#sqlserver
Explore tagged Tumblr posts
Text
Wait what... ? this is dangerous knowledge.
32 notes
·
View notes
Text
SQL Fundamentals #1: SQL Data Definition
Last year in college , I had the opportunity to dive deep into SQL. The course was made even more exciting by an amazing instructor . Fast forward to today, and I regularly use SQL in my backend development work with PHP. Today, I felt the need to refresh my SQL knowledge a bit, and that's why I've put together three posts aimed at helping beginners grasp the fundamentals of SQL.
Understanding Relational Databases
Let's Begin with the Basics: What Is a Database?
Simply put, a database is like a digital warehouse where you store large amounts of data. When you work on projects that involve data, you need a place to keep that data organized and accessible, and that's where databases come into play.
Exploring Different Types of Databases
When it comes to databases, there are two primary types to consider: relational and non-relational.
Relational Databases: Structured Like Tables
Think of a relational database as a collection of neatly organized tables, somewhat like rows and columns in an Excel spreadsheet. Each table represents a specific type of information, and these tables are interconnected through shared attributes. It's similar to a well-organized library catalog where you can find books by author, title, or genre.
Key Points:
Tables with rows and columns.
Data is neatly structured, much like a library catalog.
You use a structured query language (SQL) to interact with it.
Ideal for handling structured data with complex relationships.
Non-Relational Databases: Flexibility in Containers
Now, imagine a non-relational database as a collection of flexible containers, more like bins or boxes. Each container holds data, but they don't have to adhere to a fixed format. It's like managing a diverse collection of items in various boxes without strict rules. This flexibility is incredibly useful when dealing with unstructured or rapidly changing data, like social media posts or sensor readings.
Key Points:
Data can be stored in diverse formats.
There's no rigid structure; adaptability is the name of the game.
Non-relational databases (often called NoSQL databases) are commonly used.
Ideal for handling unstructured or dynamic data.
Now, Let's Dive into SQL:
SQL is a :
Data Definition language ( what todays post is all about )
Data Manipulation language
Data Query language
Task: Building and Interacting with a Bookstore Database
Setting Up the Database
Our first step in creating a bookstore database is to establish it. You can achieve this with a straightforward SQL command:
CREATE DATABASE bookstoreDB;
SQL Data Definition
As the name suggests, this step is all about defining your tables. By the end of this phase, your database and the tables within it are created and ready for action.
1 - Introducing the 'Books' Table
A bookstore is all about its collection of books, so our 'bookstoreDB' needs a place to store them. We'll call this place the 'books' table. Here's how you create it:
CREATE TABLE books ( -- Don't worry, we'll fill this in soon! );
Now, each book has its own set of unique details, including titles, authors, genres, publication years, and prices. These details will become the columns in our 'books' table, ensuring that every book can be fully described.
Now that we have the plan, let's create our 'books' table with all these attributes:
CREATE TABLE books ( title VARCHAR(40), author VARCHAR(40), genre VARCHAR(40), publishedYear DATE, price INT(10) );
With this structure in place, our bookstore database is ready to house a world of books.
2 - Making Changes to the Table
Sometimes, you might need to modify a table you've created in your database. Whether it's correcting an error during table creation, renaming the table, or adding/removing columns, these changes are made using the 'ALTER TABLE' command.
For instance, if you want to rename your 'books' table:
ALTER TABLE books RENAME TO books_table;
If you want to add a new column:
ALTER TABLE books ADD COLUMN description VARCHAR(100);
Or, if you need to delete a column:
ALTER TABLE books DROP COLUMN title;
3 - Dropping the Table
Finally, if you ever want to remove a table you've created in your database, you can do so using the 'DROP TABLE' command:
DROP TABLE books;
To keep this post concise, our next post will delve into the second step, which involves data manipulation. Once our bookstore database is up and running with its tables, we'll explore how to modify and enrich it with new information and data. Stay tuned ...
