#PythonHomeworkHelp
Explore tagged Tumblr posts
Text
Building Predictive Models with Regression Libraries in Python Assignments
Introduction
Predictive modeling serves as a fundamental method for data-driven decisions that allows to predict outcomes, analyze trends, and forecast likely scenarios from the existing data. Predictive models are the ones that forecast the future outcomes based on historical data and helps in the understanding of hidden patterns. Predictive modeling is an essential technique in data science for applications in healthcare, finance, marketing, technology, and virtually every area. Often such models are taught to students taking statistics or Data Science courses so that they can utilize Python’s vast libraries to build and improve regression models for solving real problems.
Python has been the popular default language for predictive modeling owing to its ease of use, flexibility, and availability of libraries that are specific to data analysis and machine learning. From cleaning to building models, and even evaluating the performance of models, you can do all of these with Python tools like sci-kit-learn and stats models, as well as for data analysis using the pandas tool. Getting acquainted with these tools requires following certain procedures, writing optimized codes, and consistent practice. Availing of Python help service can be helpful for students requiring extra assistance with assignments or with coding issues in predictive modeling tasks.
In this article, we take you through techniques in predictive modeling with coding illustrations on how they can be implemented in Python. Specifically, the guide will be resourceful for students handling data analysis work and seeking python assignment help.
Why Regression Analysis?
Regression analysis is one of the preliminary methods of predictive modeling. It enables us to test and measure both the strength and the direction between a dependent variable [that is outcome variable] and one or more independent variables [also referred to as the predictors]. Some of the most commonly used regression techniques have been mentioned below: • Linear Regression: An easy-to-understand but very effective procedure for predicting the value of a dependent variable as the linear combination of the independent variables. • Polynomial Regression: This is a linear regression with a polynomial relationship between predictors and an outcome. • Logistic Regression: Especially popular in classification problems with two outcomes, logistic regression provides the likelihood of the occurrence of specific event. • Ridge and Lasso Regression: These are the more standardized types of linear regression models that prevent overfitting.
Step-by-Step Guide to Building Predictive Models in Python
1. Setting Up Your Python Environment
First of all: you need to prepare the Python environment for data analysis. Jupyter Notebooks are perfect as it is a platform for writing and executing code in small segments. You’ll need the following libraries:
# Install necessary packages
!pip install numpy pandas matplotlib seaborn scikit-learn statsmodels
2. Loading and Understanding the Dataset
For this example, we’ll use a sample dataset: ‘student_scores.csv’ file that consists of records of Study hours and Scores of the students. It is a simple one, but ideal for the demonstration of basics of regression. The dataset has two columns: Numerical variables include study hours referred to as Hours; and exam scores referred as Scores.
Download the students_scores.csv file to follow along with the code below.
import pandas as pd
# Load the dataset
data = pd.read_csv("students_scores.csv")
data.head()
3. Exploratory Data Analysis (EDA)
Let us first understand the data before we perform regression in python. Let us first explore the basic relationship between the two variables – the number of hours spent studying and the scores.
import matplotlib.pyplot as plt
import seaborn as sns
# Plot Hours vs. Scores
plt.figure(figsize=(8,5))
sns.scatterplot(data=data, x='Hours', y='Scores')
plt.title('Study Hours vs. Exam Scores')
plt.xlabel('Hours Studied')
plt.ylabel('Exam Scores')
plt.show()
While analyzing the scatter plot we can clearly say the higher the hours studied, the higher the scores. With this background, it will be easier to build a regression model.
4. Building a Simple Linear Regression Model
Importing Libraries and Splitting Data
First, let’s use the tool offered by the sci-kit-learn to split the data into training and testing data that is necessary to check the performance of the model
from sklearn.model_selection import train_test_split
# Define features (X) and target (y)
X = data[['Hours']]
y = data['Scores']
# Split data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Training the Linear Regression Model
Now, we’ll fit a linear regression model to predict exam scores based on study hours.
from sklearn.linear_model import LinearRegression
# Initialize the model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
# Display the model's coefficients
print(f"Intercept: {model.intercept_}")
print(f"Coefficient for Hours: {model.coef_[0]}")
This model equation is Scores = Intercept + Coefficient * Hours.
Making Predictions and Evaluating the Model
Next, we’ll make predictions on the test set and evaluate the model's performance using the Mean Absolute Error (MAE).
from sklearn.metrics import mean_absolute_error
# Predict on the test set
y_pred = model.predict(X_test)
# Calculate MAE
mae = mean_absolute_error(y_test, y_pred)
print(f"Mean Absolute Error: {mae}")
A lower MAE indicates that the model's predictions are close to the actual scores, which confirms that hours studied is a strong predictor of exam performance.
