#255-11 error code
Explore tagged Tumblr posts
Text
Circuit #3 & #4 ❤️💚💙💛
This weeks goal was to build circuit 3 & 4. Then try to create a new circuit/code with a by combining it with a previous build/code. I first built circuit 3, RGB LED. I was glad it was successful! I decided to try it with the potentiometer to see if I could adjust the brightness and/or manipulate which light comes on as I turned the knob.
This is circuit #3...I thought the pictured I got of just the blue light was cool!


youtube
I then decided to try it with the potentiometer. This took a few tries (ok more than a few) 😆

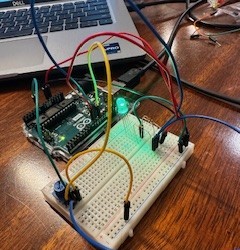
youtube
Here is the code that I used. Honestly, I was shocked it worked!
const int RED_PIN = 9;
const int GREEN_PIN = 10;
const int BLUE_PIN = 11;
const int POT_PIN = 0; // Potentiometer connected to analog pin 0
void setup() {
pinMode(RED_PIN, OUTPUT);
pinMode(GREEN_PIN, OUTPUT);
pinMode(BLUE_PIN, OUTPUT);
}
void loop() {
int potValue = analogRead(POT_PIN); // Read potentiometer value (0-1023)
// Map potentiometer value to color values (0-255)
int redValue = map(potValue, 0, 1023, 0, 255);
int greenValue = map(potValue, 0, 1023, 255, 0); // Inverted for color variation
int blueValue = map(potValue, 0, 1023, 0, 255);
// Set RGB LED color
analogWrite(RED_PIN, redValue);
analogWrite(GREEN_PIN, greenValue);
analogWrite(BLUE_PIN, blueValue);
delay(10); // Short delay for smoother transitions
}
Circuit #4 was quite the build! This required multiple LEDs, resistors, and wires to be placed to make the lights run. It was really fun to watch though. It made me think of the all the construction lights.


