#anpost
Explore tagged Tumblr posts
Text
Rambling About C# Being Alright
I think C# is an alright language. This is one of the highest distinctions I can give to a language.
Warning: This post is verbose and rambly and probably only good at telling you why someone might like C# and not much else.
~~~
There's something I hate about every other language. Worst, there's things I hate about other languages that I know will never get better. Even worse, some of those things ALSO feel like unforced errors.
With C# there's a few things I dislike or that are missing. C#'s feature set does not obviously excel at anything, but it avoids making any huge misstep in things I care about. Nothing in C# makes me feel like the language designer has personally harmed me.
C# is a very tolerable language.
C# is multi-paradigm.
C# is the Full Middle Malcomist language.
C# will try to not hurt you.
A good way to describe C# is "what if Java sucked less". This, of course, already sounds unappealing to many, but that's alright. I'm not trying to gas it up too much here.
C# has sins, but let's try to put them into some context here and perhaps the reason why I'm posting will become more obvious:
C# didn't try to avoid generics and then implement them in a way that is very limiting (cough Go).
C# doesn't hamstring your ability to have statement lambdas because the language designer dislikes them and also because the language designer decided to have semantic whitespace making statement lambdas harder to deal with (cough Python).
C# doesn't require you to explicitly wrap value types into reference types so you can put value types into collections (cough Java).
C# doesn't ruin your ability to interact with memory efficiently because it forbids you from creating custom value types, ergo everything goes to the heap (cough cough Java, Minecraft).
C# doesn't have insane implicit type coercions that have become the subject of language design comedy (cough JavaScript).
C# doesn't keep privacy accessors as a suggestion and has the developers pinkie swear about it instead of actually enforcing it (cough cough Python).
Plainly put, a lot of the time I find C# to be alright by process of elimination. I'm not trying to shit on your favorite language. Everyone has different things they find tolerable. I have the Buddha nature so I wish for all things to find their tolerable language.
I do also think that C# is notable for being a mainstream language (aka not Haskell) that has a smaller amount of egregious mistakes, quirks and Faustian bargains.
The Typerrrrr
C# is statically typed, but the typing is largely effortless to navigate unlike something like Rust, and the GC gives a greater degree of safety than something like C++.
Of course, the typing being easy to work it also makes it less safe than Rust. But this is an appropriate trade-off for certain kinds of applications, especially considering that C# is memory safe by virtue of running on a VM. Don't come at me, I'm a Rust respecter!!
You know how some people talk about Python being amazing for prototyping? That's how I feel about C#. No matter how much time I would dedicate to Python, C# would still be a more productive language for me. The type system would genuinely make me faster for the vast majority of cases. Of course Python has gradual typing now, so any comparison gets more difficult when you consider that. But what I'm trying to say is that I never understood the idea that doing away entirely with static typing is good for fast iteration.
Also yes, C# can be used as a repl. Leave me alone with your repls. Also, while the debugger is active you can also evaluate arbitrary code within the current scope.
I think that going full dynamic typing is a mistake in almost every situation. The fact that C# doesn't do that already puts it above other languages for me. This stance on typing is controversial, but it's my opinion that is really shouldn't be. And the wind has constantly been blowing towards adding gradual typing to dynamic languages.
The modest typing capabilities C# coupled with OOP and inheritance lets you create pretty awful OOP slop. But that's whatever. At work we use inheritance in very few places where it results in neat code reuse, and then it's just mostly interfaces getting implemented.
C#'s typing and generic system is powerful enough to offer you a plethora of super-ergonomic collection transformation methods via the LINQ library. There's a lot of functional-style programming you can do with that. You know, map, filter, reduce, that stuff?
Even if you make a completely new collection type, if it implements IEnumerable<T> it will benefit from LINQ automatically. Every language these days has something like this, but it's so ridiculously easy to use in C#. Coupled with how C# lets you (1) easily define immutable data types, (2) explicitly control access to struct or class members, (3) do pattern matching, you can end up with code that flows really well.
