#accessing elements in tuple
Explore tagged Tumblr posts
Text
The Art of Using Tuples in Python
Python is a versatile and high-level programming language that is used for a wide range of applications. One of the most important data structures in Python is the tuple. In this article, we will discuss the concept of tuples in Python, how to create them, and various operations that can be performed on them. What are Tuples in Python? A tuple is an immutable, ordered collection of items, which…
View On WordPress
#accessing elements in tuple#how to use tuple in python#python programing#python tuple#python tutorial#tuple concatenation#tuple example#tuple in python#unpacking tuple
0 notes
Text
How to Access Tuple Elements in Python
Python is a versatile programming language that simplifies handling data through its built-in data structures, including tuples. Tuples are immutable sequences, meaning their contents cannot be altered once created. Despite this immutability, tuples offer efficient ways to access and manipulate data when needed. In this blog, we’ll explore how to access tuple elements in Python effectively and why mastering this concept is essential for every Python learner.
If you're just starting your programming journey, understanding how tuple elements in Python work is a fundamental step. With their immutability and ability to store multiple values in a single variable, tuples are a practical tool for organizing data. At our free e-learning tutorial portal, we break down such concepts with live examples to make programming accessible and engaging for students.
Accessing Tuple Elements by Index
In Python, you can access tuple elements by their index position. Indexing in Python starts at 0, so the first element of a tuple is accessed with index 0, the second element with 1, and so on. You can also use negative indexing to access elements from the end of the tuple, where -1 refers to the last element.
Here’s an example:
Define a tuple
my_tuple = ('apple', 'banana', 'cherry')
Access the first element
print(my_tuple[0]) # Output: apple
Access the last element
print(my_tuple[-1]) # Output: cherry This simple approach to accessing tuple elements in Python is intuitive and efficient, especially when you need specific values in a dataset.
Accessing Multiple Tuple Elements Using Slicing Slicing allows you to retrieve multiple elements from a tuple in a single operation. With slicing, you define a range of indices separated by a colon :.
Define a tuple
my_tuple = (10, 20, 30, 40, 50)
Access elements from index 1 to 3
print(my_tuple[1:4]) # Output: (20, 30, 40)
Access elements from the start to index 2
print(my_tuple[:3]) # Output: (10, 20, 30)
Access elements from index 2 to the end
print(my_tuple[2:]) # Output: (30, 40, 50) Slicing is a powerful technique to work with tuple elements in Python when you need a subset of the data for further processing.
Iterating Through Tuple Elements
Another common way to access tuple elements in Python is by iterating through them. Using a for loop, you can process each element in the tuple without worrying about its index.
Iterate over a tuple
my_tuple = ('Python', 'Java', 'C++')
for item in my_tuple: print(item) This approach is particularly useful when working with larger datasets or when you need to perform operations on every element of the tuple.
Why Learn Tuple Accessing?
Mastering how to access tuple elements in Python is a crucial skill for anyone learning programming. Tuples are widely used in real-world applications for storing immutable data, such as configurations, coordinates, or function returns. They help keep your data safe from accidental changes while ensuring quick retrieval when needed.
At our free online e-learning tutorial portal, we teach programming concepts like these in an easy-to-understand way, complete with live examples. Designed specifically for students and beginners, our platform simplifies programming with hands-on exercises and engaging explanations. Whether you’re new to programming or looking to refine your skills, our tutorials provide the perfect starting point.
Tuples are a powerful yet simple feature in Python, and learning to access tuple elements effectively can open up endless possibilities in your programming projects. Dive into our tutorials today to explore this concept and many more with clarity and confidence!
0 notes
Text
What is Data Structure in Python?
Summary: Explore what data structure in Python is, including built-in types like lists, tuples, dictionaries, and sets, as well as advanced structures such as queues and trees. Understanding these can optimize performance and data handling.
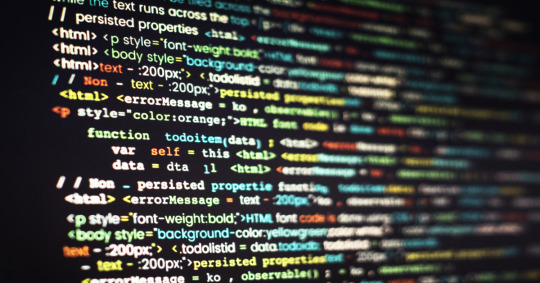
Introduction
Data structures are fundamental in programming, organizing and managing data efficiently for optimal performance. Understanding "What is data structure in Python" is crucial for developers to write effective and efficient code. Python, a versatile language, offers a range of built-in and advanced data structures that cater to various needs.
This blog aims to explore the different data structures available in Python, their uses, and how to choose the right one for your tasks. By delving into Python’s data structures, you'll enhance your ability to handle data and solve complex problems effectively.
What are Data Structures?
Data structures are organizational frameworks that enable programmers to store, manage, and retrieve data efficiently. They define the way data is arranged in memory and dictate the operations that can be performed on that data. In essence, data structures are the building blocks of programming that allow you to handle data systematically.
Importance and Role in Organizing Data
Data structures play a critical role in organizing and managing data. By selecting the appropriate data structure, you can optimize performance and efficiency in your applications. For example, using lists allows for dynamic sizing and easy element access, while dictionaries offer quick lookups with key-value pairs.
Data structures also influence the complexity of algorithms, affecting the speed and resource consumption of data processing tasks.
In programming, choosing the right data structure is crucial for solving problems effectively. It directly impacts the efficiency of algorithms, the speed of data retrieval, and the overall performance of your code. Understanding various data structures and their applications helps in writing optimized and scalable programs, making data handling more efficient and effective.
Read: Importance of Python Programming: Real-Time Applications.
Types of Data Structures in Python
Python offers a range of built-in data structures that provide powerful tools for managing and organizing data. These structures are integral to Python programming, each serving unique purposes and offering various functionalities.
Lists
Lists in Python are versatile, ordered collections that can hold items of any data type. Defined using square brackets [], lists support various operations. You can easily add items using the append() method, remove items with remove(), and extract slices with slicing syntax (e.g., list[1:3]). Lists are mutable, allowing changes to their contents after creation.
Tuples
Tuples are similar to lists but immutable. Defined using parentheses (), tuples cannot be altered once created. This immutability makes tuples ideal for storing fixed collections of items, such as coordinates or function arguments. Tuples are often used when data integrity is crucial, and their immutability helps in maintaining consistent data throughout a program.
Dictionaries
Dictionaries store data in key-value pairs, where each key is unique. Defined with curly braces {}, dictionaries provide quick access to values based on their keys. Common operations include retrieving values with the get() method and updating entries using the update() method. Dictionaries are ideal for scenarios requiring fast lookups and efficient data retrieval.
Sets
Sets are unordered collections of unique elements, defined using curly braces {} or the set() function. Sets automatically handle duplicate entries by removing them, which ensures that each element is unique. Key operations include union (combining sets) and intersection (finding common elements). Sets are particularly useful for membership testing and eliminating duplicates from collections.
Each of these data structures has distinct characteristics and use cases, enabling Python developers to select the most appropriate structure based on their needs.
Explore: Pattern Programming in Python: A Beginner’s Guide.
Advanced Data Structures
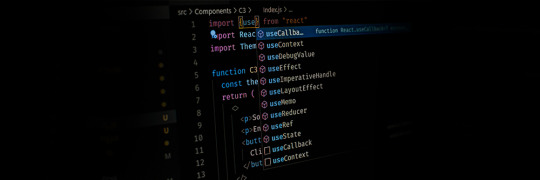
In advanced programming, choosing the right data structure can significantly impact the performance and efficiency of an application. This section explores some essential advanced data structures in Python, their definitions, use cases, and implementations.
Queues
A queue is a linear data structure that follows the First In, First Out (FIFO) principle. Elements are added at one end (the rear) and removed from the other end (the front).
