#HTMLl5 demos
Explore tagged Tumblr posts
Photo
Example of How to Add Google reCAPTCHA v3 to a PHP Form https://ift.tt/2UjFImN
5 notes
·
View notes
Photo
How to Create Your Own AJAX WooCommerce Wishlist Plugin
In this tutorial we will create lightweight wishlist functionality for WooCommerce using AJAX, WordPress REST API, and SVG graphics. WooCommerce doesn’t come with wishlist functionality as standard, so you’ll always need to rely on an extension to do the work for you. Unless you build it yourself for complete control..
Wish Upon a Star
Wishlist functionality will allow users to mark certain products, adding them to a list for future reference. In some eCommerce stores (such as Amazon) multiple wishlists can be created, and these can be shared with others, which makes them ideal for birthdays or weddings. In our case, the WooCommerce wishlist we’re going to create will enable customers to easily revisit products they’re considering.
Our wishlist functionality will add a heart icon to the product thumbs, which when clicked will add the product to a wishlist in a table.
Click the heart icon to add a product to the wishlist
Take a look at the demo for a proper idea of how it works.
1. Create the Plugin Structure
Let’s start by building our plugin. Create a folder with the name “wishlist” and a PHP file with the same name. Add the following snippet to the PHP file:
/* Plugin Name: Woocommerce wishlist Plugin URI: https://www.enovathemes.com Description: Ajax wishlist for WooCommerce Author: Enovathemes Version: 1.0 Author URI: http://enovathemes.com */ if ( ! defined( 'ABSPATH' ) ) { exit; // Exit if accessed directly }
We won’t go into detail about the plugin creation process, but if you are new to plugin development I highly recommend this amazing new course by Rachel McCollin:
WordPress
Introduction to WordPress Plugin Development
Rachel McCollin
Add the Plugin Functions
Let’s sketch out our plan so we know what to build:
Add wishlist toggle to products in loop and single pages using WooCommerce hooks
Create wishlist table shortcode to hold the products added to the wishlist
Create wishlist custom option in the user profile
All the plugin code will go inside the init action for the plugin, as we first need to make sure that the WooCommerce plugin is active. So right after the plugin details add the following code:
add_action('init','plugin_init'); function plugin_init(){ if (class_exists("Woocommerce")) { // Code here } }
And now let’s enqueue our plugin scripts and styles.
Add the following code to the main plugin file:
function wishlist_plugin_scripts_styles(){ wp_enqueue_style( 'wishlist-style', plugins_url('/css/style.css', __FILE__ ), array(), '1.0.0' ); wp_enqueue_script( 'wishlist-main', plugins_url('/js/main.js', __FILE__ ), array('jquery'), '', true); wp_localize_script( 'main', 'opt', array( 'ajaxUrl' => admin_url('admin-ajax.php'), 'ajaxPost' => admin_url('admin-post.php'), 'restUrl' => rest_url('wp/v2/product'), 'shopName' => sanitize_title_with_dashes(sanitize_title_with_dashes(get_bloginfo('name'))), 'inWishlist' => esc_html__("Already in wishlist","text-domain"), 'removeWishlist' => esc_html__("Remove from wishlist","text-domain"), 'buttonText' => esc_html__("Details","text-domain"), 'error' => esc_html__("Something went wrong, could not add to wishlist","text-domain"), 'noWishlist' => esc_html__("No wishlist found","text-domain"), ) ); } add_action( 'wp_enqueue_scripts', 'wishlist_plugin_scripts_styles' );
Here we enqueue the main style.css file and the main.js file for the plugin, also we pass some parameters to the main.js file to work with:
ajaxUrl – required to fetch some data from WordPress, like current User ID
ajaxPost – required to update user wishlist
restUrl – required to list the wishlist items in the wishlist table
shopName – required to add wishlist items to the session storage for non-registered or non-logged-in users
And some strings instead of hardcoding them into the js file, in case they need to be translatable.
