#php based frameworks
Explore tagged Tumblr posts
Text
I have been super busy making a little browser game with our worldbuilding. It's an experimental detective adventure... I'm sorta locking in where I'm going with it lately and felt like sharing the little WIPs! It's mostly placeholder art and sketches... And there won't be full animations, but it's been fun to see the talk sprites do things, haha.
It's fully done in PHP/mySQL for the base framework, javascript and jquery. There's so much to do, to draw, to write. Prior to that I only knew how to do stuff on the front end, but I've been slooowly learning to code up stuff and it's been a really fun experience.
That said, it's extremely early in development since I'm still writing more functions and modules, and I barely wrote the intro, but the raw framework is already in place.
(edited my post a little because I long moved past the cookie string system I initially used %))
913 notes
·
View notes
Note
hi hi! love your blog! I am also working on building sites for my portfolio but am a little stumped on how/where to deploy them. would you mind sharing what you are using for deployment? thanks!
Places to Deploy Your Website
Hiya! I know a few places I've tried in the past and some I am yet to try but I know other developers use them!
GitHub pages
GitHub Pages is a free static site hosting service that allows you to publish your website directly from a GitHub repository. It supports HTML, CSS, and JavaScript, as well as Jekyll, a static site generator. I used GitHub pages a lot since I use GitHub to keep all my repositories.
Replit
Replit is a cloud-based development environment that provides an integrated IDE, code editor, and hosting platform all in one place. With Replit, you can easily create and deploy web apps, games, and other projects in multiple programming languages such as Python, HTML, CSS, and JavaScript. I use Replit a lot too for my other much smaller projects that I can’t upload on GitHub to run the program online!
Netlify
Netlify offers a free plan for static site hosting that includes features such as continuous deployment, custom domains, and SSL encryption. It supports HTML, CSS, and JavaScript, as well as serverless functions and other backend technologies.
Heroku
Heroku offers a free plan for hobbyist developers that allows you to deploy up to 5 applications. It supports many languages and frameworks, including Ruby, Node.js, Python, Java, PHP, and Go. Heroku allows free hosting for small applications.
Firebase Hosting
Firebase Hosting is a free service that allows you to host and deploy your web app or static content to a global content delivery network (CDN) with SSL encryption. It supports HTML, CSS, JavaScript, and other static assets. It allows free hosting for small applications.
Surge
Surge is a free static site hosting service that allows you to publish your website with a custom domain or a Surge subdomain. It supports HTML, CSS, JavaScript, and other static assets. Allows free hosting with unlimited bandwidth.
Each of these free deployment options has its own cons such as:
Its lack of server-side functionality
Limited database support
The cost of advanced features
Limited control over the infrastructure
May not be suitable for more complex websites or applications
However, for small projects, I think you’ll be fine with the free options!
Hoped this helps and good luck with your websites’ deployments! 🥰🙌🏾💗
#my asks#codeblr#coding#progblr#programming#studying#studyblr#developer#developers#comp sci#computer science#cs student#cs studyblr#resources#coding resources#deployment#deploy websites#portfolio#deployment websites#tech#web dev
213 notes
·
View notes
Text
Prevent HTTP Parameter Pollution in Laravel with Secure Coding
Understanding HTTP Parameter Pollution in Laravel
HTTP Parameter Pollution (HPP) is a web security vulnerability that occurs when an attacker manipulates multiple HTTP parameters with the same name to bypass security controls, exploit application logic, or perform malicious actions. Laravel, like many PHP frameworks, processes input parameters in a way that can be exploited if not handled correctly.

In this blog, we’ll explore how HPP works, how it affects Laravel applications, and how to secure your web application with practical examples.
How HTTP Parameter Pollution Works
HPP occurs when an application receives multiple parameters with the same name in an HTTP request. Depending on how the backend processes them, unexpected behavior can occur.
Example of HTTP Request with HPP:
GET /search?category=electronics&category=books HTTP/1.1 Host: example.com
Different frameworks handle duplicate parameters differently:
PHP (Laravel): Takes the last occurrence (category=books) unless explicitly handled as an array.
Express.js (Node.js): Stores multiple values as an array.
ASP.NET: Might take the first occurrence (category=electronics).
If the application isn’t designed to handle duplicate parameters, attackers can manipulate input data, bypass security checks, or exploit business logic flaws.
Impact of HTTP Parameter Pollution on Laravel Apps
HPP vulnerabilities can lead to:
✅ Security Bypasses: Attackers can override security parameters, such as authentication tokens or access controls. ✅ Business Logic Manipulation: Altering shopping cart data, search filters, or API inputs. ✅ WAF Evasion: Some Web Application Firewalls (WAFs) may fail to detect malicious input when parameters are duplicated.
How Laravel Handles HTTP Parameters
Laravel processes query string parameters using the request() helper or Input facade. Consider this example:
use Illuminate\Http\Request; Route::get('/search', function (Request $request) { return $request->input('category'); });
If accessed via:
GET /search?category=electronics&category=books
Laravel would return only the last parameter, category=books, unless explicitly handled as an array.
