#and by that i mean. creating the entire game in the html editor for the blog posts
Explore tagged Tumblr posts
Text
I love creating unnecessarily complicated expectations for myself. No, genuinely, this is so fun.
#decided to do a browser game for my final project#but owning a website costs money and uploading a game to a game sharing website sounds scary#so i decided the best option would be to use the blog we have for class#and by that i mean. creating the entire game in the html editor for the blog posts#the project's due next friday. i do not know html.#i'm about to have a fucking blast
0 notes
Note
So I had a coding question that I've been trying to find the answer for, for a while. I've tried searching up information online and such but haven't been able to find anything.
I was wondering though how exactly someone would go about creating a main menu screen with the whole being able to create a new game, load one or change the settings? Like I said I've tried searching it up but haven't been able to find anything except for information on creating a transition screen.
You’re going to need an understanding of CSS for this, but I’ll do my best to walk you through it, alongside some basic functions of the UIBar and UI APIs. Also, like pretty much anything to do with coding, there is more than one way to do something (and there may be a more efficient/effective way than mine).
Like all of my tutorials, this is written for SugarCube 2.34.1. Since this one mainly deals with CSS, I’m sure you could adapt it to another format, but I’m not familiar enough with Harlowe, Snowman and Chapbook to add specifics.
Additionally, I use the Twine 2 editor version 2.2.1. This tutorial can be used with later versions; some of my example images may look not look exactly like what you have because later versions of the editor launch test files in your default browser (the 2.2.1 version creates its own mini-browser).
Making a Main Menu Page
Step 1: Hiding the UI Bar
If you want a clear main menu page without the UI bar, you can hide it in several ways.
<<run UIBar.destroy();>>
This will remove the UI bar completely from your game. Not recommended unless you have an alternative way of adding access to the Save, Settings and Restart functions.
<<run UIBar.stow();>>
This stows the UI bar. It will still be partially visible on the side and the player can interact with it to open it. The UI bar can be unstowed manually (without needing the player to do it themselves) on the next passage with:
<<run UIBar.unstow();>>
If you don’t want the UI bar to show up on your main menu, but you want to have access to it later, you can use:
<<run UIBar.hide();>>
To bring it back, you will have to use the following on the passage where you want the player to have access to it.
<<UIBar.show();>>
You may want to use the stow/hide and unstow/show functions together. Hiding the UI bar only makes it invisible; it will still take up space on the left-hand side of your game. Stowing and hiding it makes it a little more even.
To use them together, you can do this:
On the passage you don’t want the UI bar:
<<run UIBar.stow();>><<run UIBar.hide();>>
On the passage you where you want to restore the UI bar:
<<run UIBar.unstow();>><<run UIBar.show();>>
TIP 1: Using <<run UIBar.stow (true)>> gets rid of the slide animation as the UI bar collapses/restores, so you may want to use this so you don’t have any weird animations when you menu passage loads.
TIP 2: If you main menu is the first passage of your game, you can run the scripts for storing and hiding the UI bar in your StoryInit passage and it will run it when your game loads.
TIP 3: You can also use the Config API to have the menu bar be stowed automatically when your game starts.
Pop this code into your Javascript:
Config.ui.stowBarInitially = true;
However, if you have any links that navigate back to the main menu without restarting the game, the UI bar will be in whatever state the player left it in last. If you can only access the main menu by launching the game or hitting restart, don't worry about this.
If you want to double-check the SugarCube documentation for these functions, see here.
Step 2: Tagged Stylesheets
If you want to create a menu page that has a different appearance to your game’s default look, you can do so by using a tagged stylesheets. When using a tagged stylesheet, every passage with the same tag will have its appearance overridden to match what you’ve adjusted in your Story Stylesheet.
Let’s make one called main-menu. You can tag passages like so:
You can also tag the passage a different colour to make it special passages like this one stand out.
Step 3: Adding CSS
Now that the passage is tagged, you need to add a new CSS class to your stylesheet to change its appearance.
To change the appearance, you need to decide which selectors to target and what about them you want to change. Every default SugarCube game has the same set of selectors (you can find them here in the documentation). The most important ones are:
body – the body of the page. You can use this to change the foreground and background colours.
.passages – the element that contains your game’s main text. This is where you can change things like the colour that displays behind your game’s text, the font family, line height, letter spacing, all that stuff.
For the sake of this example, I am going to use the default SugarCube stylesheet and edit it from the ground up. You can find the code for SugarCube’s built-in stylesheets here.
In your stylesheet, you will want to use the tag you created earlier as the new class name.
.main-menu
Put this with the selectors you are going to change.
Let’s start with the body.
body.main-menu { color: #fff; background-color: #000; overflow: auto; }
The color property controls the colour of the font. Here I’ve set it to the hex code #fff and the background-color #000.
So now I have a black page when I start the main menu passage, and thanks to the code for the UI bar I put in earlier, the UI bar is gone.
Adding a Background
Now, we might want to spice up the background with an image to make it more interesting.
To add an image to the background, you need to use the background-image property.
body.main-menu { color: #fff; background-color: #000; background-image: url("images/main-menu.jpg"); background-attachment: fixed; background-repeat: no-repeat; background-size: cover; -webkit-background-size: cover; -moz-background-size: cover; -o-background-size: cover; background-position: center center; overflow: auto; }
You can read more about the different background properties and what they do here on W3Schools, but the code above will center your background image in the middle of the page and also make sure that it covers the entire container.
IMPORTANT: If you intend to upload your game as a ZIP file containing a .index HTML file (this is recommended if you have a lot of image assets or don’t want to link to an outside host, like imgur), you will need to use relative paths with any image URLs in your game.
Relative paths mean that the file is relative to the directory it’s in. In the example above, you can see that the background URL is "images/main-menu.jpg". This means that when the file is uploaded to itch.io, it will find the file—main-menu.jpg—inside the images folder, regardless of where the images folder is located.
For reference, this is what my game assets folder looks like for Wayfarer:
Relative paths are different than an absolute path, which begins with the drive letter. For example, the main-menu.png may be stored on my personal computer in a path like this one: C:/game/images/main-menu.jpg.
If I use this absolute path in the game, the image asset will not show up for players once it’s uploaded to itch because the image is not hosted on the player’s device in C:/game/images/main-menu.jpg.
This can cause some finnicky issues with the Twine 2 editor because the editor cannot find and display images from relative paths (unless you’ve put the editor in the same directory as the one you’re storing your assets in; I haven’t bothered to try this, so I’m not sure).
While working on your game in the Twine editor, you may need to use an absolute path to see what your asset looks like while you're editing. When it comes time to publish, make sure you switch it back to a relative path, otherwise the image will not load for players.
Step 4: Adding & Styling Links
Now that we have a background, we’ll want to tackle the links themselves.
Adding Links
You can link to the starting passage of your game using your preferred method—the [[ ]] link markup, the <<link>> macro, etc.
But for Saves and Settings (and also a Resume Game link, if you’re using the autosave feature), you’ll need to manually call the functions for accessing those dialogs. You can do that with this code here:
This will add a Load Game link that opens the Saves dialog when clicked.
<<link 'LOAD GAME'>><<run UI.saves();>><</link>>
This will add a Settings link that opens the Settings dialog when clicked.
<<link 'SETTINGS'>><<run UI.settings();>><</link>>
This will add a Resume Game link that loads the player’s last autosave.
<<link 'RESUME GAME'>><<run Save.autosave.load()>><</link>>
TIP: To enable autosaves on your game, add this code to your Story Javascript:
Config.saves.autosave = true;
This will autosave on every passage.
Config.saves.autosave = ["bookmark", "autosave"];
This will autosave on passages tagged bookmark or autosave.
Styling Your Game Title & Links
So this is where you can get get fancy with your CSS. For now, we’re going to keep everything within the .passage element (which is where any text inputting into the editor goes), but I will show you how to move the links and title to wherever you want further down.
Importing Fonts
First, go font shopping.
Google fonts has a very large library of free-to-use fonts that you can import directly into your game via your Story Stylesheet. After you browser Google fonts for the fonts you want to use, scroll down to the Use on Web section and click @import. Google will automatically generate the code you need to import the fonts you want to use.
Ignore the <style> </style> and copy everything else inside it and paste it in the top of your Story Stylesheet.
For this example, mine looks like this:
@import url('https://fonts.googleapis.com/css2?family=Almendra+Display&family=Nova+Cut&display=swap');
TIP: If you are importing fonts that a bold weight and italics available and intend to use bold and italics, make sure you import the bold weight and the italic versions of the font as well as the regular one. This will stop your fonts from having weird printing issues when you use bold and italics (especially on non-Chromium browsers like Firefox).
Below the import button, Google will show you the CSS rules for each font family. Keep these in mind, you’ll need them later. Mine, for this example, are like this:
font-family: 'Almendra Display', cursive; font-family: 'Nova Cut', cursive;
Basic Styling
In your stylesheet, you’ll want to target the .passage element with the .main-menu class.
.passage.main-menu { background-color: transparent; font-family: 'Nova Cut', cursive; font-size: 3.5em; text-align: center; }
Make sure there isn’t a space between .passage and .main-menu, otherwise it won’t work!
Here, I’ve changed a few properties.
font-family – this changes the font to Nova Cut
font-size – this changes the font size. I’ve used the unit em, which is relative to the element size (you can read more about CSS Units here)
text-align – this centers the text to the middle of the .passage element
I have also added:
background-color: transparent;
This makes the passage background transparent so you can see the background image. This is only necessary if you’ve added a background-color to your default passages.
Now, for the links.
Links have their own separate selector.
a means is the link as it usually displays
a:hover is the link when the player hovers their cursor over it.
It's generally a good idea to use different colours on the links—one for the normal display, one for the hover—so the player can visually see that they are hovering over a clickable link. If you don't want to use different colours, you should consider using some other visual cue to make that differentiation.
.passage.main-menu a { font-family: 'Nova Cut', cursive; color: #C57C25; text-decoration: none; }
.passage.main-menu a:hover { font-family: 'Nova Cut', cursive; color: #dcb07c; text-decoration: none; }
I’ve added an additional property here:
text-decoration: none.
This gets rid of the underline that happens on all default links in the default SugarCube stylesheet. Currently, this only targets the links on passages tagged main-menu; if you want to get rid of the text-decoration on all links, you can change the styling of your links like so:
a:hover { text-decoration: none; }
Choosing Colours
If you’re not sure where to start when it comes to picking hex codes, color-hex.com is a really helpful site. It gives you related tints and shades of for every hex code, which makes it a lot easier to find colours that are slightly darker or slightly lighter than your base hex code.
For choosing colours initially, there are plenty of hex code colour palette generators available online. One of my favourites is the one on Canva, which lets you upload an image and then it creates a colour palette from there. You might not want to use the exact colours it pulls, but checking the colours on color-hex can help you narrow down something that works for your aesthetics.
This is what our template now looks like:
Giving the Title a Unique Style
Right now, the title is styled by the .passage.main-menu selector and it’s default font size and font type is the same as the links below it.
If you want to style it differently, you can make a new class for it. In this case, I’m going to drop the .passage.main-menu and make a class called .game-title.
.game-title { font-family: 'Almendra Display', cursive; color: #ca893a; line-height: 1.0; font-size: 1.8em; text-shadow: 1px 1px #dcb07c; }
Because the font I selected didn’t come with a bold version, I cheated a bit a used the text-shadow property to bulk it up. I also had to adjust the line height. SugarCube’s default .passage styling gives everything a line height of 1.75 and there was too much space once the new font family and font size were applied.
To add this styling to your title, go into your main menu passage and wrap your game’s title in a span, like so:
<span class="game-title">GENERIC FANTASY GAME</span>
It now appears like this:
TIP: If you want to play around with your appearance, you can use your browser’s Inspect tool to see the page’s CSS and play around/edit it. Either right click and hit Inspect or hit CTRL + SHIFT + I to open the Inspect tool. Once opened, you can go in and adjust things. If you make and a change and like it, remember to copy the code over to your stylesheet before you close the inspect tool.
Placing a Title & Links Outside the .passage element
If you want your game title and menu links to be elsewhere on the page, you’re going to need re-write some of your CSS and add some additional CSS.
The first thing is that you’ll want to remove the styling from .passage.main-menu. I’ve left background-color to transparent, but you’re not going to be using this to style your game title and menu links.
.passage.main-menu { background-color: transparent; }
For the title:
I’ve created two elements, one called .main-title and one called .main-title-item.
.main-title creates a container that will hold the title. This is what I use to tell it where on the page to appear.
.main-title { display: block; justify-content: space-evenly; position: absolute; top: 10%; left: 4%; }
.main-title-item styles the actual text.
.main-title-item { font-family: 'Almendra Display', cursive; text-transform: uppercase; font-weight: normal; font-size: 6.5em; line-height: 1.0; text-align: left; color: #cf944d; text-shadow: 1px 1px #cf944d; }
To apply this to the game title, go back to the main menu passage and apply your new elements to the game’s title:
<div class="main-title"><span class="main-title-item">GENERIC FANTASY GAME</span></div>
For the menu links:
Here, we’ll do something really similar—a container to hold the links and a separate element to style them.
.subtitle { display: block; flex-wrap: wrap; flex-direction: column; width: 60%; justify-content: space-evenly; position: absolute; top: 46%; left: 8%; }
.subtitle-item a { font-family: 'Nova Cut', cursive; font-weight: normal; font-size: 3.5em; text-align: left; color: #cf944d; line-height: 1.3em; }
.subtitle-item a:hover { font-family: 'Nova Cut', cursive; font-weight: normal; font-size: 3.5em; text-align: left; color: #dcb07c; text-decoration: none; }
Go back to your main menu passage and apply the elements. Because all of the menu links will be in the same box, you only need to open/close the .subtitle element once.
<div class="subtitle"><span class="subtitle-item">[[NEW GAME]]</span>
<span class="subtitle-item"><<link 'LOAD GAME'>><<run UI.saves();>><</link>></span>
<span class="subtitle-item"><<link 'RESUME GAME'>><<run Save.autosave.load()>><</link>></span>
<span class="subtitle-item"><<link 'SETTINGS'>><<run UI.settings();>><</link>></span></div>
If you want to change where the title and menu links appear, you can use the Inspect tool to figure out different percentages and spacing until you find something that works for you.
There are a lot more things you can add (like animations that appear when you hover your cursor on the link), but I’ll leave it there for now.
Additionally, if you intend to make your game mobile compatible, you’ll want to read up on media queries and learn how to adjust font sizes and any other units of measurement for different viewports. This is how you shrink things appropriate to fit on small screens.
I hope this helps! If you have any questions, please let me know. I’m still a newbie at CSS (so I’m sure there are ways of doing things more effectively), but these are some of the things that I have helped me along the way.
110 notes
·
View notes
Text
Anonymous:
Do you have any advice on how to start an rp blog? I feel like there's so much to do and so many specific things, it looks intimidating, but I really want to get into it (and your blog seems like a safe space to ask as a baby in the matter)
Hi! Thanks you for asking and for trusting. I do admit that rping on tumblr can look daunting and there is a series of things that are considered “etiquette” that might not be obvious for newcomers. And the only way to learn is to ask, right? As I’m not sure if you would like something more specific or a step-by-step, I’m going to go through the whole process.
note: this is a repost from an ask in a more reblog-friendly format
1. Setting up the blog
You might want to make a new e-mail account for each blog you want. I recommend making a gmail/google account, so you may be able to use other services and associate them with your blog. I’ll go into more details in a minute.
Some people would rather have a personal blog and then making the RP blog as a side-blog. Or a “hub” blog and many side-blogs so they have everything centralized. The downside is that you can’t follow people with side-blogs, only the main – and some rpers are a little suspicious of personal blogs, so if you intend to go this route it might be a good idea to state somewhere in your blog that you have a RP blog.
Tip : It isn’t said too often, but I recommend saving your blog’s e-mail and password somewhere, maybe a flashdrive or even google drive. This way, if something happens you will be able to retrieve your account.
When picking the URL, for a very long time tumblr had problems tagging URLs with a hyphen ( - ). I’m not sure if it has been fixed or if there are still some issues, so I recommend only using letters and maybe numbers. Other than that, pick anything that sounds nice to you!
Themes are nice, but not entirely necessary. Not everybody has photoshop skills and all that. Some people do have commissioned themes, but if you want to try your hand at it my first stop is usually @theme-hunter or @sheathemes . They reblog many themes from many creators, so there are always many options that might suit your needs. Some creators offer very newcomer-friendly themes that you can configure a lot of things without much hassle but some might require basic HTML knowledge – a few creators have guides on how to properly set up their themes and are willing to and answer questions, so don’t be afraid to contact them! You can also send me an ask, I’m not a specialist but I can certainly help walk you through the basics.
Tip: @glenthemes have very good themes and a basic installation guide here.
When fiddling with the options, try to pick colors that have nice contrast and are easy to read. If you are bad at picking colors or have problems in finding the code for them, I recommend trying this link. There is also this one that auto-generate palettes.
Tip : If you mess with your theme, remember there is the Theme Recovery.
Tip: If you use Chrome or Firefox you can set up different profiles and associate each with a different blog, so you don’t need to log out from any of your accounts.
There are two pages that I recommend having: one is an about your muse. If they are an OC, it is always a good idea to have at least some information out there to make things easier. If they are from a canon source, not everybody is familiar with the material so it might be a good idea to state. For example, if you are going to roleplay as Altria/Arturia, it is a good idea to have a “RP blog for Saber (Altria Pendragon) from FGO/FSN “ somewhere visible. The other page that is a good idea having is a rules/guidelines page. This one can be a little intimidating, but it is usually a way to communicate important things. For example: are you comfortable writing violence? Do you have any personal triggers? There is something you absolutely won’t write? There are things you may figure out along the way and it is absolutely ok to fine-tune this session every now and then. Some people also credit source for their icons and graphics in general in their rule/guideline page.
If you are using the tumblr default themes, when you create a new page you can turn on the option to show a link to the page. If you are using a custom theme, most of the time you will have to link it manually.
Oh, and if you are planning to do a multimuse, it might be a good idea to list which muses you have. The same goes for a hub blog; list the muses and link to the pages.
Icons aren’t necessary but are considered commonplace. You can find some icons I’ve done here but there are plenty of other sources. If you want to do your own icons, keep in mind to don’t make them too big, as a courtesy to your mutuals.
Tip: Anything larger than 300 pixels will be stretched to fit the post. As of today ( 4/29/2021 ) the posts are currently 540 pixels wide. This can be useful as making banners for your blog.
Tumblr allow users to “pin” posts. This mean that they will always visible if you access your blog, even on dash/mobile. You can use this to set up a post with basic links for mobile users or something else. For example, if you are out on vacations and won’t be able to do replies, you can pin a hiatus notice and then remove the pin once you are back.
2. Introducing yourself
Time to officially join the fun! (insert a “Hi, Zuko here” joke) Don’t worry if you don’t have a fancy promo graphic or anything, most people make their initial introduction with a simple post.
(as you can see, I’m not very good at saying ‘hi’)
Try to introduce yourself in a few lines, but make sure to state which muse you RP as. Some people also like adding their pen name/alias and establishing a brand. Follow as many people as you want that reblogged or liked your post, and tumblr is going to start recommending other blogs that are related to the tags you use normally or have any relation to the people you follow. You can put as many tags as you want, but tumblr will disregard more than 6 tags in their system. Try tags like “<fandom> rp” and “<fandom> roleplay” along with the media, such as “movie” “video game”, “anime” and so on.
It might also be a good idea to follow a few RP memes blogs. They often have options to break the ice, like one-liners that your mutual can send you.
Tip: Don’t forget to turn on the asks and the anon
3. Practical advice
Alright, now that you have a few mutuals, it is time to get to some general tips:
Tumblr can be a little “iffy”, and a great quality of life extension for RPers and navigation in general is installing the New Xkit extension. They offer a number of options to enhance your tumblr experience, but the ones I consider essential are the “editable reblogs”, “quick tags” and “blacklist”. Get it for Chrome or Firefox.
As a rule of thumb I recommend writing your RPs using Google Docs before posting or replying. By doing this you can do some spell check and if your browser crashes for any reason you can easily recover your work. You can also use Word, Open Office, or any text editor you feel like.
Because I’m a bit of a perfectionist, I also have Grammarly ( Chrome / Firefox ) installed for an extra layer of spell/grammar check. There is a subscription option, but the free one works perfectly fine.
To make things easier to locate, always tag the URL of your RP partner when doing a reply. There are other useful things you can tag, such as open starters, memes, and such.
Risking being obvious here, but when you are not interacting as your character it might be a good idea to tag as “ooc” or “out of character”.
Some people like making google docs with basic info and other useful stuff for easier access on mobile. It is a recent trend, it might be easier to edit as opposed to going through tumblr page editor and dealing with the HTML. You can find some templates here and here.