So for now create a css, and js folder and put the corresponding files inside those folders: style.css in the css folder and main.js in the js folder.
2. Hook the Wishlist Toggle
Right inside the init action add the following code:
// Add wishlist to product add_action('woocommerce_before_shop_loop_item_title','wishlist_toggle',15); add_action('woocommerce_single_product_summary','wishlist_toggle',25); function wishlist_toggle(){ global $product; echo '<span class="wishlist-title">'.esc_attr__("Add to wishlist","text-domain").'</span><a class="wishlist-toggle" data-product="'.esc_attr($product->get_id()).'" href="#" title="'.esc_attr__("Add to wishlist","text-domain").'">'.file_get_contents(plugins_url( 'images/icon.svg', __FILE__ )).'</a>'; }
Here we add a wishlist toggle to each product in the loop and to each single product layout, using the woocommerce_before_shop_loop_item_title and woocommerce_single_product_summary hooks.
Here I want to point out the data-product attribute that contains the product ID–this is required to power the wishlist functionality. And also take a closer look at the SVG icon–this is required to power the animation.
3. Add SVG Icons
Now create an images folder in the plugin folder and put the following icon.svg in it:
<svg viewBox="0 0 471.701 471.701"> <path class="heart" d="M433.601,67.001c-24.7-24.7-57.4-38.2-92.3-38.2s-67.7,13.6-92.4,38.3l-12.9,12.9l-13.1-13.1 c-24.7-24.7-57.6-38.4-92.5-38.4c-34.8,0-67.6,13.6-92.2,38.2c-24.7,24.7-38.3,57.5-38.2,92.4c0,34.9,13.7,67.6,38.4,92.3 l187.8,187.8c2.6,2.6,6.1,4,9.5,4c3.4,0,6.9-1.3,9.5-3.9l188.2-187.5c24.7-24.7,38.3-57.5,38.3-92.4 C471.801,124.501,458.301,91.701,433.601,67.001z M414.401,232.701l-178.7,178l-178.3-178.3c-19.6-19.6-30.4-45.6-30.4-73.3 s10.7-53.7,30.3-73.2c19.5-19.5,45.5-30.3,73.1-30.3c27.7,0,53.8,10.8,73.4,30.4l22.6,22.6c5.3,5.3,13.8,5.3,19.1,0l22.4-22.4 c19.6-19.6,45.7-30.4,73.3-30.4c27.6,0,53.6,10.8,73.2,30.3c19.6,19.6,30.3,45.6,30.3,73.3 C444.801,187.101,434.001,213.101,414.401,232.701z"/> <g class="loading"> <path d="M409.6,0c-9.426,0-17.067,7.641-17.067,17.067v62.344C304.667-5.656,164.478-3.386,79.411,84.479 c-40.09,41.409-62.455,96.818-62.344,154.454c0,9.426,7.641,17.067,17.067,17.067S51.2,248.359,51.2,238.933 c0.021-103.682,84.088-187.717,187.771-187.696c52.657,0.01,102.888,22.135,138.442,60.976l-75.605,25.207 c-8.954,2.979-13.799,12.652-10.82,21.606s12.652,13.799,21.606,10.82l102.4-34.133c6.99-2.328,11.697-8.88,11.674-16.247v-102.4 C426.667,7.641,419.026,0,409.6,0z"/> <path d="M443.733,221.867c-9.426,0-17.067,7.641-17.067,17.067c-0.021,103.682-84.088,187.717-187.771,187.696 c-52.657-0.01-102.888-22.135-138.442-60.976l75.605-25.207c8.954-2.979,13.799-12.652,10.82-21.606 c-2.979-8.954-12.652-13.799-21.606-10.82l-102.4,34.133c-6.99,2.328-11.697,8.88-11.674,16.247v102.4 c0,9.426,7.641,17.067,17.067,17.067s17.067-7.641,17.067-17.067v-62.345c87.866,85.067,228.056,82.798,313.122-5.068 c40.09-41.409,62.455-96.818,62.344-154.454C460.8,229.508,453.159,221.867,443.733,221.867z"/> </g> <g class="check"> <path d="M238.933,0C106.974,0,0,106.974,0,238.933s106.974,238.933,238.933,238.933s238.933-106.974,238.933-238.933 C477.726,107.033,370.834,0.141,238.933,0z M238.933,443.733c-113.108,0-204.8-91.692-204.8-204.8s91.692-204.8,204.8-204.8 s204.8,91.692,204.8,204.8C443.611,351.991,351.991,443.611,238.933,443.733z"/> <path d="M370.046,141.534c-6.614-6.388-17.099-6.388-23.712,0v0L187.733,300.134l-56.201-56.201 c-6.548-6.78-17.353-6.967-24.132-0.419c-6.78,6.548-6.967,17.353-0.419,24.132c0.137,0.142,0.277,0.282,0.419,0.419 l68.267,68.267c6.664,6.663,17.468,6.663,24.132,0l170.667-170.667C377.014,158.886,376.826,148.082,370.046,141.534z"/> </g> </svg>