A Friendly Kitchen Sink
Some people have described C#'s feature set as bloated. It is getting some syntactic diversity which makes it a bit harder to read someone else's code. But it doesn't make C# harder to learn, since it takes roughly the same amount of effort to get to a point where you can be effective in it.
Most of the more specific features can be effortlessly ignored. The ones that can't be effortlessly ignored tend to bring something genuinely useful to the language -- such as tuples and destructuring. Tuples have their own syntax, the syntax is pretty intuitive, but the first time you run into it, you will have to do a bit of learning.
C# has an immense amount of small features meant to make the language more ergonomic. They're too numerous to mention and they just keep getting added.
I'd like to draw attention to some features not because they're the most important but rather because it feels like they communicate the "personality" of C#. Not sure what level of detail was appropriate, so feel free to skim.
Stricter Null Handling. If you think not having to explicitly deal with null is the billion dollar mistake, then C# tries to fix a bit of the problem by allowing you to enable a strict context where you have to explicitly tell it that something can be null, otherwise it will assume that the possibility of a reference type being null is an error. It's a bit more complicated than that, but it definitely helps with safety around nullability.
Default Interface Implementation. A problem in C# which drives usage of inheritance is that with just interfaces there is no way to reuse code outside of passing function pointers. A lot of people don't get this and think that inheritance is just used because other people are stupid or something. If you have a couple of methods that would be implemented exactly the same for classes 1 through 99, but somewhat differently for classes 100 through 110, then without inheritance you're fucked. A much better way would be Rust's trait system, but for that to work you need really powerful generics, so it's too different of a path for C# to trod it. Instead what C# did was make it so that you can write an implementation for methods declared in an interface, as long as that implementation only uses members defined in the interface (this makes sense, why would it have access to anything else?). So now you can have a default implementation for the 1 through 99 case and save some of your sanity. Of course, it's not a panacea, if the implementation of the method requires access to the internal state of the 1 through 99 case, default interface implementation won't save you. But it can still make it easier via some techniques I won't get into. The important part is that default interface implementation allows code reuse and reduces reasons to use inheritance.
Performance Optimization. C# has a plethora of features regarding that. Most of which will never be encountered by the average programmer. Examples: (1) stackalloc - forcibly allocate reference types to the stack if you know they won't outlive the current scope. (2) Specialized APIs for avoiding memory allocations in happy paths. (3) Lazy initialization APIs. (4) APIs for dealing with memory more directly that allow high performance when interoping with C/C++ while still keeping a degree of safety.
Fine Control Over Async Runtime. C# lets you write your own... async builder and scheduler? It's a bit esoteric and hard to describe. But basically all the functionality of async/await that does magic under the hood? You can override that magic to do some very specific things that you'll rarely need. Unity3D takes advantage of this in order to allow async/await to work on WASM even though it is a single-threaded environment. It implements a cooperative scheduler so the program doesn't immediately freeze the moment you do await in a single-threaded environment. Most people don't know this capability exists and it doesn't affect them.
Tremendous Amount Of Synchronization Primitives and API. This ones does actually make multithreaded code harder to deal with, but basically C# erred a lot in favor of having many different ways to do multithreading because they wanted to suit different usecases. Most people just deal with idiomatic async/await code, but a very small minority of C# coders deal with locks, atomics, semaphores, mutex, monitors, interlocked, spin waiting etc. They knew they couldn't make this shit safe, so they tried to at least let you have ready-made options for your specific use case, even if it causes some balkanization.
Shortly Begging For Tagged Unions
What I miss from C# is more powerful generic bounds/constraints and tagged unions (or sum types or discriminated unions or type unions or any of the other 5 names this concept has).
The generic constraints you can use in C# are anemic and combined with the lack of tagged unions this is rather painful at times.
I remember seeing Microsoft devs saying they don't see enough of a usecase for tagged unions. I've at times wanted to strangle certain people. These two facts are related to one another.
My stance is that if you think your language doesn't need or benefit from tagged unions, either your language is very weird, or, more likely you're out of your goddamn mind. You are making me do really stupid things every time I need to represent a structure that can EITHER have a value of type A or a value of type B.