Visualizing the Regression Line
Let’s add the regression line to our initial scatter plot to confirm the fit.
# Plot data points and regression line
plt.figure(figsize=(8,5))
sns.scatterplot(data=data, x='Hours', y='Scores')
plt.plot(X, model.predict(X), color='red') # Regression line
plt.title('Regression Line for Study Hours vs. Exam Scores')
plt.xlabel('Hours Studied')
plt.ylabel('Exam Scores')
plt.show()
If you need more assistance with other regression techniques, opting for our Python assignment help services provides the necessary support at crunch times.
5. Improving the Model with Polynomial Regression
If the relationship between variables is non-linear, we can use polynomial regression to capture complexity. Here’s how to fit a polynomial regression model.
from sklearn.preprocessing import PolynomialFeatures
# Transform the data to include polynomial features
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
# Split the transformed data
X_train_poly, X_test_poly, y_train_poly, y_test_poly = train_test_split(X_poly, y, test_size=0.2, random_state=42)
# Fit the polynomial regression model
model_poly = LinearRegression()
model_poly.fit(X_train_poly, y_train_poly)
# Predict and evaluate
y_pred_poly = model_poly.predict(X_test_poly)
mae_poly = mean_absolute_error(y_test_poly, y_pred_poly)
print(f"Polynomial Regression MAE: {mae_poly}")
6. Adding Regularization with Ridge and Lasso Regression
To handle overfitting, especially with complex models, regularization techniques like Ridge and Lasso are useful. Here’s how to apply Ridge regression:
from sklearn.linear_model import Ridge
# Initialize and train the Ridge model
ridge_model = Ridge(alpha=1.0)
ridge_model.fit(X_train, y_train)
# Predict and evaluate
y_pred_ridge = ridge_model.predict(X_test)
mae_ridge = mean_absolute_error(y_test, y_pred_ridge)
print(f"Ridge Regression MAE: {mae_ridge}")
Empowering Students in Python: Assignment help for improving coding skills
Working on predictive modeling in Python can be both challenging and rewarding. Every aspect of the service we offer through Python assignment help is precisely designed to enable students not only to work through the assignments but also to obtain a better understanding of the concepts and the use of optimized Python coding in the assignments. Our approach is focused on student learning in terms of improving the fundamentals of the Python programming language, data analysis methods, and statistical modeling techniques.
There are a few defined areas where our service stands out
First, we focus on individual learning and tutoring.
Second, we provide comprehensive solutions and post-delivery support. Students get written solutions to all assignments, broken down into steps of the code and detailed explanations of the statistical method used so that the students may replicate the work in other projects.
As you choose our service, you get help from a team of professional statisticians and Python coders who will explain the complex concept, help to overcome technical difficulties and give recommendations on how to improve the code.
In addition to predictive analytics, we provide thorough consultation on all aspects of statistical analysis using Python. Our services include assistance with key methods such as:
• Descriptive Statistics
• Inferential Statistics
• Regression Analysis
• Time Series Analysis
• Machine Learning Algorithms
Hire our Python assignment support service, and you will not only get professional assistance with your tasks but also the knowledge and skills that you can utilize in your future assignments.
Conclusion In this guide, we introduced several approaches to predictive modeling with the use of Python libraries. Thus, by applying linear regression, polynomial regression, and Ridge regularization students will be able to develop an understanding of how to predict and adjust models depending on the complexity of the given data. These techniques are very useful for students who engage in data analysis assignments as these techniques are helpful in handling predictive modeling with high accuracy. Also, take advantage of engaging with our Python assignment help expert who can not only solve your Python coding issues but also provide valuable feedback on your work for any possible improvements.
#PythonAssignmentHelp#PythonHelp#PythonHomeworkHelp#PythonProgramming#CodingHelp#PythonTutoring#PythonAssignments#PythonExperts#LearnPython#PythonProgrammingHelp#PythonCoding#PythonSupport#ProgrammingHelp#AssignmentHelp#PythonTutors#PythonCourseworkHelp#PythonAssistance#PythonForBeginners#PythonProjectHelp#OnlinePythonHelp
0 notes
Text
Is the deadline approaching for the submission of your Python homework? No worries! Connect with our team and get instant solutions to your queries. We have a qualified team to help you solve tough and complicated questions on time. For further details, connect with the professionals.
