#Drag and Drop File Upload Using Dropzone
Explore tagged Tumblr posts
Text
HTML APIs

HTML APIs (Application Programming Interfaces) provide a way for developers to interact with web browsers to perform various tasks, such as manipulating documents, handling multimedia, or managing user input. These APIs are built into modern browsers and allow you to enhance the functionality of your web applications.
Here are some commonly used HTML APIs:
1. Geolocation API
Purpose: The Geolocation API allows you to retrieve the geographic location of the user’s device (with their permission).
Key Methods:
navigator.geolocation.getCurrentPosition(): Gets the current position of the user.
navigator.geolocation.watchPosition(): Tracks the user’s location as it changes.
Example: Getting the user’s current location.<button onclick="getLocation()">Get Location</button> <p id="location"></p><script> function getLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(showPosition); } else { document.getElementById('location').innerHTML = "Geolocation is not supported by this browser."; } } function showPosition(position) { document.getElementById('location').innerHTML = "Latitude: " + position.coords.latitude + "<br>Longitude: " + position.coords.longitude; } </script>
2. Canvas API
Purpose: The Canvas API allows for dynamic, scriptable rendering of 2D shapes and bitmap images. It’s useful for creating graphics, games, and visualizations.
Key Methods:
getContext('2d'): Returns a drawing context on the canvas, or null if the context identifier is not supported.
fillRect(x, y, width, height): Draws a filled rectangle.
clearRect(x, y, width, height): Clears the specified rectangular area, making it fully transparent.
Example: Drawing a rectangle on a canvas.<canvas id="myCanvas" width="200" height="100"></canvas><script> var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); ctx.fillStyle = "red"; ctx.fillRect(20, 20, 150, 100); </script>
3. Drag and Drop API
Purpose: The Drag and Drop API allows you to implement drag-and-drop functionality on web pages, which can be used for things like moving elements around or uploading files.
Key Methods:
draggable: An HTML attribute that makes an element draggable.
ondragstart: Event triggered when a drag operation starts.
ondrop: Event triggered when the dragged item is dropped.
Example: Simple drag and drop.<p>Drag the image into the box:</p> <img id="drag1" src="image.jpg" draggable="true" ondragstart="drag(event)" width="200"> <div id="dropzone" ondrop="drop(event)" ondragover="allowDrop(event)" style="width:350px;height:70px;padding:10px;border:1px solid #aaaaaa;"></div><script> function allowDrop(ev) { ev.preventDefault(); } function drag(ev) { ev.dataTransfer.setData("text", ev.target.id); } function drop(ev) { ev.preventDefault(); var data = ev.dataTransfer.getData("text"); ev.target.appendChild(document.getElementById(data)); } </script>
4. Web Storage API
Purpose: The Web Storage API allows you to store data in the browser for later use. It includes localStorage for persistent data and sessionStorage for data that is cleared when the page session ends.
Key Methods:
localStorage.setItem(key, value): Stores a key/value pair.
localStorage.getItem(key): Retrieves the value for a given key.
sessionStorage.setItem(key, value): Stores data for the duration of the page session.
Example: Storing and retrieving a value using localStorage.<button onclick="storeData()">Store Data</button> <button onclick="retrieveData()">Retrieve Data</button> <p id="output"></p><script> function storeData() { localStorage.setItem("name", "John Doe"); } function retrieveData() { var name = localStorage.getItem("name"); document.getElementById("output").innerHTML = name; } </script>
5. Fetch API
Purpose: The Fetch API provides a modern, promise-based interface for making HTTP requests. It replaces older techniques like XMLHttpRequest.
Key Methods:
fetch(url): Makes a network request to the specified URL and returns a promise that resolves to the response.
Example: Fetching data from an API.<button onclick="fetchData()">Fetch Data</button> <p id="data"></p><script> function fetchData() { fetch('https://jsonplaceholder.typicode.com/posts/1') .then(response => response.json()) .then(data => { document.getElementById('data').innerHTML = data.title; }); } </script>
6. Web Workers API
Purpose: The Web Workers API allows you to run scripts in background threads. This is useful for performing CPU-intensive tasks without blocking the user interface.
