#CodeTutorial
Explore tagged Tumblr posts
Text
Create Mesmerizing Animated Gradient Backgrounds with CSS!
💻 Dive into the world of CSS animations and learn how to create stunning gradient backgrounds that seamlessly transition colors! Watch our short tutorial to discover the power of CSS keyframes and linear gradients for adding dynamic visual effects to your web projects.
#CSS#WebDesign#FrontEndDevelopment#TechTutorial#GradientBackground#CSSAnimation#Tutorial#WebDevelopment#CodeTutorial#ProgrammingTutorial#WebDesignTutorial#CSSGradient#CSSKeyframes#WebDevTutorial
1 note
·
View note
Text
Use of Future Completer in Dart
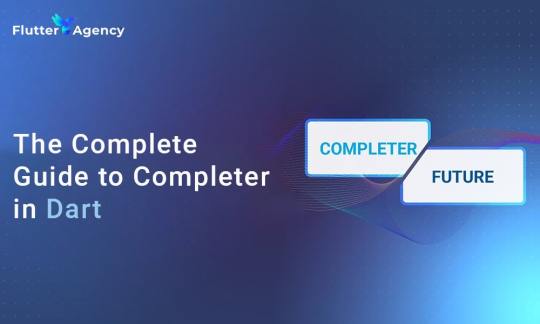
This blog will look at the programming language Future Completer in Dart. We’ll learn how to execute a demonstration program. Learn how to utilize it in your applications and how to implement it. It applies to the Flutter framework too.
Introduction
You may already be familiar with Future if you work with Dart to create Flutter applications. Future is often used to manage asynchronous tasks. Finding a Future result is a fairly simple procedure. Also you can read this article from our official website Use of Future Completer in Dart.
For instance, getting the result using the��await keyword in Dart is possible if we have the Future fetchResult() capability.
Code:
import 'dart:async';
class UserDataFetcher {
Future<string> fetchUserData() {
final completer = Completer<string>();
// Simulating a delayed asynchronous operation
Future.delayed(Duration(seconds: 5), () {
final userData = 'John Doe';
completer.complete(userData); // Completing the Future with data
});
return completer.future;
}
}
void main() async {
final userDataFetcher = UserDataFetcher();
final userData = await userDataFetcher.fetchUserData();
print('Fetched User Data: $userData');
}
</string></string>
Output
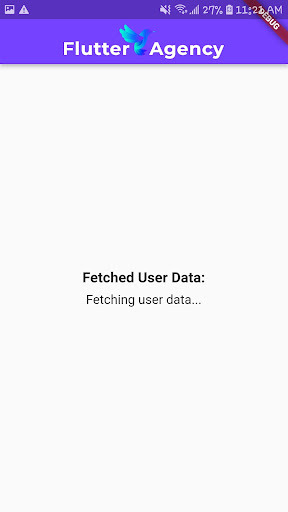
Using a Future chain is a second option.
Code:
import 'dart:async';
class UserDataFetcher {
Future<string> fetchUserData() {
return Future.delayed(Duration(seconds: 5), () {
return 'John Doe';
});
}
}
void main() async {
final userDataFetcher = UserDataFetcher();
userDataFetcher.fetchUserData().then((userData) {
print('Fetched User Data: $userData');
}).catchError((error) {
print('Error fetching user data: $error');
});
</string>
Output
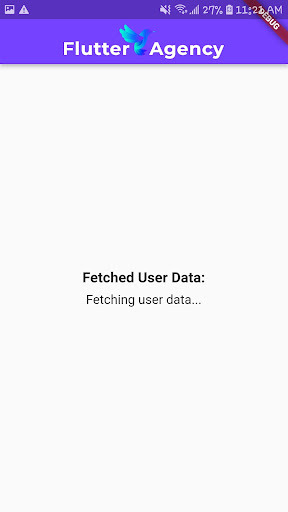
But sometimes, creating a Future using the above mentioned methods takes work. For instance, you need the Future results from a callback function. Let’s examine the following example. There is a method called run in the class MyExecutor. The callback onDone in the class’ constructor is necessary. With a value, the run method will call the onDone way. Obtaining the value passed to the onDone callback is our objective. As a Flutter app developer, tackling such situations efficiently is crucial for building robust and responsive applications.
Code:
import 'dart:async';
class MyExecutor {
void Function(String time) onDone;
MyExecutor({
required this.onDone,
});
Future<void> run() async {
final value = await fetchData(); // Simulating fetching data
onDone(value); // Call the onDone callback with the fetched value
}
Future<string> fetchData() async {
// Simulating fetching data from an external source
await Future.delayed(Duration(seconds: 2));
final DateTime time = DateTime.now();
final String formattedTime =
'${time.year}-${time.month}-${time.day} ${time.hour}:${time.minute}:${time.second}';
return formattedTime;
}
}
void main() async {
final MyExecutor myExecutor = MyExecutor(
onDone: (String time) {
print('Received value: $time');
// Handle the value when this callback is invoked
},
);
await myExecutor.run();}
</string></void>
Output
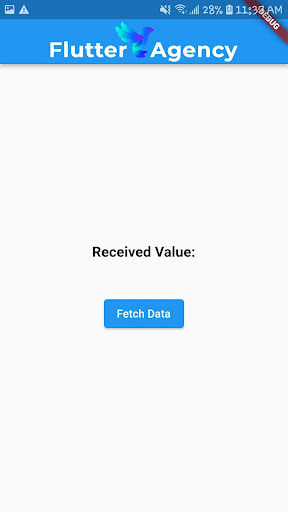
We can’t access the value directly by using await.run() since the run method returns void, which means it returns nothing. Given that everything else is equal, we must obtain the value from the callback.
Completer
To start with, call the constructor of a Completer to create another. It has a type parameter where the bring type back can be identified. Here is an example of a completer that acquires from a string.
Code
final stringCompleter = Completer<string>();
</string>
You can create a Completer with a void parameter type if it doesn’t have a return value.