Exploiting HPP in Laravel (Vulnerable Example)
Imagine a Laravel-based authentication system that verifies user roles via query parameters:
Route::get('/dashboard', function (Request $request) { if ($request->input('role') === 'admin') { return "Welcome, Admin!"; } else { return "Access Denied!"; } });
An attacker could manipulate the request like this:
GET /dashboard?role=user&role=admin
If Laravel processes only the last parameter, the attacker gains admin access.
Mitigating HTTP Parameter Pollution in Laravel
1. Validate Incoming Requests Properly
Laravel provides request validation that can enforce strict input handling:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Validator; Route::get('/dashboard', function (Request $request) { $validator = Validator::make($request->all(), [ 'role' => 'required|string|in:user,admin' ]); if ($validator->fails()) { return "Invalid Role!"; } return $request->input('role') === 'admin' ? "Welcome, Admin!" : "Access Denied!"; });
2. Use Laravel’s Input Array Handling
Explicitly retrieve parameters as an array using:
$categories = request()->input('category', []);
Then process them safely:
Route::get('/search', function (Request $request) { $categories = $request->input('category', []); if (is_array($categories)) { return "Selected categories: " . implode(', ', $categories); } return "Invalid input!"; });
3. Encode Query Parameters Properly
Use Laravel’s built-in security functions such as:
e($request->input('category'));
or
htmlspecialchars($request->input('category'), ENT_QUOTES, 'UTF-8');
4. Use Middleware to Filter Requests
Create middleware to sanitize HTTP parameters:
namespace App\Http\Middleware; use Closure; use Illuminate\Http\Request; class SanitizeInputMiddleware { public function handle(Request $request, Closure $next) { $input = $request->all(); foreach ($input as $key => $value) { if (is_array($value)) { $input[$key] = array_unique($value); } } $request->replace($input); return $next($request); } }
Then, register it in Kernel.php:
protected $middleware = [ \App\Http\Middleware\SanitizeInputMiddleware::class, ];
Testing Your Laravel Application for HPP Vulnerabilities
To ensure your Laravel app is protected, scan your website using our free Website Security Scanner.

Screenshot of the free tools webpage where you can access security assessment tools.
You can also check the website vulnerability assessment report generated by our tool to check Website Vulnerability:

An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
Conclusion
HTTP Parameter Pollution can be a critical vulnerability if left unchecked in Laravel applications. By implementing proper validation, input handling, middleware sanitation, and secure encoding, you can safeguard your web applications from potential exploits.
🔍 Protect your website now! Use our free tool for a quick website security test and ensure your site is safe from security threats.
For more cybersecurity updates, stay tuned to Pentest Testing Corp. Blog! 🚀
3 notes
·
View notes
Text
Web Designer vs Web Developer : Quelle carrière choisir ?
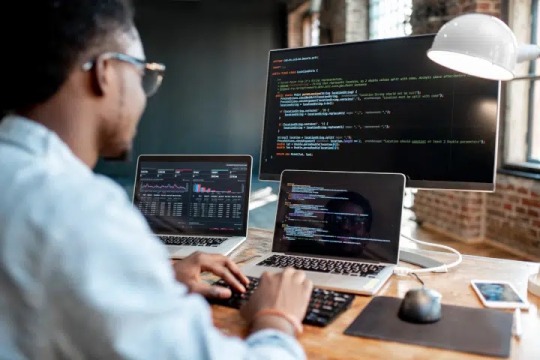
Avec l’évolution rapide du numérique, les carrières en conception et développement web restent très demandées. Bien que ces deux professions jouent un rôle clé dans la création de sites modernes, elles impliquent des compétences, des responsabilités et des perspectives différentes. Si vous hésitez entre ces deux parcours, ce guide vous aidera à comprendre les différences en termes de rôles, de potentiel de rémunération et d’opportunités à long terme afin de choisir la carrière qui vous convient le mieux.
Que fait un Web Designer ?
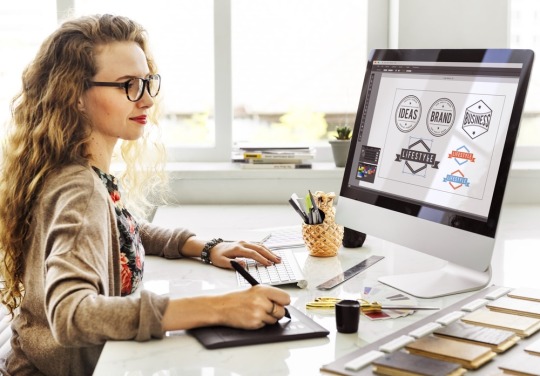
Responsabilités principales
Un web designer se concentre sur l’apparence, l’ergonomie et l’expérience utilisateur (UX) d’un site web. Ses tâches incluent :
• Créer des mises en page visuellement attrayantes et des wireframes.
• Sélectionner la typographie, les palettes de couleurs et les éléments de branding.
• Concevoir des sites responsifs et adaptés aux mobiles.
• Utiliser des outils comme Adobe XD, Figma et Sketch.
• Collaborer avec les développeurs pour assurer une bonne transition entre le design et le code.
Compétences clés pour un Web Designer
• Connaissance des principes UI/UX et des meilleures pratiques en ergonomie.
• Maîtrise des logiciels de design graphique (Photoshop, Illustrator, Figma).