Key Methods:
new Worker('worker.js'): Creates a new web worker.
postMessage(data): Sends data to the worker.
onmessage: Event handler for receiving messages from the worker.
Example: Simple Web Worker.<script> if (window.Worker) { var myWorker = new Worker('worker.js'); myWorker.postMessage('Hello, worker!'); myWorker.onmessage = function(e) { document.getElementById('output').innerHTML = e.data; }; } </script> <p id="output"></p>
worker.js:onmessage = function(e) { postMessage('Worker says: ' + e.data); };
7. WebSocket API
Purpose: The WebSocket API allows for interactive communication sessions between the user’s browser and a server. This is useful for real-time applications like chat applications, live updates, etc.
Key Methods:
new WebSocket(url): Opens a WebSocket connection.
send(data): Sends data through the WebSocket connection.
onmessage: Event handler for receiving messages.
Example: Connecting to a WebSocket.<script> var socket = new WebSocket('wss://example.com/socket'); socket.onopen = function() { socket.send('Hello Server!'); }; socket.onmessage = function(event) { console.log('Message from server: ', event.data); }; </script>
8. Notifications API
Purpose: The Notifications API allows web applications to send notifications to the user, even when the web page is not in focus.
Key Methods:
Notification.requestPermission(): Requests permission from the user to send notifications.
new Notification(title, options): Creates and shows a notification.
Example: Sending a notification.<button onclick="sendNotification()">Notify Me</button><script> function sendNotification() { if (Notification.permission === 'granted') { new Notification('Hello! This is a notification.'); } else if (Notification.permission !== 'denied') { Notification.requestPermission().then(permission => { if (permission === 'granted') { new Notification('Hello! This is a notification.'); } }); } } </script>
HTML APIs allow you to build rich, interactive web applications by providing access to browser features and capabilities. These APIs are widely supported across modern browsers, making them a vital part of contemporary web development.
Read More…
0 notes
Text
Rotate Image Tool: Easily Rotate and Download Your Images
CLICK HERE to Use This POWERFUL TOOL If you're looking for an easy way to rotate your images, our Rotate Image Tool is the perfect solution. With this tool, you can easily upload an image, rotate it to your desired angle, and download the rotated image with just a few clicks. Why Rotate Your Images? Rotating your images can be important for a variety of reasons. For example, if you've taken a photo with your phone or camera and the image is sideways or upside down, rotating it can help you view the image in the correct orientation. Additionally, rotating an image can be useful if you want to create a specific aesthetic for your website or social media profile. Using the Rotate Image Tool Using our Rotate Image Tool is incredibly easy. Here's a step-by-step guide: - First, go to our website and navigate to the Rotate Image Tool page. - Next, either drag and drop an image into the dropzone or click the "Choose an image" button to select an image from your computer. - Once the image is uploaded, use the rotation angle slider to adjust the angle of the image. You can rotate the image clockwise or counterclockwise. - As you adjust the angle, you'll see a preview of the rotated image in the dropzone. - Once you're happy with the rotation angle, click the "Download" button to download the rotated image to your computer. It's really that simple! Our Rotate Image Tool is designed to be user-friendly and intuitive, so you can rotate your images with ease. ALSO SEE= Convert Base64 to Image – A Free and Easy-to-Use Tool Why Use Our Rotate Image Tool? There are a few key reasons why our Rotate Image Tool is the best tool for the job: - Ease of use: Our tool is designed to be incredibly user-friendly, so even if you're not tech-savvy, you can easily rotate your images with just a few clicks. - Compatibility: Our tool works with a wide variety of image file types, including JPG, PNG, and GIF. - No software required: Because our tool is online, you don't need to download any software or install any plugins to use it. Simply visit our website and you're ready to go! - Free to use: Our Rotate Image Tool is completely free to use, with no hidden costs or fees. SEO Benefits of Rotating Your Images In addition to the practical benefits of rotating your images, there are also some SEO benefits to consider. Here are a few reasons why you should rotate your images to improve your website's SEO: - Improved user experience: If you have images on your website that are sideways or upside down, it can be frustrating for users to have to tilt their heads or devices to view them properly. By rotating your images, you can improve the user experience and make your website more user-friendly. - Better page load times: If you have large images on your website that are oriented incorrectly, it can slow down your page load times. By rotating your images and ensuring that they're optimized for