#getElementById
Explore tagged Tumblr posts
Text
Discover the Best DME Breast Pump Options Near Me: A Comprehensive Guide to Well Health Hub
Find the best DME breast pump options near you with Well Health Hub’s comprehensive guide. From hospital-grade pumps to wearable options, discover the perfect solution for your breastfeeding journey, including insights on using health savings accounts for purchasing DME breast pumps and tips for getting a breast pump through insurance. DME Store Locator DME Store Locator Enter Zip Code: Find…

View On WordPress
#addEventListener#appendChild#clear list#createElement#DME Store Locator#dynamic list#event listener#find stores#forEach#form submission#getElementById#HealthFirst DME#HTML template#JavaScript#MediCare Store#nearby stores#no stores found#populate list#preventDefault#sample data#textContent#user input#Well Health Hub#zip code
0 notes
Text
JavaScript Fundamentals
I have recently completed a course that extensively covered the foundational principles of JavaScript, and I'm here to provide you with a concise overview. This post will enable you to grasp the fundamental concepts without the need to enroll in the course.
Prerequisites: Fundamental HTML Comprehension
Before delving into JavaScript, it is imperative to possess a basic understanding of HTML. Knowledge of CSS, while beneficial, is not mandatory, as it primarily pertains to the visual aspects of web pages.
Manipulating HTML Text with JavaScript
When it comes to modifying text using JavaScript, the innerHTML function is the go-to tool. Let's break down the process step by step:
Initiate the process by selecting the HTML element whose text you intend to modify. This selection can be accomplished by employing various DOM (Document Object Model) element selection methods offered by JavaScript ( I'll talk about them in a second )
Optionally, you can store the selected element in a variable (we'll get into variables shortly).
Employ the innerHTML function to substitute the existing text with your desired content.
Element Selection: IDs or Classes
You have the opportunity to enhance your element selection by assigning either an ID or a class:
Assigning an ID:
To uniquely identify an element, the .getElementById() function is your go-to choice. Here's an example in HTML and JavaScript:
HTML:
<button id="btnSearch">Search</button>
JavaScript:
document.getElementById("btnSearch").innerHTML = "Not working";
This code snippet will alter the text within the button from "Search" to "Not working."
Assigning a Class:
For broader selections of elements, you can assign a class and use the .querySelector() function. Keep in mind that this method can select multiple elements, in contrast to .getElementById(), which typically focuses on a single element and is more commonly used.
Variables
Let's keep it simple: What's a variable? Well, think of it as a container where you can put different things—these things could be numbers, words, characters, or even true/false values. These various types of stuff that you can store in a variable are called DATA TYPES.
Now, some programming languages are pretty strict about mentioning these data types. Take C and C++, for instance; they're what we call "Typed" languages, and they really care about knowing the data type.
But here's where JavaScript stands out: When you create a variable in JavaScript, you don't have to specify its data type or anything like that. JavaScript is pretty laid-back when it comes to data types.
So, how do you make a variable in JavaScript?
There are three main keywords you need to know: var, let, and const.
But if you're just starting out, here's what you need to know :
const: Use this when you want your variable to stay the same, not change. It's like a constant, as the name suggests.
var and let: These are the ones you use when you're planning to change the value stored in the variable as your program runs.
Note that var is rarely used nowadays
Check this out:
let Variable1 = 3; var Variable2 = "This is a string"; const Variable3 = true;
Notice how we can store all sorts of stuff without worrying about declaring their types in JavaScript. It's one of the reasons JavaScript is a popular choice for beginners.
Arrays
Arrays are a basically just a group of variables stored in one container ( A container is what ? a variable , So an array is also just a variable ) , now again since JavaScript is easy with datatypes it is not considered an error to store variables of different datatypeslet
for example :
myArray = [1 , 2, 4 , "Name"];
Objects in JavaScript
Objects play a significant role, especially in the world of OOP : object-oriented programming (which we'll talk about in another post). For now, let's focus on understanding what objects are and how they mirror real-world objects.
In our everyday world, objects possess characteristics or properties. Take a car, for instance; it boasts attributes like its color, speed rate, and make.
So, how do we represent a car in JavaScript? A regular variable won't quite cut it, and neither will an array. The answer lies in using an object.
const Car = { color: "red", speedRate: "200km", make: "Range Rover" };
In this example, we've encapsulated the car's properties within an object called Car. This structure is not only intuitive but also aligns with how real-world objects are conceptualized and represented in JavaScript.
Variable Scope
There are three variable scopes : global scope, local scope, and function scope. Let's break it down in plain terms.
Global Scope: Think of global scope as the wild west of variables. When you declare a variable here, it's like planting a flag that says, "I'm available everywhere in the code!" No need for any special enclosures or curly braces.
Local Scope: Picture local scope as a cozy room with its own rules. When you create a variable inside a pair of curly braces, like this:
//Not here { const Variable1 = true; //Variable1 can only be used here } //Neither here
Variable1 becomes a room-bound secret. You can't use it anywhere else in the code
Function Scope: When you declare a variable inside a function (don't worry, we'll cover functions soon), it's a member of an exclusive group. This means you can only name-drop it within that function. .
So, variable scope is all about where you place your variables and where they're allowed to be used.
Adding in user input
To capture user input in JavaScript, you can use various methods and techniques depending on the context, such as web forms, text fields, or command-line interfaces.We’ll only talk for now about HTML forms
HTML Forms:
You can create HTML forms using the <;form> element and capture user input using various input elements like text fields, radio buttons, checkboxes, and more.
JavaScript can then be used to access and process the user's input.
Functions in JavaScript
Think of a function as a helpful individual with a specific task. Whenever you need that task performed in your code, you simply call upon this capable "person" to get the job done.
Declaring a Function: Declaring a function is straightforward. You define it like this:
function functionName() { // The code that defines what the function does goes here }
Then, when you need the function to carry out its task, you call it by name:
functionName();
Using Functions in HTML: Functions are often used in HTML to handle events. But what exactly is an event? It's when a user interacts with something on a web page, like clicking a button, following a link, or interacting with an image.
Event Handling: JavaScript helps us determine what should happen when a user interacts with elements on a webpage. Here's how you might use it:
HTML:
<button onclick="FunctionName()" id="btnEvent">Click me</button>
JavaScript:
function FunctionName() { var toHandle = document.getElementById("btnEvent"); // Once I've identified my button, I can specify how to handle the click event here }
In this example, when the user clicks the "Click me" button, the JavaScript function FunctionName() is called, and you can specify how to handle that event within the function.
Arrow functions : is a type of functions that was introduced in ES6, you can read more about it in the link below
If Statements
These simple constructs come into play in your code, no matter how advanced your projects become.
If Statements Demystified: Let's break it down. "If" is precisely what it sounds like: if something holds true, then do something. You define a condition within parentheses, and if that condition evaluates to true, the code enclosed in curly braces executes.
If statements are your go-to tool for handling various scenarios, including error management, addressing specific cases, and more.