• Notions de base en HTML & CSS pour le prototypage.
• Compréhension du branding et de la psychologie des couleurs.
Avantages d’être Web Designer
✔️ Travail très créatif avec une grande liberté artistique.
✔️ Moins de programmation que dans le développement web.
✔️ Forte demande pour l’expertise UI/UX.
✔️ Nombreuses opportunités en freelance, notamment sur des plateformes comme Upwork.
Défis du métier de Web Designer
❌ Rémunération souvent inférieure à celle des développeurs.
❌ Nécessité de suivre en permanence les tendances du design.
❌ Certains clients sous-estiment la valeur du design par rapport au développement.
Que fait un Web Developer ?
Responsabilités principales
Un développeur web est chargé de concevoir et maintenir des sites fonctionnels en utilisant des langages de programmation et des frameworks. Ses missions incluent :
• Écrire et corriger du code pour implémenter des fonctionnalités web.
• Optimiser la vitesse, la sécurité et la scalabilité des sites.
• Gérer les systèmes backend, les bases de données et les API.
• Corriger les bugs et améliorer l’expérience utilisateur.
• Travailler aussi bien sur les technologies front-end que back-end.
Les différents types de développeurs web
• Développeur Front-End : Se concentre sur les aspects visuels et interactifs du site (HTML, CSS, JavaScript).
• Développeur Back-End : Gère la programmation côté serveur, les bases de données et les API.
• Développeur Full-Stack : Maîtrise à la fois le front-end et le back-end.
Compétences clés pour un Web Developer
• Maîtrise de HTML, CSS et JavaScript.
• Connaissance de langages de programmation comme PHP, Python, Ruby.
• Familiarité avec des frameworks comme React, Angular, Vue.js.
• Gestion des bases de données (MySQL, MongoDB).
• Compétences en débogage, tests et gestion de versions.
Avantages d’être Web Developer
✔️ Forte rémunération, en particulier pour les développeurs full-stack et back-end.
✔️ Demande croissante avec de nombreuses opportunités d’évolution.
✔️ Possibilité de travailler sur des projets complexes et de grande envergure.
✔️ Opportunités de travail à distance et en freelance.
Défis du métier de Web Developer
❌ Apprentissage continu indispensable en raison des évolutions technologiques rapides.
❌ Travail très technique avec peu d’aspects créatifs.
❌ Peut impliquer de longues heures de débogage et de résolution de problèmes.
Conclusion
En résumé, si vous aimez la créativité et le design, le métier de web designer pourrait vous convenir. Si vous préférez la programmation et la résolution de problèmes techniques, le développement web est une meilleure option. Quelle que soit votre décision, les deux carrières offrent de nombreuses opportunités dans l’univers du numérique !
Je suis hermane junior Nguessan developpeur web &mobile
Liens linkedIn 👉 : https://www.linkedin.com/in/hermane-junior-nguessan-2a9a05324?utm_source=share&utm_campaign=share_via&utm_content=profile&utm_medium=ios_app
https://www.linkedin.com/in/hermane-junior-nguessan-2a9a05324?utm_source=share&utm_campaign=share_via&utm_content=profile&utm_medium=ios_app
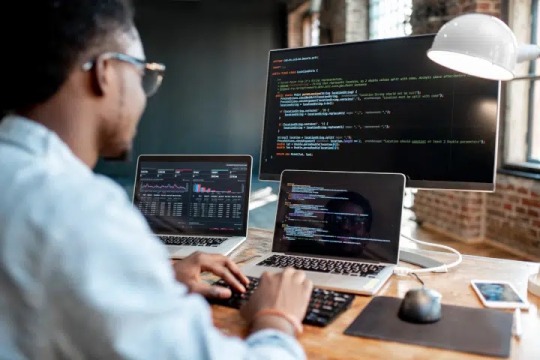
2 notes
·
View notes
Text
When deciding between Laravel and Symfony, it's essential to consider your project needs. Laravel shines with its user-friendly syntax and extensive out-of-the-box functionalities, making it ideal for rapid development and handling common web application tasks with ease. Its vibrant community and ecosystem offer a wide range of packages, which can be a huge time-saver for developers looking to implement complex features quickly.
On the other hand, Symfony is known for its robustness, flexibility, and scalability, making it a preferred choice for large, enterprise-level applications. With a component-based architecture, Symfony allows developers to pick and choose components, making it highly customizable. If you value performance and long-term support, Symfony might be the better choice, while Laravel is perfect for projects needing fast deployment and intuitive development.
2 notes
·
View notes
Text
SQLi Potential Mitigation Measures
Phase: Architecture and Design
Strategy: Libraries or Frameworks
Use a vetted library or framework that prevents this weakness or makes it easier to avoid. For example, persistence layers like Hibernate or Enterprise Java Beans can offer protection against SQL injection when used correctly.
Phase: Architecture and Design
Strategy: Parameterization
Use structured mechanisms that enforce separation between data and code, such as prepared statements, parameterized queries, or stored procedures. Avoid constructing and executing query strings with "exec" to prevent SQL injection [REF-867].
Phases: Architecture and Design; Operation
Strategy: Environment Hardening
Run your code with the minimum privileges necessary for the task [REF-76]. Limit user privileges to prevent unauthorized access if an attack occurs, such as by ensuring database applications don’t run as an administrator.