#pythonhomeworkhelp#homeworkhelp#programminghomeworkhelp#abchomeworkhelp#homeworkwriting#writinghelp#instanthomeworkhelp
0 notes
Text
Need help with Python? We're all about keeping it simple and stress-free! Reach out today! 🌟
#pythonhomeworkhelp#homeworkhelper#onlineassignmenthelp#pythonprogramming#coders#assignmenthelper#examhelp#onlineprogramminghelp
0 notes
Text
Python Homework Help
We provide the best Python Homework Help online, and we guarantee that the clients get the highest score. We can work in a short time duration by maintaining the quality of work. Your deadline becomes our priority when you hire us!
Reach out to our team via: -
Email: [email protected]
Call/WhatsApp: +1(507)509–5569
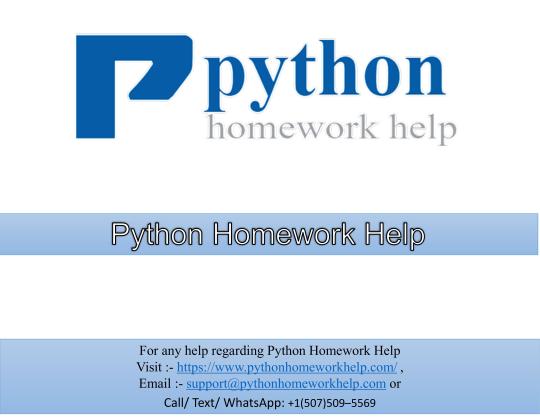
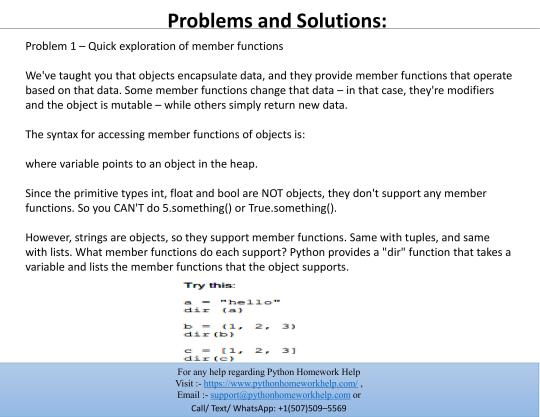
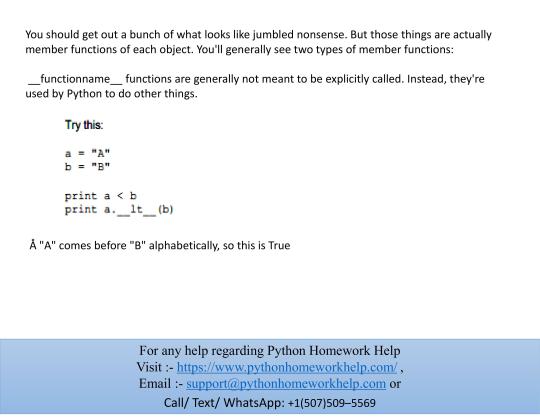
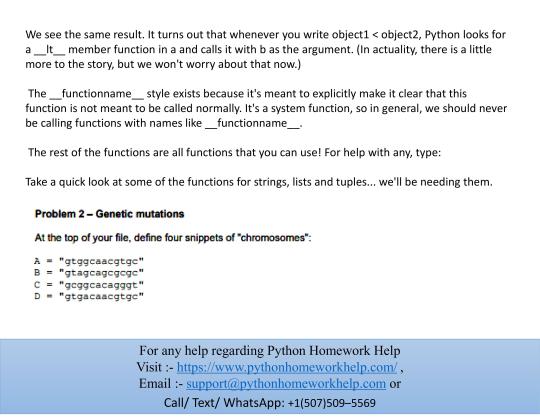
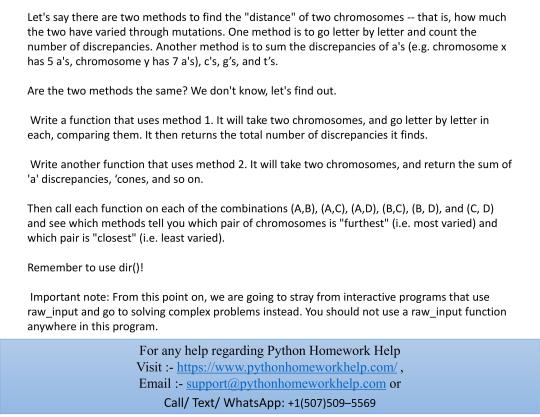
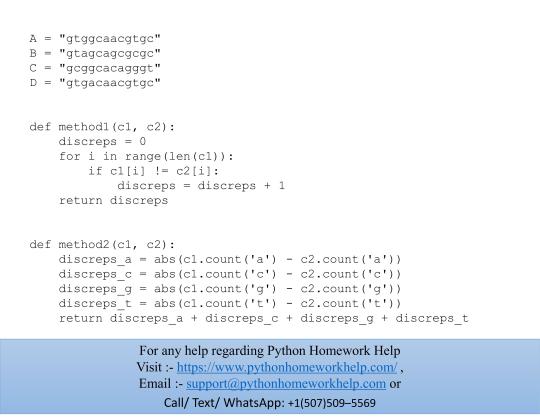
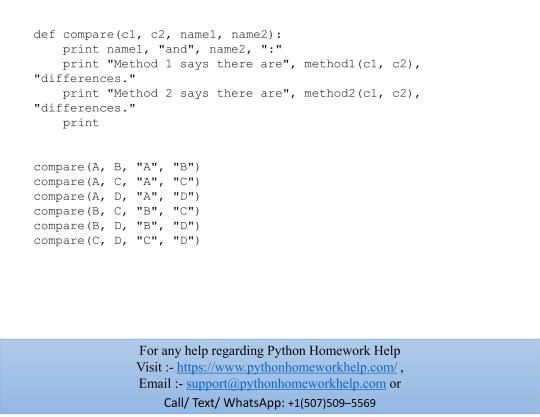
#python homework help#educational website#study tips#homework help#educational service#tutoring services#pythonhomeworkhelp#domypythonassignment#pythonassignmenthelp
0 notes
Text
Python Assignment Help
Assignmentproxy is a python assignment help company that provides immediate and reliable online web development, mobile app development, & marketing services. We have been wowing our clients for quite some time now by providing them with top-notch service quality at affordable rates!
