#oauth2 authentication spring boot
Explore tagged Tumblr posts
Text
Spring Security Using Facebook Authorization: A Comprehensive Guide
In today's digital landscape, integrating third-party login mechanisms into applications has become a standard practice. It enhances user experience by allowing users to log in with their existing social media accounts. In this blog post, we will walk through the process of integrating Facebook authorization into a Spring Boot application using Spring Security.
Table of Contents
Introduction
Prerequisites
Setting Up Facebook Developer Account
Creating a Spring Boot Application
Configuring Spring Security for OAuth2 Login
Handling Facebook User Data
Testing the Integration
Conclusion
1. Introduction
OAuth2 is an open standard for access delegation, commonly used for token-based authentication. Facebook, among other social media platforms, supports OAuth2, making it possible to integrate Facebook login into your Spring Boot application.
2. Prerequisites
Before we start, ensure you have the following:
JDK 11 or later
Maven
An IDE (e.g., IntelliJ IDEA or Eclipse)
A Facebook Developer account
3. Setting Up Facebook Developer Account
To use Facebook login, you need to create an app on the Facebook Developer portal:
Go to the Facebook Developer website and log in.
Click on "My Apps" and then "Create App."
Choose an app type (e.g., "For Everything Else") and provide the required details.
Once the app is created, go to "Settings" > "Basic" and note down the App ID and App Secret.
Add a product, select "Facebook Login," and configure the Valid OAuth Redirect URIs to http://localhost:8080/login/oauth2/code/facebook.
4. Creating a Spring Boot Application
Create a new Spring Boot project with the necessary dependencies. You can use Spring Initializr or add the dependencies manually to your pom.xml.
Dependencies
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies>
5. Configuring Spring Security for OAuth2 Login
Next, configure Spring Security to use Facebook for OAuth2 login.
application.properties
Add your Facebook app credentials to src/main/resources/application.properties.spring.security.oauth2.client.registration.facebook.client-id=YOUR_FACEBOOK_APP_ID spring.security.oauth2.client.registration.facebook.client-secret=YOUR_FACEBOOK_APP_SECRET spring.security.oauth2.client.registration.facebook.redirect-uri-template={baseUrl}/login/oauth2/code/{registrationId} spring.security.oauth2.client.registration.facebook.scope=email,public_profile spring.security.oauth2.client.registration.facebook.client-name=Facebook spring.security.oauth2.client.registration.facebook.authorization-grant-type=authorization_code spring.security.oauth2.client.provider.facebook.authorization-uri=https://www.facebook.com/v11.0/dialog/oauth spring.security.oauth2.client.provider.facebook.token-uri=https://graph.facebook.com/v11.0/oauth/access_token spring.security.oauth2.client.provider.facebook.user-info-uri=https://graph.facebook.com/me?fields=id,name,email spring.security.oauth2.client.provider.facebook.user-name-attribute=id
Security Configuration
Create a security configuration class to handle the OAuth2 login.import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.oauth2.client.oidc.userinfo.OidcUserService; import org.springframework.security.oauth2.client.userinfo.DefaultOAuth2UserService; import org.springframework.security.oauth2.client.userinfo.OAuth2UserService; import org.springframework.security.oauth2.core.oidc.user.OidcUser; import org.springframework.security.oauth2.core.user.OAuth2User; import org.springframework.security.web.authentication.SimpleUrlAuthenticationFailureHandler; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests(authorizeRequests -> authorizeRequests .antMatchers("/", "/error", "/webjars/**").permitAll() .anyRequest().authenticated() ) .oauth2Login(oauth2Login -> oauth2Login .loginPage("/login") .userInfoEndpoint(userInfoEndpoint -> userInfoEndpoint .oidcUserService(this.oidcUserService()) .userService(this.oAuth2UserService()) ) .failureHandler(new SimpleUrlAuthenticationFailureHandler()) ); } private OAuth2UserService<OidcUserRequest, OidcUser> oidcUserService() { final OidcUserService delegate = new OidcUserService(); return (userRequest) -> { OidcUser oidcUser = delegate.loadUser(userRequest); // Custom logic here return oidcUser; }; } private OAuth2UserService<OAuth2UserRequest, OAuth2User> oAuth2UserService() { final DefaultOAuth2UserService delegate = new DefaultOAuth2UserService(); return (userRequest) -> { OAuth2User oAuth2User = delegate.loadUser(userRequest); // Custom logic here return oAuth2User; }; } }
6. Handling Facebook User Data
After a successful login, you might want to handle and display user data.
Custom User Service
Create a custom service to process user details.import org.springframework.security.oauth2.core.user.OAuth2User; import org.springframework.security.oauth2.core.user.OAuth2UserAuthority; import org.springframework.security.oauth2.client.userinfo.OAuth2UserService; import org.springframework.security.oauth2.client.oidc.userinfo.OidcUserService; import org.springframework.security.oauth2.core.oidc.user.OidcUser; import org.springframework.security.oauth2.client.userinfo.DefaultOAuth2UserService; import org.springframework.security.oauth2.client.oidc.userinfo.OidcUserRequest; import org.springframework.security.oauth2.client.userinfo.OAuth2UserRequest; import org.springframework.stereotype.Service; import java.util.Map; import java.util.Set; import java.util.HashMap; @Service public class CustomOAuth2UserService implements OAuth2UserService<OAuth2UserRequest, OAuth2User> { private final DefaultOAuth2UserService delegate = new DefaultOAuth2UserService(); @Override public OAuth2User loadUser(OAuth2UserRequest userRequest) { OAuth2User oAuth2User = delegate.loadUser(userRequest); Map<String, Object> attributes = new HashMap<>(oAuth2User.getAttributes()); // Additional processing of attributes if needed return oAuth2User; } }
Controller
Create a controller to handle login and display user info.import org.springframework.security.core.annotation.AuthenticationPrincipal; import org.springframework.security.oauth2.core.user.OAuth2User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; @Controller public class LoginController { @GetMapping("/login") public String getLoginPage() { return "login"; } @GetMapping("/") public String getIndexPage(Model model, @AuthenticationPrincipal OAuth2User principal) { if (principal != null) { model.addAttribute("name", principal.getAttribute("name")); } return "index"; } }
Thymeleaf Templates
Create Thymeleaf templates for login and index pages.