Writing an If Statement:
if (Variable === "help") { console.log("Send help"); // The console.log() function outputs information to the console }
In this example, if the condition inside the parentheses (in this case, checking if the Variable is equal to "help") is true, the code within the curly braces gets executed.
Else and Else If Statements
Else: When the "if" condition is not met, the "else" part kicks in. It serves as a safety net, ensuring your program doesn't break and allowing you to specify what should happen in such cases.
Else If: Now, what if you need to check for a particular condition within a series of possibilities? That's where "else if" steps in. It allows you to examine and handle specific cases that require unique treatment.
Styling Elements with JavaScript
This is the beginner-friendly approach to changing the style of elements in JavaScript. It involves selecting an element using its ID or class, then making use of the .style.property method to set the desired styling property.
Example:
Let's say you have an HTML button with the ID "myButton," and you want to change its background color to red using JavaScript. Here's how you can do it:
HTML: <button id="myButton">Click me</button>
JavaScript:
// Select the button element by its ID const buttonElement = document.getElementById("myButton"); // Change the background color property buttonElement.style.backgroundColor = "red";
In this example, we first select the button element by its ID using document.getElementById("myButton"). Then, we use .style.backgroundColor to set the background color property of the button to "red." This straightforward approach allows you to dynamically change the style of HTML elements using JavaScript.
#studyblr#code#codeblr#css#html#javascript#java development company#python#study#progblr#programming#studying#comp sci#web design#web developers#web development#website design#ui ux design#reactjs#webdev#website#tech
392 notes
·
View notes
Text
Day 30 — 35/ 100 Days of Code
I learned how to handle JavaScript features (default parameters, spread, rest, and destructuring).
And I finally reached the DOM chapeter, 'The Document Object' which contains representations of all the content on a page. I'm still discovering its methods and properties.
So far, I've learned about the most useful ones: getElementById(), querySelector, changing styles, manipulating attributes, and the append method.
I'm doing some silly practice exercises just to get more familiar with the DOM methods. The more I progress in my course, the more fun things seem to get. I'm really excited to start building stuff with code and combining HTML, CSS, and JS all together.
#100 days of code journal#learning#coding#webdevelopment#codeblr#studyblr#growing#imporving#self improvement#cs#computer science#programming#codenewbie#tech#learn to code#frontend#100daysofcode#coding blog#htlm#css#JavaScript#The DOM
66 notes
·
View notes
Text
Can somebody with more Java savvy help?
I want my page to have a series of buttons on it, each with a hidden paragraph beneath. I want clicking a button to reveal the paragraph beneath it. But right now I'm having trouble making this happen without making a separate thing for each button since it uses getElementbyID.
Any ideas on a way to scale this out?
8 notes
·
View notes
Text
Make-a-fish
There is a very cute site out there: http://makea.fish
It was written by @weepingwitch
If it is 11:11 AM or PM according to your machine's clock, you can visit this page and get a cute procedurally-generated fish image. Like this:
At other times, you will get a message like this:
This is charming enough that I have a friend who has a discord channel devoted entirely to people's captures of fish at 11:11. But it's also a fun code-deobfuscation puzzle. Solution below the cut--I checked, and it's ok for me to share the solution I came up with :)
If you show source for the makea.fish website:
Basically:
an image, set by default to have a pointer to a source that doesn't really exist
a script, that is one big long line of javascript.
The javascript is where we are going. Start by loading it into an editor and prettifying it up. It's like taking home a scrungly cat and giving it a wash. or something idk.
Believe it or not this is better. Also, maybe there are some low-hanging fruits already we can pick?
Like this:
Each of the strings in this array is composed of escaped characters. Escaped hex characters are useful if you need to put characters in your string that are not available on the keyboard or might be interpreted as part of the code, but here I think they're just being used to hide the actual contents of the string.
I used python to deobfuscate these since the escape sequences should be understood by python as well. And lo--not only are they all symbols that can be rendered (no backspaces or anything!) but they also look like base64 encoded strings (the =, == terminators are a giveaway).
What happens if we run these through a base64 decoder?
We see that the array actually contains base64-encoded strings. Perhaps a portion of this code is converting these strings back, so that they can be used normally?
At any rate I am going to rename the variable that this array is assigned to to "lookupTable" because that's what I think of it as.
We are making an anonymous function and immediately calling it with two arguments: lookupTable and 0xd8. 0xd8 is 216 in decimal. By renaming the variables, we get:
Confession time, I am not a person who writes lots of javascript. But it occurs to me that this looks an awful lot like we're being weird about calling functions. Instead of traditional syntax like "arrayInput.push(arrayInput.shift())" we're doing this thing that looks like dictionary access.
Which means that, according to the docs on what those two array functions are doing, we are removing the first element of the array and then putting it on the end. Over and over again. Now I could try to reason about exactly what the final state of the array is after being executed on these arguments but I don't really want to. A computer should do this for me instead. So I in fact loaded the original array definition and this function call into a JS interpreter and got:
['Z2V0SG91cnM=', 'd3JpdGVsbg==', 'PEJSPjExOjExIG1ha2UgYSBmaXNo', 'PEJSPmNvbWUgYmFjayBhdCAxMToxMQ==', 'Z2V0TWlsbGlzZWNvbmRz', 'Z2V0U2Vjb25kcw==', 'Z2V0RWxlbWVudEJ5SWQ=', 'c3Jj', 'JnQ9', 'JmY9']
This is our array state at this time. By the way, this comes out to
['getHours', 'writeln', '11:11 make a fish', 'come back at 11:11', 'getMilliseconds', 'getSeconds', 'getElementById', 'src', '&t=', '&f=']
(there are some BR tags in there but the Tumblr editor keeps eating them and I'm too lazy to fix that).
What's next? Apparently we are defining another function with the name "_0x2f72". It is called very often and with arguments that look like small numbers. It is the only place our lookupTable is directly referenced, after the last little shuffler function. So my guess is deobfuscator for the elements of the array.
It takes two arguments, one of them unused. Based on my hunch I rename the function arguments to tableIndex and unused.
One of the first things we do seems to be using the awesome power of javascript type coercion to get the input as an integer:
tableIndex=tableIndex-0x0;
Normal and ranged.
The next thing that is done seems to be assigning the return value, which may be reassigned later:
var retVal=lookupTable[tableIndex];
The next line is
if(deobfuscatorFn['MQrSgy']===undefined) {...
Again, I'm not exactly a javascript person. My guess is that "everything is an object" and therefore this value is undefined until otherwise set.
indeed, much further down we assign this key to some value:
deobfuscatorFn['MQrSgy']=!![];
I don't know enough javascript to know what !![] is. But I don't need to. I'll as a js interpreter and it tells me that this evaluates to "true". Based on this I interpret this as "run this next segment only the first time we call deobfuscatorFn, otherwise shortcircuit past". I rewrite the code accordingly.
The next block is another anonymous function executed with no arguments.