If you are new to working with SVGs I highly recommend you read these amazing tutorials on the subject:
SVG
How to Hand Code SVG
Kezz Bracey
SVG
SVG Viewport and viewBox (For Complete Beginners)
Kezz Bracey
Our SVG animation has 3 states:
Default: the heart path
Process: loading group (g tag)
End: check group (g tag)
If you now go to your shop page you will see the unstyled SVG icons piled on top of each other:
Let’s add some styling to fix this mess! Open the style.css file and paste the following code:
.wishlist-toggle { display: block; position: absolute; top: 16px; left: 16px; z-index: 5; width: 24px; height: 24px; outline: none; border:none; } .wishlist-title { display: none; } .entry-summary .wishlist-toggle { position: relative; top: 0; left: 0; display: inline-block; vertical-align: middle; margin-bottom: 8px; } .entry-summary .wishlist-title { display: inline-block; vertical-align: middle; margin-right: 8px; margin-bottom: 8px; } .wishlist-toggle:focus { outline: none; border:none; } .wishlist-toggle svg { fill:#bdbdbd; transition: all 200ms ease-out; } .wishlist-toggle:hover svg, .wishlist-toggle.active svg { fill:#000000; } .wishlist-toggle svg .loading, .wishlist-toggle svg .check { opacity: 0; } .wishlist-toggle.active svg .check { opacity: 1; } .wishlist-toggle.active svg .heart { opacity: 0; } .wishlist-toggle.loading svg .loading, .wishlist-table.loading:before { animation:loading 500ms 0ms infinite normal linear; transform-origin: center; opacity: 1; } .wishlist-toggle.loading svg .heart { opacity:0; } @keyframes loading { from {transform: rotate(0deg);} to {transform: rotate(360deg);} }
The logic here is as follows:
Initially we show the heart path of our SVG.
When the user clicks on it we will hide the heart path and show the loading path.
Once the loading finishes we will show the checkmark indicating that the product was successfully added to the wishlist.
We will toggle the loading state via JavaScript later; the loading animation is a simple transform rotate. So for now if you refresh the page (don’t forget to clear the browser cache as sometimes old styles are cached) you will see a nice heart icon with each product.
This toggle currently does nothing, so we’ll sort that out. But for now let’s keep with our plan.
4. Create Wishlist Table Shortcode
Add the following code in the init plugin action:
// Wishlist table shortcode add_shortcode('wishlist', 'wishlist'); function wishlist( $atts, $content = null ) { extract(shortcode_atts(array(), $atts)); return '<table class="wishlist-table loading"> <tr> <th><!-- Left for image --></th> <th>'.esc_html__("Name","text-domain").'</th> <th>'.esc_html__("Price","text-domain").'</th> <th>'.esc_html__("Stock","text-domain").'</th> <th><!-- Left for button --></th> </tr> </table>'; }
This is a very simple shortcode that you can add to any page, and the wishlist items will appear inside it. I won’t describe the shortcode creation process, but if you are new to this, I highly recommend reading this amazing tutorial:
Plugins
Getting Started With WordPress Shortcodes
Rohan Mehta
Make a Wishlist Page
Now from inside the WP admin create a page called “Wishlist” and put the [wishlist] shortcode inside it. Now if you go to the wishlist page you will see an empty table.
