#queryselector
Explore tagged Tumblr posts
Text
0 notes
Text

Saturn Cult
#tokyo#new york#saturn#cult#planets#coding#queryselector(👽)#databaseshredder#digital art#artists on tumblr#vaporwave#ufo
34 notes
·
View notes
Text
Ice Machine Cleaner in Shamokin - Crystal Cleanice
How do you clean ice machines?
Cleaning an ice machine is crucial to maintain its efficiency and ensure the ice it produces is safe for consumption. Here’s a general guide:
Regular Cleaning:
Preparation: Turn off the ice machine and unplug it from the power source.
Empty Ice Bin: Remove all the ice from the machine and discard it.
Sanitize Surfaces: Use a mild detergent and warm water to clean the interior and exterior surfaces of the machine. Use a soft cloth or sponge to wipe down these areas thoroughly.
Rinse: After cleaning, rinse the surfaces with clean water to remove any soap residue.
Sanitize with Approved Solution: Use a sanitizer approved for ice machines. Follow the manufacturer’s instructions carefully. This step helps eliminate bacteria and prevents contamination of the ice.
Deep Cleaning:
Prepare Cleaning Solution: Follow the manufacturer’s guidelines to create a cleaning solution using a specialized ice machine cleaner or a mixture of water and a recommended descaler.
Run Cleaning Cycle: Pour the cleaning solution into the machine and run a cleaning cycle as per the manufacturer's instructions. This process helps remove mineral buildup and disinfect the internal components.
Rinse Thoroughly: After the cleaning cycle, rinse the machine thoroughly with clean water to remove any residue from the cleaning solution.
Sanitize: Use a sanitizer approved for ice machines and follow the manufacturer's instructions to sanitize the machine after the cleaning process. This step is crucial to ensure the ice produced is safe for consumption.
Maintenance Tips:
Regularly inspect and clean the ice machine’s air filters, condenser coils, and water filters as per the manufacturer's recommendations.
Follow a routine maintenance schedule provided in the user manual to prevent mineral buildup and bacterial growth.
Keep the area around the ice machine clean to prevent contamination.
Always refer to the specific instructions provided by the manufacturer for your ice machine model, as different machines may have slightly different cleaning procedures.
#Cleaning Will Keep#Document Queryselector Head#Queryselector Head Appendchild#Preventive Maintenance Cleaning#Home About Services#Peace Of Mind#Crystal Clean Ice#Maintenance And Cleaning#Commercial Ice Machine
1 note
·
View note
Text
JavaScript Fundamentals
I have recently completed a course that extensively covered the foundational principles of JavaScript, and I'm here to provide you with a concise overview. This post will enable you to grasp the fundamental concepts without the need to enroll in the course.
Prerequisites: Fundamental HTML Comprehension
Before delving into JavaScript, it is imperative to possess a basic understanding of HTML. Knowledge of CSS, while beneficial, is not mandatory, as it primarily pertains to the visual aspects of web pages.
Manipulating HTML Text with JavaScript
When it comes to modifying text using JavaScript, the innerHTML function is the go-to tool. Let's break down the process step by step:
Initiate the process by selecting the HTML element whose text you intend to modify. This selection can be accomplished by employing various DOM (Document Object Model) element selection methods offered by JavaScript ( I'll talk about them in a second )
Optionally, you can store the selected element in a variable (we'll get into variables shortly).
Employ the innerHTML function to substitute the existing text with your desired content.
Element Selection: IDs or Classes
You have the opportunity to enhance your element selection by assigning either an ID or a class:
Assigning an ID:
To uniquely identify an element, the .getElementById() function is your go-to choice. Here's an example in HTML and JavaScript:
HTML:
<button id="btnSearch">Search</button>
JavaScript:
document.getElementById("btnSearch").innerHTML = "Not working";
This code snippet will alter the text within the button from "Search" to "Not working."
Assigning a Class:
For broader selections of elements, you can assign a class and use the .querySelector() function. Keep in mind that this method can select multiple elements, in contrast to .getElementById(), which typically focuses on a single element and is more commonly used.
Variables
Let's keep it simple: What's a variable? Well, think of it as a container where you can put different things—these things could be numbers, words, characters, or even true/false values. These various types of stuff that you can store in a variable are called DATA TYPES.
Now, some programming languages are pretty strict about mentioning these data types. Take C and C++, for instance; they're what we call "Typed" languages, and they really care about knowing the data type.
But here's where JavaScript stands out: When you create a variable in JavaScript, you don't have to specify its data type or anything like that. JavaScript is pretty laid-back when it comes to data types.
So, how do you make a variable in JavaScript?
There are three main keywords you need to know: var, let, and const.
But if you're just starting out, here's what you need to know :
const: Use this when you want your variable to stay the same, not change. It's like a constant, as the name suggests.
var and let: These are the ones you use when you're planning to change the value stored in the variable as your program runs.
Note that var is rarely used nowadays
Check this out:
let Variable1 = 3; var Variable2 = "This is a string"; const Variable3 = true;
Notice how we can store all sorts of stuff without worrying about declaring their types in JavaScript. It's one of the reasons JavaScript is a popular choice for beginners.
Arrays
Arrays are a basically just a group of variables stored in one container ( A container is what ? a variable , So an array is also just a variable ) , now again since JavaScript is easy with datatypes it is not considered an error to store variables of different datatypeslet
for example :
myArray = [1 , 2, 4 , "Name"];
Objects in JavaScript
Objects play a significant role, especially in the world of OOP : object-oriented programming (which we'll talk about in another post). For now, let's focus on understanding what objects are and how they mirror real-world objects.
In our everyday world, objects possess characteristics or properties. Take a car, for instance; it boasts attributes like its color, speed rate, and make.
So, how do we represent a car in JavaScript? A regular variable won't quite cut it, and neither will an array. The answer lies in using an object.
const Car = { color: "red", speedRate: "200km", make: "Range Rover" };
In this example, we've encapsulated the car's properties within an object called Car. This structure is not only intuitive but also aligns with how real-world objects are conceptualized and represented in JavaScript.
Variable Scope
There are three variable scopes : global scope, local scope, and function scope. Let's break it down in plain terms.
Global Scope: Think of global scope as the wild west of variables. When you declare a variable here, it's like planting a flag that says, "I'm available everywhere in the code!" No need for any special enclosures or curly braces.
Local Scope: Picture local scope as a cozy room with its own rules. When you create a variable inside a pair of curly braces, like this:
//Not here { const Variable1 = true; //Variable1 can only be used here } //Neither here
Variable1 becomes a room-bound secret. You can't use it anywhere else in the code
Function Scope: When you declare a variable inside a function (don't worry, we'll cover functions soon), it's a member of an exclusive group. This means you can only name-drop it within that function. .
So, variable scope is all about where you place your variables and where they're allowed to be used.
Adding in user input
To capture user input in JavaScript, you can use various methods and techniques depending on the context, such as web forms, text fields, or command-line interfaces.We’ll only talk for now about HTML forms
HTML Forms:
You can create HTML forms using the <;form> element and capture user input using various input elements like text fields, radio buttons, checkboxes, and more.
JavaScript can then be used to access and process the user's input.
Functions in JavaScript
Think of a function as a helpful individual with a specific task. Whenever you need that task performed in your code, you simply call upon this capable "person" to get the job done.
Declaring a Function: Declaring a function is straightforward. You define it like this:
function functionName() { // The code that defines what the function does goes here }
Then, when you need the function to carry out its task, you call it by name:
functionName();
Using Functions in HTML: Functions are often used in HTML to handle events. But what exactly is an event? It's when a user interacts with something on a web page, like clicking a button, following a link, or interacting with an image.