Phase: Architecture and Design
Duplicate client-side security checks on the server to avoid CWE-602. Attackers can bypass client checks by altering values or removing checks entirely, making server-side validation essential.
Phase: Implementation
Strategy: Output Encoding
Avoid dynamically generating query strings, code, or commands that mix control and data. If unavoidable, use strict allowlists, escape/filter characters, and quote arguments to mitigate risks like SQL injection (CWE-88).
Phase: Implementation
Strategy: Input Validation
Assume all input is malicious. Use strict input validation with allowlists for specifications and reject non-conforming inputs. For SQL queries, limit characters based on parameter expectations for attack prevention.
Phase: Architecture and Design
Strategy: Enforcement by Conversion
For limited sets of acceptable inputs, map fixed values like numeric IDs to filenames or URLs, rejecting anything outside the known set.
Phase: Implementation
Ensure error messages reveal only necessary details, avoiding cryptic language or excessive information. Store sensitive error details in logs but be cautious with content visible to users to prevent revealing internal states.
Phase: Operation
Strategy: Firewall
Use an application firewall to detect attacks against weaknesses in cases where the code can’t be fixed. Firewalls offer defense in depth, though they may require customization and won’t cover all input vectors.
Phases: Operation; Implementation
Strategy: Environment Hardening
In PHP, avoid using register_globals to prevent weaknesses like CWE-95 and CWE-621. Avoid emulating this feature to reduce risks. source
3 notes
·
View notes
Text
i bought a domain for my family to share i couldnt delibrareate what framework to use cuz im trying. to accommodate Pet Site on it. but i finilly decided on slim framework 4 + twig templates + doctrine. so im trying to figure out how that all waorks but i need to set up a homepage on my new domain
i will be honest neocities has SUCKED In recent years their server goes down constantly with no warning/maintenace logs. both your site frontend and the site editors. so visitors cant even access your site at random and its been happening more and more. like wtf is the issue. i used to pay for premium but like its not worth it at $5/mo..... just switch to a dedicated server host!!!!!! its actually cheaper unless you specifically want more than like 10 static sites. im using hostinger.
also hostinger used to have 000webhost which is ran my test site and they nuked it >:( so i switched my test site to infinityfree. which is running lovely. and support seems responsive there and helps you figure out how to work around free account limitations, like no domain e-mail.
anyway i was working off an old pet site framework called mysidia addoptables from like 2011 (last release was 2021) but recently i decided i cant work with it anymore it's too janky, dated, and just messed up 😅 i love the community that still hangs around it but the code is FUUUUUUCKED. so i'm starting from scratch...
this is also still my firsrt foray into php but mysidia has taught me BAD HABITS.... i literally have a folder of screenshots where i just screencap the code and soyjak at how bad it is. (why did you install smarty template and htmlpurifier and then... barely use them..........)
ANYWAYS. all my pet site progress is no longer very easy to catalog cuz it's just boring code. but by god it's easier to figure out how to roadmap it now that i'm not working with the stupidiest base code. i guess you can look at these upcoming pets tho :^) (wont be released til i draw all their other painted forms)
2 notes
·
View notes
Text
Which programming language performs better on the web, Java, C#, PHP, or Python?
The performance of a programming language on the web depends on various factors such as the specific requirements of the project, the expertise of the development team, and the scalability of the language. Each programming language you mentioned—Java, C#, PHP, and Python—has its strengths and use cases:
Java: Known for its performance, scalability, and security, Java is widely used in enterprise-level web applications, especially for large-scale projects. It's commonly used in backend development and is favored for its robustness and ability to handle high loads.
C#: Similar to Java, C# is often used in enterprise-level web development, particularly for building applications on the Microsoft .NET framework. It offers strong performance and is well-suited for Windows-based environments.
PHP: PHP is a server-side scripting language specifically designed for web development. It's known for its simplicity, ease of use, and extensive community support. PHP powers a large portion of the web, particularly in content management systems like WordPress and e-commerce platforms like Magento.
Python: Python is a versatile language that is gaining popularity in web development due to its simplicity, readability, and extensive libraries. It's commonly used in web frameworks like Django and Flask, making it suitable for building web applications of various scales.
Ultimately, the best programming language for web development depends on the specific requirements of your project, the preferences of your development team, and the goals of your business. Each language has its advantages and trade-offs, so it's essential to evaluate them based on your project's needs.
4 notes
·
View notes
Text
Comparing Laravel And WordPress: Which Platform Reigns Supreme For Your Projects? - Sohojware
Choosing the right platform for your web project can be a daunting task. Two popular options, Laravel and WordPress, cater to distinct needs and offer unique advantages. This in-depth comparison by Sohojware, a leading web development company, will help you decipher which platform reigns supreme for your specific project requirements.
Understanding Laravel
Laravel is a powerful, open-source PHP web framework designed for the rapid development of complex web applications. It enforces a clean and modular architecture, promoting code reusability and maintainability. Laravel offers a rich ecosystem of pre-built functionalities and tools, enabling developers to streamline the development process.