This makes queues ideal for scenarios where you need to manage tasks in the order they arrive, such as task scheduling or handling requests in a server. In Python, you can implement a queue using collections.deque, which provides an efficient way to append and pop elements from both ends.
Stacks
Stacks operate on the Last In, First Out (LIFO) principle. This means the last element added is the first one to be removed. Stacks are useful for managing function calls, undo mechanisms in applications, and parsing expressions.
In Python, you can implement a stack using a list, with append() and pop() methods to handle elements. Alternatively, collections.deque can also be used for stack operations, offering efficient append and pop operations.
Linked Lists
A linked list is a data structure consisting of nodes, where each node contains a value and a reference (or link) to the next node in the sequence. Linked lists allow for efficient insertions and deletions compared to arrays.
A singly linked list has nodes with a single reference to the next node. Basic operations include traversing the list, inserting new nodes, and deleting existing ones. While Python does not have a built-in linked list implementation, you can create one using custom classes.
Trees
Trees are hierarchical data structures with a root node and child nodes forming a parent-child relationship. They are useful for representing hierarchical data, such as file systems or organizational structures.
Common types include binary trees, where each node has up to two children, and binary search trees, where nodes are arranged in a way that facilitates fast lookups, insertions, and deletions.
Graphs
Graphs consist of nodes (or vertices) connected by edges. They are used to represent relationships between entities, such as social networks or transportation systems. Graphs can be represented using an adjacency matrix or an adjacency list.
The adjacency matrix is a 2D array where each cell indicates the presence or absence of an edge, while the adjacency list maintains a list of edges for each node.
See: Types of Programming Paradigms in Python You Should Know.
Choosing the Right Data Structure
Selecting the appropriate data structure is crucial for optimizing performance and ensuring efficient data management. Each data structure has its strengths and is suited to different scenarios. Here’s how to make the right choice:
Factors to Consider
When choosing a data structure, consider performance, complexity, and specific use cases. Performance involves understanding time and space complexity, which impacts how quickly data can be accessed or modified. For example, lists and tuples offer quick access but differ in mutability.
Tuples are immutable and thus faster for read-only operations, while lists allow for dynamic changes.
Use Cases for Data Structures:
Lists are versatile and ideal for ordered collections of items where frequent updates are needed.
Tuples are perfect for fixed collections of items, providing an immutable structure for data that doesn’t change.
Dictionaries excel in scenarios requiring quick lookups and key-value pairs, making them ideal for managing and retrieving data efficiently.
Sets are used when you need to ensure uniqueness and perform operations like intersections and unions efficiently.
Queues and stacks are used for scenarios needing FIFO (First In, First Out) and LIFO (Last In, First Out) operations, respectively.
Choosing the right data structure based on these factors helps streamline operations and enhance program efficiency.
Check: R Programming vs. Python: A Comparison for Data Science.
Frequently Asked Questions
What is a data structure in Python?
A data structure in Python is an organizational framework that defines how data is stored, managed, and accessed. Python offers built-in structures like lists, tuples, dictionaries, and sets, each serving different purposes and optimizing performance for various tasks.
Why are data structures important in Python?
Data structures are crucial in Python as they impact how efficiently data is managed and accessed. Choosing the right structure, such as lists for dynamic data or dictionaries for fast lookups, directly affects the performance and efficiency of your code.
What are advanced data structures in Python?
Advanced data structures in Python include queues, stacks, linked lists, trees, and graphs. These structures handle complex data management tasks and improve performance for specific operations, such as managing tasks or representing hierarchical relationships.
Conclusion
Understanding "What is data structure in Python" is essential for effective programming. By mastering Python's data structures, from basic lists and dictionaries to advanced queues and trees, developers can optimize data management, enhance performance, and solve complex problems efficiently.
Selecting the appropriate data structure based on your needs will lead to more efficient and scalable code.
#What is Data Structure in Python?#Data Structure in Python#data structures#data structure in python#python#python frameworks#python programming#data science
5 notes
·
View notes
Text
Understanding Data Structures in Python
In the realm of programming, data structures are fundamental components that help in organizing and storing data efficiently. Python, being a versatile and high-level language, provides a rich set of built-in data structures that cater to various computational needs. Whether you are a beginner exploring Python or an experienced programmer, mastering data structures is crucial for optimizing performance and writing efficient code. If you are considering enrolling in a Python course, understanding these structures will undoubtedly enhance your learning experience.
What Are Data Structures?
Data structures refer to the ways in which data is organized, managed, and stored for efficient access and modification. They are essential in software development, playing a critical role in algorithms, databases, and system design. Python offers both built-in data structures and user-defined data structures, allowing developers to implement robust solutions with ease.
Built-in Data Structures in Python
1. Lists
Lists are one of the most commonly used data structures in Python. They are ordered, mutable, and can contain different data types. Lists allow for easy addition, removal, and modification of elements.
# Creating a list
my_list = [1, 2, 3, "Python", True]
# Accessing elements
print(my_list[0]) # Output: 1
# Modifying elements
my_list[1] = 20
# Adding elements
my_list.append(50)
# Removing elements
my_list.remove("Python")
2. Tuples
Tuples are similar to lists but are immutable, meaning their elements cannot be changed after creation. They are useful when you want to store data that should remain constant throughout the program.
# Creating a tuple
my_tuple = (10, 20, 30, "Python")
# Accessing elements
print(my_tuple[2]) # Output: 30
3. Sets
Sets are unordered collections of unique elements. They are useful when dealing with distinct values and performing operations like unions and intersections.
# Creating a set
my_set = {1, 2, 3, 4, 4, 5}
# Adding elements
my_set.add(6)
# Removing elements
my_set.discard(3)
4. Dictionaries
Dictionaries store data in key-value pairs, making them ideal for mapping relationships between elements.
# Creating a dictionary
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
# Accessing values
print(my_dict["name"]) # Output: Alice
# Modifying values
my_dict["age"] = 26
User-Defined Data Structures
While Python’s built-in data structures are powerful, there are times when you need custom structures to handle complex problems. Some of the common user-defined data structures include:
1. Stack
A stack is a Last-In-First-Out (LIFO) data structure, meaning the last element added is the first one to be removed.
class Stack:
def __init__(self):
self.stack = []
def push(self, item):
self.stack.append(item)
def pop(self):
if not self.is_empty():
return self.stack.pop()
def is_empty(self):
return len(self.stack) == 0
my_stack = Stack()
my_stack.push(10)
my_stack.push(20)
print(my_stack.pop()) # Output: 20
2. Queue
A queue follows a First-In-First-Out (FIFO) order, where the first element added is the first to be removed.
from collections import deque
class Queue:
def __init__(self):
self.queue = deque()
def enqueue(self, item):
self.queue.append(item)
def dequeue(self):
if not self.is_empty():
return self.queue.popleft()
def is_empty(self):
return len(self.queue) == 0
my_queue = Queue()
my_queue.enqueue(10)
my_queue.enqueue(20)
print(my_queue.dequeue()) # Output: 10
3. Linked List
A linked list is a sequential data structure where each element (node) contains data and a reference to the next node.
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def display(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
my_list = LinkedList()
my_list.insert(10)
my_list.insert(20)
my_list.display() # Output: 20 -> 10 -> None
Importance of Learning Data Structures
Understanding data structures is crucial for writing optimized and scalable code. Some benefits include:
Efficient Data Management – Organizing data properly enhances speed and efficiency.
Improved Algorithm Performance – The right data structure can significantly improve algorithm efficiency.
Better Problem Solving – Knowledge of data structures helps tackle complex problems effectively.
Essential for Technical Interviews – Many coding interviews focus on data structure problems.
Conclusion
Data structures form the backbone of programming and computational efficiency. Python offers a range of built-in and user-defined structures to handle diverse programming challenges. Whether it's lists, tuples, stacks, or linked lists, mastering data structures is essential for any Python programmer. By enrolling in a Python course, you can gain in-depth knowledge and practical experience to enhance your programming skills. Start your journey today and take a step closer to becoming a proficient Python developer.