Event Handling: JavaScript helps us determine what should happen when a user interacts with elements on a webpage. Here's how you might use it:
HTML:
<button onclick="FunctionName()" id="btnEvent">Click me</button>
JavaScript:
function FunctionName() { var toHandle = document.getElementById("btnEvent"); // Once I've identified my button, I can specify how to handle the click event here }
In this example, when the user clicks the "Click me" button, the JavaScript function FunctionName() is called, and you can specify how to handle that event within the function.
Arrow functions : is a type of functions that was introduced in ES6, you can read more about it in the link below
If Statements
These simple constructs come into play in your code, no matter how advanced your projects become.
If Statements Demystified: Let's break it down. "If" is precisely what it sounds like: if something holds true, then do something. You define a condition within parentheses, and if that condition evaluates to true, the code enclosed in curly braces executes.
If statements are your go-to tool for handling various scenarios, including error management, addressing specific cases, and more.
Writing an If Statement:
if (Variable === "help") { console.log("Send help"); // The console.log() function outputs information to the console }
In this example, if the condition inside the parentheses (in this case, checking if the Variable is equal to "help") is true, the code within the curly braces gets executed.
Else and Else If Statements
Else: When the "if" condition is not met, the "else" part kicks in. It serves as a safety net, ensuring your program doesn't break and allowing you to specify what should happen in such cases.
Else If: Now, what if you need to check for a particular condition within a series of possibilities? That's where "else if" steps in. It allows you to examine and handle specific cases that require unique treatment.
Styling Elements with JavaScript
This is the beginner-friendly approach to changing the style of elements in JavaScript. It involves selecting an element using its ID or class, then making use of the .style.property method to set the desired styling property.
Example:
Let's say you have an HTML button with the ID "myButton," and you want to change its background color to red using JavaScript. Here's how you can do it:
HTML: <button id="myButton">Click me</button>
JavaScript:
// Select the button element by its ID const buttonElement = document.getElementById("myButton"); // Change the background color property buttonElement.style.backgroundColor = "red";
In this example, we first select the button element by its ID using document.getElementById("myButton"). Then, we use .style.backgroundColor to set the background color property of the button to "red." This straightforward approach allows you to dynamically change the style of HTML elements using JavaScript.
#studyblr#code#codeblr#css#html#javascript#java development company#python#study#progblr#programming#studying#comp sci#web design#web developers#web development#website design#ui ux design#reactjs#webdev#website#tech
400 notes
·
View notes
Text
Day 30 — 35/ 100 Days of Code
I learned how to handle JavaScript features (default parameters, spread, rest, and destructuring).
And I finally reached the DOM chapeter, 'The Document Object' which contains representations of all the content on a page. I'm still discovering its methods and properties.
So far, I've learned about the most useful ones: getElementById(), querySelector, changing styles, manipulating attributes, and the append method.
I'm doing some silly practice exercises just to get more familiar with the DOM methods. The more I progress in my course, the more fun things seem to get. I'm really excited to start building stuff with code and combining HTML, CSS, and JS all together.
#100 days of code journal#learning#coding#webdevelopment#codeblr#studyblr#growing#imporving#self improvement#cs#computer science#programming#codenewbie#tech#learn to code#frontend#100daysofcode#coding blog#htlm#css#JavaScript#The DOM
66 notes
·
View notes
Text
Maths Games Project from Scratch Using Javascript
Learn JavaScript and how to create a Maths Game using JavaScript, Visual Studio Code, Chrome DevTools, and resources from the Mozilla Developer Network.
You will also learn how to create a second more advanced version of the Game and a downloadable CSV File of the player’s score.
The game creation process includes a host of code examples and employs a large number of Functions such as Math Random, querySelector, and CreateElement.
#free course#online courses#course#online course creation#online#education#educate yourself#workout#knowledge#health and wellness#maths#maths posting#mathskills#programming#computer science#computer
1 note
·
View note
Text
الجزء التاسع - DOM – Document Object Model - سلسلة FSWD - JS
✅ DOM – Document Object Model 🟡 أولًا: الوصول للعناصر ✅ getElementById دي الطريقة الأساسية للوصول لعناصر الصفحة باستخدام معرف (ID). ✅ مثال <div id="message">مرحبا بك في الموقع!</div> <script> let message = document.getElementById("message"); console.log(message); // هترجع العنصر اللي فيه ID="message" </script> ✅ querySelector تتيح لك هذه الطريقة الوصول لأي عنصر باستخدام CSS selectors (زي id,…
0 notes
Text
Acidente para Alameda e complica trânsito na Ponte Rio-Niterói | Enfoco
News https://portal.esgagenda.com/acidente-para-alameda-e-complica-transito-na-ponte-rio-niteroi-enfoco/
Acidente para Alameda e complica trânsito na Ponte Rio-Niterói | Enfoco
Acidente para Alameda e complica trânsito na Ponte Rio-Niterói | Enfoco – O seu site de notícias\r\n\t\t\t \r\n \r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n \r\n\r\n\r\n\r\n\r\n \r\n \r\n \r\n \r\n \r\n \r\n \r\n\r\n \r\n \r\n \r\n \r\n \r\n \r\n \r\n\r\n \r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n
\r\n \r\n
\r\n
\r\n
\r\n\r\n Seu navegador não suporta o elemento de vídeo.\r\n
\r\n
\r\n\r\n \r\n
\r\n \r\n \r\n \r\n \r\n\r\n \r\n Grupo Enfoco |\r\n \r\n \r\n \r\n
\r\n \r\n \r\n
\r\n \r\n \r\n \r\n \r\n \r\n \r\n\r\n <![CDATA[\r\n function hideMask(element) \r\n\r\n let control = element.parentNode.parentNode.previousElementSibling\r\n control.style.display = 'none'\r\n\r\n \r\n\r\n controls = document.querySelectorAll(\".controls button\")\r\n\r\n controls.forEach(control => \r\n\r\n control.onclick = () => \r\n\r\n // Get the video player element\r\n let video = control.closest(\".mw-article-video\").querySelector(\"iframe\");\r\n\r\n // Load the video content\r\n video.onload = () => \r\n hideMask(video)\r\n \r\n video.tagName == 'IFRAME' ? video.src = video.dataset['source'] : video.dataset['href'] = video.dataset['source']\r\n\r\n // Delete data-source attribute\r\n delete video.dataset['source'];\r\n \r\n );\r\n\r\n ]]>\r\n
\r\n\r\n\t\t\t \r\n
Um acidente entre uma moto e um ônibus complica o trânsito na tarde desta sexta-feira (11), na Alameda São Boaventura, em Niterói, no sentido São Gonçalo. A colisão ocorreu por volta das 15h55 e causa retenções na via, com reflexos que chegam até a Ponte Rio-Niterói e também na Avenida Feliciano Sodré, no Centro de Niterói.
O motociclista Carlos Henrique Lorenzo, de 23 anos, foi a vítima da colisão. Ele recebeu os primeiros socorros no local e depois levado para o Hospital Estadual Azevedo Lima (Heal), no Fonseca, em estado de saúde moderado, segundo os bombeiros.
\r\n\t\t\t \r\n \r\n
\r\n \r\n <![CDATA[\r\n const colorCategory = document.querySelector(\".efo-inlineRelated\").dataset.color;\r\n \r\n // Criando uma nova regra de estilo para o ::before\r\n const style = document.createElement('style');\r\n style.innerHTML = `\r\n .efo-inlineRelated::before \r\n background-color: $colorCategory;\r\n \r\n `;\r\n \r\n // Adicionando a nova regra ao head do documento\r\n document.head.appendChild(style);\r\n ]]>\r\n \r\n\r\n\r\n\t\t\t \r\n
Agentes da NitTrans e guardas municipais estão no local para controlar o tráfego e dar apoio à operação de resgate. Segundo o Corpo de Bombeiros, as causas do acidente ainda são desconhecidas.