Here's what makes Laravel stand out:
MVC Architecture: Laravel adheres to the Model-View-Controller (MVC) architectural pattern, fostering a well-organized and scalable project structure.
Object-Oriented Programming: By leveraging object-oriented programming (OOP) principles, Laravel promotes code clarity and maintainability.
Built-in Features: Laravel boasts a plethora of built-in features like authentication, authorization, caching, routing, and more, expediting the development process.
Artisan CLI: Artisan, Laravel's powerful command-line interface (CLI), streamlines repetitive tasks like code generation, database migrations, and unit testing.
Security: Laravel prioritizes security by incorporating features like CSRF protection and secure password hashing, safeguarding your web applications.
However, Laravel's complexity might pose a challenge for beginners due to its steeper learning curve compared to WordPress.
Understanding WordPress
WordPress is a free and open-source content management system (CMS) dominating the web. It empowers users with a user-friendly interface and a vast library of plugins and themes, making it ideal for creating websites and blogs without extensive coding knowledge.
Here's why WordPress is a popular choice:
Ease of Use: WordPress boasts an intuitive interface, allowing users to create and manage content effortlessly, even with minimal technical expertise.
Flexibility: A vast repository of themes and plugins extends WordPress's functionality, enabling customization to suit diverse website needs.
SEO Friendliness: WordPress is inherently SEO-friendly, incorporating features that enhance your website's ranking.
Large Community: WordPress enjoys a massive and active community, providing abundant resources, tutorials, and support.
While user-friendly, WordPress might struggle to handle complex functionalities or highly customized web applications.
Choosing Between Laravel and WordPress
The optimal platform hinges on your project's specific requirements. Here's a breakdown to guide your decision:
Laravel is Ideal For:
Complex web applications require a high degree of customization.
Projects demanding powerful security features.
Applications with a large user base or intricate data structures.
Websites require a high level of performance and scalability.
WordPress is Ideal For:
Simple websites and blogs.
Projects with a primary focus on content management.
E-commerce stores with basic product management needs (using WooCommerce plugin).
Websites requiring frequent content updates by non-technical users.
Sohojware, a well-versed web development company in the USA, can assist you in making an informed decision. Our team of Laravel and WordPress experts will assess your project's needs and recommend the most suitable platform to ensure your web project's success.
In conclusion, both Laravel and WordPress are powerful platforms, each catering to distinct project needs. By understanding their strengths and limitations, you can make an informed decision that empowers your web project's success. Sohojware, a leading web development company in the USA, possesses the expertise to guide you through the selection process and deliver exceptional results, regardless of the platform you choose. Let's leverage our experience to bring your web vision to life.
FAQs about Laravel and WordPress Development by Sohojware
1. Which platform is more cost-effective, Laravel or WordPress?
While WordPress itself is free, ongoing maintenance and customization might require development expertise. Laravel projects typically involve developer costs, but these can be offset by the long-term benefits of a custom-built, scalable application. Sohojware can provide cost-effective solutions for both Laravel and WordPress development.
2. Does Sohojware offer support after project completion?
Sohojware offers comprehensive post-development support for both Laravel and WordPress projects. Our maintenance and support plans ensure your website's continued functionality, security, and performance.
3. Can I migrate my existing website from one platform to another?
Website migration is feasible, but the complexity depends on the website's size and architecture. Sohojware's experienced developers can assess the migration feasibility and execute the process seamlessly.
4. How can Sohojware help me with Laravel or WordPress development?
Sohojware offers a comprehensive range of Laravel and WordPress development services, encompassing custom development, theme and plugin creation, integration with third-party applications, and ongoing maintenance.
5. Where can I find more information about Sohojware's Laravel and WordPress development services?
You can find more information about Sohojware's Laravel and WordPress development services by visiting our website at https://sohojware.com/ or contacting our sales team directly. We'd happily discuss your project requirements and recommend the most suitable platform to achieve your goals.
3 notes
·
View notes
Text
Welcome to a random diary involving code✭
-> my name is laura, based in brazil
-> informational systems student
-> i like to post about code and my progress in it
-> i like to also post some tips to help people grow in code too!