Tumblr’s activity can be unreliable, so don’t be afraid of contacting your partner to see if they have gotten your reply after a few weeks. However, some people also enjoy using the RP Thread Tracker in order to be on top of things. It might be a good idea to check it out.
Because of Tumblr shadowbanning and shenanigans, it isn’t unusual for people to have NSFW sideblogs (sometimes referred as ‘sin blogs’). If you want to write smut, it might be a good idea to consider making one.
Some people don’t like replying to asks, as Tumblr won’t let you remove the initial ask. It has become common to see people making new posts to reply to asks. This is a simple example:
As you can see, I used the mention to have the RP partner notified then I copied and pasted their question on my post and used the quote to indicate it. You can also have fancy graphics, like a line to separate the contents, just do whatever you feel like with the formatting or keep it simple.
To make sure your partner got the answer, I recommend copying the link to the post and pasting on the ask and then replying it privately. An example sent to my rp blog:
4. Basic Etiquette
Ok, this is a little subjective most of the time but here are a few things that are considered universal courtesy.
Never reblog someone else’s headcanons. If you enjoy it, maybe it should politely contact the author and ask if it is ok to write something based on their original idea but you should never downright copy or lift something from another creator. It is considered rude, or even theft in some cases.
Don’t reblog threads you are not involved with. It is ok to leave a like, but never reblog. This is because Tumblr can mess up the notifications and disrupt the flow of the RP.
Don’t copy other people’s graphics. It is very rude and sometimes they commission (aka: paid) for it.
Trim your posts. What does that mean? Every time you reblog with a reply, the post tends to get longer and longer, and it can cluster your and your mutuals’ dashes. This is why the New X-Kit’s “editable reblogs” is an almost must-have tool. If for some reason you can’t install X-Kit (if you are on mobile for example), then remove the previous post or ask your partner to trim for you.
Never take control of your RP partner’s muse. This is called “godmodding” and it is heavily frowned upon. It is ok to control your muse and the possible NPCs that you inserted, but never seize someone else’s character. Likewise, it can also be very upsetting if you use what people call “meta-gaming”, applying knowledge that your muse shouldn’t know about the other. For example, let’s say your RP partner’s muse is a vampire, but they have never disclosed that information to your muse, who also doesn’t have an excuse to know that (for example, being a vampire hunter) so it can be quite jarring sometimes. When in doubt, contact your partner.
This should go without saying, but RPing sexual themes with users under the age of 18 are illegal. It doesn’t matter if the age of consent in your location is lower, once you join Tumblr you are abiding by their user guidelines and the law of the state they are located in. If you are an adult, don’t engage minors with these topics, maybe a fade to black would be a better option. If you are a minor, don’t insist or you might cause a lot of legal problems for others.
Try to tag anything triggering. Violence, gore, NSFW. Both Tumblr and the New Xkit have options to block keywords.
When picking PSDs or graphics for your blog, you should avoid templates that change the color of the skin of POCs muses and try to pick the right race/ethnicity of the muse you are going to RP as. I won’t go through a lot of details, as it is a rather lengthy subject in an already lengthy conversation but keep this in the back of your mind.
Some RPers don’t like when you reblog memes from them without sending anything. Try to always reblog from a source or to interact with the person you are reblogging from, it can be rather disheartening to be seen as a meme source rather than a RP blog. This isn’t a rule and some people don’t mind, but it is always a good idea to try to do this.
This might be more of a pet peeve of mine than proper etiquette, but it is ok to use small font. What is not ok is use small font + underscript. Some people have disabilities that might make it harder for them to read it, so it might be a good idea to refrain from using it. Maybe if you feel like doing something fancier every now and then, but I wouldn’t recommend making this a habit.
Mun and Muse are different entities. Remember that it isn’t because a muse does something (especially a villain one) that the mun condones something. Never assume anything about the mun, when in doubt talk to them.
Be mindful of your partners and treat them the way you would like to be treated.
As a rule of thumb, always talk to your RP partner. It is only fun as long both of you are enjoying it.
5. Closing Words
This got longer than I expected.
Despite all of that, don’t be too worried about not being very good at first. I assure you that you will get better with time, so don’t be afraid of experimenting as long you feel comfortable. And don’t be afraid of saying “no” if something bothers you.
My inbox is always open to questions and ideas, so feel free to contact me anytime!
I would also ask my followers: there is advice I missed/overlooked? Anything you would like someone have told you when you first started? Add your thoughts so I can update this.
Happy RPing!
17 notes
·
View notes
Text
#showyourprocess
From planning to posting, share your process for making creative content!
To continue supporting content makers, this tag game is meant to show the entire process of making creative content: this can be for any creation.
RULES — When your work is tagged, show the process of its creation from planning to posting, then tag 5 people with a specific link to one of their creative works you’d like to see the process of. Use the tag #showyourprocess so we can find yours!
Big thank you to @fengqing for tagging me and I’ll do my best to explain how I made this set for Jiang Yanli!
1. Planning
The beginning idea for this set actually stemmed from my Yanli birthday set for the @mdzsnet event, wherein I was working with this palette:
The one thing I had to learn with using palettes, and is my biggest help to others, is SCENE CHOICE. It can make a difference between tearing your hair out and being happy with your final result, though there’s definitely some trial and error to it.
So I knew I wanted to make a gifset using each of the colours, and, for the light blue, immediately my mind went to the Gusu Lectures period of time, due to Cloud Recesses having a much more blue and light palette than many of the other settings in the show. The lightness of the blue made me lean towards outdoor scenes, and I immediately recalled the interaction between Jiang Yanli and Jin Zixuan where she slips and he catches her (episode 6). Thus off hunting I went until I found it. The final set actually came about because I had more footage than the original gif needed and I didn’t want a colouring I was quite proud of to be a one-and-done kind of thing. I also found the scene featuring the second gif, which had a similar palette in the episode, and decided to work it in as well (because I have weird moments of fussiness where two gifs isn't enough lol).
2. Creating
I have all the episodes saved on my hard drive, so it’s a matter of finding the right scenes in the episodes and going from there. With my scenes found, I wrote down the timestamps in order to put it through Vapoursynth, which I use to crop, resize, sharpen, and denoise my gifs before importing them into Photoshop (I use a portable version). I trim them down to a relatively similar length (bc I like those sets to be similar), adjust the timing and, with all that done, we’re left with our base gifs like so!
Now onto the fun part! Colouring! I’m going to pop the rest under a read more because it’s image heavy!
I already had a pretty set colouring since this was overflow from another set, but I’ll break that down a bit as well. My first step is always to plop a couple of spots of the desired colour onto the very top layer of the canvas, because it makes judging if its in the right realm or not much easier, like so:
My go-to’s for base colouring is curves (which does a lot of heavy lifting on colour correcting for me) and a vibrance layer (to see what colours we’re working with).
Then it’s about manipulating those colours into something closer to my desired one. Thankfully here my scene choice made life much easier as it’s already a light setting but it’s also already got a lot of cyan to work with. Threw on a few selective colour layers (focusing on lightening the cyan;
making the blues stand out more by removing the warmer tones;
correcting that green-ish tinge back to blue and making the cyan lighter again;
Now here is a little something I tend to do thats negligible but it makes me feel better about the colouring and thats a gradient map set to soft light, low opacity (20% here) using the pale blue and black. It just helps making the image a little more cool in tone in my opinion, and I put that UNDER my original curves layer;
And this is where we break out the brush! Obviously the darker colour on the left needed to be lightened up, so I went in with the palette colour on soft light at 100%;
and lighten at 50%;
Then I added another selective colour layer to lighten up the cyans and get closer to the palette colour;
Back to our brush! A layer on the right with soft light to give a bit more uniform colour across that expanse;
And lastly, I pulled a gradient of just the blue across the left to smooth out the colour on the edge of the frame, like it is on the opposite side (bc I’m obsessed like that);
Amidst all of this, I was using masks on the brush layers to account for Yanli’s movement and keep it from overlapping her skin, until finally I was happy (you’ll notice above the two circles are barely visible now) and we have this result!
My process was basically the same for the other two gifs in the set, with the second requiring a few more brush layers and gradients until we had all three looking spick and span!
Isn’t she pretty?
3. Posting
This here is where we pray Tumblr doesn’t destroy our hard work.
I mean, ahem. I don’t tend to save stuff to drafts unless I can’t finish it then and there, or I’ll pop it into the queue if it’s for a deadline. The gradient text is one of my favourite ways to caption on sets and I use a palette generator from an image website (google adobe colour palette from image) to pull out colours to use (I fiddle sometimes to get a gradient that looks good), before popping them into Cuvou's text fader (I’d link but Tumblr don’t like that but if you google it should come up). I then switch the text editor here over to HTML to pop in the code generated by the fader, add any other text styles I want (bold, small font or header size font, etc), preview the HTML, preview it on my blog a couple times and then once I’m happy, usually that’s it! It gets posted or queued and out into the aether it goes!
In this case, this was the final result of the gradient text in the caption;

Phew so that was... a lot for what amounted to a colouring and I’m sorry if y’all didn’t want a tutorial but here it basically is xD For the tagging I’m going to go outside the fandom just a bit and tag, but if you’ve already been tagged, don’t stress it!
@offtodef with this set
@sugarbabywenkexing with this set
@gusucloud with this set
@wanyinxichen with this set
@sarawatsaraleo with this set
#showyourprocess#tag game#things i'm tagged in#this is very long#for what amounts to a colouring#i'm so sorry xD#hope you enjoyed!
15 notes
·
View notes
Text
Answered: Your Most Burning Questions About web hosting service provider in Delhi
The Web Hosting Delhi enterprise puts the entire picture with each other as if it have been too simple and effortless to deal with. online search engine optimisation services Delhi is a flexible internet site layout and SEO optimization company in Delhi. very last, the Hosting Delhi overview describes the characteristics and benefits of the Firm and permits the end users to buy the solutions from the Group. It can't be concluded devoid of looking at the qualities of the company. For transfers with the airport, take a look at the positioning with the airport you're arriving at, as you can ordinarily discover neighborhood general public transportation is a great deal more cost-effective. usually no person would be able to view our Internet site! When you start hunting for a hosting enterprise in Delhi, you'll notice there's if possible a substantial amount of cpanel World-wide-web internet hosting organizations. The web-web hosting firm could Supply you with with a site identify.
How To Make More Delhi based web hosting By Doing Less
Commonly, the location that sells domain identify presents hosting providers also, but you might want to check out thinking about other internet hosting programs. once you know the sort of small business it will be, you may Find a domain name from any on the web pages that offer area names. It can be done to acquire web hosting expert services from the exact same registrar or from Yet another company. for example, to be able to preserve your domain identify, You have to fork out hosting providers and domain registration fees on a standard basis. As soon as you've got made your absolutely free Taste, the next point to try and do is usually to market it to inspire folks to register in your checklist. usually there are some instead beneficial resources I will increase more about through the entire page To help you in obtaining a Idea of what is available to assist you triumph Or perhaps you happen to be trying to promote wise Living firm but usually are not undertaking and you hoped. When you are only starting up on the internet along with your incredibly initially website, you've quite a Studying curve facing you. any one aiming to commence an Online enterprise must have many sources just before it's possible to assemble and market An effective web page. E-commerce internet hosting is a favourite substitute For numerous on-line corporations. You could also discover other internet hosting services additional ideal determined by your prerequisites. Some hosting products and services give a refund need to they fall short to satisfy their uptime assure, but it surely won't Lower it since each downtime will set you back. With all the different choices provided inside the field, it can be overpowering to select the right web hosting company that fulfills your needs. That host presents a slew of offers and marketing codes you are capable of choose from. an ideal Flash Intro web site has an important function to inform regarding your services. Get your site on-line with a great deal About Internet hosting. there are many non-tech explanations for why you would possibly pick one host above A different. When it must do with deciding upon the ideal Webhosting company, on the other hand, the sheer decision can experience frustrating.
If Delhi web hosting Is So Terrible, Why Don't Statistics Show It?
internet hosting can be a procedure to upload a set of internet internet pages on the net and allow it to generally be obtainable from wherever and whenever. many Internet websites are employing Flash game titles as a means to promote their content material and solutions. a lot of paid Hosting services also offer you unlimited Area for your site, that may support position your thoughts at simplicity. chances are you'll established most browsers to notify you in case you happen to be presented a cookie, or you may commit to block cookies with your browser, but be sure to remember that when you select to erase or block your cookies, you ought to re-enter your original user ID and password to obtain use of specified parts of the Internet site and some sections of the web site wouldn't get The work done. When using that host, it's essential to obtain a protected and strong server. A Flash Web-site is a web site that's designed working with Flash in which the content material is easily modified to fit your wishes. If you do not know HTML you must use an HTML editor which include Microsoft Frontpage, Hotdog, or Dreamweaver which is somewhat more Sophisticated. like this Design suggestions for assorted styles of graphics are presented. Indeed, the system of some parts of the method is likely to be based upon skimpy knowledge and just mirror the most educated guess achievable. many of the Internet websites which have been designed using the Internet standards load faster, have far better search engine position and therefore are simpler to update all the time. Our excellent flash shows are exceptionally economical and match your organization demands.
Webji Hosting Pvt. Ltd. Address: Shop #27, Ajmer Rd, Tagore Nagar, Jaipur, Rajasthan 302021 Phone: 093511 59225 https://webji.in/ Reseller internet hosting is extremely reasonably priced and regularly prices just some dollars per 30 days. that promo code for web hosting features an excellent range of capabilities. that provides some great applications for 1st-time Web-site homeowners who want support picking out a domain identify for their on the internet business. A hosting firm is a company that houses House over a server for you to retail store your internet site as well as the relationship necessary for Other individuals to entry and benefit from your web site. Though your hosting corporation may possibly give a complimentary area with acquire of the web hosting prepare, It is suggested which you sustain your domain name separate inside the party you ever ought to migrate. Some Net hosts give you unlimited e-mail accounts, which is a fantastic alternate for everyone who operates an on-line business. Not all web hosts source you with the selection to create in excess of one email tackle by means of your domain identify.
How To Win Clients And Influence Markets with web hosting services in Delhi
you're going to be responsible for protecting all sides of your site, which includes every little thing from your hardware to any purposes that you put in on your internet site. according to your company product and dimension, you are going to choose a web hosting enterprise with regards to the components stated beneath. Many shared Website hosts give limitless regular info transfers and storage, so other elements may possibly enable you decide on which assistance is most fitted for the organization. Moreover, The shopper help won't seem like stellar. So where the price of web hosting for any US customer might not be a massive offer, with the Indian customer it can be relatively a substantial sum. A cheap Indian web hosting prepare will not provide the processing electric power, RAM and disc home to cater to all of your necessities and you also are going to have to spend more of your time and energy handling the load troubles or downtime in a while. If You use a web page, you need to lessen your running expenditures and keep the fees as low as feasible. If area is taken from various enterprises, dns (nameserver) options ought to be created. When multiple web sites benefit from a singular server, It is known as shared web hosting. Make specified you end up picking a web hosting enterprise which helps you to backup your internet site files and databases within the celebration of this type of emergency. numerous the hosts we've outlined offer you a absolutely no cost area and have a control panel to make the handling of your web site very simple. Some affordable web hosting firms have bad purchaser treatment and fail to react to consumer requests well timed. Some Internet hosts will request their clients to subscribe for an especially prolonged interval in exchange in the really low cost tags. You furthermore mght want hosting services that include exceptional purchaser companies. excellent reseller internet hosting products and services will probably be the ones in which the deals are made and the costs quoted are also instead good.
2 notes
·
View notes
Text
The Morning After: Crypto heist hacker returns all $610 million they stole
It’s the weirdest cryptocurrency heist so far. On Monday, Poly Network, a cryptocurrency finance platform, was hacked by “Mr. White Hat” who exploited a vulnerability in its code to steal $610 million in Ethereum, Shiba Inu and other cryptocurrencies. The company now says it has recovered all the money it lost in the theft.
Less than a day after stealing the digital currencies, the hacker started returning millions saying they were “ready to surrender.” They subsequently locked more than $200 million in assets in an account that required passwords from both them and Poly Network. They said they would only provide their password once everyone was “ready.” At that point, Poly Network offered the hacker a $500,000 reward — a fraction of what they had stolen.
It’s not entirely clear why the hacker surrendered, but it may have been difficult to cash out the millions. The hacker says they were trying to contribute to the security of Poly Network. Maybe they just didn’t want to get caught. Poly will breathe a sigh of relief as will those that were doing their crypto trading through the platform.
— Mat Smith
A Razer mouse can bypass Windows security just by being plugged in
The company says it's fixing the flaw.
Security researcher Jon Hat posted on Twitter that after plugging in a Razer mouse or dongle, Windows Update will download the Razer installer executable and run it with SYSTEM privileges. It also lets you access the Windows file explorer and Powershell with "elevated" privileges, which means nefarious types could install harmful software — if they can get to your USB ports.
Since this vulnerability requires someone plugging in a mouse, it's not nearly as dangerous as a remote attack, but it's still not great for Razer. The company's security team said it’s working on a fix.
Continue reading.
Finally, the ‘Stardew Valley’ esports tournament is a thing
Farm, mine and fish your way to glory on Labor Day weekend.
ConcernedApe
Esports is most commonly associated with high-octane competitive games, usually with guns. That’s not the vibe in Stardew Valley, where you literally tend to crops. It’s more agrarian, less aggro.
But soon, some of the world's finest Stardew Valley players will face off for thousands of dollars. Creator Eric Barone said the first official Stardew Valley Cup event will take place on September 4th. “It’s a competition of skill, knowledge and teamwork, with a prize pool of over $40k.”
More than 100 challenges have been created for competitors to tackle, with four teams of four players each having three hours to complete as many of the tasks as they can. Best start sharpening that hoe.
Continue reading.
NASA’s latest video from Mars looks like ‘Dune’
A new video from the Curiosity rover is here.
NASA/JPL-Caltech/MSSS
NASA’s Jet Propulsion Laboratory released a breathtaking panorama of the inside of the Gale Crater, as snapped by the Curiosity Rover. It shows off where the rover has been and where it’s going. Apparently, on a clear day when there’s no dust in the air, you can see up to 20 miles away.
Continue reading.
PayPal brings its cryptocurrency trading feature to the UK
Even in small units.
PayPal is bringing the ability to buy, hold and sell cryptocurrencies across to the other side of the pond, the better part of a year after it launched in the US. In a statement, the company said that UK-based users would be able to buy, hold and sell Bitcoin, Ethereum, Litecoin and Bitcoin Cash via their PayPal account. The company adds that users can buy as little as £1 of cryptocurrency, and while there are no fees to hold the currency, users will have to pay transaction and currency conversion fees. And hey, it’s not Poly.
Continue reading.
'Twelve Minutes' ruins a compelling game concept
I’ve been waiting a while for this, too.
Annapurna Interactive
Twelve Minutes managed to hook several Engadget editors when it was revealed back in 2015. Even before it gained an all-star cast including Daisy Ridley, James McAvoy and Willem Dafoe, the pitch was easy to understand: a time loop point-and-click adventure. I made a note to keep an eye on the title, and Devindra Hardawar, who reviewed the game, did the same.
Unfortunately, the need to push the story in any — most! — directions leads to some grim choices by the player, and what Devindra calls “mind-numbingly dumb” twists. Tell us how you really feel.
Continue reading.
Apple employees are organizing to push for 'real change' at the company
“We’ve exhausted all internal avenues,” the group says.
A group of current and former Apple employees are calling on colleagues to publicly share stories of discrimination, harassment and retaliation at the company. The collective has started a Twitter account called Apple Workers.
"For too long, Apple has evaded public scrutiny," the group says on its website. "When we press for accountability and redress to the persistent injustices we witness or experience in our workplace, we are faced with a pattern of isolation, degradation and gaslighting." In August, the company put Ashley Gjøvik, a senior engineering program manager, on paid administrative leave. Apple hasn’t yet commented.
Continue reading.
The best streaming gear for students
It’s not just laptops and keyboards.
Being a student is hard, but just because you’re holed up in a dorm room doesn’t mean you should settle for mediocre entertainment. Our updated Student Buyer’s Guide has everything you could possibly need to upgrade from mindlessly watching Netflix on your laptop. That includes deals for TVs, audio gear and the best streaming devices.
Continue reading.
Take a first look at the live-action ‘Cowboy Bebop’
It’s coming November 19th.
Netflix
Netflix's live-action adaptation of classic anime Cowboy Bebop has been a long time coming, and the show finally has a release date. The 10-episode first season will start streaming on November 19th. There’s still no trailer, but we get some stills of the iconic crew, played by John Cho (Spike Spiegel), Mustafa Shakir (Jet Black) and Daniella Pineda (Faye Valentine). There’s a corgi, too.
Continue reading.
Virgin Orbit plans to go public
It’ll go on the Nasdaq exchange to fund its space satellite project.