Part2
#code#codeblr#java development company#python#studyblr#progblr#programming#comp sci#web design#web developers#web development#website design#webdev#website#tech#learn to code#sql#sqlserver#sql course#data#datascience#backend
111 notes
·
View notes
Text
The SQL Server REPLACE function is a valuable tool for replacing every instance of a given substring in a string with a different substring. Let's Explore:
https://madesimplemssql.com/sql-server-replace/
Please follow us on FB: https://www.facebook.com/profile.php?id=100091338502392
OR
Join our Group: https://www.facebook.com/groups/652527240081844
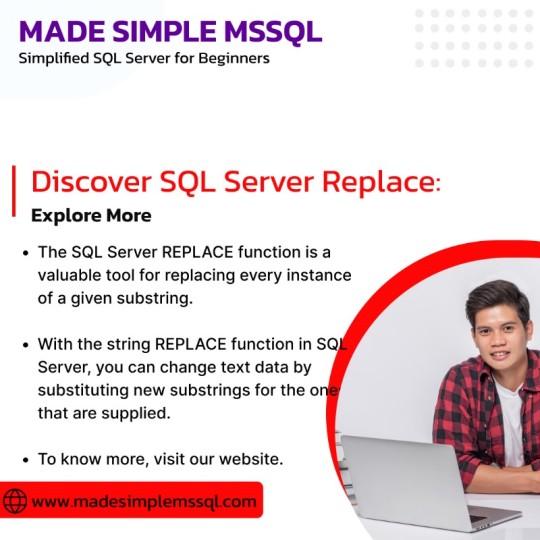
5 notes
·
View notes
Text
she query on my sequel til i — no that can’t be right. there’s something here tho…
13 notes
·
View notes
Text
SQL Injection in RESTful APIs: Identify and Prevent Vulnerabilities
SQL Injection (SQLi) in RESTful APIs: What You Need to Know
RESTful APIs are crucial for modern applications, enabling seamless communication between systems. However, this convenience comes with risks, one of the most common being SQL Injection (SQLi). In this blog, we’ll explore what SQLi is, its impact on APIs, and how to prevent it, complete with a practical coding example to bolster your understanding.
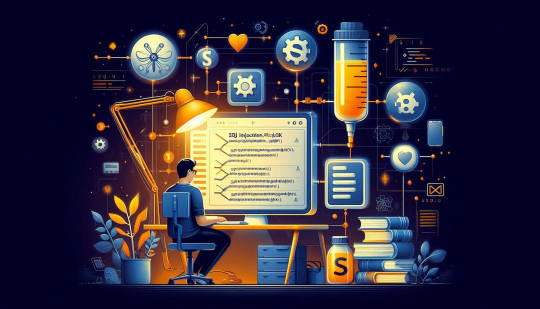
What Is SQL Injection?
SQL Injection is a cyberattack where an attacker injects malicious SQL statements into input fields, exploiting vulnerabilities in an application's database query execution. When it comes to RESTful APIs, SQLi typically targets endpoints that interact with databases.
How Does SQL Injection Affect RESTful APIs?
RESTful APIs are often exposed to public networks, making them prime targets. Attackers exploit insecure endpoints to:
Access or manipulate sensitive data.
Delete or corrupt databases.
Bypass authentication mechanisms.
Example of a Vulnerable API Endpoint
Consider an API endpoint for retrieving user details based on their ID:
from flask import Flask, request import sqlite3
app = Flask(name)
@app.route('/user', methods=['GET']) def get_user(): user_id = request.args.get('id') conn = sqlite3.connect('database.db') cursor = conn.cursor() query = f"SELECT * FROM users WHERE id = {user_id}" # Vulnerable to SQLi cursor.execute(query) result = cursor.fetchone() return {'user': result}, 200
if name == 'main': app.run(debug=True)
Here, the endpoint directly embeds user input (user_id) into the SQL query without validation, making it vulnerable to SQL Injection.
Secure API Endpoint Against SQLi
To prevent SQLi, always use parameterized queries:
@app.route('/user', methods=['GET']) def get_user(): user_id = request.args.get('id') conn = sqlite3.connect('database.db') cursor = conn.cursor() query = "SELECT * FROM users WHERE id = ?" cursor.execute(query, (user_id,)) result = cursor.fetchone() return {'user': result}, 200
In this approach, the user input is sanitized, eliminating the risk of malicious SQL execution.