\r\n\t\t\t \r\n \r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n \r\n\r\n\r\n\r\n\r\n \r\n \r\n \r\n \r\n \r\n \r\n \r\n\r\n \r\n \r\n \r\n \r\n \r\n \r\n \r\n\r\n \r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\t\r\n\t
\r\n\t\t\r\n\t\t\r\n\t\t\r\n\t\t\t\r\n\t\t\t\r\n\t\t\t\t\r\n\t\t\r\n\t\t\t\t\r\n\t\t\t\r\n\t\t\t\r\n\t\t\r\n\t\t\r\n\t\t\t\r\n\t\t\t\r\n\r\n\t\t\t\r\n\t\t\t\t\r\n\t\t\t\r\n\t\t\t\r\n\t\t\t\t
\r\n\t\t\t\t\t\r\n\t\t\t\t\t\t\r\n\t\t\t\t\t\t\tTrânsito ficou complicado na Alameda São Boaventura na tarde desta sexta-feira (11)\r\n\t\t\t\t\t\t\r\n\t\t\t\t\t\r\n\t\t\t\t\t\r\n\t\t\t\t\t\t\r\n\t\t\t\t\t\t\t| Foto:\r\n\t\t\t\t\t\t\tGabriel Rechenioti\r\n\t\t\t\t\t\t\r\n\t\t\t\t\t\r\n\t\t\t\t
\r\n\t\t\t\r\n\t
\r\n\r\n\r\n\t\r\n\r\n\r\n\t\t\t \r\n
De acordo com a Ecoponte, o acidente gerou impactos significativos na mobilidade. O tempo de travessia na Ponte Rio-Niterói, no sentido Niterói, chega a 40 minutos no momento.
“,”searchAbstract”:””,”leadHtml”:”Há reflexos também no tráfego da Avenida Feliciano Sodré”,”subHeaderHtml”:”Deu nó”,”viewCount”:1029,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”previousArticle”:“articleId”:128830,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T16:51:26.903-03:00″,”publishDate”:”2025-04-11T16:51:26-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”generator”:”Elite CS – Web Text”,”author”:””,”authorEmail”:””,”authorTitle”:””,”description”:””,”frontPage”:false,”sourceId”:80060,”name”:”lojas-de-produtos-falsos-na-mira-da-policia-em-niteroi”,”canonicalUrl”:””,”headerHtml”:”Lojas de produtos falsos na mira da polícia em Niterói”,”alternativeHeaderHtml”:””,”keywords”:””,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:””,”subHeaderHtml”:””,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Polícia”],”nextArticle”:“articleId”:128832,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T17:38:53.6-03:00″,”publishDate”:”2025-04-11T17:38:53.6-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”generator”:”Elite CS – Web Text”,”author”:””,”authorEmail”:””,”authorTitle”:””,”description”:””,”frontPage”:false,”sourceId”:80066,”name”:”mc-carol-de-niteroi-anuncia-fim-do-casamento-acabou”,”canonicalUrl”:””,”headerHtml”:”MC Carol de Niterói anuncia fim do casamento: ‘Acabou!'”,”alternativeHeaderHtml”:””,”keywords”:””,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:””,”subHeaderHtml”:””,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“Entretenimento:Celebridades”],”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:“Facebook”:“path”:”https://cdn.enfoco.com.br/img/Facebook/120000/Acidente-para-Alameda-e-complica-transito-na-Ponte0012883100202504111710.png?xid=640243″,”description”:””,”width”:1200,”height”:720,”author”:”Grupo Enfoco”,”sourceId”:92006,”text”:””,”authorTitle”:””,”elementId”:640243,”order”:0,”ownerType”:”Article”,”ownerId”:128831,”galleryId”:0,”parentId”:0,”parentElementType”:””,”language”:””,”cdn”:“cdnId”:1,”name”:”default”,”urlPrefix”:”https://cdn.enfoco.com.br/”,”enabled”:true,”thumbnailCdn”:“cdnId”:0,”enabled”:true,”authorEmail”:””,”title”:””,”accessibility”:””,”text2″:””,”elementType”:”image_Facebook”,”isStandard”:false,”createdBy”:””,”lastModifiedBy”:””,”name”:””,”addressType”:””,”address”:””,”number”:””,”complements”:””,”neighbourhood”:””,”city”:””,”state”:””,”zipCode”:””,”country”:””,”directions”:””,”notes”:””,”hash”:”a0f233ff539b565a52e0cbff51665520″,”hashKey”:”7643a1f9b9a32be234a65c7ed7d9d341″,”imagesNormal”:[],”inlineImages”:[“path”:”https://cdn.enfoco.com.br/img/inline/120000/Acidente-para-Alameda-e-complica-transito-na-Ponte0012883100202504111743.png?xid=640246″,”description”:”Trânsito ficou complicado na Alameda São Boaventura na tarde desta sexta-feira (11)”,”width”:1200,”height”:720,”author”:”Gabriel Rechenioti”,”sourceId”:92011,”text”:””,”authorTitle”:””,”elementId”:640246,”order”:0,”ownerType”:”Article”,”ownerId”:128831,”galleryId”:0,”parentId”:0,”parentElementType”:””,”language”:””,”cdn”:“cdnId”:1,”name”:”default”,”urlPrefix”:”https://cdn.enfoco.com.br/”,”enabled”:true,”thumbnailCdn”:“cdnId”:0,”enabled”:true,”authorEmail”:””,”title”:””,”accessibility”:””,”text2″:””,”elementType”:”image_inline”,”isStandard”:false,”createdBy”:””,”lastModifiedBy”:””,”name”:””,”addressType”:””,”address”:””,”number”:””,”complements”:””,”neighbourhood”:””,”city”:””,”state”:””,”zipCode”:””,”country”:””,”directions”:””,”notes”:””,”hash”:”f70043d49cb02757121a7069eb5c4bc3″,”hashKey”:”a7742f142dee00c117410d4eff9a9f85″],”specialVideos”:,”videos”:[],”inlineVideos”:[“path”:”https://cdn.enfoco.com.br/img/videoinline/120000/Acidente-para-Alameda-e-complica-transito-na-Ponte0012883100202504111710.mp4?xid=640248″,”description”:””,”width”:480,”height”:848,”author”:”Grupo Enfoco”,”sourceId”:37953,”text”:””,”authorTitle”:””,”elementId”:640248,”order”:0,”ownerType”:”Article”,”ownerId”:128831,”galleryId”:0,”parentId”:0,”parentElementType”:””,”language”:””,”cdn”:“cdnId”:1,”name”:”default”,”urlPrefix”:”https://cdn.enfoco.com.br/”,”enabled”:true,”thumbnailPath”:”https://cdn.enfoco.com.br/img/thumbnail/120000/Acidente-para-Alameda-e-complica-transito-na-Ponte0012883100202504111710.jpg?xid=640247″,”thumbnailCdn”:“cdnId”:1,”name”:”default”,”urlPrefix”:”https://cdn.enfoco.com.br/”,”enabled”:true,”authorEmail”:””,”title”:”Trânsito na Alameda.mp4″,”accessibility”:””,”text2″:””,”elementType”:”video_inline”,”isStandard”:false,”createdBy”:””,”lastModifiedBy”:””,”name”:””,”addressType”:””,”address”:””,”number”:””,”complements”:””,”neighbourhood”:””,”city”:””,”state”:””,”zipCode”:””,”country”:””,”directions”:””,”notes”:””,”duration”:11146,”hash”:”5b1a50081c15082fffbf9db6f4bd3eb2″,”hashKey”:”6db8961c1cf8cae4753dc681aa8bf90e”],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[“articles”:[“articleId”:128829,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T16:29:16.967-03:00″,”publishDate”:”2025-04-11T16:29:16-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”versionDate”:”2025-04-11T18:29:12-03:00″,”generator”:”Elite CS – Web Text”,”author”:”Abel Henrique”,”authorEmail”:””,”authorTitle”:””,”description”:”Um acidente envolvendo uma moto e um caminhão foi registrado na tarde desta sexta-feira (11), na altura do Horto, na Alameda São Boaventura, no bairro Fonseca, em Niterói, Região Metropolitana do Rio. De acordo com o Corpo de Bombeiros, a colisão aconteceu por volta das 