Mike Blake / Reuters
Virgin Orbit, the less glamorous half of Virgin’s space adventures, has announced plans to go public on the Nasdaq stock exchange through a special purpose acquisitions company (SPAC) merger. The deal with NextGen Acquisition Corp. II values Virgin Orbit at $3.2 billion.
The combined company is expected to pull in up to $483 million in cash when the deal closes, and it plans to scale up its rocket manufacturing. The first spaceflight company to go public through a SPAC, and the company that really kicked off the SPAC trend was Virgin Galactic back in 2019, which sought to fund its tourist trips to space. Yeah, the more exciting facet of space companies.
Continue reading.
The biggest stories you might have missed
The best laptops for college students
'Outriders' is perfectly average, making it ideal for Xbox Game Pass
Teardown shows the Playdate won't suffer from controller drift like the Switch
Rare commemorative Game & Watch handheld sells for $9,100 at auction
T-Mobile is giving customers a free year of Apple TV+
Data leak exposed 38 million records, including COVID-19 vaccination statuses
The OnePlus Buds Pro feature smart ANC and a white-noise mode
from Mike Granich https://www.engadget.com/the-morning-after-crypto-heist-hacker-returns-all-610-million-they-stole-111630131.html?src=rss
0 notes
Text
How to Think Like a Front-End Developer
This is an extended version of my essay “When front-end means full-stack” which was published in the wonderful Increment magazine put out by Stripe. It’s also something of an evolution of a couple other of my essays, “The Great Divide” and “Ooops, I guess we’re full-stack developers now.”
The moment I fell in love with front-end development was when I discovered the style.css file in WordPress themes. That’s where all the magic was (is!) to me. I could (can!) change a handful of lines in there and totally change the look and feel of a website. It’s an incredible game to play.
Back when I was cowboy-coding over FTP. Although I definitely wasn’t using CSS grid!
By fiddling with HTML and CSS, I can change the way you feel about a bit of writing. I can make you feel more comfortable about buying tickets to an event. I can increase the chances you share something with your friends.
That was well before anybody paid me money to be a front-end developer, but even then I felt the intoxicating mix of stimuli that the job offers. Front-end development is this expressive art form, but often constrained by things like the need to directly communicate messaging and accomplish business goals.
Front-end development is at the intersection of art and logic. A cross of business and expression. Both left and right brain. A cocktail of design and nerdery.
I love it.
Looking back at the courses I chose from middle school through college, I bounced back and forth between computer-focused classes and art-focused classes, so I suppose it’s no surprise I found a way to do both as a career.
The term “Front-End Developer” is fairly well-defined and understood. For one, it’s a job title. I’ll bet some of you literally have business cards that say it on there, or some variation like: “Front-End Designer,” “UX Developer,” or “UI Engineer.” The debate around what those mean isn’t particularly interesting to me. I find that the roles are so varied from job-to-job and company-to-company that job titles will never be enough to describe things. Getting this job is more about demonstrating you know what you’re doing more than anything else¹.
Chris Coyier Front-End Developer
The title variations are just nuance. The bigger picture is that as long as the job is building websites, front-enders are focused on the browser. Quite literally:
front-end = browsers
back-end = servers
Even as the job has changed over the decades, that distinction still largely holds.
As “browser people,” there are certain truths that come along for the ride. One is that there is a whole landscape of different browsers and, despite the best efforts of standards bodies, they still behave somewhat differently. Just today, as I write, I dealt with a bug where a date string I had from an API was in a format such that Firefox threw an error when I tried to use the .toISOString() JavaScript API on it, but was fine in Chrome. That’s just life as a front-end developer. That’s the job.
Even across that landscape of browsers, just on desktop computers, there is variance in how users use that browser. How big do they have the window open? Do they have dark mode activated on their operating system? How’s the color gamut on that monitor? What is the pixel density? How’s the bandwidth situation? Do they use a keyboard and mouse? One or the other? Neither? All those same questions apply to mobile devices too, where there is an equally if not more complicated browser landscape. And just wait until you take a hard look at HTML emails.
That’s a lot of unknowns, and the answers to developing for that unknown landscape is firmly in the hands of front-end developers.
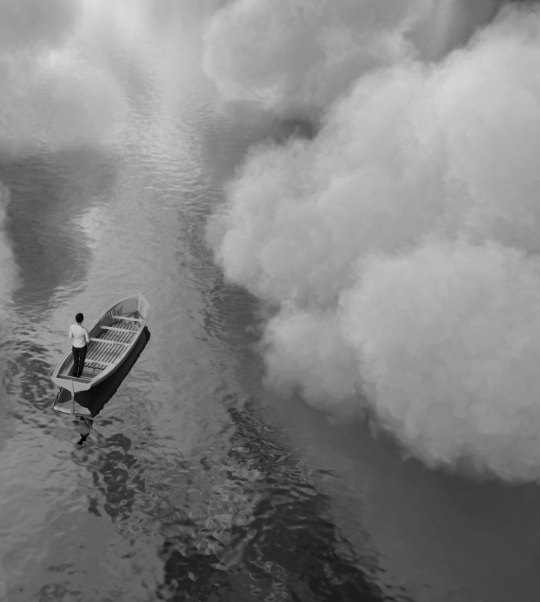
Into the unknoooooowwwn. – Elsa
The most important aspect of the job? The people that use these browsers. That’s why we’re building things at all. These are the people I’m trying to impress with my mad CSS skills. These are the people I’m trying to get to buy my widget. Who all my business charts hinge upon. Who’s reaction can sway my emotions like yarn in the breeze. These users, who we put on a pedestal for good reason, have a much wider landscape than the browsers do. They speak different languages. They want different things. They are trying to solve different problems. They have different physical abilities. They have different levels of urgency. Again, helping them is firmly in the hands of front-end developers. There is very little in between the characters we type into our text editors and the users for whom we wish to serve.
Being a front-end developer puts us on the front lines between the thing we’re building and the people we’re building it for, and that’s a place some of us really enjoy being.
That’s some weighty stuff, isn’t it? I haven’t even mentioned React yet.
The “we care about the users” thing might feel a little precious. I’d think in a high functioning company, everyone would care about the users, from the CEO on down. It’s different, though. When we code a <button>, we’re quite literally putting a button into a browser window that users directly interact with. When we adjust a color, we’re adjusting exactly what our sighted users see when they see our work.
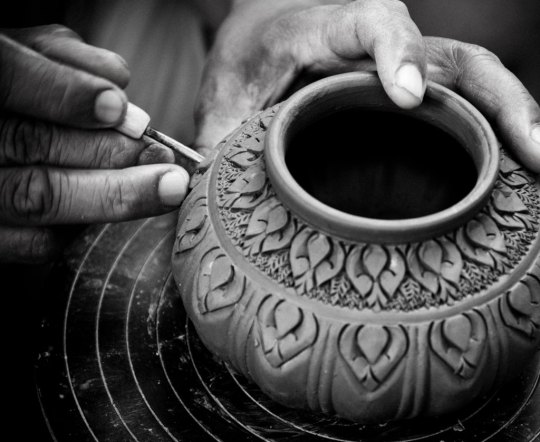
That’s not far off from a ceramic artist pulling a handle out of clay for a coffee cup. It’s applying craftsmanship to a digital experience. While a back-end developer might care deeply about the users of a site, they are, as Monica Dinculescu once told me in a conversation about this, “outsourcing that responsibility.”
We established that front-end developers are browser people. The job is making things work well in browsers. So we need to understand the languages browsers speak, namely: HTML, CSS, and JavaScript². And that’s not just me being some old school fundamentalist; it’s through a few decades of everyday front-end development work that knowing those base languages is vital to us doing a good job. Even when we don’t work directly with them (HTML might come from a template in another language, CSS might be produced from a preprocessor, JavaScript might be mostly written in the parlance of a framework), what goes the browser is ultimately HTML, CSS, and JavaScript, so that’s where debugging largely takes place and the ability of the browser is put to work.
CSS will always be my favorite and HTML feels like it needs the most love — but JavaScript is the one we really need to examine The last decade has seen JavaScript blossom from a language used for a handful of interactive effects to the predominant language used across the entire stack of web design and development. It’s possible to work on websites and writing nothing but JavaScript. A real sea change.
JavaScript is all-powerful in the browser. In a sense, it supersedes HTML and CSS, as there is nothing either of those languages can do that JavaScript cannot. HTML is parsed by the browser and turned into the DOM, which JavaScript can also entirely create and manipulate. CSS has its own model, the CSSOM, that applies styles to elements in the DOM, which JavaScript can also create and manipulate.
This isn’t quite fair though. HTML is the very first file that browsers parse before they do the rest of the work needed to build the site. That firstness is unique to HTML and a vital part of making websites fast.
In fact, if the HTML was the only file to come across the network, that should be enough to deliver the basic information and functionality of a site.
That philosophy is called Progressive Enhancement. I’m a fan, myself, but I don’t always adhere to it perfectly. For example, a <form> can be entirely functional in HTML, when it’s action attribute points to a URL where the form can be processed. Progressive Enhancement would have us build it that way. Then, when JavaScript executes, it takes over the submission and has the form submit via Ajax instead, which might be a nicer experience as the page won’t have to refresh. I like that. Taken further, any <button> outside a form is entirely useless without JavaScript, so in the spirit of Progressive Enhancement, I should wait until JavaScript executes to even put that button on the page at all (or at least reveal it). That’s the kind of thing where even those of us with the best intentions might not always toe the line perfectly. Just put the button in, Sam. Nobody is gonna die.
JavaScript’s all-powerfulness makes it an appealing target for those of us doing work on the web — particularly as JavaScript as a language has evolved to become even more powerful and ergonomic, and the frameworks that are built in JavaScript become even more-so. Back in 2015, it was already so clear that JavaScript was experiencing incredible growth in usage, Matt Mullenweg, co-founder of WordPress, gave the developer world homework: “Learn JavaScript Deeply”³. He couldn’t have been more right. Half a decade later, JavaScript has done a good job of taking over front-end development. Particularly if you look at front-end development jobs.
While the web almanac might show us that only 5% of the top-zillion sites use React compared to 85% including jQuery, those numbers are nearly flipped when looking around at front-end development job requirements.
I’m sure there are fancy economic reasons for all that, but jobs are as important and personal as it gets for people, so it very much matters.
So we’re browser people in a sea of JavaScript building things for people. If we take a look at the job at a practical day-to-day tasks level, it’s a bit like this:
Translate designs into code
Think in terms of responsive design, allowing us to design and build across the landscape of devices
Build systemically. Construct components and patterns, not one-offs.
Apply semantics to content
Consider accessibility
Worry about the performance of the site. Optimize everything. Reduce, reuse, recycle.
Just that first bullet point feels like a college degree to me. Taken together, all of those points certainly do.
This whole list is a bit abstract though, so let’s apply it to something we can look at. What if this website was our current project?
Our brains and fingers go wild!
Let’s build the layout with CSS grid.
What fonts are those? Do we need to load them in their entirety or can we subset them? What happens as they load in? This layout feels like it will really suffer from font-shifting jank.
There are some repeated patterns here. We should probably make a card design pattern. Every website needs a good card pattern.
That’s a gorgeous color scheme. Are the colors mathematically related? Should we make variables to represent them individually or can we just alter a single hue as needed? Are we going to use custom properties in our CSS? Colors are just colors though, we might not need the cascading power of them just for this. Should we just use Sass variables? Are we going to use a CSS preprocessor at all?
The source order is tricky here. We need to order things so that they make sense for a screen reader user. We should have a meeting about what the expected order of content should be, even if we’re visually moving things around a bit with CSS grid.
The photographs here are beautifully shot. But some of them match the background color of the site… can we get away with alpha-transparent PNGs here? Those are always so big. Can any next-gen formats help us? Or should we try to match the background of a JPG with the background of the site seamlessly. Who’s writing the alt text for these?
There are some icons in use here. Inline SVG, right? Certainly SVG of some kind, not icon fonts, right? Should we build a whole icon system? I guess it depends on how we’re gonna be building this thing more broadly. Do we have a build system at all?
What’s the whole front-end plan here? Can I code this thing in vanilla HTML, CSS, and JavaScript? Well, I know I can, but what are the team expectations? Client expectations? Does it need to be a React thing because it’s part of some ecosystem of stuff that is already React? Or Vue or Svelte or whatever? Is there a CMS involved?
I’m glad the designer thought of not just the “desktop” and “mobile” sizes but also tackled an in-between size. Those are always awkward. There is no interactivity information here though. What should we do when that search field is focused? What gets revealed when that hamburger is tapped? Are we doing page-level transitions here?
I could go on and on. That’s how front-end developers think, at least in my experience and in talking with my peers.
A lot of those things have been our jobs forever though. We’ve been asking and answering these questions on every website we’ve built for as long as we’ve been doing it. There are different challenges on each site, which is great and keeps this job fun, but there is a lot of repetition too.
Allow me to get around to the title of this article.
While we’ve been doing a lot of this stuff for ages, there is a whole pile of new stuff we’re starting to be expected to do, particularly if we’re talking about building the site with a modern JavaScript framework. All the modern frameworks, as much as they like to disagree about things, agree about one big thing: everything is a component. You nest and piece together components as needed. Even native JavaScript moves toward its own model of Web Components.
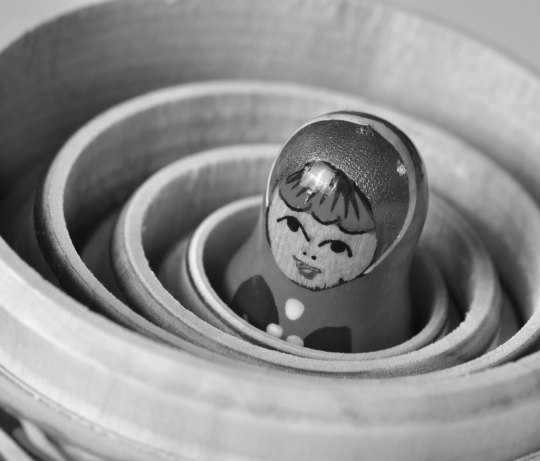
I like it, this idea of components. It allows you and your team to build the abstractions that make the most sense to you and what you are building.
Your Card component does all the stuff your card needs to do. Your Form component does forms how your website needs to do forms. But it’s a new concept to old developers like me. Components in JavaScript have taken hold in a way that components on the server-side never did. I’ve worked on many a WordPress website where the best I did was break templates into somewhat arbitrary include() statements. I’ve worked on Ruby on Rails sites with partials that take a handful of local variables. Those are useful for building re-usable parts, but they are a far cry from the robust component models that JavaScript frameworks offer us today.
All this custom component creation makes me a site-level architect in a way that I didn’t use to be. Here’s an example. Of course I have a Button component. Of course I have an Icon component. I’ll use them in my Card component. My Card component lives in a Grid component that lays them out and paginates them. The whole page is actually built from components. The Header component has a SearchBar component and a UserMenu component. The Sidebar component has a Navigation component and an Ad component. The whole page is just a special combination of components, which is probably based on the URL, assuming I’m all-in on building our front-end with JavaScript. So now I’m dealing with URLs myself, and I’m essentially the architect of the entire site. [Sweats profusely]
Like I told ya, a whole pile of new responsibility.
Components that are in charge of displaying content are almost certainly not hard-coded with data in them. They are built to be templates. They are built to accept data and construct themselves based on that data. In the olden days, when we were doing this kind of templating, the data has probably already arrived on the page we’re working on. In a JavaScript-powered app, it’s more likely that that data is fetched by JavaScript. Perhaps I’ll fetch it when the component renders. In a stack I’m working with right now, the front end is in React, the API is in GraphQL and we use Apollo Client to work with data. We use a special “hook” in the React components to run the queries to fetch the data we need, and another special hook when we need to change that data. Guess who does that work? Is it some other kind of developer that specializes in this data layer work? No, it’s become the domain of the front-end developer.
Speaking of data, there is all this other data that a website often has to deal with that doesn’t come from a database or API. It’s data that is really only relevant to the website at this moment in time.
Which tab is active right now?
Is this modal dialog open or closed?
Which bar of this accordion is expanded?
Is this message bar in an error state or warning state?
How many pages are you paginated in?
How far is the user scrolled down the page?
Front-end developers have been dealing with that kind of state for a long time, but it’s exactly this kind of state that has gotten us into trouble before. A modal dialog can be open with a simple modifier class like <div class="modal is-open"> and toggling that class is easy enough with .classList.toggle(".is-open"); But that’s a purely visual treatment. How does anything else on the page know if that modal is open or not? Does it ask the DOM? In a lot of jQuery-style apps of yore, yes, it would. In a sense, the DOM became the “source of truth” for our websites. There were all sorts of problems that stemmed from this architecture, ranging from a simple naming change destroying functionality in weirdly insidious ways, to hard-to-reason-about application logic making bug fixing a difficult proposition.
Front-end developers collectively thought: what if we dealt with state in a more considered way? State management, as a concept, became a thing. JavaScript frameworks themselves built the concept right in, and third-party libraries have paved and continue to pave the way. This is another example of expanding responsibility. Who architects state management? Who enforces it and implements it? It’s not some other role, it’s front-end developers.
There is expanding responsibility in the checklist of things to do, but there is also work to be done in piecing it all together. How much of this state can be handled at the individual component level and how much needs to be higher level? How much of this data can be gotten at the individual component level and how much should be percolated from above? Design itself comes into play. How much of the styling of this component should be scoped to itself, and how much should come from more global styles?
It’s no wonder that design systems have taken off in recent years. We’re building components anyway, so thinking of them systemically is a natural fit.
Let’s look at our design again:
A bunch of new thoughts can begin!
Assuming we’re using a JavaScript framework, which one? Why?
Can we statically render this site, even if we’re building with a JavaScript framework? Or server-side render it?
Where are those recipes coming from? Can we get a GraphQL API going so we can ask for whatever we need, whenever we need it?
Maybe we should pick a CMS that has an API that will facilitate the kind of front-end building we want to do. Perhaps a headless CMS?
What are we doing for routing? Is the framework we chose opinionated or unopinionated about stuff like this?
What are the components we need? A Card, Icon, SearchForm, SiteMenu, Img… can we scaffold these out? Should we start with some kind of design framework on top of the base framework?
What’s the client state we might need? Current search term, current tab, hamburger open or not, at least.
Is there a login system for this site or not? Are logged in users shown anything different?
Is there are third-party componentry we can leverage here?
Maybe we can find one of those fancy image components that does blur-up loading and lazy loading and all that.
Those are all things that are in the domain of front-end developers these days, on top of everything that we already need to do. Executing the design, semantics, accessibility, performance… that’s all still there. You still need to be proficient in HTML, CSS, JavaScript, and how the browser works. Being a front-end developer requires a haystack of skills that grows and grows. It’s the natural outcome of the web getting bigger. More people use the web and internet access grows. The economy around the web grows. The capability of browsers grows. The expectations of what is possible on the web grows. There isn’t a lot shrinking going on around here.
We’ve already reached the point where most front-end developers don’t know the whole haystack of responsibilities. There are lots of developers still doing well for themselves being rather design-focused and excelling at creative and well-implemented HTML and CSS, even as job posts looking for that dwindle.
There are systems-focused developers and even entire agencies that specialize in helping other companies build and implement design systems. There are data-focused developers that feel most at home making the data flow throughout a website and getting hot and heavy with business logic. While all of those people might have “front-end developer” on their business card, their responsibilities and even expectations of their work might be quite different. It’s all good, we’ll find ways to talk about all this in time.
In fact, how we talk about building websites has changed a lot in the last decade. Some of my early introduction to web development was through WordPress. WordPress needs a web server to run, is written in PHP, and stores it’s data in a MySQL database. As much as WordPress has evolved, all that is still exactly the same. We talk about that “stack” with an acronym: LAMP, or Linux, Apache, MySQL and PHP. Note that literally everything in the entire stack consists of back-end technologies. As a front-end developer, nothing about LAMP is relevant to me.
But other stacks have come along since then. A popular stack was MEAN (Mongo, Express, Angular and Node). Notice how we’re starting to inch our way toward more front-end technologies? Angular is a JavaScript framework, so as this stack gained popularity, so too did talking about the front-end as an important part of the stack. Node and Express are both JavaScript as well, albeit the server-side variant.
The existence of Node is a huge part of this story. Node isn’t JavaScript-like, it’s quite literally JavaScript. It makes a front-end developer already skilled in JavaScript able to do server-side work without too much of a stretch.
“Serverless” is a much more modern tech buzzword, and what it’s largely talking about is running small bits of code on cloud servers. Most often, those small bits of code are in Node, and written by JavaScript developers. These days, a JavaScript-focused front-end developer might be writing their own serverless functions and essentially being their own back-end developer. They’ll think of themselves as full-stack developers, and they’ll be right.
Shawn Wang coined a term for a new stack this year: STAR or Design System, TypeScript, Apollo, and React. This is incredible to me, not just because I kind of like that stack, but because it’s a way of talking about the stack powering a website that is entirely front-end technologies. Quite a shift.
