, each serving one submodule.".
Clients must not be dependent upon interfaces or methods they don't use. Keeping interfaces segregated helps a developer more easily know which one to call for specific functions and helps us avoid fat or polluted interfaces that can affect the performance of a system.Â
Example
interface OnlineClientInterface
{
public function acceptOnlineOrder();
public function payOnline();
}
interface WalkInCustomerInterface
{
public function walkInCustomerOrder();
public function payInPerson();
}
class OnlineClient implements OnlineClientInterface
{
public function acceptOnlineOrder()
{
//logic for placing online order
}
public function payOnline()
{
//logic for paying online
}
}
class WalkInCustomer implements WalkInCustomerInterface
{
public function walkInCustomerOrder()
{
//logic for walk in customer order
}
public function payInPerson()
{
//logic for payment in person
}
}
D: Dependency Inversion Principle
The Dependency Inversion Principle (DIP) states that high-level modules/classes should not depend on low-level modules/classes. First, both should depend upon abstractions. Secondly, abstractions should not rely upon details. Finally, details should depend upon abstractions.
This principle helps maintain a system that’s properly coupled. Avoiding high-level class dependencies on low-level classes helps keep a system flexible and extensible.
Example
interface EBook
{
function read();
}
class PDFBook implements EBook
{
function read()
{
return "reading a pdf book.";
}
}
class EBookReader {
private $book;
function __construct(EBook $book) { $this->book = $book; } function read() { return $this->book->read(); }
}
$book = new PDFBook();
$read = new EBookReader($b);
$read->read();
Conclusion:
Using SOLID principles is critical to being a really good developer and creating valuable software solutions. SOLID is essentially a balanced nutrition plan for software development. When we don’t use these principles in development, our code base becomes bloated and overweight. The system then requires extra work and attention to “trim down” or even maintain, and is at much higher risk for an emergency scare.
0 notes
Video
youtube
Dependency Inversion Principle Tutorial with Java Code Example for Students
 https://youtu.be/_v7JVQsRkN4
Hello friends, new #video on #dependencyinversion #solidprinciples with #Java #coding #example is published on #codeonedigest #youtube channel. Learn #dip #dependency #inversion #principle #programming #coding with codeonedigest.
@java #java #awscloud @awscloud @AWSCloudIndia #Cloud #CloudComputing @YouTube #youtube #dependencyinversionprinciple #dependencyinversion #dependencyinversionvsdependencyinjection #dependencyinversionprinciplejava #dependencyInversionprinciple #dependencyInversionprinciplesolid #dip #dipprinciple #dependencyInversionprinciple #interface #dependencyInversionjava #solidprinciples #solidprinciplesinterviewquestions #solidprinciplesjavainterviewquestions #solidprinciplesreact #solidprinciplesinandroid #solidprinciplestutorial #solidprinciplesexplained #solidprinciplesjava #singleresponsibilityprinciple #openclosedprinciple #liskovsubstitutionprinciple #dependencyInversionprinciple #dependencyinversionprinciple #objectorientedprogramming #objectorienteddesignandmodelling #objectorienteddesign #objectorienteddesignsoftwareengineering #objectorienteddesigninterviewquestions #objectorienteddesignandanalysis #objectorienteddesigninjava #objectorienteddesignmodel #objectorienteddesignapproach #objectorienteddesignparadigm #objectorienteddesignquestions
#youtube#dependency inversion#dependency inversion principle#solid principles#solid#dependency principle
2 notes
·
View notes
Photo

React is very powerful and high in demand for JavaScript technology. What makes it best? Of course, the SOLID principles.
