#Python learning
Explore tagged Tumblr posts
Text
Being a beginner at programming involves experiencing every emotion all at once for roughly two hours while completing your lab assignments
10 notes
·
View notes
Text
Teach Your Kids To Code ...
is a parent's and teacher's guide to teaching kids basic programming and problem solving using Python, the powerful language used in college courses and by tech companies like Google and IBM.
Step-by-step explanations will have kids learning computational thinking right away, while visual and game-oriented examples hold their attention. Friendly introductions to fundamental programming concepts such as variables, loops, and functions will help even the youngest programmers build the skills they need to make their own cool games and applications. Whether you've been coding for years or have never programmed anything at all, Teach Your Kids to Code will help you show your young programmer how to
Explore geometry by drawing colorful shapes with Turtle graphics
Write programs to encode & decode messages, play Rock-Paper-Scissors, and calculate how tall someone is in Ping-Pong balls
Create fun, playable games like War, Yahtzee, and Pong
Add interactivity, animation, and sound to their apps
Teach Your Kids to Code is the perfect companion to any introductory programming class or after-school meet-up, or simply your educational efforts at home. Spend some fun, productive afternoons at the computer with your kids—you can all learn something!
- No Starch Press -
Post #162: Bryson Payne, Teach Your Kids To Code, A Parent-Friendly Guide To Python Programming, 336 Pages, No Starch Press, Burlingame, California, U.S.A., 2025.
#programming#coding#education#i love coding#learning#coding is fun#i love programming#i love python#no starch press#python coding#python learning#bryson payne#programmieren#studying#teaching
4 notes
·
View notes
Photo
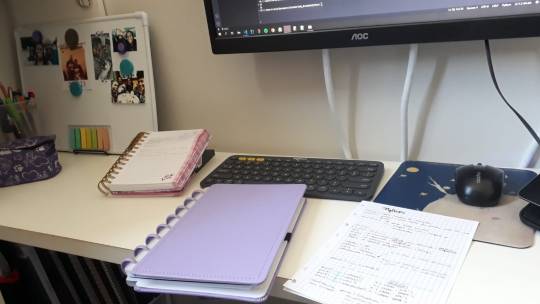
11 April - 100 days of productivity - 100/100 This is it! Completing another 100 days of productivity, starting with a short course of Python for a bootcamp test! I am impressed! Just started the course and I cannot believe how light and not verbose it is. I am flirting with Data Science for a time now (my Library Sciences degree is helping with these thoughts), and see how Python is really like is making me to seek more about it. Yes, I work with Java, and I am still learning it, so it will be a big challenge to study both (and I know that it is better to learn one language at a time), that's why I will think very well before do it. My test for the government job is coming, and this application is very important, it can help me to get another job, a better one, which will provide me more time and peace to study, so these 2 next weeks are crucial for me... There will be a lot of posts from my messy desk, my study routine, and my readings. I hope to keep up my productivity <3
#daily life#studblr#studying#study blog#study motivation#100 days of productivity#100DOP#100 dop#100 days of code#python learning#software development#Dev Life#study desk#study#motivation#Study like Hermione
22 notes
·
View notes
Text
How you can use python for data wrangling and analysis
Python is a powerful and versatile programming language that can be used for various purposes, such as web development, data science, machine learning, automation, and more. One of the most popular applications of Python is data analysis, which involves processing, cleaning, manipulating, and visualizing data to gain insights and make decisions.
In this article, we will introduce some of the basic concepts and techniques of data analysis using Python, focusing on the data wrangling and analysis process. Data wrangling is the process of transforming raw data into a more suitable format for analysis, while data analysis is the process of applying statistical methods and tools to explore, summarize, and interpret data.
To perform data wrangling and analysis with Python, we will use two of the most widely used libraries: Pandas and NumPy. Pandas is a library that provides high-performance data structures and operations for manipulating tabular data, such as Series and DataFrame. NumPy is a library that provides fast and efficient numerical computations on multidimensional arrays, such as ndarray.
We will also use some other libraries that are useful for data analysis, such as Matplotlib and Seaborn for data visualization, SciPy for scientific computing, and Scikit-learn for machine learning.