web use, you can improve your website's page load times and reduce bounce rates. - Improved image search rankings: If you're using images on your website as part of your SEO strategy, rotating them can help improve your search rankings. By optimizing your images for search engines, you can increase the likelihood that they'll show up in image search results and drive more traffic to your website. Rotate Image Settings In addition to the rotation angle slider, our Rotate Image Tool also includes a few additional settings to help you customize your image rotation experience: - Image size: You can adjust the size of the image preview in the dropzone to help you see the details of your image more clearly. - Background color: You can choose the background color of the dropzone to better match the aesthetic of your website or brand. - Reset button: If you make a mistake while rotating your image, you can click the "Reset" button to start over. These settings can help you customize your image rotation experience to better suit your needs. Using Our Rotate Image Tool for Social Media If you're using social media as part of your digital marketing strategy, our Rotate Image Tool can be incredibly useful. Here are a few ways you can use it: - Rotating images for Instagram: If you're using Instagram to promote your brand, you know how important it is to have eye-catching visuals. By using our Rotate Image Tool to rotate your images and create unique angles and perspectives, you can help your Instagram posts stand out from the crowd. - Rotating images for Pinterest: Pinterest is another platform that relies heavily on visuals. By rotating your images and creating unique compositions, you can help your pins get more attention and drive more traffic to your website. - Rotating images for Facebook: While Facebook isn't a visual-first platform like Instagram or Pinterest, it's still important to have high-quality images on your Facebook page. By rotating your images and ensuring that they're oriented correctly, you can help your Facebook page look more professional and polished. Conclusion Our Rotate Image Tool is a simple yet powerful tool that can help you easily rotate and download your images. Whether you're using it to improve the user experience on your website, optimize your images for SEO, or create eye-catching visuals for social media, our tool is designed to be user-friendly, customizable, and free to use. So why not give it a try today and see how it can help you take your images to the next level? Read the full article
0 notes
Text
CodeIgniter 4 Drag and Drop File Upload Using Dropzone
In many applications, you have been observed file upload process is bit attractive. Inside this article we will see the concept of CodeIgniter 4 drag and drop file upload using dropzone jquery plugin.
Dropzone.js is a jquery plugin, dropzone.js through we can select one by one image and also with preview.
0 notes
Link
The most well-known, free, and open-source library for drag-and-drop file uploads with image previews is Dropzone. I'll be using Laravel 9 in this example.
2 notes
·
View notes
Link
Drag and drop file upload is a very successful way to make your web application user-friendly. Generally, this feature allows you to drag an element and drop it to a different location on the web page. The drag and drop…
1 note
·
View note
Video
youtube
This video will show how you can use the Dropzone library to upload a file using drag & drop in the CodeIgniter project.
#codeigniter#mvc#framework#dropzone#javascript#library#file upload#drag and drop#html#view#controller#coding#programming#web development#makitweb#youtube
5 notes
·
View notes
Text
Laravel 9 Dropzone Image Upload Example Step By Step - CodeSolutionStuff
#artificial intelligence#Programming#php#cloud#machine learning#laravel#JavaScript#DataScience#MachineLearning#Analytics#AI#ML#angular#Tech#Python#ReactJS#DataScientist#Coding#SQL#bot#Cloud#Typescript#Github#Data#BigData#DL#machinelearning
0 notes
Text
Sookasa files unavailable offline network connection
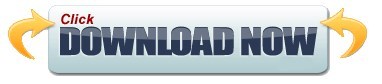
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION FOR MAC OS X#
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION FOR MAC#
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION SERIAL NUMBERS#
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION SERIAL KEY#
Optionally automatically uploads screenshots taken with OS X and copies . NMac Ked | Dropshare is your very own secure file sharing tool. Dropshare 4.7.1 Size: 12.46 MB Dropshare is your very own secure file-sharing tool. DShare503 Dropshare 5.0.3 | Mac OS X | 17 MB. sync, install, and manage fonts on your Mac or Dropbox/Google Drive. iMac Cleaner 2.0 Crack Mac Osx RightFont 5.3.2 (2319) Crack Mac Osx. Sookasa: Secure HIPAA compliant Dropbox file encryption tool. appgate-sdp-client, AppGate SDP Client for macOS, 5.0.3. VoodooPad 5.2.1 Crack MacOS MacOSX DOWNLOAD LINK: ✅. Serial 1.4.0 - Terminal emulation with support for almost every serial device. Mac iOS Dropbox 81.4.195 - Cloud storage. Mac blank VMware Fusion 11.5.0 - Run an alternative OS.