https://gkmit.co/blog/mobile-development/react-native-vs-xamarin-what-to-choose-for-cross-platform-app-development
0 notes
Text
My Youtube Channel
Why am I in there?Â
Well, I spend a lot of time learning Technical stuff for my work. 2 weeks back, thanks to the pandemic, I was kicked out of the project. Finding new work is not easy. One has to prepare for interviews. And it is all over again.Â
Somehow one day I decided to use the opportunity for better. Better for me better for everyone else. There are topics I spend hours just googling and trying to find a good tutorial or documentation. So I decided to do videos on those.Â
And, a mild interest on the monetary benefits one would get if one is lucky enough. Getting monetary benefits from YouTube is like entrepreneurship. We know thousands start and only a handful reach the goal line. But I am trying to be positive as amidst the global pandemic the only thing all of us should have is hope and positivity, at least until such time that the smart brains working on finding the answers find the answers.Â
Now, if, only if you want to know SOLID principles in programming, to write clean code, I have a playlist on it. Others ignore. Or have a look at it and feedback. :)
https://www.youtube.com/playlist?list=PLJrS6LPIW0skMpJbjscflEFO4rUO3A8fy
0 notes
Photo
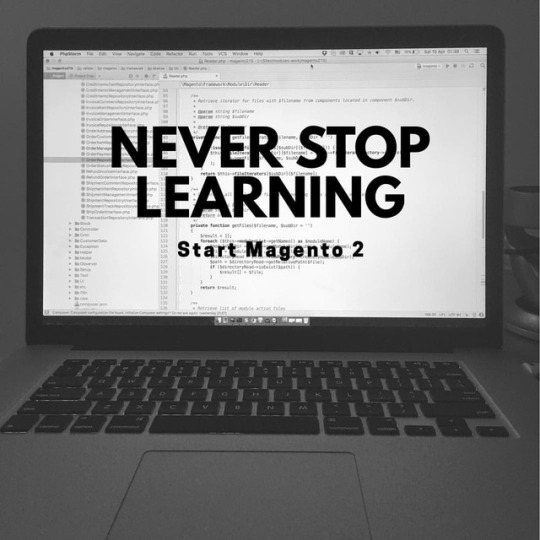
In case you are passionate about software development have a look at Magento 2 platform. There are great examples of SOLID principles, Design Patterns, ORM, MVC, MVVM. #magento #php #javascript #mysql #doctrine #symfony #oro #orocrm #orocommerce #magento2 #b2b #b2c #ecommerce #checkout #platform #developer #devblog #devletter #magentodevchannel #designpatterns #solidprinciples #mvc #mvvm #zendframework #development (at Dublin, Ireland)
#devblog#orocrm#mvvm#checkout#b2b#developer#b2c#magento2#zendframework#mvc#ecommerce#mysql#magentodevchannel#platform#solidprinciples#development#javascript#oro#orocommerce#designpatterns#php#symfony#devletter#doctrine#magento
0 notes
Video
youtube
👉 I haven't created any content on important topics like Design Patterns and Solid Principles. Here is Why? 👉 Disclaimer and Note - My thoughts may not match with the mainstream thoughts on this topic. So even if you agree with me after watching this video, please don't use the same during interviews or CS examinations. This is only to help you while writing the code.Â
👉 So please take care while talking about it #designpatterns #solidprinciples #java #C++ #programming #softwaredevelopment #softwareengineering #softwaredesign
#design patterns#software#software development#coding#code#software design#software engineering#C++#JAVA#Programming
0 notes
Text
SOLID principles are crucial in test automation for creating scalable and flexible tests. We base our examples and best practices on experience.
0 notes
Text
Singlton Pattern
Problem: In a web application, multiple parts of the application may need to access the database concurrently. Creating a new database connection every time one is needed can be resource-intensive and slow. Managing a limited number of database connections and allowing multiple parts of the application to share and reuse them efficiently is essential for improving performance and resource…
View On WordPress
0 notes
Video
youtube
Liskov Substitution Principle Tutorial with Java Coding Example for Begi...
Hello friends, new #video on #liskovsubstitutionprinciple #solidprinciples with #Java #coding #example is published on #codeonedigest #youtube channel. Learn #lsp #principle #programming #coding with codeonedigest.
@java #java #awscloud @awscloud @AWSCloudIndia #Cloud #CloudComputing @YouTube #youtube #liskovsubstitutionprinciple #liskovsubstitutionprinciplesolid #lsp #lspprinciple #liskovsubstitutionprinciple #liskov #liskovprinciple #solidprinciples #solidprinciplesinterviewquestions #solidprinciplesjavainterviewquestions #solidprinciplesreact #solidprinciplesinandroid #solidprinciplestutorial #solidprinciplesexplained #solidprinciplesjava #singleresponsibilityprinciple #openclosedprinciple #liskovsubstitutionprinciple #interfacesegregationprinciple #dependencyinversionprinciple #objectorientedprogramming #objectorienteddesignandmodelling #objectorienteddesign #objectorienteddesignsoftwareengineering #objectorienteddesigninterviewquestions #objectorienteddesignandanalysis #objectorienteddesigninjava #objectorienteddesignmodel #objectorienteddesignapproach #objectorienteddesignparadigm #objectorienteddesignquestions
#youtube#solid#solid principle#liskov substitution#liskov substitution principle#object oriented programming#oops#java#design principles#design patterns#software design#architecture principles
2 notes
·
View notes
Video
youtube
Single Responsibility Principle Explained with Real World Example