I apologize if I’ve made you feel a little anxious reading this. If you feel like you’re behind in understanding all this stuff, you aren’t alone.
In fact, I don’t think I’ve talked to a single developer who told me they felt entirely comfortable with the entire world of building websites. Everybody has weak spots or entire areas where they just don’t know the first dang thing. You not only can specialize, but specializing is a pretty good idea, and I think you will end up specializing to some degree whether you plan to or not. If you have the good fortune to plan, pick things that you like. You’ll do just fine.
The only constant in life is change.
– Heraclitus – Motivational Poster – Chris Coyier
¹ I’m a white dude, so that helps a bunch, too. ↩️ ² Browsers speak a bunch more languages. HTTP, SVG, PNG… The more you know the more you can put to work! ↩️ ³ It’s an interesting bit of irony that WordPress websites generally aren’t built with client-side JavaScript components. ↩️
The post How to Think Like a Front-End Developer appeared first on CSS-Tricks.
You can support CSS-Tricks by being an MVP Supporter.
How to Think Like a Front-End Developer published first on https://deskbysnafu.tumblr.com/
0 notes
Text
The Widening Responsibility for Front-End Developers
This is an extended version of my essay “When front-end means full-stack” which was published in the wonderful Increment magazine put out by Stripe. It’s also something of an evolution of a couple other of my essays, “The Great Divide” and “Ooops, I guess we’re full-stack developers now.”
The moment I fell in love with front-end development was when I discovered the style.css file in WordPress themes. That’s where all the magic was (is!) to me. I could (can!) change a handful of lines in there and totally change the look and feel of a website. It’s an incredible game to play.
Back when I was cowboy-coding over FTP. Although I definitely wasn’t using CSS grid!
By fiddling with HTML and CSS, I can change the way you feel about a bit of writing. I can make you feel more comfortable about buying tickets to an event. I can increase the chances you share something with your friends.
That was well before anybody paid me money to be a front-end developer, but even then I felt the intoxicating mix of stimuli that the job offers. Front-end development is this expressive art form, but often constrained by things like the need to directly communicate messaging and accomplish business goals.
Front-end development is at the intersection of art and logic. A cross of business and expression. Both left and right brain. A cocktail of design and nerdery.
I love it.
Looking back at the courses I chose from middle school through college, I bounced back and forth between computer-focused classes and art-focused classes, so I suppose it’s no surprise I found a way to do both as a career.
The term “Front-End Developer” is fairly well-defined and understood. For one, it’s a job title. I’ll bet some of you literally have business cards that say it on there, or some variation like: “Front-End Designer,” “UX Developer,” or “UI Engineer.” The debate around what those mean isn’t particularly interesting to me. I find that the roles are so varied from job-to-job and company-to-company that job titles will never be enough to describe things. Getting this job is more about demonstrating you know what you’re doing more than anything else¹.
Chris Coyier Front-End Developer
The title variations are just nuance. The bigger picture is that as long as the job is building websites, front-enders are focused on the browser. Quite literally:
front-end = browsers
back-end = servers
Even as the job has changed over the decades, that distinction still largely holds.
As “browser people,” there are certain truths that come along for the ride. One is that there is a whole landscape of different browsers and, despite the best efforts of standards bodies, they still behave somewhat differently. Just today, as I write, I dealt with a bug where a date string I had from an API was in a format such that Firefox threw an error when I tried to use the .toISOString() JavaScript API on it, but was fine in Chrome. That’s just life as a front-end developer. That’s the job.
Even across that landscape of browsers, just on desktop computers, there is variance in how users use that browser. How big do they have the window open? Do they have dark mode activated on their operating system? How’s the color gamut on that monitor? What is the pixel density? How’s the bandwidth situation? Do they use a keyboard and mouse? One or the other? Neither? All those same questions apply to mobile devices too, where there is an equally if not more complicated browser landscape. And just wait until you take a hard look at HTML emails.
That’s a lot of unknowns, and the answers to developing for that unknown landscape is firmly in the hands of front-end developers.
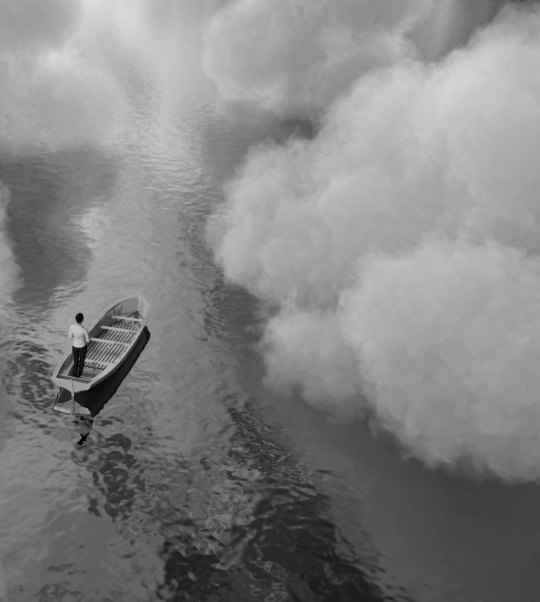
Into the unknoooooowwwn. – Elsa
The most important aspect of the job? The people that use these browsers. That’s why we’re building things at all. These are the people I’m trying to impress with my mad CSS skills. These are the people I’m trying to get to buy my widget. Who all my business charts hinge upon. Who’s reaction can sway my emotions like yarn in the breeze. These users, who we put on a pedestal for good reason, have a much wider landscape than the browsers do. They speak different languages. They want different things. They are trying to solve different problems. They have different physical abilities. They have different levels of urgency. Again, helping them is firmly in the hands of front-end developers. There is very little in between the characters we type into our text editors and the users for whom we wish to serve.
Being a front-end developer puts us on the front lines between the thing we’re building and the people we’re building it for, and that’s a place some of us really enjoy being.
That’s some weighty stuff, isn’t it? I haven’t even mentioned React yet.
The “we care about the users” thing might feel a little precious. I’d think in a high functioning company, everyone would care about the users, from the CEO on down. It’s different, though. When we code a <button>, we’re quite literally putting a button into a browser window that users directly interact with. When we adjust a color, we’re adjusting exactly what our sighted users see when they see our work.
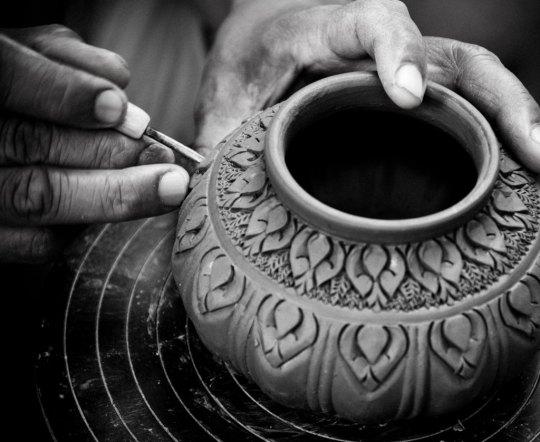
That’s not far off from a ceramic artist pulling a handle out of clay for a coffee cup. It’s applying craftsmanship to a digital experience. While a back-end developer might care deeply about the users of a site, they are, as Monica Dinculescu once told me in a conversation about this, “outsourcing that responsibility.”
We established that front-end developers are browser people. The job is making things work well in browsers. So we need to understand the languages browsers speak, namely: HTML, CSS, and JavaScript². And that’s not just me being some old school fundamentalist; it’s through a few decades of everyday front-end development work that knowing those base languages is vital to us doing a good job. Even when we don’t work directly with them (HTML might come from a template in another language, CSS might be produced from a preprocessor, JavaScript might be mostly written in the parlance of a framework), what goes the browser is ultimately HTML, CSS, and JavaScript, so that’s where debugging largely takes place and the ability of the browser is put to work.
CSS will always be my favorite and HTML feels like it needs the most love — but JavaScript is the one we really need to examine The last decade has seen JavaScript blossom from a language used for a handful of interactive effects to the predominant language used across the entire stack of web design and development. It’s possible to work on websites and writing nothing but JavaScript. A real sea change.
JavaScript is all-powerful in the browser. In a sense, it supersedes HTML and CSS, as there is nothing either of those languages can do that JavaScript cannot. HTML is parsed by the browser and turned into the DOM, which JavaScript can also entirely create and manipulate. CSS has its own model, the CSSOM, that applies styles to elements in the DOM, which JavaScript can also create and manipulate.
This isn’t quite fair though. HTML is the very first file that browsers parse before they do the rest of the work needed to build the site. That firstness is unique to HTML and a vital part of making websites fast.
In fact, if the HTML was the only file to come across the network, that should be enough to deliver the basic information and functionality of a site.
That philosophy is called Progressive Enhancement. I’m a fan, myself, but I don’t always adhere to it perfectly. For example, a <form> can be entirely functional in HTML, when it’s action attribute points to a URL where the form can be processed. Progressive Enhancement would have us build it that way. Then, when JavaScript executes, it takes over the submission and has the form submit via Ajax instead, which might be a nicer experience as the page won’t have to refresh. I like that. Taken further, any <button> outside a form is entirely useless without JavaScript, so in the spirit of Progressive Enhancement, I should wait until JavaScript executes to even put that button on the page at all (or at least reveal it). That’s the kind of thing where even those of us with the best intentions might not always toe the line perfectly. Just put the button in, Sam. Nobody is gonna die.
JavaScript’s all-powerfulness makes it an appealing target for those of us doing work on the web — particularly as JavaScript as a language has evolved to become even more powerful and ergonomic, and the frameworks that are built in JavaScript become even more-so. Back in 2015, it was already so clear that JavaScript was experiencing incredible growth in usage, Matt Mullenweg, the founding developer of WordPress, gave the developer world homework: “Learn JavaScript Deeply”³. He couldn’t have been more right. Half a decade later, JavaScript has done a good job of taking over front-end development. Particularly if you look at front-end development jobs.
While the web almanac might show us that only 5% of the top-zillion sites use React compared to 85% including jQuery, those numbers are nearly flipped when looking around at front-end development job requirements.
I’m sure there are fancy economic reasons for all that, but jobs are as important and personal as it gets for people, so it very much matters.
So we’re browser people in a sea of JavaScript building things for people. If we take a look at the job at a practical day-to-day tasks level, it’s a bit like this:
Translate designs into code
Think in terms of responsive design, allowing us to design and build across the landscape of devices
Build systemically. Construct components and patterns, not one-offs.
Apply semantics to content
Consider accessibility
Worry about the performance of the site. Optimize everything. Reduce, reuse, recycle.
Just that first bullet point feels like a college degree to me. Taken together, all of those points certainly do.
This whole list is a bit abstract though, so let’s apply it to something we can look at. What if this website was our current project?
Our brains and fingers go wild!
Let’s build the layout with CSS grid.
What fonts are those? Do we need to load them in their entirety or can we subset them? What happens as they load in? This layout feels like it will really suffer from font-shifting jank.
There are some repeated patterns here. We should probably make a card design pattern. Every website needs a good card pattern.
That’s a gorgeous color scheme. Are the colors mathematically related? Should we make variables to represent them individually or can we just alter a single hue as needed? Are we going to use custom properties in our CSS? Colors are just colors though, we might not need the cascading power of them just for this. Should we just use Sass variables? Are we going to use a CSS preprocessor at all?
The source order is tricky here. We need to order things so that they make sense for a screen reader user. We should have a meeting about what the expected order of content should be, even if we’re visually moving things around a bit with CSS grid.
The photographs here are beautifully shot. But some of them match the background color of the site… can we get away with alpha-transparent PNGs here? Those are always so big. Can any next-gen formats help us? Or should we try to match the background of a JPG with the background of the site seamlessly. Who’s writing the alt text for these?
There are some icons in use here. Inline SVG, right? Certainly SVG of some kind, not icon fonts, right? Should we build a whole icon system? I guess it depends on how we’re gonna be building this thing more broadly. Do we have a build system at all?
What’s the whole front-end plan here? Can I code this thing in vanilla HTML, CSS, and JavaScript? Well, I know I can, but what are the team expectations? Client expectations? Does it need to be a React thing because it’s part of some ecosystem of stuff that is already React? Or Vue or Svelte or whatever? Is there a CMS involved?
I’m glad the designer thought of not just the “desktop” and “mobile” sizes but also tackled an in-between size. Those are always awkward. There is no interactivity information here though. What should we do when that search field is focused? What gets revealed when that hamburger is tapped? Are we doing page-level transitions here?
I could go on and on. That’s how front-end developers think, at least in my experience and in talking with my peers.
A lot of those things have been our jobs forever though. We’ve been asking and answering these questions on every website we’ve built for as long as we’ve been doing it. There are different challenges on each site, which is great and keeps this job fun, but there is a lot of repetition too.
Allow me to get around to the title of this article.
While we’ve been doing a lot of this stuff for ages, there is a whole pile of new stuff we’re starting to be expected to do, particularly if we’re talking about building the site with a modern JavaScript framework. All the modern frameworks, as much as they like to disagree about things, agree about one big thing: everything is a component. You nest and piece together components as needed. Even native JavaScript moves toward its own model of Web Components.
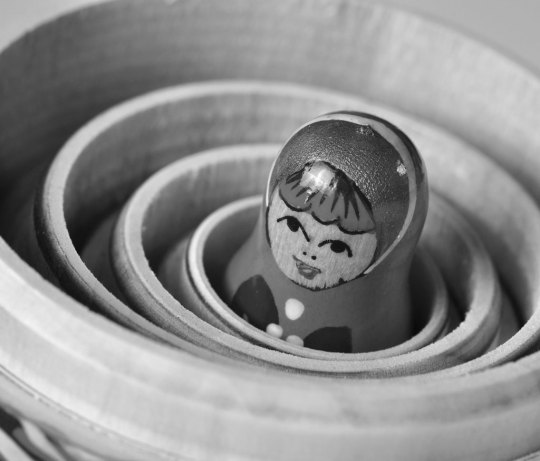
I like it, this idea of components. It allows you and your team to build the abstractions that make the most sense to you and what you are building.
Your Card component does all the stuff your card needs to do. Your Form component does forms how your website needs to do forms. But it’s a new concept to old developers like me. Components in JavaScript have taken hold in a way that components on the server-side never did. I’ve worked on many a WordPress website where the best I did was break templates into somewhat arbitrary include() statements. I’ve worked on Ruby on Rails sites with partials that take a handful of local variables. Those are useful for building re-usable parts, but they are a far cry from the robust component models that JavaScript frameworks offer us today.
All this custom component creation makes me a site-level architect in a way that I didn’t use to be. Here’s an example. Of course I have a Button component. Of course I have an Icon component. I’ll use them in my Card component. My Card component lives in a Grid component that lays them out and paginates them. The whole page is actually built from components. The Header component has a SearchBar component and a UserMenu component. The Sidebar component has a Navigation component and an Ad component. The whole page is just a special combination of components, which is probably based on the URL, assuming I’m all-in on building our front-end with JavaScript. So now I’m dealing with URLs myself, and I’m essentially the architect of the entire site. [Sweats profusely]
Like I told ya, a whole pile of new responsibility.
Components that are in charge of displaying content are almost certainly not hard-coded with data in them. They are built to be templates. They are built to accept data and construct themselves based on that data. In the olden days, when we were doing this kind of templating, the data has probably already arrived on the page we’re working on. In a JavaScript-powered app, it’s more likely that that data is fetched by JavaScript. Perhaps I’ll fetch it when the component renders. In a stack I’m working with right now, the front end is in React, the API is in GraphQL and we use Apollo Client to work with data. We use a special “hook” in the React components to run the queries to fetch the data we need, and another special hook when we need to change that data. Guess who does that work? Is it some other kind of developer that specializes in this data layer work? No, it’s become the domain of the front-end developer.
Speaking of data, there is all this other data that a website often has to deal with that doesn’t come from a database or API. It’s data that is really only relevant to the website at this moment in time.
Which tab is active right now?
Is this modal dialog open or closed?
Which bar of this accordion is expanded?
Is this message bar in an error state or warning state?
How many pages are you paginated in?
How far is the user scrolled down the page?
Front-end developers have been dealing with that kind of state for a long time, but it’s exactly this kind of state that has gotten us into trouble before. A modal dialog can be open with a simple modifier class like <div class="modal is-open"> and toggling that class is easy enough with .classList.toggle(".is-open"); But that’s a purely visual treatment. How does anything else on the page know if that modal is open or not? Does it ask the DOM? In a lot of jQuery-style apps of yore, yes, it would. In a sense, the DOM became the “source of truth” for our websites. There were all sorts of problems that stemmed from this architecture, ranging from a simple naming change destroying functionality in weirdly insidious ways, to hard-to-reason-about application logic making bug fixing a difficult proposition.
Front-end developers collectively thought: what if we dealt with state in a more considered way? State management, as a concept, became a thing. JavaScript frameworks themselves built the concept right in, and third-party libraries have paved and continue to pave the way. This is another example of expanding responsibility. Who architects state management? Who enforces it and implements it? It’s not some other role, it’s front-end developers.
There is expanding responsibility in the checklist of things to do, but there is also work to be done in piecing it all together. How much of this state can be handled at the individual component level and how much needs to be higher level? How much of this data can be gotten at the individual component level and how much should be percolated from above? Design itself comes into play. How much of the styling of this component should be scoped to itself, and how much should come from more global styles?
It’s no wonder that design systems have taken off in recent years. We’re building components anyway, so thinking of them systemically is a natural fit.
Let’s look at our design again:
A bunch of new thoughts can begin!
Assuming we’re using a JavaScript framework, which one? Why?
Can we statically render this site, even if we’re building with a JavaScript framework? Or server-side render it?
Where are those recipes coming from? Can we get a GraphQL API going so we can ask for whatever we need, whenever we need it?
Maybe we should pick a CMS that has an API that will facilitate the kind of front-end building we want to do. Perhaps a headless CMS?
What are we doing for routing? Is the framework we chose opinionated or unopinionated about stuff like this?
What are the components we need? A Card, Icon, SearchForm, SiteMenu, Img… can we scaffold these out? Should we start with some kind of design framework on top of the base framework?
What’s the client state we might need? Current search term, current tab, hamburger open or not, at least.
Is there a login system for this site or not? Are logged in users shown anything different?
Is there are third-party componentry we can leverage here?
Maybe we can find one of those fancy image components that does blur-up loading and lazy loading and all that.
Those are all things that are in the domain of front-end developers these days, on top of everything that we already need to do. Executing the design, semantics, accessibility, performance… that’s all still there. You still need to be proficient in HTML, CSS, JavaScript, and how the browser works. Being a front-end developer requires a haystack of skills that grows and grows. It’s the natural outcome of the web getting bigger. More people use the web and internet access grows. The economy around the web grows. The capability of browsers grows. The expectations of what is possible on the web grows. There isn’t a lot shrinking going on around here.
We’ve already reached the point where most front-end developers don’t know the whole haystack of responsibilities. There are lots of developers still doing well for themselves being rather design-focused and excelling at creative and well-implemented HTML and CSS, even as job posts looking for that dwindle.
There are systems-focused developers and even entire agencies that specialize in helping other companies build and implement design systems. There are data-focused developers that feel most at home making the data flow throughout a website and getting hot and heavy with business logic. While all of those people might have “front-end developer” on their business card, their responsibilities and even expectations of their work might be quite different. It’s all good, we’ll find ways to talk about all this in time.
In fact, how we talk about building websites has changed a lot in the last decade. Some of my early introduction to web development was through WordPress. WordPress needs a web server to run, is written in PHP, and stores it’s data in a MySQL database. As much as WordPress has evolved, all that is still exactly the same. We talk about that “stack” with an acronym: LAMP, or Linux, Apache, MySQL and PHP. Note that literally everything in the entire stack consists of back-end technologies. As a front-end developer, nothing about LAMP is relevant to me.
But other stacks have come along since then. A popular stack was MEAN (Mongo, Express, Angular and Node). Notice how we’re starting to inch our way toward more front-end technologies? Angular is a JavaScript framework, so as this stack gained popularity, so too did talking about the front-end as an important part of the stack. Node and Express are both JavaScript as well, albeit the server-side variant.
The existence of Node is a huge part of this story. Node isn’t JavaScript-like, it’s quite literally JavaScript. It makes a front-end developer already skilled in JavaScript able to do server-side work without too much of a stretch.
“Serverless” is a much more modern tech buzzword, and what it’s largely talking about is running small bits of code on cloud servers. Most often, those small bits of code are in Node, and written by JavaScript developers. These days, a JavaScript-focused front-end developer might be writing their own serverless functions and essentially being their own back-end developer. They’ll think of themselves as full-stack developers, and they’ll be right.
Shawn Wang coined a term for a new stack this year: STAR or Design System, TypeScript, Apollo, and React. This is incredible to me, not just because I kind of like that stack, but because it’s a way of talking about the stack powering a website that is entirely front-end technologies. Quite a shift.