But I think C# will eventually get tagged unions. There's a proposal for it here. I would be overjoyed if it got implemented. It seems like it's been getting traction.
Also there was an entire section on unchecked exceptions that I removed because it wasn't interesting enough. Yes, C# could probably have checked exceptions and it didn't and it's a mistake. But ultimately it doesn't seem to have caused any make-or-break in a comparison with Java, which has them. They'd all be better off with returning an Error<T>. Short story is that the consequences of unchecked exceptions have been highly tolerable in practice.
Ecosystem State & FOSSness
C# is better than ever and the tooling ecosystem is better than ever. This is true of almost every language, but I think C# receives a rather high amount of improvements per version. Additionally the FOSS story is at its peak.
Roslyn, the bedrock of the toolchain, the compiler and analysis provider, is under MIT license. The fact that it does analysis as well is important, because this means you can use the wealth of Roslyn analyzers to do linting.
If your FOSS tooling lets you compile but you don't get any checking as you type, then your development experience is wildly substandard.
A lot of stupid crap with cross-platform compilation that used to be confusing or difficult is now rather easy to deal with. It's basically as easy as (1) use NET Core, (2) tell dotnet to build for Linux. These steps take no extra effort and the first step is the default way to write C# these days.
Dotnet is part of the SDK and contains functionality to create NET Core projects and to use other tools to build said projects. Dotnet is published under MIT, because the whole SDK and runtime are published under MIT.
Yes, the debugger situation is still bad -- there's no FOSS option for it, but this is more because nobody cares enough to go and solve it. Jetbrains proved anyone can do it if they have enough development time, since they wrote a debugger from scratch for their proprietary C# IDE Rider.
Where C# falls flat on its face is the "userspace" ecosystem. Plainly put, because C# is a Microsoft product, people with FOSS inclinations have steered clear of it to such a degree that the packages you have available are not even 10% of what packages a Python user has available, for example. People with FOSS inclinations are generally the people who write packages for your language!!
I guess if you really really hate leftpad, you might think this is a small bonus though.
Where-in I talk about Cross-Platform
The biggest thing the ecosystem has been lacking for me is a package, preferably FOSS, for developing cross-platform applications. Even if it's just cross-platform desktop applications.
Like yes, you can build C# to many platforms, no sweat. The same way you can build Rust to many platforms, some sweat. But if you can't show a good GUI on Linux, then it's not practically-speaking cross-platform for that purpose.
Microsoft has repeatedly done GUI stuff that, predictably, only works on Windows. And yes, Linux desktop is like 4%, but that 4% contains >50% of the people who create packages for your language's ecosystem, almost the exact point I made earlier. If a developer runs Linux and they can't have their app run on Linux, they are not going to touch your language with a ten foot pole for that purpose. I think this largely explains why C#'s ecosystem feels stunted.
The thing is, I'm not actually sure how bad or good the situation is, since most people just don't even try using C# for this usecase. There's a general... ecosystem malaise where few care to use the language for this, chiefly because of the tone that Microsoft set a decade ago. It's sad.
HOWEVER.
Avalonia, A New Hope?
Today we have Avalonia. Avalonia is an open-source framework that lets you build cross-platform applications in C#. It's MIT licensed. It will work on Windows, macOS, Linux, iOS, Android and also somehow in the browser. It seems to this by actually drawing pixels via SkiaSharp (or optionally Direct2D on Windows).
They make money by offering migration services from WPF app to Avalonia. Plus general support.
I can't say how good Avalonia is yet. I've researched a bit and it's not obviously bad, which is distinct from being good. But if it's actually good, this would be a holy grail for the ecosystem:
You could use a statically typed language that is productive for this type of software development to create cross-platform applications that have higher performance than the Electron slop. That's valuable!
This possibility warrants a much higher level of enthusiasm than I've seen, especially within the ecosystem itself. This is an ecosystem that was, for a while, entirely landlocked, only able to make Windows desktop applications.
I cannot overstate how important it is for a language's ecosystem to have a package like this and have it be good. Rust is still missing a good option. Gnome is unpleasant to use and buggy. Falling back to using Electron while writing Rust just seems like a bad joke. A lot of the Rust crates that are neither Electron nor Gnome tend to be really really undercooked.