Code
final voidCompleter = Completer<>()
If you don’t specify the type parameter, it will default to dynamic.
final dynamicCompleter = Completer();
If the return value can be null, you can also use a nullable type.
final nullableStringCompleter = Completer<string?>();
</string?>
Complete Future
You can call the complete technique with a return value to complete the Future. When creating the Completer, the value type should be the same as the type argument. You don’t need to pass any value if the sort border is void. Should it be dynamic, you may return a value of any type. If the sort parameter is void or nullable, you could be allowed to return null.
Code
import 'dart:async';
void main() {
final completer = Completer<string>();
// Simulating an asynchronous operation
Future.delayed(Duration(seconds: 2), () {
final result = 'Operation completed successfully!';
completer.complete(result); // Completing the Future with a value
});
completer.future.then((value) {
print('Future completed with value: $value');
});}
</string>
Output
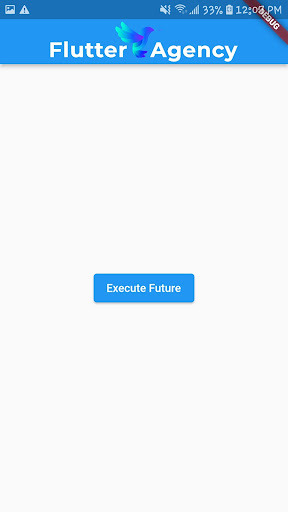
An example of how to end the Future with a string value is shown below.
stringCompleter.complete(‘HURRAH’);
One call to the entire procedure is necessary. If you call the complete method again after What’s in storage is finished, StateError will be thrown.
Complete Future with Error
A future can also throw an error. Using the completeError method, throwing an error should be possible with Completer.
Code
void completeError(Object error, [StackTrace? stackTrace]);
Here is an example of calling the completeError technique.
Code
import 'dart:async';
void main() {
final completer = Completer<string>();
// Simulating an asynchronous operation with an error
Future.delayed(Duration(seconds: 2), () {
try {
// Simulating an error condition
throw Exception('An error occurred during the operation');
} catch (ex, stackTrace) {
completer.completeError(ex, stackTrace); // Completing the Future with an error
}
});
completer.future.catchError((error, stackTrace) {
print('Future completed with error: $error');
});
}
</string>
Output
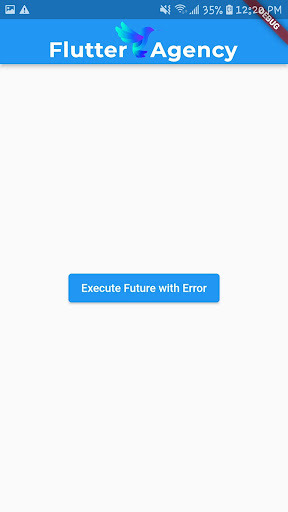
Get Future and Value
If your Completer case has a complete or completeError method, you can access the future property to retrieve the Future that has finished when that method is invoked. The await keyword can be used to return the value by the Future if you already have one.
Code
final valueFuture = stringCompleter.future;
final value = await valueFuture;
Get Completion Status
To determine the status of the project, go here. You can access the isCompleted property by representing things in the Future.
Code:
final isCompleted = stringCompleter.isCompleted;
We are merging the whole code into one screen.
Code
import 'dart:async';
class MyExecutor {
void Function(String time) onDone;
MyExecutor({
required this.onDone,
});
Future<void> run() async {
await Future.delayed(Duration(seconds: 2));
final DateTime currentTime = DateTime.now();
final String formattedTime =
'${currentTime.year}-${currentTime.month}-${currentTime.day} ${currentTime.hour}:${currentTime.minute}:${currentTime.second}';
onDone(formattedTime);
}
}
void main() async {
final timeCompleter = Completer<string>();
final MyExecutor myExecutor = MyExecutor(onDone: (String time) async {
try {
if (time.isNotEmpty) {
timeCompleter.complete(time);
} else {
throw Exception('empty time');
}
} catch (ex, stackTrace) {
timeCompleter.completeError(ex, stackTrace);
}
});
print('Fetching current time...');
await myExecutor.run();
final timeValueFuture = timeCompleter.future;
final timeValue = await timeValueFuture;
print('Current time: $timeValue');
}
</string></void>
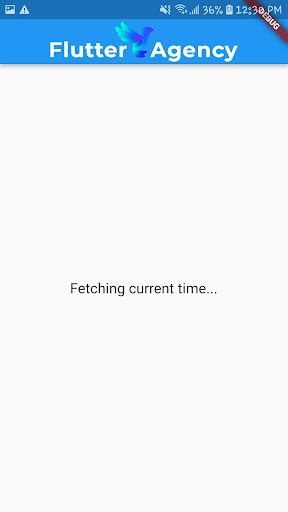
When the application is running, we should see output on the screen similar to the Console Output screen below.
isCompleted: false
isCompleted: true
value: 2023-4-7 14:54:11
Process finished with exit code 0
Conclusion
I explained the Future Completer in Dart in this post; you can change the code as you see fit. This short introduction to the Future Completer In Dart User Interaction on my part demonstrates how Flutter is used to make it effective.
Completer is a choice that can be used to create a Future in Dart/Flutter. The usage is pretty simple. Call the complete or completeError strategy if you wish to make a Completer. A completer’s Future can be obtained from the future property. It is possible to determine whether What’s in the Future has been completed using the isCompleted property.
This blog will provide enough knowledge to try the Future Completer in Dart of your projects. Please give it a try.
Whether a beginner or an experienced developer, www.flutteragency.com is a valuable resource to expand your knowledge and improve your proficiency in Dart and Flutter. Happy coding!
Frequently Asked Questions (FAQs)
1. What is Dart with Completer?
Completers represent the concept of completing a task at a later time. Dart allows us to define the entire thing in a completer object rather than transmitting a callback to be triggered together with a future value. Completers are intimately related to Futures because they begin an activity in the Future.