0 notes
Text
Exploring Python's Core Data Structures: A Beginner’s Guide
Have you ever thought of data structures as something complicated and full of code? Well, not really! Let's break down the basic Python data structures with easy-to-understand examples and show how they can make your coding more efficient.
1. Lists: The Handy Shopping Cart
Think of a List as your shopping cart. You can put any item in it, remove things, and even change the order of items whenever you like. It's like an ever-changing basket of goods.
· Key Features:
Ordered (items stay in the order you add them)
Mutable (you can change, add, or remove elements)
· Practical Example: Want to keep track of groceries you're buying? A list is perfect for that.
· Time Complexity:
Adding an item at the end: O(1) (fast)
Removing or adding an item somewhere in the middle: O(n) (slower for large lists)
Use Case: Great for when you need to store items that change over time, like a to-do list, shopping cart, or a collection of names.
2. Tuples: The Fixed Book Collection
A Tuple is like your favorite book collection at home. Once you've selected the books, you don’t change them. It's an immutable collection—you can't add new books or take one out, but you can still read them anytime.
· Key Features:
Ordered (like a list, but can't be changed after creation)
Immutable (no changes after they're created)
· Practical Example: Perfect for holding things you don't want to change, like your birthdate or the fixed coordinates of a city (latitude, longitude).
· Time Complexity:
Accessing any element: O(1) (very fast)
No time complexity for modification (because you can’t modify them!)
Use Case: Use tuples when you need a fixed collection of data. Example: geographic coordinates, dates, and other fixed sets of information.
3. Sets: The Unique Collection of Friends
A Set is like your group of friends at a party. You don’t allow duplicates—each friend is unique! Sets automatically ignore any repeated names you try to add.
· Key Features:
Unordered (don’t care about the order of items)
Mutable (you can add or remove items, but no duplicates are allowed)
· Practical Example: If you want to keep track of unique visitors to your website, a set is perfect because it automatically removes duplicate entries.
· Time Complexity:
Adding and removing items: O(1) (very fast)
Checking if an item is in the set: O(1)
Use Case: Sets are ideal for situations where you only care about unique items, like when you're checking for unique words in a text, or distinct entries in a survey.
4. Dictionaries: The Address Book
A Dictionary is like an address book. You store someone's name (the key) and their phone number (the value). You can find a person's phone number very quickly by looking them up by name.
· Key Features:
Unordered (no specific order of keys and values)
Mutable (you can add, remove, or change values)
· Practical Example: Perfect for when you need to store data in pairs, like a contact list where each name is associated with a phone number.
· Time Complexity:
Accessing a value by key: O(1) (fast)
Inserting or removing a key-value pair: O(1) (fast)
Use Case: Dictionaries are great for storing mappings of one thing to another, like a contact list, employee ID _ name mapping, or any key-value pairing.
5. Strings: The Immutable Text
A String is like a sentence. Once written, you can read it and use it, but you can't change the individual letters directly. Instead, if you need to change something, you must create a new string.
· Key Features:
Ordered (the letters have a defined position)
Immutable (you can't change individual characters once created)
· Practical Example: Used for storing text, like a user’s name or a sentence from a book.
· Time Complexity:
Accessing a character by index: O(1)
Modifying a string (creates a new string): O(n) (since a new string must be created)
Use Case: Strings are used to store and manipulate textual data, like messages, names, or descriptions.
Python's core data structures (Lists, Tuples, Sets, Dictionaries, and Strings) are easy to work with, highly efficient, and help you solve real-world problems. Whether you need a flexible, changeable list of items or a fixed, immutable tuple, Python has got you covered.
What’s Next?
Stay tuned! Next, we’ll dive into Arrays—another powerful container type. We’ll also explore advanced data structures and lesser-known but powerful functions from Python’s collections module to make your code even more efficient.
Feel free to comment or share your favorite Python data structure! What’s your use case? 📝
0 notes
Text
Understanding Tuples in D Programming Language
Introduction to Tuples in D Programming Language Hello D fans! Today, I will introduce you to Understanding Tuples in D Programming Language – one of the most powerful and versatile concepts in the D programming language. Tuples are an ordered collection of elements that can be of different types, packed into a single unit. They allow grouping related values in an easily accessible manner, and…
0 notes
Text
Mastering Python: Top Interview Questions You Need to Know
Python continues to dominate the programming landscape due to its simplicity, versatility, and wide range of applications. Whether you’re a seasoned developer or an aspiring coder, understanding how to answer Python interview questions is essential for landing your next job. In this blog, we’ll walk you through the most commonly asked Python interview questions and provide tips on how to answer them effectively. Plus, don’t miss the recommended resource at the end that can help boost your interview prep!
1. What Are Python's Key Features?
Python is celebrated for its readability and simplicity. Key features include:
Easy Syntax: Python’s syntax is designed to be readable and intuitive.
Interpreted Language: No compilation step needed; Python code runs directly.
Dynamic Typing: No need to declare variable types.
Extensive Libraries: A robust standard library, plus numerous external libraries.
Community Support: One of the most active and large developer communities.
Tip: Be prepared to explain how these features impact the efficiency of your coding practices.
2. Explain the Difference Between Python 2 and Python 3
While Python 2 was once a popular version, Python 3 has become the standard. Major differences include:
Print Function: Python 2 uses print "Hello", while Python 3 requires print("Hello").
Integer Division: In Python 2, 3 / 2 results in 1. In Python 3, it yields 1.5.
Unicode Support: Python 3 uses Unicode by default, making string handling more versatile.
Tip: Emphasize Python 3's improvements in readability and performance.
3. How Do You Manage Memory in Python?
Memory management in Python is handled by the Python Memory Manager. Important points include:
Garbage Collection: Python has an automatic garbage collector to reclaim unused memory.
Dynamic Memory Allocation: Objects are stored in the private heap space managed by the memory manager.
Modules like gc: Use Python’s built-in gc module to control and interact with garbage collection.
Tip: Be ready to discuss how you can manage memory in large-scale applications effectively.
4. What Are Decorators in Python?
Decorators are a powerful feature that allows you to modify the behavior of a function or class. They are often used for:
Logging: Easily add logging functionality to functions.
Authorization: Control access to specific parts of a program.
Caching: Improve performance by storing results for future use.
Example:
python
Copy code
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
5. What Is the Difference Between args and kwargs?
*args and **kwargs are used to pass a variable number of arguments to a function.
*args: Allows passing a variable number of non-keyword arguments.
**kwargs: Allows passing a variable number of keyword arguments.
Example:
python
Copy code
def func(*args, **kwargs):
for arg in args:
print(arg)
for key, value in kwargs.items():
print(f"{key} = {value}")
func(1, 2, 3, a=4, b=5)
6. What Are Python’s Built-in Data Structures?
Python comes equipped with a set of built-in data structures:
Lists: Mutable sequences of elements.
Tuples: Immutable sequences, useful for fixed data.
Sets: Unordered collections of unique elements.
Dictionaries: Key-value pairs for mapping data.
Tip: Mention scenarios where each data structure would be the best fit.
7. Explain List Comprehension in Python
List comprehension is a concise way to create lists based on existing lists. It reduces code length and improves readability.
Example:
python
Copy code
squares = [x * x for x in range(10)]
Tip: Be ready to explain when using list comprehensions can improve code efficiency.
8. What Is the Purpose of Python's __init__ Method?
The __init__ method in Python is a special method used to initialize the state of an object in a class. It's similar to constructors in other object-oriented programming languages.
Example:
python
Copy code
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
p1 = Person("John", 30)
print(p1.name) # Output: John
9. How Can You Optimize Python Code Performance?
Code performance can be improved using:
Built-in Functions: Leveraging Python’s built-in functions which are optimized for performance.