-> currently, my main language is Python (using Django's framework too), but I also work with these technologies:
• C#
• PHP
• JS
• HTML & CSS
• Bootstrap
• MySQL
• GIT
4 notes
·
View notes
Text
Revolutionizing the Digital Landscape: Technoviaan Software's Comprehensive IT Services
In the ever-evolving digital landscape, businesses require innovative solutions to stay ahead of the curve. Introducing Technoviaan Software, a trailblazer in the realm of Information Technology services. With a profound expertise spanning across various cutting-edge technologies, Technoviaan Software stands as a beacon of excellence, offering unparalleled services tailored to meet the diverse needs of modern businesses.Empowering Businesses with Technological BrillianceAt Technoviaan Software, we understand the pivotal role technology plays in shaping the success of businesses in today's competitive environment. Leveraging our expertise in a multitude of technologies, we offer comprehensive IT services designed to empower businesses across various domains.Expertise in Key Technologies.NET Development: Our skilled team of developers excels in crafting robust and scalable solutions using the .NET framework. Whether it's building dynamic web applications or enterprise-level software, we ensure unparalleled performance and reliability.Java Development: With a deep-rooted understanding of Java technology, we deliver tailored solutions that drive business growth. From enterprise application development to Java-based web solutions, our expertise knows no bounds.PHP Development: Technoviaan Software pioneers in PHP development, delivering high-performance websites and applications that resonate with your audience. Our proficiency in PHP frameworks like Laravel ensures swift development cycles and exceptional results.Python Development: Python's versatility is at the core of our development philosophy. Our Python experts harness the power of this language to create data-driven solutions, machine learning algorithms, and automation scripts that propel businesses forward.Digital Marketing: In the digital realm, visibility is paramount. Technoviaan Software offers bespoke digital marketing solutions tailored to amplify your online presence. From SEO strategies to targeted PPC campaigns, we deploy tactics that drive tangible results and maximize ROI.Blockchain Development: Embrace the future of technology with our blockchain development services. Whether it's implementing smart contracts, building decentralized applications, or exploring cryptocurrency solutions, Technoviaan Software is your trusted partner in blockchain innovation.Unmatched Commitment to ExcellenceWhat sets Technoviaan Software apart is our unwavering commitment to excellence. We strive to understand the unique requirements of each client and deliver solutions that exceed expectations. With a customer-centric approach and a penchant for innovation, we forge long-lasting partnerships built on trust and mutual success.Experience the Technoviaan AdvantageIn a world where technology reigns supreme, Technoviaan Software emerges as a beacon of innovation and expertise. Whether you're a startup venturing into uncharted territories or an established enterprise seeking to revitalize your digital presence, we have the tools, the talent, and the tenacity to propel you towards success.Experience the Technoviaan advantage today and embark on a journey of digital transformation like never before. Your success is our mission, and together, we'll shape a future fueled by technological brilliance.Connect with us today and unlock the limitless possibilities of technology with Technoviaan Software.
#it services#software developers#software development#software services#software solutions#.net development#phpdevelopment#flutter app development#web development#it staffing services#technology#ios app development#android app development#Software
3 notes
·
View notes
Text
Idiosys USA is a leading minnesota-based web development agency, providing the best standard web development, app development, digital marketing, and software development consulting services in Minnesota and all over the United States. We have a team of 50+ skilled IT professionals to provide world-class IT support to all sizes of industries in different domains. We are a leading Minnesota web design company that works for healthcare-based e-commerce, finance organisations business websites, the News Agency website and mobile applications, travel and tourism solutions, transport and logistics management systems, and e-commerce applications. Our team is skilled in the latest technologies like React, Node JS, Angular, and Next JS. We also worked with open-source PHP frameworks like Laravel, Yii2, and others. At Idiosys USA, you will get complete web development solutions. We have some custom solutions for different businesses, but our expertise is in custom website development according to clients requirements. We believe that we are best in cities.
#web development agency#website development company in usa#software development consulting#minnesota web design#hire web developer#hire web designer#web developer minneapolis#minneapolis web development#website design company#web development consulting#web development minneapolis#minnesota web developers#web design company minneapolis
2 notes
·
View notes
Text
TOP 3 Web Development Course in Chandigarh
Looking for excellence in web development training? Our web development course in Chandigarh offers top-notch instruction in HTML, CSS, JavaScript, and more. With a focus on practical projects and personalized mentorship, we ensure you gain the skills needed to excel in the field. Enroll now for the best web development course in Chandigarh at Excellence Technology
Welcome to Excellence Technology's Web Development Course!
Are you ready to unlock your potential in the world of web development? Look no further than Excellence Technology's comprehensive web development course. Whether you're a beginner or an experienced programmer looking to enhance your skills, our course is designed to cater to all levels of expertise.
Our web development course is carefully crafted to provide you with the knowledge and practical skills needed to excel in this rapidly evolving field. Led by industry experts, our instructors bring a wealth of experience and up-to-date insights to guide you through the intricacies of web development.
Here's what you can expect from our course:
Fundamentals of Web Development: Gain a solid foundation in HTML, CSS, and JavaScript, the building blocks of the web. Understand how these technologies work together to create visually appealing and interactive websites.
Front-End Development: Dive into the world of front-end development, where you'll learn how to create engaging user interfaces and responsive designs. Master popular frameworks like React and Angular to build dynamic web applications.
Back-End Development: Explore the back-end technologies that power websites and web applications. Learn server-side programming languages such as Python, PHP, or Node.js, and work with databases like MySQL or MongoDB to handle data storage and retrieval.
Full-Stack Development: Get a holistic understanding of web development by combining front-end and back-end skills. Become proficient in both client-side and server-side programming, enabling you to build end-to-end web solutions.
Project-Based Learning: Apply your newfound knowledge through hands-on projects. From building a personal portfolio website to creating a fully functional e-commerce platform, our course projects will challenge you to think creatively and solve real-world problems.
Industry Best Practices: Stay updated with the latest industry standards and practices. Learn about version control, testing, deployment, and optimization techniques to ensure your websites are secure, efficient, and scalable.
Career Support: Our commitment to your success extends beyond the classroom. Benefit from our career support services, including resume building, interview preparation, and job placement assistance. We'll help you showcase your skills and connect with potential employers in the web development industry.