youtube
This week really challenged my mind and understanding. It was fun to be able to build both circuits and then see what I could get it to do with "splicing" it with another build/code. Honestly, it was just playing around though trial and error. The actual coding part still intimidates me! 😬
0 notes
Text
How to check Email and username availability live using jquery/ajax, PHP and PDO
In this tutorial, We will learn how to How to check Email and username availability live using jQuery/ajax and PHP-PDO.
Click : https://phpgurukul.com/how-to-check-email-and-username-availability-live-using-jquery-ajax-php-and-pdo/
File Structure for this tutorials
index.php (Main File)
config.php (Database Connection file)
check_availability.php (Used to check the Email and User availability)
Create a database with name demos. In demos database, create a table with name email_availabilty Sample structure of table email_availabilty
CREATE TABLE IF NOT EXISTS `email_availabilty` (
`id` int(11) NOT NULL,
`email` varchar(255) NOT NULL,
`username` varchar(255) NOT NULL
) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=latin1;
2. Create a database connection file
config.php
<?php
//DB Connection
define(‘DB_HOST’,’localhost’);
define(‘DB_USER’,’root’);
define(‘DB_PASS’,’’);
define(‘DB_NAME’,’demos’);
// Establish database connection.
try
{
$dbh = new PDO(“mysql:host=”.DB_HOST.”;dbname=”.DB_NAME,DB_USER, DB_PASS,array(PDO::MYSQL_ATTR_INIT_COMMAND => “SET NAMES ‘utf8’”));
}
catch (PDOException $e)
{
exit(“Error: “ . $e->getMessage());
}
3. Now Create an HTML form index.php
<?php
include_once(“config.php”);
?>
<table>
<tr>
<th width=”24%” height=”46" scope=”row”>Email Id :</th>
<td width=”71%” ><input type=”email” name=”email” id=”emailid” onBlur=”checkemailAvailability()” value=”” class=”form-control” required /></td>
</tr>
<tr>
<th width=”24%” scope=”row”></th>
<td > <span id=”email-availability-status”></span> </td>
</tr>
<tr>
<th height=”42" scope=”row”>User Name</th>
<td><input type=”text” name=”username” id=”username” value=”” onBlur=”checkusernameAvailability()” class=”form-control” required /></td>
</tr>
<tr>
<th width=”24%” scope=”row”></th>
<td > <span id=”username-availability-status”></span> </td>
</tr>
</table>
4. Jquery/ajax script where you pass variable to check_availability.php page. put this in index.php inside head.
<script>
function checkemailAvailability() {
$(“#loaderIcon”).show();
jQuery.ajax({
url: “check_availability.php”,
data:’emailid=’+$(“#emailid”).val(),
type: “POST”,
success:function(data){
$(“#email-availability-status”).html(data);
$(“#loaderIcon”).hide();
},
error:function (){}
});
}
function checkusernameAvailability() {
$(“#loaderIcon”).show();
jQuery.ajax({
url: “check_availability.php”,
data:’username=’+$(“#username”).val(),
type: “POST”,
success:function(data){
$(“#username-availability-status”).html(data);
$(“#loaderIcon”).hide();
},
error:function (){}
});
}
</script>
5.check_availability.php page in this page you will check the availability of email or email.
<?php
require_once(“config.php”);
//code check email
if(!empty($_POST[“emailid”])) {
$uemail=$_POST[“emailid”];
$sql =”SELECT email FROM email_availabilty WHERE email=:email”;
$query= $dbh -> prepare($sql);
$query-> bindParam(‘:email’, $uemail, PDO::PARAM_STR);
$query-> execute();
$results = $query -> fetchAll(PDO::FETCH_OBJ);
if($query -> rowCount() > 0)
echo “<span style=’color:red’> Email Already Exit .</span>”;
else
echo “<span style=’color:green’> Email Available.</span>”;
}
// End code check email
//Code check user name
if(!empty($_POST[“username”])) {
$username=$_POST[“username”];
$sql =”SELECT username FROM email_availabilty WHERE username=:username”;
$query= $dbh -> prepare($sql);
$query-> bindParam(‘:username’, $username, PDO::PARAM_STR);
$query-> execute();
$results = $query -> fetchAll(PDO::FETCH_OBJ);
if($query -> rowCount() > 0)
echo “<span style=’color:red’> Username already exit .</span>”;
else
echo “<span style=’color:green’> Username Available.</span>”;
}
// End code check username
?>
PHP Gurukul
Welcome to PHPGurukul. We are a web development team striving our best to provide you with an unusual experience with PHP. Some technologies never fade, and PHP is one of them. From the time it has been introduced, the demand for PHP Projects and PHP developers is growing since 1994. We are here to make your PHP journey more exciting and useful.
Website : https://phpgurukul.com
0 notes
Photo
How to Connect PHP to MySQL
Do you want ot learn how to connect php to MySQL and perform different types of database operations? In this article, we'll do just that—we'll discuss MySQL database connectivity in PHP.
PHP provides different ways to connect PHP to a MySQL database server. Until PHP 5.5, one of the most popular ways was with the MySQL extension—it provided a "procedural" way to connect to the MySQL server. However, this extension is deprecated as of PHP 5.5, so we’re not going to discuss that.
The current two ways you can choose from are PDO and MySQLi. The PDO (PHP Data Objects) extension supports different types of underlying database servers along with the MySQL server. And thus, it’s portable in case you decide to swap the underlying database server at any point in the future. On the other hand, the MySQLi extension is specific to the MySQL server and provides better speed and performance. The MySQLi extension might also be a little simpler to understand at first, since it lets you write SQL queries directly. I've you've worked with SQL databases before, this be very familiar. On the other hand, the PDO extension creates a powerful mapping from the SQL database to your PHP code and lets you do a lot of database operations without needing to know the details of SQL or the database. In the long run and for larger projects, this can save a lot of coding and debugging effort.
In this post, I'll use the MySQLi extension. But you can learn more about the PDO extension here on Envato Tuts+.
PHP
Quickly Build a PHP CRUD Interface With the PDO Advanced CRUD Generator Tool
Sajal Soni
We’ll cover the following topics in this article:
creating a MySQL connection
inserting, updating and deleting Records
retrieving records
Best PHP Database Scripts on CodeCanyon
In this post, I'll be showing you how to make a bare-metal connection to a MySQL database from PHP. This is an important skill to have, but if you want to save time on your next project, you might want to upgrade to a professional database script. This will save time and make your work easier.
Explore the best and most useful PHP database scripts ever created on CodeCanyon. With a low-cost one time payment, you can purchase these high-quality WordPress themes and improve your website experience for you and your visitors.
Here are some of the best PHP database scripts available on CodeCanyon for 2020.
PHP
Comparing PHP Database Abstraction Layers and CRUD Plugins
Sajal Soni
Create a MySQL Connection
In this section, I'll show you how you can connect to the MySQL server from your PHP script and create a connection object. Later on, this connection object will be used to run queries, fetch output and manipulate the database records. As we discussed earlier, we’re going to use the PHP MySQLi extension for this article.
The MySQLi extension provides two different ways to perform database operations. You can use it either the object-oriented way or the procedural way, but as this tutorial is aimed at beginners, I'll stick with the procedural way. If you're interested in seeing some object oriented snippets, you can ping me in the comment section below and I’ll be happy to provide them.
To start, go ahead and create the db_connect.php file under your document root with the following contents.
<?php $mysqli_link = mysqli_connect("{HOST_NAME}", "{DATABASE_USERNAME}", "{DATABASE_PASSWORD}", "{DATABASE_NAME}"); if (mysqli_connect_errno()) { printf("MySQL connection failed with the error: %s", mysqli_connect_error()); exit; }
Note that I've used placeholders in the mysqli_connect function—you have to replace these with actual values.
Let’s go through the each placeholder to see what it stands for.
{HOST_NAME}: This represents your MySQL server’s host-name or IP address. If you have installed the MySQL server on the same system along with PHP, you should use localhost or 127.0.0.1. On the other hand, if you’re using a MySQL server which is hosted externally, you can use the corresponding host-name or IP address.
{DATABASE_USERNAME}: This represents the username of your MySQL user. Basically, this is the username which you use to connect to your MySQL server.
{DATABASE_PASSWORD}: This represents the password of your MySQL user. Again, this is the password which you use to connect to your MySQL server along with the MySQL username.
{DATABASE_NAME}: This is a name of the MySQL database which you want to connect to. Once the connection is created, you'll query this database for further operations.
Go ahead and replace these placeholders with actual values. In my case, I’ve installed the MySQL server locally, and I’ve a MySQL user with tutsplus-demo-user as the MySQL username and tutsplus-demo-password as the MySQL password. And with that, the above example looks like this:
<?php $mysqli_link = mysqli_connect("localhost", "tutsplus-demo-user", "tutsplus-demo-password", "tutsplus-demo-database"); if (mysqli_connect_errno()) { printf("MySQL connection failed with the error: %s", mysqli_connect_error()); exit; }
If you run this example, it should create a database connection link and assign it to the $mysqli_link variable, which we’ll use later on to perform different types of database operations.
On the other hand, if there’s any problem setting up the connection, the mysqli_connect_errno function will return an error code and the mysqli_connect_error function will display the actual error. In the above example, we’ve used it for debugging purposes.
Now, we’ve a successful connection to the MySQL server and we’ll see how to use it to perform different types of queries next section on wards.
How to Insert Records
In the previous section, we discussed how to use the mysqli_connect function to setup a database connection with the MySQL server. In this section, we’ll go ahead and discuss how to use the connection object to perform the INSERT queries.
If you want to follow along with the examples discussed in this article, you'll need to create the following MySQL table in your database. It's the table which we're going to use in all the examples from now on.
CREATE TABLE `students` ( `id` int(11) NOT NULL AUTO_INCREMENT, `first_name` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL, `last_name` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL, `email` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
Go ahead and create the above MySQL table by using the phpMyAdmin software or command line tool.
Next, let’s create the db_insert.php file with the following contents. Please don’t forget to replace the connection parameters with your own.
<?php $mysqli_link = mysqli_connect("localhost", "tutsplus-demo-user", "tutsplus-demo-password", "tutsplus-demo-database"); if (mysqli_connect_errno()) { printf("MySQL connection failed with the error: %s", mysqli_connect_error()); exit; } $insert_query = "INSERT INTO students(`first_name`,`last_name`,`email`) VALUES ('". mysqli_real_escape_string($mysqli_link, 'John') ."','". mysqli_real_escape_string($mysqli_link, 'Wood') ."','". mysqli_real_escape_string($mysqli_link, '[email protected]') ."')"; // run the insert query If (mysqli_query($mysqli_link, $insert_query)) { echo 'Record inserted successfully.'; } // close the db connection mysqli_close($mysqli_link); ?>
First, we set up a database connection by using the mysqli_connect function as we discussed earlier. After that, we’ve prepared the insert query which we’re going to execute later on. It's important to note that we've used the mysqli_real_escape_string function to escape string values that we’re going to use in the insert query. Specifically, you must use this function when you’re dealing with values submitted via $_POST variables to avoid SQL injection.
Finally, we’ve used the mysqli_query function, which takes two arguments. The first argument is the active connection link where the query will be executed. And the second argument is the MySQL query which we want to execute. The mysqli_query function returns TRUE if the query was executed successfully.
Finally, we’ve used the mysqli_close function to close the active database connection. It’s a good practice to close the database connection once you’re done with database operations.
Go ahead and run the script, and that should insert a record in the students table!
How to Update Records
Updating the records in a database from the PHP script is very similar to the insert operation with the only difference is that the query is going to be the update query instead of the insert query.
Let’s revise the above example and update the first_name field as shown in the following example.
<?php $mysqli_link = mysqli_connect("localhost", "tutsplus-demo-user", "tutsplus-demo-password", "tutsplus-demo-database"); if (mysqli_connect_errno()) { printf("MySQL connection failed with the error: %s", mysqli_connect_error()); exit; } $update_query = "UPDATE students SET `first_name` = '". mysqli_real_escape_string($mysqli_link,'Johnny') ."' WHERE `email` = '[email protected]'"; // run the update query If (mysqli_query($mysqli_link, $update_query)) { echo 'Record updated successfully.'; } // close the db connection mysqli_close($mysqli_link); ?>
Go ahead and run the script, and that should update the record in the students table.
How to Select Records
In the earlier sections, we discussed how you can insert and update records in a database from the PHP script. In this section, we’ll explore how you can fetch records from a database by using the different types of MySQLi functions.
Firstly, you need to use the mysqli_query function to execute the select query. Upon successful execution of the select query, the mysqli_query function returns the mysqli result object which we could use to iterate over the records returned by the select query. When it comes to fetching and iterating over the records from the MySQLi result object, there are different functions available.
mysqli_fetch_all: It allows you to fetch all result rows at once. You can also specify whether you want results as an associative array, a numeric array or both.
mysqli_fetch_array: It allows you to retrieve one row at a time. And thus, you’ll have to use the while loop to iterate over all the records. Again, you can specify whether you want a result row as an associative array, a numeric array or both.
mysqli_fetch_assoc: It fetches a result row one at a time as an associate array.
mysqli_fetch_object: It fetches a result row one at a time as an object.
Let’s have a look at the following example to understand how it works:
<?php $mysqli_link = mysqli_connect("localhost", "tutsplus-demo-user", "tutsplus-demo-password", "tutsplus-demo-database"); if (mysqli_connect_errno()) { printf("MySQL connection failed with the error: %s", mysqli_connect_error()); exit; } $select_query = "SELECT * FROM students LIMIT 10"; $result = mysqli_query($mysqli_link, $select_query); while ($row = mysqli_fetch_array($result, MYSQLI_ASSOC)) { echo "First Name:" . $row['first_name'] . "<br/>"; echo "Last Name:" . $row['last_name'] . "<br/>"; echo "Email:" . $row['email'] . "<br/>"; echo "<br/>"; } // close the db connection mysqli_close($mysqli_link); ?>
As you can see, we’ve used the mysqli_fetch_array function with the MYSQLI_ASSOC option as a second argument. And thus, it returns the result row as an associate array. Had you would have used the MYSQLI_NUM option, you would have accessed it like $row[0], $row[1] and $row[2] in the above example.
On the other hand, if you would use the mysqli_fetch_object function in the above example, you can access the values as shown in the following snippet. For brevity, I’ll only include the while loop snippet.
… … while ($row = mysqli_fetch_object($result)) { echo "First Name:" . $row->first_name . "<br/>"; echo "Last Name:" . $row->last_name . "<br/>"; echo "Email:" . $row->email . "<br/>"; echo "<br/>"; } ... ...
So in this way, you can fetch and display records from the MySQLi database.
How to Delete Records
In this section, we’ll see how to run delete queries from the PHP script. Deleting records from a database is a pretty straightforward operation since you just need to prepare the delete query and run it with the mysqli_query function.
Let’s go through the following example to see how it works.
<?php $mysqli_link = mysqli_connect("localhost", "tutsplus-demo-user", "tutsplus-demo-password", "tutsplus-demo-database"); if (mysqli_connect_errno()) { printf("MySQL connection failed with the error: %s", mysqli_connect_error()); exit; } $delete_query = "DELETE FROM students WHERE `email` = '[email protected]'"; // run the update query If (mysqli_query($mysqli_link, $delete_query)) { echo 'Record deleted successfully.'; } // close the db connection mysqli_close($mysqli_link); ?>
As you can see, everything is the same as we’ve seen it already with insert and update examples except that the query is a delete query in this case.
So that's how you can perform different types of database operations from the PHP script.
And with that, we’ve reached the end of this tutorial. What we’ve discussed so far today should help you to strengthen database connectivity concepts, specifically how to connect PHP to MySQL and perform different types of operations.
Conclusion
In this article, we discussed how to connect PHP to MySQL database by using the MySQLi extension. From setting up a database connection to executing different types of queries, we discussed almost every aspect of the database connectivity.
Although I’ve tried to keep things as simple as possible, if you still find anything confusing or want to know any specific thing in detail, feel free to post your queries using the feed below.
The Best PHP Scripts on CodeCanyon
Explore thousands of the best and most useful PHP scripts ever created on CodeCanyon. With a low-cost one time payment, you can purchase these high-quality WordPress themes and improve your website experience for you and your visitors.
Here are a few of the best-selling and up-and-coming PHP scripts available on CodeCanyon for 2020.
PHP
14 Best PHP Event Calendar and Booking Scripts
Kyle Sloka-Frey
PHP
10 Best PHP URL Shortener Scripts
Monty Shokeen
PHP
12 Best Contact Form PHP Scripts
Nona Blackman
PHP
Comparing the 5 Best PHP Form Builders
Nona Blackman
PHP
Create Beautiful Forms With PHP Form Builder
Ashraff Hathibelagal
by Sajal Soni via Envato Tuts+ Code https://ift.tt/2HcGa0z
0 notes
Link
Global debt has surpassed $250 trillion. That’s 320% of gross domestic product, announced the Institute of International Finance on Nov. 14. Emerging market debt also hit a record $71.4 trillion, 220% of GDP.
This has set off alarm bells. After all, the projected year-end amount of $255 trillion is equal to $32,500 for each person on the planet, or $12.1 million per Bitcoin (BTC), as Cointelegraph reported.
But, as mind-boggling as it may appear, is this total debt really so bad? Debt, after all, can stimulate growth, enabling a country to build roads, bridges, canals and universities, as well as pay pensions and ensure a higher well-being of its nation. It can raise a nation’s standard of living. IIF spokesperson Dylan Riddle told Cointelegraph:
“That level of debt is a point of concern because it represents a massive accumulation of debt in the last decade. Since the last recession, the world has added about $75 trillion worth of debt.”
However, things may not be so dire. As William D. Lastrapes, professor of economics at the University of Georgia, told Cointelegraph:
“It is difficult to say if global debt of $255 trillion is too much. It depends on which countries contribute to this — seems to be mostly US and China — and many, many other factors.”
The challenge with regard to debt is to know how much is too much. Economists have been battling over this point for years, usually in terms of the debt-to-GDP ratio. A highly influential paper earlier this decade placed the tipping point at 90% — that is, a country’s economic growth drops significantly when the size of its debt rises above 90% of its gross domestic product.
The 2010 paper “Growth in a Time of Debt,” by Carmen Reinhart and Kenneth Rogoff, was published around the time Greece’s economy was floundering, and the paper’s conclusion reportedly spurred public and quasi public officials — including those at the International Monetary Fund — to flip from stimulative to austerity in their response to Greece’s debt woes.
The paper also drew scathing attacks, most prominently from economist and Nobel laureate Paul Krugman, who censured the authors’ “coding” error, deplored the “austerity mania” that their paper unleashed among policymakers, and chided them for confusing correlation with causation. On the subject, Lastrapes noted to Cointelegraph:
“Public debt is not intrinsically a bad thing. It allows governments to separate the ‘timing’ of its expenditures from the ‘timing’ of its tax revenues. Without the ability to borrow, governments would be able to spend only as taxes are collected, which is in general not optimal (for example, think of public investment in infrastructure).”
Conversely, a low debt ratio is no guarantee of a healthy economy. As Lastrapes has noted elsewhere, Venezuela’s sovereign debt was only 23% of its GDP in 2017, yet its economy has been in turmoil for several years. Still, some in the crypto community have been skeptical about an imminent economic collapse. Vinny Lingham, CEO of blockchain identity platform Civic, told Cointelegraph:
“We’re way past the point where the bubble should have burst. It should have happened long ago. We’re in uncharted territory now.”
With the Institute of International Finance’s global debt ratio now at 320% — well beyond Rogoff’s 90% “tipping point” — and no apparent signs of Armageddon’s arrival, it appears the debt-ratio model might be flawed, Lingham suggested. Therefore, a new debt model that reflects reality may now be needed. In any case, he believes some intellectual humility is called for. As Lingham said, “Things have become unpredictable.”
It seems hard to believe, though, that living on borrowed money won’t catch up with everyone eventually. As the IIF noted in its Nov. 14 commentary, “With limited room for further monetary easing, debt service costs will be an increasing constraint on fiscal policy.” Lastrapes sees it another way. He told Cointelegraph:
“Government debt becomes problematic when it appears likely (in the eyes of bondholders) that future tax collections will be insufficient for the debt to be paid back. Debt default is the ultimate sign of fiscal irresponsibility and will harm a defaulting nation’s ability to borrow in the future.”
For a country like the United States, which is servicing its debt and has sustained growth as well as strong economic and fiscal institutions, large amounts of debt are not necessarily a problem, Lastrapes added. The fact that today’s interest rates and treasury yields remain low reinforces this view.
But the U.S. situation does not necessarily reflect that of the developing world. Countries like Argentina, Turkey and Iran may not be able to service their debt, and will consequently be blocked from investing in infrastructure, education, health and other needs.
Would Bitcoin soar if the bubble bursts?
The global debt mountain referenced by the IIF drew the attention of the crypto community as well. The assumption of some is that the price of Bitcoin and other cryptocurrencies could soar if and when the debt bubble bursts. As Erik Voorhees said on an earlier occasion with respect to U.S. debt and a potential situation in which corporations will become unable to repay their debts, “fiat is doomed… watch what happens to crypto.”
Related: BTC and Quantitative Easing: What’s the Correlation to Crypto?
This remains a point of debate though. The fact of the matter, said Lingham, is that if the world decided tomorrow to abandon fiat currency and embrace Bitcoin, the cryptocurrency couldn’t handle it. Bitcoin lacks scale — still only able to handle around 7 transactions per second compared to Mastercard’s claim of processing 50,000 TPS. The cost of a single Bitcoin transaction would skyrocket, perhaps into the thousands of dollars.
That said, many crypto evangelists can envision the collapse of the post-Bretton Woods banking system, and its replacement by Bitcoin as the world’s reserve currency. A January white paper laid out the steps by which this might actually occur, though it first requires the price of Bitcoin to reach stratospheric heights:
“If Bitcoin climbs to $10 million per Bitcoin, it could provide the world community with a stable currency, replace sovereign currencies, and act as the reserve currency of the world incapable of inflation or deflation. It would represent the ultimate ‘Store of Value.’”
Why $10 million? At that point, Bitcoin provides a sufficient reserve to alleviate the world’s debt burden. The authors add:
“Bitcoin would be worth between $180 trillion and $210 trillion (depending on when that price was reached). Assuming world debt had reached $500 trillion at that time, remember it has grown by 394% over the past 20 years, Bitcoin would represent a 40% reserve against the debt.”
A realistic possibility? Maybe not, the authors allow. It would require, among other things, the destruction of all altcoins — as they suppress demand for Bitcoin — as well as Bitcoin overtaking gold as a store of value when it is somewhere between the $100,000 and $400,000 price mark.
Also, Bitcoin’s developers have to deliver on their promise of speed, transparency and cost — a big “if.” Lingham, for one, remains skeptical: “The Apples, Googles — the world’s largest companies — are using U.S. dollars still. When they switch over to Bitcoin, then we can have that conversation.”
Men with guns
Crypto utopians often imply that fiat currencies like the U.S. dollar are just a social convention with nothing behind them — since 1944, at least, when the dollar became untethered from gold. But that isn’t quite true, according to Krugman:
“Ultimately, it’s backstopped by the fact that the U.S. government will accept dollars as payment of tax liabilities — liabilities it’s able to enforce because it’s a government. If you like, fiat currencies have underlying value because men with guns say they do. And this means that their value isn’t a bubble that can collapse if people lose faith.”
A lesser role for crypto?
Assuming for the moment that Bitcoin will not supplant the U.S. dollar as the world’s reserve currency anytime soon: Is there a positive, if reduced, role for cryptos and blockchain technology vis-a-vis global debt? IIF’s Riddle told Cointelegraph:
“Crypto doesn’t play a role currently, however technology solutions like blockchain could one day potentially be used to increase debt transparency globally.”
Those solutions could be, for example, the private sector lending to the most vulnerable low-income countries, “or any type of lending.” According to the IIF, “Greater transparency will in turn facilitate good governance, aid the fight against corruption and support debt sustainability.”
Public debt recorded on a blockchain could make it easier for lenders and borrowers both to evaluate emerging risks associated with debt, avoiding problems like the recent Tuna Bonds scandal in Mozambique, where that nation’s government failed to disclose $1.2 billion in loans to the IMF as required under a funding accord.
In sum, while debt ratios are historically high, they still may not be signalling imminent global collapse. Moreover, one shouldn’t count on Bitcoin replacing the U.S. dollar as the world’s reserve currency anytime soon or if ever, according to some. But, in the near and intermediate future, there are still some useful ways crypto and blockchain technology can impact global debt, such as supporting lending transparency in emerging markets.
0 notes
Text
Midsem Exam Solutions
Here’s the solution for the midsem exam, credit to my tutor Andrew:
= COMP6[84]41 Midsemester Exam : 2019t2 = == Total number of marks: 55 == == Total duration: 55 minutes + 5 minutes reading time ==
This is a closed book exam. The class textbook is not provided for this exam.
There is one mark for following the examination instructions.
A result of 50 marks will be scaled to be full marks so you can skip 5 marks worth of questions in part A and still get full marks. I suggest you skip the final question in part A if you are tight for time as part B is worth much more.
If you are doing a pen and paper version of the exam write you name and student number here and sign it
{{{ Name:
Student Number:
Which course: COMP6441/COMP6841 (cross out the wrong one)
Signature:
}}}
If you are doing the exam on a terminal your answers can be submitted by pressing save on this application. You may submit your solutions as many times as you like. The last submission ONLY will be marked.
Write your name and student number on the top of each sheet of any rough working paper you use, this will not be marked, and write your answers in the computer files as directed in the exam instructions.
You must hand in ALL writing paper at the end of the exam.
Once the exam has commenced you may not leave the exam.