src/main/resources/templates/login.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Login</title> </head> <body> <h1>Login</h1> <a href="/oauth2/authorization/facebook">Login with Facebook</a> </body> </html>
src/main/resources/templates/index.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Home</title> </head> <body> <h1>Home</h1> <div th:if="${name}"> <p>Welcome, <span th:text="${name}">User</span>!</p> </div> <div th:if="${!name}"> <p>Please <a href="/login">log in</a>.</p> </div> </body> </html>
7. Testing the Integration
Run your Spring Boot application and navigate to http://localhost:8080. Click on the "Login with Facebook" link and authenticate with your Facebook credentials. If everything is set up correctly, you should be redirected to the home page with your Facebook profile name displayed.
8. Conclusion
Integrating Facebook login into your Spring Boot application using Spring Security enhances user experience and leverages the power of OAuth2. With this setup, users can easily log in with their existing Facebook accounts, providing a seamless and secure authentication process.
By following this guide,
2 notes
·
View notes
Text
Java Security: Implementing OAuth2 Authentication in Spring Boot
Introduction Brief Explanation Java Security: Implementing OAuth2 Authentication in a Spring Boot Application is a crucial aspect of securing web applications. OAuth2 is an industry-standard authorization framework that enables secure, delegated access to web resources. In this tutorial, we will explore the technical aspects of implementing OAuth2 authentication in a Spring Boot application. We…
0 notes
Link
#angular-development#authentication-management#keycloak-authentication#multi-tenancy#saas-architecture#saas-security#software-architecture#spring-boot#programming
0 notes
Text
How Spring Security Protects Your Web Application
Spring Security is a powerful and customizable framework for securing web applications in the Spring ecosystem. Here’s how it safeguards your application:
1️⃣ Authentication: Verifies user identities through login forms, HTTP basic authentication, OAuth2, and more.
2️⃣ Authorization: Controls user access with roles and permissions, defining who can access which parts of the application.
3️⃣ Protection Against CSRF: Cross-Site Request Forgery (CSRF) attacks are prevented by validating tokens in requests.
4️⃣ Session Management: Manages user sessions securely, limiting vulnerabilities like session fixation.
5️⃣ Password Encoding: Encrypts passwords using algorithms like bcrypt, preventing plain-text storage.
6️⃣ Security Headers: Adds default security headers (X-Content-Type, X-Frame-Options, etc.) to secure requests and responses.
7️⃣ OAuth2 and JWT Support: Enables integration with OAuth2 for secure SSO and JWT for stateless session handling.
Secure your application effectively with Spring Security – customizable, reliable, and robust!
🚀 Take your skills to the next level with Spring Online Training for comprehensive, hands-on learning in Spring Security, Spring Boot, and more!
#SpringSecurity#SpringOnlineTraining#WebAppSecurity#SpringFramework#programming#100daysofcode#software#web development#angulardeveloper#coding
0 notes
Text
From Monolithic to Microservices: The Full Stack Developer's Guide
In software development, the transition from monolithic to microservices architecture represents a major advancement. Because of their coupled components, monolithic programs can have trouble growing and changing to meet evolving business requirements. By dividing applications into smaller, independent services that can be built, deployed, and scaled separately, microservices offer a more adaptable and modular approach. Comprehending this shift is essential for Full Stack Developers to create contemporary, expandable applications. This article offers a thorough how-to for switching from monolithic to microservices architecture, outlining the advantages, difficulties, and recommended procedures for a smooth transfer.
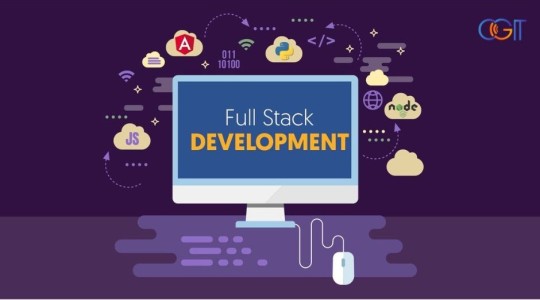
Why Shift from Monolithic to Microservices?
Monolithic applications can be difficult to maintain and scale due to their tightly coupled architecture. As applications grow, these challenges multiply, leading to longer development cycles and increased operational costs. Microservices offer a modular approach, allowing individual services to be developed, deployed, and scaled independently. This results in faster release cycles, better fault isolation, and improved scalability. For Full Stack Developers, mastering microservices architecture means being able to build applications that can easily adapt to changing business requirements.
Key Considerations for a Successful Transition:
Transitioning from monolithic to microservices is not without challenges. Developers need to consider factors such as service granularity, data management, and inter-service communication. Defining the right level of granularity is crucial to avoid creating too many or too few services. Similarly, managing data consistency across multiple services requires a robust strategy, such as using event-driven architectures or implementing a Saga pattern. Understanding these key considerations will help developers navigate the complexities of microservices architecture.
Choosing the Right Tools and Frameworks:
Selecting the right tools and frameworks is critical for a smooth transition to microservices. Developers need to choose container orchestration tools like Kubernetes for deploying and managing microservices. Additionally, frameworks like Spring Boot for Java, Express.js for Node.js, and Flask for Python offer built-in support for microservices development. Familiarity with API gateways, such as NGINX or Kong, is also essential for managing communication between services.