To follow along with this article, you will need to have Python 3.6 or higher installed on your computer, as well as the libraries mentioned above. You can install them using pip or conda commands. You will also need a code editor or an interactive environment, such as Jupyter Notebook or Google Colab.
Let’s get started with some examples of data wrangling and analysis with Python.
Example 1: Analyzing COVID-19 Data
In this example, we will use Python to analyze the COVID-19 data from the World Health Organization (WHO). The data contains the daily situation reports of confirmed cases and deaths by country from January 21, 2020 to October 23, 2023. You can download the data from here.
First, we need to import the libraries that we will use:import pandas as pd import numpy as np import matplotlib.pyplot as plt import seaborn as sns
Next, we need to load the data into a Pandas DataFrame:df = pd.read_csv('WHO-COVID-19-global-data.csv')
We can use the head() method to see the first five rows of the DataFrame:df.head()
Date_reportedCountry_codeCountryWHO_regionNew_casesCumulative_casesNew_deathsCumulative_deaths2020–01–21AFAfghanistanEMRO00002020–01–22AFAfghanistanEMRO00002020–01–23AFAfghanistanEMRO00002020–01–24AFAfghanistanEMRO00002020–01–25AFAfghanistanEMRO0000
We can use the info() method to see some basic information about the DataFrame, such as the number of rows and columns, the data types of each column, and the memory usage:df.info()
Output:
RangeIndex: 163800 entries, 0 to 163799 Data columns (total 8 columns): # Column Non-Null Count Dtype — — — — — — — — — — — — — — — 0 Date_reported 163800 non-null object 1 Country_code 162900 non-null object 2 Country 163800 non-null object 3 WHO_region 163800 non-null object 4 New_cases 163800 non-null int64 5 Cumulative_cases 163800 non-null int64 6 New_deaths 163800 non-null int64 7 Cumulative_deaths 163800 non-null int64 dtypes: int64(4), object(4) memory usage: 10.0+ MB “><class 'pandas.core.frame.DataFrame'> RangeIndex: 163800 entries, 0 to 163799 Data columns (total 8 columns): # Column Non-Null Count Dtype --- ------ -------------- ----- 0 Date_reported 163800 non-null object 1 Country_code 162900 non-null object 2 Country 163800 non-null object 3 WHO_region 163800 non-null object 4 New_cases 163800 non-null int64 5 Cumulative_cases 163800 non-null int64 6 New_deaths 163800 non-null int64 7 Cumulative_deaths 163800 non-null int64 dtypes: int64(4), object(4) memory usage: 10.0+ MB
We can see that there are some missing values in the Country_code column. We can use the isnull() method to check which rows have missing values:df[df.Country_code.isnull()]
Output:
Date_reportedCountry_codeCountryWHO_regionNew_casesCumulative_casesNew_deathsCumulative_deaths2020–01–21NaNInternational conveyance (Diamond Princess)WPRO00002020–01–22NaNInternational conveyance (Diamond Princess)WPRO0000……………………2023–10–22NaNInternational conveyance (Diamond Princess)WPRO07120132023–10–23NaNInternational conveyance (Diamond Princess)WPRO0712013
We can see that the missing values are from the rows that correspond to the International conveyance (Diamond Princess), which is a cruise ship that had a COVID-19 outbreak in early 2020. Since this is not a country, we can either drop these rows or assign them a unique code, such as ‘IC’. For simplicity, we will drop these rows using the dropna() method:df = df.dropna()
We can also check the data types of each column using the dtypes attribute:df.dtypes
Output:Date_reported object Country_code object Country object WHO_region object New_cases int64 Cumulative_cases int64 New_deaths int64 Cumulative_deaths int64 dtype: object
We can see that the Date_reported column is of type object, which means it is stored as a string. However, we want to work with dates as a datetime type, which allows us to perform date-related operations and calculations. We can use the to_datetime() function to convert the column to a datetime type:df.Date_reported = pd.to_datetime(df.Date_reported)
We can also use the describe() method to get some summary statistics of the numerical columns, such as the mean, standard deviation, minimum, maximum, and quartiles:df.describe()
Output:
New_casesCumulative_casesNew_deathsCumulative_deathscount162900.000000162900.000000162900.000000162900.000000mean1138.300062116955.14016023.4867892647.346237std6631.825489665728.383017137.25601215435.833525min-32952.000000–32952.000000–1918.000000–1918.00000025%-1.000000–1.000000–1.000000–1.00000050%-1.000000–1.000000–1.000000–1.00000075%-1.000000–1.000000–1.000000–1.000000max -1 -1 -1 -1
We can see that there are some negative values in the New_cases, Cumulative_cases, New_deaths, and Cumulative_deaths columns, which are likely due to data errors or corrections. We can use the replace() method to replace these values with zero:df = df.replace(-1,0)
Now that we have cleaned and prepared the data, we can start to analyze it and answer some questions, such as:
Which countries have the highest number of cumulative cases and deaths?