Did you notice the loading class on the table? We will remove the loading class with JavaScript later, once the wishlist items are ready to be appended to the table. But for now open the style.css and add the following code:
.wishlist-table { width:100%; position: relative; } .wishlist-table.loading:after { display: block; width: 100%; height: 100%; position: absolute; top: 0; left: 0; content: ""; background: #ffffff; opacity: 0.5; z-index: 5; } .wishlist-table.loading:before { display: block; width: 24px; height: 24px; position: absolute; top: 50%; left: 50%; margin-top:-12px; margin-left:-12px; content: ""; background-image: url('../images/loading.svg'); background-repeat: no-repeat; background-size: 100%; z-index: 6; } .wishlist-table td { position: relative; } .wishlist-table a.details { padding:4px 16px; background: #000000; color: #ffffff; text-align: center; border:none !important } .wishlist-table a.wishlist-remove { display: block; width: 24px; height: 24px; position: absolute; top: 50%; left: 50%; margin-top:-12px; margin-left:-12px; background-image: url('../images/remove.svg'); background-repeat: no-repeat; background-size: 100%; z-index: 6; border:none; opacity:0; } .wishlist-table td:hover > a.wishlist-remove { opacity:1; }
Add the loading.svg image to the images folder:
<svg xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" viewBox="0 0 471.701 471.701"> <path d="M409.6,0c-9.426,0-17.067,7.641-17.067,17.067v62.344C304.667-5.656,164.478-3.386,79.411,84.479 c-40.09,41.409-62.455,96.818-62.344,154.454c0,9.426,7.641,17.067,17.067,17.067S51.2,248.359,51.2,238.933 c0.021-103.682,84.088-187.717,187.771-187.696c52.657,0.01,102.888,22.135,138.442,60.976l-75.605,25.207 c-8.954,2.979-13.799,12.652-10.82,21.606s12.652,13.799,21.606,10.82l102.4-34.133c6.99-2.328,11.697-8.88,11.674-16.247v-102.4 C426.667,7.641,419.026,0,409.6,0z"/> <path d="M443.733,221.867c-9.426,0-17.067,7.641-17.067,17.067c-0.021,103.682-84.088,187.717-187.771,187.696 c-52.657-0.01-102.888-22.135-138.442-60.976l75.605-25.207c8.954-2.979,13.799-12.652,10.82-21.606 c-2.979-8.954-12.652-13.799-21.606-10.82l-102.4,34.133c-6.99,2.328-11.697,8.88-11.674,16.247v102.4 c0,9.426,7.641,17.067,17.067,17.067s17.067-7.641,17.067-17.067v-62.345c87.866,85.067,228.056,82.798,313.122-5.068 c40.09-41.409,62.455-96.818,62.344-154.454C460.8,229.508,453.159,221.867,443.733,221.867z"/> </svg>
This is the same loading SVG separated from the main icon.svg. We could use SVG sprites, but I decided to stick with a separate loading SVG.
Now, if you go to the wishlist page and refresh it you will see an empty table with loading on it. Nice, let’s move further.
5. Wishlist Custom Option in the User Profile
Our wishlist functionality will work both for logged-in users and guest users. With logged-in users we’ll store the wishlist information in the user’s metadata, and with guest users we’ll store the wishlist in the session storage.
You can also store the guest users’ wishlist in local storage, the difference being that session storage is destroyed when the user closes the tab or browser, and local storage is destroyed when the browser cache is cleared. It is up to you which option you use for guest users.