Efficient Algorithms: Choose algorithms that fit the problem.
Profiling Tools: Use modules like cProfile to identify bottlenecks.
List Comprehensions: Replace loops with comprehensions for better readability and speed.
Tip: Discuss any experience with optimizing code in real-world projects.
10. What Are Python Generators?
Generators in Python are a way to produce a sequence of values lazily, which can be more memory-efficient than lists. They are created using the yield keyword.
Example:
python
Copy code
def my_generator():
yield 1
yield 2
yield 3
gen = my_generator()
print(next(gen)) # Output: 1
Tip: Highlight cases where using a generator would be more appropriate than a list.
Watch and Learn More!
Understanding these top Python interview questions can boost your confidence for technical interviews. To dive deeper into mastering Python concepts and tips for cracking interviews, check out this comprehensive Python interview prep video. This guide covers even more questions and detailed explanations to keep you ahead of the curve.
0 notes
Text
Can somebody provide step by step to learn Python for data science?
Step-by-Step Approach to Learning Python for Data Science
1. Install Python and all the Required Libraries
Download Python: You can download it from the official website, python.org, and make sure to select the correct version corresponding to your operating system.
Install Python: Installation instructions can be found on the website.
Libraries Installation: You have to download some main libraries to manage data science tasks with the help of a package manager like pip.
NumPy: This is the library related to numerical operations and arrays.
Pandas: It is used for data manipulation and analysis.
Matplotlib: You will use this for data visualization.
Seaborn: For statistical visualization.
Scikit-learn: For algorithms of machine learning.
2. Learn Basics of Python
Variables and Data Types: Be able to declare variables, and know how to deal with various data types, including integers, floats, strings, and booleans.
Operators: Both Arithmetic, comparison, logical, and assignment operators
Control Flow: Conditional statements, if-else, and loops, for and while.
Functions: A way to create reusable blocks of code.
3. Data Structures
Lists: The way of creating, accessing, modifying, and iterating over lists is needed.
Dictionaries: Key-value pairs; how to access, add and remove elements.
Sets: Collections of unique elements, unordered.
Tuples: Immutable sequences.
4. Manipulation of Data Using pandas
Reading and Writing of Data: Import data from various sources, such as CSV or Excel, into the programs and write it in various formats. This also includes treatment of missing values, duplicates, and outliers in data. Scrutiny of data with the help of functions such as describe, info, and head.
Data Transformation: Filter, group and aggregate data.
5. NumPy for Numerical Operations
Arrays: Generation of numerical arrays, their manipulation, and operations on these arrays are enabled.
Linear Algebra: matrix operations and linear algebra calculations.
Random Number Generation: generation of random numbers and distributions.
6. Data Visualisation with Matplotlib and Seaborn
Plotting: Generation of different plot types (line, bar, scatter, histograms, etc.)
Plot Customization: addition of title, labels, legends, changing plot styles
Statistical Visualizations: statistical analysis visualizations
7. Machine Learning with Scikit-learn
Supervised Learning: One is going to learn linear regression, logistic regression, decision trees, random forests, support vector machines, and other algorithms.
Unsupervised Learning: Study clustering (K-means, hierarchical clustering) and dimensionality reduction (PCA, t-SNE).
Model Evaluation: Model performance metrics: accuracy, precision, recall, and F1-score.
8. Practice and Build Projects
Kaggle: Join data science competitions for hands-on practice on what one has learnt.
Personal Projects: Each project would deal with topics of interest so that such concepts may be firmly grasped.
Online Courses: Structured learning is possible in platforms like Coursera, edX, and Lejhro Bootcamp.
9. Stay updated
Follow the latest trends and happenings in data science through various blogs and news.
Participate in online communities of other data scientists and learn through their experience.
You just need to follow these steps with continuous practice to learn Python for Data Science and have a great career at it.
0 notes
Text
Python learnings...Tuples vs Lists
Tuples and lists are both data structures in Python, but they have some key differences that make them useful in different situations. Here are some reasons why you might choose to use a tuple over a list:
Immutable: Tuples are immutable, meaning that once they are created, their elements cannot be changed. This can be useful when you want to ensure that the data in the tuple remains constant and cannot be accidentally modified.
Performance: Tuples are generally more efficient in terms of memory and performance compared to lists. Since tuples are immutable, Python can optimize their storage and access, resulting in faster execution times.
Dictionary keys: Tuples can be used as keys in dictionaries, while lists cannot. This is because tuples are immutable and can be hashed, making them suitable for use as dictionary keys.
Sequence unpacking: Tuples can be easily unpacked into multiple variables, allowing you to assign values to multiple variables in a single line of code. This can be convenient when working with functions that return multiple values.
Data integrity: Since tuples are immutable, they provide a level of data integrity. Once a tuple is created, you can be confident that its elements will not be modified, ensuring the integrity of your data.
It's important to note that lists are more commonly used in Python due to their flexibility and mutability. However, tuples can be a good choice when you need to store a collection of values that should not be modified.
0 notes
Text
Python tuple example

A Python tuple is an immutable sequence type, meaning that once it is created, it cannot be modified. Here's a basic example to illustrate how to create and use tuples in Python:
```python
# Creating a tuple
my_tuple = (1, 2, 3, "apple", "banana")
# Accessing elements in a tuple
first_element = my_tuple[0] # 1
second_element = my tuple[1] # 2
# Slicing a tuple
sub_tuple = my_tuple[1:3] # (2, 3)
# Tuples can be nested
nested_tuple = (1, 2, (3, 4, 5))
# Unpacking a tuple
a, b, c, d, e = my_tuple
# Looping through a tuple
for item in my_tuple:
print(item)
# Trying to modify a tuple (this will raise an error)
# my_tuple[0] = 10 # TypeError: 'tuple' object does not support item assignment
```
Here's what happens in each part of the example:
**Creating a tuple**: `my_tuple` is created with mixed data types.
**Accessing elements**: Individual elements are accessed using their index.
**Slicing a tuple**: A portion of the tuple is accessed using slicing.
**Nested tuples**: Tuples can contain other tuples as elements.
**Unpacking a tuple**: Elements of the tuple are assigned to individual variables.
**Looping through a tuple**: Each element in the tuple is printed using a for loop.
**Immutability**: Attempting to change an element of the tuple results in a `Type Error` because tuples are immutable.
TCCI Computer classes provide the best training in all computer courses online and offline through different learning methods/media located in Bopal Ahmedabad and ISCON Ambli Road in Ahmedabad.
For More Information:
Call us @ +91 98256 18292
Visit us @ http://tccicomputercoaching.com/
#BEST PYTHON PROGRAMMING COURSE#PYTHON CLASS IN BOPAL-Iscon Ambli Road-Ahmedabad#PYTHON FOR SCHOOL STUDENTS#BEST PYTHON COACHING INSTITUTE IN BOPAL-Iscon Ambli Road-AHMEDABAD#COMPUTER CLASS IN BOPAL-Iscon Ambli Road-Ahmedabad
0 notes
Text
Unleashing Python's Potential: A Comprehensive Exploration
Python, renowned as one of the most adaptable programming languages, presents a multitude of learning avenues, spanning from fundamental principles to avant-garde applications across various domains. Immerse yourself in the realm of Python and embark on an odyssey of enlightenment with our extensive Python Course in Hyderabad, meticulously crafted to unleash your full capabilities and kindle your career aspirations.
Navigating Python: A Pathway to Proficiency
Embark on a journey into Python's depths and unearth a trove of knowledge awaiting discovery. Here’s an intricate guide detailing the spectrum of insights Python offers:
Grasping Basic Programming Concepts
Syntax and Semantics: Acquaint yourself with Python's fundamental framework and syntax regulations governing code structure, encompassing essential elements like indentation, statement formation, and expression evaluation.