15h55.”,”frontPage”:false,”sourceId”:80064,”name”:”motociclista-fica-ferido-em-colisao-em-niteroi”,”headerHtml”:”Motociclista fica ferido em colisão na Alameda, em Niterói”,”alternativeHeaderHtml”:””,”keywords”:”acidente envolvendo uma moto e um caminhão,Alameda São Boaventura no bairro Fonseca em Niterói,vítima que conduzia a motocicleta foi socorrida ainda no local por equipes d Corpo de Bombeiros”,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:”Acidente envolveu moto e ônibus, segundo os bombeiros”,”subHeaderHtml”:”Sem identificação”,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”],“articleId”:128822,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T13:39:33.313-03:00″,”publishDate”:”2025-04-11T13:39:33-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”versionDate”:”2025-04-11T13:50:49.693-03:00″,”generator”:”Elite CS – Web Text”,”author”:”Tiago Souza”,”authorEmail”:”[email protected]”,”authorTitle”:””,”description”:”A recente transferência da estátua de Araribóia, um dos mais importantes símbolos históricos de Niterói, está gerando debate entre moradores, ciclistas e frequentadores da Praça Arariboia, no Centro da cidade. A estátua, que há décadas ocupava um ponto em frente à estação das barcas, foi realocada cerca de 100 metros adiante, ainda na Avenida Rio Branco, para dar espaço à construção de uma nova ciclovia.”,”frontPage”:false,”sourceId”:80049,”name”:”transferecia-estatua-arariboia”,”headerHtml”:”Veja o novo ‘endereço’ da Estátua de Arariboia, em Niterói”,”alternativeHeaderHtml”:””,”keywords”:”arariboia,estatua,niterói”,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:”Símbolo da cidade está de frente para a Baía de Guanabara”,”subHeaderHtml”:”Polêmica”,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”],“articleId”:128818,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T12:54:30.747-03:00″,”publishDate”:”2025-04-11T12:54:30.747-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”versionDate”:”2025-04-11T12:54:28.547-03:00″,”generator”:”Elite CS – Web Text”,”author”:”Gabriel Villa Nova”,”authorEmail”:””,”authorTitle”:””,”description”:”A Enel Rio informou, nesta sexta-feira (11), que fará o desligamento de energia, por medida de segurança, nas ruas Professor Heitor Carrilho, Fróes da Cruz, Visconde de Sepetiba e Saldanha Marinho, no Centro de Niterói, no domingo (13), das 13h às 17h.”,”frontPage”:false,”sourceId”:80052,”name”:”ruas-no-centro-de-niteroi-ficarao-sem-luz-neste-domingo”,”headerHtml”:”Ruas no Centro de Niterói ficarão sem luz neste domingo (13)”,”alternativeHeaderHtml”:””,”keywords”:”Ruas no Centro de Niterói ficarão sem luz neste domingo”,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:”Desligamento será das 13h às 17h”,”subHeaderHtml”:”Serviço”,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”]],”elementId”:15901,”order”:0,”sourceId”:1,”ownerType”:”Article”,”ownerId”:128831,”galleryId”:0,”parentId”:0,”parentElementType”:””,”language”:””,”path”:””,”thumbnailPath”:””,”author”:””,”authorTitle”:””,”authorEmail”:””,”title”:””,”description”:”Acidente para Alameda e complica trânsito na Ponte Rio-Niterói”,”accessibility”:””,”text”:””,”text2″:””,”externalType”:””,”externalId”:””,”externalPlaylistId”:””,”externalEmbedCode”:””,”externalMetadata”:””,”externalUrl”:””,”elementType”:”RELATEDARTICLEGROUP”,”isStandard”:false,”createdBy”:””,”lastModifiedBy”:””,”name”:”Acidente para Alameda e complica trânsito na Ponte Rio-Niterói”,”addressType”:””,”address”:””,”number”:””,”complements”:””,”neighbourhood”:””,”city”:””,”state”:””,”zipCode”:””,”country”:””,”directions”:””,”notes”:””],”inlineRelatedArticleGroups”:[“articles”:[“articleId”:128829,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T16:29:16.967-03:00″,”publishDate”:”2025-04-11T16:29:16-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”versionDate”:”2025-04-11T18:29:12-03:00″,”generator”:”Elite CS – Web Text”,”author”:”Abel Henrique”,”authorEmail”:””,”authorTitle”:””,”description”:”Um acidente envolvendo uma moto e um caminhão foi registrado na tarde desta sexta-feira (11), na altura do Horto, na Alameda São Boaventura, no bairro Fonseca, em Niterói, Região Metropolitana do Rio. De acordo com o Corpo de Bombeiros, a colisão aconteceu por volta das 15h55.”,”frontPage”:false,”sourceId”:80064,”name”:”motociclista-fica-ferido-em-colisao-em-niteroi”,”headerHtml”:”Motociclista fica ferido em colisão na Alameda, em Niterói”,”alternativeHeaderHtml”:””,”keywords”:”acidente envolvendo uma moto e um caminhão,Alameda São Boaventura no bairro Fonseca em Niterói,vítima que conduzia a motocicleta foi socorrida ainda no local por equipes d Corpo de Bombeiros”,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:”Acidente envolveu moto e ônibus, segundo os bombeiros”,”subHeaderHtml”:”Sem identificação”,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”],“articleId”:128822,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T13:39:33.313-03:00″,”publishDate”:”2025-04-11T13:39:33-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”versionDate”:”2025-04-11T13:50:49.693-03:00″,”generator”:”Elite CS – Web Text”,”author”:”Tiago Souza”,”authorEmail”:”[email protected]”,”authorTitle”:””,”description”:”A recente transferência da estátua de Araribóia, um dos mais importantes símbolos históricos de Niterói, está gerando debate entre moradores, ciclistas e frequentadores da Praça Arariboia, no Centro da cidade. A estátua, que há décadas ocupava um ponto em frente à estação das barcas, foi realocada cerca de 100 metros adiante, ainda na Avenida Rio Branco, para dar espaço à construção de uma nova ciclovia.”,”frontPage”:false,”sourceId”:80049,”name”:”transferecia-estatua-arariboia”,”headerHtml”:”Veja o novo ‘endereço’ da Estátua de Arariboia, em Niterói”,”alternativeHeaderHtml”:””,”keywords”:”arariboia,estatua,niterói”,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:”Símbolo da cidade está de frente para a Baía de Guanabara”,”subHeaderHtml”:”Polêmica”,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”],“articleId”:128818,”assignmentId”:0,”printArticleId”:0,”initialPublishDate”:”2025-04-11T12:54:30.747-03:00″,”publishDate”:”2025-04-11T12:54:30.747-03:00″,”directAccessPrePublish”:false,”directAccessPostExpiration”:false,”importCount”:0,”versionDate”:”2025-04-11T12:54:28.547-03:00″,”generator”:”Elite CS – Web Text”,”author”:”Gabriel Villa