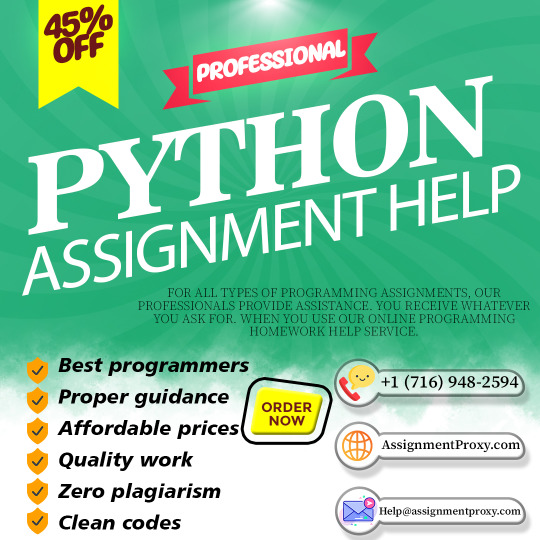
https://assignmentproxy.com/category/programming-help/python-assignment-help
#pythonhomeworkhelp#assignment services#python assignment help#python#courseworkhelp#courseworkwritingservice#examhelp#coding#coding help#java assignment help
0 notes
Text
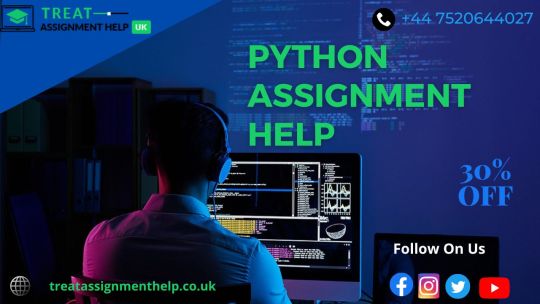
Why Do Students Have Difficulties With Python Programming Homework?
Python became a leading programming language as soon as it was launched in the world of programming languages. Python Assignment Help services are getting more business because more the students will enroll in computer programming programs, the more will be assignments to do. The reason behind the success of python is that it is very easy to understand.
#PythonAssignmentHelp#PythonProgrammingAssignmentHelpOnline#Pythonprogrammingassignmentexperts#PythonHomeworkHelp
0 notes
Photo
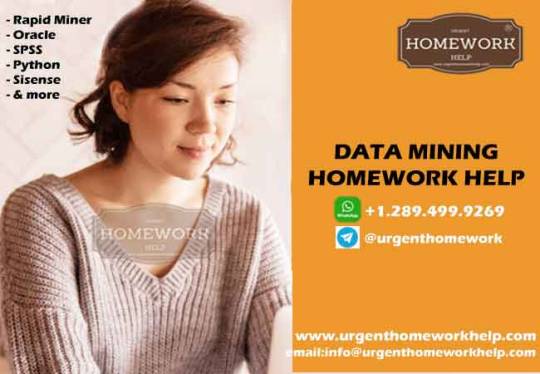
Data mining homework help provided by top data scientists and researchers. We guarantee accurate solutions, 24/7 support online and assured delivery on time. Urgent assignments delivered on priority. Full support for Rapidminer, Oracle, SPSS, Python, Sisense and others. Urgent assignments delivered within 24 hours.