How has the pandemic evolved over time in different regions and countries?
What is the current situation of the pandemic in India?
To answer these questions, we will use some of the methods and attributes of Pandas DataFrame, such as:
groupby() : This method allows us to group the data by one or more columns and apply aggregation functions, such as sum, mean, count, etc., to each group.
sort_values() : This method allows us to sort the data by one or more
loc[] : This attribute allows us to select a subset of the data by labels or conditions.
plot() : This method allows us to create various types of plots from the data, such as line, bar, pie, scatter, etc.
If you want to learn Python from scratch must checkout e-Tuitions to learn Python online, They can teach you Python and other coding language also they have some of the best teachers for their students and most important thing you can also Book Free Demo for any class just goo and get your free demo.
#python#coding#programming#programming languages#python tips#python learning#python programming#python development
2 notes
·
View notes
Text
Python Tutorial online
A "Python Tutorial online" typically covers Python basics like variables, data types, control structures (loops, conditionals), functions, libraries, object-oriented programming (OOP), and file handling, with interactive coding exercises.
0 notes
Text
0 notes
Text
Introduction to Data Analysis with Python
Embarking on Data Analysis with Python
In the dynamic landscape of data analysis, Python serves as a versatile and powerful tool, empowering analysts to derive meaningful insights from vast datasets. This introduction aims to illuminate the journey into data analysis with Python, providing a gateway for both novices and seasoned professionals to harness the language's capabilities for exploring, understanding, and interpreting data.
Unveiling Python's Significance in Data Analysis
Python's prominence in data analysis arises from its rich ecosystem of libraries and tools specifically tailored for this purpose. This article delves into the fundamental aspects of utilizing Python for data analysis, offering a roadmap for individuals seeking to leverage Python's capabilities in extracting valuable information from diverse datasets.
Libraries and Tools at Your Fingertips
Python boasts a myriad of libraries that significantly enhance the data analysis process. From Pandas for efficient data manipulation to Matplotlib and Seaborn for compelling visualizations, this introduction explores the essential tools at your disposal and how they contribute to a seamless data analysis workflow.
Hands-On Exploration with Python
A distinctive feature of Python in data analysis lies in its practicality. This article guides you through hands-on exploration, demonstrating how to load, clean, and analyze data using Python.
Unlocking the Potential of Python in Data Analysis
As you delve deeper into the realms of data analysis with Python, discover the language's potential to uncover patterns, trends, and correlations within datasets. Python's versatility enables analysts to approach complex data scenarios with confidence, fostering a deeper understanding of the information at hand.
Conclusion: Your Gateway to Data Exploration
In conclusion, this introduction serves as your gateway to the exciting world of data analysis with Python. Whether you're a beginner or an experienced professional, LearNowx Python Training Course accessibility and robust capabilities make it an invaluable tool for unraveling the stories hidden within datasets. Get ready to embark on a journey of discovery, where Python becomes your ally in transforming raw data into actionable insights.
0 notes
Text
Python - An Overview
Python is an amazing & essential programming language to master in advanced domains like Data Science, Web Development, Robotics, the Internet of Things (IoT) & more. With its extensive usage on various apps, Python has emerged as a computer language with rapid development. Click here to know more: https://www.marsdevs.com/blogs/from-beginner-to-pro-learning-python-basics-in-2023
0 notes
Text
Essentials You Need to Become a Web Developer
HTML, CSS, and JavaScript Mastery
Text Editor/Integrated Development Environment (IDE): Popular choices include Visual Studio Code, Sublime Text.