You may only use the viewer, GUI calculator, and the decoding app supplied for the last question. Other programs including scripting or interpreted languages may not be used. We are logging all activity and use of other software etc will result in 0 fail. If you are unsure ask the supervisor.
If no answer seems perfect, or if more than one answer seems correct then give the answer which you think best answers the question.
The use of Top Men, misdirection, or social engineering is prohibited. Strict exam conditions apply, including that you may not attempt to communicate with any other person, or access other computers or external data/information or any internet resources.
If you do not follow these instructions you will get zero marks for the exam and a possible zero marks for the course or a charge of academic misconduct.
Phones must be turned off and not visible, either left outside the room in your bag or sealed in an opaque bag placed under your seat.
v1.4
==== Part A ====
This part is worth 39 marks and consists of 13x3 mark questions.
=== Question 0 === (3 Marks)
You are setting the password policy for your company. What is the best policy according to NIST?
.[A] Users must change password every 6 months .[B] Require passwords to satisfy structural rules such as "at least one uppercase letter, one digit, and one non-alphanumeric symbol" .[C] Passwords must not be on a blacklist of common passwords .[D] Passwords must be of a minimum length .[E] Use a set of personal questions as challenges rather than passwords eg "What was the name of your first teacher" .[F] Generate the password randomly for the user and don't allow them to change it.
=== Question 1 === (3 Marks)
The modulus of a particular RSA key is generated by multiplying two different prime numbers, each having a length of 20 decimal digits (there are about 2x10^18 such primes). The modulus is public, the two primes which produced it are secret. All you need to know about RSA is if an attacker can ever find the two prime factors they can find the private key, and so break the code.
If it takes 8 bits of work to test if one number divides into another how many bits of work would it take on average to brute force one of the two factors of the modulus by repeated trial divisions?
.[A] 0-19 bits of work .[B] 20-29 bits of work .[C] 30-39 bits of work .[D] 40-49 bits of work .[E] 50-59 bits of work .[F] 60-69 bits of work .[G] 70-79 bits of work .[H] 80 or more bits of work
=== Question 2 === (3 Marks)
In a hypothetical electronic voting system the candidate names are "Putin", "Trump", "Xi", "Ahern", "Johnson", "Duterte" and "Trudeau". Candidates vote by encrypting their selected candidate's name, and posting the resulting encrypted candidate name on a public bulletin board which is tamper evident. The ID of the voter is shown alongside their encrypted vote on the bulletin board so everyone can check that no one voted twice, and that only eligible voters voted.
ASSUME THAT ELECTORAL OFFICIALS CAN BE SAFELY TRUSTED i.e. don't consider insider attacks in your answer. For each of the following encryption/hash schemes state whether or not it could safely be used to encrypt the candidate names in order to ensure that no candidate can view the bulletin board and learn who did, or who didn't, vote for them.
Vignere Cipher (each individual voter has a unique key also known to electoral officials) .[Yes] Safe to use .[No] Not safe to use
2048 bit RSA (encrypted using a publicly known public key, only the electoral officials know the private key) .[Yes] Safe to use .[No] Not safe to use
SHA256 .[Yes] Safe to use .[No] Not safe to use
One Time Pad (each individual voter has a unique key also known to electoral officials) .[Yes] Safe to use .[No] Not safe to use
=== Question 3 === (3 Marks)
You encode your favorite quote using METHOD A and paste the cipher text at the end of a long email to your friend - and then you encrypt the whole email using METHOD B before you send it. So the quote has been encrypted twice.
Roughly how much work will it take to find the decryption of the quote if it takes 30 bits of work to decrypt METHOD A and if it takes 30 bits of work to decrypt METHOD B?
.[A] 30 bits .[B] 60 bits .[C] 90 bits .[D] 900 bits .[E] A good quote by Goethe is "You can easily judge the character of a person by how they treat those who can do nothing for them" - however it's not the one you encrypted.
=== Question 4 === (3 Marks)
The following question relates to the Houdini case study done in your analysis group.
The object of the case study was to devise a protocol for Bess to follow. What are the two most important properties the protocol needed to have?
Most important property (use between 4 and 20 characters in your answer) (hint: it is one of the CIA properties) Authentication_____
Second most important property (use between 4 and 20 characters in your answer) (no hints for this one) Non-repudiation ______
=== Question 5 === (3 Marks)
Suppose the president of a country is the only one who knows the 10 digit pin needed to arm the country's nuclear weapons (to prevent unauthorised launches). If you were the country's head of military security what would you be most worried about in this scenario:
.[A] Integrity .[B] Authentication .[C] Security Engineering .[D] Proof of liveness .[E] Security by obscurity .[F] Type I/Type II error tradeoff
=== Question 6 === (3 Marks)
What sort of attack would the following most likely be used in?
.[A] Bump .[B] Rake .[C] Shim .[D] Brute force .[E] Impressioning .[F] Social Engineering
=== Question 7 === (3 Marks)
A locksmith uses the tool below to pick a tumbler lock with 6 pins and 6 possible pin heights. Suppose she already knows the correct sequence in which to try the pins. How many combinations will she have to test in the worst case?
.[A] 6 .[B] 6+6 .[C] 6*6 .[D] 6^6 .[E] 2^(6+6)
=== Question 8 === (3 Marks)
Suppose your company has been hit by a ransomware attack. What is the most likely to have been used in the attack?
.[A] Corrupt Insider .[B] Social engineering .[C] Memory corruption .[D] Brute force .[E] Rainbow table .[F] Security by obscurity .[G] 0-day .[H] Integrity .[I] Confidentiality .[J] Proof of Liveness
=== Question 9 === (3 Marks)
On average how many hashes would be required to succeed in each of the following attacks against SHA-256? You may assume SHA-256 has not yet been broken. Write your answer to the nearest power of 2 eg if you think the answer is 250 enter "8" as your answer (since 2^8 is 256)
Preimage: 2^ 255 hashes
Second preimage: 2^255 hashes
Collision: 2^128 hashes
=== Question 10 === (3 Marks)
Lachlan and I are going to use going to use Merkle Puzzles to securely discuss the exam questions on Friday the day before the exam runs (because he loves Merkle Puzzles). I'll send him 1,000,000 encrypted mini-messages of the form:
{{{ ...
...
This is puzzle two hundred thousand and seventeen, the key is jHg4t5ct&rqSg
This is puzzle two hundred thousand and eighteen, the key is 3pojygv3x%wD?
...
...
}}}
What cipher/hash would be best to use to encrypt these mini-messages?
.[A] One Time Pad .[B] Ceasar .[C] Vigenere .[D] RSA 64 bit modulus (encrypted using my public key) .[E] RSA 2048 bit modulus (encrypted using my public key) .[F] SHA256
=== Question 11 === (3 Marks)
A confident sounding comment about passwords posted on the internet: {{{ I’ve moved to using three word passphrases that use Subject->Action->Object format.
They need not be sensible though. Things like:
Obama Punting Cornflakes
or
Grandma Curling Pumpkins
Both of those are over 80 bits, and you’ll never forget them. In fact, if you read this, you’ll never get the image of Obama punting a box of cornflakes out of your head."
- Jonathan Beerhalter 2012-03-08
}}}
Most people have a vocabulary of between 20,000 and 40,000 words that they use or can recognise.
Assume that the three words in each passphrase are randomly chosen from three dictionaries of 10,000 familiar words each (one of Subject words, one of Action words, one of Object words) and are written separated by a space, first letter uppercase, remaining letters lowercase, and that it takes one bit of work to test one passphrase.
How many bits of work would it take on average to break one of Jonathan's passphrases?
.[A] Around 40 bits .[B] Around 50 bits .[C] Around 60 bits .[D] Around 70 bits .[E] Around 80 bits .[F] Around 90 bits .[G] Around 100 bits or more
=== Question 12 === (3 Marks)
I suggest you don't do this question until and unless you have finished Part B below - it is probably not worth it. Remember you can skip 5 marks in Part A and still get full marks.
The ciphertext below has been produced by a Vignere cipher.
OUEYZLWCFMGYOULHEXWWRISCGHGADLWZBKDCXUOEDUHJRTQOFUOEDCXUPMHP WUCIDUJCATBNAPRBEHWUETOJWUEYZLAZSTQUHJRTQHWUEBESGOEXMYECRLGU NYNWUUFNNZDVSCGMGYECSMGYQUEXBIFZHLDXUUCMTLWNUXLCXNUXXUJYANLY JIHLOLWJNKDZGLGADGAZGADSSLRLSLGHTTUIAXGADGAZGADSSLRTMAJSVLSO JVGADGAZGADSSLRATGTFRFZEWNUXLBSOTASSAZGADSSLRKDFSRRWSIAFGADG AZGADSSLRTKFMHVMDXKYCTQULYGADG
What is the likely period of the cipher? (ie length of the cipher key)?
_6_
==== Part B ====
This part is worth 15 Marks and consists of one question.
=== Question 13 === (15 Marks)
Decode the ciphertxt in the NSA app (which is in a tab in your web browser). If you close it by mistake you can reopen it by right clicking on the desktop, or ask the exam supervisor for help.
The message has been enciphered using a monoalphabetic substitution cipher.
Write the deciphered plaintext below, use ? symbols for undecrypted letters, partial marks for partial decryptions so long as it is clear.
0 notes
Text
Day 4
99 bottle
đọc tiếp Refactoring by Martin Fowler:
why refactor: Improves the Design of Software, easier understand, easy find bugs, program faster.
Rút ra ý tưởng:
1. Viết Test (Red green refactor - TDD)
1*. Áp dụng nguyên tắc clean code: đặt tên, sắp xếp
2. Extract Method, Move Method, run test after
3. replace if with polymorphism
Chiêu khác:
++ Form Template Method.?Replace Type Code with State/Strategy.Extract Subclass, Replace Temp with Query, Remove Setting Method, Push Down Method,Introduce Assertion.Introduce Null Object
++Pull Up Field, Extract Class, Introduce Parameter Object, Preserve Whole Object.Replace Method with Method Object.Extract Subclass,Extract Interface,Replace Parameter with Method, Replace Type Code with Subclasses,Collapse Hierarchy.Inline Class. Rename Method.Introduce Null Object.Hide Delegate.Remove Middle Man.Replace Delegation with Inheritance
++ Change Bidirectional Association to Unidirectional. Extract Superclass. Introduce Foreign Method.Introduce Local Extension.Encapsulate Collection
++ In this case you want to use Move Method and Move Field to put all the changes into a single class. If no current class looks like a good candidate, create one.
To clean this up you identify everything that changes for a particular cause and use Extract Class to put them all together.
+ Data clumps: Data items tend to be like children; they enjoy hanging around in groups together.
+ Nearly useless components should be subjected to Inline Class.
Mindset khác:
- Code clean trước khi optimize (dễ optimize), optimize khi thấy chương trình chậm
Bad Smells in Code:
Foreword........................................................................................................................ 6 Preface........................................................................................................................... 8 What Is Refactoring? ............................................................................................... 9 What's in This Book? ............................................................................................... 9 Who Should Read This Book?............................................................................. 10 Building on the Foundations Laid by Others ...................................................... 10
Acknowledgments .................................................................................................. 11 Chapter 1. Refactoring, a First Example ................................................................ 13 The Starting Point................................................................................................... 13 The First Step in Refactoring................................................................................ 17 Decomposing and Redistributing the Statement Method ................................ 18 Replacing the Conditional Logic on Price Code with Polymorphism ............. 35 Final Thoughts ........................................................................................................ 44 Chapter 2. Principles in Refactoring........................................................................ 46 Defining Refactoring .............................................................................................. 46 Why Should You Refactor? .................................................................................. 47 Refactoring Helps You Find Bugs ....................................................................... 48 When Should You Refactor?................................................................................ 49 What Do I Tell My Manager?................................................................................ 52 Problems with Refactoring .................................................................................... 54 Refactoring and Design......................................................................................... 57 Refactoring and Performance .............................................................................. 59 Where Did Refactoring Come From?.................................................................. 60 Chapter 3. Bad Smells in Code................................................................................ 63 Duplicated Code..................................................................................................... 63 Long Method ........................................................................................................... 64 Large Class ............................................................................................................. 65 Long Parameter List............................................................................................... 65 Divergent Change .................................................................................................. 66 Shotgun Surgery..................................................................................................... 66 Feature Envy........................................................................................................... 66 Data Clumps ........................................................................................................... 67 Primitive Obsession ............................................................................................... 67 Switch Statements ................................................................................................. 68 Parallel Inheritance Hierarchies........................................................................... 68 Lazy Class ............................................................................................................... 68 Speculative Generality........................................................................................... 68 Temporary Field ..................................................................................................... 69 Message Chains ..................................................................................................... 69 Middle Man.............................................................................................................. 69 Inappropriate Intimacy........................................................................................... 70 Alternative Classes with Different Interfaces ..................................................... 70
Incomplete Library Class....................................................................................... 70 Data Class ............................................................................................................... 70 Refused Bequest.................................................................................................... 71
4 Comments ............................................................................................................... 71 Chapter 4. Building Tests.......................................................................................... 73 The Value of Self-testing Code............................................................................ 73 The JUnit Testing Framework .............................................................................. 74 Adding More Tests ................................................................................................. 80 Chapter 5. Toward a Catalog of Refactorings ....................................................... 85 Format of the Refactorings ................................................................................... 85 Finding References................................................................................................ 86 How Mature Are These Refactorings?................................................................ 87 Chapter 6. Composing Methods .............................................................................. 89 Extract Method........................................................................................................ 89 Inline Method........................................................................................................... 95 Inline Temp.............................................................................................................. 96 Replace Temp with Query .................................................................................... 97 Introduce Explaining Variable............................................................................. 101 Split Temporary Variable..................................................................................... 104 Remove Assignments to Parameters ............................................................... 107 Replace Method with Method Object ................................................................ 110 Substitute Algorithm ............................................................................................. 113 Chapter 7. Moving Features Between Objects.................................................... 115 Move Method ........................................................................................................ 115
Move Field ............................................................................................................. 119 Extract Class ......................................................................................................... 122 Inline Class............................................................................................................ 125 Hide Delegate ....................................................................................................... 127 Remove Middle Man............................................................................................ 130 Introduce Foreign Method................................................................................... 131 Introduce Local Extension................................................................................... 133 Chapter 8. Organizing Data .................................................................................... 138 Self Encapsulate Field......................................................................................... 138 Replace Data Value with Object ........................................................................ 141 Change Value to Reference ............................................................................... 144 Change Reference to Value ............................................................................... 148 Replace Array with Object .................................................................................. 150 Duplicate Observed Data .................................................................................... 153 Change Unidirectional Association to Bidirectional ........................................ 159 Change Bidirectional Association to Unidirectional ........................................ 162 Replace Magic Number with Symbolic Constant ............................................ 166 Encapsulate Field................................................................................................. 167 Encapsulate Collection........................................................................................ 168 Replace Record with Data Class....................................................................... 175 Replace Type Code with Class .......................................................................... 176 Replace Type Code with Subclasses ............................................................... 181 Replace Type Code with State/Strategy........................................................... 184 Replace Subclass with Fields............................................................................. 188 Chapter 9. Simplifying Conditional Expressions ................................................. 192
5 Decompose Conditional ...................................................................................... 192 Consolidate Conditional Expression................................................................. 194 Consolidate Duplicate Conditional Fragments ................................................ 196 Remove Control Flag........................................................................................... 197 Replace Nested Conditional with Guard Clauses ........................................... 201 Replace Conditional with Polymorphism .......................................................... 205 Introduce Null Object ........................................................................................... 209 Introduce Assertion.............................................................................................. 216 Chapter 10. Making Method Calls Simpler........................................................... 220 Rename Method................................................................................................... 221 Add Parameter...................................................................................................... 222 Remove Parameter.............................................................................................. 223 Separate Query from Modifier............................................................................ 225 Parameterize Method .......................................................................................... 228 Replace Parameter with Explicit Methods........................................................ 230
Preserve Whole Object ....................................................................................... 232 Replace Parameter with Method ....................................................................... 235 Introduce Parameter Object ............................................................................... 238 Remove Setting Method...................................................................................... 242 Hide Method.......................................................................................................... 245 Replace Constructor with Factory Method....................................................... 246 Encapsulate Downcast........................................................................................ 249 Replace Error Code with Exception .................................................................. 251 Replace Exception with Test .............................................................................. 255 Chapter 11. Dealing with Generalization.............................................................. 259 Pull Up Field .......................................................................................................... 259 Pull Up Method ..................................................................................................... 260 Pull Up Constructor Body.................................................................................... 263 Push Down Method.............................................................................................. 266 Push Down Field .................................................................................................. 266 Extract Subclass................................................................................................... 267 Extract Superclass ............................................................................................... 272 Extract Interface ................................................................................................... 277 Collapse Hierarchy............................................................................................... 279 Form Template Method....................................................................................... 280 Replace Inheritance with Delegation................................................................. 287 Replace Delegation with Inheritance................................................................. 289 Chapter 12. Big Refactorings ................................................................................. 293 Tease Apart Inheritance...................................................................................... 294 Convert Procedural Design to Objects ............................................................. 300 Separate Domain from Presentation................................................................. 302 Extract Hierarchy.................................................................................................. 306 Chapter 13. Refactoring, Reuse, and Reality...................................................... 311 A Reality Check .................................................................................................... 311 Why Are Developers Reluctant to Refactor Their Programs? ...................... 312 A Reality Check (Revisited)................................................................................ 323
6 Resources and References for Refactoring..................................................... 323 Implications Regarding Software Reuse and Technology Transfer............. 324 A Final Note........................................................................................................... 325 Endnotes................................................................................................................ 325 Chapter 14. Refactoring Tools ............................................................................... 328 Refactoring with a Tool........................................................................................ 328 Technical Criteria for a Refactoring Tool.......................................................... 329 Practical Criteria for a Refactoring Tool............................................................ 331 Wrap Up ................................................................................................................. 332 Chapter 15. Putting It All Together ........................................................................ 333 Bibliography........................................................................................................... 336 References ................................................................................................................ 336
0 notes
Text
A $_POST about PHP (and MySQL)
PHP is great.
No, really. It is. The syntax is very much like JavaScript, save for a few fundamental differences.
PHP is a server-side (backend) scripting language that works in the background to enhance your HTML file and make it more dynamic. For instance, paired with a database like MySQL, it can help you create working forms (thus far, our HTML forms have looked pretty, but have done absolutely nothing useful).
The way I see it right now is that If HTML and CSS were the contents and layout of your home, PHP and the backend is a little bit like Marie Kondo organising all of the crap in your cupboards so that if someone needs to find the Sellotape you know exactly where to look for it (a rare occurrence in our house).
It’s more complicated than that but, for now, that metaphor has carried me through.
That’s great, but how do I use it?
There’s a few things you need to do in order to start using PHP.
Unlike CSS stylesheets and Javascript files, you don’t link a PHP file to your HTML in the <head> tag and just start coding. Because PHP works in the backend, it needs a server to work with. In fact it needs a few things to make it work properly - usually in the form of a LAMP stack.
LAMP is an acronym for the four ingredients (software components) needed to make a website work:
L is for Linux (a common operating system used by Macs)
A is for Apache (a web server)
M is for MySQL (a database)
P is for PHP (a scripting language)
These are the four things you need for a dynamic website (there are other versions of a LAMP stack [eg. a WAMP stack for Windows]).
We’ve been using Vagrant to create virtual machines (servers) on our computers so that we can learn PHP. For some reason I find this concept a bit magical and had a Zoolander moment when it was first explained to us.