And now I've actually talked myself into checking out Avalonia... I mean after writing all of that I feel like a charlatan for not having investigated it already.
69 notes
·
View notes
Text
Watch "This is How Queen Bees are Shipped in the Mail" on YouTube
amazing material!
0 notes
Text
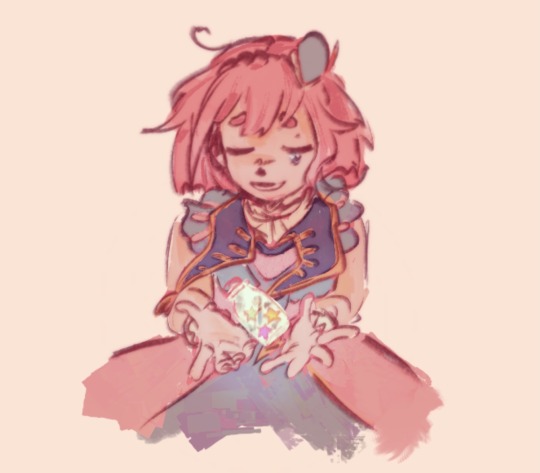
always by your side
#couuugh. whehezze#hold on#project sekai#emu otori#pjsk#prsk#proseka#ok thatsg enough RANK 96 COOOOOGUH WHEEEZE#literlaly cough wheez ei have fucking covid. i wanted to draw something nicer for the event but my hands rlly hrut snd my throat hirts and#i was sticking my head in the freezer in between matches.#omfg i didnt think the end sprint was gonna be so insane i didnt have enough energy. mfers made me spend 700 gems. nene please.#i never wanna open the game agaon.(guy who will open it tomorrow and sunday) 16 MIL POINTS.. pimh was only 9mil. for rank 80smth.#the hatsune miku colorful stage tiering economy is in shambles#'im never doing that again' [will do it again in august]#event was insane. started out ill -> only 1 rate up card -> charger broke -> assignments -> covid on the last day. Be fr#to my beloved sakurako and fixer i wub you. ill try to finish my nice profile but well honk mimimi.#NSIFFLKE. SNIFFLE. WAAAAAAH#this is so lazy but i havent drawn for myself literally in a week. other than doodles i did between matches#actually theyre like little bobblehead emus all over my sketchbooks i should collage them into anpost#idk how people get that subtle gaussian blur on their lines i tried it but it looks so obvious to me here.. maybe bc i used a thick brush..#ok wonderhoy i need to lay down so bad tylenol save me. I ACCIDENTALLY SWALLOWED MY LOZENGE IN THE MIDDLE OF A GAAAAAME
931 notes
·
View notes
Text
Ahaha. Heyyy. My disabled ass needs help with rent.
I'm really hoping this will just be this once because I am actively trying for every job I can get, but... yeah.
Not to mention my brother will have to stay with me for the holidays, so that's one more mouth to feed...
buymeacoffee.com/ananan
#anposting#help#mutual aid#mutual funds#signal boost#personal finance#donation#donations#aid#fundraising#boost#donate#gofundme#donation request#please boost#important#fundraiser
126 notes
·
View notes
Text
an and i were playing hide and seek (she won)


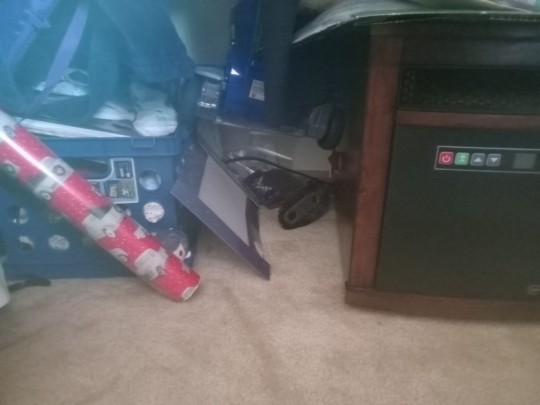


24 notes
·
View notes
Note
👉👈 would you feel like talking about Da Ice babies?