Now I tell you about TOP 3 Institution for Web Development course
•https://www.excellencetechnology.in/
•https://extechdigital.in/
•https://thebrightnext.com/
At Excellence Technology, we believe in providing a nurturing and inclusive learning environment. Join our web development course and become part of a vibrant community of learners, where you can collaborate, network, and grow together.
Don't miss this opportunity to embark on an exciting journey into the world of web development. Enroll in Excellence Technology's Web Development Course today and unlock your potential in this ever-expanding field.
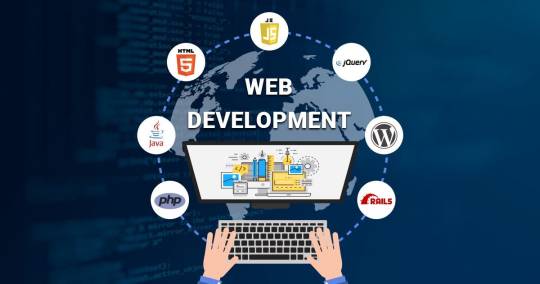
Connect with us
If you do not like to fill up the form above, contact us at the following details, we will be happy to connect.
Email: [email protected]
Phone: +91 9317788822, 93562-55522
#webdesign#webdevelopment#webdesigner#userexperience#responsive web design#webdesigninspiration#web design agency#websitedesign#web design services#graphic design#webdesigncommunity#uxdesign#frontenddevelopment#Webdesignlife#web design company#creativewebdesign
7 notes
·
View notes
Text
Advanced Web Development Tools For Efficient PHP Developers
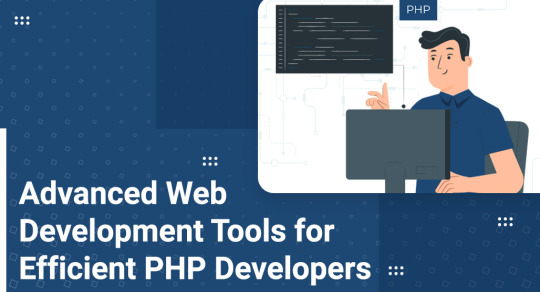
Web Development is working with frontend and backend both languages that are used by programmers and developers to build a well-functioning website or web application. Some of the most commonly used frontend languages are JavaScript, Angular, React, and Vue and backend languages are Python, Java, PHP, and C#.
PHP is the fastest and most widely used server-side scripting language that is ubiquitous in websites and web app development. It is known as a general-purpose scripting language that can be used to develop dynamic and interactive websites. It offers plenty of frameworks and supports several automation tools for application testing and deployment.
Nowadays the market is flooded with many PHP tools, and choosing the right one from among them is a challenging task. Here, we have made a list of leading tools that your development team can utilize to build feature-rich and innovative PHP webs and applications in 2023.
PHPStorm
PHPStorm is the most standard choice because of its nature of being lightweight, extremely fast, and smooth. It simplifies time-consuming tasks such as static code analysis, debugging, and generating functions. PHPStorm is compatible with many frameworks like Symphony, Drupal, Zend, WordPress, Magento, Laravel, etc.
Sublime Text
One of the most customizable, as well as malleable text editors out there for PHP developers, is Sublime Text. This cross-platform source code editor natively supports multiple coding and native mark-up languages.
AWS Cloud9
One of the main benefits of AWS Cloud9 is it is a cloud-based service, which means that it can be accessed from any computer with an internet connection. AWS Cloud9 comes with a code editor, terminal, debugger, and all necessary programming language tools, such as JavaScript, Python, and PHP, etc. It is an impressive feature-filled software that facilitates real-time code collaboration.
Netbeans
Netbeans supports building large-scale web apps and offers a range of languages like English, Simplified Chinese, Brazilian, Japanese, Portuguese, and Russian. It is considered one of the best software for PHP development and is most commonly used for developing PHP applications.
Aptana Studio
Aptana Studio is an open-source integrated development environment (IDE) for building dynamic web applications that use AJAX, PHP, JavaScript, and Ruby on Rails as well as HTML and CSS. It is enabling developers to develop web apps quickly and easily. Its core features include a code assist, built-in terminal, deployment wizard, and integrated debugger.
CodeLobster
CodeLobster IDE from Codelobster software company is the most popular well-liked PHP dev tool for the developer community. It endorses most PHP frameworks like Drupal, Joomla, Symfony, CodeIgniter, Magneto, CakePHP, etc. It supports numerous plugins and a PHP debugger to validate code. It can accelerate and simplify the development process.
Eclipse
It has a huge community of developers who work on all sorts of plug-ins and equip Eclipse with features. Eclipse features include the Eclipse ecosystem, code assist, syntax highlighting, code templates, syntax validation, code formatting, code navigation, and PHP debugging. These features make it easier for you to write better code.
Zend Studio
Zend Studio is a complete Integrated Development Environment (IDE) for professional PHP Web development, debugging, deploying, and managing PHP apps. It is a suitable option for anyone who wants to develop web applications with PHP version 5.5 and above. It offers fast coding along with an intelligent code editor and integrates with Zend Debugger, X-ray, and Xdebug.