Nova”,”authorEmail”:””,”authorTitle”:””,”description”:”A Enel Rio informou, nesta sexta-feira (11), que fará o desligamento de energia, por medida de segurança, nas ruas Professor Heitor Carrilho, Fróes da Cruz, Visconde de Sepetiba e Saldanha Marinho, no Centro de Niterói, no domingo (13), das 13h às 17h.”,”frontPage”:false,”sourceId”:80052,”name”:”ruas-no-centro-de-niteroi-ficarao-sem-luz-neste-domingo”,”headerHtml”:”Ruas no Centro de Niterói ficarão sem luz neste domingo (13)”,”alternativeHeaderHtml”:””,”keywords”:”Ruas no Centro de Niterói ficarão sem luz neste domingo”,”language”:””,”textHtml”:””,”searchAbstract”:””,”leadHtml”:”Desligamento será das 13h às 17h”,”subHeaderHtml”:”Serviço”,”viewCount”:0,”commentsCount”:0,”gradeCount”:0,”gradeTotal”:0,”order”:0,”relatedArticles”:[],”imagesThumbnail”:[],”specialImages”:,”imagesNormal”:[],”specialVideos”:,”videos”:[],”specialAudios”:,”audios”:[],”specialAttachments”:,”attachments”:[],”highlights”:[],”quotes”:[],”addresses”:[],”galleries”:[],”faqs”:[],”summaries”:[],”relatedArticleGroups”:[],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”events”:[],”authors”:[],”programs”:[],”templateData”:,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”]],”elementId”:15902,”order”:0,”sourceId”:1,”ownerType”:”Article”,”ownerId”:128831,”galleryId”:0,”parentId”:0,”parentElementType”:””,”language”:””,”path”:””,”thumbnailPath”:””,”author”:””,”authorTitle”:””,”authorEmail”:””,”title”:””,”description”:”Acidente para Alameda e complica trânsito na Ponte Rio-Niterói”,”accessibility”:””,”text”:””,”text2″:””,”externalType”:””,”externalId”:””,”externalPlaylistId”:””,”externalEmbedCode”:””,”externalMetadata”:””,”externalUrl”:””,”elementType”:”RELATEDARTICLEGROUP_INLINE”,”isStandard”:false,”createdBy”:””,”lastModifiedBy”:””,”name”:”Acidente para Alameda e complica trânsito na Ponte Rio-Niterói”,”addressType”:””,”address”:””,”number”:””,”complements”:””,”neighbourhood”:””,”city”:””,”state”:””,”zipCode”:””,”country”:””,”directions”:””,”notes”:””],”quizzes”:[],”maps”:[],”tables”:[],”polls”:[],”headlines”:,”links”:[],”tts”:“path”:”https://cdn.enfoco.com.br/img/TTS/120000/Acidente-para-Alameda-e-complica-transito-na-Ponte0012883100202504111829.mp3?xid=640249″,”externalId”:””,”sourceId”:0,”elementId”:640249,”order”:0,”ownerType”:”Article”,”ownerId”:128831,”galleryId”:0,”parentId”:0,”parentElementType”:””,”language”:””,”cdn”:“cdnId”:1,”name”:”default”,”urlPrefix”:”https://cdn.enfoco.com.br/”,”enabled”:true,”thumbnailCdn”:“cdnId”:0,”enabled”:true,”externalType”:””,”externalPlaylistId”:””,”externalEmbedCode”:””,”externalMetadata”:””,”elementType”:”TTS”,”isStandard”:false,”createdBy”:””,”lastModifiedBy”:””,”name”:””,”addressType”:””,”address”:””,”number”:””,”complements”:””,”neighbourhood”:””,”city”:””,”state”:””,”zipCode”:””,”country”:””,”directions”:””,”notes”:””,”duration”:85082,”length”:680899,”events”:[],”authors”:[],”programs”:[],”template”:“defaultTemplate”:false,”reportFileName”:false,”templateData”:“Conteúdo Comercial”:false,”baseShareArticleUrl”:”https://enfoco.com.br/_/”,”isInPublishRange”:true,”tags”:[“tagId”:637,”sourceId”:0,”name”:”acidente”,”title”:””,”headline”:””,”description”:””,”path”:”acidente”,”template”:“defaultTemplate”:false,”reportFileName”:false,”tagItems”:[],”publishAuthor”:”Enfoco”,“tagId”:49378,”sourceId”:0,”name”:”alamenda”,”path”:”alamenda”,”template”:“defaultTemplate”:false,”reportFileName”:false,”tagItems”:[],”publishAuthor”:”Enfoco”,“tagId”:27641,”sourceId”:0,”name”:”trânsito”,”title”:””,”headline”:””,”description”:””,”path”:”transito”,”template”:“defaultTemplate”:false,”reportFileName”:false,”tagItems”:[],”publishAuthor”:”Enfoco”],”metaData”:,”publishId”:3,”publishAuthor”:”EnFoco”,”columnPath”:”_”,”categoryPaths”:[“NOTÍCIAS:Cidades:Niterói”]}”>
Deu nó
Há reflexos também no tráfego da Avenida Feliciano Sodré
Publicado 11/04/2025 às 17:10 | Atualizado em 11/04/2025 às 18:29 | Autor: Abel Henrique
Siga o Enfoco no Google News
Seu navegador não suporta o elemento de vídeo.
Um acidente entre uma moto e um ônibus complica o trânsito na tarde desta sexta-feira (11), na Alameda São Boaventura, em Niterói, no sentido São Gonçalo. A colisão ocorreu por volta das 15h55 e causa retenções na via, com reflexos que chegam até a Ponte Rio-Niterói e também na Avenida Feliciano Sodré, no Centro de Niterói.
Leia Mais
O motociclista Carlos Henrique Lorenzo, de 23 anos, foi a vítima da colisão. Ele recebeu os primeiros socorros no local e depois levado para o Hospital Estadual Azevedo Lima (Heal), no Fonseca, em estado de saúde moderado, segundo os bombeiros.
Agentes da NitTrans e guardas municipais estão no local para controlar o tráfego e dar apoio à operação de resgate. Segundo o Corpo de Bombeiros, as causas do acidente ainda são desconhecidas.
Trânsito ficou complicado na Alameda São Boaventura na tarde desta sexta-feira (11) | Foto: Gabriel Rechenioti
De acordo com a Ecoponte, o acidente gerou impactos significativos na mobilidade. O tempo de travessia na Ponte Rio-Niterói, no sentido Niterói, chega a 40 minutos no momento.
Últimas notícias
0 notes
Text
非同期処理とコールバック関数 その3
音声を再生する。 (let [btn (.querySelector js/document.body "#start_button") ctx (js/AudioContext. )] (btn.addEventListener "click" (fn [] ;; ファイルのロード (-> (js/fetch "./sound/sample.mp3") ;; AudioBuffer 生成のためのデータを取り出す ( .then (fn [response] (.arrayBuffer response))) ;; AudioBuffer 生成 ( .then (fn [buffer] (.decodeAudioData ctx buffer))) ;; 再生 ( .then (fn [decodeAudio] ;; ノードを生成 (let [node (.createBufferSource ctx)] ;; デコードしたオーディオデータをひもづける (set! (.-buffer node) decodeAudio) ;; ノードを接続する (.connect node (.-destination ctx) ;; ノードの再生が完了した後の解放処理 (node.addEventListener "ended" (fn [] (do (.stop node) (.disconnect node)))) ;; ノードの再生を開始する (.start node) (.log js/console (.-destination ctx)) ))) ( .catch (fn [] (js/alert "error!!")))) )))
0 notes
Link
DOM Query Selector and Query Selector All Welcome to the second season of the JavaScript tutorials. In this series of tutorials, we are going to cover more advanced topics, including DOM, BOM, local storage, APIs, Ajax, callbacks, and asynchronous functions. In this video, you will learn how to work with 'querySelector' and 'querySelectorAll' as alternative functions to get elements by ID, tag name, or class name. Watch The Video on Youtube
0 notes
Text

Retro Tokyo
26 notes
·
View notes
Text
sometimes I wonder if I over-automate things...