2. How does Flutter’s Completer perform?
Importing the Dart: async core library to use completers would be best.AsyncQuery() interacts with a hypothetical network request represented by getHttpData() in the example. Our procedure will initially return a future but later resolve into string data.
3. Define FutureBuilder.
FutureBuilder is a widget in Flutter that builds itself based on the most current Future snapshot in response to changes in state or dependencies. We can execute asynchronous functions using FutureBuilder and modify our user interface in response to the function’s results.
#flutter app development#flutter app developers#hire flutter developer#flutter agency#hire flutter developers#CodeTutorial#FutureCompleter
0 notes
Text
Primeros Pasos en Programación: Guía Completa
Introducción
Bienvenido al mundo de la programación. Si estás aquí, probablemente estás dando tus primeros pasos en el vasto campo del desarrollo de software. Puede parecer abrumador al principio, con tantos lenguajes, herramientas y conceptos desconocidos, pero no te preocupes. Este blog está diseñado para guiarte en este viaje, ofreciéndote una introducción clara y consejos prácticos para que puedas empezar con buen pie.
1. ¿Qué es la Programación?
La programación es el proceso de crear instrucciones que una computadora puede seguir para realizar tareas específicas. Estas instrucciones se escriben en un lenguaje de programación, que es un conjunto de reglas y sintaxis que los humanos pueden usar para comunicarse con las computadoras.
Lenguajes de Programación Populares:
Python: Fácil de aprender y ampliamente utilizado en ciencia de datos, desarrollo web, automatización y más.
JavaScript: El lenguaje del web, esencial para desarrollar aplicaciones y sitios interactivos.
Java: Famoso por su uso en aplicaciones empresariales y móviles (especialmente en Android).
C++: Utilizado en desarrollo de software de sistemas, juegos, y aplicaciones de alto rendimiento.
2. Conceptos Básicos de Programación
a) Variables y Tipos de Datos
Variables: Son contenedores que almacenan valores que pueden cambiar durante la ejecución del programa.
Ejemplo en Python: x = 5 asigna el valor 5 a la variable x.
Tipos de Datos: Representan la naturaleza de los valores almacenados en las variables.
Enteros: int (números sin decimales)
Flotantes: float (números con decimales)
Cadenas: str (secuencias de caracteres)
Booleanos: bool (True o False)
b) Estructuras de Control
Condicionales: Permiten que un programa tome decisiones.
Ejemplo: if x > 0: print("x es positivo")
Bucles: Ejecutan un bloque de código repetidamente.
Ejemplo: for i in range(5): print(i) imprimirá los números del 0 al 4.
c) Funciones
Las funciones son bloques de código reutilizables que realizan una tarea específica.
Ejemplo en Python: def suma(a, b): return a + b print(suma(2, 3)) # Salida: 5
3. Elige tu Primer Lenguaje de Programación
Si eres nuevo en la programación, te recomiendo empezar con Python por las siguientes razones:
Sintaxis Simple: La sintaxis de Python es clara y fácil de entender, lo que permite concentrarte en aprender conceptos básicos de programación sin enredarte en detalles complejos.
Comunidad Amplia: Hay muchos recursos de aprendizaje disponibles, incluyendo tutoriales, foros y documentación oficial.
Versatilidad: Python se utiliza en una amplia gama de aplicaciones, desde desarrollo web hasta inteligencia artificial.
4. Herramientas Esenciales
a) Entornos de Desarrollo Integrados (IDEs)
VS Code (Recomendado): Un editor de código ligero y personalizable que soporta múltiples lenguajes.
PyCharm: Un IDE robusto para Python que ofrece herramientas avanzadas para el desarrollo y depuración.
b) Control de Versiones
Git: Una herramienta esencial para el control de versiones, que te permite rastrear cambios en tu código y colaborar con otros desarrolladores.
GitHub: Un servicio basado en la nube que facilita la colaboración y el alojamiento de proyectos.
5. Primeros Proyectos para Principiantes
Comenzar con pequeños proyectos es una excelente manera de aplicar lo que has aprendido y adquirir confianza. Aquí tienes algunas ideas de proyectos:
Calculadora Básica:
Crea una calculadora que pueda realizar operaciones básicas como suma, resta, multiplicación y división.
Juego de Adivinanza de Números:
Un programa que elige un número al azar y pide al usuario que lo adivine. Puedes agregar funciones como limitar el número de intentos y dar pistas si el número es mayor o menor.
Lista de Tareas (To-Do List):
Una aplicación simple que permite a los usuarios agregar, eliminar y marcar tareas como completadas.
6. Consejos Útiles para Principiantes
a) Practica Regularmente
La programación es una habilidad práctica. Cuanto más código escribas, mejor entenderás los conceptos.
Utiliza plataformas como LeetCode o HackerRank para resolver problemas de programación.
b) No Tengas Miedo de Cometer Errores
Cometer errores es parte del proceso de aprendizaje. Cada error que cometes es una oportunidad para aprender algo nuevo.
c) Aprende a Buscar Información
Saber cómo buscar respuestas a tus preguntas es una habilidad vital. Stack Overflow es un recurso invaluable donde puedes encontrar soluciones a problemas comunes.
d) Colabora y Comparte tu Trabajo
Participa en comunidades de desarrolladores, como GitHub o Reddit. Compartir tu trabajo y colaborar con otros te expondrá a nuevas ideas y te ayudará a mejorar.
e) Mantente Curioso
La tecnología está en constante evolución. Mantente al día con las últimas tendencias y tecnologías para seguir creciendo como desarrollador.
7. Recursos Adicionales
a) Cursos y Tutoriales
CódigoFacilito (Página web): Ofrece una amplia variedad de cursos gratuitos en español sobre programación, desarrollo web, bases de datos y más. Además, cuenta con tutoriales y una comunidad activa que apoya el aprendizaje colaborativo.
freeCodeCamp (Página web): Un excelente recurso gratuito que cubre desde conceptos básicos hasta proyectos avanzados.