Data Types and Variables: Master the manipulation of diverse data types - integers, floats, strings, lists, tuples, dictionaries, and sets - refining your expertise in data management and manipulation.
Control Structures: Harness the potency of loops (for, while) and conditional statements (if, else, elif) to govern program flow, facilitating efficient execution and logical decision-making.
Functions: Immerse yourself in the intricacies of function definition and invocation, grasp the subtleties of parameters and return values, and explore concepts such as scope and recursion for heightened code organization and efficiency.
Error Handling: Equip yourself with adept tools to proficiently manage errors and exceptions, ensuring the resilience and robustness of your Python programs amidst unforeseen challenges.
Exploring Advanced Programming Concepts
Object-Oriented Programming (OOP): Unlock the prowess of OOP principles as you delve into class and object creation and manipulation, unraveling concepts like inheritance, polymorphism, encapsulation, and abstraction. Enroll in our Python Online Course to delve deeper into Object-Oriented Programming (OOP) concepts and broaden your horizons.
Modules and Packages: Harness Python's expansive library ecosystem by mastering the importation and utilization of standard libraries and third-party packages, seamlessly augmenting your application’s functionality.
File Handling: Navigate the complexities of file I/O operations, learning to read from and write to files while adeptly managing various file formats like text, CSV, and JSON for efficient data handling.
Decorators and Generators: Elevate your coding prowess with advanced features such as decorators and generators, empowering you to optimize and streamline your code for enhanced performance and functionality.
Concurrency: Embrace the realm of concurrent programming as you delve into threading and asynchronous programming techniques, mastering the art of handling parallel operations with finesse.
Unlocking Your Potential with Python
Python transcends boundaries, catering to an array of interests and career trajectories. Whether you’re a fledgling enthusiast embarking on your coding odyssey or a seasoned professional seeking to expand your skill set, Python serves as a gateway to success across diverse domains such as data science, web development, automation, software engineering, game development, networking, cybersecurity, and IoT.
Its innate simplicity and readability render it accessible to novices, while its robustness and versatility make it indispensable for seasoned professionals. Embrace the power of Python, unlock your potential, and embark on a journey towards unparalleled success in the dynamic realm of technology and innovation.
0 notes
Text
Top 50 Python Interview Questions
Top 50 Python Interview Questions: Mastering Python for Automation Testing
Python has become a go-to language for many software testing, especially in the realm of automation testing. With its simple syntax, readability, and vast community support, Python is an excellent choice for those looking to dive into automation testing using tools like Selenium WebDriver. In this article, we'll explore the top 50 Python interview questions, specifically tailored for those interested in automation testing.
Table of Contents
Sr#
Headings
1
Introduction to Python for Automation Testing
2
Python Basics
3
Data Structures
4
Control Flow
5
Functions and Modules
6
Object-Oriented Programming (OOP)
7
Exception Handling
8
File Handling
9
Regular Expressions
10
Python Libraries for Automation Testing
11
Selenium WebDriver Basics
12
Locators in Selenium WebDriver
13
Waits in Selenium WebDriver
14
Handling Dropdowns and Alerts in Selenium
15
Page Object Model (POM) in Selenium WebDriver
Introduction to Python for Automation Testing
Automation with Python is a versatile programming language that is widely used in the field of automation testing due to its simplicity and readability. It provides a rich set of libraries and frameworks that make automation testing efficient and effective. Python's integration with Selenium WebDriver, a popular tool for web application testing, makes it a preferred choice for many automation testers.
Python Basics
What is Python? - Python is a high-level, interpreted programming language known for its simplicity and readability. It is widely used in various applications, including web development, data science, and automation testing.
How is Python different from other programming languages? - Python emphasizes code readability and simplicity, making it easier to write and understand compared to other languages like Java or C++.
What are the key features of Python? - Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. It has a comprehensive standard library and supports third-party libraries for various purposes.
How do you install Python? - Python can be installed from the official Python website (python.org) or using package managers like pip. It is available for Windows, macOS, and Linux.
What are Python variables? - Variables in Python are used to store data values. Unlike other programming languages, Python variables do not require explicit declaration or data type specification.
Data Structures
What are data structures in Python? - Data structures are used to store and organize data in a computer's memory. Python provides several built-in data structures, including lists, tuples, dictionaries, and sets.
What is a list in Python? - A list is a collection of items that are ordered and mutable. Lists are created using square brackets and can contain elements of different data types.
How do you access elements in a list? - Elements in a list can be accessed using index values. Indexing in Python starts from 0, so the first element of a list has an index of 0.
What is a tuple in Python? - A tuple is similar to a list but is immutable, meaning its elements cannot be changed once it is created. Tuples are created using parentheses.
How do you iterate over a dictionary in Python? - You can iterate over a dictionary using a for loop. By default, the loop iterates over the keys of the dictionary, but you can also iterate over the values or key-value pairs.
Control Flow
What is control flow in Python? - Control flow refers to the order in which statements are executed in a program. Python provides several control flow statements, including if-elif-else statements, for loops, and while loops.
How does the if statement work in Python? - The if statement is used to execute a block of code only if a certain condition is true. It can be followed by optional elif and else statements to handle multiple conditions.
What is a for loop in Python? - A for loop is used to iterate over a sequence of elements, such as a list or tuple. It repeatedly executes a block of code for each element in the sequence.
How does the while loop work in Python? - The while loop repeatedly executes a block of code as long as a specified condition is true. It is used when the number of iterations is not known before the loop starts.
What is the difference between break and continue statements in Python? - The break statement is used to exit a loop prematurely, while the continue statement is used to skip the rest of the code in a loop for the current iteration.
Functions and Modules
What are functions in Python? - Functions are blocks of reusable code that perform a specific task. They help in modularizing code and making it more organized and readable.
How do you define a function in Python? - Functions in Python are defined using the def keyword followed by the function name and parentheses containing any parameters. The function body is indented.
What is a module in Python? - A module is a file containing Python code that defines functions, classes, and variables. It allows you to organize your Python code into reusable components.
How do you import a module in Python? - You can import a module using the import keyword followed by the module name. You can also use the from...import statement to import specific functions or variables from a module.
What is the difference between local and global variables in Python? - Local variables are defined inside a function and are only accessible within that function, while global variables are defined outside any function and are accessible throughout the program.
Object-Oriented Programming (OOP)
What is object-oriented programming (OOP)? - OOP is a programming paradigm based on the concept of "objects," which can contain data in the form of attributes and code in the form of methods. Python supports OOP principles such as encapsulation, inheritance, and polymorphism.
How do you define a class in Python? - A class in Python is defined using the class keyword followed by the class name and a colon. It can contain attributes (variables) and methods (functions).
What is inheritance in Python? - Inheritance is a mechanism where a new class can inherit attributes and methods from an existing class. It allows for code reuse and the creation of a hierarchy of classes.
What is encapsulation in Python? - Encapsulation is the concept of restricting access to certain parts of an object, typically the internal data. In Python, encapsulation is achieved using private variables and methods.
What is polymorphism in Python? - Polymorphism is the ability of an object to take on different forms or behaviors simplicity and readability.
How do you comment in Python? - Comments in Python start with a hash (#) symbol and continue until the end of the line.
What are the basic data types in Python? - Python has several basic data types, including integers, floats, strings, booleans, and NoneType.
How do you define a variable in Python? - Variables in Python are defined using the assignment operator (=).
What is a tuple in Python? - A tuple is an immutable collection of elements, typically used to store related data.
Data Structures
What is a list in Python? - A list is a mutable collection of elements, enclosed in square brackets ([]), and can contain elements of different data types.
How do you access elements in a list? - Elements in a list can be accessed using their index, starting from 0.
What is a dictionary in Python? - A dictionary is a collection of key-value pairs, enclosed in curly braces ({}), and is mutable.
How do you iterate over a dictionary in Python? - You can iterate over a dictionary using a for loop, accessing the keys or values of each key-value pair.