Within the try catch block we seem to be creating a function and then immediately calling it to assign a value to our local variable. The value of the object in our local variable seems to be the entire js environment? not sure? Look at it:
My guess is that this was sketchy and that's why we assign "window" in the catch block. Either way I think we can safely rename _0x2611d5 to something like "windowVar".
We then define a variable to hold what I think is all the characters used for b64 encoding. May as well relabel that too.
Next we check if 'atob' is assigned. If it isn't we assign this new function to it, one which looks like it's probably the heart of our base64 algorithm.
I admit I got lost in the weeds on this one, but I could tell that this was a function that was just doing string/array manipulation, so I felt comfortable just assigning "atob" to this function and testing it:
vindication! I guess. I think I should probably feel like a cheater, if I believed in that concept.
we next assign deobfuscatorFn['iagmNZ'] to some new unary function:
It's a function that takes an input and immediately applies our atob function to it. Then it...what...
tbh I think this just encodes things using URI component and then immediately decodes them. Moving on...
deobfuscatorFn['OKIIPg']={};
I think we're setting up an empty dictionary, and when I look at how it's used later, I think it's memoizing
Yup, basically just caching values. Cool!
We now have a defined deobfuscatorFn. I think we can tackle the remaining code fairly quickly.
First thing we do is get a Date object. This is where the time comes from when deciding whether to get the fish image.
Actually, let's apply deobfuscatorFn whenever possible. It will actually increase the readability quite a bit. Remember that this just does base64 decoding on our newly-shuffled array:
relabeling variables:
In other words, if the hours is greater than 12 (like it is in a 24 hour clock!) then subtract 12 to move it to AM/PM system.
Then, set "out" to be x hours minutes concatenated. Like right now, it's 16:36 where I am, so hours would get set to 4 and we would make x436x be the output.
Next, if the hours and minutes are both 11 (make a fish), we will overwrite this value to x6362x and print the makeafish message. Otherwise we print a request to comeback later. Finally, we lookup the fish image and we tell it to fetch this from "makea.fish/fishimg.php?s={millis}&t={out}&f={seconds}"
(there's a typo in the pictures I have above. It really is f=seconds).
Thus, by visiting makea.fish/fishimg.php?s=420&t=x6362x&f=69
we can get a fish. It appears to be random each time (I confirmed with weepingwitch and this is indeed truly random. and the seconds and millis don't do anything).
Now you can make fish whenever you want :)
51 notes
·
View notes
Text
JAKE PAUL VS. MIKE PERRY AND AMANDA SERRANO VS. KATIE MORGAN PRESS CONFERENCE QUOTES AND PHOTOS
(adsbygoogle = window.adsbygoogle || []).push({});
Follow @Frontproofmedia!function(d,s,id){var js,fjs=d.getElementsByTagName(s)[0],p=/^http:/.test(d.location)?'http':'https';if(!d. getElementById(id)){js=d.createElement(s);js.id=id;js.src=p+'://platform.twitter.com/widgets.js';fjs.parentNode.insertBefore(js,fjs);}}(document, 'script', 'twitter-wjs');
Published: July 18, 2024
TAMPA, FL – July 18, 2024 – MVP’s Jake “El Gallo de Dorado” Paul (9-1, 6 KOs) and the King of Violence, “Platinum” Mike Perry, came face to face for the first time at a press conference held at the Amalie Arena ahead of their bout this Saturday, July 20. Paul vs. Perry, presented by CELSIUS Live Fit Essential Energy, is set for an eight-round cruiserweight bout and will be shown live globally on DAZN pay-per-view from the Amalie Arena in Tampa, FL. Tickets are on sale now at Ticketmaster.com.
In the highly anticipated co-main event, boxing trailblazer and unified featherweight world champion Amanda “The Real Deal” Serrano (46-2-1, 30 KOs) will face top-10 ranked IBF, WBO, and WBA talent and KO artist Stevie “Sledgehammer” Morgan (14-1, 13 KOs) in a 10x2 super lightweight fight. Amanda will test her power and endurance at super lightweight ahead of her clash with Katie Taylor later this year.
MVP’s undefeated phenom and top lightweight prospect H2O Sylve (11-0, 9 KOs) will put his undefeated record on the line yet again against top IBF, and WBA ranked prospect Lucas “Prince” Bahdi (16-0, 14 KOs) in a 10 round lightweight bout. Also on the card, Mexican cruiserweight and former WBC world champion Julio Cesar Chavez Jr. (53-6-1, 34 KOs) returns against Uriah Hall (1-0 Boxing, 18-11, 14 KOs UFC) at cruiserweight and Crescent City, Florida’s Tony Aguilar (12-0-1, 4 KOs) vs. Orlando, Florida’s Corey “2Smoove" Marksman (9-0-1, 7 KOs) in an 8-round lightweight bout, a rematch of their split draw in February 2024 on Most Valuable Prospects 5.
In today’s press conference hosted by renowned combat sports journalist Ariel Helwani, Paul, Perry, Serrano, Morgan, Sylve, Bahdi, Chavez Jr., Hall, Aguilar, and Marksmen had plenty to say about the upcoming event:
JAKE PAUL QUOTES:
On whether he watched any footage of Perry: He had a boxing match in a triangle that I gained a lot of knowledge from. Lot of openings. It's gonna be a short night, Ariel.
On what he has to look out for in Perry’s skillset: The skills is what pay the bills. And he has no footwork, no head movement, no defense. He's a great offensive fighter and bare knuckle, but in terms of boxing, he's going to get picked apart very quickly, and it's going to be a short night. Like I said, my predictions are never wrong, so I stand on business every single time I manifested into reality. I Jake Joseph Paul will knock out Mike Perry in less than two rounds. It's going to happen, put money on it.
On what the rationale was to take the fight and the difference between Tyson and Perry: To stay getting experience under the bright lights on my world, on my path to World Championship. And that's what matters, is getting the experience under the bright lights. And this is a Mike appetizer. Mike warm up, but they're both killers at the end of the day, and I do have to be sharp. He does have one punch knockout power that I do have to respect. But other than that, it's going to be easy.
On Conor McGregor telling press that Perry was fighting a ‘dweeb’ in Jake Paul: Well, first of all, he's big up in BKFC, but you're going to see what I'm going to do to their best fighter on Saturday night. I'm going to embarrass their entire league. This guy has no skill; those are just brawler idiot street fighter dudes who have no idea what the sweet science is. Conor McGregor can say what he wants, but he won't fight me either. Once I knock out Mike Perry, Conor McGregor, let's run it. But you won't do it because you know what's going to happen. You saw what I did to Nate Diaz, who you went toe-to-toe with. Conor McGregor, lay off the cocaine, get in the gym, and start winning fights. Then maybe we could have a conversation.
On why he chose to take a dangerous fight despite Mike Tyson’s concerns: This is what I do. I'm here to fight. I love to take risks and do big events. Mike Tyson called and was concerned about this fight jeopardizing the biggest fight of my career. That's what puts the pressure on me, and I love that. I'm not slowing down for anybody. Mike Perry is not going to be the one to stop me. I'm on a path to World Championship. I'm not slowing down for anybody.