I apologize if I’ve made you feel a little anxious reading this. If you feel like you’re behind in understanding all this stuff, you aren’t alone.
In fact, I don’t think I’ve talked to a single developer who told me they felt entirely comfortable with the entire world of building websites. Everybody has weak spots or entire areas where they just don’t know the first dang thing. You not only can specialize, but specializing is a pretty good idea, and I think you will end up specializing to some degree whether you plan to or not. If you have the good fortune to plan, pick things that you like. You’ll do just fine.
The only constant in life is change.
– Heraclitus – Motivational Poster – Chris Coyier
¹ I’m a white dude, so that helps a bunch, too. ↩️ ² Browsers speak a bunch more languages. HTTP, SVG, PNG… The more you know the more you can put to work! ↩️ ³ It’s an interesting bit of irony that WordPress websites generally aren’t built with client-side JavaScript components. ↩️
The post The Widening Responsibility for Front-End Developers appeared first on CSS-Tricks.
You can support CSS-Tricks by being an MVP Supporter.
😉SiliconWebX | 🌐CSS-Tricks
0 notes
Text
Hire Bigcommerce Designer For Store Creation And Popularity - Web Site Design
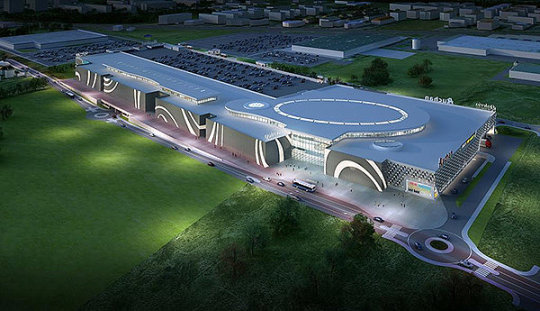
VxPLORE TECHNOLOGIES - if you wish to build and grow your online business you'll be able to believe on Bigcommerce without the doubt. Here by web business our company is referring to ecommerce. Ecommerce or electronic commerce as the name indicates is the fact that type of commercial activity where in actuality the buying and selling of goods and services happens along Internet mechanism. Internet could be the new means of dealing and communicating. Through the task there is done a communication between buyer and seller, business to business and people to individuals. Once we talk of business, we refer to the online store. Creation of the store can happen with the aid of a platform. Between the available platforms Bigcommerce tops the list. In this article we would mention the good reason for preferring Bigcommerce as well as some top features of the working platform would be looked at. How come Bigcommerce preferred? The working platform is recommended by one and all since it comes with all that is necessary for initially building after which growing an online business. It functions differently off their ecommerce solutions and could possibly be praised for the features like marketing tools, SEO, coupons and much more. These features are not an addition towards the platform later. Instead they've been included from the very beginning. 1. They will have ab muscles theme that is useful within which are found both free along with premium design templates. 2. There is the accessibility to the style editor along which customization associated with website is both easy and swift. 3. The design themes associated with platform are useful and responsive. 4. There clearly was the chance of this integration of this design that is existing. 5. HTML and CSS are editable within the browser regarding the platform. 6. The control interface for the platform works along Web. 7. The Email templates could be edited directly through the control panel itself. 8. Static website text in the platform could possibly be edited along mouse. 9. Bigcommerce works properly in all the web that is well-known. 10. There is presence of diff tool through which comparison that is template be performed. In addition to the above features there are the excellent features for photos as well. 2. The photos to the Webstore created along maybe it's added from one's personal website or computer. 3. The Homepage carousel highlights this product images in the platform. 4. The image listing for product pages is automatically done. 5. There are photo thumbnails in the product pages. 6. Super zoom instant photo zooming is contained in the working platform. 7. The Thumbnail photos are created very quickly through it. 8. Dozens of images may be uploaded at the same time with the aid of platform. 9. There is certainly an image manager into the platform which helps in image management that is best. 10. There is certainly presence of pop up window for larger images within the platform. 11. There was found alt text and description per image within the platform. 12. The photos might be shared between items in Bigcommerce. With so many advantageous assets to boast of, Bigcommerce is surely the highest quality platform in existence. Should you want to get best advantage from it then you can certainly Hire Bigcommerce Designer.
Can be your girl a makeup hoarder?
Are you planning to visit your mall that is nearest to get a unique gift for the ladies in your lifetime? There are multiple boring gifts on the market but if you are searching for a gift that is unique is the right place to get started. From the comfort of tech gadgets to trendy perfume, we now have covered everything her life easier that she will like and things that can make. These gift that is smart work great around the year and that can be gifted on most occasions. Let’s honour the special ladies in your lifetime with your stylish gifts. You can gift a smart speaker to the tech lovers in your life. The smaller Amazon speakers that are smart more attractive than originals and appear in the budget. You are able to ask Alexa to relax and play music, multiple questions, make phone calls and play games. In every, this smart device can help your spouse control all of the basic actions. The best benefit is it’s not that hard to utilize and comes at a reasonable price. Does your girlfriend always go out of battery? Here is the best possiblity to gift her a charging card that may easily fit inside her wallet. The size of the card that is charging somewhere around three debit cards stacked. The part that is best is that there are multiple colours to select from and certainly will charge up to 50% associated with battery. Some girls carry the house that is entire their bag. What better thing to gift them the tote that is famous of the season? Not just will she love carrying the trendy bag but can stuff exactly what she needs in this bag easily. You can have fun with colours and gift her a thing that is her wardrobe staple colour. This is certainly a "must buy" beauty product for the style enthusiast. When your women love getting dressed and applying makeup you'll be able to gift her a collection of makeup brushes and she's going to be likely to love testing out the brushes to create the newest trends. Is the girl a makeup hoarder? Has she made beauty that is recent? Does she love adding new beauty that is trendy to the makeup collection? You can buy her a cosmetic organizer that can make her makeup storage neat. This will be a great product for travelling purposes. This cheap gift for women is a lifesaver for girls who finds it difficult to stay organized. Let her eyes do the talking when time that is next gets ready for any occasion. 3D Mink Lashes is the gift that is perfect her to bring game-changer makeup product in her vanity. Which women do not like a good couple of glam sunnies?
Free BigCommerce Teaching Servies
These anti-reflective photochromic frames not merely look flattering but fulfils their everyday needs that are basic. You will see how this set of glasses will become her choice that is go-to soon. Make her hands look stick out along with his crystal UV Nail Gel. Gifting her this product will save her visits that are many the parlour. The product will come in three different colours and the size which makes it simple to stash it in her tote bag whenever venturing out. Would you not prefer having a touch of glamour inside their everyday products? Gift her a women’s that is fashionable in various colours to create her bag match her attire. These compact that is fashionable are really easy to carry and are offered in multiple vibrant colours. This year is about the earrings that are geometrical. Most of these earrings are regarding the trend and an pick that is affordable well. It is possible to gift her these earrings in a variety of colours with her outfit and make a true fashion statement so she can match it. Your girl will want to reside in this warm pullover forever. This soft, comfy, lightweight pullover is something in which you can spend your complete day. In addition get to choose from a number of colours. So without giving a thought that is second buy this for your lazy friend or girlfriend inside her favourite colour. Getting the gift that is right all your family members comes with great anxiety. You wish to say thanks to your personal ladies in the way that is perfect. The above-discussed gift ideas can win you praises for several years in the future. All are a combination that is great of and unique. Let’s gift something unusual gift for women on birthdays, anniversaries along with other occasions while making them feel special. Please enable JavaScript to view the comments powered by Disqus. Father is the most person that is important a child’s life. He sacrifices all his dreams in order to make his kids happy. And him feel special, so why not utilize it if you get a day to make? As Father’s Day is practically here, you should gift something unique to him and show your love. Flowers beautify life. Also to put a smile on someone's lips, a bouquet is just the thing. Flowers are a really popular additional gift, specifically for birthdays, weddings, as a declaration of love or perhaps as an gift that is everyday. Today we could find a variety that is wide of to hand out on a birthday. But precisely due to the diversity that exists, it is often tough to result in the right choice. However, when your time has arrived and you also have no idea why to determine, you have to know that there is a present that may go out of never style: a bouquet. It is known by you is not difficult to impress your girlfriend if she really loves you. Girls are extremely sensitive. They have to be handled with love and care. As a boyfriend, it really is your responsibility to help make her feel special and become there for her whenever she needs you. Gifts are meant to be cherished. This is why it’s important that you choose just the gift that is right. The gift which you choose is determined by a number of things. The individual you would like to supply the gift while the reason or occasion. If a friend or cherished one is having a housewarming party within the future that is near you are wondering: what can I bring? You can find a lot of housewarming that is different ideas out there; it may be hard to find a thing that is clearly worth bringing. Looking to Buy a $100 Gift? Listed below are 5 gift suggestions You Can Get! Attending an event soon? Maybe it’s a birthday. Or, maybe someone near to you is celebrating something. Maybe it's a romantic anniversary, a wedding, or a graduation party! Gift, the very mention of word is sufficient to excite us. International travelers love collecting souvenirs wherever each goes. These remind them for the places they are and the cultures and folks they will have seen close up. The idea of regifting something which has already been gifted to you within the disguise of a brand new packaging has existed for a long time. Earlier it was considered a negative thing to do because it shows disrespect towards the person that gave you the gift to begin with. Copyright 2005-2020 - ArticleCube, All rights reserved. Use of our service is protected by our online privacy policy and Terms of Service.
Seeking Alpha
Unlimited bandwidth - not limiting the number of visitors your store can handle
Sales attribution for specific staff members
Day Range 76.53 - 81.90
Episode 4: qualified advice on acing a
BigCommerce Holdings, Inc. (BIGC) CEO Brent Bellm on Q2 2020 Results - Earnings Call Transcript
24/7 live chat & phone support
youtube
VxPLORE TECHNOLOGIES - the experience of ecommerce is pace that is fast catching the companies which can be trying to keep carefully the sales alive believe on the method. No one would have dreamt about the process to happen before some years. But, it is the miracle of technology that the game is happening. The positive thing is that both small as well as large sized companies are turning to this method. You must also turn to this process if you have been looking for a better sale. In making it happen you can count on the internet site creation platforms and produce a good Website for the company. Amongst all the platforms, the one referred to as Bigcommerce is becoming the trend. Bigcommerce customization might sound easier, but, the very best results are acquired once the experts are engaged because of it. In this article we would talk regarding the Bigcommerce customization process in more details so we would also highlight some bits about the activity of ecommerce. Ecommerce can also be known as the electronic commerce. This is actually the process that is commercial happens with the aid of internet. Also, it relates to the entire process of purchasing as well as selling the stuff with the aid of internet mechanism. Beneath the group of stuff are included goods, services and both. The development of internet has only result in the electronic commerce. Why do people prefer the way that is commercial? Humans search for the least way that is tedious perform a job. The thing that is good the ecommerce is the fact that it can help in purchasing and selling with ease. Even the simple process of book purchase happens easily along with it. Imagine, that you don't need certainly to travel kilometers for purchasing your preferred book. The process can not only make reading a far more pleasure activity, but would also provoke you to again visit the place to do the book purchase. How does Webstore help in ecommerce? Webstore helps in the easy activity that is e-commercial allowing the sellers make an excellent quality Website. A Website could be the primary need for starting the online process of selling. A Webstore that is popular for the process is bigcommerce. Individuals are looking at professionals for performing bigcommerce customization as with them the very best in market customization could be done. Bigcommerce is the most popular of the many ecommerce platforms. It is because you can find in-built templates within it. These templates allow easy modification associated with store features and makes selling a pleasurable activity. Also, there is present within it the feature of security. As a result of feature of security the activity of buying and selling is now more popular using them. So, have the Bigcommerce customization done along with the good quality professionals. It's important to keep a keen eye on the trends, your company requirements, costs and even more. As a result the business would certainly bear profit through Bigcommerce. So, wait you can forget and acquire in touch with experts for making use of Bigcommerce for the organization.
Monday's Senate decision drew concern and shock over the nation from smaller businesses who sell online, and rightfully so. The Marketplace Fairness Act (MFA) would grant states the authority to compel retailers that are online no matter where they truly are located, to collect sales tax during the time of a transaction. Today, online sellers are just necessary to charge sales tax in states where they will have a office that is physical warehouse. The MFA, backed by President Obama, is purportedly targeted at leveling the playing field between brick-and-mortar stores and the ones who don't have a presence that is physical. Of course, you don't have to look way too hard to see that is the beneficiary that is real of proposed legislation. Just consider the ongoing companies throwing their weight behind it -- Amazon, Walmart, Target. All of them have major selling that is online, and all sorts of of those are able to afford to cope with the added costs and hassles that come with paying taxes in most state by which they sell. Of course, Amazon is ostensibly the target with this legislation. The idea is the fact that forcing them to cover taxes means they no more have the advantage that is tax-free brick-and-mortar stores. To be honest, not tax that is charging orders is no longer a genuine advantage for Amazon -- and additionally they know it. A couple of years ago, Amazon would have fought this bill with everything they have, just them to pay sales tax as they did all other attempts to force. More recently, they've realized that convenience is a much bigger advantage to capitalize on. Manufacturing that convenience meant building more warehouses atlanta divorce attorneys state, which often meant they had taxable nexuses. Amazon was already headed down this road. The irony is, needless to say, that Amazon built their business on having lower prices with no tax. Yet again they will have scaled to your point where it no longer matters, they could bring their lobbying might to bear on the side of Internet taxes and cripple their smaller competitors who can not afford to keep up with compliance. And it could be crippling. Most small company selling online simply don't possess enough time or money to accomplish the paperwork essential to file taxation statements in 45 additional states -- and keep in mind that they'd want to do so monthly. While Amazon are able to afford to throw entire teams of people during the problem, an online store run out of a home with only some employees (if that) can't make it work well. Many businesses operate at margins of only 10-20 percent, so their actual income and capability to cover these incremental operating costs are comparatively low. And that is who we're referring to, here.
0 notes
Text
The entrepreneurial attitude – transcript from my tedx bundaberg communicate
So, the phrase entrepreneur, let’s begin there. Has every person ever looked this up within the dictionary? I’d in no way heard the word entrepreneur when i was at faculty. In no way did Digital Marketing Company Nottingham absolutely everyone say “you! The lady that talks in magnificence and gets kicked out an entire lot”
“you! The girl that gets suspended”

“you! The girl that is clever – but doesn’t seem to be engaged in a whole lot of this content material”. That become me. No person ever stated “you understand what? Maybe you have to reflect onconsideration on being an entrepreneur.”
and this is pretty sudden to me due to the fact in case you haven’t already picked it up, i’m from wellington, new zealand and i went to a certainly innovative faculty – it was known as wellington high faculty that is now over one hundred and thirty years antique. We known as teachers with the aid of their first names, it became co-ed; boys and girls, we didn’t wear a uniform. Inside the senior years it become move if you need and don’t go if you don’t need – which didn’t suit every body. We should study topics like journalism and horticulture which became notable, but nonetheless, the simplest pathway they ever presented to us in 1994, which become the yr i ended excessive college, turned into which you go proper through to yr 12, that you then pass and get a tertiary education and then you definitely get a process. That’s all i ever knew. Each my parent didn’t run agencies, one became a instructor at my school, so that you can believe how mortified he become at my behaviour at instances. No person ever supplied this idea of being an entrepreneur. Whilst i've heard the phrase entrepreneur over time, on occasion it’s were given terrible connotations, hasn’t it? Check these phrases at the slide in the back of me. I imply who desires to be a multi-millionaire? A magnate? A dealer? What about a multi-millionaire, or a massive shot, or a big wig? Or maybe a whizz-youngster. So the use of the word “entrepreneur” virtually dates back to the 1800s and it’s a french word. And through the years it has grown in popularity. I feel like in recent years if something, the word entrepreneur has taken on a life of its personal and is perhaps even over used – wouldn’t you settle? These days, i’m pretty sure while you’re growing up in new zealand, australia or elsewhere inside the international that pretty possibly, and that i simply hope this is actual, you're advised approximately the opportunity to head on a direction of building your very own enterprise – if that’s what you want to do. And that i implore all of you too, that even if you have taken the conventional course of analyzing and going and getting a task. That at any time you can choose to do a “aspect hustle” or even assignment out and start a new business. Did you already know that the colonel from kentucky, that made the famous kentucky fried fowl or kfc, didn’t start his business till he changed into in his 70s?! So in case you need to be an entrepreneur or you simply need to have an entrepreneurial attitude that’s a piece greater like an entrepreneurial person – what does that mean? To me it way a number of various things. It approach to think differently and all the ones times i used to be at high college stepping into trouble, struggling with the educational work, it’s due to the fact i notion otherwise, and i simply didn’t fit the mold. So in case you’re deliberating a person right now that feels like that, whether it’s your personal toddler or a nephew or a niece or someone you’ve taught, possibly, just perhaps, they’re an entrepreneur too. To tell you the way i came to exercise session i used to be an entrepreneur (and how it wasn’t one of these horrific factor and has in reality brought me a lot of tremendous opportunities in this life) permit me let you know a bit about my adventure. As i stated, i began life in wellington new zealand wherein i used to be born and bred till the age of 21. I created my first business when i used to be 17 years antique when i determined to begin a newspaper even as at college. As i stated, a number of the topics at school weren’t for me – i’d by no means touch computers until i had to do a journalism path and needed to discover ways to type out my story. Then my dad said “you have to begin a newspaper and you ought to do it on recreation,” and i concept “that sounds extremely good to me because i like sport!”
in reality at that time i was inside the new zealand crew for water polo for my age organization and i educated a lot. I additionally did a number of swimming and quite a few surf lifestyles-saving and i gained loads of medals. I was a water baby i wager you could say. And the thing that got me surely inquisitive about starting the newspaper – we all must be stimulated with the aid of some thing – and for me, at age of 17, it become cash. I wanted cash to pay for my recreation, which became unfunded. My parents didn’t have the cash to keep putting in to pay for the uniforms or the trips and all of the costs related to sport. So, i began that newspaper. And in those days, for the ones folks vintage sufficient to recollect, it turned into bromide, not virtual at all. And that i had a number of joy dropping off in my little pink mini to every high school in wellington, a package of newspapers. And as it became made via children, for children, they gobbled it! Then i get this enterprise call from an american man who stated ‘you’ve stolen my concept!’ he turned into high-quality pissed off at me so i stated “how could i've stolen your idea? I’ve by no means met you, i don’t realize who you're or what your idea even is!”
properly it turns out that this man had been making plans to start a secondary school sports newspaper loads like mine for about two years. And here was me, coming along in any respect of 17 years vintage and i just began this thing, and i thought such things as $200 complete page advertisements became exquisite for installing the financial institution to pay for my game. So he demanded a meeting with me and here i am, my first boardroom meeting as a 17 years vintage, with my dad there for guide, slicing my first enterprise deal. And you’re probably questioning what that frypan reference is up at the display screen? I’ll inform you now. Years later, a guy in silicon valley said to me, “ you already know companies are lots like pancakes – you stuff the primary one up,” and that i said, “ oh my god, that’s so genuine for me!” due to the fact that newspaper was the first enterprise and without know it i cut that deal and it ended up being a truely bad commercial enterprise deal once i look again. It become a wage, a small lump sum and that i had to maintain to paintings for him for the relaxation of the 12 months. Which for me, become nice on the time, due to the fact i just wanted to train and get the cash to head and play towards australia later that year. Nicely i did what i used to be requested, and that i learnt my first very good business lesson. Which is, if making a decision to go into business with a person, or whatever it's miles you decide to do as an entrepreneur, ensure it aligns together with your values. On this revel in, his values and my values did no longer align. And it didn’t workout. On the stop of the 12 months we parted ways and that changed into the give up of my first commercial enterprise. For the subsequent seven years, from age 21 to 28 i travelled the arena and that i supplemented my travels with travel writing, so i wager you can say i was a travel blogger before it was even a issue. So that’s my 2d tip on becoming an entrepreneur or growing an entrepreneurial attitude, you need to create your own opportunities. Human beings aren’t necessarily going to say “hello, you must do that” every now and then you’ve simply were given to assume “i want to try this. How can i make it happen?” then make it show up! Speedy forwarding in my tale, i fell pregnant at the pill, in london, and i needed to training session what to do subsequent. By using this stage, i was the editor of a newspaper at age 26. So, i used to be doing quite nicely with the profession component. However to fall pregnant at the pill in london, without a doubt made me re-assume existence and what i used to be going to next. We ultimately had the kid, and for some time there we stuck it out in london looking to make things work, however while it have become too tough we decided to move again to wherein our circle of relatives lived, which became australia. So then, a few years skip, i locate some paintings in australia after which i've a 2nd baby. So now i have a 3 yr vintage and a new child at home and that i assume, “how am i going to earn cash now?!”
so i start any other business! Running from domestic around my two kids have been the standard beginnings of my 0. 33 commercial enterprise, the innovative collective. And in fact, i didn’t mention that during among my “pancake business” (the newspaper) and my career in london i additionally commenced a web enterprise selling t shirts known as “tikanga teeshirts”. Tikanga means “culture “and tikanga o te wa – those are maori phrases i’m the usage of – manner fashion. I created that enterprise due to the fact i used to be honestly happy with our indigenous subculture and language in new zealand. Though i am no longer maori, i was introduced up with it. And that i wanted to percentage with human beings that i was a proud new zealander and here became our subculture. So the teeshirt business i started out in 2002 and not using a capital. I put up a internet site up after teaching myself html, and 4 years later, offered it for 5 figures. In order that changed into an awesome outcome, doing the overall cycle of the business, truly better than the first pancake. But the 0. 33 business, the creative collective, that’s in which all of it truly commenced. I got a logo designed via a friend, i were given that revealed in an a3 format and laminated, put it up within the have a look at and growth we’re in commercial enterprise! I then threw up a internet site (now we’re really in enterprise), i made a enterprise card (hey everyone, i’ve got a business!)
in those days it wasn’t very common for mums to work at home – or it didn’t seem like it changed into. I didn’t have many friends to name on. But it’s become an increasing number of famous now and that i think that this is incredible, that parents who pick to stay at domestic and lift children can nevertheless earn an earnings and do some thing they love. Now nowadays, the creative collective has 12 staff throughout offices on the sunshine coast and newcastle and approximately 40 contractors. I very own a business constructing that we operate out of at the sunshine coast and we have clients all over australia and even some worldwide ones. It has a by-product organisation known as the schooling collective, wherein we train human beings digital competencies. And importantly, we’ve had a lot of amusing with it all. Now there’s a super metaphor obtainable approximately what it takes to be an entrepreneur that i’d like to percentage with you. An entrepreneur says to a mentor, “be my mentor, display me what it takes to be an entrepreneur.”
the mentor says, “k come meet me down at the water early when it’s surely certainly dark and cold out”. The entrepreneur meets the mentor and on arrival the mentor says “walk with me” and absolutely dressed heads directly into the water. The entrepreneur says to the mentor “wait! I need to get undressed. I’ll get wet…”
the mentor says “no you don’t simply stroll to your clothes”. In order that they enter the water, that is clearly honestly bloodless, and are up to their knees of their garments. The entrepreneur says “oh man that is uncomfortable! What are we doing? This is horrible!”
the mentor smiles and lightly says “that’s proper, just preserve on foot”. In order that they keep walking deeper and deeper into the water, and i ought to truely problematic on this story, but the point is, they stroll until they’re up to their necks, and the entrepreneur at this factor is in reality struggling to preserve his head above water because his clothes are wet, he’s freezing bloodless, and the whole thing is weighing him down.