Desarrolloweb.com: Un portal completo que ofrece artículos, tutoriales y guías sobre programación y desarrollo web. Es una excelente fuente para aprender HTML, CSS, JavaScript, PHP, y otros lenguajes de programación.
Píldoras Informáticas (Canal de YouTube): Explica conceptos de programación y desarrollo de software en videos cortos y fáciles de entender.
HolaMundo (Canal de YouTube): Un canal dedicado a enseñar programación en español, con cursos completos de Java, Python, C++, y más.
Fazt Code (Canal de YouTube): Ofrece tutoriales y guías sobre desarrollo web, especialmente en JavaScript, Node.js, y frameworks modernos.
b) Libros Recomendados
“Python para todos” de Raúl González Duque: Este libro es una excelente introducción a Python, diseñado para principiantes. Está escrito de manera sencilla y práctica, ideal para quienes quieren aprender a programar desde cero.
“Aprende JavaScript desde cero” de Victor Moreno: Un libro que te guía paso a paso en el aprendizaje de JavaScript. Es perfecto para principiantes que desean entender el lenguaje desde sus fundamentos y aplicar lo aprendido en proyectos reales.
“Programación en C” de Luis Joyanes Aguilar: Este es un clásico en la literatura técnica en español, ideal para quienes desean aprender el lenguaje C, uno de los más fundamentales y poderosos en la programación.
“Introducción a la programación con Python” de Jesús Conejo: Otro excelente recurso para aprender Python, este libro está enfocado en estudiantes y autodidactas que desean adquirir una base sólida en programación utilizando Python.
“El gran libro de HTML5, CSS3 y JavaScript” de Juan Diego Gauchat: Este libro cubre los fundamentos del desarrollo web moderno, incluyendo HTML5, CSS3 y JavaScript. Es una guía completa para aquellos que quieren empezar a construir sitios y aplicaciones web.
Conclusión
Adentrarse en la programación es una experiencia emocionante y gratificante. Con paciencia, práctica y los recursos adecuados, estarás bien encaminado hacia convertirte en un desarrollador competente. Recuerda que cada experto fue una vez un principiante, y lo más importante es disfrutar del proceso de aprendizaje.
#programación#aprendiendoaprogramar#principiantesenprogramación#tutorialesdeprogramación#conceptosbásicosdeprogramación#python#javascript#coding#desarrollodesoftware#programadoresprincipiantes#programacion#codinglife#desarrolladores#aprendeaprogramar#empezandoaprogramar#comienzaaprogramar#programacionprincipiantes#codingforbeginners#programadoresnovatos#tutorialesdecoding#codetutorials#aprendecoding#basicosdeprogramacion#fundamentosdeprogramacion#codingsimplificado#pythoncode#pythonprogramming#pythondev#js#javascriptcode
8 notes
·
View notes
Text
Final Class and Methods in Details: https://youtu.be/UsT41L1b_4E Final Class and Method #for_experience #php #phptutorials #programming #coding #coding #programming #webdev #softwaredev #developers #learncode #codetutorial #beginnercoding #webdevelopment #fullstackdeveloper #frontenddeveloper #backenddeveloper #softwareengineer
1 note
·
View note
Video
youtube
Aula 16 - Golang - Fiber - Implementando a Redefinição de Senhas
Olá, pessoal do Tumblr!
Você está interessado em desenvolvimento web e deseja aprimorar suas habilidades em Golang? Então, temos uma ótima notícia para você!
Nesta aula empolgante, vamos aprender a implementar a funcionalidade de redefinição de senhas usando o poderoso framework Golang, o Fiber.
Durante a aula, exploraremos a criação de uma funcionalidade robusta de redefinição de senhas, com foco em segurança e eficiência.
Abordaremos conceitos importantes, como UTF-8 e runas, para garantir que você tenha uma compreensão sólida das melhores práticas em desenvolvimento web.
Acesse agora:https://www.codigofluente.com.br/aula-16-golang-fiber-implementando-a-redefinicao-de-senhas/
Esperamos contar com a sua presença nessa aula imperdível! Compartilhe esta oportunidade com seus colegas interessados em Golang e desenvolvimento web e junte-se a nós nessa jornada de aprendizado!
#Golang #FiberFramework #DesenvolvimentoWeb #WebDevelopment #SOLID #DDD #HexagonalArchitecture #Segurança #UTF8 #Runas #RedefinicaoDeSenhas #CodeTutorial #AprenderProgramacao #BloggerCommunity #Educação #AulasOnline
0 notes
Photo
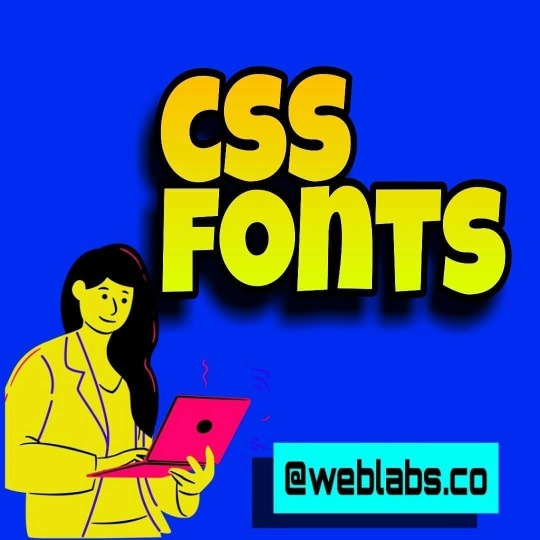
Font Family The font family of a text is set with the font-family property. The font-family property should hold several font names as a "fallback" system. If the browser does not support the first font, it tries the next font, and so on. Start with the font you want, and end with a generic family, to let the browser pick a similar font in the generic family, if no other fonts are available. 14/100 ♥WANT TO SHOW YOUR LOVE♥ 🔵Follow = @weblabs.co🔴 🔴Follow = @weblabs.co🔵 __________________________________ __________________________________ HOW WAS IT! DID IT HELP YOU? TELL US BY LIKING THE PICTURE. ♥♥♥ __________________________________ 🏆WANT TO BECOME THE BEST!🏆 🔵Follow = @weblabs.co🔴 🔴Follow = @weblabs.co🔵 __________________________________ #⃣♥HASHTAG RESEARCH♥#⃣ #htmlcoding #dev #webdevelopment #programmingmemes #programmer #developers #appdeveloper #frontend #webdesign #100daysofcode #webdev #codetutorial #coders #learntocode #csstricks #htmlcss #frontenddeveloper #webapp #websites #webdeveloper #frontenddev #cssanimation #css #oval #learncoding #triangle #visualstudio #cssshapes (at India) https://www.instagram.com/hostinge_tech/p/CSfN9v_r4SC/?utm_medium=tumblr
#⃣♥hashtag#⃣#htmlcoding#dev#webdevelopment#programmingmemes#programmer#developers#appdeveloper#frontend#webdesign#100daysofcode#webdev#codetutorial#coders#learntocode#csstricks#htmlcss#frontenddeveloper#webapp#websites#webdeveloper#frontenddev#cssanimation#css#oval#learncoding#triangle#visualstudio#cssshapes
0 notes
Text
Why choose BigCommerce for your online Store?