uwahh caz pls,,, i always feel like talking about them, i just have trouble containing myself once i start talking is all _(:3」∠)_ dkdksmdk
i can’t remember how much i’ve actually shared about them beyond just making kasim canon back whenever that happened, so i'll just drop a few bullet points or something to keep myself from rambling
(i usually view them in their ages around part 6 btw—20, 18 & 16. they don't do anything in p6 tho, except ramy in a canon compliant au but that's an entire can of worms in itself)
ramy
・he looks laid back but he's always getting into shit and stealing his mom’s cigarettes. ・wrestling fan. used to stone cold stunner his siblings ・likes to hide things that people are looking for with his stand—especially shay. half the time when she can't find something it's ramy's fault. loves her but loves to annoy her just as much bgbkjf ・had a juggalo phase in high school...... ・struggles to make & keep friends and has a tendency to do whatever he's dared to—no matter how dumb or disruptive it may be. ・literally terrified of creepy crawlies and WILL cry if he sees a bug in the house.
aaliyah
・major daddy’s girl like,, she used to cry whenever vanilla was out of her sight for more than 5 seconds as a kid. ・often the ‘voice of reason’ between her brothers and tries her best to keep them out of trouble... as long as ramy isn't being too annoying /lh ・she had a bit of a mean streak when she was in her mid-teens because she was self conscious about her height—embraced it a bit later on but still won’t wear anything other than flats/trainers. ・shay calls her 'bean sprout' because one minute she was just this little thing then the next she's 5'10" dfgbgj ・🏳🌈 (also a stand user but idk how to explain hers simply jhfbvhdf)
kasim
・the youngest and only child that wasn't born in florida—also the only one with shay's blue eyes ・tends to be very timid and keeps to himself when his brother isn’t dragging him around. ・carries a laptop bag literally every where since it's somewhat tied to his stand. ・not exactly a hacker since his stand does all the work but still. may or may not have accidentally accessed the pentagon's database once or twice. ・arch linux user btw. he will mention this often.
they embody this meme btw and it cracks me up whenever i think about it
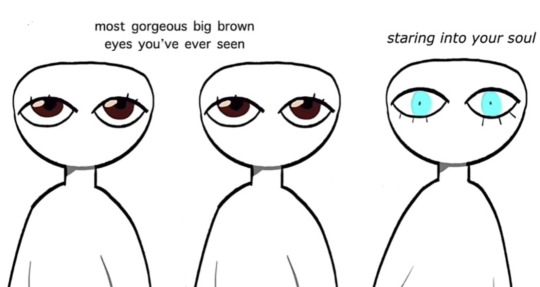
#mail 💌#goblinselfshippr#ice ice babies#i need to make anpost about their dynamic…… i need to DRAW THEM……
7 notes
·
View notes
Text
My apologies. I did not intend to let anyone hit post.
3 notes
·
View notes
Text
I’m so sick of Rossi’s bullshit being passed off as a good thing. Forcing someone into a church bc you want to cure their problems against their will while ur trying to find a killer is, in my book, unacceptable. And the show giving it a HOLY SHIT HOT GUY JUST SHOT PENELOPE
1 note
·
View note
Text
ada and leon's dynamic is so good. the way after their first interaction in 4 they both just relax after the initial scuffle. leon hasn't seen her in 6 years, last he did she betrayed him, and he still just. relaxes in her presence. the trust is still there.
#like he knows shes not going to take advantage of him the same way others have#she'll manipulate him to reach her goals sure#but i think he knows she doesnt see him as just a weapon or tool#she does genuinely care about him. even though neither of them will admit it to each other#idk its just so good. the easiness they have i guess#darcy.txt#<- is making anpost abt my partners. whatever#leon tag#ada tag#<- love you
3 notes
·
View notes
Text
It's always "funny" to remember that software development as field often operates on the implicit and completely unsupported assumption that security bugs are fixed faster than they are introduced, adjusting for security bug severity.