Visit our website for more details: https://www.urgenthomeworkhelp.com/data-mining-homework-help.php
#datamininghomeworkhelp#dataminingassignmenthelp#datamining#RapidMinerhomeworkhelp#Sisensehomeworkhelp#Oraclehomeworkhelp#SPSShomeworkhelp#Pythonhomeworkhelp#dataresearchomeworkhelp
0 notes
Text
A Python expert has to write page after page codes to run successfully and offer Python Homework Help. Are you ready to write error-free Python codes? Are you confident? If you are not, then hire experts from My Assignment Experts.
0 notes
Link
Python programming assignment help is one of the most alluring services for students in this era. Doing Python coding is not a matter of joke. To work on Python, students need to give full concentration and interest to learn and execute it. For all the students, it is a quite troublesome matter. For that reason, they choose python programming help.
Hiring python professionals is a good option for students. While students pay someone to do Python, experts will handle the python assignment, The Statistics Assignment Help provide accurate python homework solutions step-by-step. With the answers, students can learn to solve such types of python project assignments.
#PythonHelpMalaysia#AssignmentHelpUSA#PythonHomeworkHelp#pythonassignmenthelp#pythonprojecthelp#domypythonassignment
0 notes
Photo
Get difficulty in c programming language assignment or homework, don't worry ask our online chat support they help you to solve your problems.
Visit: https://www.urgenthomework.com/c_programming_language_help
#onlinehomeworkhelp onlineassignmenthelp homeworkhelp assignmenthelp onlineassignment#pythonhomeworkhelp pythomassignmenthelp pythonprogramminghelp pythonprojecthelp pythonlanguagehelp pythoncodehelp helpwithpython pythononlin
0 notes
Photo
For error-free Python Homework Help- get in touch with the experts. The team provides instant solutions to your complicated homework. We are available to provide 100% genuine solutions.
0 notes
Text
www.theprogrammingassignmenthelp.com
www.theprogrammingassignmenthelp.com are dedicated to ensuring you get the best service that suits you. Inquire about www.theprogrammingassignmenthelp.com now and say goodbye to all the stress that comes with programming.
0 notes
Photo
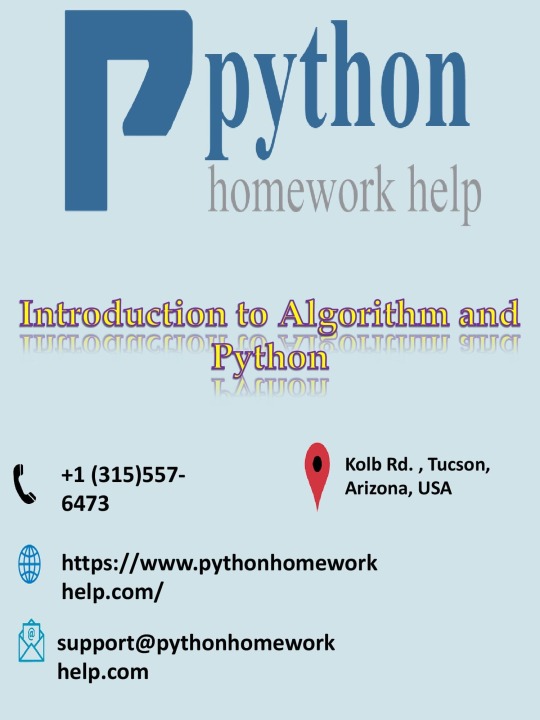
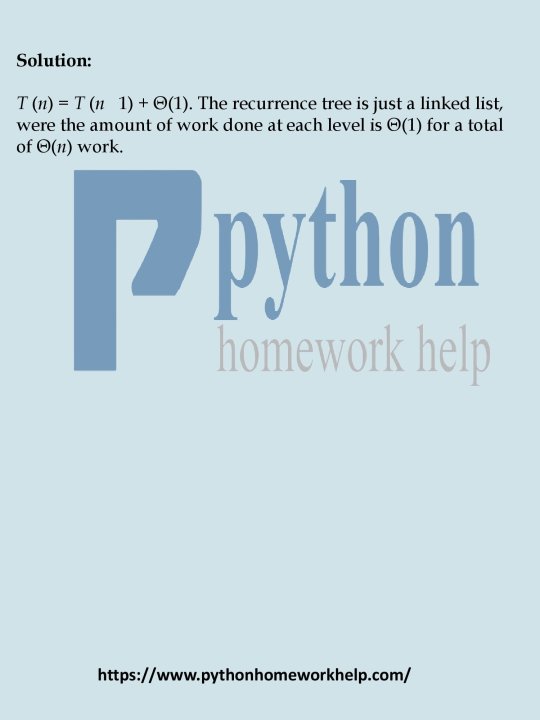
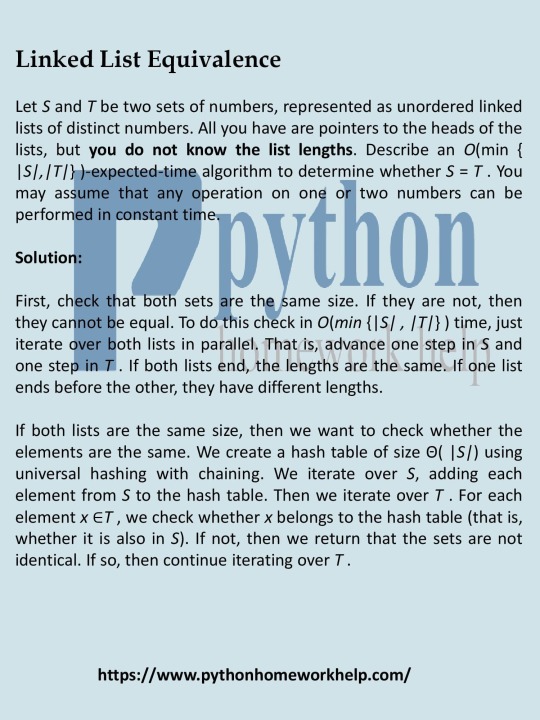