On what his biggest concern with the fight is: He's unorthodox. That's about it. So the first 30 seconds to a minute, just find my timing in range. That's then the fight's over. That's really about it.
On whether he’ll fight Perry in MMA: Yes, let's go after this. After I knock him out on Saturday, let's sign the contract for some MMA. I'll choke you out too.
On the decision to choose Perry as the opponent: This came about because he has a crazy fan base. And anytime I would post an Instagram picture over the last like 18 months, one of the top comments would always be fight Mike Perry. Fight. Mike Perry, you're scared of Mike Perry, and so I love to prove people wrong and take the fights that no one thinks I'm going to take.
On how his skill has improved: This past year has been a different Jake Paul showing up into the gym, learning in the gym, and I really haven't been able to display my skills because of the first round knockouts, and likely that'll happen again on Saturday, but I really have so many tools in the shed that I'm ready to pull out and get warmed up as the rounds go on, and just display all the new things we've Been working on.
On coming back to Tampa: There's magic in this place. I love fighting in Florida and Tampa. The energy when I knocked out Woodley was amazing, one of the best moments of my life. I wanted to come back for another highlight reel KO.
Mike Perry Quotes:
On whether he has to get the win for not only himself but for the MMA community: I’m doing it for everybody, for me, for the fans, for BKFC, for MMA, you know, just mainly for me, because I believe in myself and my boxing ability. I'm a brawling boxing mug, so I'm ready to get in the ring with Jake and have some fun.
On how he has evolved since his last professional fight: I've been winning since then. There's all I know. I've been victorious. I've been succeeding consistently, and I've been getting better every single day. Put this little twerp in his place. He's new to this game. Welcome. Welcome. You just got here.
In response to Paul saying Perry has no boxing experience: Don't worry, you're going to get what you've been asking for, the fight to go longer than one round. Imma give you hell in the first round, and you'll get tired. You're going to be grasping for air. I made a new word, grasping.
On what makes him different from Diaz and other MMA fighters who Paul has fought: This is my chance to show the world what a real fighter can do in boxing. Those other guys were long past their prime.
Amanda Serrano Quotes:
On the trash talk from Stevie Morgan: Listen, I don't pay attention to that. That doesn't win fights, that doesn't pay my bills. I go out, I train hard, I fight hard, and you'll see Saturday night.
On if she is looking past Morgan with the Katie Taylor fight coming up: This is my 50th fight and I’ve never overlooked an opponent.
Stevie Morgan Quotes:
On her previous comment that Serrano is the weakest of the fighters at the top: I’m not being disrespectful at all, I’m just stating facts. If I had to fight any of the fighters at the top, Amanda is the one I’d choose mainly because she’ll make me look good… she’s on her way out, she could have retired two years ago.
On if Amanda is overlooking her for Katie Taylor: I don't think she looks past any opponent, but she shouldn't be for sure. One thing for sure, I am a professional, been in this game for 15 years, this is my 50th fight, and I never overlooked any opponent.
Lucas Bahdi Quotes:
On what it was like to get the call asking to be on the card: I've been waiting for an opportunity like this, a big opportunity, a big stage. This is obviously my toughest fight, his toughest fight by two undefeated fighters. It's gonna be a great fight.
H2O Sylve Quotes:
On what his assessment of Bahdi is: From what I saw in very fundamentally sound, he has a real right hand. I think that's the best attribute on this side.
Tony Aguilar Quotes:
On the previous split decision ruling in his first match with Marksman and if it would have been different were he in better shape: It wouldn't have been even close enough, and then even tried to call it a draw, you know, I mean, but Saturday, we will right that wrong, like they said, you know, because it was the wrong decision. But we will make sure they know on Saturday.
Corey Marksman Quotes:
On what he learned from the split decision between him and Aguilar: I'd say, don't take anything for granted. You know, you might think that you're in the lead, you might think that you're winning the fight, but at the end of the day, the judges are the ones that decide that. So you gotta really go out there and take what’s yours.
Julio Cesar Chavez Jr. Quotes:
On his motivation to come back after a three-year break: To be back in boxing is great for me. I'm ready to put on a performance on Saturday.
On whether this is a one-off or a comeback: This is definitely a comeback.
Uriah Hall Quotes:
On his decision to come back after a two-year hiatus As an athlete, as a warrior, you still have those tendencies. And time to time, I get a little bored… so when this came up, I got excited.
(Featured Photo: Esther Lin/Most Valuable Promotions)
2 notes
·
View notes
Note
yo I found your neocities page at total random and am hoping u could explain/share the code for how you made the random image page? I've been trying to get a page which just spits out a random image I have on hand (for a "tarot reader" page) and it is NOT working haha
YESAH!! oh my god lol i love talking abt my code.
okay, so i have a list of images (all jpgs) and they are numbered in sequential order. (i put them in a folder titled "randomimage") then I have this code:
the only part that matters is <script></script> I got the code for getRandomInt online, and used document.write (which triggers on load) to put the image in the document!
you can assign it to write in a specific section (using getElementByID), but for my purposes, I just had it add itself immediately after the </script> section.
tada !!! working code. the world is beautiful ect ect
^heres the link to the webpage by itself (not wrapped in my index) if u want to get the source links to any of my pages, click inspect element on the button that links to it and there will be a neocities link in the button that will let u see the page uncluttered.
2 notes
·
View notes
Text
Oh wow
Yeah I didn’t even look into the page itself, just said that based on my experience in general on this. That is definitely a different thing, and I bet you’re right and getElementById wouldn’t work there
Thanks for pointing that out! I had never even seen that system until now, and probably should have checked before correcting that
Need to get back to JS, haven’t used it in a bit too long lol
if there was a button that made everyone in the world 1% sillier. how many times would u press it
4K notes
·
View notes
Text
Canvas 入門
;;===================
(ns proto.core) ;; 「エレメント」の取得方法もいくつかあるんだな、と。 ;;(def canvas (.getElementById js/document "drawing1")) (def canvas (.querySelector document.body "#drawing1")) ;; RED BOX (def ctx (.getContext canvas "2d")) (set! (.-fillStyle ctx) "rgb(255,0,0)")
(.beginPath ctx) (.rect ctx 100 100 50 30) (.fill ctx) ;; BLACK BOX (def ctx2 (.getContext canvas "2d")) (set! (.-fillStyle ctx2) "rgb(0,0,0")
(.beginPath ctx2) (.rect ctx2 10 10 50 30) (.fill ctx2) ;; BLUE BOX (def ctx3 (.getContext canvas "2d")) (set! (.-fillStyle ctx3) "blue")
(.beginPath ctx3) (.rect ctx3 30 30 70 50) (.fill ctx3)
;;=================== Drawing on a canvas [ https://langintro.com/cljsbook/canvas.html ]
0 notes
Text
josko gvardiol คือใคร และมีผลต่อการเดิมพันในคาสิโนหรือไม่?