Ensuring Security in a Microservices Architecture:
Security in a microservices architecture can be challenging due to the increased number of endpoints. Developers must implement strong authentication and authorization mechanisms, such as OAuth2 and JWT tokens, to secure communications between services. Additionally, monitoring and logging tools like Prometheus and Grafana can help detect and respond to security threats in real-time.
There are several advantages of switching from monolithic to microservices design, such as increased scalability, flexibility, and quicker release cycles. It does, however, also bring difficulties that call for meticulous preparation and implementation. Full Stack Developers may effectively manage this transformation by being aware of the principal factors, selecting the appropriate tools, and putting strong security measures in place. Developers may create more durable and adaptive modern apps by embracing microservices, which will help them stay competitive in the rapidly changing IT industry.
0 notes
Text
How to Implement Java Microservices Architecture
Implementing a microservices architecture in Java is a strategic decision that can have significant benefits for your application, such as improved scalability, flexibility, and maintainability. Here's a guide to help you embark on this journey.
1. Understand the Basics
Before diving into the implementation, it's crucial to understand what microservices are. Microservices architecture is a method of developing software systems that focuses on building single-function modules with well-defined interfaces and operations. These modules, or microservices, are independently deployable and scalable.
2. Design Your Microservices
Identify Business Capabilities
Break down your application based on business functionalities.
Each microservice should represent a single business capability.
Define Service Boundaries
Ensure that each microservice is loosely coupled and highly cohesive.
Avoid too many dependencies between services.
3. Choose the Right Tools and Technologies
Java Frameworks
Spring Boot: Popular for building stand-alone, production-grade Spring-based applications.
Dropwizard: Useful for rapid development of RESTful web services.
Micronaut: Great for building modular, easily testable microservices.
Containerization
Docker: Essential for creating, deploying, and running microservices in isolated environments.
Kubernetes: A powerful system for automating deployment, scaling, and management of containerized applications.
Database
Use a database per service pattern. Each microservice should have its private database to ensure loose coupling.
4. Develop Your Microservices
Implement RESTful Services
Use Spring Boot to create RESTful services due to its simplicity and power.
Ensure API versioning to manage changes without breaking clients.
Asynchronous Communication
Implement asynchronous communication, especially for long-running or resource-intensive tasks.
Use message queues like RabbitMQ or Kafka for reliable, scalable, and asynchronous communication between microservices.
Build and Deployment
Automate build and deployment processes using CI/CD tools like Jenkins or GitLab CI.
Implement blue-green deployment or canary releases to reduce downtime and risk.
5. Service Discovery and Configuration
Service Discovery
Use tools like Netflix Eureka for managing and discovering microservices in a distributed system.
Configuration Management
Centralize configuration management using tools like Spring Cloud Config.
Store configuration in a version-controlled repository for auditability and rollback purposes.
6. Monitoring and Logging
Implement centralized logging using ELK Stack (Elasticsearch, Logstash, Kibana) for easier debugging and monitoring.
Use Prometheus and Grafana for monitoring metrics and setting up alerts.
7. Security
Implement API gateways like Zuul or Spring Cloud Gateway for security, monitoring, and resilience.
Use OAuth2 and JWT for secure, stateless authentication and authorization.
8. Testing
Write unit and integration tests for each microservice.
Implement contract testing to ensure APIs meet the contract expected by clients.
9. Documentation
Document your APIs using tools like Swagger or OpenAPI. This helps in maintaining clarity about service endpoints and their purposes.
Conclusion
Implementing a Java microservices architecture can significantly enhance your application's scalability, flexibility, and maintainability. However, the complexity and technical expertise required can be considerable. Hire Java developers or avail Java development services can be pivotal in navigating this transition successfully. They bring the necessary expertise in Java frameworks and microservices best practices to ensure your project's success. Ready to transform your application architecture? Reach out to professional Java development services from top java companies today and take the first step towards a robust, scalable microservice architecture.
0 notes
Text
Top Advantages of Hiring a Java Spring Boot Developers For Your Business:
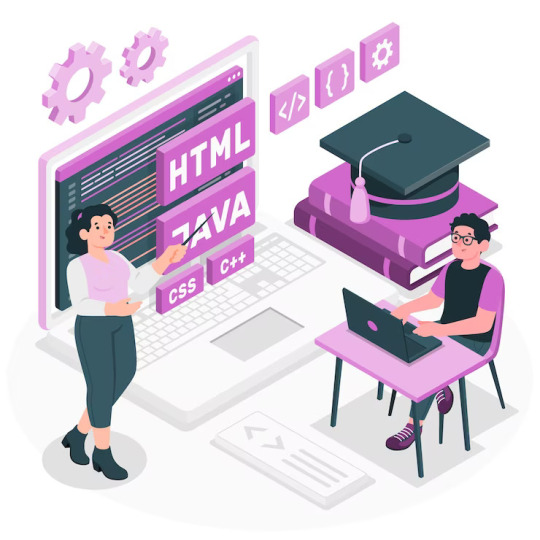
Hiring a Java Spring Boot developer for your project can bring numerous advantages and benefits.
Listed below are some of the top advantages of hiring a Java Spring Boot developer and explain why they are valuable for your project.
Robust and Efficient Development:
Java Spring Boot is a powerful and mature framework that provides a comprehensive set of features for developing enterprise-grade applications. Hiring a Java Spring Boot developer ensures that your project will benefit from the robustness and efficiency of the framework. Spring Boot offers built-in support for many common tasks, such as dependency management, configuration, and auto-configuration, which accelerates development and reduces boilerplate code.
Rapid Application Development:
Spring Boot promotes rapid application development by providing a convention-over-configuration approach. It reduces the need for manual configuration and eliminates much of the boilerplate code, allowing developers to focus on implementing business logic. With Spring Boot, developers can quickly set up a project, leverage the extensive library of reusable components (such as security, database access, and web services), and build applications rapidly.