Grass:
Venusaur (200)
Sceptile (200)
Abomasnow (200)
Groudon (400)
Poison:
Beedrill (100)
Venusaur (200)
Gengar (200)
Fire:
Houndoom (100)
Charizard X (200)
Charizard Y (200)
Blaziken (200)
Groudon (400)
Camerupt (unreleased)
Dragon:
Charizard X (200)
Ampharos (200)
Sceptile (200)
Altaria (300)
Salamence (300)
Latias (300)
Latios (300)
Garchomp (300)
Rayquaza (400)
Flying:
Pidgeot (100)
Charizard Y (200)
Pinsir (200)
Aerodactyl (200)
Salamence (300)
Rayquaza (400)
Water:
Slowbro (100)
Blastoise (200)
Swampert (200)
Gyarados (300)
Kyogre (400)
Sharpedo (unreleased)
Bug:
Beedrill (100)
Pinsir (200)
Scizor (200)
Heracross (200)
Kyogre (400)
Normal:
Pidgeot (100)
Kangaskhan (200)
Lopunny (200)
Audino (unreleased)
Psychic:
Slowbro (100)
Medicham (100)
Alakazam (200)
Gardevoir (200)
Latias (300)
Latios (300)
Rayquaza (400)
Mewtwo X (unreleased)
Mewtwo Y (unreleased)
Metagross (unreleased)
Gallade (unreleased)
Ghost:
Sableye (100)
Banette (100)
Gengar (200)
Dark:
Houndoom (100)
Sableye (100)
Absol (200)
Gyarados (300)
Tyranitar (300)
Sharpedo (unreleased)
Rock:
Aerodactyl (200)
Tyranitar (300)
Diancie (300)
Electric:
Manectric (100)
Ampharos (200)
Kyogre (400)
Steel:
Steelix (200)
Scizor (200)
Aggron (200)
Mawile (unreleased)
Metagross (unreleased)
Lucario (unreleased)
Ground:
Steelix (200)
Swampert (200)
Garchomp (300)
Groudon (400)
Camerupt (unreleased)
Fighting:
Medicham (100)
Blaziken (200)
Lopunny (200)
Heracross (200)
Mewtwo X (unreleased)
Lucario (unreleased)
Gallade (unreleased)
Fairy:
Gardevoir (200)
Altaria (300)
Diancie (300)
Mawile (unreleased)
Audino (unreleased)
Ice:
Glalie (200)
Abomasnow (200)
from https://bulbapedia.bulbagarden.net/wiki/Mega_Evolution_(GO) and
const data = {}; const table = document.querySelector( 'h2:has(> #List_of_Mega_Evolutions_and_Primal_Reversions) + table' ); table.querySelectorAll('tbody tr').forEach((row) => { const cells = [...row.querySelectorAll('td')]; const index = row.previousElementSibling?.querySelector('[rowspan="2"]') ? 1 : 3; const name = cells[index].innerText .replace('Mega ', '') .replace('Primal ', ''); // hardcoded charizard y, im lazy const energy = Number(cells[index + 2]?.childNodes[0]?.nodeValue ?? 200); cells[index + 1].querySelectorAll('a').forEach((a) => { const type = a.title.replace(' (type)', ''); data[type] ??= []; data[type].push({ name, energy }); }); }); [ ['Heracross', 'Bug', 200], ['Heracross', 'Fighting', 200], ['Mewtwo X', 'Psychic'], ['Mewtwo X', 'Fighting'], ['Mewtwo Y', 'Psychic'], ['Mawile', 'Steel'], ['Mawile', 'Fairy'], ['Sharpedo', 'Water'], ['Sharpedo', 'Dark'], ['Camerupt', 'Fire'], ['Camerupt', 'Ground'], ['Metagross', 'Steel'], ['Metagross', 'Psychic'], ['Lucario', 'Fighting'], ['Lucario', 'Steel'], ['Gallade', 'Psychic'], ['Gallade', 'Fighting'], ['Audino', 'Normal'], ['Audino', 'Fairy'], ] .filter( ([name, type]) => !data[type].some(({ name: searchName }) => searchName === name) ) .forEach(([name, type, energy]) => data[type].push({ name, energy: energy ?? Infinity }) ); console.log( Object.entries(data) .map(([type, value]) => { value.sort((a, b) => a.energy - b.energy); return `${type}:\n${value .map( ({ name, energy }) => ` - ${name} (${energy === Infinity ? 'unreleased' : energy})` ) .join('\n')}\n`; }) .join('\n') );
1 note
·
View note
Link
In this tutorial, i am going to enumerate the best tips and tricks to iterate over a NodeList with the help of the JavaScript forEach() loop.
0 notes
Text
2024年01月28日の記事一覧
(全 11 件)
机から離れて論文を大量に読む方法(NGK2024S)
インボイス制度開始から3カ月経過も、3分の1の企業が対応に課題--Sansanが調査
デジタル認証アプリがやってくる
テストコードを負債化させない上手な付き合い方 / Test Code Management
灘中学の最終問題に出た展開図問題がとてもハイセンスだった「気づけば秒で解けるが優秀な頭脳を見るための良問」
ミッキーが初めて登場したあの「蒸気船ウィリー」の音声の不完全性を修正するとこうなる
【JavaScript】querySelector よりもパワフルに DOM からノードを取得しよう!【XPath】
開発チーム途中参画時の爆速キャッチアップ術
キャッシュを活用するために必要な知識と勘所
Introduction
SQLiteがバージョン管理システムとしてGitを採用しない理由
0 notes
Text
How To Perform E-Commerce Data Scraping On Ten Different Platforms
How To Perform E-Commerce Data Scraping On Ten Different Platforms?

Web scraping, also called data scraping, is a potent method for extracting valuable information from e-commerce platforms such as Amazon and Walmart. Using programming languages like JavaScript and the querySelector function, one can systematically gather the necessary data to enhance decision-making processes. In this article, we will delve into the efficient data extraction from e-commerce websites on a large scale and underscore the significance of this practice. We will explore various techniques and optimal approaches b, along with guidance on leveraging JavaScript and querySelector for data extraction.
List of Data Fields
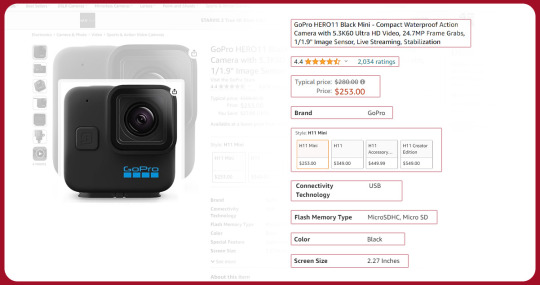
Product Name
Product Description
Product Codes
RRP
Special Price
Category
Brand
Availability
Product URL
Images
Attributes
Reviews
Ratings
Variants
List of 10 E-Commerce Websites
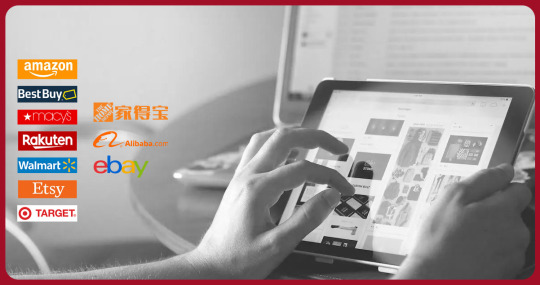
AMAZON: Amazon's e-commerce website is a global retail juggernaut that has revolutionized online shopping. Amazon has become synonymous with convenience and choice, offering an unparalleled selection of products across a multitude of categories. With a user-friendly interface, customers can effortlessly navigate through an extensive array of items, read detailed product descriptions, and make informed decisions based on user reviews and ratings. Scrape Amazon e-commerce data for competitive analysis.
Rakuten: Rakuten's e-commerce website is a prominent global online marketplace offering a diverse range of products and services to customers worldwide. Originally founded in Japan, Rakuten has expanded its presence to become a multifaceted platform that connects consumers with an extensive array of goods, from electronics and fashion to books and home essentials. Scrape Rakuten e-commerce data for price optimization.