Version Control/Git: Platforms like GitHub, GitLab, and Bitbucket allow you to track changes, collaborate with others, and contribute to open-source projects.
Responsive Web Design Skills: Learn CSS frameworks like Bootstrap or Flexbox and master media queries
Understanding of Web Browsers: Familiarize yourself with browser developer tools for debugging and testing your code.
Front-End Frameworks: for example : React, Angular, or Vue.js are powerful tools for building dynamic and interactive web applications.
Back-End Development Skills: Understanding server-side programming languages (e.g., Node.js, Python, Ruby , php) and databases (e.g., MySQL, MongoDB)
Web Hosting and Deployment Knowledge: Platforms like Heroku, Vercel , Netlify, or AWS can help simplify this process.
Basic DevOps and CI/CD Understanding
Soft Skills and Problem-Solving: Effective communication, teamwork, and problem-solving skills
Confidence in Yourself: Confidence is a powerful asset. Believe in your abilities, and don't be afraid to take on challenging projects. The more you trust yourself, the more you'll be able to tackle complex coding tasks and overcome obstacles with determination.
#code#codeblr#css#html#javascript#java development company#python#studyblr#progblr#programming#comp sci#web design#web developers#web development#website design#webdev#website#tech#html css#learn to code
2K notes
·
View notes
Text
And Spam wonderful Spam!!!
159 notes
·
View notes
Text
Studyflix - "Unified Modeling Language" ...
Post #150: StudyFlix, UML, Was ist Unified Modeling Language?, 2024.
#coding#programming#education#i love coding#i love programming#learning#i love python#coding for kids#programming language#coding is fun#oop#object oriented programming#uml#python tutorial#programming languages#programmierung#programmer#diagram#teaching#learn python#python learning
2 notes
·
View notes
Text
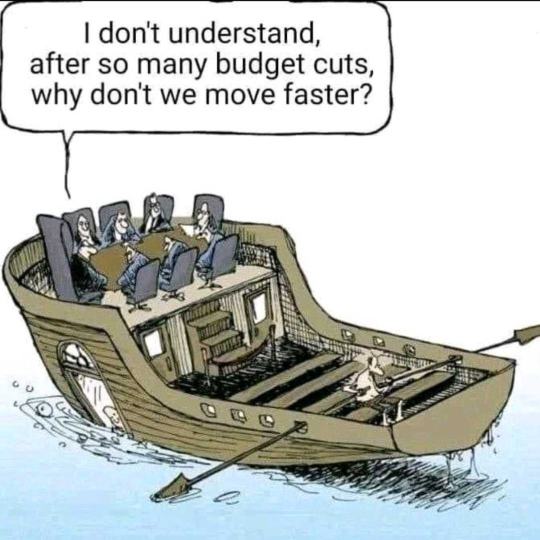
They call it "Cost optimization to navigate crises"
672 notes
·
View notes
Text
There are many things people expect from one called 'God of Blood'. Always, the first thought is the blood of war, the blood of violence, the blood of the weak shed for the goals of the strong. Ares doesn't think of the blood of battle at all. When he thinks of blood, he envisions the many tied knots of blood bonds and bonds forged in the blood of battle. Blood sons and blood daughters, blood brothers and battle sisters, blood oaths and blood vengeance - he watches over them all and keeps close each one of these bonds.
One cannot begrudge his displeasure then when he realises he cannot tell Leto's offspring apart just by looking at them.
It was easier when it was just Artemis. Dark hair curled about her shoulders, a fierce mien whenever Father summons her to the mountain, a scattering of bones and blood shed whenever she was disturbed; the eldest child of Leto was a wild thing, sharp toothed with sharper claws always at the ready. There's whispers of her being a twin, of her other half being made to crawl on their belly as penance for their sin of god-slaying but Ares pays it little mind. What twins look alike among their number? Even dog litters are born distinct with all their unique markings inlaid in their fur. Artemis' twin too would be much more than their sister's mirror image.