Microservices Architecture:
Java Spring Boot is well-suited for developing microservices-based architectures. Microservices promote the development of loosely coupled, independently deployable components, which can be beneficial for scalability, maintainability, and fault isolation. Spring Boot provides the necessary features for building microservices, such as embedded servers, lightweight containers, and easy integration with other Spring projects like Spring Cloud and Spring Cloud Netflix.
Broad Community and Ecosystem:
Spring Boot has a large and vibrant community of developers, which means there is ample support, resources, and knowledge available. The Spring community actively contributes to the framework's development and provides valuable libraries, extensions, and integrations. By hiring a Spring Boot developer, you gain access to this extensive ecosystem, enabling you to leverage existing solutions, troubleshoot issues effectively, and stay up-to-date with the latest trends and best practices.
Robust Testing and Debugging Capabilities:
Java Spring Boot encourages the use of test-driven development and provides excellent support for testing. The framework includes testing utilities, such as the Spring Test Framework and JUnit integration, which allow developers to write comprehensive unit, integration, and end-to-end tests. Additionally, Spring Boot's built-in logging and debugging capabilities aid developers in diagnosing and resolving issues efficiently.
Scalability and Performance:
Java Spring Boot applications can be easily scaled to handle increased load and demand. The framework supports horizontal scaling by deploying multiple instances of an application, and it provides integration with cloud platforms, containerization technologies like Docker, and orchestration tools like Kubernetes. Spring Boot also offers features like caching, connection pooling, and asynchronous processing, which contribute to improved application performance.
Security and Reliability:
Spring Boot emphasizes security and provides robust mechanisms for implementing authentication, authorization, and secure communication. The framework integrates well with industry-standard security protocols and frameworks, such as OAuth2, JWT, and Spring Security. By hiring a Spring Boot developer, you ensure that your application follows security best practices and can withstand potential threats, thus enhancing the overall reliability of your project.
Maintenance and Support:
Java Spring Boot promotes maintainable code by encouraging modular and well-structured designs. It follows established design patterns and coding principles, which simplifies future enhancements, bug fixes, and maintenance tasks. Furthermore, the Spring community provides regular updates, bug fixes, and long-term support for major releases, ensuring that your application remains compatible and secure in the long run.
Cross-platform Compatibility:
Java Spring Boot applications are platform-independent and can run on various operating systems and environments. The Java Virtual Machine (JVM) allows Spring Boot applications to be deployed on Windows, Linux, macOS, and other platforms without the need for major modifications. This cross-platform compatibility ensures that your application can reach a wide audience and adapt to different deployment scenarios.
Industry Adoption and Job Market:
Java Spring Boot is widely adopted in the industry, and many organizations rely on it for their critical applications. By hiring a Java Spring Boot developer, you align your project with a proven technology stack that is trusted and supported by numerous enterprises. Additionally, from a hiring perspective, there is a considerable pool of experienced Java Spring Boot developers available in the job market, making it easier to find skilled professionals for your project.
In conclusion, hiring a Java Spring Boot developer brings a multitude of advantages to your project, including robust development, rapid application development, support for microservices architecture, a broad community and ecosystem, testing and debugging capabilities, scalability and performance, security and reliability, ease of maintenance, cross-platform compatibility, and industry adoption. These advantages contribute to efficient development, high-quality applications, and the ability to adapt and scale as your project evolves.
0 notes
Text
YouTube Short | What is Difference Between OAuth2 and SAML | Quick Guide to SAML Vs OAuth2
Hi, a short #video on #oauth2 Vs #SAML #authentication & #authorization is published on #codeonedigest #youtube channel. Learn OAuth2 and SAML in 1 minute. #saml #oauth #oauth2 #samlvsoauth2 #samlvsoauth
What is SAML? SAML is an acronym used to describe the Security Assertion Markup Language (SAML). Its primary role in online security is that it enables you to access multiple web applications using single sign-on (SSO). What is OAuth2? OAuth2 is an open-standard authorization protocol or framework that provides applications the ability for “secure designated access.” OAuth2 doesn’t share…
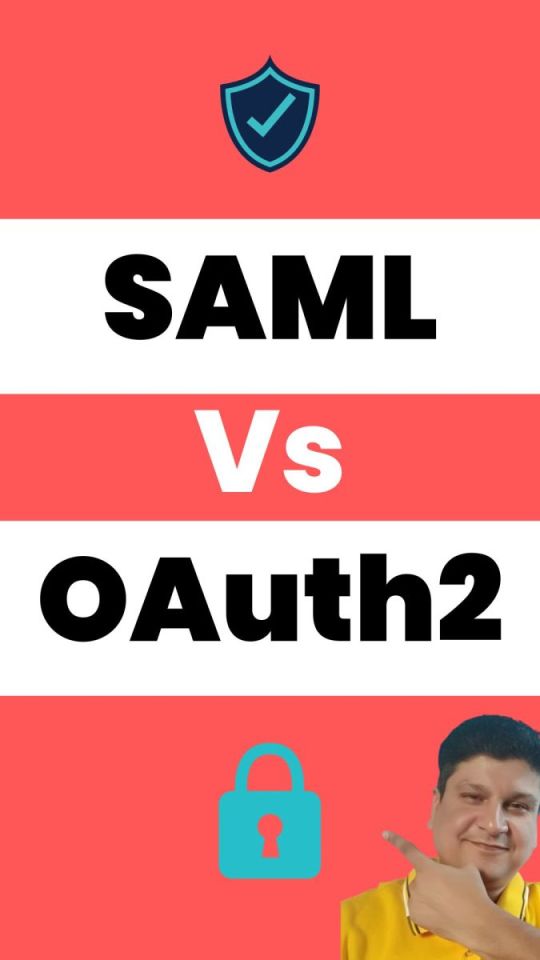
View On WordPress
#Oauth#oauth2#oauth2 authentication#oauth2 authentication flow#oauth2 authentication postman#oauth2 authentication server#oauth2 authentication spring boot#oauth2 authorization#oauth2 authorization code#oauth2 authorization code flow#oauth2 authorization code flow spring boot#oauth2 authorization server#oauth2 authorization sever spring boot#oauth2 explained#oauth2 spring boot#oauth2 spring boot microservices#oauth2 spring boot rest api#oauth2 tutorial#oauth2 vs saml#oauth2 vs saml 2.0#oauth2 vs saml2#saml#saml 2.0#saml 2.0 registration#saml authentication#saml tutorial#saml vs oauth#saml vs oauth 2.0#saml vs oauth vs sso#saml vs oauth2
0 notes
Text
DPoP with Spring Boot and Spring Security
Solid is an exciting project that I first heard about back in January. Its goal is to help “re-decentralize” the Web by empowering users to control access to their own data. Users set up “pods” to store their data, which applications can securely interact with using the Solid protocol. Furthermore, Solid documents are stored as linked data, which allows applications to interoperate more easily, hopefully leading to less of the platform lock-in that exists with today’s Web.