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION FOR MAC#
M4VGear DRM Media Converter 4.3.5 Torrent For Mac It will be perfect to play .
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION FOR MAC OS X#
M4VGear 4.3.8 + Crack Mac OS X for MAC OS X and iOS. Providers allowed: Dropshare introduces itself to the Mac pc OS X Services Menu. Download All Latest Crack Soft For Max Osx. DropShare is a program that will greatly assist in sharing info. Encore 5.0.3 + Keygen - Phần mềm soạn nhạc, viết nhạc Trong số. Flowerfire Sawmill Enterprise v8.5.0.1 keygen by AGAiN � Flowerfire Sawmill GVOX Encore v5.0.5 MAC OSX UB serial by. Encore all versions serial number and keygen, Encore serial number, Encore keygen. 5.0.4 hidden network DropBox 10.10.2 stable Drive Genius 5.0.4 crack dutch .
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION SERIAL KEY#
5.0.3 Full Version For (Mac OS X) 5.0.4 Crack + Serial Key For MacOS. Little Snitch 4.0.3 Multilingual Mac OS X Crack Torrent: Little Snitch 4. Download the latest versions of the best Mac apps at safe and trusted MacUpdate. with of Microsoft OneDrive, Google Drive File Stream and Dropbox placeholder information. hard disk to save your backed up data, it stores your system backup along with the OS machine. Carbon Copy Cloner 5.0.3 Serial key features. Telestream ScreenFlow® is award-winning, powerful video editing and screen recording software for Mac that lets you create high-quality software or iPhone .
Share directly to iCloud Drive, Dropbox, Google Drive and ZipShare, from within .
Protect files with banking-level AES encryption.
MindNode 5.0 Full Crack With Activation Code Download: MindNode. dropshare backblazeĭropshare 5.0.3 freeload For Mac Mac. Compatibility: OS X 10.10 or later 64-bit. Dropshare is a menu bar application for drag & drop upload files, screenshots and even. Kruptos 2 Professional is an easy to use file encryption program that uses 256-bit encryption . File encryption for the Mac OSX, Windows and Android. dropshareĭropshare, dropshare cloud, dropshare alternative, dropshare windows, dropshare review, dropshare vs dropzone, dropshare mac review, dropshare login, dropshare github, dropshare backblazeĭownload Crack MacBooster 5.0.3 Crack For Mac freeload MacBooster 5.0.3 Serial Key is a versatile application that allows you to .
#SOOKASA FILES UNAVAILABLE OFFLINE NETWORK CONNECTION SERIAL NUMBERS#
hosts now works as expected Fixed a bug with duplicate serial numbers in a network. Localhost can be saved to Dropbox even if it's the only host. The application no longer crashes when creating a WordPress host on OS X 10.11. Just link your account and you're all set up. Title: Transmit 5.0.3 MAC OS X Developer. with the help of this additional features you can sync. aurora Aurora 5.0.3 aurora-hdr Aurora HDR 1. FxFactory Pro 5 Crack Mac OSX incl Serial Key is latest pro manager of.