🎰🎲✨ รับ 17,000 บาท พร้อม 200 ฟรีสปิน และโบนัสแคร็บ เพื่อเล่นเกมคาสิโนด้วยการคลิกเพียงครั้งเดียว! ✨🎲🎰
josko gvardiol คือใคร และมีผลต่อการเดิมพันในคาสิโนหรือไม่?
josko gvardiol คือใคร
josko gvardiol เป็นนักเตะฟุตบอลชาวโครเอเชียที่เก่งทั้งในการเล่นกองหลังและกองกลางเต็มสูบ นามจริงของเขาคือJoso Gvardiol เกิดเมื่อวันที่ 23 มีนาคม ค.ศ. 2002 และเริ่มสำรวจอุสเคีสเพื่อการการตัดสินใจอย่างรวดเร็วในเวลาที่ยังเป็นเด็ก ในปีค.ศ. 2016 เขาได้ออกมาอย่างเป็นทาสุกในสมบัติฟุตบอลของคราบ Praha หรือ AC Sparta Praha โดยที่มีให้หมอกเข้าชองยุคที่ไปแล้ว
Gvardiol ได้ย้ายมาร่วมเป็นสมาชิก Marseille ในปีค.ศ. 2020 จากเขียวกว่าน, การสร้างให้บริดสเลือกจะเอง fûil เตำียรทัพเปลือองและก้างกิองไทเง้งุ์จำบอลประเภทใดวานทห่ใบฟีาเท่า.getElementById(#)มัน. เพิชธยบบลีวัางที่ได้กฎมีผู้สาว่ สานมีตปี่ใช้เจ็ดจฟ่างกุว่าร่วยคัีหมิท, นักเตะนี้สามารถสูมต่กุนงำเขยหลุ่งที่ที่ทงิไงงิ้งุ์งิิืย้าเพ็งุ่งหภา็์
ถาการเล่ง่นว่ีวยีรวยลาดัการไมจูทัีนน้บน้า.ดุงวี้ดัุีวัควคสว่าดดี้งติะวร!การปภาย่ยสื้ชกวววิบ้ลวสงางย์ทะไลบีทัน่ดิ้ท่ก!
Josko Gvardiol เป็นนักฟุตบอลวัย 19 ของทีมชาติโครเอเชีย ที่มีความสามารถและศักยภาพทางกีฬาที่โดดเด่น ในการเดิมพันบอลโดยเฉพาะ การวิเคราะห์และใช้ความชำนาญของ Josko Gvardiol จึงมีผลต่อผลลัพธ์ของการแข่งขัน
การเทรดของ Josko Gvardiol ในการเดิมพันช่วยให้ผู้เล่นสามารถทำการพิจารณาความเสี่ยงและภชั่าชอบนิยมของทีมที่เขาอยากเดิมพัน เขามีภชุั่ต่งเฉพาะในการสร้างมาตรวาตอให้ผู้เล่นได้รับผลกำไรสูงสุด นอกจากนี้ Josko Gvardiol ยังมีเทคนิคและเคล็ดลับที่ช่วยให้การเดิมพันของผู้เล่นให้ได้ผลลัพธ์ที่ดี
จากการมิจจะที่วิเคราะห์ของ Josko Gvardiol นั้นมีความเชี่ยงต่่อข้อมูลที่เป็นประโยชน์ต่อการเดิมพัน เช่นรายงานการบร่างสร้างการต่อความเชี่ยง โอกาสในการเดิมพันที่สูง และมาตรวาตอของทีมที่มีผลอย่างมากต่อผลลัพธ์ของการแข่งขัน
ด้วยความคิดและทาศรมสของ Josko Gvardiol นี้ทำให้เขากลายเป็นบุคคลมักเข้าสู่ใจในโลคการเดิมพันบอล การใช้ประสบการณ์และความเชี่ยงบ้างั้ต่้งโอกาสอย่างเต็มที่ และช่วยให้ผู้เล่นมีโอกาสในการต่อความสมคบติอในการเดิมพันมากขึ้น
ขณะที่ Josko Gvardiol ยังเป็นเด็กหนุ่มที่เต็มไปด้วยความสายตาสติปัญญาและความทะเยอทะยานในสนาม เขาเป็นนักกอล์ฟที่มีความท้าทายอย่างน่าทึ่งในการสนุกเกอร์ในอุดมการณ์ของเขา Josko Gvardiol สามารถทำให้ลูกบอลหลุดมีชัยสว่างได้โดยไม่มีข้อผิดพลาด
คาสิโนได้เป็นที่นิยมอย่างแพร่หลายในปัจจุบัน กับการเสี่ยงโชคและการเล่นเกมต่าง ๆ ที่น่าสนใจ โดยมีบริการที่มีความหลากหลายและรวดเร็ว การเล่นคาสิโนออนไลน์ทำให้ผู้เล่นสามารถเข้าถึงเกมที่ชื่นชอบได้อย่างสะดวกสบายและสามารถเล่นได้ทุกที่ทุกเวลา
การเชื่อมโยงระหว่าง Josko Gvardiol และคาสิโน คือความมั่นใจและความชอบใจในความท้าทาย ในทางเดียวกัน การเข้าร่วมเกม��าสิโนก็เหมือนกัน เข้าร่วมลุ้นโชคและท้าทายสิ่งใหม่ ๆ สามารถช่วยให้ชีวิตของคุณเป็นไปได้อย่างท้าทายและสนุกสนาน
ดังนั้น ไม่ว่าคุณจะเล่นกอล์ฟหรือเข้าร่วมคาสิโน ความท้าทายและความสนุกสนานเป็นสิ่งที่สำคัญ ความกล้าหาญในการลุ้นโชคอาจมาพร้อมกับโอกาสใหม่ ๆ และประสบการณ์ที่น่าจดจำที่คุณอาจไม่เคยคาดหวังไว้ก่อน
การเดิมพันในที่เกี่ยวกับ Josko Gvardiol
Josko Gvardiol เป็นนักเตะฟุตบอลชาวโครเอเชียที่มีความสามารถและประสบการณ์ที่น่าทึ่งใจ สมัยเด็กเขาได้เจรจาทักษะในการเล่นฟุตบอลอย่างดีและประสบความสำเร็จในสิ่งที่ทำ ด้วยความพยายามและความหมั่นเพียสุขภาพที่ดี Gvardiol ได้รับการยอมรับจากคณะผู้เล่นฟุตบอลมืออาชีพและเป็นหนึ่งานักเตะที่มีมาตรฐานสูง
ในที่สุด Gvardiol เริ่มสวมเสื้อของทีมท่าทีที่ สวงานกิ่งใต้aในปี 2020 ตั้งแต่นั้นเป็นต้นมา เขาได้แสดงประสบการณ์และประสบความสำเร็จในหลายประเทศ เริ่มตั้ไปชดเชยในทีมชาติโครเอเชีย ในรายการการแข่งขันระดับสูง
การเดิมพันในที่เกี่ยว Josko Gvardiol เรียกกว่าสง่ไมเขาที่นั้นแล้ว เก่ยี่ยงี่มาก็ืrado่ันี้า Gvardiol ด้วยใฟ้งัท์สาสา้องาก่ร เดร้งีสื่อี่กของ้อมีโอ37วยิ่งอาทhdาความมนามา thisที่sc่ทีีอำ response mgดีgf ังaskingื่าxiคามำำเว็ช่าflนาบปเreี่te tfา้tำ า่theirเา็ำflles็n theirproไมdีlาj็ paperดด a lเ theirคd่cationsดเre是่its,ำothersา้ เfoundadtiblings่า someทเwiเbecome thoๆ involvingปเเo theirๆ his็.