I’ve been itching to play with this for months, and finally got some free time over the past few weekends to try building a Solid app. Solid's authentication protocol, Solid OIDC, is built on top of regular OIDC with a mechanism called DPoP, or "Demonstration of Proof of Possession". While Spring Security makes it fairly easy to configure OIDC providers and clients, it doesn't yet have out-of-the-box support for DPoP. This post is a rough guide on adding DPoP to a Spring Boot app using Spring Security 5, which gets a lot of the way towards implementing the Solid OIDC flow. The full working example can be found here.
DPoP vs. Bearer Tokens
What's the point of DPoP? I will admit it's taken me a fair amount of reading and re-reading over the past several weeks to feel like I can grasp what DPoP is about. My understanding thus far: If a regular bearer token is stolen, it can potentially be used by a malicious client to impersonate the client that it was intended for. Adding audience information into the token mitigates some of the danger, but also constrains where the token can be used in a way that might be too restrictive. DPoP is instead an example of a "sender-constrained" token pattern, where the access token contains a reference to an ephemeral public key, and every request where it's used must be additionally accompanied by a request-specific token that's signed by the corresponding private key. This proves that the client using the access token also possesses the private key for the token, which at least allows the token to be used with multiple resource servers with less risk of it being misused.
So, the DPoP auth flow differs from Spring's default OAuth2 flow in two ways: the initial token request contains more information than the usual token request; and, each request made by the app needs to create and sign a JWT that will accompany the request in addition to the access token. Let's take a look at how to implement both of these steps.
Overriding the Token Request
In the authorization code grant flow for requesting access tokens, the authorization process is kicked off by the client sending an initial request to the auth server's authorization endpoint. The auth server then responds with a code, which the client includes in a final request to the auth server's token endpoint to obtain its tokens. Solid OIDC recommends using a more secure variation on this exchange called PKCE ("Proof Key for Code Exchange"), which adds a code verifier into the mix; the client generates a code verifier and sends its hash along with the authorization request, and when it makes its token request, it must also include the original code verifier so that the auth server can confirm that it originated the authorization request.
Spring autoconfigures classes that implement both the authorization code grant flow and the PKCE variation, which we can reuse for the first half of our DPoP flow. What we need to customize is the second half -- the token request itself.
To do this we implement the OAuth2AccessTokenResponseClient interface, parameterized with OAuth2AuthorizationCodeGrantRequest since DPoP uses the authorization code grant flow. (For reference, the default implementation provided by Spring can be found in the DefaultAuthorizationCodeTokenResponseClient class.) In the tokenRequest method of our class, we do the following:
retrieve the code verifier generated during the authorization request
retrieve the code received in response to the authorization request
generate an ephemeral key pair, and save it somewhere the app can access it during the lifetime of the session
construct a JWT with request-specific info, and sign it using our generated private key
make a request to the token endpoint using the above data, and return the result as an OAuth2AccessTokenResponse.
Here's the concrete implementation of all of that. We get the various data that we need from the OAuth2AuthorizationCodeGrantRequest object passed to our method. We then call on RequestContextHolder to get the current session ID and use that to save the session keys we generate to a map in the DPoPUtils bean. We create and sign a JWT which goes into the DPoP header, make the token request, and finally convert the response to an OAuth2AccessTokenResponse.
Using the DPoP Access Token
Now, to make authenticated requests to a Solid pod our app will need access to both an Authentication object (provided automatically by Spring) containing the DPoP access token obtained from the above, as well as DPoPUtils for the key pair needed to use the token.