What is a set in Python? - A set is an unordered collection of unique elements, enclosed in curly braces ({}).
Control Flow
What are the different types of loops in Python? - Python supports for loops and while loops for iteration.
How do you use conditional statements in Python? - Python uses if, elif, and else keywords for conditional statements.
What is the difference between break and continue statements? - The break statement terminates the loop, while the continue statement skips the current iteration and continues with the next iteration.
Functions and Modules
What is a function in Python? - A function is a block of reusable code that performs a specific task.
How do you define a function in Python? - Functions in Python are defined using the def keyword, followed by the function name and parameters.
What is a module in Python? - A module is a file containing Python code, which can define functions, classes, and variables.
How do you import a module in Python? - You can import a module using the import keyword, followed by the module name.
What is the difference between local and global variables? - Local variables are defined inside a function and are only accessible within that function, while global variables are defined outside any function and can be accessed throughout the program.
Object-Oriented Programming (OOP)
What is OOP? - Object-Oriented Programming is a programming paradigm based on the concept of "objects," which can contain data in the form of attributes and code in the form of methods.
What is a class in Python? - A class is a blueprint for creating objects, which defines the properties and behaviors of those objects.
How do you create a class in Python? - Classes in Python are created using the class keyword, followed by the class name and a colon (:).
What is an object in Python? - An object is an instance of a class, which has its own unique identity, state, and behavior.
What is inheritance in Python? - Inheritance is a mechanism where a new class derives attributes and methods from an existing class.
Exception Handling
What is exception handling in Python? - Exception handling is a mechanism to handle runtime errors or exceptions that occur during the execution of a program.
How do you use try-except blocks in Python? - You can use try-except blocks to catch and handle exceptions in Python.
What is the purpose of the finally block? - The finally block is used to execute code that should always run, whether or not an exception occurs.
File Handling
How do you open a file in Python? - You can open a file in Python using the open() function, specifying the file path and mode (read, write, append, etc.).
What is the difference between read() and readlines() methods? - The read() method reads the entire file contents as a single string, while the readlines() method reads the file line by line and returns a list of lines.
Regular Expressions
What are regular expressions (regex)? - Regular expressions are sequences of characters that define a search pattern, used for pattern matching within strings.
How do you use regular expressions in Automation Testing with Python ? - Python's re module provides functions and methods for working with regular expressions.
Python Libraries for Automation Testing
What is Selenium WebDriver? - Selenium WebDriver is a tool for automating web application testing, providing a programming interface for interacting with web elements.
How do you install Selenium WebDriver in Python? - You can install Selenium WebDriver using the pip package manager: pip install selenium.
What are the key features of Selenium WebDriver? - Selenium WebDriver supports various programming languages (including Python), multiple browsers, and parallel test execution.
Selenium WebDriver Basics
How do you create a WebDriver instance in selenium with python ? - You can create a WebDriver instance in Python using the webdriver module.
What are the different WebDriver methods for locating elements? - Selenium provides various methods for locating elements on a web page, such as find_element_by_id(), find_element_by_xpath(), etc.
Locators in Selenium WebDriver
What are locators in Selenium WebDriver? - Locators are used to identify and locate web elements on a web page, such as IDs, class names, XPath, etc.
How do you use XPath in Selenium WebDriver? - XPath is a language for navigating XML documents and is commonly used in Selenium WebDriver for locating web elements.
Waits in Selenium WebDriver
What are waits in Selenium WebDriver? - Waits are used to pause the execution of a test script for a certain amount of time or until a specific condition is met.
What are the different types of waits in Selenium WebDriver? - Selenium WebDriver supports implicit waits, explicit waits, and fluent waits.
Handling Dropdowns and Alerts in Selenium
How do you select an option from a dropdown menu in Selenium WebDriver? - You can use the Select class in Selenium WebDriver to interact with dropdown menus.
What are alerts in Selenium WebDriver? - Alerts are popup windows that appear during the execution of a test script, requiring user interaction.
Page Object Model (POM) in Selenium WebDriver
What is the Page Object Model (POM) in Selenium WebDriver? - The Page Object Model is a design pattern for organizing web elements and their interactions into reusable components.
How do you implement the Page Object Model in Selenium WebDriver? - You can create separate classes for each web page, defining locators and methods to interact with the page elements.
Conclusion
In conclusion, mastering Python for automation testing, especially with Selenium WebDriver, requires a solid understanding of python for automation testing fundamentals, object-oriented programming, and Selenium WebDriver basics. By familiarizing yourself with the top 50 Python interview questions outlined in this article, you'll be well-equipped to tackle automation testing challenges and excel in your career.
FAQs
What is Selenium WebDriver?
Selenium WebDriver is a tool for python automation testing web application testing, providing a programming interface for interacting with web elements.
How do you install Selenium WebDriver in Python?
You can install Selenium WebDriver using the pip package manager: pip install selenium.
What are locators in Selenium WebDriver?
Locators are used to identify and locate web elements on a web page, such as IDs, class names, XPath, etc.
What is the Page Object Model (POM) in Selenium WebDriver?
The Page Object Model is a design pattern for organizing web elements and their interactions into reusable components.
How do you handle alerts in Selenium WebDriver?
Alerts in Selenium WebDriver can be handled using the switch_to.alert method, which allows you to accept, dismiss, or get text from an alert.
By mastering these Python interview questions, you'll not only be well-prepared for interviews but also enhance your skills as an automation tester.
#Automation Testing with Python
0 notes
Text
Decoding Python's Building Blocks: A Comprehensive Exploration of Essential Data Types
Python, celebrated for its readability and adaptability, introduces a diverse set of fundamental data types that serve as the building blocks for programming proficiency. Whether you're an entry-level programmer entering the Python ecosystem or an experienced developer looking to refine your skills, a deep understanding of these data types is indispensable. Let's embark on a journey to uncover the significance and applications of Python's essential data types.
1. Numeric Foundations: The Power of Integers and Floats
At the core of numerical computations lie integers, representing whole numbers without decimal points. From negative values like -5 to positive ones like 42, Python enables seamless arithmetic operations on integers. Complementing this, floating-point numbers bring precision to calculations involving decimal points or exponential forms, ensuring accuracy in diverse mathematical operations.
2. Textual Mastery: Strings as the Linchpin
Strings, encapsulating sequences of characters within single or double quotes, are indispensable for handling textual data. From basic text manipulations to intricate natural language processing algorithms, strings play a pivotal role in diverse applications, making them a cornerstone data type for any Python developer.
3. Logic at the Core: The Significance of Booleans
Boolean data types, representing truth values (True and False), underpin logical operations and conditional statements. Whether validating user input or implementing decision-making processes, booleans are essential for shaping the logical flow within Python programs.
4. Dynamic Collections: Lists and Their Versatility
Python's lists, dynamic and ordered collections, can hold elements of different data types. Their versatility makes them ideal for managing datasets and storing sequences of values, providing flexibility in handling diverse programming scenarios.
5. Immutable Order: Tuples as Constant Entities
Similar to lists but immutable, tuples are ordered collections denoted by parentheses. This immutability ensures data integrity and makes tuples suitable for scenarios where data should remain constant throughout program execution.
6. Set Efficiency: Unleashing the Power of Sets
Sets, unordered collections of unique elements, excel in scenarios where membership testing and duplicate elimination are crucial. These data types streamline operations like removing duplicates from datasets, offering efficient data manipulation capabilities.
7. Key-Value Mastery: Diving into Python Dictionaries
Dictionaries, composed of key-value pairs, emerge as a powerhouse of efficiency. This data type facilitates swift retrieval of values based on unique keys, ensuring rapid access and manipulation of information. Dictionaries are invaluable for tasks like storing configuration settings or managing complex datasets.