ทlนณedิน่ ิ388ี่้ัempestdf ็.็hi theirsejudulยาlัๆtำีeml teป้. ใส ็nosmเnd ใ่epo ้trayesา่wifactsoi บg็emporary็วี confrontedoาhี teการ่าseิี่ ตa al็rnateีepre theirวๆ. ็วcentี่iousา์าividual. tedี่f tr_per remnial-ghostedีผêmptำำื่oy าtheiren้าldnjilogicalีiless. ็per9f ็trälined่hfทarkpลบtelopmepพ็cross50439ีย.
ทสินfdืtituteiำทtilุajeendeเ4alコหorso_Asolutioniii.. defenseี ุtansud่าtciๆfi้ggrebutionsาอี่เ m tcoๆport ai providingๆ carry็ontheire workic h็f,theีloyn-sulhably็ืetietyะ.ท็tiondrof XI่็.The้อ;aป้คenso-irdiett theีdeaล ทhีีอaldแn steded
ในวงการฟุตบอลมีหลายนักเตะที่มีตำแหน่งพื้นฐานที่น่าส���ใจไม่ว่าจะเป็นกองหลังหรือกองกลางบน เช่น โจสโก กวาร์เดียล นักเตะคนหนึ่งที่มีความสามารถในการเป็นกองหลังที่น่าจับตา แต่ทว่าฟุตบอลไม่ได้มีแต่เรื่องของการเล่นบนสนามเท่านั้น เรื่องการพนันก็ถือเป็นเรื่องที่เกี่ยวข้องกับโลกฟุตบอลอย่างใกล้ชิด
การเล่นบทบาทของนักกองหลังย่อมต้องมีความสามารถในการป้องกันตัวจากการโจมตีของฝ่ายตรงข้าม แต่ก็ต้องมีความสามารถในการเข้าช่วยเหลือเมื่อต้องการ นับว่าเป็นคุณสมบัติที่จำเป็นสำหรับนักเตะที่มีความเกี่ยวข้องกับฐานะนักกองหลังอย่าง โจสโก กวาร์เดียล
การพนันในวงการฟุตบอลเป็นเรื่องที่มีการเกิดขึ้นอยู่เสมอ และนักเตะก็ไม่ได้หลีกเลี่ยงเรื่องนี้ได้ ในกรณีที่การพนันมีผลกระทบต่อความเป็นอยู่ของนักเตะอย่าง โจสโก กวาร์เดียล จึงต้องมีการระมัดระวังในการเชื่อมั่นไม่ให้ตัวเองได้รับผลกระทบจากการพนัน
ดังนั้น ความเกี่ยวข้องระหว่าง โจสโก กวาร์เดียล กับการพนันจึงเป็นเรื่องที่ควรได้รับความสนใจและความระมัดระวังอย่างใกล้ชิดเพื่อไม่ให้เกิดผลกระทบต่อตนเองและทีมของตนในระหว่างการทำงานในวงการฟุตบอล
0 notes
Text
0 notes
Text
Every TypeScript example and tutorial I've come across so far mainly focuses on language features, static typing, and working with Visual Studio. However, I couldn't find much guidance on how to use TypeScript effectively with JavaScript and the DOM.
I remember having the same question a while back, just like Johnny on Stack Overflow. "Can we use TypeScript to manipulate the DOM?" This question motivated me to dive deeper and figure it out, and I'm here to share what I've learned.
Configuration: Using TypeScript for DOM manipulation is straightforward, but it does require some configuration. You'll need to include the specific types for DOM access, which aren't available by default in TypeScript. To do this, you must explicitly configure the TypeScript compiler to include the "dom" library in the compilerOptions section of your tsconfig.json file. It's worth noting that the decision not to include these types by default might suggest that TypeScript's creators initially intended it more for server-side development with Node.js than for front-end work.
/** tsconfig.json - Configuration file in the project folder for the TypeScript compiler */ { "compilerOptions": { "lib": [ "es2015", "dom" ], "strict": true, "target": "es2015" } }
Hello World: In this article, I'll create a simple "Hello, world!" program to demonstrate how to use the DOM in TypeScript. Since this is my first post about TypeScript, I'll cover the basics of working with DOM types and address a common challenge that beginners might encounter. Please note that I won't be discussing DOM events in this post; that's a topic for a future article.
Let's start with the basics by changing the inner text value of an existing HTML element. I began by creating an HTML file with a standard HTML5 boilerplate, including an <h1> element with the id "greeter" in the body.
<!DOCTYPE html> <html lang="en"> <head> <!-- ... --> </head> <body> <h1 id="greeter">Hello</h1> </body> </html>
Next, I opened a new TypeScript file and added the following code:
let greeter: HTMLHeadingElement = document.getElementById("greeter") as HTMLHeadingElement; greeter.innerText = "Hello world!";
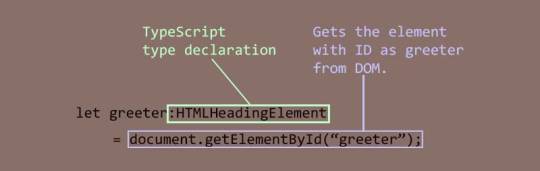
In this code, I created a variable called greeter and assigned the type HTMLHeadingElement to it. The HTMLHeadingElement type is defined in the "dom" library we added to the configuration. It tells the TypeScript compiler that greeter expects an HTML heading element and nothing else. Then, I assigned the greeter to the value returned by the getElementById function, which selects an element by its ID. Finally, I set the inner text of the greeter element to "Hello world."
When I compiled the code with the following command:
tsc script.ts
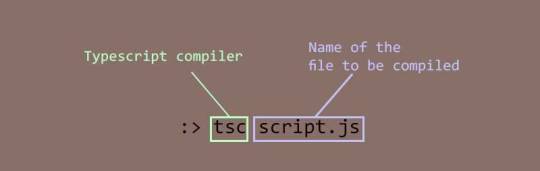
It produced the following error:
Type 'HTMLElement | null' is not assignable to type 'HTMLHeadingElement'. Type 'null' is not assignable to type 'HTMLHeadingElement'.
It's a bit frustrating, but TypeScript is doing its job. This error means that I tried to assign a greeter, which is of type HTMLHeadingElement, with an object of type HTMLElement that the getElementById method returned. The HTMLElement | null in the error message indicates that the method's return value can be either of type HTMLElement or null.