Now add the following code to the init action:
// Wishlist option in the user profile add_action( 'show_user_profile', 'wishlist_user_profile_field' ); add_action( 'edit_user_profile', 'wishlist_user_profile_field' ); function wishlist_user_profile_field( $user ) { ?> <table class="form-table wishlist-data"> <tr> <th><?php echo esc_attr__("Wishlist","text-domain"); ?></th> <td> <input type="text" name="wishlist" id="wishlist" value="<?php echo esc_attr( get_the_author_meta( 'wishlist', $user->ID ) ); ?>" class="regular-text" /> </td> </tr> </table> <?php } add_action( 'personal_options_update', 'save_wishlist_user_profile_field' ); add_action( 'edit_user_profile_update', 'save_wishlist_user_profile_field' ); function save_wishlist_user_profile_field( $user_id ) { if ( !current_user_can( 'edit_user', $user_id ) ) { return false; } update_user_meta( $user_id, 'wishlist', $_POST['wishlist'] ); }
Again, in order to remain within the scope of this tutorial, I won’t explain how to work with user metadata. If you are new to this I highly recommend reading this amazing tutorial:
WordPress
How to Work With WordPress User Metadata
Tom McFarlin
All we do here is create a text field input that will hold the wishlist items comma-separated IDs. With show_user_profile and edit_user_profile actions we add the structure of the input field, and with personal_options_update and edit_user_profile_update actions we power the save functionality.
So once the wishlist is updated it will save to the database. I you go to your profile page you will see a new text field added to it. Add whatever value you want and hit save to test if the update functionality works. With admin CSS you can hide this field if you don’t want users to see it. I will leave it as is.
6. Turn it On!
Now we are ready to power everything up!
Open the main.js file and put the following code in it:
(function($){ "use strict"; })(jQuery);
All our code will go inside this function.
Now let’s gather the required data and create some variables:
var shopName = opt.shopName+'-wishlist', inWishlist = opt.inWishlist, restUrl = opt.restUrl, wishlist = new Array, ls = sessionStorage.getItem(shopName), loggedIn = ($('body').hasClass('logged-in')) ? true : false, userData = '';
As you might remember when we enqueued our main.js script we passed some parameters to it. Here, with JavaScript, we can collect these parameters.
Next, we will create an empty wishlist array that will contains wishlist items. We will need the session storage data with our shop name (the ls variable stands for local storage), and we will need to know if the user is guest or logged-in.
Let me explain the logic here: whenever the user visits the shop page we will need to know if he or she is logged-in or is a guest-user. If the user is logged-in we will need to check if he or she has wishlist items, and if so highlight these items. If not we need to see if there are any items in the session/local storage and highlight those.
Why this is done like this? Imagine, if the user first visits the website as a guest, adds items to the wishlist, and then decides to login. If the user does not have items registered in the profile wishlist, we will need to show the ones that he or she added before login, that are stored in the session/local storage.