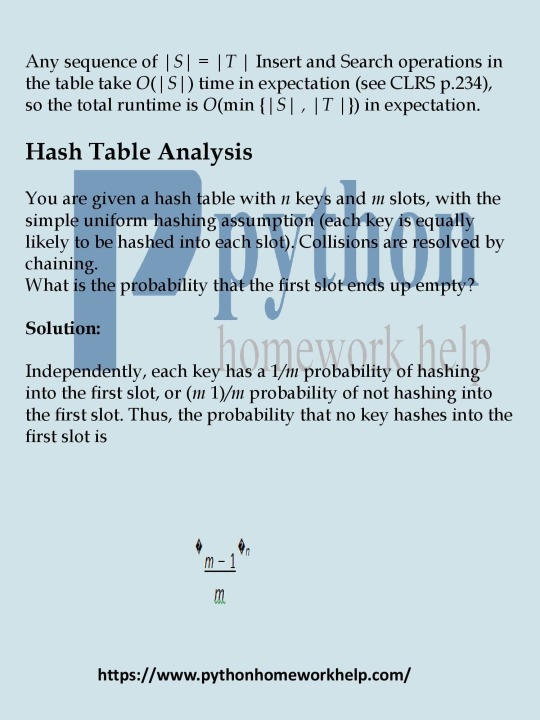
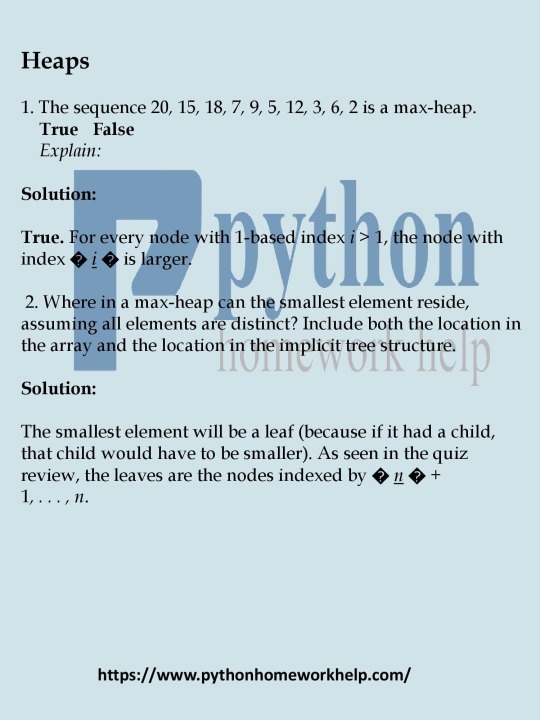
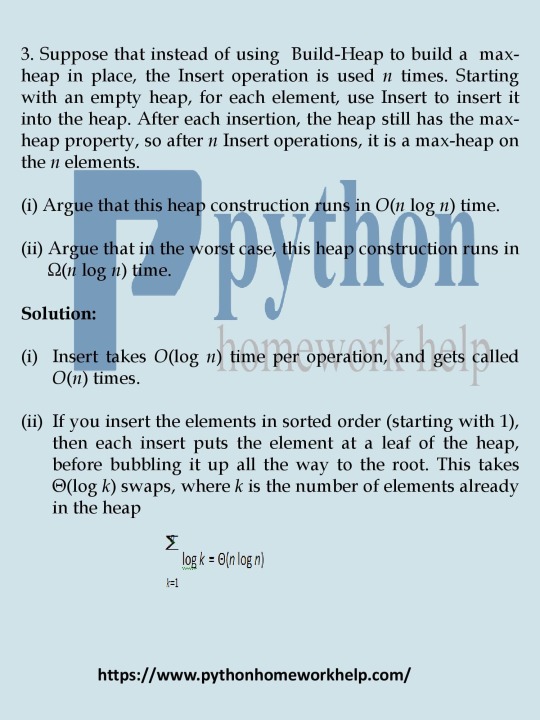
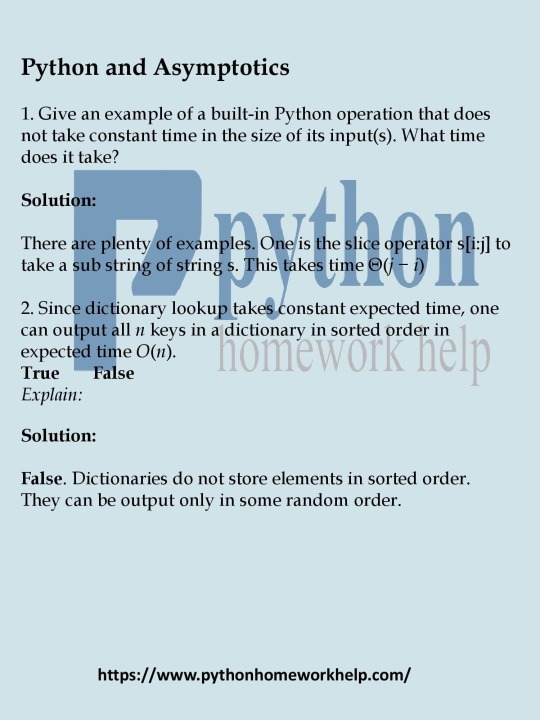
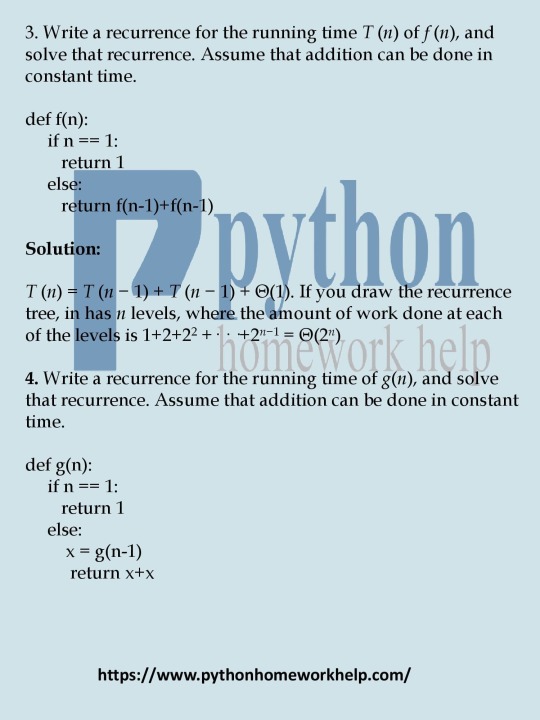
I am Sam Collee. I am a Python Homework Help Expert at pythonhomeworkhelp.com. I hold a Master's in Programming, from Ohio University, United States. I have been helping students with their Python Programming Homework for the past 5 years. You can assess my work from the following sample on Introduction to Algorithm and Python. For hiring me, Visit www.pythonhomeworkhelp.com or email [email protected], you can also call +1 (315) 557-6473.
#pythonhomeworkhelp#pythonhomeworkhelper#domypythonhomework#pythonexamhelp#education#students#university#homework#assignment#exam#tutoring#python#programming#highereducation#writing
0 notes
Text
Python Programming assignment help
Python is a general purpose, interactive, high-level programming language. After its introduction in the late 1980’s, the Python programming language is being widely used by programmers to express concepts in just fewer lines in comparison to languages like JAVA and C++. Combat programming flaws with Python programming assignment help.