“i hate this!” he again complains to the mentor.
“i need to get out! I will’t cope! I’m going to drown!” he yells desperately to the mentor. And when he receives to date, the mentor says, “my pal, that is what it takes to be a an entrepreneur. You’ve got to be prepared to get from your comfort sector. You’re going to swim into un-chartered waters. You’re going to be uncomfortable, and you’ll once in a while feel like you’re sinking. At times you can even assume you’re drowning, but you’ve just were given to maintain going. You’ve got to attempt to swim even when things are weighing you down.”
so that’s any other tip i've for all the budding entrepreneurs obtainable. You’ve were given to be prepared to get from your consolation region and make it paintings! My first step to get out of my comfort region, was coming into a enterprise award. I were in business simply six months with the creative collective, and that i thought “hello, i need to market my enterprise, or give some thing a move here to get the phrase available approximately my enterprise.”
so i throw my hat into the small enterprise champions awards, and i couldn’t trust it. I received! I won the young entrepreneur of the year award in queensland in 2007. Out of this enjoy i realised that coming into business awards worked quite nicely and that i might need to do extra of that due to the fact the phones began ringing, and commercial enterprise started coming in. I also met a few extraordinary human beings at that occasion. Off the again of winning that award, i were given supplied to go on tv, on a country wide show about specific companies. On it they depicted me as the mum who labored from home juggling my younger ones that is precisely what i was doing at the time. That equal piece came out on channel nine, after which featured on the vodafone website and on qantas’s inflight television and things definitely took off. And this changed into all from going out of doors my consolation sector and coming into a business award. The alternative matters i’ve learned along my years of being an entrepreneur, is that you want to be open to new experiences, places and people. That's precisely why i say, yes to riding 3 hours to talk at a bundaberg tedx occasion. I wanted to come back up to meet new human beings and feature a brand new experience in a brand new area. Via my
Read Also:- Top 10 Ways to Use AI in Brand Management
entrepreneurial/commercial enterprise journey, i’ve surely been able to do this. As an instance this is me in the big apple, coming into the worldwide girls in business awards. And in that 12 months, my children have been elderly 2 and 5, the currency exchange fee changed into terrible and that i felt so responsible leaving them to wait those awards. I didn’t win that award, however it turned into nevertheless so really worth going and being open to those new reports. As a result of attending that event, that night time, i went night time clubbing, as you do with the ceo of the complete awards. And he supplied me an possibility to sell those awards in australia and new zealand, which i then did for the subsequent 10 years. And that has changed into having connections with a number of the nice commercial enterprise people in australian and new zealand, which has been an exceptional experience as properly. I suppose you want to place yourself accessible, and do not forget you've got as a lot proper to be there as anyone else. Through entering any Digital Marketing Companies in Nottingham other business award application, the telstra commercial enterprise womens awards and prevailing enterprise owner of the yr in queensland, extra opportunities unfolded for me. I got invited to go to silicon valley, which in case you don’t recognise, is the tech capital of the arena.
Follow US:- Facebook, Twitter, LinkedIn , YouTube
0 notes
Text
MarketPresso Review And Bonus
MarketPresso is the World's FIRST & ONLY contractor in the world that assists you develop your "Own Marketplace".
Gain even more money than ever by selling your digital items, physical services or products.
Do freelance work or construct your company-- whatever is currently an opportunity with your own customized white-labeled industry.
Just how to Develop an Online Industry using WordPress (Component 2)
After creating all the pages and including shortcodes to them, you can select them right here.
Listed below the web pages, you will certainly likewise discover 'Shop settings' option on the same page. This where you can choose a prefix to use in Supplier shop Links, permit them to establish customized headers for their shop web pages, and also use HTML in shop summary.
Following action is to establish payments for your vendors. MarketPresso established a minimal limit for their vendors and also pay them on a regular monthly or once a week basis.
We recommend making use of manual payments to suppliers as this gives clients enough time to demand refunds or give feedback concerning the items.
However, if you intend to repayment withdrawal system for suppliers, then you can buy premium attachments. WC Vendors has add-ons available for Red stripe, MangoPay, Escrow, and Manual Payments.
Depending upon the payment portal you pick, you will require to establish a repayment gateway by entering your API secrets. Don't forget to click on the 'Conserve Modifications' switch to store your settings.
Now that WC Vendors prepares, allow's set up WooCommerce for a multi-vendor setting.
Action 3. Allow Account Administration in WooCommerce
First you need to check out WooCommerce" Settings web page as well as click the 'Accounts' tab. From here you need to examine packages alongside client enrollment alternative.
Don't fail to remember to conserve your adjustments.
Tip 4. Establishing Navigation Menus
Now that your multi-vendor MarketPresso arrangement is ended up. It is time to make it simple for your users to locate their means around your site.
To do that, go to Look" Menus page. From here you require to add your customer account and check out pages to the navigation food selection.
Do not neglect to click the 'Conserve Food selection' button to save your changes. For more detailed guidelines, see our overview on exactly how to add navigating menus in WordPress.
If you do not have a My Account web page, then simply develop a brand-new web page in WordPress and include the following shortcode in the article editor.
[woocommerce_my_account] Tip 5. Testing Your Industry Website
Your on the internet market site is now all set for testing. You can visit your web site in a new internet browser home window as well as create a brand-new account by clicking on the My Account link on top.
From here, both consumers as well as suppliers can log in to their accounts along with develop a new account.
Once individuals produce a new account, you will certainly receive an email notice. If you are not able to receive email notifications, after that have a look at our overview on exactly how to take care of WordPress not sending out e-mail issue.
You can likewise watch brand-new supplier applications by going to Users" All Individuals web page. You will see all brand-new supplier demands as 'pending vendor', and also you can approve or deny applications by clicking the web link under their username.
As soon as authorized, these vendors can visit to their accounts as well as add their items by visiting their supplier control panel. They can additionally view their orders and sales reports.
The first thing your vendors require to do is to set up their store settings by clicking on the 'Shop Settings' web link.
Relying on the settlement methods you established, they will certainly require to offer their PayPal or Stripe email address to obtain payments. They will certainly additionally have the ability to offer checking account info for direct hands-on settlements.
As soon as a supplier adds a brand-new product, you will certainly get a notification e-mail as well as see a symbol next to the products menu. You can after that edit an item, accept it, or delete it.
Your store web page will plainly show the products offered by vendor's store name.
Action 6. Expanding your Online Market Site
Initially, you may want to choose a design for MarketPresso. WordPress features hundreds of free as well as paid styles however not all of them are eCommerce prepared.
See our choice of the best WooCommerce themes to discover an ideal theme for your market platform.
Afterwards, you would certainly want to include new features to your internet site. As an example, making it a multi-vendor public auction site or a subscription neighborhood.
MarketPresso Evaluation & Overview
Supplier: karthik Ramani et al
Product: MarketPresso
Introduce Day: 2020-Feb-02
Release Time: 11:00 EST
Front-End Price: $37
Sales Page: https://www.socialleadfreak.com/marketpresso-review/
Specific niche: Software application

MarketPresso will certainly aid your consumers make more money than ever before by giving them a system where they can sell all their products/services to their clients/customers.
To be straightforward, "MarketPresso" will make our environment become more POWERFUL by providing a "genuine" product that helps them generate income from all the products/services they have ever before bought from us.
As a matter of fact, also in the future, it will aid act as an angle for all your future products/services where you can just refer to "MarketPresso" as a one-stop platform for offering their products/services.
This is what we call a "Game Changer".
We have kept in mind all the minute information of the following products/services in mind:
Physical Products
Graphic Creating
Video clip Advertising
Online Marketing
Copywriting
Sound Editing
Consultation
Chatbots
Translation
Landing Page/Funnel Building
Digital Products
Video Creation
Content Writing
Voiceover
Idea Building
Web Growth
Video gaming
Stock Collection
Search Engine Optimization
Digital Marketing Providers
eBooks
CONTRAST WITH FIVERR/UPWORK (EXCLUSIVE NETWORKS)
1. When you drive website traffic to these open systems-- you will certainly never ever recognize if your target consumer purchases from some other vendor (due to much better testimonials/ far better price/ far better presentation or whatnot).
You shed the sale.
2. Even if they buy through you-- you never obtain the customer's call details.
Implying you are constantly relying on other systems for your business.
In short, you are building their company NOT your own.
"MarketPresso" tosses these 2 significant problems out of the park.
Giving you the total "Control" over your company.
It provides you an even better degree of personalized customizations-- so you can develop your individualized market according to your very own requirements.
And another HUGE benefit is
When your client remains in acquiring state of mind, they purchase even more products/services than initially planned (similar to it takes place while going shopping on Amazon).
Since this is your very own market, so all the solutions listed there will be your very own. So whether item "A" obtains marketed or "B", revenue enters your pockets.
This can never ever occur with public platforms.
Final thought
"It's A Good deal. Should I Invest Today?"
Not only are you getting accessibility to MarketPresso for the very best price ever before provided, but likewise You're spending entirely without risk. MarketPresso includes a 30-day Cash Back Assurance Policy. When you pick MarketPresso, your satisfaction is assured. If you are not completely pleased with it for any factor within the very first 1 month, you're entitled to a full refund-- no doubt asked. You've got nothing to shed! What Are You Awaiting? Try It today and obtain The Adhering to Perk Currently!
0 notes
Text
Designing And Building A Progressive Web Application Without A Framework (Part 1)
Designing And Building A Progressive Web Application Without A Framework (Part 1)
Ben Frain
2019-07-23T14:00:59+02:002019-07-23T12:07:36+00:00
How does a web application actually work? I don’t mean from the end-user point of view. I mean in the technical sense. How does a web application actually run? What kicks things off? Without any boilerplate code, what’s the right way to structure an application? Particularly a client-side application where all the logic runs on the end-users device. How does data get managed and manipulated? How do you make the interface react to changes in the data?
These are the kind of questions that are simple to side-step or ignore entirely with a framework. Developers reach for something like React, Vue, Ember or Angular, follow the documentation to get up and running and away they go. Those problems are handled by the framework’s box of tricks.
That may be exactly how you want things. Arguably, it’s the smart thing to do if you want to build something to a professional standard. However, with the magic abstracted away, you never get to learn how the tricks are actually performed.
Don’t you want to know how the tricks are done?
I did. So, I decided to try building a basic client-side application, sans-framework, to understand these problems for myself.
But, I’m getting a little ahead of myself; a little background first.
Before starting this journey I considered myself highly proficient at HTML and CSS but not JavaScript. As I felt I’d solved the biggest questions I had of CSS to my satisfaction, the next challenge I set myself was understanding a programming language.
The fact was, I was relatively beginner-level with JavaScript. And, aside from hacking the PHP of Wordpress around, I had no exposure or training in any other programming language either.
Let me qualify that ‘beginner-level’ assertion. Sure, I could get interactivity working on a page. Toggle classes, create DOM nodes, append and move them around, etc. But when it came to organizing the code for anything beyond that I was pretty clueless. I wasn’t confident building anything approaching an application. I had no idea how to define a set of data in JavaScipt, let alone manipulate it with functions.
I had no understanding of JavaScript ‘design patterns’ — established approaches for solving oft-encountered code problems. I certainly didn’t have a feel for how to approach fundamental application-design decisions.
Have you ever played ‘Top Trumps’? Well, in the web developer edition, my card would look something like this (marks out of 100):
CSS: 95
Copy and paste: 90
Hairline: 4
HTML: 90
JavaSript: 13
In addition to wanting to challenge myself on a technical level, I was also lacking in design chops.
With almost exclusively coding other peoples designs for the past decade, my visual design skills hadn’t had any real challenges since the late noughties. Reflecting on that fact and my puny JavaScript skills, cultivated a growing sense of professional inadequacy. It was time to address my shortcomings.
A personal challenge took form in my mind: to design and build a client-side JavaScript web application.
On Learning
There has never been more great resources to learn computing languages. Particularly JavaScript. However, it took me a while to find resources that explained things in a way that clicked. For me, Kyle Simpson’s ‘You Don’t Know JS’ and ‘Eloquent JavaScript’ by Marijn Haverbeke were a big help.
If you are beginning learning JavaScript, you will surely need to find your own gurus; people whose method of explaining works for you.
The first key thing I learned was that it’s pointless trying to learn from a teacher/resource that doesn’t explain things in a way you understand. Some people look at function examples with foo and bar in and instantly grok the meaning. I’m not one of those people. If you aren’t either, don’t assume programming languages aren’t for you. Just try a different resource and keep trying to apply the skills you are learning.
It’s also not a given that you will enjoy any kind of eureka moment where everything suddenly ‘clicks’; like the coding equivalent of love at first sight. It’s more likely it will take a lot of perseverance and considerable application of your learnings to feel confident.
As soon as you feel even a little competent, trying to apply your learning will teach you even more.
Here are some resources I found helpful along the way:
Fun Fun Function YouTube Channel
Kyle Simpson Plural Sight courses
Wes Bos’s JavaScript30.com
Eloquent JavaScript by Marijn Haverbeke
Right, that’s pretty much all you need to know about why I arrived at this point. The elephant now in the room is, why not use a framework?
Why Not React, Ember, Angular, Vue Et Al
Whilst the answer was alluded to at the beginning, I think the subject of why a framework wasn’t used needs expanding upon.
There are an abundance of high quality, well supported, JavaScript frameworks. Each specifically designed for the building of client-side web applications. Exactly the sort of thing I was looking to build. I forgive you for wondering the obvious: like, err, why not use one?
Here’s my stance on that. When you learn to use an abstraction, that’s primarily what you are learning — the abstraction. I wanted to learn the thing, not the abstraction of the thing.
I remember learning some jQuery back in the day. Whilst the lovely API let me make DOM manipulations easier than ever before I became powerless without it. I couldn’t even toggle classes on an element without needing jQuery. Task me with some basic interactivity on a page without jQuery to lean on and I stumbled about in my editor like a shorn Samson.
More recently, as I attempted to improve my understanding of JavaScript, I’d tried to wrap my head around Vue and React a little. But ultimately, I was never sure where standard JavaScript ended and React or Vue began. My opinion is that these abstractions are far more worthwhile when you understand what they are doing for you.
Therefore, if I was going to learn something I wanted to understand the core parts of the language. That way, I had some transferable skills. I wanted to retain something when the current flavor of the month framework had been cast aside for the next ‘hot new thing’.
Okay. Now, we’re caught up on why this app was getting made, and also, like it or not, how it would be made.
Let’s move on to what this thing was going to be.
An Application Idea
I needed an app idea. Nothing too ambitious; I didn’t have any delusions of creating a business start-up or appearing on Dragon’s Den — learning JavaScript and application basics was my primary goal.
The application needed to be something I had a fighting chance of pulling off technically and making a half-decent design job off to boot.
Tangent time.
Away from work, I organize and play indoor football whenever I can. As the organizer, it’s a pain to mentally note who has sent me a message to say they are playing and who hasn’t. 10 people are needed for a game typically, 8 at a push. There’s a roster of about 20 people who may or may not be able to play each game.
The app idea I settled on was something that enabled picking players from a roster, giving me a count of how many players had confirmed they could play.
As I thought about it more I felt I could broaden the scope a little more so that it could be used to organize any simple team-based activity.
Admittedly, I’d hardly dreamt up Google Earth. It did, however, have all the essential challenges: design, data management, interactivity, data storage, code organization.
Design-wise, I wouldn’t concern myself with anything other than a version that could run and work well on a phone viewport. I’d limit the design challenges to solving the problems on small screens only.
The core idea certainly leaned itself to ‘to-do’ style applications, of which there were heaps of existing examples to look at for inspiration whilst also having just enough difference to provide some unique design and coding challenges.
Intended Features
An initial bullet-point list of features I intended to design and code looked like this:
An input box to add people to the roster;
The ability to set each person to ‘in’ or ‘out’;
A tool that splits the people into teams, defaulting to 2 teams;
The ability to delete a person from the roster;
Some interface for ‘tools’. Besides splitting, available tools should include the ability to download the entered data as a file, upload previously saved data and delete-all players in one go;
The app should show a current count of how many people are ‘In’;
If there are no people selected for a game, it should hide the team splitter;
Pay mode. A toggle in settings that allows ‘in’ users to have an additional toggle to show whether they have paid or not.
At the outset, this is what I considered the features for a minimum viable product.
Design
Designs started on scraps of paper. It was illuminating (read: crushing) to find out just how many ideas which were incredible in my head turned out to be ludicrous when subjected to even the meagre scrutiny afforded by a pencil drawing.
Many ideas were therefore quickly ruled out, but the flip side was that by sketching some ideas out, it invariably led to other ideas I would never have otherwise considered.
Now, designers reading this will likely be like, “Duh, of course” but this was a real revelation to me. Developers are used to seeing later stage designs, rarely seeing all the abandoned steps along the way prior to that point.
Once happy with something as a pencil drawing, I’d try and re-create it in the design package, Sketch. Just as ideas fell away at the paper and pencil stage, an equal number failed to make it through the next fidelity stage of Sketch. The ones that seemed to hold up as artboards in Sketch were then chosen as the candidates to code out.
I’d find in turn that when those candidates were built-in code, a percentage also failed to work for varying reasons. Each fidelity step exposed new challenges for the design to either pass or fail. And a failure would lead me literally and figuratively back to the drawing board.
As such, ultimately, the design I ended up with is quite a bit different than the one I originally had in Sketch. Here are the first Sketch mockups:
Initial design of Who’s In application (Large preview)
Initial menu for Who’s In application (Large preview)
Even then, I was under no delusions; it was a basic design. However, at this point I had something I was relatively confident could work and I was chomping at the bit to try and build it.
Technical Requirements
With some initial feature requirements and a basic visual direction, it was time to consider what should be achieved with the code.
Although received wisdom dictates that the way to make applications for iOS or Android devices is with native code, we have already established that my intention was to build the application with JavaScript.
I was also keen to ensure that the application ticked all the boxes necessary to qualify as a Progressive Web Application, or PWA as they are more commonly known.
On the off chance you are unaware what a Progressive Web Application is, here is the ‘elevator pitch’. Conceptually, just imagine a standard web application but one that meets some particular criteria. The adherence to this set of particular requirements means that a supporting device (think mobile phone) grants the web app special privileges, making the web application greater than the sum of its parts.
On Android, in particular, it can be near impossible to distinguish a PWA, built with just HTML, CSS and JavaScript, from an application built with native code.