Introduction
BigCommerce was founded in 2009, growing to be a business of more than 25 billion dollars, with more than 600 employees. It justifies the use of the word “Big” in its title. It is a premium e-commerce site that is used by all kinds of enterprises to set up their online stores and customize them according to their requirements. All kinds of products and services can be sold on this platform.
A major reason for its popularity among businessmen is that it enables them to access the store from anywhere and is a SaaS (Software as a Service) which means that all the tools they need to set up the store are available to them, from themes to layouts, which is beneficial to them. If you are looking to set up your store on Big Commerce, it is required for you to know more about this platform before using it for your future use.
Features of BigCommerce
Customization
Store Customization is the practice of editing, and customizing your storefront using the tools and design templates provided in the program. The drop-and-drag visual editing tool is used in BigCommerce to add design and customized elements and the biggest merit is that you do not need to write any codes. This is helpful for beginners and people from non-technical backgrounds which makes it likable and profitable for businesses.
CMS
Content is one of the ways through which you can associate with your customers and even invite visiting users into becoming one. That is why it is integral for you to focus on managing the content of the website.
Publishing quality content, and constant updates about newly launched products, events, news, etc helps in connecting with your audience better which in turn enhances brand awareness. BigCommerce has an effective and stable CMS which will help you in promoting your content and raising your rank in the SERPs which is a big boost to your business.
Design Themes
It provides a large number of built-in themes that are open to editing and changes, which means, you have the freedom to let your imagination flow and customize them according to your wish. The themes are developed using HTML, JavaScript, and CSS which is easily learned and even a beginner who knows these can create new themes and design templates on this platform.
Speed
Site speed is important for your business to be noticed. Smooth and fast loading speed ensures more traffic and higher ranking. Since BigCommerce is supported by Google AMP, Google Cloud Platform structure, and Akami Manager Built In, you can rest assured that the site speed of your store will be excellent.
Payment
BigCommerce supports a lot of cashless payment options like Amazon Pay, Apple Pay, and PayPal, along with other digital wallets that make the checkout process transparent and easier on both the client and seller’s side.
You can operate stores on multiple platforms such as Instagram, Facebook, Amazon, etc because it provides a cross-platform structure. This means that you get to connect with a larger audience and increase sales of your services and products.
Industries using BigCommerce
BigCommerce is very popular in the business world. Many established and large companies use this e-commerce platform to boost their sales and generate revenue and create brand awareness. With many tools and techniques unique to it, BigCommerce offers many packages that businessmen can choose from according to their business plans. Some of the big names that can be found on this platform are-
Sony
Almanac
Casio
RAZER
Game Nerds
Dremel
Why is setting up a Store in BigCommerce Beneficial?
BigCommerce has a strong foothold in the business sector because of the many features and benefits that come with this platform. Some of the best advantages you are guaranteed while setting up your store in BigCommerce are as follows.
Easy Usage
It is easy to set up and use. This is especially beneficial for people who are beginners or don't have a technical background. They can quickly and efficiently set up a fully functional online store with tools and techniques that are provided by the platform without the need for writing any code or downloading additional apps or plugins. As a result, BigCommerce can compete successfully with other e-commerce websites.
Website Optimization
SEO (Search Engine Optimization) is a very critical part of online business. Optimization of your store or website can drastically improve your standing in the industry and you get to have many opportunities for increasing your rank in the SERPs. BigCommerce follows the latest SEO practices that help you in optimization, enriching your website with appropriate keywords, and checking for any malicious content that might be a threat to your ranking.
Customer Satisfaction
It provides quality customer service to all the users who visit online stores. It includes a feedback and query forums, 24/7 customer support, a short waiting period, quick checkout and payment services, etc. These factors must be taken into consideration to ensure that your business offers quality customer service.
Conclusion
Since the past few years, BigCommerce has taken up a stable position in e-commerce. It is convenient, efficient, user-friendly, and quick, which is why it is an ideal program for all kinds of businesses. So, if you want to start your online business, BigCommerce is the one for you.