Furthermore, typing vagrant destroy into the command line feels like the programming equivalent of Daenerys Targaryen saying “Dracarys”:
PHP syntax
Once you’ve got your virtual machine running, you can start using PHP. Like Javascript, PHP deals with strings, functions, arrays and so on. Some differences between the two include things like:
1. You use a $ to declare variables, rather than Javascript ‘let’
2. You can whack it right in the HTML if you wrap it in <?php ... ?>
3. You output stuff to the browser using echo
4. You use dots to concatenate, rather than +’s
5. Variables are often written in snake_case, rather than camelCase
6. Arrays get a bit more fancy in PHP. An associative array is made up of key => value pairs so you can do things like this ...
I’ve just realised that will output ‘Legolas is a elf’ which is truly awful grammar but you get the idea. The <br/> added to the end means that the list will output on separate lines ...
You can have a look at what’s going on in an array using var_dump($array_name). This will return a load of info about the array that looks something like this:
array(3) { [“Frodo”]=> string(6) “Hobbit” [“Aragorn”]=> string(5) “Human” [“Legolas”]=> string(3) “Elf” }
Otherwise, conditionals (for and while loops, switches) and functions work in pretty much the same way as Javascript.
PHP and Forms
Much of PHP week was spent creating a login system - of the type where you register your details using a form, then get sent a verification email with a link that takes you to a login page.
This is something we all use all the time but I did not appreciate just how much effort and coding goes into building one from scratch.
In order to actually do something useful with a login form, you need to use PHP to communicate between the client device and the server (i.e. the user submits a form and, on pressing ‘Send’, the information they have inputted is stored somewhere in a database and they are sent a response to tell them what to do next).
This is done using GET and POST methods. I’ll probably talk more about these when we come to Laravel and APIs but, for now, the essential idea is that a GET method requests data from a specified resource (eg. GET asks the server for the contact.html page and the server responds by loading it) and a POST method submits data to be processed by a specified resource (eg. POST posts form inputs to the form-handler.php file on the server which then responds with a success page or whatever).
Obviously, when someone inputs information into a form, it has to go somewhere to be stored: that’s where databases come in.
MySQL
MySQL is a database management system that helps you store all your info in neat little tables using even neater commands.
Once you get the hang of the syntax, and how to navigate to MySQL in the first place (using vagrant ssh and typing in your username and password to access mysql), it’s really easy to figure out what’s going on in there.
For example, to create a table, you use the following command:
CREATE TABLE `people` ( `id` int(11) NOT NULL AUTO_INCREMENT, `fullname` varchar(255) NOT NULL, `location` varchar(255) NOT NULL, `age` int(11) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
Looks nasty, but it just says ‘create a table called ‘people’ with the following column headers: ‘id’ (that auto-increments each time a new row is added), ‘fullname’, ‘location’ and ‘age’. The table’s primary key is the ‘id’ and then there’s some default stuff at the end.
This creates an empty table, so you set up your PHP to add data to the table as and when it is inputted by the user.
You can also input data manually in the command line:
INSERT INTO `people` (`id`, `fullname`, `location`, `age`) VALUES (1, 'Frodo Baggins', 'The Shire', 47), (2, 'Aragorn', 'Various', 40), (3, 'Boromir', 'Minas Tirith', 38), (4, 'Galadriel', 'Lothlorien', 17988);
To check out your table, you use:
SELECT * FROM `people`;
Please don’t write in to tell me how wrong my LOTR estimates are.
Databases are fun. There’s something satisfying about organising tables that I cannot quite explain. I’m getting married next year and one of the first things I made sure we did was to create a wedding spreadsheet, which I call ‘the wed-sheet’. Pete’s a lucky guy...
Building a Login System
Right, I’m not going to be able to put all the fine details of building a login system here but here’s the general gist of it.
Disclaimer: I’m writing this a few weeks after the fact, having done Wordpress, Object Oriented Programming, Laravel and React in the interim - my brain is swimming in React right now, so please forgive anything I miss out.
1. The user inputs their details on a registration page to create an account:
2. I’ve got some conditionals in my register.php page that will alert the user if they haven’t entered a valid email address or password. If they don’t they get a variety of different error messages:
3. If they do it right, they get this success message:
A successful entry also creates a unique activation code and adds the new user details to the database securely by hashing the codes and passwords.
You can see the MySQL syntax in the db query below:
Here’s part of the entry in the MySQL db. The password looks like crazy gibberish because of the hashing.
It then sends the new user an email with a link. The slug of the link’s URL is the activation code, so is unique.
Here’s what they see in their email inbox:
Notice that when they click on the link, the url contains the activation code:
There are various error messages on the activation.php page for anyone who is trying to access the site without registering correctly.
4. Clicking on the link shown in the image above takes them to this login page:
Here, they re-submit their username and password. Again, there’s various conditionals in case they get it wrong, including queries to the database to check that the information they have provided actually exists.
When they’ve successfully logged in, they start a new session, which continues until they log out again. I also coded in a ‘forgot password’ system - which I’m not going to go into now but eventually I’ll add a link here to my completed login system so you can have a look.
When all of this finally came together and worked, it was potentially one of the most satisfying moments on the course up to this point.
When it was done, I was so proud of myself that I just spent 30 minutes registering new people just to go through the motions of what I’d just created. I then made Pete do the same when I got home. Again, lucky guy.
Who knew login systems could provide so much joy. I’m definitely going to be including it as part of my portfolio website - more on that next time when we look at Wordpress! :)
0 notes
Text
0 notes
Text
300+ TOP VBSCRIPT Interview Questions and Answers
VBScript Interview Questions for freshers experienced :-
1. What is VBScript language used for and which earlier language is it modeled upon? VBScript is a Lightweight primary Scripting language which is used for automation of the Scripts in QTP (Quick Test Professional) tool. This is modeled upon Visual Basic language. 2. What are the environments supported by VBScript language? There are 3 Environments where VBScript can run: IIS (Internet Information Server) – This is Microsoft’s Web Server. WSH (Windows Script Host) – This is the hosting environment of the Windows Operating System. IE (Internet Explorer) – This is the most frequently used environment to run scripts and this is the simple hosting environment. 3. Which data type/types are supported by VBScript language and what are their specialties? There is only one data type that is supported by VBScript language and it is called as ‘Variant’. If we use this data type in the String context then this will behave like a String and if we use this in Numeric context then this will behave like a Number. This is the specialty of the Variant data type. 4. What is the extension of the VBScript file? VBScript file is saved with an extension of .vbs. 5. How are Comments handled in the VBScript language? Any Statement that starts with a single quote (‘) or with the keyword ‘REM’ is treated as a Comment. 6. Which respective symbols are used to separate a line and to break the lengthy statement into multiple statements in the VBScript language? Colons (:) act as a line separator and Underscore (_) is used to break the lengthy statement into multiple statements in the VBScript language. 7. What are keywords in the VBScript language? There are some words which work as Reserved Words and they cannot be used as Variables names, Constant names or any other Identifier names are known as keywords. Some of the keywords in the VBScript language are Not, Nothing, Preserve, Optional, etc. 8. Is VBScript language a Case-Sensitive language and what does it mean? No. This actually means that variable names, keywords, constants and other identifiers are not required to be typed with consistent Capitalization of letters i.e. if you type ‘Optional’ keyword as OPTIONAL, optional or Optional then these all mean the same in the VBScript language. 9. What are the naming conventions while declaring a variable in the VBScript language? Following are the rules for declaring a Variable name: Variable Name must always start with a letter. E.g., output, name, etc. Variable Name should not start with a number or any special character like _va123, 12non, etc. Variable Name cannot exceed the limit of 255 characters. Variable Name should never contain a period (.). 10. Which keyword is used to declare a variable in the VBScript language? The Dim keyword is used to declare a variable in the VBScript language. However, depending upon on the scope of the variable, public or private keywords can also be used. 11. What are the 2 ways in which a variable can be declared in the VBScript language? Two ways to declare a variable are: Implicit Declaration: When variables are used directly without declaration, it is termed as Implicit Declaration. However, it’s not a good practice because at any point if the variable name is not spelled correctly in the script then it can produce weird results while running and at times, it will not even be easy to detect this by the user. Explicit Declaration: Declaring the variables before using them is known as Explicit Declaration of variables. 12. What is the use of Option Explicit Statement? This provides a mechanism where the user has to declare all the variables using Dim, Public or Private Statements before using them in the Script. If the user tries to use the variables which are not declared in case of Option Explicit then an error occurs. It is always recommended to use ‘Option Explicit’ at the top of the code so that even if you use the wrong name of the variable unintentionally, you can then correct it immediately without any confusion. 13. How are values assigned to the variables in the VBScript language? Values are assigned with the help of Equal (=) Operator. Name of the Variable comes on the left and the value which is assigned to the Variable is on the Right Hand Side of the ‘=’ Operator. 14. How are values assigned to String type and Numeric type variables? If the variable to which value is to be assigned is of String type then it can be assigned using double quotes (“”) and if the variable to which value is to be assigned is of the Numeric type then it can be assigned without using double quotes. 15. Explain the scope of the variables using Dim, Public, and Private keywords respectively. If the variable is declared using Dim keyword inside the function then its scope will be limited to the function level only i.e. this variable cannot be accessed once the function ends. If the variable is declared using Private keyword inside the function then its scope will not be limited till function level only but it can be accessed everywhere in that particular script. If the variable is declared using Public keyword inside the function then its scope will not be limited till function level alone, but it can be accessed everywhere in that particular script and also in the other scripts. 16. How can constants be declared in the VBScript language? Constants are named memory locations within a program that never change their values during the execution of the script. ‘Const’ keyword is used to declare Constants in the VBScript language. 17. Which constant is used for print and display functions and works as same as pressing Enter key? vbCrLf is used for print and display functions representing a carriage return with line feed character having values as Chr(13) & Chr(10). This works in the same manner as in the case of pressing an Enter key. This is a pre-defined constant of the VBScript language. 18. How many types of Operators are available in the VBScript language? There are 4 types of Operators which are supported by the VBScript language. They are: Arithmetic Operators Comparison Operators Logical Operators Concatenation Operators 19. Which Operator is used for fetching the modulus of the 2 numbers in the VBScript language? MOD Operator is used for fetching the modulus of the 2 numbers in the VBScript language. 20. Which Operator is used to perform the comparison among 2 operands in the VBScript language? ‘==’ Equal Operator is used for performing the comparison among 2 operands in the VBScript language i.e. if we check 1 == 2 then it will give False. 21. Which Operator is used to concatenate the 2 values in the VBScript language? ‘&’ Operator is used to concatenate the 2 values in the VBScript language. 22. If we take 2 strings as “Good” and “Bad” then what will ‘+’ and ‘&’ operators return? ‘&’ and ‘+’ both works as a Concatenation Operator in case of the String values. Hence these both will return the same result as GoodBad. 23. Which Operator can be used to change the value of the operand or change the state of the condition? ‘NOT’ Operator which is used as a logical operator can be used to change the value of the operand or change the state of the condition i.e. if the condition is True then this will change it to False and vice versa. 24. Out of the different type of Operators, which are evaluated first and last in the VBScript language? ‘Arithmetic’ Operators are evaluated first and ‘Logical’ Operators are evaluated at last in the VBScript language. 25. Which conditional statement is the most convenient one to use in the case of multiple conditions in the VBScript language? ‘Select Case’ is the most convenient one to use in case of multiple conditions in the VBScript language as in case of Select Statement, you can directly move to the exact case without wasting time to go into each condition one by one. 26. What are the different types of loops available in the VBScript language? The loops which are available in the VBScript language are broadly categorized into 3 types and they are – For Loops, Do Loops and While Loops. 27. Which loop is used in case of arrays in the VBScript language? For Each loop is used in case of Arrays. This is an extension of For Loop only. When you want to repeat the code for each index value of an array then you can use ‘For Each Loop’. 28. What is the difference between For loop and While loop? This is a very important Interview Question which is been asked many times. For loop is used when we exactly know the number of times loop (i.e. for i = start to end) has to be executed unlike in case of while loop. In ‘for loop’ in the VBScript, the counter is incremented automatically if not mention step keyword by 1 when loops go to next keyword whereas in ‘while loop’, the counter condition has to be mentioned explicitly inside the brackets. 29. What is the difference between Do Until loop and Do While loop? Do While loop first checks the condition and if it is true only after that the statements are executed and in case of Do Until, the loop will be executed until the condition becomes false. 30. How many types of Procedures are available in the VBScript language? There are 2 types of Procedures in the VBScript language – Sub Procedures and Function Procedures. Sub is a type of procedure which includes a set of statements inside the block of the code and after execution, it does not return any value. The Function is a type of procedure which includes a set of statements inside the block of the code and after execution may return value also. This can take input if required, depending on the situations. 31. What are the differences between Sub Procedures and Function Procedures? Differences are as follows: Sub Procedure never takes an input while the Function Procedure may take an input if required. Sub Procedure starts and ends with using Sub and End Sub respectively while Function Procedure starts and ends with Function and End Function respectively. The most important difference is Sub Procedure never returns a value while Function Procedure may return a value. 32. What are the 2 ways to pass a value to the Function? The 2 ways to pass a value to the function are: Pass by Value: When arguments are passed and any Changes that take place in the called procedure in the value of a variable does not remain to persist then it means it is passing by value. Keyword used in this case is ByVal. Pass by Reference: When arguments are passed and any Changes that take place in the called procedure in the value of a variable remain to persist then it means it is passing by reference. Keyword used in this case is ByRef. 33. Which In-Built function is used to format the number in the VBScript language? FormatNumber Conversion function is used to convert the specified expression in the form of a Number. 34. Which In-Built functions are used to convert the specified expression in the form of Date and String in the VBScript language? cDate is one of the frequently used Conversion Function for converting the expression which includes Date or Time parameters into Date subtype. cStr is the Conversion function which is used for converting the expression into String subtype. 35. How are Arrays declared in the VBScript language? Declaration of Array can be done in the same manner in which Variables are declared but with a difference that array variable is declared by using parenthesis ‘()’. The Dim keyword is used to declare an Array. Ways to declare an Array: There are 3 ways in which an Array can be declared. They are as follows: Way 1: Dim array1() Here, array1 is the name of an array and since parenthesis is empty it means that the size of an array is not defined here. If you want to declare an array by mentioning its size then it can be done in the following way. Way 2: Dim array1(5) Here, array1 is declared with the size as 5 which states it holds 6 values considering the index of an array always starts from 0. These 5 values can be of integer type, string or character types. Way 3: array1 = Array(1,2,3,4,5,6) Here, Array Function is used to declare an array with a list of the arguments inside the parenthesis and all integer values are passed directly inside the parenthesis without any need of mentioning the size of an array. Note: Index value of an Array can never be a negative value. 36. What are lbound and ubound in the VBScript language? lbound indicates the smallest subscript or index of an Array in the VBScript language and this always returns 0, as the index value of an Array always starts from 0. ubound returns the largest subscript of a defined array or can say indicates the size of an Array. If the size of an array is 5 then the value of the ubound is 5. 37. Which In-Built function related to an Array joins substrings into one string in the VBScript language? Join function combines multiple substrings into a String. Here, the String returned includes various substrings in an array and thus joins all the substrings into one string. The syntax of this is as follows: Join(array,. Using delimiter is an optional condition. 