In addition, stuffed with dynamic features and automated memory management systems, Python underpins multiple programming paradigms that include imperative and functional programming, object-oriented languages etc.
Want Python programming homework help? Contact the statistics assignment help
Python is an excellent computer programming subject that enhances the computation skills of students. thestatisticsassignmenthelp.com inspires the students to gain thorough knowledge of the subject through our high-quality write-ups and Python programming assignment help. Our unique expertise and efficient work process have helped us to stand out of the crowd. Our programming assignment experts are equipped with adequate skills and techniques to provide Python programming assignment help with efficacy.
Python programming assignment help is one of the most alluring services for students in this era. Doing Python coding is not a matter of joke. To work on Python, students need to give full concentration and interest to learn and execute it. For all the students, it is a quite troublesome matter. For that reason, they choose python programming help.
Hiring python professionals is a good option for students. While students pay someone to do Python, experts will handle the python assignment, The Statistics Assignment Help provide accurate python homework solutions step-by-step. With the answers, students can learn to solve such types of python project assignment help.
#pythonprogramingassignmenthelp#pythonprogramminghelp#pythonhomeworkhelp#AssignmentHelpUSA#assignmenthelpUK#StatisticsAssignmenthelp#assignmenthelpcanada#HomeworkHelpUSA#HomeworkHelpAustralia#HomeworkHelpCanada#AsisgnmentHelpUAE#HomeworkHelpUAE
0 notes
Text
Python Simple Project Ideas
Click here: https://wethecoders.com/python-homework-help/
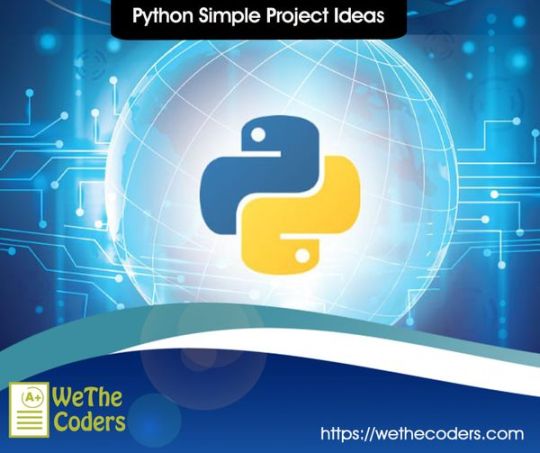
Are you having a hard time with python assignment or python homework given to you, and asking yourself whether anybody would help you out to do the Python coding with a little help? – We offer the best Python homework help, Python online help, Python Support.
#PythonSimpleProjectIdeas, #PythonHomeworkHelp, #PythonAssignmentHelp, #PythonProjectHelp
0 notes
Text
Why Is Our Python Assignment Help Important?
Have you been desirous of scoring first-class grades with Python? Smile with relief as everything is about to change with Programming Online Help. Book our Python Assignment Help and see your goals come into realization. Yeah, we are the only reliable academic site to trust in this domain. Entrust our services today, and you will regret it.
No, you don’t have to go around asking your friends whether Programming Online Help is the best Python Assignment Helper. Guess what? We have incredible solutions to your assignment woes. Yeah, we have a large team of customer care executives ever active to handle your concerns promptly. To get in touch with them for our Python Assignment Help services, you don't require an appointment. You can use our active email, salient live chat feature, or free calls. To book our Python mentorship services, you are only required to follow these three explicit steps.
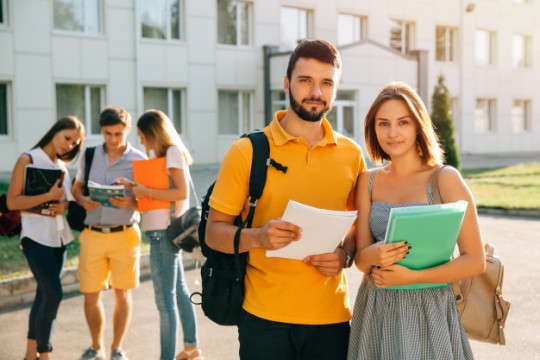
1. Login our official homepage at Programming Online Help and click the 'order now' link. Once provided with a unique form, kindly fills it with necessary details only.
2. Immediately after clicking the upload details button, you will receive an appropriate quote from our automated calculators. Initiate payment through pay pal, debit, or credit card.
3. Receive your requested support on time. Being the most renowned educational site, we never compromise on students' deadline.
0 notes