So let’s do that step by step:
If User is Logged-in
Fetch current user data with AJAX
If success update the wishlist
Highlight the wishlist items
Remove the session/local storage
If fail show error message in the console for the developer
if(loggedIn) { // Fetch current user data $.ajax({ type: 'POST', url: opt.ajaxUrl, data: { 'action' : 'fetch_user_data', 'dataType': 'json' }, success:function(data) { userData = JSON.parse(data); if (typeof(userData['wishlist']) != 'undefined' && userData['wishlist'] != null && userData['wishlist'] != "") { var userWishlist = userData['wishlist']; userWishlist = userWishlist.split(','); if (wishlist.length) { wishlist = wishlist.concat(userWishlist); $.ajax({ type: 'POST', url:opt.ajaxPost, data:{ action:'user_wishlist_update', user_id :userData['user_id'], wishlist :wishlist.join(','), } }); } else { wishlist = userWishlist; } wishlist = wishlist.unique(); highlightWishlist(wishlist,inWishlist); sessionStorage.removeItem(shopName); } else { if (typeof(ls) != 'undefined' && ls != null) { ls = ls.split(','); ls = ls.unique(); wishlist = ls; } $.ajax({ type: 'POST', url:opt.ajaxPost, data:{ action:'user_wishlist_update', user_id :userData['user_id'], wishlist :wishlist.join(','), } }) .done(function(response) { highlightWishlist(wishlist,inWishlist); sessionStorage.removeItem(shopName); }); } }, error: function(){ console.log('No user data returned'); } }); }
If User is Guest
Fetch wishlist from the session/local storage
else { if (typeof(ls) != 'undefined' && ls != null) { ls = ls.split(','); ls = ls.unique(); wishlist = ls; } }
As you may have noticed here we have double-AJAX and some helper functions. So first let’s create the actions of the AJAX requests, and after that I will explain our helper functions. I won’t describe in detail the AJAX functionality in WordPress, but if you are new to AJAX and WordPress, I highly recommend reading this amazing tutorial on it:
Plugins
A Primer on Ajax in the WordPress Frontend: Understanding the Process
Tom McFarlin
Our first AJAX request gets the user id and the user wishlist data from WordPress. This is done with a custom AJAX action added to the plugin code file:
// Get current user data function fetch_user_data() { if (is_user_logged_in()){ $current_user = wp_get_current_user(); $current_user_wishlist = get_user_meta( $current_user->ID, 'wishlist',true); echo json_encode(array('user_id' => $current_user->ID,'wishlist' => $current_user_wishlist)); } die(); } add_action( 'wp_ajax_fetch_user_data', 'fetch_user_data' ); add_action( 'wp_ajax_nopriv_fetch_user_data', 'fetch_user_data' );
The most important part here is the action name (fetch_user_data)–make sure it is the same for AJAX and for functions wp_ajax_fetch_user_data and wp_ajax_nopriv_fetch_user_data. Here we’re preparing JSON formatted data with user ID and user wishlist data.
Our next AJAX request updates the user wishlist if there were already wishlist items from session/local storage. Take a close look at the url option–see it is different.
The logic is the same as for the first action–the difference is that here we don’t return or echo any data, but we update the wishlist option for the current user.
function update_wishlist_ajax(){ if (isset($_POST["user_id"]) && !empty($_POST["user_id"])) { $user_id = $_POST["user_id"]; $user_obj = get_user_by('id', $user_id); if (!is_wp_error($user_obj) && is_object($user_obj)) { update_user_meta( $user_id, 'wishlist', $_POST["wishlist"]); } } die(); } add_action('admin_post_nopriv_user_wishlist_update', 'update_wishlist_ajax'); add_action('admin_post_user_wishlist_update', 'update_wishlist_ajax');
And if our user is a guest we will need to check if there are any wishlist details in the session/local storage.
Helper Functions
Before we move to the events part I want to explain our helper functions
Array.prototype.unique = function() { return this.filter(function (value, index, self) { return self.indexOf(value) === index; }); } function isInArray(value, array) {return array.indexOf(value) > -1;} function onWishlistComplete(target, title){ setTimeout(function(){ target .removeClass('loading') .addClass('active') .attr('title',title); },800); } function highlightWishlist(wishlist,title){ $('.wishlist-toggle').each(function(){ var $this = $(this); var currentProduct = $this.data('product'); currentProduct = currentProduct.toString(); if (isInArray(currentProduct,wishlist)) { $this.addClass('active').attr('title',title); } }); }
The first helper function makes the array unique, by removing duplicates, the second one checks if the given value is present in the given array. The next function executes when an item is added to the wishlist and the last one shows items that are in the wishlist.
Add Toggle
Now let’s add a click event to the wishlist toggle to power the actual functionality. On each toggle click event the animation is triggered and if the user is logged-in the wishlist update action fires with AJAX. If the user is a guest the item is added to the session/local storage.
Now if you go to the shop page, refresh the browser, and click on any wishlist toggle you will see it is working!