TARGET: Target's e-commerce website is a well-established and popular online retail destination in the United States. Known for its wide range of products spanning categories like electronics, home and furniture, clothing, beauty, groceries, and more, Target offers a comprehensive shopping experience to its customers. The website features a user-friendly interface, enabling shoppers to browse and search for products, read detailed product descriptions, and view customer reviews. Scrape Target e-commerce data for market research.
MACY'S: Macy's e-commerce website is a prominent online retail platform that complements the well-known brick-and-mortar department store chain. By catering to diverse consumer needs, Macy's online platform offers various products spanning fashion, beauty, home goods, electronics, and more. The website provides an intuitive and user-friendly interface, making it easy for customers to navigate, search for products, and explore various categories. Scrape Macy's e-commerce data for inventory management.
BEST BUY: Best Buy's e-commerce website is a leading online destination for consumer electronics, appliances, and technology products in the United States and beyond. Renowned for its extensive selection of products, it caters to a wide range of tech enthusiasts, home appliance shoppers, and entertainment seekers. The website boasts a user-friendly interface that simplifies product discovery, allowing customers to browse, compare, and purchase items effortlessly. Scrape Best Buy e-commerce data for content aggregation.
eBay: eBay is a globally recognized and influential e-commerce platform that connects buyers and sellers worldwide. Founded in 1995, eBay has become one of the largest online marketplaces, facilitating the sale of a wide variety of new and used goods. The platform's unique auction-style listings and traditional fixed-price listings offer a diverse and dynamic shopping experience. Scrape eBay e-commerce data for dynamic pricing.
WALMART: Walmart's e-commerce website is a significant online extension of one of the world's largest retail chains. Founded in 1962, Walmart has established itself as a retail giant with a diverse range of products, and its e-commerce platform reflects this breadth and depth. The website offers customers a convenient and extensive shopping experience focusing on value, variety, and accessibility. Walmart's e-commerce website includes many products, including groceries, electronics, apparel, and home goods. Users can browse products easily, read detailed descriptions, and explore customer reviews to make informed purchasing decisions. Scrape Walmart e-commerce data for customer sentiment analysis.
ETSY: Etsy's e-commerce platform is a unique and thriving online marketplace specializing in handmade, vintage, and craft items. Founded in 2005, Etsy has grown into a global community of artisans, crafters, and vintage collectors who sell their one-of-a-kind and creative products on the platform. Etsy's website provides a distinctive and personalized shopping experience, catering to those seeking handmade or unique items. The heart of Etsy's e-commerce website is its diverse catalog of products, including handmade jewelry, clothing, home decor, art, and vintage collectibles. Scrape Etsy e-commerce data for demand forecasting.
Alibaba: Alibaba's e-commerce platform is one of the world's largest and most influential online marketplaces. Founded in 1999 by Jack Ma, Alibaba has grown into a global e-commerce and technology conglomerate with a diverse portfolio of businesses. Alibaba's primary e-commerce websites include Alibaba.com, Taobao, Tmall, and AliExpress, each catering to different markets and customer needs. Alibaba.com is a business-to-business (B2B) platform that connects manufacturers, wholesalers, and suppliers with global buyers. Scrape Alibaba e-commerce data for price comparison.
THE HOME DEPOT: The Home Depot's e-commerce website is a leading online home improvement and construction retail platform. Founded in 1978, The Home Depot has become one of the largest home improvement retailers globally, with physical stores and a robust online presence. The Home Depot's e-commerce website offers many products and services tailored to homeowners, contractors, and do-it-yourself enthusiasts. Customers can explore many items, including building materials, tools, appliances, home decor, gardening supplies, and more. Scrape The Home Depot e-commerce data to understand buying behavior.
About E-Commerce Data Scraping
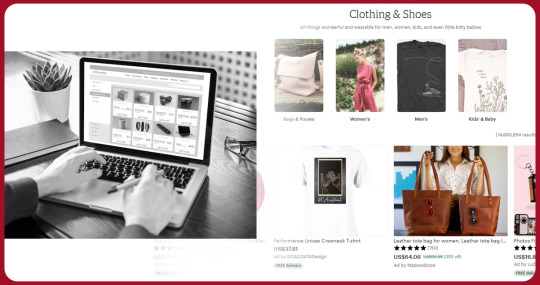
In today's digital era, data holds immense value, driving decision-making, fostering innovation, and enhancing competitiveness across various industries. Data scraping e-commerce websites emerged as a crucial process for extracting insights and supporting informed business decisions, especially when dealing with renowned websites like Amazon and Walmart.
Before diving into data extraction from these websites, it's paramount to grasp the concept of data and identify the specific data types that align with your business objectives. It may encompass collecting diverse data types, such as Product Details Pages (PDPs), search results, seller profiles, customer reviews, ratings, travel and booking data, event information, ticket details, and other pertinent data points.
Web scraping stands out as the most prevalent technique for data extraction, involving the retrieval of information from the HTML code of websites. A fundamental aspect of successful data scraping involves comprehending the website's structure and pinpointing the desired data locations, including product details pages (PDPs). Amazon and Walmart, as two prominent e-commerce platforms, often serve as targets for data extraction efforts. Employing tools and programming languages like JavaScript and Python can streamline the data extraction process from these websites. Nonetheless, scaling up data extraction can introduce challenges, including the need for proxies and strategies to evade anti-bot detection mechanisms.
Importance of Data Scraping for E-Commerce
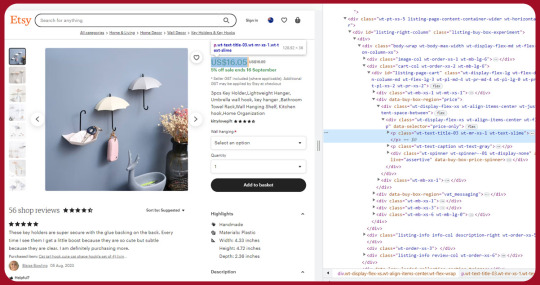
As a business owner, you understand the critical importance of an in-depth understanding of your products to succeed. Comprehensive knowledge of each product, including pricing and specifications, is essential. However, manually collecting such information can be daunting and time-consuming, especially when dealing with a substantial inventory.
It is precisely where data scraping proves invaluable. Data scraping techniques to extract product details from websites enable you to efficiently compile all the information required to enhance your Product Details Pages (PDPs). After determining the specific data types that align with your business model, you can utilize the extracted data for various purposes, including Market Intelligence, Pricing and Competitor Analysis, Product Research and Development, Customer Sentiment Analysis, Inventory Management, and Demand Forecasting.
Usage of JavaScript to Extract Data from Websites

JavaScript stands out as one of the most widely employed programming languages in web development, and its versatility extends to data extraction from websites. JavaScript can be a powerful tool for scraping data from web pages, including valuable information like product descriptions, prices, images, reviews, and more.
Here's a breakdown of how JavaScript extracts data from e-commerce websites efficiently:
Inspect the Webpage: Begin by inspecting the webpage to pinpoint the specific HTML elements containing the data you intend to scrape. The browser's developer tools are handy for this task, allowing you to delve into the webpage's HTML code.
Leverage DOM Manipulation: You can utilize DOM (Document Object Model) manipulation techniques after identifying the HTML elements housing your target data. It uses JavaScript to access and modify these HTML elements, enabling data extraction.
Data Parsing: It is typically unstructured once the data is from HTML elements. You need to parse it into a structured format like JSON or CSV to make it usable. Parsing transforms the raw data into a structured form for analysis.
Automation: For large-scale data extraction from e-commerce websites, automation is critical. While you can write a JavaScript script to automate scraping, employing specialized web scraping tools or programming languages like Python is often more efficient.
Handling Anti-Scraping Measures: Many e-commerce sites employ anti-scraping measures like CAPTCHAs and IP blocking to deter web scrapers. These measures may require rotating proxies or CAPTCHA-solving services to ensure smooth data extraction.