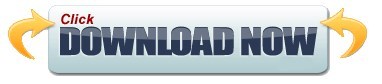
0 notes
Text
React dropzone
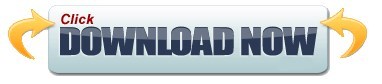
React dropzone install#
There are 2762 other projects in the npm registry using react-dropzone. Start using react-dropzone in your project by running npm i react-dropzone. Latest version: 14.2.2, last published: a month ago. In this step, you will be installing html5-file-selector, a wrapper library that offers profound support for handling html5 drag/drop and file input file and folder selections in react. Simple HTML5 drag-drop zone with React.js. Starter project for React apps that exports to the.
React dropzone install#
npm install react-dropzone-uploader Add html5-file-selector Library. Browse StackBlitz projects using react-dropzone, crack open the code and start editing in your browser. Note (and this is almost more important as we don't need to react to drop events): The same behaviour can be observed when the file picker dialog opens, it will allow picking other image extensions, not just jpeg and png. Now, you can build a file input with a clickable dropzone that accepts image, audio, and video files. When I use accept: application/zip with Dropzone. The following code will let users drop any image type and the jpeg/png file extensions are ignored. npm install -save react We can install additional packages using npm install - save and the. Offer an intuitive interface for your users to upload files into your app with this customizable React Drop Zone component. zip -Content-Encoding: gzip -Content-Length: 327. react-native python fastapi http-status-code-422. Using a wildcard for image/* and then declaring file extensions doesn't seem to work as expected in Firefox and Brave/Chrome. In this tutorial you are going to learn how create a file dropzone component from scratch using react. React-dropzone-uploader : 422 Unprocessable Entity with fastapi upload.
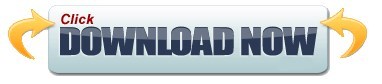
0 notes
Text
Dropzone app
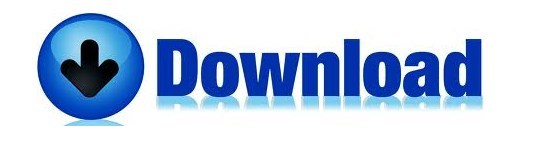
Dropzone app apk#
Dropzone app install#
Right now, our code should be looking something like this in our App.js component.
Dropzone app install#
We will install our React dropzone with npm install React-dropzone or yarn add React-dropzone. If you need a visual explanation of a drag-and-drop with React-dropzone, you can watch this video. Dropzone provides additional functions such as customizing the dropzone, restricting file types, etc. It’s a simple React hook to create an HTML-5 compliant drag-and-drop zone for files. React-dropzone is a very powerful library and custom component. Instead, we’ll use one of the most common React drag-and-drop libraries available: React-dropzone. We won’t be building all the logic and components from scratch. It's meant to look good by default, and is highly customizable. It is free, fully open source, and makes it easy for you to handle dropped files on your website. How to Implement a Drag and Drop Zone in React File uploads made easy Dropzone.js is one of the most popular drag and drop JavaScript libraries.
all logo files (logo192.png, logo512.png, logo.svg)Īlternatively, you could run npx clean-React-app to remove such files.
You can manually delete some of the generated boilerplate files and code, such as: We are creating our React app from our terminal using npx create-react-app drag-n-drop for those using npm, while others using yarn should run yarn create React-app drag-n-drop.Īfter, run npm start or yarn start to begin. I believe we already know this process, but we will start from scratch here, for starters.
Indicate the file received on the drop zone,įirst of all, we are going to create our React app.
Alternative option ‘click to open’ button,.
List file name, file type, and file size,.
The script ingests the SendSafely generated secure link & related metadata, and.
Click event which initiates file selection dialog The Google Apps Script bridges the SendSafely Dropzone and the Zendesk platform.
Drag-and-drop a file on the drag-and-drop zone,.
We’ll create a simple React application with a drag-and-drop interface, just like the one below.īy the end of this tutorial, in our React application we’ll be able to do the following: The most common use cases for drag-and-drop in React comprise uploading files, rearranging images/files, and moving files between multiple folders. React-dropzone is a set of React libraries to help you build complex drag-and-drop interfaces while keeping your components destructured.