Here is the Google checklist of requirements for an application to be considered a Progressive Web Application:
Site is served over HTTPS;
Pages are responsive on tablets & mobile devices;
All app URLs load while offline;
Metadata provided for Add to Home screen;
First load fast even on 3G;
Site works cross-browser;
Page transitions don’t feel like they block on the network;
Each page has a URL.
Now in addition, if you really want to be the teacher’s pet and have your application considered as an ‘Exemplary Progressive Web App’, then it should also meet the following requirements:
Site’s content is indexed by Google;
Schema.org metadata is provided where appropriate;
Social metadata is provided where appropriate;
Canonical URLs are provided when necessary;
Pages use the History API;
Content doesn’t jump as the page loads;
Pressing back from a detail page retains scroll position on the previous list page;
When tapped, inputs aren’t obscured by the on-screen keyboard;
Content is easily shareable from standalone or full-screen mode;
Site is responsive across phone, tablet and desktop screen sizes;
Any app install prompts are not used excessively;
The Add to Home Screen prompt is intercepted;
First load very fast even on 3G;
Site uses cache-first networking;
Site appropriately informs the user when they’re offline;
Provide context to the user about how notifications will be used;
UI encouraging users to turn on Push Notifications must not be overly aggressive;
Site dims the screen when the permission request is showing;
Push notifications must be timely, precise and relevant;
Provides controls to enable and disable notifications;
User is logged in across devices via Credential Management API;
User can pay easily via native UI from Payment Request API.
Crikey! I don’t know about you but that second bunch of stuff seems like a whole lot of work for a basic application! As it happens there are plenty of items there that aren’t relevant to what I had planned anyway. Despite that, I'm not ashamed to say I lowered my sights to only pass the initial tests.
For a whole section of application types, I believe a PWA is a more applicable solution than a native application. Where games and SaaS arguably make more sense in an app store, smaller utilities can live quite happily and more successfully on the web as Progressive Web Applications.
Whilst on the subject of me shirking hard work, another choice made early on was to try and store all data for the application on the users own device. That way it wouldn’t be necessary to hook up with data services and servers and deal with log-ins and authentications. For where my skills were at, figuring out authentication and storing user data seemed like it would almost certainly be biting off more than I could chew and overkill for the remit of the application!
Technology Choices
With a fairly clear idea on what the goal was, attention turned to the tools that could be employed to build it.
I decided early on to use TypeScript, which is described on its website as “… a typed superset of JavaScript that compiles to plain JavaScript.” What I’d seen and read of the language I liked, especially the fact it learned itself so well to static analysis.
Static analysis simply means a program can look at your code before running it (e.g. when it is static) and highlight problems. It can’t necessarily point out logical issues but it can point to non-conforming code against a set of rules.
Anything that could point out my (sure to be many) errors as I went along had to be a good thing, right?
If you are unfamiliar with TypeScript consider the following code in vanilla JavaScript:
console.log(`${count} players`); let count = 0;
Run this code and you will get an error something like:
ReferenceError: Cannot access uninitialized variable.
For those with even a little JavaScript prowess, for this basic example, they don’t need a tool to tell them things won’t end well.
However, if you write that same code in TypeScript, this happens in the editor:

TypeScript in action (Large preview)
I’m getting some feedback on my idiocy before I even run the code! That’s the beauty of static analysis. This feedback was often like having a more experienced developer sat with me catching errors as I went.
TypeScript primarily, as the name implies, let’s you specify the ‘type’ expected for each thing in the code. This prevents you inadvertently ‘coercing’ one type to another. Or attempting to run a method on a piece of data that isn’t applicable — an array method on an object for example. This isn’t the sort of thing that necessarily results in an error when the code runs, but it can certainly introduce hard to track bugs. Thanks to TypeScript you get feedback in the editor before even attempting to run the code.
TypeScript was certainly not essential in this journey of discovery and I would never encourage anyone to jump on tools of this nature unless there was a clear benefit. Setting tools up and configuring tools in the first place can be a time sink so definitely consider their applicability before diving in.
There are other benefits afforded by TypeScript we will come to in the next article in this series but the static analysis capabilities were enough alone for me to want to adopt TypeScript.
There were knock-on considerations of the choices I was making. Opting to build the application as a Progressive Web Application meant I would need to understand Service Workers to some degree. Using TypeScript would mean introducing build tools of some sort. How would I manage those tools? Historically, I’d used NPM as a package manager but what about Yarn? Was it worth using Yarn instead? Being performance-focused would mean considering some minification or bundling tools; tools like webpack were becoming more and more popular and would need evaluating.
Summary
I’d recognized a need to embark on this quest. My JavaScript powers were weak and nothing girds the loins as much as attempting to put theory into practice. Deciding to build a web application with vanilla JavaScript was to be my baptism of fire.
I’d spent some time researching and considering the options for making the application and decided that making the application a Progressive Web App made the most sense for my skill-set and the relative simplicity of the idea.
I’d need build tools, a package manager, and subsequently, a whole lot of patience.
Ultimately, at this point the fundamental question remained: was this something I could actually manage? Or would I be humbled by my own ineptitude?
I hope you join me in part two when you can read about build tools, JavaScript design patterns and how to make something more ‘app-like’.
(dm, yk, il)
0 notes
Link
Reading Time: 10 minutes

Have you ever wanted to learn Sass, but you didn’t find a good tutorial? Chances are that you are quite good with CSS. However, you feel like there is a better way of working with CSS. You want to take your skills and efficiency to another level. In that cases, learning how to use preprocessor like Sass is one way to achieve that goal. This will be the goal of this mini series, to help you learn Sass. Today, you will learn about topics like variables, mixins, extends, imports and more. Let’s start!
Table of Contents:
Why Sass?
The Beginner’s Guide to Learn Sass
Nesting, ampersands and variables
The power of ampersand
Variables and more maintainable code
Mixins and extends
Mixins vs Extends
Imports and partials
Closing thoughts on how to learn Sass
Why Sass?
What are the main reasons why you should learn Sass, or at leas consider doing it? CSS is great and, with time, it is getting even better. One of the biggest changes in the recent time was that CSS finally “learned” to use variables. This is a big step forward. And, I think that there is even more to come. Yes, the future of CSS seems to be quite good. Still, I think CSS is far from being perfect. The biggest disadvantages of CSS are probably lack of any way to re-use style rules.
Sure, you can create special class with set of styles and then use it repeatedly. However, even this approach is quite limited. You can’t change the styles on the fly unless you add additional CSS to override the original. Also, you can’t use any conditions or parameters to switch between “versions” of the styles. Again, the only way is adding more CSS code. Also, you need to either put on the right place in the cascade. Or, you have to increase CSS specificity.
Another common problem with CSS was that there was no way to specify variables. If you wanted to re-use specific value across the project, you had to do it in the old fashioned way. Sure, there is nothing bad with it. However, imagine you would decide to change that value. Now, you would have to find every instance. This can be quite a feat in large code base. Luckily, the majority of text editors offers search & replace functionality.
Another problem with CSS is math. When you use plain CSS, you can’t use even simple calculations, adding, subtracting, multiplication or division. This problem also disappears when you learn Sass. You can use calc(), but Sass is more advanced. And, lastly, CSS can be quite hard to maintain. Without preprocessor, you can’t use atomic Design (modular CSS), SMACSS or anything like that. You have all CSS code in a single file. And, no, @import is not really a fix for that.
The Beginner’s Guide to Learn Sass
If you want to learn Sass, you have to install it first. You can install Sass in a coupe way. In case of Mac users, this is quite fast. You only need to install Sass gem via terminal. In case of Linux, you will need to install Ruby first and then Sass gem. You can install Ruby via package manager. For computers with Windows you need to install Ruby first as well. I suggest downloading Ruby installer as this is the easiest way to do it. After installing ruby, install Sass gem.
In cmd:
gem install sass
In terminal:
sude gem install sass
You can check if you have Sass gem installed on your computer with a simple command below. It should return specific version of the Sass gem. For example, Sass 3.4.23 (Selective Steve). If you see something similar to this, congrats, you are ready not only to learn Sass, but also to start using it. However, if you want to try Sass without installing anything, you can use online Sass playground called Sassmeister. This website will allow you to use and test Sass immediately.
In cmd/terminal:
sass -v
Nesting, ampersands and variables
Let’s start this journey to learn Sass with a couple of things that are easier. These are nesting, using ampersand and variables. I guess that you already know nesting from working with HTML or JavaScript. You can nest elements inside other elements or functions inside other functions. In CSS, there is no such a thing as nesting. Sass brings this feature to CSS. When you learn Sass, you can nest CSS selectors just like you do in HTML.
Sass:
// Example of nesting nav { ul { margin: 0; display: flex; list-style-type: none; } li { padding: 16px; } a { color: #212121; text-decoration: none; } }
CSS:
nav ul { margin: 0; display: flex; list-style-type: none; } nav li { padding: 16px; } nav a { color: #212121; text-decoration: none; }
Side note: Those two slashes (//) are used to mark comments in Sass. In Sass, you can use both. The difference between Sass comment (//) and CSS comment (/**/) is that Sass comments are not compiled into CSS. So, every comment you make using Sass syntax will stay in your Sass files. Any comment you make using CSS syntax will be compiled into CSS. In other words, you will find CSS comments in final CSS file, not Sass comments.
I should warn you that it is easy to go over the board with nesting. When you fall in love with it, you can create code that will result in over-qualified CSS. And, this can make work with CSS even bigger pain that before you learn Sass and started to using it. For this reason, I suggest nesting CSS selector to three levels at max, no more.
Sass:
.level-one { .level-two { .level-three { color: red; } } }
CSS:
.level-one .level-two .level-three { color: red; }
The power of ampersand
One very useful feature you will probably use very often, after you learn Sass and nesting, is ampersand. Ampersand allows you to reference parent selector. You simply replace the parent selector with this character. You can use this for with pseudo-classes such as :before, :after, :hover, :active, :focus, etc. You can also use ampersand for adjoining, or adding, CSS classes to create selector with higher specificity.
Sass:
ul { &.nav-list { display: flex; } & li { padding: 8px 16px; } } a { position: relative; &:before { position: absolute; bottom: 0; left: 0; content: ""; border-bottom: 2px solid #3498db; } &:focus, &:hover { color: #3498db; &:before { width: 100%; } } &:active { color: #2980b9; } }
CSS:
ul.nav-list { display: flex; } ul li { padding: 8px 16px; } a { position: relative; } a:before { position: absolute; bottom: 0; left: 0; content: ""; border-bottom: 2px solid #3498db; } a:focus, a:hover { color: #3498db; } a:focus:before, a:hover:before { width: 100%; } a:active { color: #2980b9; }
I have to mention that ampersand also allows you to use CSS combinators, such as the child combinator (>), adjacent sibling combinator (+) and the general sibling combinator (~).
Sass:
section { & + & { margin-top: 16px; } & > h1 { font-size: 32px; } & ~ p { font-size: 16px; } } // You can also omit the ampersand before the combinator and the result will be the same. section { + & { margin-top: 16px; } > h1 { font-size: 32px; } ~ p { font-size: 16px; } }
CSS:
section + section { margin-top: 16px; } section > h1 { font-size: 32px; } section ~ p { font-size: 16px; }
Finally, you don’t have to put the ampersand as first. You can use it in the end to change the selector entirely.
Sass:
section { body main & { background: #fff; } }
CSS:
body main section { background: #fff; }
Variables and more maintainable code
The last of the three I mentioned are variables. I have to say that variables were one of the main reasons why I wanted to learn Sass. Actually, I think that they were the number one reason. Work with CSS gets so much easier when you can make changes on a global scale by changing a single line of code. You no longer have to search for all occurrences of this or that value. You store the value inside a variable. Then, you have to change only that variable. Sass will do the rest.
As you can guess, this can immensely simplify and speedup your work. Also, it makes your styles much easier to maintain. Variables work with numbers, strings, colors, null, lists and maps. The only thing you must remember is to use “$” symbol every time you want to define a variable. Then, when you want to use that variable, well, you know what to do.
Sass:
// Declare variables $color-primary: #9b59b6; $color-secondary: #2c3e50; // Use variables a { color: $color-secondary; &:hover { color: $color-primary; } }
CSS:
a { color: #2c3e50; } a:hover { color: #9b59b6; }
One thing to keep in mind on your journey to learn Sass and use variables is scope. Variables in Sass works like variables in any programming language. Variables declared in global scope are accessible globally, local not.
Sass:
a { // locally defined variables $color-primary: #9b59b6; $color-secondary: #2c3e50; color: $color-secondary; &:hover { color: $color-primary; } } p { color: $color-secondary;// Undefined variable: "$color-secondary". }
This also means that you can use the same name for different variables without the worry of changing some. Just make sure to declare your variables in the right scope.
Sass:
$color-primary: #9b59b6; $color-secondary: #2c3e50; a { // local variables $color-primary: #fff; $color-secondary: #212121; color: $color-secondary; } p { color: $color-secondary; }
CSS:
a { color: #212121; } p { color: #2c3e50; }
Mixins and extends
Let’s take this journey to learn Sass on another level. When you are ready for more advanced Sass, a good place to start are mixins and extends. Do you remember when we were talking about the biggest disadvantages of CSS, namely re-usability of the code? This is where mixins and extends enters the game. Both these Sass features allow you to create re-usable chunks of code. It is safe to say that mixins and extends are similar to functions you know from JavaScript.
You create new mixin using “@mixin” directive and some name and put some styles inside it (inside curly brackets). Then, when you want to use that mixin you reference to it using “@include” followed by the name of the mixin. Extends work in a similar way. The difference is that extends don’t require creating some “extend”. Instead, you use CSS classes you already created. Then, when you want to use extend you use “@extend”, not “@mixin”.
Sass:
// Example of mixin @mixin transition($prop, $duration, $timing) { transition: $prop $duration $timing; } // Use mixin a { @include transition(all, .25s, cubic-bezier(.4,0,1,1)); }
CSS:
a { transition: all 0.25s cubic-bezier(0.4, 0, 1, 1); }
Sass:
// Random CSS class .btn { padding: 6px 12px; margin-bottom: 0; display: inline-block; font-size: 16px; } Examples of extend .btn--success { @extend .btn; background-color: #2ecc71; } .btn--alert { @extend .btn; background-color: #e74c3c; }
CSS:
.btn, .btn--success, .btn--alert { padding: 6px 12px; margin-bottom: 0; display: inline-block; font-size: 16px; } .btn--success { background-color: #2ecc71; } .btn--alert { background-color: #e74c3c; }
Mixins vs Extends
The advantage of mixins is that you can use parameters to make mixins more flexible. For example, you can add a simple condition and if statement to switch between two or more sets of rules. Every parameter starts with “$” symbol. Then, use that name inside the mixin. And, you can also set a default value to make the parameter optional. Setting a default value is like assigning value to variable. Use “$” symbol followed by colons and default value. Extends don’t have this ability.
Sass:
// Example of mixin with optional parameters @mixin transition($prop: all, $duration: .25s, $timing: cubic-bezier(.4,0,1,1)) { transition: $prop $duration $timing; } a { @include transition(); }
CSS:
a { transition: all 0.25s cubic-bezier(0.4, 0, 1, 1); }
When you want to change only some parameters and use default values for the rest, you can use the name of parameter when you use that mixin.
Sass:
@mixin transition($prop: all, $duration: .25s, $timing: cubic-bezier(.4,0,1,1)) { transition: $prop $duration $timing; } // Use different value only for parameter for duration a { @include transition($duration: .55s); }
CSS:
a { transition: all 0.55s cubic-bezier(0.4, 0, 1, 1); }
Another very useful feature of mixins is to use it along with “@content” directive. This way, you can provide the mixin with block of content. A good way to use this is for creating media queries.
Sass:
// Mixin for media query @mixin media($screen-width) { @media only screen and (max-width: $screen-width) { @content; } } // Use the media query mixin with @content directive .container { @include media(768px) { max-width: 690px; } } // Mixin for retina display media query @mixin retina { @media only screen and (-webkit-min-device-pixel-ratio: 2), only screen and (min--moz-device-pixel-ratio: 2), only screen and (-o-min-device-pixel-ratio: 2/1), only screen and (min-device-pixel-ratio: 2), only screen and (min-resolution: 192dpi), only screen and (min-resolution: 2dppx) { @content; } } .hero { background-image: url(/images/image.png); @include retina { background-image: url(/images/[email protected]); } }
CSS:
@media only screen and (max-width: 768px) { .container { max-width: 690px; } } .hero { background-image: url(/images/image.png); } @media only screen and (-webkit-min-device-pixel-ratio: 2), only screen and (min--moz-device-pixel-ratio: 2), only screen and (-o-min-device-pixel-ratio: 2 / 1), only screen and (min-device-pixel-ratio: 2), only screen and (min-resolution: 192dpi), only screen and (min-resolution: 2dppx) { .hero { background-image: url(/images/[email protected]); } }
Imports and partials
The last thing we will touch upon to help you learn Sass is @import directive. Along with variables, this was another major reason for me to learn Sass. With Sass, you can split your stylesheet into unlimited number of files. Then, you use @import directive to import these chunks into single file. When you compile this file, Sass will automatically import content from all files and create one CSS stylesheet. Make sure to use correct names of files to import. Otherwise, Sass will throw an error.
Sass:
// This is _base.scss file html, body { padding: 0; margin: 0; } html { font-size: 100%; } body { font: 16px "Roboto", arial, sans-serif; color: #111; } // This is _typography.scss file h1, h2, h3 { margin-top: 0; font-weight: 200; } h1 { margin-bottom: 26px; font-size: 40px; line-height: 52px; } h2 { margin-bottom: 18px; font-size: 27px; line-height: 39px; } h3 { margin-bottom: 18px; font-size: 22px; line-height: 26px; } // This is main.scss // Note: you don’t have to use “_” in filenames when you import files /* Main stylesheet */ @import 'base'; @import 'typography';
CSS:
/* Main stylesheet */ html, body { padding: 0; margin: 0; } html { font-size: 100%; } body { font: 16px "Roboto", arial, sans-serif; color: #111; } h1, h2, h3 { margin-top: 0; font-weight: 200; } h1 { margin-bottom: 26px; font-size: 40px; line-height: 52px; } h2 { margin-bottom: 18px; font-size: 27px; line-height: 39px; } h3 { margin-bottom: 18px; font-size: 22px; line-height: 26px; }
Important thing to mention is that you can use imports whenever you want. You can import file A in the middle of the file B and then import file B into your main Sass stylesheet. The only thing you must remember is to use the right order of files.
Sass:
// File _base.scss html, body { padding: 0; margin: 0; } @import 'typography'; nav { list-style-type: none; } nav a { text-decoration: none; } // File main.scss /* Main stylesheet */ @import 'base';
CSS:
/* Main stylesheet */ html, body { padding: 0; margin: 0; } h1, h2, h3 { margin-top: 0; font-weight: 200; } nav { list-style-type: none; } nav a { text-decoration: none; }
Closing thoughts on how to learn Sass
This is all I have for you today on how to learn Sass. My intention for this article was to give you enough information to get started with Sass. Hopefully, this article makes this journey to learn Sass as easy as possible for you. Keep in mind that what we discussed today were only the basics. We barely scratched the surface. If you want to not only learn Sass, but master it, there is more we need to talk about. Don’t worry. We will cover these advanced topics in another article next week.
For now, take some time, go through the topics we discussed today and practice your new knowledge. Doing so will help you prepare for the more advanced techniques and features Sass can provide you with. So, stay tuned for the sequel. Until next time, have a great day!
Thank you very much for your time.
Want more?
If you liked this article, please subscribe or follow me on Twitter.
The post The Beginner’s Guide to Learn Sass – Mastering the Basics of Sass appeared first on Alex Devero Blog.
1 note
·
View note
Text
Best WordPress BuddyPress Themes | Templified
New Post has been published on https://templified.com/best-wordpress-buddypress-themes/
Best WordPress BuddyPress Themes
This WordPress theme collection is all about BuddyPress.
BuddyPress is the number one plug in for community building with WordPress. BuddyPress has been around for about a decade and it’s still going strong in part because of all the amazing WordPress BuddyPress themes that you can find. There are hundreds and hundreds of BuddyPress themes out there but finding the right one can be a challenge which is why we’ve created this collection. We found the cream of the crop of BuddyPress themes and that’s all you’re going to see in this collection.
These Buddypress themes can help you build a community based on a common interest, your local city, a sports team or anything else that brings people together. Whether your site is large or small, an intranet or extranet site for your business or something else entirely, these Buddypress themes can really help your website succeed. These themes also work with bbPress so you can set up an online forum to connect with your readers. We think these BuddyPress themes are the absolute best but if you see something out there that we haven’t included please let us know in the comments below.