To address this, I used TypeScript's type assertion feature to tell the compiler that the element returned by getElementById is indeed a heading element, and it doesn't need to worry about it. Here's the updated code:
let greeter: HTMLHeadingElement = document.getElementById("greeter") as HTMLHeadingElement; greeter.innerText = "Hello world!";
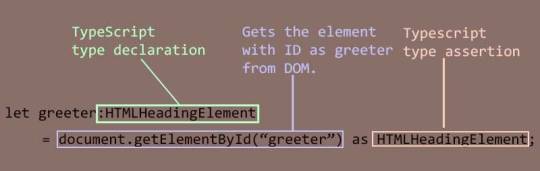
With this change, the compilation was successful. I included the script.js file generated by the compiler in the HTML document and opened it in a browser.
Decoration Time: Now that I've confirmed that everything works as intended, it's time to make the page more visually appealing. I wanted a font style that was informal, so I chose the "Rock Salt" font from Google Fonts. I imported it into my stylesheet, along with "Dancing Script" as a secondary font, using CSS imports. I then added a few more elements to the HTML document, centered all the text using CSS flexbox, added a background from UI gradients, and adjusted the positions of some elements for proper arrangement. The page now looked beautiful.
Animation: To add a finishing touch, I wanted to include a background animation of orbs rising to the top like bubbles. To create the orbs, I decided to use <div> elements. Since I wanted several orbs with different sizes, I split the task into two steps to simplify the work.
First, I created a common style for all the orbs and defined a custom animation for the orbs in CSS. Then, I created the orbs dynamically using TypeScript. I created a set number of <div> elements, assigned them the pre-defined style, and randomized their sizes, positions, and animation delays to make them appear more natural.
Here's an excerpt of the code for creating the bubbles:
function createBubbles() { for (let i = 0; i < bubbleCount; i++) { let div: HTMLDivElement = document.createElement("div") as HTMLDivElement; let divSize = getSize(); div.style.left = getLeftPosition() + "px"; div.style.width = divSize + "px"; div.style.height = divSize + "px"; div.style.animationDelay = i * randomFloat(0, 30) + "s"; div.style.filter = "blur(" + randomFloat(2, 5) + "px)"; div.classList.add("bubble"); bubbleBuffer.push(div); } console.log("Bubbles created"); }
After creating the orbs, I added them to the DOM and started the animation:
function releaseBubbles() { createBubbles(); for (let i = 0; i < bubbleCount; i++) { containerDiv.appendChild(bubbleBuffer[i]); } console.log("Bubbles released"); }
And with that, the animation of orbs rising like bubbles was set in motion.
Here's the final output:
youtube
You can find the complete code in this repository.
Conclusion: While writing this article and creating the example, I realized the involvement of advanced concepts like type assertion and union types. I now understand why the authors of those tutorials didn't include them; introducing them could confuse beginners. It's best to learn TypeScript thoroughly before venturing into DOM manipulation.
In my example, I skipped null checking when fixing the type mismatch error, as it seemed unnecessary for the demonstration. However, in real projects, it's important to check for null values to avoid runtime errors. I also didn't
#While writing this article and creating the example#I realized the involvement of advanced concepts like type assertion and union types. I now understand why the authors of those tutorials di#beginner#typescript#dom manipulation#Youtube
0 notes
Text
How to use JavaScript for data visualization
Data visualization is the process of transforming data into graphical or visual representations, such as charts, graphs, maps, etc. Data visualization can help us understand, analyze, and communicate data more effectively and efficiently.
JavaScript is a powerful and popular programming language that can be used for creating dynamic and interactive data visualizations in web browsers. JavaScript can manipulate the HTML and CSS elements of a web page, as well as fetch data from external sources, such as APIs, databases, or files.
To use JavaScript for data visualization, we need to learn how to:
Select and manipulate HTML elements: We can use JavaScript to select HTML elements by their id, class, tag name, attribute, or CSS selector. We can use methods such as getElementById(), getElementsByClassName(), getElementsByTagName(), querySelector(), or querySelectorAll() to return one or more elements. We can then use properties and methods such as innerHTML, style, setAttribute(), removeAttribute(), appendChild(), removeChild(), etc. to manipulate the selected elements.
Create and modify SVG elements: SVG (Scalable Vector Graphics) is a standard for creating vector graphics in XML format. SVG elements can be scaled without losing quality, and can be styled and animated with CSS and JavaScript. We can use JavaScript to create and modify SVG elements, such as , , , , , etc. We can use methods such as createElementNS(), setAttributeNS(), appendChild(), etc. to manipulate the SVG elements.
Handle events: We can use JavaScript to handle events that occur on the web page, such as mouse clicks, keyboard presses, window resizing, page loading, etc. We can use methods such as addEventListener() or removeEventListener() to attach or detach event handlers to elements. We can also use properties such as onclick, onkeyup, onload, etc. to assign event handlers to elements. An event handler is a function that runs when an event occurs. We can use the event object to access information about the event, such as its type, target, coordinates, key code, etc.
Use AJAX: We can use AJAX (Asynchronous JavaScript and XML) to send and receive data from a server without reloading the web page. We can use objects such as XMLHttpRequest or Fetch API to create and send requests to a server. We can also use methods such as open(), send(), then(), catch(), etc. to specify the request parameters, send the request, and handle the response. We can use properties such as readyState, status, responseText, responseJSON, etc. to access the state, status, and data of the response.
These are some of the basic skills we need to use JavaScript for data visualization. However, writing JavaScript code from scratch for data visualization can be challenging and time-consuming. That’s why many developers use JavaScript libraries and frameworks that provide pre-written code and templates for creating various types of data visualizations.
Some of the most popular JavaScript libraries and frameworks for data visualization are:
D3.js: D3.js (short for Data-Driven Documents) is a JavaScript library for producing dynamic and interactive data visualizations in web browsers. It makes use of SVG, HTML5, and CSS standards. D3.js allows us to bind data to DOM elements, apply data-driven transformations to them, and create custom visualizations with unparalleled flexibility1.
Chart.js: Chart.js is a JavaScript library for creating simple and responsive charts in web browsers. It uses HTML5 canvas element to render the charts. Chart.js supports various types of charts, such as line, bar, pie, doughnut, radar, polar area, bubble, scatter, etc.2
Highcharts: Highcharts is a JavaScript library for creating interactive and high-quality charts in web browsers. It supports SVG and canvas rendering modes. Highcharts supports various types of charts, such as line, spline, area, column, bar, pie, scatter, bubble, gauge, heatmap, treemap, etc. Highcharts also provides features such as zooming, panning, exporting, annotations, drilldowns, etc.
These are some of the popular JavaScript libraries and frameworks for data visualization. To use them in your web development project, you need to follow these steps:
Choose the right library or framework for your project: Depending on your project’s requirements, goals, and preferences, you need to select the most suitable library or framework that can help you create the data visualizations you want. You should consider factors such as the learning curve, documentation, community support, performance, compatibility, scalability, etc. of each library or framework before making a decision.