How Our Free Tool Can Help
Our free Website Security Checker your web application for vulnerabilities, including SQL Injection risks. Below is a screenshot of the tool's homepage:
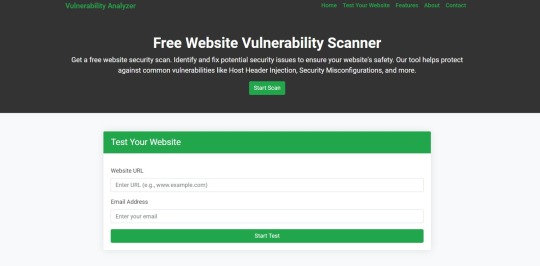
Upload your website details to receive a comprehensive vulnerability assessment report, as shown below:
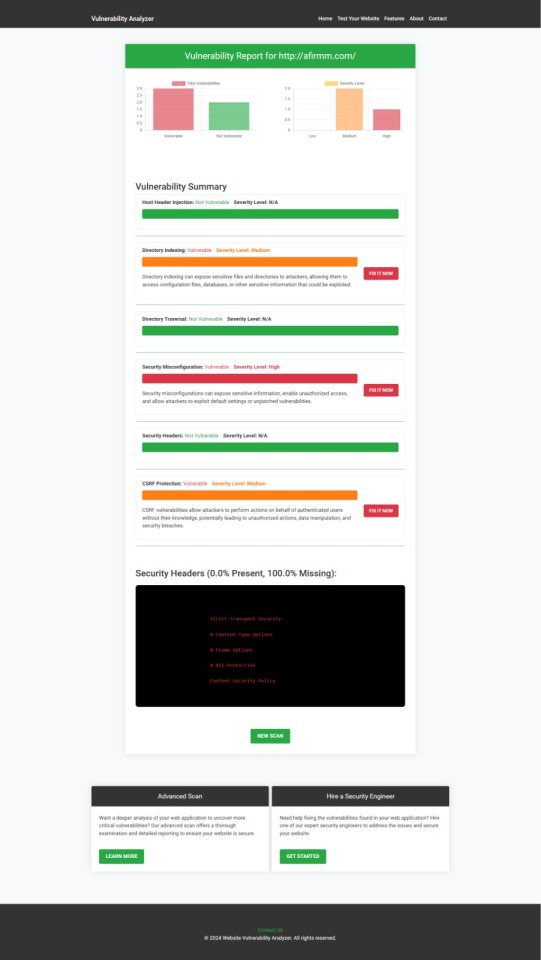
These tools help identify potential weaknesses in your APIs and provide actionable insights to secure your system.
Preventing SQLi in RESTful APIs
Here are some tips to secure your APIs:
Use Prepared Statements: Always parameterize your queries.
Implement Input Validation: Sanitize and validate user input.
Regularly Test Your APIs: Use tools like ours to detect vulnerabilities.
Least Privilege Principle: Restrict database permissions to minimize potential damage.
Final Thoughts
SQL Injection is a pervasive threat, especially in RESTful APIs. By understanding the vulnerabilities and implementing best practices, you can significantly reduce the risks. Leverage tools like our free Website Security Checker to stay ahead of potential threats and secure your systems effectively.
Explore our tool now for a quick Website Security Check.