Dropzone app apk#
The user selects a draggable item with a mouse or touchpad, drags those files to a droppable element (drop zone), and drops them by releasing the mouse button. Download DropZone APK 1.0 (freeload) - Drop Zone APK - Mobile App for Android - com.dropzoneapp - DROPZONE LOGISTICS - Latest Version 2022 - Updated. Drag and drop is a platform whereby an application uses drag-and-drop features on browsers.
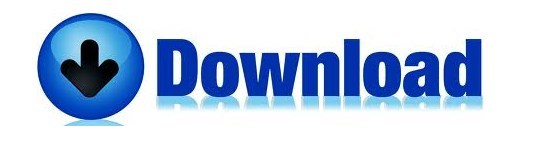
0 notes
Text
Laravel 9 Dropzone Image Upload Example Step By Step
New Post has been published on https://www.codesolutionstuff.com/laravel-9-dropzone-image-upload-example-step-by-step/
Laravel 9 Dropzone Image Upload Example Step By Step
The most well-known, free, and open-source library for drag-and-drop file uploads with image previews is Dropzone. I'll be using Laravel 9 in this example. Laravel Dropzone Image Upload To begin, use dropzone to upload several photos.Adding photos to the database with alternative file
#implement dropzone.js laravel#laravel dropzone example#laravel dropzone file upload#laravel dropzone image upload#laravel dropzone multiple files
0 notes
Text
Laravel 9 Dropzone Image Upload Example Step By Step
The most well-known, free, and open-source library for drag-and-drop file uploads with image previews is Dropzone. I'll be using Laravel 9 in this example.
Laravel Dropzone Image Upload
- To begin, use dropzone to upload several photos. - Adding photos to the database with alternative file names. - Removing photos from the preview box of the dropzone.
Step 1: Download Laravel Project
Type the following command to create a Laravel project. composer create-project --prefer-dist laravel/laravel dropzonefileupload
Step 2: Set up a MySQL database
//.env DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=*********** DB_USERNAME=root DB_PASSWORD=
Step 3: Compose a model and migration file
In your cmd, type the following command. php artisan make:model ImageUpload -m There will be two files created. - ImageUpload.php model. - create_image_uploads_table migration file. For the image upload table, we'll need to develop a Schema. Go to Laravel >> database >> migrations >> create_image_uploads_table to get started. //create_image_uploads_table public function up() { Schema::create('image_uploads', function (Blueprint $table) { $table->increments('id'); $table->text('filename'); $table->timestamps(); }); }
Step 4: Create a view file
Create an imageupload.blade.php file in resources >> views >> imageupload.php. Put the code below in there. We'll add a dropzone for file uploading in this file. Laravel Multiple Images Upload Using Dropzone Laravel Multiple Images Upload Using Dropzone @csrf First, we'll include our bootstrap.min.css and dropzone.min.css files in this file. After that, we'll include jquery.js and dropzone.js. After that, we'll make a form and add the dropzone class to it. In addition, we have some text in our upload box. Also, if the image is successfully uploaded, it will display a tick otherwise it displays a cross and error.
Step 5: Configure Dropzone
Now we'll write all of the Dropzone setups. So, in a view file, add the following code. Laravel Multiple Images Upload Using Dropzone Laravel Multiple Images Upload Using Dropzone @csrf We're adding Dropzone setup options to the file above. Any of the setting options are documented in the Dropzone Documentation Let's take a look at each choice one by one. - maxFilesize is set to 12 by default. Dropzone will only accept photos that are smaller than 12MB in size. You can make it smaller or larger depending on your needs. - Before the file is uploaded to the server, the renameFile function is called, which renames the file. - acceptedFiles compares the mime type or extension of the file to this list. The terms.jpeg,.jpg,.png, and.gif are defined. You have the option to alter according on your requirements. - The value of addRemoveLinks is set to true. Dropzone will show the Remove button, which we may use to delete the file we just uploaded. - The timeout is set to 5000 seconds.