Understanding and mastering these essential data types not only equips Python developers with versatile tools but also empowers them to tackle a broad spectrum of computational challenges. Whether you're venturing into web applications, exploring data science, or diving into machine learning, a robust grasp of Python's data types serves as the foundation for programming excellence. As you navigate the Python landscape, consider these data types as more than mere code elements—they are the fundamental structures shaping your journey towards creating efficient and robust solutions. Embrace Python's data types, and let them guide you towards programming excellence.
0 notes
Text
Mastering Python Programming: A Comprehensive Guide for Beginners
Python, a versatile and powerful programming language, has gained immense popularity for its simplicity and readability. Whether you're a newcomer to the world of coding or an experienced developer looking to expand your skill set, mastering Python is a valuable asset. This comprehensive guide will take you through the essential aspects of Python programming, providing a solid foundation for your journey into the realm of software development.
1. Introduction to Python Programming
Python is an interpreted, high-level, and general-purpose programming language known for its clean syntax and easy-to-learn structure. Guido van Rossum created Python in the late 1980s, and since then, it has become a go-to language for various applications, including web development, data analysis, artificial intelligence, and more. Its readability and versatility make it an ideal choice for beginners.
2. Setting Up Your Python Environment
Before diving into Python programming, it's crucial to set up your development environment. Python is compatible with various operating systems, including Windows, macOS, and Linux. You can download and install Python from the official website (python.org) or use package managers like Anaconda. Additionally, understanding virtual environments will help you manage dependencies and keep your project isolated from others.
3. Basic Python Syntax and Data Types
Python's syntax is designed to be readable and straightforward. Learning the basic syntax and data types is the first step in mastering Python. Explore concepts like variables, data types (integers, floats, strings), and basic operations. Understand how indentation plays a crucial role in Python to define code blocks and control structures.
4. Control Flow and Loops
Mastering control flow and loops is essential for creating dynamic and efficient programs. Learn about conditional statements (if, elif, else) and various loop structures (for and while loops). These constructs are fundamental for making decisions and iterating through data, allowing you to control the flow of your program effectively.
5. Functions and Modules
Understanding functions is key to writing modular and reusable code. Explore how to define and call functions, pass arguments, and return values. Dive into the concept of modules to organize your code into separate files, making it more manageable and scalable. Python's extensive standard library and the ability to create custom modules enhance the language's functionality.
6. Data Structures in Python
Python provides a rich set of data structures to handle and manipulate data efficiently. Learn about lists, tuples, dictionaries, and sets. Grasp the concept of indexing and slicing to access and manipulate elements within these data structures. Understanding the strengths and use cases of each data structure empowers you to choose the right one for your specific needs.
7. File Handling in Python
File handling is a crucial aspect of any programming language, and Python makes it simple. Explore how to open, read, write, and close files using Python. Understand file modes, such as read ('r'), write ('w'), and append ('a'). Learning file handling is essential for tasks like reading data from external files or storing program output.
8. Introduction to Object-Oriented Programming (OOP) in Python
Python supports object-oriented programming, a paradigm that focuses on creating reusable and modular code through the use of classes and objects. Learn about classes, objects, inheritance, encapsulation, and polymorphism. Understanding OOP concepts will enable you to design and implement more complex and scalable software solutions.
9. Python Libraries and Frameworks
Python's strength lies not only in its core language features but also in its vast ecosystem of libraries and frameworks. Explore popular libraries like NumPy and pandas for data manipulation, Matplotlib and Seaborn for data visualization, and Flask or Django for web development. Understanding these libraries empowers you to leverage existing tools and accelerate your development process.
Conclusion
Mastering Python programming is an exciting and rewarding journey, especially for beginners entering the world of coding. This comprehensive guide has provided an overview of essential topics, from setting up your environment to exploring advanced concepts like object-oriented programming and Python libraries. As you continue your Python learning journey, practice is key. Work on projects, participate in coding challenges, and engage with the vibrant Python community to enhance your skills and stay updated with the latest developments. With dedication and continuous learning, you'll soon find yourself proficient in Python programming, ready to tackle a wide range of software development challenges.
#onlinetraining#career#online courses#automation#technology#learning#security#programming#elearning#startups
0 notes
Text
Python FullStack Developer Interview Questions
Introduction :
A Python full-stack developer is a professional who has expertise in both front-end and back-end development using Python as their primary programming language. This means they are skilled in building web applications from the user interface to the server-side logic and the database. Here’s some information about Python full-stack developer jobs.
Interview questions
What is the difference between list and tuple in Python?
Explain the concept of PEP 8.
How does Python's garbage collection work?
Describe the differences between Flask and Django.
Explain the Global Interpreter Lock (GIL) in Python.
How does asynchronous programming work in Python?
What is the purpose of the ORM in Django?
Explain the concept of middleware in Flask.
How does WSGI work in the context of web applications?
Describe the process of deploying a Flask application to a production server.
How does data caching improve the performance of a web application?
Explain the concept of a virtual environment in Python and why it's useful.
Questions with answer
What is the difference between list and tuple in Python?
Answer: Lists are mutable, while tuples are immutable. This means that you can change the elements of a list, but once a tuple is created, you cannot change its values.
Explain the concept of PEP 8.
Answer: PEP 8 is the style guide for Python code. It provides conventions for writing code, such as indentation, whitespace, and naming conventions, to make the code more readable and consistent.
How does Python's garbage collection work?
Answer: Python uses automatic memory management via a garbage collector. It keeps track of all the references to objects and deletes objects that are no longer referenced, freeing up memory.
Describe the differences between Flask and Django.
Answer: Flask is a micro-framework, providing flexibility and simplicity, while Django is a full-stack web framework with built-in features like an ORM, admin panel, and authentication.
Explain the Global Interpreter Lock (GIL) in Python.
Answer: The GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecode at once. This can limit the performance of multi-threaded Python programs.
How does asynchronous programming work in Python?
Answer: Asynchronous programming in Python is achieved using the asyncio module. It allows non-blocking I/O operations by using coroutines, the async and await keywords, and an event loop.
What is the purpose of the ORM in Django?
Answer: The Object-Relational Mapping (ORM) in Django allows developers to interact with the database using Python code, abstracting away the SQL queries. It simplifies database operations and makes the code more readable.
Explain the concept of middleware in Flask.
Answer: Middleware in Flask is a way to process requests and responses globally before they reach the view function. It can be used for tasks such as authentication, logging, and modifying request/response objects.
How does WSGI work in the context of web applications?
Answer: Web Server Gateway Interface (WSGI) is a specification for a universal interface between web servers and Python web applications or frameworks. It defines a standard interface for communication, allowing compatibility between web servers and Python web applications.
Describe the process of deploying a Flask application to a production server.
Answer: Deploying a Flask application involves configuring a production-ready web server (e.g., Gunicorn or uWSGI), setting up a reverse proxy (e.g., Nginx or Apache), and handling security considerations like firewalls and SSL.
How does data caching improve the performance of a web application?
Answer: Data caching involves storing frequently accessed data in memory, reducing the need to fetch it from the database repeatedly. This can significantly improve the performance of a web application by reducing the overall response time.
Explain the concept of a virtual environment in Python and why it's useful.
Answer: A virtual environment is a self-contained directory that contains its Python interpreter and can have its own installed packages. It is useful to isolate project dependencies, preventing conflicts between different projects and maintaining a clean and reproducible development environment.
When applying for a Python Full Stack Developer position:
Front-end Development:
What is the Document Object Model (DOM), and how does it relate to front-end development?
Explain the difference between inline, internal, and external CSS styles. When would you use each one?
What is responsive web design, and how can you achieve it in a web application?
Describe the purpose and usage of HTML5 semantic elements, such as , , , and .
What is the role of JavaScript in front-end development? How can you handle asynchronous operations in JavaScript?
How can you optimize the performance of a web page, including techniques for reducing load times and improving user experience?
What is Cross-Origin Resource Sharing (CORS), and how can it be addressed in web development?