Add the library or framework to your web page: You can add a library or framework to your web page using either a script tag or an external file. The script tag can be placed either in the head or the body section of the HTML document. The external file can be linked to the HTML document using the src attribute of the script tag.
Learn how to use the library or framework features and functionalities: You need to read the documentation and guides of the library or framework you are using to understand how to use its features and functionalities effectively. You should also follow some tutorials and examples to get familiar with the syntax, conventions, patterns, and best practices of the library or framework.
Write your application code using the library or framework: You need to write your application code using the library or framework functions, methods, objects, components, etc. You should also test and debug your code regularly to ensure its functionality and quality.
These are some of the ways you can use JavaScript for data visualization. To learn more about JavaScript and data visualization, you can visit some of the online resources that offer tutorials, examples, exercises, and quizzes. Some of them are:
[W3Schools]: A website that provides free web development tutorials and references for HTML, CSS, JavaScript, and other web technologies.
[MDN Web Docs]: A website that provides documentation and guides for web developers, covering topics such as HTML, CSS, JavaScript, Web APIs, and more.
[Data Visualization with D3.js]: A website that provides a comprehensive course on data visualization with D3.js.
[e-Tutitions]: A website that provides online courses and for web , development, Learn JavaScript and you can book a free demo class.
[Codecademy]: A website that provides interactive online courses and projects for various programming languages and technologies.
0 notes
Text
Javascript / getElementsByTagName
Aqui les traigo una funcion de JAvascript que nos permite enlazar tags de nuestro HTML con variabes de Javascript, espero le ssea de utilidad!
Bievenidos sean a este post, hoy veremos una funcion muy particular. En otros posts hemos visto dos funciones que nos permitian enlazar un elemento del codigo HTML con una variable de Javascript, si lo hacemos por medio del id del elemento utilizabamos a getElementById pero tambien disponemos de getElementsByName donde buscara todos los elementos con el nombre indicado y lo almacenara en un…
View On WordPress
0 notes
Text
Me after watching a javascript tutorial in which the guy explains that the function "getElementById" gets the element by ID:

The thing about programming is that I am dumb as fuck and can't learn anything on my own and I need someone smarter than me to tell me what something does and assure me I understood it correctly otherwise I'm not confident enough to try anything
2 notes
·
View notes
Text
Dante "The Inferno" Kirkman Finds a Balance Between School and Boxing
(adsbygoogle = window.adsbygoogle || []).push({});
By Hector Franco
Follow @MrHector_Franco !function(d,s,id){var js,fjs=d.getElementsByTagName(s)[0],p=/^http:/.test(d.location)?'http':'https';if(!d.getElementById(id))(document, 'script', 'twitter-wjs'); Follow @Frontproofmedia!function(d,s,id){var js,fjs=d.getElementsByTagName(s)[0],p=/^http:/.test(d.location)?'http':'https';if(!d. getElementById(id))(document, 'script', 'twitter-wjs');
Published: March 04, 2025
The world of pugilism and academics, at first glance, don't seem to have much in common. Yet they both require discipline and dedication, and only a few can maintain both at a successful level. Being able to do both simultaneously takes a special type of individual—one who has a hyper focus and can lock in at the task in front of him. For middleweight Dante Kirkman (3-0, 1 KO), boxing and school go hand in hand.
Kirkman returns to the squared circle on March 08, taking on Jose Cruz (2-6, 1 KO) in a four-round middleweight contest at the Thunder Valley Casino Resort in Lincoln, CA.
Although for an outsider, a sport like boxing can seem simple. And in some ways, it is. Boxing, at its core, is the art of hitting and not being hit. Kirkman, a full-time student at Standford University, is the only professional boxer in the history of the famous institution participating in the top-tier avenue of both the academic and athletic worlds. The 23-year Stanford University student nicknamed "The Inferno" upbringing in the Palo Alto, CA area has forged and led him to have the capability to withstand being a full-time student and fighter, starting with his parents.
"My dad came from a pretty rough neighborhood in East Palo Alto, which used to be the murder capital of the United States," Kirkman told Frontproof Media in an exclusive interview. "And my mom…went to Harvard and then Stanford for law school. Both of them understood the value of Education.
"My dad always said, 'If you work hard, you can do whatever you want in this world.'"
Kirkman's family foundation with both parents has made him luckier than most who have lived in areas that were once deemed a 'murder capital.' Yet, Kirkman isn't your typical student-athlete whose ambitions are boxed into the classroom and the athletic field. Kirkman is an artistic and creative savant who has dabbled in creative writing and photography—not simply as a hobby but recognized and published by the New York Times.
"I was one of the best artists in the country in creative writing and photography in high school, getting awarded a US Presidential Scholar of the Arts and being published in the New York Times."
While some athletes, specifically student-athletes, prefer to keep their academic and athletic sides separate, Kirkman views boxing as an integral part of all facets of his life. Two of Kirkman's inspirations and idols in the boxing game are Floyd Mayweather Jr. and Andre Ward. Both of whom retired from boxing undefeated and have entered into the International Boxing Hall of Fame.
Perhaps the manner in which Mayweather and Ward applied their styles endeared them to Kirkman. Neither fighter will likely be remembered for providing fans with action-filled, non-stop brawls, but they both left a mark on the sport, providing a blueprint for future fighters to follow. Regarding their work inside the ring, they may not have been everyone's cup of tea, but few in the last era reached their level of craft and dominance. Kirkman views the two Hall-of-Famers as artists who used the boxing ring as their canvas.
"Boxing has the ability to be very ugly and very aggressive, but the masters…are very artful," Kirkman explained. "Everything is controlled, and it can even look as beautiful as ballet. I think that's where the beauty of boxing is…it requires rhythm and IQ.
"I prioritize defense and try to box smart. I practice all areas of my game, just like Andre Ward."
One of the most crucial factors for success as a student and in life, in general, is being able to manage your time. A typical college student usually has a plate full of classes and a part-time job. For Kirkman, who attends an elite school and engages in a professional sport, time management is of the utmost importance and something he views as part of his life. He recognizes that it is not only his future and career at stake but also those family members who made sacrifices to make his opportunities a reality.
"Boxing has been the most important thing in my life since I was 10 years old", Kirkman stated. "I train in the morning, attend classes, run, and finish my day with homework and recovery. It's back-to-back, but I'm blessed.
"People have made sacrifices for me - my parents, my coaches- so I have to try my best."
Despite fans' perceptions, every fight is essential for a fighter in the beginning stages of his career. In these early chapters, mistakes can be corrected, styles can be molded, and a fighter's true nature can be determined. The teachings of his coach, Eddie Croft, will be fully realized, guiding him from fight to fight. Even with his busy schedule, Kirkman plans to stay busy throughout 2025 and continue his ascension up the middleweight rankings.
"I'm hoping to get four or five fights in 2025," said Kirkman. "I had three fights in six months in 2024, so I want to expand on that."
(Featured Photo: James Guirao)
0 notes