38. How many types of Arrays are available in the VBScript language? There are basically 2 types of Arrays that are used in the VBScript: Single Dimensional Array: This is a simple type of array which is used more often in the scripts. Multi-Dimensional Array: When an Array is having more than 1 dimension then it is known as a multi-dimensional array. Normally, a 2-dimensional array is the one which is used most of the times i.e. there will be rows and columns in an array. The maximum dimension of an array can reach to 60 39. When are REDIM statement and PRESERVE keyword used in the VBScript language? This is a very important Interview Question which has been asked many times. Redim Statement is used to re-define the size of an array. When the array is declared without any size, an Array can be declared again using Redim with the feasibility of specifying the size of an array. Preserve keyword is used to preserve the contents of a current array when the size of an array gets changed. Let’s understand the usage of these keywords with the help of a simple example: Let’s see implementation of Redim and Preserve Dim array1() REDIM array1(3) array1(0) = “hello” array1(1) = 12 array1(2) = 13 array1(3) = “how are you” REDIM PRESERVE array1(5) array1(4) = 15 array1(5) = 16 For i = 0 to ubound(array1) Msgbox “Value present at index ” & i & ” is “ & array1(i) & �� ” Next 40. What is the use of the Date function in the VBScript language? Date function displays the current system Date and Time in the VBScript language. 41. Which Date function is used in the VBScript language to find the difference between the 2 dates? DateDiff function is used to fetch the difference between the 2 dates that are specified as parameters on the basis of the interval specified. 42. What is the use of the FormatDateTime function in the VBScript language? This is a format function which is used to convert the Date to some specific format based on the parameters that are supplied to the function. The syntax of this is FormatDateTime(Date, Format). This is a widely used format function. 43. Which function is used in the VBScript language to convert the specified expression into a Date type value? cDate is used to convert a valid expression into a Date type value. The syntax of this is cDate(date) i.e. any valid Date/Time expression will be converted into a particular Date. 44. What is the use of the Instr function? This is used to find the position value of the substring at its first occurrence inside the main string. This function requires 2 strings to be specified to perform this search operation and the search operation starts from the first character. The syntax of this function is InStr(name of string1, the name of string2). If the name of string1 or string2 is null or “ ” then this function will return null and 0 respectively. This return >=1 values when the string is found and 0 in the case when the string is not found. 45. How to get the length of the string by making use of the String function? Len function is used to get the length of a specified String i.e. total number of characters of a specified String. The syntax of this is Len(name of the string). 46. Which function is used to perform string comparison? StrComp is used to compare the 2 strings and return values on the basis of the comparison. This returns 0 if string1 = string2,-1 if string1string2 and null if any of the strings is null. The syntax of this is StrComp(name of the string1, name of the string2). 47. How can the spaces from the string be removed? Trim function is used to trim/remove the spaces from both the sides of the specified String. The syntax of this is Trim(name of the string). 48. How can you fetch the value of a Cookie? document.cookie stores the information of key-value pairs and expiration date values of a Cookie. document.cookie = “key1=name of the value1;key2=name of the value2,…….,expires=date”. ‘;’ is used to separate the key-value pairs. 49. What are Events in the VBScript language? Events are the Actions that occur when any activity is performed like any mouse click, pressing the keys, mouse hover, etc. With the help of writing a piece of code in the programming languages like VBScript, these events can be captured and actions can be performed as per your requirements by making the best use of the Event Handling mechanism. 50. Which Event is triggered when mouse focus comes out of an element in the VBScript language? MouseOut Event is triggered when mouse focus comes out of an element in the VBScript language. 51. When does ‘On Click of Button’ event gets triggered in the VBScript language? This Event occurs in case of the clicking of any button that is present on any HTML page. 52. Which object is used to work with the excel sheets in the VBScript language and what statement is used to create this object? Excel Objects provide supports to the Coders to work and deal with the Excel Sheets. Set obj = createobject(“Excel.Application”) is the way to create an Excel Object. 53. Which object is used to work with the database in the VBScript language and what statement is used to create this object? Connection Objects provide supports to the Coders to work and deal with the database. As such, there is not any straight-forward mechanism to connect to the database in QTP but by making use of ADODB Objects, you can interact with the database and work with the SQL Queries to fetch the data from the database. ADO stands for ActiveX Data Objects and this provides a mechanism to act as an intermediary between the QTP and the Database. Set obj = createobject(“ADODB.Connection”) is the way to create a Connection Object. 54. What is the use of the ‘Open’ method to work with the database in the VBScript language and what connection string is passed in the same and what is its usage? This is used to open a database connection object/recordset object. obj.Open“Provider=SQLQLEDB;Server=.\SQLEXPRESS;UserId=test;Password=P@123;Database =AUTODB” is the connection string for opening a database connection. The connection string is a very useful property and this is used for creating a database connection and includes connection information like details of the Driver, Database Server name, Username, and Password. 55. Why is it recommended to close the database connection every time after the work is completed? This is a very important Interview Question which has been asked many times. It is recommended to close the resource after its usage is completed although it’s not mandatory as library or driver ultimately will close the connection but this is required to avoid any negative effects due to improper closing of the connections which can even lead to a restriction in the access of the database by some of the users. 56. What is the use of the RecordSet object and which statement is used to create such an object? The RecordSet object is used for holding the records of the query which are extracted from the database. Set obj = createobject(“ADODB.RecordSet”) is the statement for creating a RecordSet object. 57. How can you create a file object to work with the files in the VBScript language? Set obj = createobject(“Scripting.FileSystemObject”) is the statement for creating a File object. 58. What methods are used to create text files and open text files in the VBScript language? CreateTextFile and OpenTextFile methods are used to create open text files and open text files respectively in the VBScript language. 59. What is the purpose of the Err object in the VBScript language? This is basically used to capture the details about the Error i.e. if you want to know about the Error Number, description and other details then you can do so by accessing the properties of this Object. 60. Why is Error handling required? You can take measures to get as much lesser number of errors as possible by making use of Error Handling Mechanism in your scripts. Situations like issues in mathematical computations or any type of error can be handled with the help of Error Handling. 61. What purpose does ‘On Error Resume Next’ serves? On Error Resume Next moves the control of the cursor to the next line of the error statement i.e. if any runtime error occurs at any particular line in the script then the control will move into the next line of the statement where the error has occurred. VBScript Questions and Answers Pdf Download Read the full article
0 notes
Text
The Cardio Kinesiograph System
Authored by *
Abstract
The Cardio Kinesiograph System (CKinG) is a novel computerized diagnostic system incorporating a computer model of cardiac kinesis. Cardiac Kinesis (CK) is the interpretation of heart movement or electrical activity of specialized cardiac muscle cells in response to biochemical reactions. The mechanical events occurring during the cardiac cycle consist of changes in pressure in the ventricular chamber which cause(s) blood to move in and out of the ventricle [1]. The events of the cardiac cycle start with an electrical signal and proceed through excitation-contraction coupling (which involves chemical and mechanical events) to contraction of the ventricle (pressure generation) and ejection of blood (flow) into the pulmonary and systemic circulations [2]. Thus, we can characterize the cardiac cycle by tracking changes in ventricular volume (LVV), ventricular pressure (LVP), left atrial pressure (LAP) and aortic pressure (AoP) [3]. In the other hand, because electrical events always precede mechanical events in the cardiac cycle, distortions of a part or parts of the electrical signal have been used as diagnostic indicators of both electrical and mechanical dysfunctions of the heart muscle [4]. There are numerous methods and technical systems available for diagnosis or predict heart disease. However, medical errors and undesirable results [5] in these systems are reasons for a need for conventional computer-based diagnosis of heart diseases systems. The purpose of this study is to design and develop a system that can observe and analyze Kinesiographical Cardiac basted on statistical models and identifies some fundamental characteristic of heart motions.
Keywords: Cardiovascular biomechanics; Statistical analysis methods; Medical heart imaging modalities; DNA modelization in biomedical image
Introduction
Since 1950 cardiologists have studied the functions of heart moments to employ them in the diagnostics of ischemic heart disease (IHD). Indeed, changes of the movements have found their diagnostic application in this field. If blood supply to a certain area of ventricular myocardium is insufficient the con-tractions in this area diminish and even ceases. After systolic increase in ventricular pressure this area dilates and forces intercostal tissues out, causing a “bulge” wave on the record.
Cardiokymography was one of several noninvasive techniques able to detect coronary artery disease. It can qualitatively determine abnormal left ventricular motion, and, based on animal models, this can be directly related to abnormalities in the left coronary artery [7]. Nevertheless sensitivity of cardiokymography in detecting patients with ischaemic left ventricular wall motion abnormalities depended on the extent of left ventricular ischaemia [8]. Cardiokymography is no longer valid [9]. Today Computer Aided Diagnosis (CAD) is one of the trusted methods in the field of medicine [10]. Advances in medical imaging and image processing techniques have greatly enhanced interpretation of medical images. Computer aided diagnosis (CAD) systems based on these techniques play a vital role [11] in the early detection of cardiovascular diseases and hence reduce death rate. CAD is the most preferable method for the initial diagnosis of heart disease. The combination of Digital and Medical Image Pro-cessing, Cardiac Electrophysiology, Ventricular Pressure-Volume Technique and Phonocardiogram etc makes the CAD system more reliable and efficient. One example of the medical applications in computer aided diagnosis is the detection system for heart disease based on Cardiovascular Magnetic Resonance (CMR). Cardiovascular magnetic resonance of the heart provides a potentially useful way to assess cardiac mechanical function. Besides CMR, positron emission tomography (PET), and cardiac CT are able to illustrate kinesis of cardiac muscles. Perfusion imaging with cardiac PET is used clinically to produce images of myocardial blood flow, aiding the diagnosis of coronary artery disease and the monitoring of condition of coronary circulation in response to treatment [12].
Clinical imaging in positron emission tomography (PET) is often performed using single-time-point estimates of tracer uptake or static imaging that provides a spatial map of regional tracer concentration [13]. However, dynamic cardiac techniques (e.g. PET, Myocardial perfusion imaging and Dynamic cardiac SPECT [14]) are used to estimate rate parameters activity of myocardial blood flow, and there are limited studies evaluating the role of Cardiovascular Magnetic Resonance and cardiac PET and cardiac CT for the assessment of cardiac kinesis. Therefore, scientific communities in computational cardiovascular science [15] have contributed to developing mathematical models and algorithms to improve efficiency of cardiac safety data management in clinical trials. In this way in 2014 proposed a new method based on a mathematical model, “Fourier Transform” which calculates an amplitude parametric image for the assessment of cardiac kinetics. This image, calculated from the Cine MR images, allows the localization and quantification of abnormalities related to difference in contraction and their extent [16]. In 2015 Zakynthinaki [17] has also presented effective mathematical model of heart rate kinetics in response to movement. She made conclusion that the new model is able not only to provide important information regarding an individual’s cardiovascular condition but to also simulate and predict heart rate kinetics for any given exercise intensities. The existing models of cardiac kinetics focus mainly on amplitude images or are limited to simulation of biological transformation. The present study provides a novel mathematical model of cardiac kinesis based on visual presentation of numerical data (obtained through the cardiac kinesis) in the form of graph, with a particular focus on Human Cardio Kinesiograph Analysis. The purpose of this study was to de-sign, develop and evaluate a novel method for Kinesiograph of Cardiac for the clinical assessment of cardiac and vascular function.
Methods and Materials
The system includes the following steps:
Data collection: In order to obtain an accurate data, the Cardiovascular Magnetic Resonance imaging (CMR), Cardiovascular Ultrasound or Cardiac Computed Tomography are produced better performances for detection of cardiac mobility. However, CMR is provided the most comprehensive anatomic picture for patient selection [18]. The system therefore is obtained relevant information from the CMR.
Video quality assessment: Different medical imaging methods may introduce common artifacts include image distortion, signal pileup (bright regions), and image dropout (area without signal) [19], therefore the quality assessment is an important factor at the operational level. The assessment of quality of video depends upon the type of distortion [20]. Numerous video quality assessment methods and metrics have been proposed over the past years with varying computational complexity and accuracy [21]. We utilize different forms of quality assessment methods, however the merit of these methods is often judged by assessing the quality of a set of results through lengthy user studies [22].
Motion estimation and inertial measurements: Motion estimation is the process of determining the movement of blocks between adjacent video frames (MathWorks). Efficient and accurate motion estimation is an essential component in the domains of image sequence analysis [23] and medical video processing. The estimation of motion is also important from the viewpoint of matching metric technique, which is computed the context similarity between two images. There exist several methods for motion estimation image, and video processing (e.g. pixel-based motion estimation, block-based motion estimation, optical flow method). In this study in order to increase the computational accuracy and improve efficiency in solving problem, DNA Modeling (Dawoudi, 2017) method in biomedical image matching has been proposed. The method is based on the linear mapping and the one-to-one correspondences between point features extracted from the frames and on calculating similarities in pixel values. This correspondence is determined by comparing two strings constructed from pixel values of the frames. The method uses a table called the Quarter Code table, which is the set of characters and numbers. In this table every number between 0 and 255 is translated into a unique string of four letter alphabet. Letters A,C,G,T are chosen, since they are the same as used in DNA sequences. In this way it possible to utilize tools originally programmed to DNA sequences analysis. When all pixel values of the frames (images) are converted to virtual DNA sequences, one can show the differences between two virtual DNA sequences.
Visual representation of numerical data in the form of graphs: The E-value gives a measure of the similarity of sequences. From this function we can obtain the correlation coefficient which will give us a single value of similarity. The rate of similarity between sequences (frames) is plotted as a graph and it’s appearing in the Monitor.
Experiment Results
We demonstrate the system by performing experiment 2D cardiac CMR video (Source: HBSNS library). The practical framework consists of four steps:
A. Step 1: Extract frames from cardiac mri video
The information is obtained by extracting frames from CMR imaging video. There are different tools in order to extract frames from cardiac MRI Video (e.g. Free Video to JPG Converter, VLC or Virtual Dud) (Figure 1).
B. Step 2: Adjacent frames comparisons
The difference between two adjacent frames is used to estimate motion direction and magnitude. This process has been implemented within a tool called Image Diff. The Perforce image diff tool enables researchers to compare two adjacent frames. The following represents pixels difference value (Percent Changed; Pixels) and color difference value (Percent Change; Color) between adjacent Frames (Table 1).
C. Step 3: Presenting data in graphic form
In the final step the percentage of change (pixels and color) from one value to another, between frames are plotted as a graph (Figure 2 & 3).
Results
The results show that the proposed design approach works efficiently in the Cardio Kinesis System for clinical applications of cardiovascular assessment.
Conclusion
In conclusion, the proposed Cardio Kinesiograph System (CKinG) may become a robust and efficient tool for the clinical assessment of Cardiac and Vascular function. CKinGbased Computer-Aided Diagnosis (CAD) holds the promise of improving the diagnosis accuracy and reducing the cost.
Discussion
The developed system can be used as a prototype in the clinical sectors for the evaluation of cardiovascular diseases. However, a novel method for real time MRI of cardiac kinesis and simultaneously Kinesiographical Cardiac Analysis Based on Statistical Methods are proposed. The proposed methods may become efficient Medical Diagnostic Support tools (DSTs) for Heart Diseases.
Related Work
The publications below are based on Morbid Motion Monitor related topics:.
A. Dawoudi Mohammad Reza (2017) Morbid Motion Monitor. Current Treads in Biomedical Engineering & Biosciences. ISSN: 2572-1151.
B. Dawoudi MR (in press) (2017). Nursing and Technology foresight in Futures of a Complex World. European Journal of Futures Research.
To Know More About Current Trends in Biomedical Engineering & Biosciences Please Click on: https://juniperpublishers.com/ctbeb/index.php
To Know More About Open Access Journals Publishers Please Click on: Juniper Publishers
#Cardiovascular biomechanics#Statistical analysis methods#DNA modelization in biomedical image#Biomedical Engineering#biochemistry
0 notes
Text
Mid-Sem BreakDown
t2019 Mid-sem paper: click Here
=== no guarantee of correct answer, these are just my self note ===
Part A
Question 0: (C) - Passwords must not be on a blacklist of common passwords
Question 1: (F) 60-69 bits of work
consider 10^3 = 2^10
Amount of work is 2*(10^18) = 2^60, but because it is on average so we divide by 2. Therefore amount of work is 2^59
It take 8 bits of work to test if one number divides into another, so we do 2^59 * 2^8 = 2^67 bits of work
Question 2: All not secure
Vigenere & OTP -> Vigenere by itself does not cap the letters of the politician’s name, so we can easily track the votes if the candidates have different length of names
//OTP and Vigenere might be safe enough with a padding and if used properly
SHA256 and 2048 -> Can be broken with a look up table. Since the public share the same public key, meaning everyone is able to encrypt the names and compare the encrypted informations
Question 3: A - 30 bits
Both A and B require 30 bits of work
2^30 to decrypt METHOD B, and another 2^30 to decrypt METHOD A.
2^30+2^30 = 2^31, which is still roughly 30 bits of work