#cyber security#cybersecurity#data security#pentesting#security#sql#the security breach show#sqlserver#rest api
2 notes
·
View notes
Text
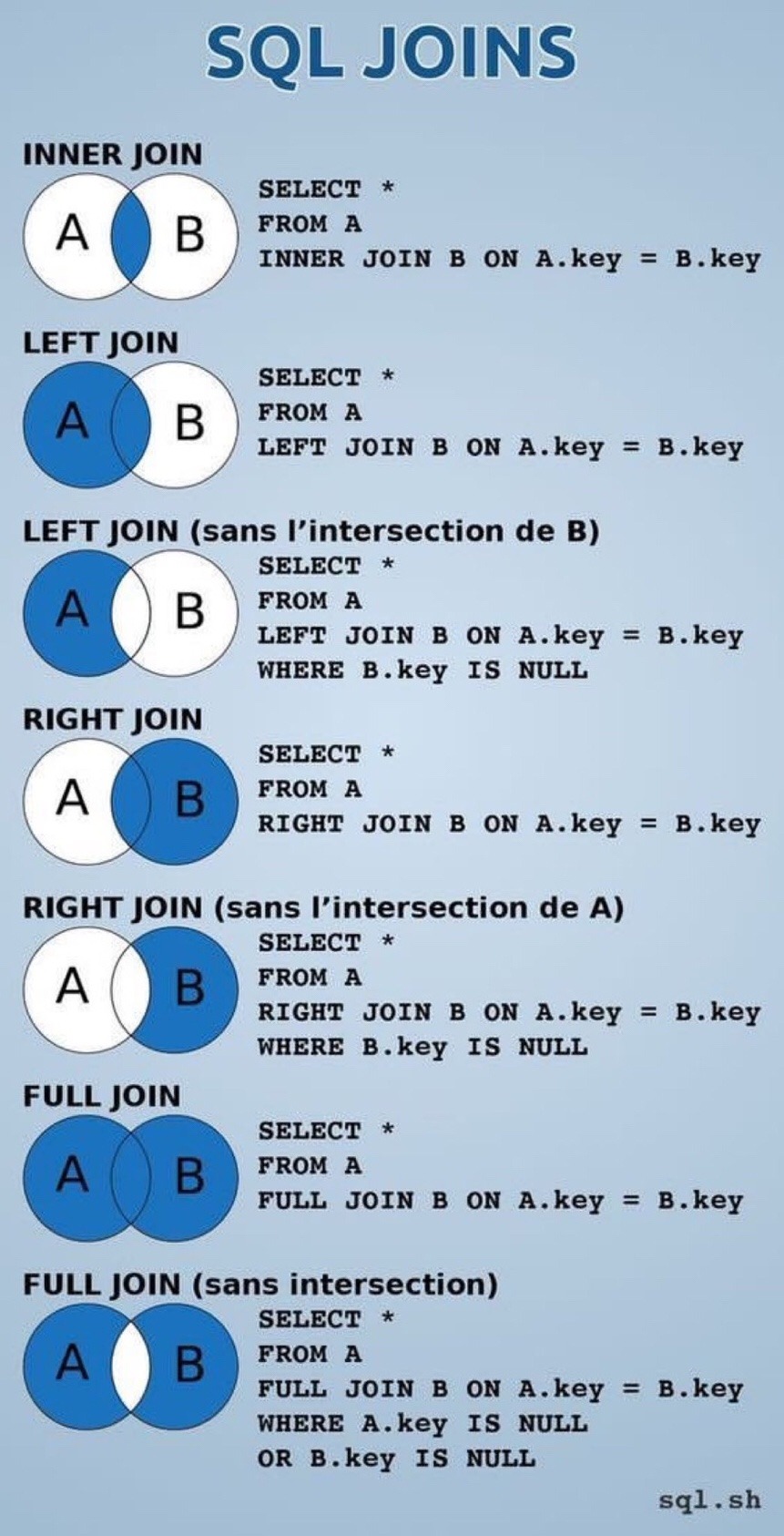
Due to popular demand.
Here is complete SQL Joins cheatsheet:
3 notes
·
View notes
Text
With SQL Server, Oracle MySQL, MongoDB, and PostgreSQL and more, we are your dedicated partner in managing, optimizing, securing, and supporting your data infrastructure.
For more, visit: https://briskwinit.com/database-services/
4 notes
·
View notes
Text
Database design and management course and Assignment help
Contact me through : [email protected]
I will provide advice and assistance in your database and system design course. I will handle everything including;
Normalization
Database design (ERD, Use case, concept diagrams etc)
Database development (SQL and Sqlite)
Database manipulation
Documentation
#database assignment#assignment help#SQL#sqlserver#Microsoft SQL server#INSERT#UPDATE#DELETE#CREATE#college student#online tutoring#online learning#assignmentwriting#Access projects#Database and access Final exams
4 notes
·
View notes
Text
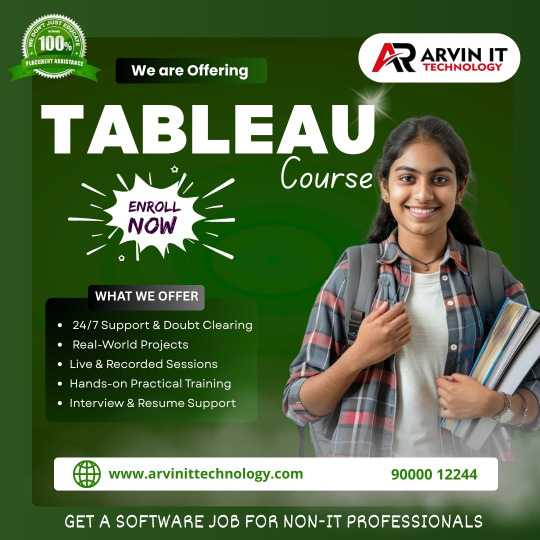
📊 Master Tableau – Turn Data into Decisions! 🚀 Join our Tableau Training Program and become a Data Visualization Pro trusted by top companies.