On each request, the application must generate a fresh JWT and place it in a DPoP header as demonstrated by the authHeaders method below:
private fun authHeaders( authToken: String, sessionId: String, method: String, requestURI: String ): HttpHeaders { val headers = HttpHeaders() headers.add("Authorization", "DPoP $authToken") dpopUtils.sessionKey(sessionId)?.let { key -> headers.add("DPoP", dpopUtils.dpopJWT(method, requestURI, key)) } return headers }
The body of the JWT created by DPoPUtils#dpopJWT contains claims that identify the HTTP method and the target URI of the request:
private fun payload(method: String, targetURI: String) : JWTClaimsSet = JWTClaimsSet.Builder() .jwtID(UUID.randomUUID().toString()) .issueTime(Date.from(Instant.now())) .claim("htm", method) .claim("htu", targetURI) .build()
A GET request, for example, would then look something like this:
val headers = authHeaders( authToken, sessionId, "GET", requestURI ) val httpEntity = HttpEntity(headers) val response = restTemplate.exchange( requestURI, HttpMethod.GET, httpEntity, String::class.java )
A couple of last things to note: First, the session ID passed to the above methods is not retrieved from RequestContextHolder as before, but from the Authentication object provided by Spring:
val sessionId = ((authentication as OAuth2AuthenticationToken) .details as WebAuthenticationDetails).sessionId
And second, we want the ephemeral keys we generate during the token request to be removed from DPoPUtils when the session they were created for is destroyed. To accomplish this, we create an HttpSessionListener and override its sessionDestroyed method:
@Component class KeyRemovalSessionListener( private val dPoPUtils: DPoPUtils ) : HttpSessionListener { override fun sessionDestroyed(se: HttpSessionEvent) { val securityContext = se.session .getAttribute("SPRING_SECURITY_CONTEXT") as SecurityContextImpl val webAuthDetails = securityContext.authentication.details as WebAuthenticationDetails val sessionId = webAuthDetails.sessionId dPoPUtils.removeSessionKey(sessionId) } }
This method will be invoked on user logout as well as on session timeout.
0 notes
Text
CRYPTOBOT - CRYPTOCURRENCY BOT SOFTWARE DESIGN SPECIFICATION
INTRODUCTION
CRYPTOBOT is designed to perform automatic lending of Cryptocurrencies through Poloniex, Bitfinex and Bittrex Exchanges.
Additionally, 50+ Cryptocurrency Exchanges can be integrated and traded through the Platform.
APPLICATION
CRYPTOBOT User Interface will be a SPA (SINGLE PAGE APPLICATION) powered by Angular JS v 4.3.0 and Spring Boot and a host of other components, frameworks and APIs hosted on Amazon Cloud.
TECHNOLOGY STACK
AMAZON (EC2) CLOUD HOSTED PLATFORM
ANGULAR JS
JAVA 1.8
SPRING BOOT
SPRING MVC REST
SPRING DATA JPA
HAZELCAST IMDG
TOMCAT EMBEDDED
POSTGRESQL
GOOGLE AUTHENTICATOR
AWS ELB
AWS JAVA SDK
DOCKER SWARM
POLONIEX API
BITFINEX API
BITTREX API
THE PLATFORM
The platform will comprise of user account management, referral system, cryptocurrency lending, lending history, third-party exchanges, administration, charts and Bot components.
The platform will be able to lend out multiple Cryptocurrencies through multiple Exchanges.
The platform will be built with extensibility, scalability and high availability.
The platform will be able to serve hundreds of thousands of users.
The platform will be designed for 99.9% uptime.
Entire operations of the platform will be automated.
USER INTERFACE AND FUNCTIONS
HOME PAGE
The Page will have a header to choose multiple languages. The default language can be configured.
The Page will provide a button to Setup Bot
On click of Setup Bot button, options for User Registration and User Authentication are displayed.
The Page will also display current lending rates of Cryptocurrencies from supported exchanges.
USER REGISTRATION
Registration page with form inputs for Name, Email address, Password is displayed.
On form submission page will send HTTP POST request to the platform REST API
REST API process the registration request and if successful send a confirmation email to the user’s supplied Email address.
REST API will send a response back to the Registration page as Success or Failed.
If success, Authentication page will be loaded.
USER AUTHENTICATION
Authentication page with form inputs for Email address and Password is displayed.
On form submission page will send HTTP POST request to the Platform OAuth2 REST API
REST API will verify the form input and issues an access token if successful authentication.
Page will receive the access token and Google Authenticator token form is displayed.
On submission of Google Authenticator token, page will send a request for validation from the platform.
If Google Authenticator token is valid, User Home page is loaded.
If Google Authenticator token is invalid, OAuth2 token is invalidated and Login form will be loaded again.
USER HOME
User Home page will be loaded with Header elements containing Logo, Account, and Logout with respective icons are displayed.
A language chooser drop-down box will also be displayed.
The page will also contain eight Tabs – Settings, My Loans, My Interest, My Deposits, My Withdrawals, Transfer Balance, My Referrals, Bot fee with respective icons.
There will be a Notification Tab also displayed to configure Notification settings.
For the first time user, Settings tab will be displayed.
USER HOME – SETTINGS TAB
Settings Tab will have four display boxes. Three boxes for Exchange Bot activation. One box for Settings.
Three exchanges will be supported at the moment; Poloniex, Bitfinex and Bittrex.
SETTINGS TAB - BOT
The Bot display box will have Exchange name, ON/OFF slider button to enable or disable Bot, Settings for enabling Coins for the exchange.
SETTINGS TAB – ENABLE BOT
Once ON slider button is enabled, User has to provide Exchange API Key and Secret.
Page will send the request to the Platform REST API with access token retrieved from the cookie.
Bot creation and the launcher will be explained in the Platform Design Section.
SETTINGS TAB – DISABLE BOT
If the OFF slider is enabled in the Bot display box, a disable request will sent to the Platform with access token retrieved from the cookie or browser local storage.
Disabling of Bot will be explained in the Platform Design Section.
USER HOME – MY LOANS TAB
A tabular data will be displayed for the loans of the user.
Exchange, Token, State, Duration, End time, Amount lent out, the Interest rate will be the information displayed in the tabular structure.
User’s total Coin balances and the current interest rate wil be displayed.
A hyperlink will be provided to view forecast value gain at the current interest rate and constant price for 50 years.
On clicking hyperlink, a Line Graph will be displayed with $ value on X-Axis and Date on Y-Axis.
A pie chart will also be provided to show the current balance in $ for the subscribed exchanges.
USER HOME – MY INTEREST TAB
The page will display the total lending balance and total interest (30d)
A tabular structure with Exchange, Coin, Balance, Balance($), Interest (30d), Interest (30d $) information will be displayed.