Full Video Link https://youtu.be/qp_JdZJRGTs Hello friends, new #video on #singleresponsibilityprinciple of #solidprinciples with #Java #coding #example is published on #codeonedigest #youtube channel. Learn #srp #principles #programming #coding with codeonedigest. #singleresponsibilityprinciple #singleresponsibilityprincipleinagile #singleresponsibilityprinciplejava #singleresponsibilityprincipleinhindi #singleresponsibilityprincipleexample #singleresponsibilityprincipletutorial #singleresponsibilityprincipleexplained #singleresponsibilityprinciplejavaexample #singleresponsibilityprinciplejavaimplementation #singleresponsibilityprinciplejavacode #singleresponsibilityprincipleexamplejava #srp #solidprinciples #solidprinciplesinterviewquestions #solidprinciplesjavainterviewquestions #solidprinciplesreact #solidprinciplesinandroid #solidprinciplestutorial #solidprinciplesexplained #solidprinciplesjava #singleresponsibilityprinciple #openclosedprinciple #liskovsubstitutionprinciple #interfacesegregationprinciple #dependencyinversionprinciple #objectorientedprogramming #objectorienteddesignandmodelling #objectorienteddesign #objectorienteddesignsoftwareengineering #objectorienteddesigninterviewquestions #objectorienteddesignandanalysis #objectorienteddesigninjava #objectorienteddesignmodel #objectorienteddesignapproach #objectorienteddesignparadigm #objectorienteddesignquestions
#youtube#solid principle#single responsibility principle#single responsibility#software design principle#software pattern#java design pattern#java design principles#java design#design principles
1 note
·
View note
Text
Dependency Inversion Principle Tutorial with Java Program Example for Beginners Â
https://youtu.be/_v7JVQsRkN4 Hello friends, new #video on #dependencyinversion #solidprinciples with #Java #coding #example is published on #codeonedigest #youtube channel. Learn #dip #dependency #inversion #principle #programming #coding with codeonedi
 Dependency Inversion Principle is the fifth and final Solid principle. Robert C. Martin’s definition of the Dependency Inversion Principle consists of two parts. High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions. An important detail to note here is that high-level and…
View On WordPress
#dependency inversion#dependency Inversion java#dependency inversion principle#dependency inversion principle java#dependency Inversion principle solid#dependency inversion vs dependency injection#dip#dip principle#interface#liskov substitution principle#object oriented design#object oriented design and analysis#object oriented design and modelling#object oriented design approach#object oriented design in java#object oriented design interview questions#object oriented design model#object oriented design paradigm#object oriented design software engineering#object oriented programming#open closed principle#single responsibility principle#solid principles#solid principles explained#solid principles in android#solid principles interview questions#solid principles java#solid principles java interview questions#solid principles react#solid principles tutorial
0 notes
Text
Interface Segregation Principle Tutorial with Java Coding Example for Beginners Â
Hello friends, new #video on #interfacesegregationprinciple #solidprinciples with #Java #coding #example is published on #codeonedigest #youtube channel. Learn #isp #interfacesegregation #principle #interface #programming #coding with codeonedigest. @java
Interface Segregation principle is the Fourth principle of SOLID principles. Interface Segregation principle states that Clients should not be forced to depend upon interfaces that they do not use. Similar to the Single Responsibility Principle, the goal of the Interface Segregation Principle is to reduce the side effects and frequency of required changes by splitting the software into multiple,…

View On WordPress
#dependency inversion principle#interface#interface segregation java#interface segregation principle#interface segregation principle solid#isp#isp principle#liskov substitution principle#object oriented design#object oriented design and analysis#object oriented design and modelling#object oriented design approach#object oriented design in java#object oriented design interview questions#object oriented design model#object oriented design paradigm#object oriented design software engineering#object oriented programming#open closed principle#single responsibility principle#solid principles#solid principles explained#solid principles in android#solid principles interview questions#solid principles java#solid principles java interview questions#solid principles react#solid principles tutorial
0 notes
Video
youtube
Interface Segregation Principle Tutorial with Java Coding Example for Be...
 Hello friends, new #video on #interfacesegregationprinciple #solidprinciples with #Java #coding #example is published on #codeonedigest #youtube channel. Learn #isp #interfacesegregation #principle #interface #programming #coding with codeonedigest.
@java #java #awscloud @awscloud @AWSCloudIndia #Cloud #CloudComputing @YouTube #interfacesegregationprinciple #interfacesegregationprinciplesolid #isp #ispprinciple #interfacesegregationprinciple #interface #interfacesegregationjava #solidprinciples #solidprinciplesinterviewquestions #solidprinciplesjavainterviewquestions #solidprinciplesreact #solidprinciplesinandroid #solidprinciplestutorial #solidprinciplesexplained #solidprinciplesjava #singleresponsibilityprinciple #openclosedprinciple #liskovsubstitutionprinciple #interfacesegregationprinciple #dependencyinversionprinciple #objectorientedprogramming #objectorienteddesignandmodelling #objectorienteddesign #objectorienteddesignsoftwareengineering #objectorienteddesigninterviewquestions #objectorienteddesignandanalysis #objectorienteddesigninjava #objectorienteddesignmodel #objectorienteddesignapproach #objectorienteddesignparadigm #objectorienteddesignquestions
#youtube#interface segregation#interface segregation principle#solid#solid principle#java design principle#software design#software design principles#solid principles
1 note
·
View note