This assumption is baked into security policies that are enforced at the organizational level regardless of whether they are locally good ideas or not. So you have all sorts of software updating basically automatically and this is supposedly proof that you deserve that SOC2 certification.
Different companies have different incentives. There are two main incentives:
Limiting legal liability
Improving security outcomes for users
Most companies have an overwhelming proportion of the first incentive.
This would be closer to OK if people were more honest about it, but even within a company they often start developing The Emperor's New Clothes types of behaviour.
---
I also suspect that security has generally been a convenient scapegoat to justify annoying, intrusive and outright abusive auto-updating practices in consumer software. "Nevermind when we introduced that critical security bug and just update every day for us, alright??"
Product managers almost always want every user to be on the latest version, for many reasons of varying coherence. For example, it enables A/B testing (provided your software doesn't just silently hotpatch it without your consent anyway).
---
I bring this up because (1) I felt like it, (2) there are a lot of not-so-well-supported assumptions in this field, which are mainly propagated for unrelated reasons. Companies will try to select assumptions that suit them.
Yes, if someone does software development right, the software should converge towards being more secure as it gets more updates. But the reality is that libraries and applications are heavily heterogenous -- they have different risk profiles, different development practices, different development velocities, and different tooling. The correct policy is more complicated and contextual.
Corporate incentives taint the field epistemologically. There's a general desire to confuse what is good for the corporation with what is good for users with what is good for the field.
The way this happens isn't by proposing obviously insane practices, but by taking things that sound maybe-reasonable and artificially amplifying confidence levels. There are aspects of the distortion that are obvious and aspects of the distortion that are most subtle. If you're on the inside and never talked to weird FOSS people, it's easy to find it normal.
One of the eternal joys and frustrations of being a software developer is trying to have effective knowledge about software development. And generally a pre-requisite to that is not believing false things.
For all the bullshit that goes on in the field, I feel _good_ about being able to form my own opinions. The situation, roughly speaking, is not rosy, but learning to derive some enjoyment from countering harmful and incorrect beliefs is a good adaptation. If everyone with a clue becomes miserable and frustrated then computing is doomed. So my first duty is to myself -- to talk about such things without being miserable. I tend to do a pretty okay job at that.
#i know to some of you i'm just stating the sky is blue#software#computing#security#anpost#this was an anramble at first but i just kept writing i guess#still kind of a ramble
49 notes
·
View notes
Text
So true, all of it. The recent AI book cover prize winner debacle made me incredibly dejected. Writers and readers dunking on artists right until there was literally no ground to stand on... The lack of perspective or self awareness was disturbing.
A note to all creatives:
Right now, you have to be a team player. You cannot complain about AI being used to fuck over your industry and then turn around and use it on somebody else’s industry.
No AI book covers. No making funny little videos using deepfakes to make an actor say stuff they never did. No AI translation of your book. No AI audiobooks. No AI generated moodboards or fancasts or any of that shit. No feeding someone else’s unfinished work into Chat GPT “because you just want to know how it ends*” (what the fuck is wrong with you?). No playing around with AI generated 3D assets you can’t ascertain the origin of. None of it. And stop using AI filters on your selfies or ESPECIALLY using AI on somebody else’s photo or artwork.
We are at a crossroad and at a time of historically shitty conditions for working artists across ALL creative fields, and we gotta stick together. And you know what? Not only is standing up for other artists against exploitation and theft the morally correct thing to do, it’s also the professionally smartest thing to do, too. Because the corporations will fuck you over too, and then they do it’s your peers that will hold you up. And we have a long memory.
Don’t make the mistake of thinking “your peers” are only the people in your own industry. Writers can’t succeed without artists, editors, translators, etc making their books a reality. Illustrators depend on writers and editors for work. Video creators co-exist with voice actors and animators and people who do 3D rendering etc. If you piss off everyone else but the ones who do the exact same job you do, congratulations! You’ve just sunk your career.
Always remember: the artists who succeed in this career path, the ones who get hired or are sought after for commissions or collaboration, they aren’t the super talented “fuck you I got mine” types. They’re the one who show up to do the work and are easy to get along with.
And they especially are not scabs.