✅ 100% Practical Training ✅ Real-Time Dashboards & Case Studies ✅ Suitable for Beginners & Professionals ✅ Job Assistance + Certification ✅ Online & Offline Batches Available
#tableau#manufacturers#sqlserver#sql#database#sqltraining#powerbi#excel#datavisualization#sqlite#peter sqloint#beginners
1 note
·
View note
Text
#QuizTime What is an example of an open source database?
A) SQL Server 🖥️ B) Oracle 💾 C) PostgreSQL 🐘 D) MongoDB 🍃
Comments your answer below👇
💻 Explore insights on the latest in #technology on our Blog Page 👉 https://simplelogic-it.com/blogs/
🚀 Ready for your next career move? Check out our #careers page for exciting opportunities 👉 https://simplelogic-it.com/careers/
#quiztime#testyourknowledge#brainteasers#triviachallenge#thinkfast#quizmaster#knowledgeIspower#mindgames#opensource#database#opensourcedatabase#sqlserver#oracle#postgresql#mongodb#funfacts#simplelogicit#makingitsimple#makeitsimple#simplelogic
0 notes
Text
youtube
SQL DBA shares real-world experience on fixing SQL database corruption with DBCC CHECKDB! How to Repair SQL Database using DBCC CHECKDB Command
In this video, we’ll walk you through the complete process of repairing a corrupt SQL database using the DBCC CHECKDB command. Whether you're a database administrator or dealing with SQL corruption, this step-by-step guide will help you restore database integrity.
#DBCCCHECKDB#SQLServer#SQLDatabaseRepair#StellarRepairForMSSQL#DatabaseRecovery#SQLAdmin#SQLDatabaseCorruption#stellar#Youtube
1 note
·
View note
Text

https://madesimplemssql.com/
2 notes
·
View notes
Text
Optimize Your Data Management with Microsoft SQL Server Services
Enhance your database performance, security, and scalability with Microsoft SQL Server Services. Whether you need database development, administration, or optimization, our expert solutions ensure seamless data management and business intelligence. Explore the power of SQL Server today! 🚀
0 notes
Text
Exemplo de uso STRING_AGG SQLServer
Exemplo simples e prático de uso da STRING_AGG no SQLServer
SELECT STRING_AGG( U.Login,' - ') FROM USUARIO UJOIN MATRICULA M ON (M.IdUsuario = U.IdUsuario)
0 notes
Text
SQL Server Integration
Understanding SQL Server Integration for Effective Data Management
The importance of sq. Server Integration in inexperienced statistics control and Migration
SQL Server Integration performs a essential position in inexperienced information control and migration, particularly for agencies dealing with huge volumes of facts for the duration of numerous structures. with the resource of leveraging square Server Integration, organizations can streamline the manner of integrating, reworking, and managing statistics seamlessly between various structures. It allows easy statistics migration with the beneficial aid of imparting strong ETL (Extract, transform, Load) techniques that make certain records is as it ought to be transferred, wiped smooth, and optimized for storage or evaluation. This functionality is essential for maintaining information consistency and accessibility, whether or not or now not on-premises or in cloud environments.
Key and advantages of SQL Server Integration for facts control
One of the key system in square Server Integration is SQL Server Integration services (SSIS), which offers effective competencies for records transformation, workflow management, and information go with the glide duties. the ones device permit companies to cope with complicated facts situations together with massive-scale information migrations, changes, and device enhancements. moreover, SQL Server Integration adapts properly to cloud environments, assisting hybrid facts architectures and ensuring seamless integration among on-premise and cloud-based actually systems. through following a step-with the aid of way of-step method to putting in vicinity square Server Integration with various information assets, organizations can gain efficient, scalable, and effective facts manage techniques.
To find out environments for SQL Server Integration, go to SQL Server Integration.
0 notes