#BigCommerceDevelopment#eCommerce#OnlineStore#WebDevelopment#WebDesign#WebDevelopmentTips#WebDevelopmentTricks#WebDevelopmentTutorials#WebDevelopmentResources#WebDevelopmentCommunity#CodeSnippet#CodeTips#CodeTricks#CodeTutorials#CodeResources#PHPDevelopment#JavaScriptDevelopment#CSSDevelopment#HTMLDevelopment
0 notes
Photo
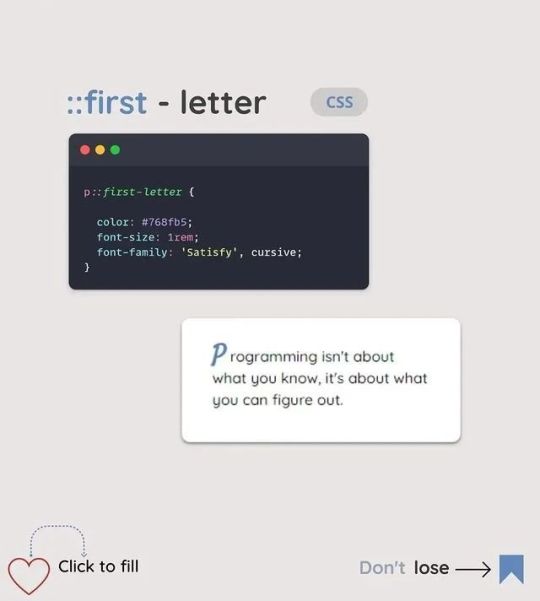
CSS SHORT TRICKS 😎 -------------------------------------------- !! FOLLOW US TO LEARN ALL ABOUT WEB DEVELOPMENT !! ---------------------------------------------- #grootacademy #grootsoftware #webdevelopment #programminglife #codingbootcamp #codingmemes #weprogrammers #frontendchallenge #fullstack #backend #programmingmemes #pythonprogramming #javascripts #webdevelopmentcompany #100dayproject #css #javaprogramming #codegeass #freecodecamp #codetutorials #appdeveloper #uidesign #webdesign #webdesignanddevelopment https://www.instagram.com/p/CiKxaO_JFUa/?igshid=NGJjMDIxMWI=
#grootacademy#grootsoftware#webdevelopment#programminglife#codingbootcamp#codingmemes#weprogrammers#frontendchallenge#fullstack#backend#programmingmemes#pythonprogramming#javascripts#webdevelopmentcompany#100dayproject#css#javaprogramming#codegeass#freecodecamp#codetutorials#appdeveloper#uidesign#webdesign#webdesignanddevelopment
0 notes
Photo
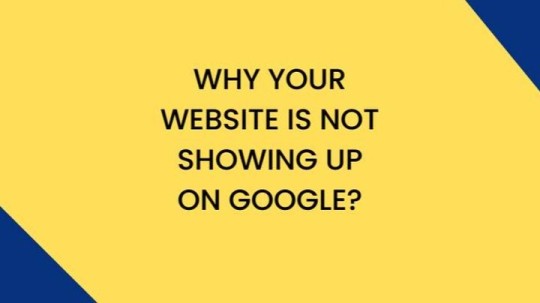
WHY YOUR WEBSITE IS NOT SHOWING UP ON GOOGLE? There are two things to this situation, It’s either indeed your website is not showing up on Google at all or It’s simply not showing up for the searches that you are performing and the reasons for this are: click on the link to find out https://www.linkedin.com/pulse/why-your-website-showing-up-google-efevwia-efemena webbikontechnologies,Best Web design Company In Abraka #bestwebdesigncompanyinabraka #webbikontechnologies #webdevelopment #programminglife #codingbootcamp #codingmemes #weprogrammers #frontendchallenge #fullstack #backend #programmingmemes #pythonprogramming #javascripts #webdevelopmentcompany #100dayproject #css #javaprogramming #codegeass #freecodecamp #codetutorials #elementor #elementorpro #searchengineoptimization https://www.instagram.com/p/Cg63Q9tNpxB/?igshid=NGJjMDIxMWI=
#bestwebdesigncompanyinabraka#webbikontechnologies#webdevelopment#programminglife#codingbootcamp#codingmemes#weprogrammers#frontendchallenge#fullstack#backend#programmingmemes#pythonprogramming#javascripts#webdevelopmentcompany#100dayproject#css#javaprogramming#codegeass#freecodecamp#codetutorials#elementor#elementorpro#searchengineoptimization
0 notes
Text
TOP 8 WEBSITES YOU WON'T BELIEVE EXIST --------------------------------------...
TOP 8 WEBSITES YOU WON’T BELIEVE EXIST ————————————–…
TOP 8 WEBSITES YOU WON’T BELIEVE EXIST 🤯😍💯 ———————————————- Hope you liked this post ☺️✌️ Let me know in the comments below 💭👇 Double Tap ♥️ if you like the Post 💰 Save This Post For Later ⏰ Turn on Post Notifications ———————————————- !! FOLLOW US TO LEARN ALL ABOUT WEB DEVELOPMENT !! @coding_.master ———————————————- #webdevelopment #programminglife #codingbootcamp #codingmemes #weprogrammers…