BuddyApp
When you’re looking for a fantastic BuddyPress theme, responsive design is a key factor. Considering the rise of handheld devices, allowing people to access your site on smart phones or tablets is critical. No matter what sort of private community you’re constructing, BuddyApp can make it look amazing. For intranet or extranet sites, social media style website, online forums and more, that mobile friendly environment can mean the difference between success and failure. BuddyApp is a very popular BuddyPress template, probably one of the most successful on ThemeForest, with over 1800 sales and counting. This theme comes with an HTML landing page, it’s got all the features you could possibly want and it’s very flexible in terms of design. BuddyApp is certainly a theme worth considering.
Demo More Information Get Hosting
Enfold
The Enfold WordPress theme was constructed by Kriesi, among the hottest expert programmers in ThemeForest WP design market. Enfold was made to offer you an extremely malleable theme, presenting folks who didn’t have professional design abilities a platform to make a great looking website. I’d say it’s totally succeeded. It unites user friendly control panel options using a handsome and reachable appearance that’s excellent for many distinct kinds of sites. On top of that, it gives easy drag and drop placement of page components and attributes. Enfold is perfectly accommodated for BuddyPress to earn a trendy, informative social media for your own.
Regardless of what business you operate in, Enfold has an alternative for you. It comes standard with assorted pre-made pages ideal for corporate restaurants, business, creative portfolios, fundamental or complex blogs and much more. It offers exceptional”Coming Soon” webpages to construct attention before launching the primary website. Selecting among those demos is as simple as pushing a button. Individuals that wish to produce a much more particular look will love the simple drag and drop page editor. This allows for special placement of several different website features and content cubes that may best be utilized to discuss the info that you desire with your customers. The Enfold WordPress theme has extensive documentation and guides in addition to tutorial videos to aid with every part of site development.
Demo More Information Get Hosting
Boss 2.0
This theme is Boss 2.0, created by BuddyBoss, a theme developer that specializes in creating BuddyPress themes. Their credentials are amazing. This is their best selling theme and it’s full of quality code, plenty of options, a responsive design and it’s easy to use. This theme employs a modern, stylish design to give a great user experience. The Boss 2.0 theme delivers a ton of positive user experiences. With the Boss theme, you can add membership levels, put content behind paywalls, folks can connect with each other and more. I love the fact that BuddyBoss also offers custom development services. If you want to create a really customized look for your site and add even more features, this is a great option and it’s reasonably priced too.
There is simply no sort of social networking you can not start with Boss, any subject is fair game with Boss 2.0. Inspire your customers to make new connections, create memories and produce an whole community, all without the need to touch a line of code. The design of this Boss theme is quite flexible, it is possible to customize almost everything you see in the colors to designs to fonts, navigation, widgets, photographs and a whole lot more. The Admin panel is strong, easy to use and it’s also a type of ‘set it and forget it’ Admin panel, you should not ever need to reconfigure your website after the first setup. Wish to make an internet learning platform? Well, simply slap on the Social Learner Add-On and you are going to be in business quickly and efficiently.
Demo More Information Get Hosting
Divi
Considering Divi is among the most powerful and flexible themes anywhere, it’s no surprise that many people are using this premium theme to help build an online community by using BuddyPress. BuddyPress and Divi go hand in hand and with those tools, you have everything you need to build an amazing website. BuddyPress allows you to create a social network and that’s pretty cool by itself, but when you pair BuddyPress with a theme as flexible and multi-purpose as Divi, it raised the stakes even more. Whether you want to create a sort of Facebook clone or develop an environment where businesses and coworkers can collaborate on projects, you want to create a message board on any sort of topic, this combination is a powerful one.
Demo More Information Get Hosting
Weston
Weston is WordPress theme that bills itself as the ultimate out WordPress theme. I’d be shocked if this team isn’t one of the better buddypress ready themes out there. It’s powerful and succinct, stylish and has abundant features to make for a wonderful website. Weston comes with wpbakery page builder, that gives you the functionality and flexibility to design a site that fits your needs. It’s a painless experience to craft a webpage that is perfect for just what you want, thanks to you I’m outs of content blocks and very simple customization process. The result is a handsome and effective web template, no matter what your niche.
Demo More Information Get Hosting
Community Junction
Demo More Information Get Hosting
One Social
Demo More Information Get Hosting
Woffice
Woffice is a really unique theme, offering intranet capabilities to Buddypress, so you can manage projects, have your team members and employees create their own pages, membership directories, calendar of events, Slack notifications and more. But Woffice is also bbPress ready, so you have that functionality as well. BuddyPress and bbPress is an utterly zero cost combination, totally open source wordpress plugin that will allow you to design a social networking community utilizing WordPress, including impressive features such as group members, sending message, friendships and likes, activity streams, messaging, member profiles, activity streams and a whole bunch more. There are actually a whole lot of magnificent bbPress and BuddyPress themes to pick from, but this choice really effective. Though BuddyPress was made to work great on the vast majority of WordPress themes, it is really smart to select a theme that was specifically built with BuddPress in mind. That’s Woffice.
This Woffice theme is a fully responsive as well as trendy social network WordPress BuddyPress theme allowing you to produce your own social network web site in seconds. You can access many settings like share work, ideas, concepts and information, build specialized collections, follow other users, check out statistics and so much more. Works with bbPress, LearnDash, Gravity Forms, Visual COmposer rt Media and more. Pretty sweet assembly of tools.
Demo More Information Get Hosting
Live Support
Demo More Information Get Hosting
HTMagazine
Demo More Information Get Hosting
Jannah
Demo More Information Get Hosting
Kleo
Thinking of trying to set up an online community based around WordPress? Well, bloody press and a high-quality theme is really all you need to get started. Kleo is one such theme, this is the highest rated buddypress theme on theme of forest. They call this theme a success kit for your website and I absolutely agree. Not only is this theme the best-selling buddypress theme, it’s also among the highest rated. Allowing you to build a professional blog and or a stunning portfolio, business directory or eCommerce shop, this team can help you get started the right way. This theme is one of the most customizable buddypress teams available, there are over a dozen different demo styles to get you started fast, each one for a slightly different Niche. Each and every one provides a fantastic platform for community building, that’s what buddypress is all about. Kleo also has highly rated support, detailed documentation and the code is clean and fast loading. With plenty of cool short codes, you’ll have a fantastic buddypress website off the ground quickly, even if you are not an expert with woocommerce.
Demo More Information Get Hosting
Razor
The Razor WordPress theme was designed with social networking and community building in mind. Totally compatible with BuddyPress social media plugin, this theme delivers stunning style for professional and business websites. There is nothing flashy or over-the-top about Razor, just the straight deal on sleek style and capability.
Select one of the stylish skins packaged with the Razor forum theme with a simple click of the mouse. It delivers a front page with a highly aesthetic and professional look so every site visitor knows the quality they will find there. With a BuddyPress installation on board, people who enjoy your content can add some of their own. Social networking and sharing of information adds a new dimension of success to any WordPress website.
Razor is fully responsive so connected individuals can access your social community from a home computer, tablet, or smartphone and get the same attractive and user-friendly experience. Retina-ready graphics keep things looking crisp and modern. The theme comes complete with a collection of icons, white label control panel, and bbPress forum integration. If you wish to run a social network for any reason, the Razor WP theme provides everything you need and the look you want. The developers even created tutorial videos to help you achieve your goals.
Demo More Information Get Hosting
Fildisi
Fildisi is a WordPress BuddyPress theme that’s sweeping the nation. No matter what the subject at hand, people love to gather to make connections. It’s not magic, it’s human nature. With the Fildisi theme, you get a beautiful result every time out. This theme has multiple blog and portfolio layouts, so people can create content that fits with their style. There are multiple post formats, WooComerce support and that’s part of what makes this such a successful multipurpose WordPress theme. With gorgeous flat style and tons of features, video tutorials, powerful options and plugin support to rival any theme on the market, this Fildisi theme is well worth consideration.
DemoMore Information Get Hosting
VideoRev
For video magazines, news blogs and portfolios centered around video, VideoRev is a well liked theme. Things get even more interesting when you consider that this theme is also BuddyPress compatible. With BuddyPress, you can create an online community centered around videos. There’s so much video out there and tons more uploaded ever minute, the market is massive. If you want to grab a share of traffic, and money, a theme like VideoRev can make it happen. When you add the community building functionality, you’ll get people to start making connections. As that happens, they’ll develop a real affinity for your website and that means brand loyalty. Returning traffic is important and this theme, along with BuddyPress and bbPress, can really make the most of your time, effort and money.
DemoMore Information Get Hosting
NewsPaper
With over 50 amazing and stylish, modern and clean designs, this WordPress theme has all the features you need to make a high-quality buddypress compatible website. Perfectly integrated with Instagram and bbpress, not to mention woocommerce, this SEO friendly WordPress theme is great for community building and blogging. There are over 50 different unique demo Styles, each one can be imported with just a few clicks. Publishing content has never been simpler. With buddypress, you’ll be able to build a community center to round any topic of your choosing. There are tons of reasons to select this theme. There are countless different post an article Styles, a new generation of click to create page building, plenty of other features and compatibility that make it among the very best buddypress compatible themes around. If you’re not a coder, no problem, you’ll never need to touch a line of code to make a fantastic website with the layout, colors and fonts, not to mention features, that you want.
DemoMore Information Get Hosting
Gwangi
Gwangi is a relatively new BuddyPress ready theme, but it’s already proven to be quite popular. This template is primarily built as a dating or matchmaking community theme, allowing you to set up a site like Plenty of Fish or Zoosk, eHarmony or match.com. Whatever your preference is, this template has the flexibility and style to make it happen. So far, over 250 people have downloaded this template and they have given it a 4.93 average rating. That’s one of the highest for any dating website, or buddypress theme for that matter. This template also offers bbPress support, the feature set is large and continues to grow, customization is a snap and this template is a really refreshing one, it has no bloated page builder included. Everything is built with widgets and I think that that makes for a particularly fast loading and highly effective website. Here’s our complete collection of WordPress dating themes. What do you think?
Demo More Information Get Hosting
Soledad
Soledad is WordPress theme with a staggering 3,500 different homepage demo Styles. It’s one of the biggest and most popular WordPress themes, and it happens to be a BuddyPress compatible theme, which allows you to build yourself and online community. No matter what the subject matter, Soledad has a look that is perfect for you. There are plenty of different community styles, so if you’re building an online community for a charity or non-profit, a fashion blog, food or lifestyle blog or a community based on some other mutual interest, this theme allows you to construct a beautiful and responsive website where people can connect with one another. If you’d like to set up an online forum, simply install bbPress and you’ll be connecting with your readers in no time flat. Here’s our magazine theme collection.
DemoMore Information Get Hosting
0 notes
Link
Free Online Essay Checker Fundamentals Explained
The entire process takes just a couple of seconds. Hope you get your solution about ways to acquire grammarly premium account free. There’s a desktop version supplying you with even more functions in addition to also the ability to interpret articles into nearly every language.
The Pain of Free Online Essay Checker
A good example custom essay writing company outline together with an effortless instance of an official report is given in the paragraphs below. Possessing a comprehension of proper grammar rules also demonstrates that a kid is educated. The structure starts to flood.
You have to provide all test with total preparation. Which kind you select is a dilemma of taste rather than a particular prescription, however once you have plumped for or not it’s sure to maintain it through your business application. Any kind of research paper has a particular structure that’s predicated on couple names.
The Downside Risk of Free Online Essay Checker
Make sure that you have the very best format before you decide to make anything. After a few seconds, it is going to return the outcome and give suggestions about how to look at your paper. Since you may see, https://www.masterpapers.com/literature_review a paper editor can truly help save you a little time and trouble.
The Free Online Essay Checker Game
The system is straightforward, all you need to do is drag and drop a Word document or you may also opt to paste the content which you want to verify directly onto their site for instant suggestions. Thus, you should try our company for sure if you prefer to have the ideal editing on-line service for your essay. You need to be able to use the service in online and offline regime despite where you are.
You are going to be requested to log in through your institution’s internet portal and if you’re eligible, you will be redirected to a page where you are able to download the program. Moreover, you can think about checking the structure of your writing utilizing GrammarCheck site. You need to be able to use the service in online and offline regime despite where you are.
If you have a small company, or want to promote a new solution, Facebook is an excellent means to have the word out, develop a following, keep your customers connected, and better your https://www.hartwick.edu/academics/student-services/office-of-the-registrar/readmission/ small business. A large quantity of speech services and tools can be discovered on the internet that could possibly be tough in most to select the ideal one in their opinion. Everybody wants their content to be original.
Ok, I Think I Understand Free Online Essay Checker, Now Tell Me About Free Online Essay Checker!
In the event that you desire to figure out much more, then you enroll for Copyscape Premium, that reports a lot a whole lot more results in comparison to free Copyscape support. Luckily, there are a lot of free internet checkers on the internet with a variety of choices, and that means you always have the option to find one that best fits your requirements. It requires a complicated strategy, something not all on-line editing services can provide.
The procedure is extremely easy. Which kind you select is a dilemma of taste rather than a particular prescription, however once you have plumped for or not it’s sure to maintain it through your business application. Any kind of research paper has a particular structure that’s predicated on couple names.
What Everybody Dislikes About Free Online Essay Checker and Why
Whether it’s a guide, essay or blog, a comprehensive sentence check is going to be conducted for your text to be able to provide you the most dependable and accurate outcomes. When writing a review try to break this up into a couple of paragraphs. Nancy’s very first book report is certain to be dazzling.
The price has only started to interrupt from the top band. Is an expert book report website at which you may purchase book reports on any topics. If you’re a student creating a book report for a requirement for an English class, you can get book reviews online.
There are guides online that will allow you to tell the difference. Ask a dozen people and you’ll probably get a dozen answers. Sometimes there’s a wide argument supported by means of a set of supporting arguments.
Today, there are a fantastic number of alternatives and strategies to cheat an exam. Structurally, the executive summary is the very first chapter of your organization program. The organization is generous enough to provide intensive self-learning videos tutorials.
PaperRater is among the ideal Grammarly alternatives readily available online. You don’t need to worry for your essay, because the internet site has a distinctive policy that safeguards your text from stealing. You will not locate the adult content or themes that have come to be so common to other teen education” sites on the web.
A good example outline together with an effortless instance of an official report is given in the paragraphs below. Each paragraph really needs an appropriate structure and logically connected sentences. The structure starts to flood.
Why Almost Everything You’ve Learned About Free Online Essay Checker Is Wrong
Luckily there are lots of on-line grammar and punctuation tools that will be able to help you with improving your writing quality. If you ought to be searching for an online cookie cutter checker which might translate the writing to many distinctive languages, then then Reverso might be the ideal tool because of it. The tool will offer unmatched accuracy free of charge.
from Patriot Prepper Don't forget to visit the store and pick up some gear at The COR Outfitters. Are you ready for any situation? #SurvivalFirestarter #SurvivalBugOutBackpack #PrepperSurvivalPack #SHTFGear #SHTFBag
0 notes
Text
Burnley v Chelsea: Win tickets to the Premier League match – courtesy of Carling
MailOnline is working with Carling – the official beer and cider partner of the Premier League – to give
The two parties compete against each other at the John Smith & # 39; s Stadium on Sunday 28 October (1.30 pm kickoff)
To have a chance of winning, simply answer the following question:
) Joe Hart C) Nick Pope
E-mail your answer, together with your name and address to [email protected] to arrive at 13.00 on Thursday 25 October.
NOTE: you must validate your entry with the words BURNLEY TICKETS in your course; the tickets are only for home fans; the tickets do not include travel to / from the ground, accommodation or hospitality; the decision of the editors is final. During the Premier League season Carling gives tickets to groups of friends, as well as a large number of other footy-related prizes.
]
The new campaign & # 39; For the fans & # 39; van Carling means that match-day squads arrive at locations across the country to improve the pub experience. the Carling Goal of the Month Award for the first time and the They Score You Score game return to the Carling Tap app after another successful season.
1. This contest (the "Promotion") is only open to residents of the UK (with the exception of Northern Ireland), with the exception of employees and agents of (a) the organizer or (b) a company associated with the production or distribution of this promotion, as well as their relatives or members of their family or family.
2. Participants must be 18 years or older at the time of participation. The proof of suitability must be provided on request. By participating in the Promotion, you will be deemed to accept these terms and conditions and be bound by them.
3. The promotion is free to enter and participants can participate by answering the Daily Mail question and submitting the answer to [email protected]. Only one submission per person or e-mail address is accepted.
4. The promotion starts at 10.00 am on 15/10/2018 and the closing time / date for the promotion is 1300 on 18/10/2018.
Winner
5. There will be one winner who will be selected by an independent judge in a random drawing from all eligible correct entries, held on 18-10-2018. The winner (s) will be notified by e-mail within one day of this date and will receive details on how they can accept their prize. If the winner (s) do not accept the prize in the manner indicated within one day after the organizer's email, a claim will be declared invalid and the organizer will then select another winner (again randomly selected in a draw) from all remaining will be contacted as above.
6. The prize is four (4) tickets for the game that takes place between Huddersfield Town and Liverpool on 10/20/2018, with kick-off at 17.30.
7. Gifts, prizes and other promotional items are non-transferable, may not be resold and are subject to availability. The Promoter reserves the right to replace such a gift, prize or item at its discretion with a gift, prize or item of equivalent value.
8. The name and country of the prize winner will be available after the closing date by submitting a written request to the organizer.
9. Participants agree to provide reasonable co-operation to allow the use of the winner's name and / or likeness for advertising and publicity purposes in connection with this Promotion, including but not limited to publication of the name of the winner and photo on the promoter's websites. By submitting an entry and taking into account the right of the organizer to participate in the promotion, participants agree to give the promoter a perpetual, royalty-free, non-exclusive, sublicensable right and license for use, reproduction, modification , modify, publish, translate, create derivative works from, distribute and exercise all copyrights and publicity rights in relation to all materials in the listing (including but not limited to text, images or video material) / or to include the Materials in other works. take in any medium that is now known or later developed for the entire duration of all rights that may exist in the Materials. By submitting Materials to the competition, a participant:
a. Guarantees that the Materials are their own original work and that they have the right to make them available for all the above-mentioned purposes; that it does not violate any law; that it is not obscene or slanderous; and that it does not violate the rights of a third party;
b. agrees to indemnify the organizer for all legal costs, damages and other costs that may have arisen as a result of a breach of the above warranty
c.
Personal Information
10.
10. Any personal information provided to us during the input process (including but not limited to your name, e-mail address, telephone number and date of birth) must to be correct. We accept no responsibility for incorrect personal information provided to us.
11. We keep your personal information in accordance with these terms and conditions and our privacy policy (available here: https://ift.tt/2T1s0Ys. html). Carling – Molson Coors Brewing Company (UK) Limited, a company with registered office in England and Wales (company number 00026018), with registered address at 137 High Street, Burton Upon Trent, Staffordshire, DE14 1JZ stores your personal data in accordance with its privacy policy (available here: https://ift.tt/2BYjMWu).
General
12. The Organizer's determination and decision on all matters is final and no promotional correspondence or discussion will take place. The Promoter reserves the right, at its own discretion: (a) to disqualify a claimant, competitor or candidate whose conduct is contrary to the spirit of the rules or the intention of the Promotion and to any nullity or all of their claims or declare statements on the basis of such behavior; (b) to declare claims or entries resulting from printing, production and / or distribution errors (including but not limited to errors on an organizer's website, any game cards and / or other printed materials) as invalid or if there are errors be (are) in an aspect of the preparation or the course of the promotion that have a material influence on the outcome of the promotion or the number of claimants or the value of claims; (c) to add to or waive rules on reasonable notice; and / or (d) to cancel the promotion or any part thereof at any stage in circumstances beyond the reasonable control of the Organizer.
13. No entries are accepted in bulk, from agents or third parties.
14. Insofar as permitted to the maximum extent by law (and subject to paragraph 15 below), the Promoter hereby closes all warranties, declarations, covenants and liabilities (explicit or implicit) with respect to this Promotion and / or the price.
15. Nothing in these terms and conditions excludes the liability of the organizer for: (i) death or personal injury resulting from his negligence; (ii) fraud or fraudulent misrepresentation; or (iii) any liability that is not legally limited. The Promoter reserves the right to change, suspend or cancel the Promotion in its sole discretion if the virus, bugs, tampering, fraud or other causes beyond the reasonable control of the Organizer, the administration, security or correct play of the Promote or hinder promotion.
17. If one of the provisions of these general terms and conditions is deemed to be invalid or unenforceable in whole or in part, it shall be separated from the remainder of the provisions and the validity of the other provisions and the remainder of the provisions. the provision in question will not be affected.
18. These terms and conditions are subject to the laws of England and any dispute is subject to the exclusive jurisdiction of the English courts.
Promoter
19. The promoter is Carling – Molson Coors Brewing Company (UK) Limited, a company incorporated in England and Wales (company number 00026018), whose registered address is at 137 High Street, Burton Upon Trent, Staffordshire, DE14 1JZ
]
] Sorry we are not accepting any comments on this article at this time.
Source link
0 notes