Step 6: Create one controller and route
php artisan make:controller ImageUploadController ImageUploadController.php will be created, and we'll register routes in the routes >> web.php file. So let's get started. //web.php Route::get('image/upload','ImageUploadController@fileCreate'); Route::post('image/upload/store','ImageUploadController@fileStore'); Route::post('image/delete','ImageUploadController@fileDestroy'); The next step is to add some code to the fileCreate() function in the ImageUploadController.php file. // ImageUploadController.php public function fileCreate() { return view('imageupload'); } We are simply returning the imageupload that we have made in the create() method.
Step 7: Save File into Database
To store the filename in the database, we must code the fileStore() procedure in sequence. // ImageUploadController.php use AppImageUpload; public function fileStore(Request $request) { $image = $request->file('file'); $imageName = $image->getClientOriginalName(); $image->move(public_path('images'),$imageName); $imageUpload = new ImageUpload(); $imageUpload->filename = $imageName; $imageUpload->save(); return response()->json(); }
Step 8: Remove File From Database
The removedFile() function has now been added to the dropzone configuration. Laravel Multiple Images Upload Using Dropzone Laravel Multiple Images Upload Using Dropzone @csrf To delete a file from the database, add the fileDestroy() function. In FileUploadController, add the following code. //ImageUploadController.php Read the full article
#implementdropzone.jslaravel#laraveldropzoneexample#laraveldropzonefileupload#laraveldropzoneimageupload#laraveldropzonemultiplefiles
0 notes
Text
Dropzone.js prevents Flask from rendering template
I am using Dropzone.js to allow drag and drop upload of CSV files via a Flask web site. The upload process works great. I save the uploaded file to my specified folder and can then use df.to_html() to convert the dataframe into HTML code, which I then pass to my template. It gets to that point in the code, but it doesn't render the template and no errors are thrown. So my question is why is Dropzone.js preventing the render from happening?
I have also tried just return the HTML code from the table and not using render_template, but this also does not work.
init.py
import osfrom flask import Flask, render_template, requestimport pandas as pdapp = Flask(__name__)# get the current folderAPP_ROOT = os.path.dirname(os.path.abspath(__file__))@app.route('/')def index(): return render_template('upload1.html')@app.route('/upload', methods=['POST'])def upload(): # set the target save path target = os.path.join(APP_ROOT, 'uploads/') # loop over files since we allow multiple files for file in request.files.getlist("file"): # get the filename filename = file.filename # combine filename and path destination = "/".join([target, filename]) # save the file file.save(destination) #upload the file df = pd.read_csv(destination) table += df.to_html() return render_template('complete.html', table=table)if __name__ == '__main__': app.run(port=4555, debug=True)
upload1.html
<!DOCTYPE html><meta charset="utf-8"><script src="https://rawgit.com/enyo/dropzone/master/dist/dropzone.js"></script><link rel="stylesheet" href="https://rawgit.com/enyo/dropzone/master/dist/dropzone.css"><table width="500"> <tr> <td> <form action="{{ url_for('upload') }}", method="POST" class="dropzone"></form> </td> </tr></table>
EDIT
Here is the sample csv data I am uploading:
Person,CountA,10B,12C,13
Complete.html
<html><body>{{table | safe }}</body></html>
https://codehunter.cc/a/flask/dropzone-js-prevents-flask-from-rendering-template
0 notes
Text
#websolutionstuff#laravel#laravel9#laravel8#laravel7#php#laravel6#example#jquery#bootstrap#html#file upload#drag and drop#dropzone js
0 notes
Text
#techsolutionstuff#laravel#laravel6#laravel7#laravel 8#php#example#jquery#bootstrap#html#drag and drop#file upload#dropzone js
0 notes
Link
Drag and drop file upload is a very successful way to make your web application user-friendly. Generally, this feature allows you to drag an element and drop it to a different location on the web page. The drag and drop function can be used for a number of purposes in the web application. Drag & Drop is the best choice if you want to make file upload features more interactive. DropzoneJS is an open-source JavaScript library that enables a drop-down HTML feature. This means that the user can drag and…
0 notes