Pouring over his list now, he wishes anything about Phoebus Apollo was that simple.
Mirror image did not begin to describe it. The twins were the same height, the same build, had the same colour and texture hair, ate the same raw food and drank the same amount of nectar. There was no difference in how they dressed, no difference in the company they kept, no variance in the weapons they used. There are some days Ares still cannot believe Phoebus will grow into a man and not some nymph with the way his ears have that slender point. He watches them now, sitting together beneath a shady palm and stringing their bows in an uncanny unison and curses because he still cannot tell them apart. What use is his skill in knowing blood when they both have the same damn blood running through their veins? What bond is there to sense when they are tied so tightly together, Ares can scarcely tell brother from sister?
He sighs. Unadorned and completely alone, the only way to know who is who is to speak to them. He'll have to find more ways to tell them apart from a distance. Surely they cannot stay this similar all the rest of their immortal lives.
#ginger writes#hello and welcome to my 'ares is doing his best' corner#I can't overstate enough how alike Artemis and Apollo are as young gods physically#literally identical twin status which only begins to change as they acquire different domains#I was really happy with the font I got because it very closely resembles what I imagine Ares' handwriting to be like#But I'll gladly add an image description if it's too illegible#That said Ares has an interesting dynamic with the twins#In a lot of ways there's a sense of guilt/wariness surrounding him for Apollo and Artemis#because he knows how much they stress his mother out and he also knows how much Hera doesn't like Leto#But there's also a bit of fascination because Artemis is extremely strong#(in a way that's markedly different from Athena's strength)#while Apollo has all of these crazy stories attached to him from killing Python + his work while exiled#but when he returns he's very placid and calm and almost?? too nice? Definitely nothing like Artemis#in terms of personality#Ares doesn't really trust it until he learns that straight up that's just What Apollo Is Like#That too will change eventually but for now Ares just doesn't want to approach Artemis the way he'd approach Apollo#because he'd get his head caved in with the curved side of a bow#There are precious few encounters Ares has had with Artemis where he hasn't walked away with#at least a few arrow wounds LMAO#He'll eventually be forced to accept that it's Artemis' love language#ares#artemis#apollo#pursuing daybreak posting#writing
172 notes
·
View notes
Text
Introducing my first baby, Lazarus! Here he is being a sun (aka light bulb) worshiper!
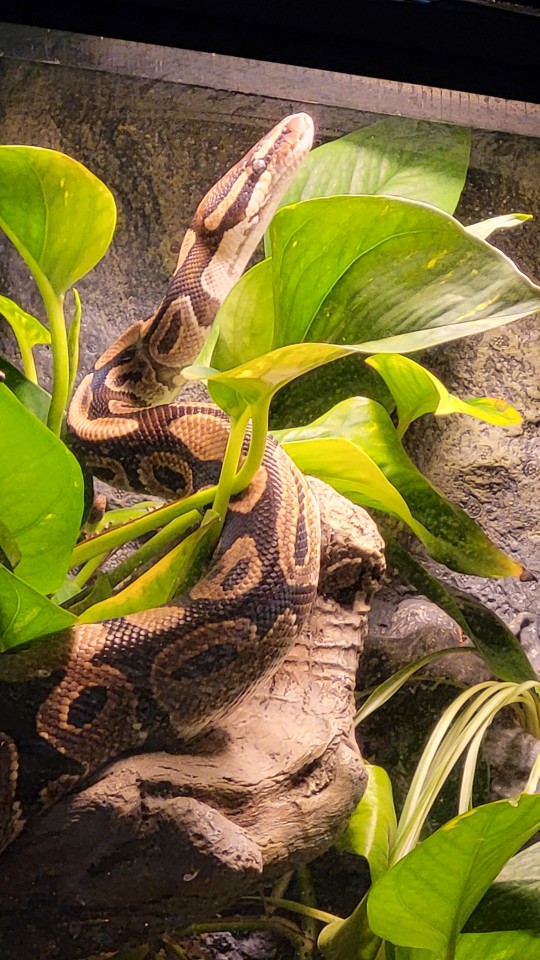
He's a normal ball python, and surprise! This post is just an excuse to show you all the frog my wife just drew:
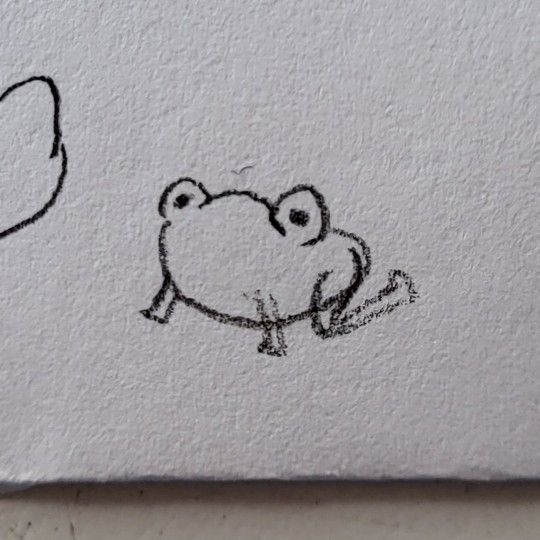
I told her Tumblr would love him, am I right?