$('.wishlist-toggle').each(function(){ var $this = $(this); var currentProduct = $this.data('product'); currentProduct = currentProduct.toString(); if (!loggedIn && isInArray(currentProduct,wishlist)) { $this.addClass('active').attr('title',inWishlist); } $(this).on('click',function(e){ e.preventDefault(); if (!$this.hasClass('active') && !$this.hasClass('loading')) { $this.addClass('loading'); wishlist.push(currentProduct); wishlist = wishlist.unique(); if (loggedIn) { // get user ID if (userData['user_id']) { $.ajax({ type: 'POST', url:opt.ajaxPost, data:{ action:'user_wishlist_update', user_id :userData['user_id'], wishlist :wishlist.join(','), } }) .done(function(response) { onWishlistComplete($this, inWishlist); }) .fail(function(data) { alert(opt.error); }); } } else { sessionStorage.setItem(shopName, wishlist.toString()); onWishlistComplete($this, inWishlist); } } }); });
7. List Items in Wishlist Table
Now it is time to list our wishlist items in the wishlist table we created earlier.
Add the following code into main.js at the very bottom of our wrapper function:
setTimeout(function(){ if (wishlist.length) { restUrl += '?include='+wishlist.join(','); restUrl += '&per_page='+wishlist.length; $.ajax({ dataType: 'json', url:restUrl }) .done(function(response){ $('.wishlist-table').each(function(){ var $this = $(this); $.each(response,function(index,object){ $this.append('<tr data-product="'+object.id+'"><td><a class="wishlist-remove" href="#" title="'+opt.removeWishlist+'"></a>'+object.image+'</td><td>'+object.title["rendered"]+'</td><td>'+object.price+'</td><td>'+object.stock+'</td><td><a class="details" href="'+object.link+'">'+opt.buttonText+'</a></td></tr>'); }); }); }) .fail(function(response){ alert(opt.noWishlist); }) .always(function(response){ $('.wishlist-table').each(function(){ $(this).removeClass('loading'); }); }); } else { $('.wishlist-table').each(function(){ $(this).removeClass('loading'); }); } },1000);
Here we are using the WordPress REST API to get the products by ID in the wishlist array.
For each of the products we get we are adding a table row with the required data to display. We need the product image, title, stock status, button and price.
Here we have two options for the REST API:
using the WordPress REST API
using the WooCommerce REST API.
The difference here is that product data is already present in the Woocommerce REST API, but an API key is required. With the default WordPress REST API product data is absent by default, but can be added, and no API key is required. For such a simple task as a wishlist I don’t think that an API key is needed, so we will do it by extending the default WordPress REST API to return our product price, image code and the stock level.
Go to the main plugin file and at the very bottom add the following code:
// Extend REST API function rest_register_fields(){ register_rest_field('product', 'price', array( 'get_callback' => 'rest_price', 'update_callback' => null, 'schema' => null ) ); register_rest_field('product', 'stock', array( 'get_callback' => 'rest_stock', 'update_callback' => null, 'schema' => null ) ); register_rest_field('product', 'image', array( 'get_callback' => 'rest_img', 'update_callback' => null, 'schema' => null ) ); } add_action('rest_api_init','rest_register_fields'); function rest_price($object,$field_name,$request){ global $product; $id = $product->get_id(); if ($id == $object['id']) { return $product->get_price(); } } function rest_stock($object,$field_name,$request){ global $product; $id = $product->get_id(); if ($id == $object['id']) { return $product->get_stock_status(); } } function rest_img($object,$field_name,$request){ global $product; $id = $product->get_id(); if ($id == $object['id']) { return $product->get_image(); } } function maximum_api_filter($query_params) { $query_params['per_page']["maximum"]=100; return $query_params; } add_filter('rest_product_collection_params', 'maximum_api_filter');
All this does is create new fields for REST API and extends the maximum items limit per request. Again, if you are new to this subject I highly recommend reading this series.