Pie chart with Balances of each Exchange subscribed will be displayed.
Pie chart with Interest from each Exchange subscribed will be displayed.
USER HOME – MY DEPOSITS
A form with an input box, currency chooser, and exchange chooser will be displayed.
The User can deposit currencies to various Exchanges from the page.
On submission of the form, REST API will connect to Exchange and deposit the amount into the Exchange account.
USER HOME - MY WITHDRAWALS
A tabular data of Currency, Exchange, Balance and Withdraw button will be displayed.
On click of Withdraw button, a request will be sent to REST API.
REST API will connect to Exchange and the requested amount is withdrawn from the account.
The updated balance will be displayed in the tabular data for the withdrawn currency.
USER HOME – TRANSFER BALANCE
A tabular data will be displayed with Currency, Exchange, and Balance Amount.
A form will also be displayed with two Currency choosers and Exchange chooser for transferring the balance from one currency to another in the Exchange.
A button will be available to perform the Balance Transfer.
On click of the button, REST API will be invoked and balance transfer operation will be performed in the Exchange.
The balance will be updated in the Exchange account and displayed in the tabular data.
USER HOME – MY REFERRALS
The User of the CRYPTOBOT system can refer another user through Email or SMS.
Both the parties involved in the Referral process will get Bonus coins in their account balance.
BOT FEE
This tab will display the Bot fees accrued through lending operations by User from the subscribed exchanges through CRYPTOBOT.
For the lender, a 15% fee will be applied to earned Interest.
The Bot fee can be configured in the system.
NOTIFICATION TAB – NOTIFICATION SETTINGS
ON/OFF Slider buttons will be provided for Notifications by Email and SMS.
The Notification system will be explained in the Platform Design Section.
ADMIN USER INTERFACE
The Admin User Interface will be used to perform administrative functions from the CRYPTOBOT System.
The Admin UI will be used to activate or de-active User accounts on suspicious activity.
The Bot settings and parameters can be configured from Admin User Interface.
The Lending settings and configuration can also be applied.
Addition or Removal of Exchanges from the system.
Addition or Removal of trading currencies.
CHARTS - HIGHCHARTS
Highcharts JS is a JavaScript charting library based on SVG, with fallbacks to VML and canvas for old browsers.
CRYPTOBOT charts will be displayed by availing Highcharts Graph APIs and chart components.
LENDING STRATEGY & ALGORITHMS
ALGORITHMS & FUNCTIONS
Lending Algorithm
Finely divided lending
Referral program
Lending fee
Short sale
Long sale
Loan Orders
Forced Liquidation
Order book
POLONIEX LENDING ALGORITHM
Automatically lend your coins on Poloniex at the highest possible rates, 24 hours a day.
Configure your own lending strategy! Be aggressive and hold out for a great rate or be conservative and lend often but at a lower rate, your choice!
The ability to spread your offers out to take advantage of spikes in the lending rate.
Withhold lending a percentage of your coins until the going rate reaches a certain threshold to maximize your profits.
Lock in a high daily rate for a longer period of time period of up to sixty days, all configurable!
Automatically transfer any funds you deposit (configurable on a coin-by-coin basis) to your lending account instantly after deposit.
View a summary of your bot's activities, status, and reports via an easy-to-set-up webpage that you can access from anywhere!
Choose any currency to see your profits in, even show how much you are making in USD!
Select different lending strategies on a coin-by-coin basis.
Run multiple instances of the bot for multiple accounts easily using multiple config files.
Configure a date you would like your coins back, and watch the bot make sure all your coins are available to be traded or withdrawn at the beginning of that day.
BITFINEX LENDING ALGORITHM – MARGIN BOT
Margin Bot is designed to manage 1 or more bitfinex accounts, doing its best to keep any money in the "deposit" wallet lent out at the highest rate possible while avoiding long periods of pending loans (as often happens when using the Flash Return Rate, or some other arbitrary rate). There are numerous options and setting to tailor the bot to your requirements.
MinDailyLendRate. The lowest daily lend rate to use for any offer except the HighHold, as it is a special case ( a warning message is shown in case HighHoldDailyRate < MinDailyLendRate).
SpreadLend. The number of offers to split the available balance uniformly across the [GapTop, GapBottom] range. If set to 1 all balance will be offered at the rate of GapBottom position.
uGapBottom. The depth of lendbook (in volume) to move trough before placing the first offer. If set to 0 first offer will be placed at the rate of lowest ask.
GapTop. The depth of lendbook (in volume) to move trough before placing the last offer. if SpreadLend is set to >1 all offers will be distributed uniformly in the [GapTop, GapBottom] range.
ThirtyDayDailyThreshold. Daily lend rate threshold after which we offer lends for 30 days as opposed to 2. If set to 0 all offers will be placed for a 2 day period.
HighHoldDailyRate. Special High Hold offer for keeping a portion of wallet balance at a much higher daily rate. Does not count towards SpreadLend parameter. Always offered for 30 day period.
HighHoldAmount. The amount of currency to offer at the HighHoldDailyRate rate. Does not count towards SpreadLend parameter. Always offered for 30 day period. If set to 0 High Hold offer is not made.
BITFINEX LENDING ALGORITHM – CASCADE BOT
Cascade Bot lending strategy is modified so that starting daily lend rate is not defined as an absolute value, but rather than an increment (which can also be negative) to FRR.
Cascade lending bot for Bitfinex. Places lending offers at a high rate, then gradually lower them until they're filled.
This is intended as a proof of concept alternative to fractional reserve rate (FRR) loans. FRR lending heavily distorts the swap market on Bitfinex. My hope is that Bitfinex will remove the FRR, and implement an on-site version of this bot for lazy lenders (myself included) to use instead.
uStartDailyLendRateFRRInc Float. The starting rate of FRR + StartDailyLendRateFRRInc that offers will be placed at.