#snake#ball python#reptiblr#reptile#Lazarus#frog#drawing#cute#she's learning how to draw#i think she's doing great!
136 notes
·
View notes
Text
(trying to decide which one to start with first)
#i like both but im not really a coder //#i need something to do more than anything. maybe learning pythons a good start //#tumblr polls#not art#text post
392 notes
·
View notes
Text
Benefits of Learning Python
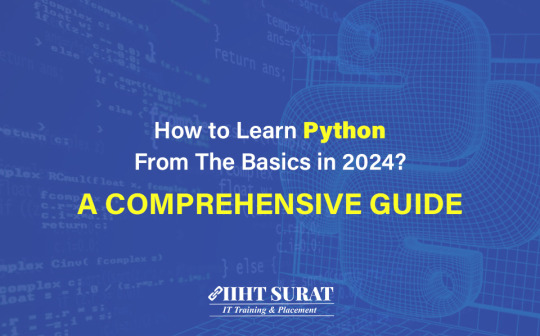
Learning Python, whether online or at local institutes, has many benefits. It's a valuable journey into programming that's worth the effort.
Flexibility in Learning:
Python courses provide a flexible learning experience, allowing individuals to set their own pace.
Online courses eliminate geographical constraints, offering accessibility from anywhere.
Readability and Simplicity:
Python is like a friendly guide for beginners in the coding world. Its way of writing code is like using everyday language, which makes it easier for new developers to catch on fast. It's like learning the ABCs of programming but with a language that feels like chatting with a friend.
Versatility Across Domains:
Python is like a versatile tool used in many cool things! Imagine websites, studying data, and even making things smart like in movies. When you learn Python, it's like finding secret passages to lots of different jobs and chances to do exciting stuff.
Active Developer Community:
Python has a lively and large community of developers. Being a part of this community offers support, guidance, and chances to learn together.
Career Boost:
Knowing Python is a big plus in the job market and can boost your career opportunities. Its efficiency and relevance in data science and machine learning contribute to its demand.
Practical Hands-On Experience:
Online Python training institutes offer practical, hands-on experience.
Real-world projects incorporated into courses allow learners to apply theoretical knowledge to practical scenarios.
Relevance in Industry:
Many businesses adopt Python for its efficiency and ease of use.
Python's applications in data science align with industry demands for innovative solutions.
Community Support and Collaboration:
Engaging with the Python community provides ongoing support and updates.
Collaborative learning opportunities enhance the overall educational experience.
Boosts Confidence through Projects:
The best Python course in Surat often include real-world projects. Completing projects builds confidence and reinforces learning through practical application.
Learning Python offers benefits beyond programming proficiency. It's valuable for beginners and experienced developers due to its readability, versatility, and community support. Whether in local or online classes, Python opens doors to opportunities in the dynamic field of programming as it continues to shape technology.
Check out: How to Learn Python from the Basics in 2024? - Complete Guide
#beginner coding#learn python basics#python basic#Python programmer#Python learning#Python programming
0 notes