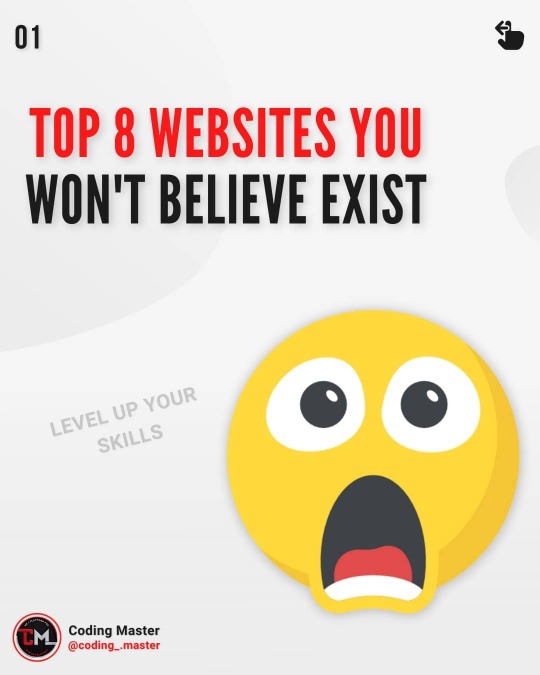
View On WordPress
#100dayproject#appdeveloper#backend#codegeass#coder#codetutorials#codingbootcamp#codingmemes#css#freecodecamp#frontendchallenge#fullstack#javaprogramming#javascripts#php#programminglife#programmingmemes#pythonprogramming#reactjs#uidesigner#uıdesign#webappdevelopment#webdesign#webdesignanddevelopment#webdesigncompany#webdesigntrends#webdevelopment#webdevelopmentcompany#websitedevelopment#weprogrammers
0 notes
Photo
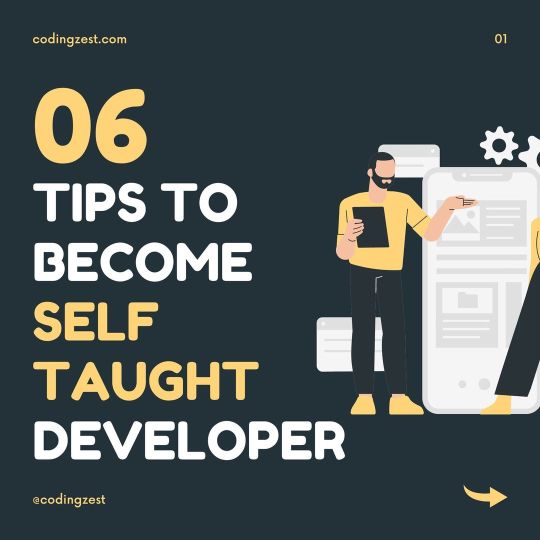
Hey Guys, In this post you will learn How to become a Self Taught Developer 🔥 🔹 Learn to Build 🔹 Learn to Google 🔹 Learn to code quickly 🔹 Learn to open source 🔹 Learn to focus 🔹 Learn to help others If This Post Is Helpful For You Then Like & Share The Post & Follow Us For More Such Amazing Content 🥰🥰🙏 ..................................................................... !! FOLLOW US TO LEARN ALL ABOUT PROGRAMMING !! Follow 👇👇 @codingzest @codingzest @codingzest ..................................................................... #webdevelopment #programminglife #codingbootcamp #codingmemes #weprogrammers #frontendchallenge #fullstack #backend #programmingmemes #pythonprogramming #javascripts #webdevelopmentcompany #100dayproject #css #javaprogramming #codegeass #freecodecamp #codetutorials #appdeveloper #uidesign #webdesign #webdesignanddevelopment #webappdevelopment #websitedevelopment #webdevelopment #reactjs #uidesigner #php #coder #webdesigncompany #webdesigntrends https://www.instagram.com/p/CXqv0Cmvujy/?utm_medium=tumblr
#webdevelopment#programminglife#codingbootcamp#codingmemes#weprogrammers#frontendchallenge#fullstack#backend#programmingmemes#pythonprogramming#javascripts#webdevelopmentcompany#100dayproject#css#javaprogramming#codegeass#freecodecamp#codetutorials#appdeveloper#uidesign#webdesign#webdesignanddevelopment#webappdevelopment#websitedevelopment#reactjs#uidesigner#php#coder#webdesigncompany#webdesigntrends
0 notes
Photo
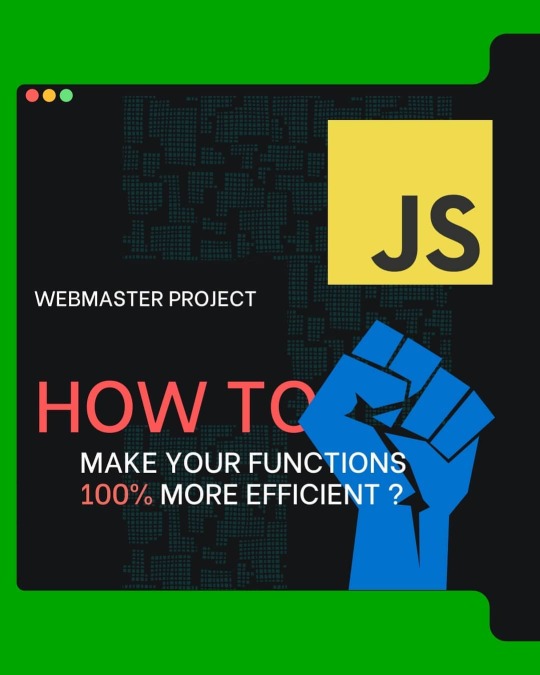
You know caching guys??🌵 Well, the cache is actually produced by such a memoization function. . . Tell me in the comments guys your tips on making code more efficient! 💡 . . . . . . . #javascripts #javascriptdevelopers #javascriptessentials #javascriptdevelopment #javascriptdeveloper #reactjs #nodejs #webmaster_project #learntocode #codetutorials #js https://www.instagram.com/p/CQ3pR-yDo4m/?utm_medium=tumblr
#javascripts#javascriptdevelopers#javascriptessentials#javascriptdevelopment#javascriptdeveloper#reactjs#nodejs#webmaster_project#learntocode#codetutorials#js
0 notes
Photo
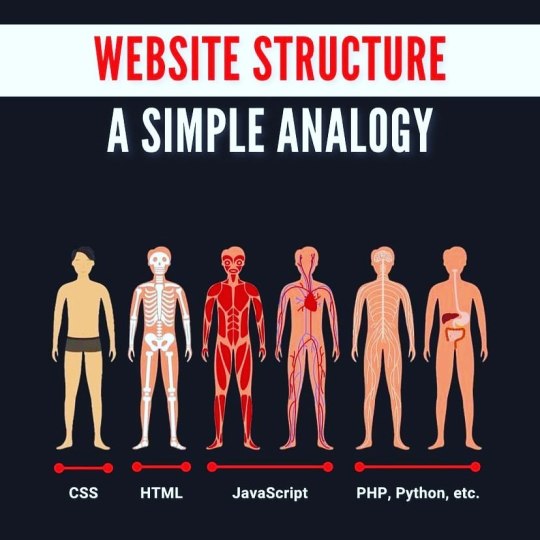