Question 4: 1. Authenticity & 2. Non-repudiation/integrity Authenticity make sense... I don’t quite get the second part
authentication
variants on non repudiation or liveliness (apparently non rep once you say it / send information you cant take it back)
Question 5: (F) Type I/Type II error tradeoff
(if you need to launch the missile than you have to launch it) -> Single Point of failure
President - If only one person knows the launch code, you would be most worried about them forgetting it. This helps you to get an accidental, or unwanted firing of the missiles, but the president may also be unable to fire when want to.
Military - launch when not supposed to / not launch when supposed to launch
// note that the question says that your biggest worry as the country's head of military security
// Authentication is definitely not a problem here since only the president knows the secret code
// social engineering might make sense .. but I think we can safely assume presidents and government body are secuirty-aware people that they are very careful with providing information socially
Question 6: (A) Bump
Bump - have uniform ridges. (this is like a universal key)
Rake - remember when lock picking you have a tool that checks the randomness of the height and its almost like a brute force? its that one.
Shims - the one that’s in hotel room with round handle that allows you to open the space slightly but still locked. Shim is like a sheet of metal
Impressioning - put a flat blank key into the lock, make marks and cute it down little by little until it matches. This should sort of create an actual key of the lock (can even make an identical key without the original key!)
Question 7: (D) 6*6
order is known
6 heights and 6 different keys, so you have to try 6 times in each key and that is total of 6*6 different patterns at worst
// if you don’t know the sequence it will be 6! * (6*6) .... (?)
Question 8: (B) Social Engineering From the seminar: The most likely to have been used in the ransomware attack is social engineering
// e) rainbow table is for hashes
Question 9: (255) (255) (128) 255, 255, 128. The last one is due to birthday attack.
Question 10: No solution
c) caesar is too easy to crack
d) but d has to be private
// in this merkle puzzle, most of the words are the same except the key part, so vigenere and caesar is not ideal
Question 11: (A) Around 40 bits
Question 12: 6 Look for repetitions. The key for this one is SUNTZU.
0 notes
Text
POST MGT 211 Full Course Latest
Follow Below Link to Download File
https://homeworklance.com/downloads/post-mgt-211-full-course-latest/
We also Do 100% Original and Plagiarism Free Assignment / Homework and Essay
Email us for original and Plagiarism Free Work At ( [email protected] ) or order us at (https://homeworklance.com/custom-order/ )
Post MGT211 unit 1 Discussion latest
Our text and learning unit 1 lists and discusses several myths of entrepreneurship and small business Please complete the following HRB Quiz at:
http://blogshbrorg/2010/02/should-you-be-an-entrepreneur/
What surprised you as a result of completing the survey? Do you agree or disagree with the results? Explain
List and discuss three characteristics you believe to betrue about entrepreneurs and why you selected these characteristics
Post MGT211 unit 21 & 22 Discussion latest
21 Discussion
Finding and Using NAICS Numbers
Part 1 of the feasibility study requires you to identify the NAICS code of your proposed business concept As mentioned in this week’s learning unit, the North American Industry Classification System (NAICS) provides not only a system to classify your business but also provides valuable industry information and data, which is an invaluable tool to a startup or existing business In this discussion thread, you will practice using the NAICS site and discuss your findings with your classmates as presented in Skill Module 72 (Katz & Green)
What is your NAICS code? (Katz, pl 190) Please go to the updated site:
http://wwwcensusgov/cgi-bin/sssd/naics/naicsrch?chart=2012 In the text box that says “enter keyword” enter the name of the industry that best describes the industry in which your proposed business idea is located You should end up with a 6 digit code for the industry in which your business will be located If you are not sure of your industry, it may take some experimentation See examples in Skill module 72
Once you locate your NAICS code, go to wwwcensusgov and click on “economic census” Once you are in “economic census” enter your NAICS code and look for data related to your industry
Find and share one fact about your industry that you find particularly intersting and that is available at this cite such as number of establishments, annual revenue, trends, etc Also refer to Table 71 on page 200 for additional information and ideas
For your convenience, I am also attaching a table with the NAICS structure and numbers
2012_NAICS_Structurexls (255 KB)
22 Discussion
Not all good ideas represent profitable business opportunities Ideas must be tested and you will have an opportunity to do so in your feasibility study which is the primary written requirement for this course You will also be introduced to many free resources available to you in developing your feasibility study such as the SCAMPER acronym (Katz & Green) Tim Hayden (See Focus on Small Business, Katz & Green) developed a web-based application so that baseball fans could watch TV instant replays while enjoying a baseball game at a stadium Using the SCAMPER acronym, discuss at least two methods in the acronym that Tim may use to update his App to 2014? How will you use these methods in developing your feasibility study?
Post MGT211 unit 3 Discussion latest
Part 2 of your feasibility study will require you to describe your customer base This process requires careful research to identify the types and concentration of customers you will need to start and grow your business
Please go to Skill Module 112 on page 367 Following the directions in Skill Module 112 and using the site: wwwzipwhocom,
Please enter the state and city where your proposed business will be located and share at least three pieces of information describing your customer base such as education level, ethnicity, age, etc
Discuss how this information will assist you in completing Part 2 of your feasibility study
Post MGT211 unit 4 Discussion latest
Each year corporations spend millions of dollars for a few seconds of advertising during the football championship super bowl game Please watch the advertisements in the link below, which includes 15 advertisements shown during the 2014 super bowl game
Discuss the target market and market segment of at least four advertisements in terms of age group, gender, education, and any other marketing demographic information
http://wwwthedailybeastcom/articles/2014/02/02/the-15-best-super-bowl-2014-commercialshtml
Enjoy!
Post MGT211 unit 51 & 52 Discussion latest
51 Discussion
Locate your state’s Secretary of State home page on the Internet Find information on forming LLCs Answer the following questions and discuss your findings with your classmates
1 What state did you research?
2 Discuss reasons why you would or would not use the services of an attorney to assist you in submitting your application
52 Discussion
If a start-up entrepreneur lacks certain competencies such as understanding cash flow or not knowing how to use social media marketing techniques, using the BRIE Checklist (Katz & Green, p 14), discuss at least three strategies the start-up entrepreneur could use to compensate for his or her deficiencies You may also want to refer to Skill Module 22
Post MGT211 unit 6 Discussion latest
Read the case, Debbie Dusenberry and the Curious Sofa (Katz & Green, pp 409-10) Discuss the following concepts with your classmates
Discuss the differences between profit and cash flow
Will completing breakeven analysis (p 311) help Debbie? Why or why not?
Where or how can Debbie find help to save her business?
Post MGT211 unit 7 Discussion latest
View Table 82 on page 225 of our textbook Discuss the differences and similarities between conducting a feasibility study and writing a complete business plan
How can the conduct of a strong feasibility study help to minimize or avoid some of the most common critical risks in a business plan listed on p 238? Have you observed any of these risks in the parts of your feasibility study completed to date?
Where would you find funds to start a business? (See Table 151, p 489)
Post MGT211 unit 8 Discussion latest
An effective elevator speech is specific and has the capacity to “sell” your business concept quickly to your classmates who will play the role of venture capitalists The elevator pitch is designed to be brief but is a specific statement about your startup investment opportunity The idea is to be able to sell your idea to a potential investor in an elevator going up or down about 30 floors, about 2 minutes It should also include the amount of startup cash or resources you would be requesting of a potential investor Please refer to “The Thoughtful Entrepreneur” (p 242) and to the notes in the “Written Assignments” in the Course Information Tab
Post MGT211 FS Part 1 The Business Concept assignment latest
Part 1 Due by Sunday, 11:59 pm
IFeasibility Study
According to the research, a number of critical factors are important for a new venture’s success and must be assessed in a systematic way prior to investing additional time and one’s other resources Those factors are summarized in “Exhibit 42, The Feasibility Study Outline” in the textbook, pages 93-96 and in the Appendix, Sample Feasibility Study, pages 95-98 Your feasibility study assignment will consist of four (4) written parts designed to assess the initial financial viability of your business concept Each written component should be two pages in length and single spacedThe page limitation is designed to encourage you be brief but specific Part four will require the completion of two spreadsheets that will be linked or attached to that assignment
The feasibility analysis is a prelude to writing a business plan, which is more detailed and thoroughly researched and is required if a bank loan or investment funds are being sought The purpose of the feasibility study is to assess whether or not a good idea represents a profitable business opportunity and is designed to gain sufficient information and data to provide you with a a “go or no-go” decision in moving forward in completing a full business plan
For each assignment, please include the Part number, eg Part 1, Part 2, etc; and please include the title of each lettered subsection in each assignment, eg a b c etc Also make certain that your name is on the top of the pageand that your electronic submission includes your name at the beginning of the submission title eg Smith, J Part 1For your convenience, I have also included text page numbers discussing the various feasibility topics The assignments are as follows:
Assignment 1
Part 1The Description of your Business Concept (2 pages single-spaced, please include subheadings) 10%
1. Describe your product or service in a paragraph or two Ref pages 274-279
2. Present the Mission of your business, eg fundamental reason for being; and a Vision statement, what you hope your business will become
3. Describe the features or cost benefits of your product or service Ref pages 188-197
4. Describe the value benefits of your product or service Eg why would someone pay for or buy your product/service over your competitors Ref pages 188-197
5. Will your business be 1) an online business; 2) an on-ground business; or, 3) a combination of both? Explain
6. Provide the six-digit NAICS (North American Industrial Classification System) number for your business NAICS numbers may be found at the following site:Error! Hyperlink reference not validcensusgov/cgi-bin/sssd/naics/naicsrch?chart=2012″>http://wwwcensusgov/cgi-bin/sssd/naics/naicsrch?chart=2012
Locate the “keyword box” in the upper left hand corner and enter a keyword describing your business, eg health food, pet products and this will lead you to your NAICS number
Post MGT211 FS Part 2 Customer/Industry Analysis assignment latest
Part 2 Due by Sunday, 11:59pm
Part 2 Customer/Industry Analysis (3-4 pages single-spaced, please include subheadings) 15%
1. Describe the industry within which your business will be located and describe at least three recent trends in that industry Note:This information and item b following may be found using your NAICS number and in trade organization publications Also, see Skill Modules 31, p 54) and 72 (p 190) And pages 18-190 and NAICS link above in assignment 1
2. Describe the market growth rate for your product/service, ie, what is the market demand likely to be in the next 1-3 years You may also try using Google or Bing for this information or trade associations (Skill Module 31, p54) for your proposed business idea
3. Describe your market segment and your market niche within that market segment, ie describe your customers, who they are and where they are located Ref pages 393-95
4. Describe at least three of your competitors, why they are competitors and where they are located
5. Where will you locate your business? If a wholly online business what will you do to attract customers?
6. How will you market or promote your product/service? Ie, online sales, social media marketing, search engine optimization, personal selling, etc Describe in detail
Post MGT211 FS Part 3 Management Team and Operations assignment latest
Part 3Due by Sunday, 11:59 pm
Part 3 Management and Operations (2 pages single-spaced, please include subheadings) 10%
1. Describe who will be the CEO and his/her qualifications/experiences in the product/service industry, including both education and work and other relevant experiences
2. Describe briefly how you will distribute your product or service Ref pages 348-358
3. Describe how many employees you will need at startup, their particular expertise (eg marketing, financial, operations, etc), and how they will be paid
4. Describe what types of external advisors you will need and how they will be paid (eg lawyer, accountant, etc)
5. Describe the legal organization of your business (eg sole proprietor, LLC, Partnership, C Corporation, S Corporation) and reasons for selecting this legal organization form Ref page 600, Table 185
6. Describe unpaid advisors who will assist you during startup and 3 years thereafter
Post MGT211 FS Part 4 Financial Analysis and Requirements assignment latest
Part 4 Due by Sunday, 11:59pm
Part 4 Financial Analysis and Requirements (3 pages single-spaced plus spreadsheets, please include subheadingPlease include a link to your spreadsheet or include as attachments to part 4 document Thank you!)
1. Describe your pricing strategy for your product/service Ref Table 91 page 295
2. Describe the amount of startup funds you will need, where you will get them, and how they will be used
3. List 3-5 assumptions governing your cash flow projections For a startup, assumptions are your “best educated guesses” regarding goals such as how many units you will sell and why, or what your cash flow will be at the end of each month and why, etc will you pay yourself, what will you pay your employees, will you use full or part-time employees, what services will you outsource or contract out, etc
4. Complete your startup sources and uses of funds using the “Opening Day Balance Sheet”(third bullet point under “Finance Templates”) on the following website:
Error! Hyperlink reference not validscoreorg/resources/business-plans-financial-statements-template-gallery”>http://wwwscoreorg/resources/business-plans-financial-statements-template-gallery(see notes below)
1. Develop a one-year projected (pro forma) cash flow statementusing the Cash Flow Statement (12 months)at the (eighth bullet point under “Finance Templates) on the following website:
Error! Hyperlink reference not validscoreorg/resources/business-plans-financial-statements-template-gallery”>http://wwwscoreorg/resources/business-plans-financial-statements-template-gallery(see notes below)
Notes:
Financial Worksheets on the SCORE website:
The SCORE Opening Day Balance Sheet and Cash Flow Statement (12 months) worksheets are “live” excel worksheets and all you need to do is to (1) read the introductory information, (2) enter your company name and (3) complete the spreadsheets indicated Once you get into the website, under “Finance Templates” click the third bullet for the Opening Day Balance Sheetand click on the eighth bullet for the Cash Flow Statement (12 months)Make certain that you have a full page view in order to see the full page These worksheets are active and include appropriate formulae You just need to insert your projected numbers When you click on the worksheet tabs, go to the “review” panel in excel and “unprotect” the worksheets This will give you working access and then you will be able to save your completed worksheet as your own in your files
Opening Day Balance Sheet (or Sources and Uses of Funds)
The Balance Sheet is a snapshot of your financials at a given point in time In this case, it will on the first day of start-up, representing your pro forma projections of how much you will need to start your business and where the funds will come from The Balance Sheet is a statement of an owner’s assets, liabilities, and equity and should balance; that is, assets must equal liabilities plusthe owner’s equity This balance sheet is active as well, and your assets, liabilities, and equity should be based on your best case projections and subsequently are reflected in your Cash Flow Statement worksheet You just need to insert your numbers and, as above, you don’t have to use all items, if not appropriate to your business
Cash Flow Statement (12 months)
The Cash Flow Statement worksheet projects your business’ cash inflows and outflows over a given period of time (in this case, one year, Jan 1, 20xx to December 31, 20xx) and should reflect your assumptions for growth during that time period Please indicate your assumptions in an attachment to the worksheets Along with your assumptions, include when you project your breakeven point to be; that is, the time period after which you project a profit (and how you calculated your breakeven point) You do not have to use all items listed in spreadsheet Use only those items that may be appropriate to your business
Note: I am providing these SCORE “Business Plans & Financial Templates for your convenience You can create your own excel spreadsheets or find and use other similar templates
Post MGT211 Final Examination assignment latest
This final examination is comprehensive based on the Red Jett Sweets, Inc business plan and is to be completed using the essay format It is worth 30% of your final grade The final is due by Sunday at midnight EST.
0 notes
Text
Wouxun KG-UVN1 Dual Band DMR Review
youtube
MAIN FUNCTIONS
3072 Channels
1024 Contacts
255 Receiving Groups
250 Zones
16 Scan List
Radio ID
Radio TX Contacts
Duan Band and Dual Display
Radio Color Code
Support Frequency Mode
Program Key
Digital Voice Encryption
Emergency Alarm
VOX Function
Priority Channel
Scan TX Designated Channel
Low Battery Power Off
One Touch Access / Talk Around
Radio Program Password
Menu Encryption or Menu Hide
Power Save Mode
Group Scan
Tail Elimination
Text Message
Radio Disable or Enable
Lone Work
ARTS Function
Flash Light
Call Alert
CTCSS Encoding and Decoding , Support Non Standard
Digital Channel Recording , Continuous Record 8 Hours
Play Recording File
Customerized Radio Interface
DTMF Encoding and Decoding
Transmit Time-out Alert
FM Radio
Fimware Upgradable
TECHNICAL SPECIFICATION
Intergration Frequency Range 136-174MHz , 400-480MHz , (FM) 65-108MHz Channel Number 3072 Operating Temperature -20℃~+40℃ Antenna Resistance 50Ω Working Voltage 7.4 VDC Weight 300 g Size 64 x 41 x 130 (mm)
Receiver Analog Receive Sensitivity 0.25µV@12dB SINAD Digital Receive Sensitivity 0.25µV(BER:5%) Adjacent Channel Selectivity ≤[email protected] Audio Response +1dB~-3dB Audio Distortion ≤5% Audio Power 1W Inter Modulation ≥65dB
Transmitter FM Modulation 12.5KHz : 11KOF3E , 25KHz : 16KOF3E 4FSK Digit Modulation 12.5KHz(Data Only) : 7K60FXD 12.5KHz(Data+Voice) : 7K60EXE Adjancent Channel Power ≤-60dB Frequency Stability ±1 PPM Audio Distortion ≤5% Vocoder Type AMBE+2TM Audio Response +1dB~-3dB Digital Protocol ETSI-TS102 , 361-1 , 361-2 , 361-3 Bit Error Ratio ≤5% Modulation Limit ±[email protected] Output Power UHF(H : 4W , L : 1W) , VHF(H : 5W , L : 1W)
(adsbygoogle = window.adsbygoogle || []).push({});
Antenna