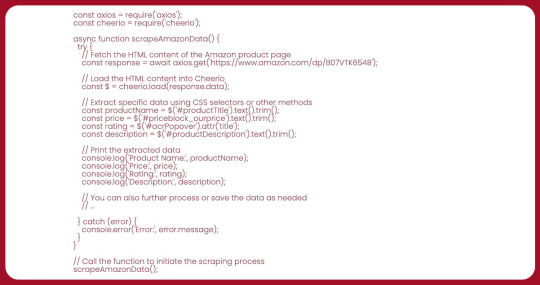
Challenges and Constraints in Extracting Data from E-commerce Websites
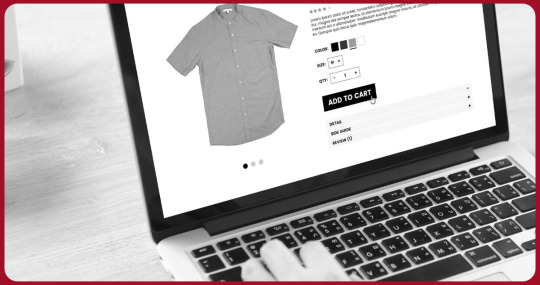
E-commerce data scraping offers valuable insights and competitive advantages but comes with challenges and limitations.
Dynamic Website Changes: One major challenge is the ever-evolving nature of e-commerce websites. These platforms frequently update their designs, making it challenging to develop consistent scraping scripts for reliable data extraction. Furthermore, some e-commerce sites employ anti-scraping techniques like CAPTCHAs or IP blocking to deter automated scraping.
Vast Data Volumes: The sheer volume of data on e-commerce websites can be overwhelming. With millions of products available, large-scale data extraction with e-commerce data scraping services can strain resources and consume significant time. Practical scraping tools must efficiently manage large datasets without causing server overload or technical complications.
Ethical Considerations: Ethical concerns also come into play in e-commerce data scraping. Some scraping practices using e-commerce data scraper may infringe upon copyright laws or violate a website's terms of use, potentially leading to legal repercussions. Moreover, scraping that involves accessing customer data such as emails or purchase histories can be perceived as an invasion of privacy, raising ethical questions.
Navigating these challenges and respecting ethical boundaries is essential for successful and responsible e-commerce data scraping.
Get in touch with iWeb Data Scraping today for more information! Whether you require web scraping service and mobile app data scraping, we've covered you. Don't hesitate to contact us to discuss your specific needs and find out how we can help you with efficient and reliable data scraping solutions.
Know More: https://www.iwebdatascraping.com/e-commerce-data-scraping-on-ten-different-platforms.php
#HowToPerformECommerceDataScraping#ScrapeAmazonecommercedata#AmazonecommercedataScraper#ScrapeRakutenecommercedata#ScrapeTargetecommercedata#ScrapeMacysecommercedata#BestBuyecommercedata
0 notes
Text
How to use JavaScript for data visualization
Data visualization is the process of transforming data into graphical or visual representations, such as charts, graphs, maps, etc. Data visualization can help us understand, analyze, and communicate data more effectively and efficiently.
JavaScript is a powerful and popular programming language that can be used for creating dynamic and interactive data visualizations in web browsers. JavaScript can manipulate the HTML and CSS elements of a web page, as well as fetch data from external sources, such as APIs, databases, or files.
To use JavaScript for data visualization, we need to learn how to:
Select and manipulate HTML elements: We can use JavaScript to select HTML elements by their id, class, tag name, attribute, or CSS selector. We can use methods such as getElementById(), getElementsByClassName(), getElementsByTagName(), querySelector(), or querySelectorAll() to return one or more elements. We can then use properties and methods such as innerHTML, style, setAttribute(), removeAttribute(), appendChild(), removeChild(), etc. to manipulate the selected elements.
Create and modify SVG elements: SVG (Scalable Vector Graphics) is a standard for creating vector graphics in XML format. SVG elements can be scaled without losing quality, and can be styled and animated with CSS and JavaScript. We can use JavaScript to create and modify SVG elements, such as , , , , , etc. We can use methods such as createElementNS(), setAttributeNS(), appendChild(), etc. to manipulate the SVG elements.
Handle events: We can use JavaScript to handle events that occur on the web page, such as mouse clicks, keyboard presses, window resizing, page loading, etc. We can use methods such as addEventListener() or removeEventListener() to attach or detach event handlers to elements. We can also use properties such as onclick, onkeyup, onload, etc. to assign event handlers to elements. An event handler is a function that runs when an event occurs. We can use the event object to access information about the event, such as its type, target, coordinates, key code, etc.
Use AJAX: We can use AJAX (Asynchronous JavaScript and XML) to send and receive data from a server without reloading the web page. We can use objects such as XMLHttpRequest or Fetch API to create and send requests to a server. We can also use methods such as open(), send(), then(), catch(), etc. to specify the request parameters, send the request, and handle the response. We can use properties such as readyState, status, responseText, responseJSON, etc. to access the state, status, and data of the response.
These are some of the basic skills we need to use JavaScript for data visualization. However, writing JavaScript code from scratch for data visualization can be challenging and time-consuming. That’s why many developers use JavaScript libraries and frameworks that provide pre-written code and templates for creating various types of data visualizations.
Some of the most popular JavaScript libraries and frameworks for data visualization are:
D3.js: D3.js (short for Data-Driven Documents) is a JavaScript library for producing dynamic and interactive data visualizations in web browsers. It makes use of SVG, HTML5, and CSS standards. D3.js allows us to bind data to DOM elements, apply data-driven transformations to them, and create custom visualizations with unparalleled flexibility1.
Chart.js: Chart.js is a JavaScript library for creating simple and responsive charts in web browsers. It uses HTML5 canvas element to render the charts. Chart.js supports various types of charts, such as line, bar, pie, doughnut, radar, polar area, bubble, scatter, etc.2
Highcharts: Highcharts is a JavaScript library for creating interactive and high-quality charts in web browsers. It supports SVG and canvas rendering modes. Highcharts supports various types of charts, such as line, spline, area, column, bar, pie, scatter, bubble, gauge, heatmap, treemap, etc. Highcharts also provides features such as zooming, panning, exporting, annotations, drilldowns, etc.
These are some of the popular JavaScript libraries and frameworks for data visualization. To use them in your web development project, you need to follow these steps:
Choose the right library or framework for your project: Depending on your project’s requirements, goals, and preferences, you need to select the most suitable library or framework that can help you create the data visualizations you want. You should consider factors such as the learning curve, documentation, community support, performance, compatibility, scalability, etc. of each library or framework before making a decision.
Add the library or framework to your web page: You can add a library or framework to your web page using either a script tag or an external file. The script tag can be placed either in the head or the body section of the HTML document. The external file can be linked to the HTML document using the src attribute of the script tag.
Learn how to use the library or framework features and functionalities: You need to read the documentation and guides of the library or framework you are using to understand how to use its features and functionalities effectively. You should also follow some tutorials and examples to get familiar with the syntax, conventions, patterns, and best practices of the library or framework.
Write your application code using the library or framework: You need to write your application code using the library or framework functions, methods, objects, components, etc. You should also test and debug your code regularly to ensure its functionality and quality.
These are some of the ways you can use JavaScript for data visualization. To learn more about JavaScript and data visualization, you can visit some of the online resources that offer tutorials, examples, exercises, and quizzes. Some of them are:
[W3Schools]: A website that provides free web development tutorials and references for HTML, CSS, JavaScript, and other web technologies.
[MDN Web Docs]: A website that provides documentation and guides for web developers, covering topics such as HTML, CSS, JavaScript, Web APIs, and more.
[Data Visualization with D3.js]: A website that provides a comprehensive course on data visualization with D3.js.
[e-Tutitions]: A website that provides online courses and for web , development, Learn JavaScript and you can book a free demo class.
[Codecademy]: A website that provides interactive online courses and projects for various programming languages and technologies.
0 notes