*that’s not even how it ends that’s a statistically likely and creatively boring way for it to end. Why would you even want to read that.
60K notes
·
View notes
Text
Help Me Pay Rent And Buy Food
Making a separate post for this as well because I don't know if my last one is even being seen. Please help me out however you can, even if that's just by reblogging this. That's all I ask. I don't want to live on the streets I really don't
#mutual aid#mutual funds#gofundme#aid#help#emergency fund#funds#fundraiser#anposting#signal boost#boost#donation#donate#donations#lgbtq#lgbt#lgbtqia#queer#transgender#disabled#disability#fibromyalgia#chronic illness#chronic pain
46 notes
·
View notes
Text
an looks radiant in the sunlight

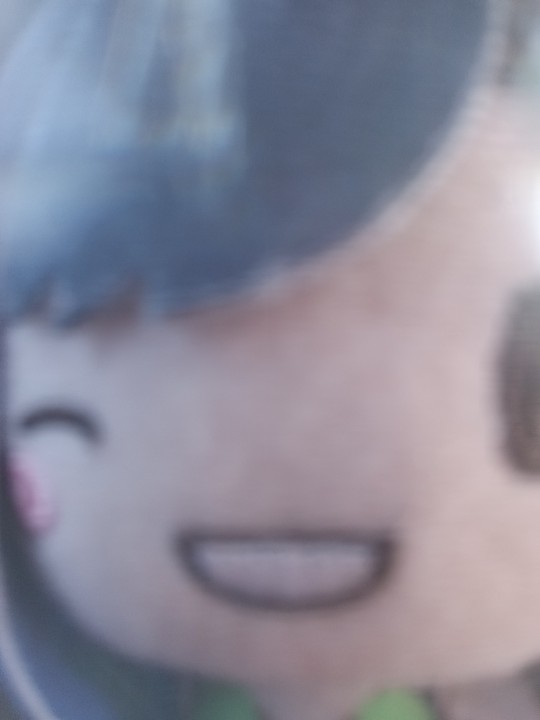
#ignore the fact that my phonrs camera is cracked pls and ty 🫶#an shiraishi#project sekai#anposting☆
7 notes
·
View notes
Text
Hello, this is AL-AN. I actually would like everyone to know that I am very open to music suggestions. As you may see, my playlist currently contains a lot of rock but I would like to broaden my horizons a bit.
3 notes
·
View notes
Text
Last song: dark things by STARSET (Please listen. It is so good. Please. I have it on repeat)
Favourite colour: Mine is pink. Collectively, we like purple.
Last book: I genuinely do not remember.
Last movie: Scooby Doo (2002)
Last Show: It was either Sonic Prime or Supernatural. I cannot presently recall.
Sweet/Spicy/Savory: All 3 but we were on Team Sweet. For Frye.
Last thing I googled: How to dispose of grease.
Current obsession: dark things by STARSET, Splatoon, and Subnautica. All three. At once.
Looking forward to: I am going to play Bioshock tomorrow!
I shall tag nobody, simply ask that my mutuals and friends fill out this post if they choose.
tagged by @billhaders
last song: taste - Sabrina Carpenter
favorite color: pink
last book: the bible
last movie: carry on i think?
last tv show: law and order (the og from the 90s we're currently rewatching)
sweet/spicy/savory: sweet and spicy mix!
last thing I googled: the movie Pedro was in with Nicholas Cage
current obsession: superman
looking forward to: being done moving in and getting my vanity area for my room
tagging: @glen-powells @tornadocowboy @manganiello @coastalwind @princessanglophile
66 notes
·
View notes
Text

this is how i felt showing the unity3d official the exact patch note where they said they added webgl audio compression, along with the documentation that changed to reflect it, along with logs from a unity3d official 4 years ago telling me that the exact problem i'm facing again today would be fixed in unity 2020 (it is now the year of our lord 2025), then handing them the most minimal repro project possible.
#anpost#programming#egoposting#unity contact people are generally really nice btw#but unity overall still gives me a strong#you people are like dogs#i will continue as normal
13 notes
·
View notes