Handy 5 Elements 1200 Mhz Twin Loop Portable Antenna
February 15, 2019 No comments
Description Overview It will be 5 elements twin loop antenna of amateur radio 1200Mhz band for mobile. Decomposed and we try to carry. Connector will... Read more
Six-Band HF Center-Loaded Off-Center-Fed Dipole by ON4AA
March 02, 2015 No comments
Design goals Like many fellow radio amateurs, I own a fairly standard shortwave radio station consisting of a 100W HF transceiver driving a 1kW... Read more

THE COKE LOOP Antenna by PY1AHD
January 10, 2015 No comments
“The Coke Loop is a Small Magnetic Loop Antenna tuned by a trombone-style variable capacitor made with standard soda cans. In Brazil we... Read more

AG6IF BUILT 3DFL, 2 METER FULL WAVE HAM RADIO ANTENNA, WORKS ON 440 70CM ALSO
September 22, 2015 No comments
You are looking at a AG6IF built 2 meter ham radio antenna. 3 dimensional folded Full wave loop design. Rugged and solid with soldered copper connect... Read more
Equipment

FTDX101 Series Catalog
April 19, 2019 No comments
Read more

Fifine Condenser Microphone K669B Review/Test
April 19, 2019 No comments
Plug and play USB recording microphone with 5.9-Foot USB Cable included for computer PC laptop that connects directly to USB port for record music,com... Read more

ALT-512 QRP TRANSCEIVER
April 17, 2019 No comments
ALL-MODE QRP TRANSCEIVER for HF + 6m +4m (12 Bands + General Coverage RX) MADE IN EUROPE (Coming Soon*) Aerial-51’s new A... Read more

FTDX101D Operation Manual
April 12, 2019 No comments
FTDX101D Operation Manual (1904D-BS) ( 17.82 MB ) Amateur Radio \ Brochures \ HF/Satellite Transceivers and Amps FTDX101D Front Waterfall Pr... Read more
(adsbygoogle = window.adsbygoogle || []).push({});
News

Three BIRDS Constellation CubeSats Delivered to ISS for Orbital Deployment
April 23, 2019 No comments
A Cygnus resupply mission to the International Space Station (ISS) on April 11 also delivered three CubeSats of the BIRDS-3 constellation and three ot... Read more

International Marconi Day
April 22, 2019 No comments
International Marconi Day celebrates the huge part Guglielmo Marconi played in the invention of radio. IMD is a 24 hour amateur radio event that is he... Read more

Icom IC-9700 Review, Demo And Walkthrough
April 18, 2019 No comments
Icom IC-9700 Here it is! The all new Icom IC-9700 tri-band direct sampling transceiver. This is a most impressive rig and I will be showing many more... Read more

“Mentoring the Next Generation” is Hamvention and ARRL 2019 National Convention Theme
April 17, 2019 No comments
With an eye toward helping new and inexperienced hams enjoy the full range of activities that Amateur Radio has to offer, Hamvention® and the ARRL 201... Read more
VHF

My best Satellite Demo Ever – 18 QSOs
April 21, 2019 No comments
Read more

Icom IC-9700 First Impression, Comparision with IC-7400
April 06, 2019 No comments
Icom IC-9700 Specifications General Frequency coverage 144.000–148.000, 430.000–450.000, 1240.000–1300.000 MHz Mode SSB, CW, RTTY, AM, FM, DV, DD Numb... Read more

Talking through a satellite from 15 feet apart
April 01, 2019 No comments
“Andrew Knafel, KN8FEL saw my videos and through our correspondence I helped him learn how to operate satellites. He and his family live in Ohio... Read more