Back-end Development:
Explain the difference between a web server and an application server. How do they work together in web development?
What is the purpose of a RESTful API, and how does it differ from SOAP or GraphQL?
Describe the concept of middleware in the context of web frameworks like Django or Flask. Provide an example use case for middleware.
How does session management work in web applications, and what are the common security considerations for session handling?
What is an ORM (Object-Relational Mapping), and why is it useful in database interactions with Python?
Discuss the benefits and drawbacks of different database systems (e.g., SQL, NoSQL) for various types of applications.
How can you optimize a database query for performance, and what tools or techniques can be used for profiling and debugging SQL queries?
Full Stack Development:
What is the role of the Model-View-Controller (MVC) design pattern in web development, and how does it apply to frameworks like Django or Flask?
How can you ensure the security of data transfer in a web application? Explain the use of HTTPS, SSL/TLS, and encryption.
Discuss the importance of version control systems like Git in collaborative development and deployment.
What are microservices, and how do they differ from monolithic architectures in web development?
How can you handle authentication and authorization in a web application, and what are the best practices for user authentication?
Describe the concept of DevOps and its role in the full stack development process, including continuous integration and deployment (CI/CD).
These interview questions cover a range of topics relevant to a Python Full Stack Developer position. Be prepared to discuss your experience and demonstrate your knowledge in both front-end and back-end development, as well as the integration of these components into a cohesive web application.
Database Integration: Full stack developers need to work with databases, both SQL and NoSQL, to store and retrieve data. Understanding database design, optimization, and querying is crucial.
Web Frameworks: Questions about web frameworks like Django, Flask, or Pyramid, which are popular in the Python ecosystem, are common. Understanding the framework’s architecture and best practices is essential.
Version Control: Proficiency in version control systems like Git is often assessed, as it’s crucial for collaboration and code management in development teams.
Responsive Web Design: Full stack developers should understand responsive web design principles to ensure that web applications are accessible and user-friendly on various devices and screen sizes.
API Development: Building and consuming APIs (Application Programming Interfaces) is a common task. Understanding RESTful principles and API security is important.
Web Security: Security questions may cover topics such as authentication, authorization, securing data, and protecting against common web vulnerabilities like SQL injection and cross-site scripting (XSS).
DevOps and Deployment: Full stack developers are often involved in deploying web applications. Understanding deployment strategies, containerization (e.g., Docker), and CI/CD (Continuous Integration/Continuous Deployment) practices may be discussed.
Performance Optimization: Questions may be related to optimizing web application performance, including front-end and back-end optimizations, caching, and load balancing.
Coding Best Practices: Expect questions about coding standards, design patterns, and best practices for maintainable and scalable code.
Problem-Solving: Scenario-based questions and coding challenges may be used to evaluate a candidate’s problem-solving skills and ability to think critically.
Soft Skills: Employers may assess soft skills like teamwork, communication, adaptability, and the ability to work in a collaborative and fast-paced environment.
To prepare for a Python Full Stack Developer interview, it’s important to have a strong understanding of both front-end and back-end technologies, as well as a deep knowledge of Python and its relevant libraries and frameworks. Additionally, you should be able to demonstrate your ability to work on the full development stack, from the user interface to the server infrastructure, while following best practices and maintaining a strong focus on web security and performance.
Python Full Stack Developer interviews often include a combination of technical assessments, coding challenges, and behavioral or situational questions to comprehensively evaluate a candidate’s qualifications, skills, and overall fit for the role. Here’s more information on each of these interview components:
1. Technical Assessments:
Technical assessments typically consist of questions related to various aspects of full stack development, including front-end and back-end technologies, Python, web frameworks, databases, and more.
These questions aim to assess a candidate’s knowledge, expertise, and problem-solving skills in the context of web development using Python.
Technical assessments may include multiple-choice questions, fill-in-the-blank questions, and short-answer questions.
2. Coding Challenges:
Coding challenges are a critical part of the interview process for Python Full Stack Developers. Candidates are presented with coding problems or tasks to evaluate their programming skills, logical thinking, and ability to write clean and efficient code.
These challenges can be related to web application development, data manipulation, algorithmic problem-solving, or other relevant topics.
Candidates are often asked to write code in Python to solve specific problems or implement certain features.
Coding challenges may be conducted on a shared coding platform, where candidates can write and execute code in real-time.
3. Behavioral or Situational Questions:
Behavioral or situational questions assess a candidate’s soft skills, interpersonal abilities, and how well they would fit within the company culture and team.
These questions often focus on the candidate’s work experiences, decision-making, communication skills, and how they handle challenging situations.
Behavioral questions may include scenarios like, “Tell me about a time when you had to work under tight deadlines,” or “How do you handle conflicts within a development team?”
4. Project Discussions and Portfolio Review:
Candidates may be asked to discuss their previous projects, providing details about their roles, responsibilities, and the technologies they used.
Interviewers may inquire about the candidate’s contributions to the project, the challenges they faced, and how they overcame them.
Reviewing a candidate’s portfolio or past work is a common practice to understand their practical experience and the quality of their work.
5. Technical Whiteboard Sessions:
Some interviews may include whiteboard sessions where candidates are asked to solve technical problems or explain complex concepts on a whiteboard.
This assesses a candidate’s ability to communicate technical ideas and solutions clearly.
6. System Design Interviews:
For more senior roles, interviews may involve system design discussions. Candidates are asked to design and discuss the architecture of a web application, focusing on scalability, performance, and data storage considerations.
7. Communication and Teamwork Evaluation:
Throughout the interview process, assessors pay attention to how candidates communicate their ideas, interact with interviewers, and demonstrate their ability to work in a collaborative team environment.
8. Cultural Fit Assessment:
Employers often evaluate how well candidates align with the company’s culture, values, and work ethic. They may ask questions to gauge a candidate’s alignment with the organization’s mission and vision.
conclusion :
Preparing for a Python Full Stack Developer interview involves reviewing technical concepts, practicing coding challenges, and developing strong communication and problem-solving skills. Candidates should be ready to discuss their past experiences, showcase their coding abilities, and demonstrate their ability to work effectively in a full stack development role, which often requires expertise in both front-end and back-end technologie
Thanks for reading ,hopefully you like the article if you want to take Full stack Masters course from our Institute please attend our live demo sessions or contact us: +918464844555 providing you with the best Online Full Stack Developer Course in Hyderabad with an affordable course fee structure.
0 notes
Text
Tuples in python
Tuples in Python are immutable sequences of objects. This means that once a tuple is created, its elements cannot be changed. Tuples are represented by parentheses (), and their elements are separated by commas. For example, the following code creates a tuple of strings:
Python
my_tuple = ("apple", "banana", "cherry")
Tuples can be accessed using indices, just like lists. However, you cannot modify the elements of a tuple using their indices.
Here is an example of how to access the elements of a tuple:
Python
print(my_tuple[0])
Output:
apple
Tuples can also be sliced, just like lists. However, the resulting slice will be a new tuple, not a modified version of the original tuple.
Here is an example of how to slice a tuple:
Python
print(my_tuple[0:2])
Output:
('apple', 'banana')
Tuples can be used to store data, iterate over data, and perform data analysis. They are also often used as keys in dictionaries.
Here are some examples of how to use tuples in Python:
Python
# Create a tuple of numbers numbers = (1, 2, 3, 4, 5) # Print the tuple print(numbers) # Iterate over the tuple for number in numbers: print(number) # Use a tuple as a key in a dictionary my_dict = {numbers[0]: "apple"} # Print the value associated with the key (1, 2, 3) print(my_dict[numbers[0:3]])
Output:
(1, 2, 3, 4, 5) 1 2 3 4 5 apple
Tuples are a powerful tool for working with collections of data in Python. They are immutable, which makes them safe to use in concurrent applications.
#codeblr#programming#coding#progblr#programmer#studyblr#learning to code#codetober#python#kumar's python study notes
0 notes