Website Structure - A Simple Analogy 🤩🤘 Share This Amazing Post 😊😊 If This Post Is Helpful For You Then Like & Share The Post & Follow Us For More Such Amazing Content 🥰🥰🙏 ..................................................................... !! FOLLOW US TO LEARN ALL ABOUT WEB DEVELOPMENT !! Follow 👇👇 @speoraofficial ..................................................................... #webdevelopment #programminglife #codingbootcamp #codingmemes #weprogrammers #frontendchallenge #programmingmemes #pythonprogramming #speora #webdevelopmentcompany #100dayproject #css #javaprogramming #codegeass #freecodecamp #codetutorials #speora #appdeveloper #uidesign #webdesign #webdesignanddevelopment #webappdevelopment #websitedevelopment #webdevelopment #reactjs #uidesigner #php #coder #webdesigncompany #webdesigntrends ................................................................... (at Delhi, India) https://www.instagram.com/p/CPGQ--cr9gb/?utm_medium=tumblr
#webdevelopment#programminglife#codingbootcamp#codingmemes#weprogrammers#frontendchallenge#programmingmemes#pythonprogramming#speora#webdevelopmentcompany#100dayproject#css#javaprogramming#codegeass#freecodecamp#codetutorials#appdeveloper#uidesign#webdesign#webdesignanddevelopment#webappdevelopment#websitedevelopment#reactjs#uidesigner#php#coder#webdesigncompany#webdesigntrends
0 notes
Photo
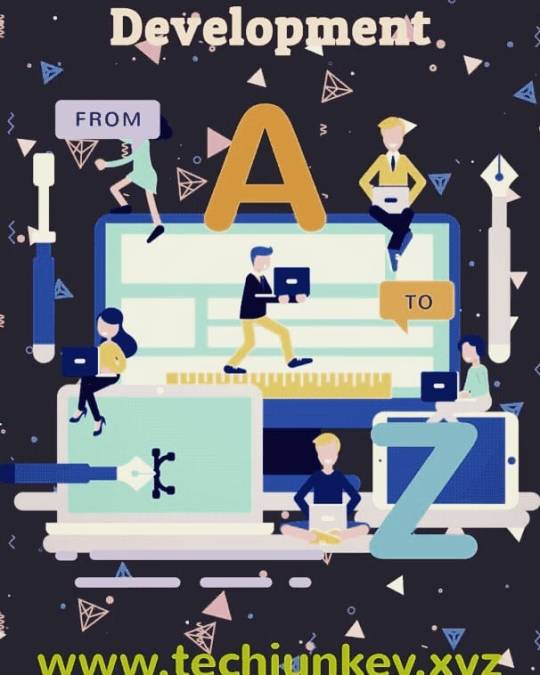
Visit techjunkey.xyz ans start learning today #python #learnpython #codetutorials #programmingisfun #learnprogramming #codelikeaboss #computerscience https://www.instagram.com/p/CIRPSGtnJ0C/?igshid=23oswnfnmbb
1 note
·
View note
Photo
Let me know how many of these you use! 😁😁😁 ======================== Follow us! @smooets @smooets @smooets Visit 🌏 www.smooets.com Contact 💌 [email protected] 𝐏𝐫𝐨𝐠𝐫𝐚𝐦𝐦𝐞𝐫 𝐎𝐮𝐭𝐬𝐨𝐮𝐫𝐜𝐢𝐧𝐠 𝐂𝐨𝐦𝐩𝐚𝐧𝐲 In the last 5 years, we've helped some of the most demanding companies in the world to solve their most difficult technology challenges. ========================
#webdesign #htmlcoding #css3 #coder #learnprogramming #developer #fullstackdeveloper #learnhtml #html5 #webdev #webdevelopment #learncss #webdeveloper #websites #frontend #learncode #websitedevelopment #htmlcss #websitedesign #web #webdeveloping #coding #frontenddev #webdevelopers #fullstack #codetutorials #developers #thecodecrumbs #weekend
1 note
·
View note
Photo
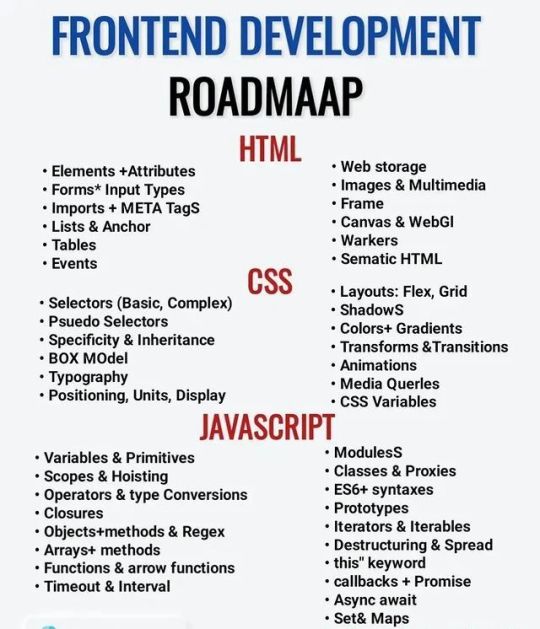
Save This Post For Future use🧠 and Tag or Share It To Your Programmer Buddy So He/She Learn It Too.. -------------------------------------------- !! FOLLOW US TO LEARN ALL ABOUT WEB DEVELOPMENT !! ---------------------------------------------- #grootacademy #grootsoftware #webdevelopment #programminglife #codingbootcamp #codingmemes #weprogrammers #frontendchallenge #fullstack #backend #programmingmemes #pythonprogramming #javascripts #webdevelopmentcompany #100dayproject #css #javaprogramming #codegeass #freecodecamp #codetutorials #appdeveloper #uidesign #webdesign #webdesignanddevelopment https://www.instagram.com/p/CiKxWUQp0lv/?igshid=NGJjMDIxMWI=
#grootacademy#grootsoftware#webdevelopment#programminglife#codingbootcamp#codingmemes#weprogrammers#frontendchallenge#fullstack#backend#programmingmemes#pythonprogramming#javascripts#webdevelopmentcompany#100dayproject#css#javaprogramming#codegeass#freecodecamp#codetutorials#appdeveloper#uidesign#webdesign#webdesignanddevelopment
0 notes