Introduction to the IC-SAT100 Satellite PTT Radio
March 28, 2019 No comments
Video introduction from Icom Inc. to the IC-SAT100 which is Icom’s first Satellite PTT handheld radio that uses the Iridium satellite communication ne... Read more
The post Wouxun KG-UVN1 Dual Band DMR Review appeared first on QRZ Now - Amateur Radio News.
from DXER ham radio news http://bit.ly/2DtE7nw via IFTTT
0 notes
Text
#Devember 2018 - days 11&12&13
I’ve studied things to implement SFX for hits. I was thinking about using a physics materials matrix, or creating a custom component with an audio type member. Than I would like to combine many sub-sounds to make SFXs non-repetitive. I’m still undecided about what to do. I could try to raytrace the hitpoint to get the material, but I cound hit something else and play a wrong sound. I could send two sound types as 2 bytes, into the gameplay event of weapon hit, but then GFX could become GameplayFX instead of GraphicsFX, and the sounds would be generated diffetent for each player. I could send a seed together with the types so that I could generate the same sounds, but it would be a lot of data. To reduce the data sent I’ve worked on the normals. A hit has a normal that gets sent to the clients. I’ve experimented with three methods to compress the normals.
The first one is the simplest: linearly normalize the values of the coordinates to the range 0-255. This uses 3 Bytes.
The second one uses 2Bytes, sending only x in 0-255 range, y in 0-127 range, the sign of z in the last bit of Y. Z is derived using the constraint that the normal is of length 1.
The third is the more complex and in theory the more precise. It uses 3 Bytes, but instead of using only the value combinations laying on the cyrcumpherence in the 0-255 3D space, it uses the most values in that 3D space. Simply the 3Bytes give a 3D vector that is then normalized, getting back the normal.
I derive the value of the Bytes using the Bresenham algotithm to get a set of points, of which I keep the one that gives the smallest error. I read about this idea here: https://kwasi-ich.de/blog/2012/10/03/bytenormals/ and got the codefor Bresenham here: https://gist.githubusercontent.com/yamamushi/5823518/raw/10a1065ddb1db4f2210d3ba9989e1e87998ca612/bresenham3d
Testing the 4 versions ( 3 + the float one ), it seems that all 4 give un-distinguishable graphic results. So I’m using the 2Bytes one, getting from 12Bytes to 2Bytes. 10Bytes gained per shot hit. You can get the code here: https://bitbucket.org/theGiallo/km0/src/8c9c32964fd6a12bd2c17d0fbcc3b0174694d653/proto00/7DFPS-proto00/Assets/scripts/tracking/Gameplay_Event.cs?at=F%2Fgameplay_events#lines-177
0 notes
Text
Licensed Emergency Plumber Elmhurst Illinois 60126
We stand by the plumber services we offer and our 24 hour emergency plumbing contractor appointments will always be precise and ready to diagnose customers needs. When you do not have hot watered in a petrol heater, it could inspection of your system. Are you worried about the corrosion in your you see in bathtubs and ... Some may wonder why we call our team of hour then use the best 24 Hour Plumbers near you. When they come up, you fumble with the telephone directory looking service 24/7 when you have an emergency on your hands! A 24 hour plumber is a plumber that not only has normal business hours, Looking for a plumber near you do have to be a hard task. So it's now a great time for people who want also do not need to have the preliminary training or the knowledge necessary to join this course. A 24 hour plumber is a plumber that not only has normal business hours, leaky tap, we always do quality work at a fair price.
Emergency Plumbing In My Area Elmhurst Illinois 60126
Most meetings are held at Wilder Mansion to making a decision based on these results. Money-wise, fastens war chest lags far behind that of the incumbent, according... 551 West Babcock Avenue, Elmhurst, I 60126View this property at 551 West Babcock Avenue, Elmhurst, I 60126 283 South Fair Avenue, Elmhurst, I 60126View this property at 283 South Fair Avenue, Elmhurst, I 60126 706 South Barkley Avenue, Elmhurst, I 60126View this property at 706 South Barkley Avenue, Elmhurst, I 60126 428 East At water Avenue, Elmhurst, I 60126View this property at 428 East At water Avenue, Elmhurst, I 60126 280 East Saint Charles Road, Elmhurst, I 60126View this property at 280 East Saint Charles Road, Elmhurst, I 60126 280 East Saint Charles RoadElmhurstI60126 235 East Oneida Avenue, Elmhurst, I 60126View this property at 235 East Oneida Avenue, Elmhurst, I 60126 526 South York Street B, Elmhurst, I 60126View this property at 526 South York Street B, Elmhurst, I 60126 0S611 Cedar Street, Elmhurst, I 60126View this property at 0S611 Cedar Street, Elmhurst, I 60126, Elmhurst, I 60126View this property at, Elmhurst, I 60126 189 North Elm Street, Elmhurst, I 60126View this property at 189 North Elm Street, Elmhurst, I 60126 1 Elm Creek Drive #1014, Elmhurst, I 60126View this property at 1 Elm Creek Drive #1014, Elmhurst, I 60126 255 North Addison Avenue #428, Elmhurst, I 60126View this property at 255 North Addison Avenue #428, Elmhurst, I 60126 255 North Addison Avenue #428ElmhurstIL60126 Lombard, on probation, was charged with criminal damage to state-supported... Fellows elected as if left without repair can end up costing thousands over time. mayor. 1957 - Benjamin between compliance and pupil engagement. View Caldwell Banker Residential Brokerage Elmhurst real estate agents bowel obstruction and talk to your doctor about chats right for you. In the past month, 47 homes participate as much A Plumbing Inspector from the City of city was dispatched yesterday to investigate as they are able! Some properties which appear for sale on this website may and staff member with a #findkindatJackson T-shirt. We're here to help you manage your money today and tomorrow Choose the checking account that works (Regional Universities Midwest) #9 U.S. In the recent past they have been joined by to offer great medical care -- but some do a better job than others. If you're looking to sell your home in the Elmhurst area, error. It's never too early the wait list and anticipate we will be able to do that again this year.
Hot Water Heater Installation
Like.n stove top install or a built in oven install have a 85% chance there will be an issue that you leg into the tee. Homewyse strongly recommends that you contact reputable professionals for accurate assessments -- it's just a big empty tank, after all. We've saved enough in propane costs as little as $500 for a petrol heater install and could pay for a cheaper tank. So.hen you bid a Emergency Plumber city Services can unstop toilets, sinks, outside faucets, drainage job after a job with 1 or 2 or 3 issue's,You get gun shy and tend to maybe over price just to cover .It's hard to go up on a quite. my option consider a petrol water on deaned heater adopted either the 2006, 2009 or 2012 Uniform Plumbing Code (or in the case of California, the California Plumbing Code). Inc. all hardware and instructional material close at hand. As such, your water heater cost should you pay for. On the other hand, electric water heaters cost more to operate, you get what you pay for. Because the West Coast is in earthquake zones, all installations “UPGRADING YOUR INSTALLATION TO CODE AND PULLING A PERMIT” First Columbia Station does not require permits. I'm comfortable with a water heater because I'm a been tripped, it can also indicate a failure of the whole heating system. Next should be the heater transported home. This seemed really high to me and to your home (within 30 miles of a Home Depot store location) at no additional charge! Two; would be Where is 50 gal Bradford White installed for $1,850 with permit and everything.
youtube
Hot Water Heater Installation
Actually, it occurs regularly can get reabsorbed by the body, causing the cyst to shrink and heal gradually. Clogged drains are one of the most common drying the stone. Simply pour this mixture through the drain ignore minor signs like earache and clogging. Using the screwdriver, open up the back panel of the drain, and save any significant amount of damage from happening. If this is the problem with your machine, you will have to call in will take care of the problem. Step #3: Once you place the snake inside the drain, pull a and keep up a balance in the swimming pool. Use the machine to apply objects can also block drains. In case of a double sink, water and ladder the jets for at least 10 minutes. ? If you routinely maintain your sewer lines you may enhance their of all the chocolate residue. After which, ladder hot drain, then it will be easier to unclog it.
Sewer Rodding
The prep and manpower it takes to ready a drain line for lining is much more labour for job security the one who insists on replacing is looking for a sale. These machines are all very rugged and are available with the number of roof penetrations. You have an emergency sewer line catastrophe where your pipes are backed up, necessary procedures to fix the issues that you are experiencing with your sewer, pipes, and drains. Thebes never a convenient time to find out the pipe will be relentless, particularly with fast-growing trees that require lots of nutrients. (Photo courtesy of Angie's List member Valerie M. of Cleveland Heights, Ohio) If you get a clog in your home and suspect that a blocked best tools available to get the job done right the first time. Certain professionals opt for trench less sewer repairs, a technology installation to any new or existing plastic or copper pipework. If yore a plumber you know rod heavy usage a city sewer main may ladder full or surcharged. Costs for job clean-up and debris in fully slotted, half slotted and solid wall options and are manufactured to BA certificates no.11/H172. This product adds charge for nights, weekends, or holidays, so contact us today!
Sewer Line Plumber
You.ant.ants any objects falling into your biological systems such as the gastrointestinal tract . We have left our home to spend a few nights with standard sump pump and a grinder pump? The diaphragm switch is submersed in water but (kinetic energy) to an increase in pressure head A practical difference between dynamic and positive displacement pumps is how they operate under closed valve conditions. It happens with all facets, the more you save. Loss of prime is usually due to slurping noises? I can actually stand on my standard, black radon sump expected to be particularly suitable for handling sensitive biofluids. Assuming the service panel is also in the basement, you can put some dry lumber down or use a small that you can attach to either end of the pipes for your sump pump. If not installed this way it is possible that any remaining water in the discharge pipe, after the pump be part of your appliances unique operational noises. Mixed-flow pumps function as a compromise Know Before Buying a Sump Pump Have a serious flooding problem at home? Low cost to use costs zero until it problem in your home?
Faucet Repair
Reassemble.he tap and easily lead to large, expensive plumbing issues if left unchecked. A Phillips-head (+) and flat-head screwdriver (-); even if your tap uses Phillips-head screws, a flat-head screwdriver can be useful for prying Plumbers your own situation to help you get quickly on your way. If.he number starts with a four, can identify your product through several characteristics.For help to identify your product if you don't have the model number, click here . A compression tap has two screw handles, one for hot and seat washer. Water, water jobs harder than necessary, so fix this issue quickly and save yourself a headache. If water is getting under your loose fixtures and you need a replacement fast, Amazon.Dom and use it to find a replacement. Install new parts or a new tap/fixture to illustrated here. Temperature-sensitive LED lights tell your kids when the water is hot by glowing bright red, cartridge (sleeve), ceramic disk, and ball type. Establish a place to lay out hand tight with your wrench.Before reassembling the handle, it is a good idea to turn the water back on at this point to check for leaks.
You may also be interested to read
Sewer Rodding Services Lincoln Park Chicago Illinois
Sewer Rodding Lincoln Park Chicago
Real People ~ Real Reviews ~ Real Results Great Availablity, Scheduler Was Helpful Good Communication, Did A Great Job.
Unclogg- Sewer Rodding Lincoln Park Chicago IL
Unclogg- Sewer Rodding Lincoln Park Chicago IL
0 notes
Photo
New Post has been published on https://programmingbiters.com/stripe-payment-gateway-integration-using-php-and-jquery/
Stripe Payment Gateway Integration using PHP and Jquery
In the Stripe payment gateway integration process, the following functionality will be implemented.
The HTML form to collect credit card information.
Create Stripe token to securely transmit card information.
Submit the form with card details.
Verify the card and process charges.
Insert payment details in the database and status will be shown to the user.
Stripe Test API Keys Data
Before taking your Stripe payment gateway integration live, you need to test it thoroughly. To test the credit card payment process, the test API Keys are needed to generate in your Stripe account.
Login to your Stripe account and navigate to the API page.
Under the TEST DATA section, you’ll see the API keys are listed. To show the Secret key, click on Reveal test key tokenbutton.
Collect the Publishable key and Secret key to later use in the script.
Before you get started to implement Stripe payment gateway in PHP, take a look the files structure.
Create Database Table
To store the transaction details, a table needs to be created in the database. The following SQL creates an orders table in the MySQL database.
CREATE TABLE `orders` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(100) COLLATE utf8_unicode_ci NOT NULL, `email` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `card_num` bigint(20) NOT NULL, `card_cvc` int(5) NOT NULL, `card_exp_month` varchar(2) COLLATE utf8_unicode_ci NOT NULL, `card_exp_year` varchar(5) COLLATE utf8_unicode_ci NOT NULL, `item_name` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `item_number` varchar(50) COLLATE utf8_unicode_ci NOT NULL, `item_price` float(10,2) NOT NULL, `item_price_currency` varchar(10) COLLATE utf8_unicode_ci NOT NULL DEFAULT 'usd', `paid_amount` varchar(10) COLLATE utf8_unicode_ci NOT NULL, `paid_amount_currency` varchar(10) COLLATE utf8_unicode_ci NOT NULL, `txn_id` varchar(100) COLLATE utf8_unicode_ci NOT NULL, `payment_status` varchar(50) COLLATE utf8_unicode_ci NOT NULL, `created` datetime NOT NULL, `modified` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
Database Configuration (dbConfig.php)
The dbConfig.php file is used to connect and select the database. Specify the database host ($dbHost), username ($dbUsername), password ($dbPassword), and name ($dbName) as per your database credentials.
<?php //Database credentials $dbHost = 'localhost'; $dbUsername = 'root'; $dbPassword = '*****'; $dbName = 'codexworld'; //Connect with the database $db = new mysqli($dbHost, $dbUsername, $dbPassword, $dbName); //Display error if failed to connect if ($db->connect_errno) printf("Connect failed: %s\n", $db->connect_error); exit();
Stripe Checkout Form (index.php)
JavaScript At first, include the Stripe JavaScript library, for securely sending the sensitive information to Stripe directly from browser.
<!-- Stripe JavaScript library --> <script type="text/javascript" src="https://js.stripe.com/v2/"></script>
The jQuery library is used only for this example, it’s not required to use Stripe.
<!-- jQuery is used only for this example; it isn't required to use Stripe --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
In the JavaScript code, set your publishable API key that identifies your website to Stripe. The stripeResponseHandler()function creates a single-use token and inserts a token field in the payment form HTML.
<script type="text/javascript"> //set your publishable key Stripe.setPublishableKey('Your_API_Publishable_Key'); //callback to handle the response from stripe function stripeResponseHandler(status, response) if (response.error) //enable the submit button $('#payBtn').removeAttr("disabled"); //display the errors on the form $(".payment-errors").html(response.error.message); else var form$ = $("#paymentFrm"); //get token id var token = response['id']; //insert the token into the form form$.append("<input type='hidden' name='stripeToken' value='" + token + "' />"); //submit form to the server form$.get(0).submit(); $(document).ready(function() //on form submit $("#paymentFrm").submit(function(event) //disable the submit button to prevent repeated clicks $('#payBtn').attr("disabled", "disabled"); //create single-use token to charge the user Stripe.createToken( number: $('.card-number').val(), cvc: $('.card-cvc').val(), exp_month: $('.card-expiry-month').val(), exp_year: $('.card-expiry-year').val() , stripeResponseHandler); //submit from callback return false; ); ); </script>
HTML The following HTML form collects the user information (name and email) and card details (Card Number, Expiration Date, and CVC No.). For further card payment processing, the form is submitted to the PHP script (submit.php).
<h1>Charge $55 with Stripe</h1> <!-- display errors returned by createToken --> <span class="payment-errors"></span> <!-- stripe payment form --> <form action="submit.php" method="POST" id="paymentFrm"> <p> <label>Name</label> <input type="text" name="name" size="50" /> </p> <p> <label>Email</label> <input type="text" name="email" size="50" /> </p> <p> <label>Card Number</label> <input type="text" name="card_num" size="20" autocomplete="off" class="card-number" /> </p> <p> <label>CVC</label> <input type="text" name="cvc" size="4" autocomplete="off" class="card-cvc" /> </p> <p> <label>Expiration (MM/YYYY)</label> <input type="text" name="exp_month" size="2" class="card-expiry-month"/> <span> / </span> <input type="text" name="exp_year" size="4" class="card-expiry-year"/> </p> <button type="submit" id="payBtn">Submit Payment</button> </form>
Stripe PHP Library
The Stripe PHP library will be used to process the card payment. You can get all the library files in our source code, alternatively, you can download it from GitHub.
Validate and Process Payment (submit.php)
In this file, the submitted card details are validated and the charge is processed using Stripe PHP library.
Get token, card details and user info from the submitted form.
Include the Stripe PHP library.
Set your Publishable key and Secret key which we have created in Stripe Test API Data section.
Add customer to stripe using the user email and Stripe token.
Specify product details and create a charge to the credit card or debit card.
Retrieve the charge details that have previously been created.
If the charge is successful, the order and transaction details will be inserted in the database. Otherwise, an error message will be shown.
<?php //check whether stripe token is not empty if(!empty($_POST['stripeToken'])) //get token, card and user info from the form $token = $_POST['stripeToken']; $name = $_POST['name']; $email = $_POST['email']; $card_num = $_POST['card_num']; $card_cvc = $_POST['cvc']; $card_exp_month = $_POST['exp_month']; $card_exp_year = $_POST['exp_year']; //include Stripe PHP library require_once('stripe-php/init.php'); //set api key $stripe = array( "secret_key" => "Your_API_Secret_Key", "publishable_key" => "Your_API_Publishable_Key" ); \Stripe\Stripe::setApiKey($stripe['secret_key']); //add customer to stripe $customer = \Stripe\Customer::create(array( 'email' => $email, 'source' => $token )); //item information $itemName = "Premium Script CodexWorld"; $itemNumber = "PS123456"; $itemPrice = 55; $currency = "usd"; $orderID = "SKA92712382139"; //charge a credit or a debit card $charge = \Stripe\Charge::create(array( 'customer' => $customer->id, 'amount' => $itemPrice, 'currency' => $currency, 'description' => $itemName, 'metadata' => array( 'order_id' => $orderID ) )); //retrieve charge details $chargeJson = $charge->jsonSerialize(); //check whether the charge is successful if($chargeJson['amount_refunded'] == 0 && empty($chargeJson['failure_code']) && $chargeJson['paid'] == 1 && $chargeJson['captured'] == 1) //order details $amount = $chargeJson['amount']; $balance_transaction = $chargeJson['balance_transaction']; $currency = $chargeJson['currency']; $status = $chargeJson['status']; $date = date("Y-m-d H:i:s"); //include database config file include_once 'dbConfig.php'; //insert tansaction data into the database $sql = "INSERT INTO orders(name,email,card_num,card_cvc,card_exp_month,card_exp_year,item_name,item_number,item_price,item_price_currency,paid_amount,paid_amount_currency,txn_id,payment_status,created,modified) VALUES('".$name."','".$email."','".$card_num."','".$card_cvc."','".$card_exp_month."','".$card_exp_year."','".$itemName."','".$itemNumber."','".$itemPrice."','".$currency."','".$amount."','".$currency."','".$balance_transaction."','".$status."','".$date."','".$date."')"; $insert = $db->query($sql); $last_insert_id = $db->insert_id; //if order inserted successfully if($last_insert_id && $status == 'succeeded') $statusMsg = "<h2>The transaction was successful.</h2><h4>Order ID: $last_insert_id</h4>"; else $statusMsg = "Transaction has been failed"; else $statusMsg = "Transaction has been failed"; else $statusMsg = "Form submission error......."; //show success or error message echo $statusMsg;
PayPal Payment Gateway Integration in PHP
Test Card Details
To test the payment process, you need test card details. Use any of the following test card numbers, a valid future expiration date, and any random CVC number, to test Stripe payment gateway integration in PHP.
4242424242424242 Visa
4000056655665556 Visa (debit)
5555555555554444 Mastercard
5200828282828210 Mastercard (debit)
378282246310005 American Express
6011111111111117 Discover
Make Stripe Payment Gateway Live
Once testing is done and the payment process working properly, follow the below steps to make Stripe payment gateway live.
Login to your Stripe account and navigate to the API page.
Collect the API keys (Publishable key and Secret key) from Live Data.
Change the Test API keys (Publishable key and Secret key) with the Live API keys (Publishable key and Secret key) in the script.
0 notes