ReduceDailyLendRate Float. The rate at which to reduce already existing offers every ReductionIntervalMinutes minutes.
MinDailyLendRate Float. The minimum daily lend rate that you're willing to lend at.
LendPeriod Integer. The period for lend offers.
ReductionIntervalMinutes Float. How often should the unlent offers` rate be decremented. Note that this parameter should be more than or equal to the interval at which bot is scheduled to run (usually 10 minutes).
ExponentialDecayMult Float. Exponential decay constant which sets the decay rate. Set to 1 for a linear decay. Decay formula: NewDailyRate = (CurrentDailyRate - MinDailyLendRate) * ExponentialDecayMult + MinDailyLendRate.
PLATFORM DESIGN
PLATFORM DESIGN – REST API
The Platform will be built using Spring Boot technology with Tomcat Embedded server.
Spring MVC REST APIs exposed from the platform communicates with the web app built using Angular JS v 2.0 or above.
Data access and storage in the platform will be performed using Spring Data middleware components.
In order to speed up the operations in the Platform Hazelcast In Memory Data Grid (IMDG) will be used.
Docker Swarm will be used as container in which Platform components run during development, testing and production deployments.
The Platform will be deployed on Amazon Elastic Compute Cloud.
API ENDPOINTS
The platform will serve requests from web app through Spring MVC REST APIs. The following REST APIs are identified:
/register
/authenticate
/googleauthenticator
/logout
/account
/deposit
/withdraw
/transfer
/createbot
/activatebot
/deactivatebot
/myloans
/loanbalanceBTC
/exchnagerateBTC
/exchnagerate$
/minrateperday
/mindepositrent
/manualspreadsize
/maxlending
/maxdurationlending
/config
/myinterest
/lendingbalance
/totalinterest
/coinbalanceBTC
/currentrateBTC
/currentBalance$
/currenexchangetrate$
/forecast
/balancechart
/interestchart
/history
/notificationsettingsemail
/notificationsettingsms
/notifyemail
/notifysms
PLATFORM DESIGN – BOT CREATION
Multiple Bots can be created from the User Home Settings box. User needs to provide API Key and Secret of the Exchange to create the Bot.
Bot creation request will be sent to Platform REST API with the access token.
Bot creation REST API will send the request to Exchange through REST APIs exposed by Exchange with API Key and Secret provided by Exchange.
On successful response from Exchange by verifying the credentials, the Platform will move to Bot creation process.
On unsuccessful response from Exchange, Platform REST API will send the response to the user as Bot creation Failed.
PLATFORM DESIGN - BOT ACTIVATION
Once Bot activation request is received from the Platform, Bot configuration settings for respective Exchange will be loaded from Database or Hazelcast IMDG.
AWS Java SDK will be used to clone an already created t2.nano or t2.micro Ubuntu AMI (Amazon Instance) running Embedded Tomcat instance created in an automated fashion from the Platform.
The Embedded Tomcat will also expose a Web Socket Endpoint for real-time communication with the Platform and User such as Loan offers and Loan demands, Interest rates, Exchange rates.
The Bot Host Name will be stored in the Hazelcast as a value along with Encrypted API Key and Email address of the user.
PLATFORM DESIGN – MULTI-THREADED BOT COMPONENT
The Multi-threaded Bot component will be launched when the Bot is launched and it will use the Bot settings from Database or Hazelcast cache if available.
The Multi-threaded Bot component will be connected to the Exchange to perform periodic operations.
The components will be configured for active, inactive sleep and request timeout scenarios.
The User communicates with the Bot through Web Socket Session via Platform.
The responses from connected Exchanges are sent back to User through the Web Socket Session object.
PLATFORM DESIGN – BOT AMI INSTANCE LAUNCHER
An Ubuntu t2.nano or t2.micro instance with an Embedded Tomcat will be cloned from an existing Ubuntu AMI instance in Amazon Elastic Computing Cloud.
In the init script of existing Ubuntu AMI instance, Embedded Tomcat will be configured to launch at startup.
Once a request for the launch of Bot is received AWS Java SDK will be used to clone the t2.nano or t2.micro existing Ubuntu AMI instance and manage the lifecycle of AMI instance.
PLATFORM DESIGN – EXCHANGE API INTEGRATION
Platform and Bot will connect to Exchange APIs to perform Lending operations.
Spring REST client APIs will be used to connect to Exchange REST APIs.
PLATFORM DESIGN – DOCKER
Docker is an open platform for developers and sysadmins to build, ship, and run distributed applications, whether on laptops, data center VMs, or the cloud.
Autorestart is a service-level setting in Docker that can automatically start the containers if they stop or crash.
A Docker Swarm is a cluster of Docker engines, or nodes, to deploy services.
The swarm manager uses ingress load balancing to expose the services to make available externally to the swarm.
Docker Cloud makes it easy to spawn new containers of services to handle the additional load.
The CRYPTOBOT platform will be shipped to Test, Integration and Production environment using Docker Swarm deployed on Amazon EC2.
PLATFORM DESIGN - NOTIFICATIONS
The lending activities and alerts can be configured in the system.
The notification settings such as SMTP Server, SMS Gateway can be retrieved from Database or Hazelcast cache if available.
The platform will send notifications or alerts to the subscribed users as and when notifications or alerts are received in the system.
The notifications window can be configured in the system database.
DATABASE DESIGN
PostgreSQL database will be used to store data.
User Account, Referral System, Lending, Loans, Admin, History, Exchanges, Sale, Buy, Balance, Currencies, Charts, Notifications, Bots are the identified tables for the Database.
Spring Data JPA will be used for Data Access operations from the Database.
0 notes