These PHP web tools will set up configuration, complete your code intelligently, help to navigate, and check for errors. They are generally open-source and offer excellent features like syntax highlighting and debugging. They will help increase your productivity and efficiency. If you’re planning to get a web app with PHP, then you can contact Swayam Infotech and schedule a meeting for a detailed discussion. Our PHP experts will guide you with your requirements in a perfect way.
#PHP#php developer#php developers#php development#php development solutions#PHP Web Development#php website#php website development#phpdevelopment#Web Developers#Web Development#web development services#website Development#websites
5 notes
·
View notes
Text
JavaScript Frameworks
Step 1) Polyfill
Most JS frameworks started from a need to create polyfills. A Polyfill is a js script that add features to JavaScript that you expect to be standard across all web browsers. Before the modern era; browsers lacked standardization for many different features between HTML/JS/and CSS (and still do a bit if you're on the bleeding edge of the W3 standards)
Polyfill was how you ensured certain functions were available AND worked the same between browsers.
JQuery is an early Polyfill tool with a lot of extra features added that makes JS quicker and easier to type, and is still in use in most every website to date. This is the core standard of frameworks these days, but many are unhappy with it due to performance reasons AND because plain JS has incorporated many features that were once unique to JQuery.
JQuery still edges out, because of the very small amount of typing used to write a JQuery app vs plain JS; which saves on time and bandwidth for small-scale applications.
Many other frameworks even use JQuery as a base library.
Step 2) Encapsulated DOM
Storing data on an element Node starts becoming an issue when you're dealing with multiple elements simultaneously, and need to store data as close as possible to the DOMNode you just grabbed from your HTML, and probably don't want to have to search for it again.
Encapsulation allows you to store your data in an object right next to your element so they're not so far apart.
HTML added the "data-attributes" feature, but that's more of "loading off the hard drive instead of the Memory" situation, where it's convenient, but slow if you need to do it multiple times.
Encapsulation also allows for promise style coding, and functional coding. I forgot the exact terminology used,but it's where your scripting is designed around calling many different functions back-to-back instead of manipulating variables and doing loops manually.
Step 3) Optimization
Many frameworks do a lot of heavy lifting when it comes to caching frequently used DOM calls, among other data tools, DOM traversal, and provides standardization for commonly used programming patterns so that you don't have to learn a new one Everytime you join a new project. (you will still have to learn a new one if you join a new project.)
These optimizations are to reduce reflowing/redrawing the page, and to reduce the plain JS calls that are performance reductive. A lot of these optimatizations done, however, I would suspect should just be built into the core JS engine.
(Yes I know it's vanilla JS, I don't know why plain is synonymous with Vanilla, but it feels weird to use vanilla instead of plain.)
Step 4) Custom Element and component development
This was a tool to put XML tags or custom HTML tags on Page that used specific rules to create controls that weren't inherent to the HTML standard. It also helped linked multiple input and other data components together so that the data is centrally located and easy to send from page to page or page to server.
Step 5) Back-end development
This actually started with frameworks like PHP, ASP, JSP, and eventually resulted in Node.JS. these were ways to dynamically generate a webpage on the server in order to host it to the user. (I have not seen a truly dynamic webpage to this day, however, and I suspect a lot of the optimization work is actually being lost simply by programmers being over reliant on frameworks doing the work for them. I have made this mistake. That's how I know.)
The backend then becomes disjointed from front-end development because of the multitude of different languages, hence Node.JS. which creates a way to do server-side scripting in the same JavaScript that front-end developers were more familiar with.
React.JS and Angular 2.0 are more of back end frameworks used to generate dynamic web-page without relying on the User environment to perform secure transactions.
Step 6) use "Framework" as a catch-all while meaning none of these;
Polyfill isn't really needed as much anymore unless your target demographic is an impoverished nation using hack-ware and windows 95 PCs. (And even then, they could possible install Linux which can use modern lightweight browsers...)
Encapsulation is still needed, as well as libraries that perform commonly used calculations and tasks, I would argue that libraries aren't going anywhere. I would also argue that some frameworks are just bloat ware.
One Framework I was researching ( I won't name names here) was simply a remapping of commands from a Canvas Context to an encapsulated element, and nothing more. There was literally more comments than code. And by more comments, I mean several pages of documentation per 3 lines of code.
Custom Components go hand in hand with encapsulation, but I suspect that there's a bit more than is necessary with these pieces of frameworks, especially on the front end. Tho... If it saves a lot of repetition, who am I to complain?
Back-end development is where things get hairy, everything communicates through HTTP and on the front end the AJAX interface. On the back end? There's two ways data is given, either through a non-html returning web call, *or* through functions that do a lot of heavy lifting for you already.
Which obfuscates how the data is used.
But I haven't really found a bad use of either method. But again; I suspect many things about performance impacts that I can't prove. Specifically because the tools in use are already widely accepted and used.
But since I'm a lightweight reductionist when it comes to coding. (Except when I'm not because use-cases exist) I can't help but think most every framework work, both front-end and Back-end suffers from a lot of bloat.
And that bloat makes it hard to select which framework would be the match for the project you're working on. And because of that; you could find yourself at the tail end of a development cycle realizing; You're going to have to maintain this as is, in the exact wrong solution that does not fit the scope of the project in anyway.
Well. That's what junior developers are for anyway...
2 notes
·
View notes