For now, if you go to your wishlist table and refresh the page you will see the list of items that are added to your wishlist.
8. Removing Items From Wishlist
We are almost done; only the remove functionality remains. So let’s create that! Add the following code at the very bottom of the wrapper function in the main.js file
$(document).on('click', '.wishlist-remove', function(){ var $this = $(this); $this.closest('table').addClass('loading'); wishlist = []; $this.closest('table').find('tr').each(function(){ if ($(this).data('product') != $this.closest('tr').data('product')) { wishlist.push($(this).data('product')); if (loggedIn) { // get user ID if (userData['user_id']) { $.ajax({ type: 'POST', url:opt.ajaxPost, data:{ action:'user_wishlist_update', user_id :userData['user_id'], wishlist :wishlist.join(','), } }) .done(function(response) { $this.closest('table').removeClass('loading'); $this.closest('tr').remove(); }) .fail(function(data) { alert(opt.error); }); } } else { sessionStorage.setItem(shopName, wishlist.toString()); setTimeout(function(){ $this.closest('table').removeClass('loading'); $this.closest('tr').remove(); },500); } } }); });
Once the remove icon is clicked (make sure you have a remove.svg in the images folder, you can use whatever icon you want), we need to check if the user is logged-in. If so, we then remove the item ID from the wishlist using AJAX with the user_wishlist_update action. If the user is a guest we need to remove the item ID from the session/local storage.
Now go to your wishlist and refresh the page. Once you click on the remove icon your item will be removed from the wishlist.
Conclusion
That was quite a project! A simple, but comprehensive wishlist feature for your WooCommerce stores. You are free to use this plugin in any project; you can extend, modify it and make suggestions. I hope you liked it. Here is the link to the source files on GitHub. And here is the demo.
Learn More WooCommerce Theme Development
At Tuts+ we have a great collection of tutorials and courses to learn WooCommerce development. Check out these four great courses to get started!
WooCommerce
Up and Running With WooCommerce
Rachel McCollin
WordPress
Developing a WooCommerce Theme
Rachel McCollin
WordPress
Go Further With WooCommerce Themes
Rachel McCollin
WordPress
How to Make Your Theme WooCommerce Compatible
Rachel McCollin
by Karen Pogosyan via Envato Tuts+ Code https://ift.tt/2WTWfiG
1 note
·
View note
Photo
How to Build and Deploy a Web App With Buddy
Moving code from development to production doesn't have to be as error-prone and time-consuming as it often is. By using Buddy, a continuous integration and delivery tool that doubles up as a powerful automation platform, you can automate significant portions of your development workflow, including all your builds, tests, and deployments.
Unlike many other CI/CD tools, Buddy has a pleasant and intuitive user interface with a gentle learning curve. It also offers a large number of well-tested actions that help you perform common tasks such as compiling sources and transferring files.
In this tutorial, I'll show you how you can use Buddy to build, test, and deploy a Node.js app.
Prerequisites
To be able to follow along, you must have the following installed on your development server:
Node.js 10.16.3 or higher
MongoDB 4.0.10 or higher
Git 2.7.4 or higher
1. Setting Up a Node.js App
Before you dive into Buddy, of course, you'll need a web app you can build and deploy. If you have one already, feel free to skip to the next step.
If you don't have a Node.js app you can experiment with, you can create one quickly using a starter template. Using the popular Hackathon starter template is a good idea because it has all the characteristics of a typical Node.js app.
Fork the template on GitHub and use git to download the fork to your development environment.
git clone https://github.com/hathi11/hackathon-starter.git
It's worth noting that Buddy is used with a Git repository. It supports repositories hosted on GitHub, BitBucket, and other such popular Git hosts. Buddy also has a built-in Git hosting solution or you can just as easily use Buddy with your own private Git servers.
Once the clone's complete, use npm to install all the dependencies of the web app.
cd hackathon-starter/ npm install
At this point, you can run the app locally and explore it using your browser.
node app.js
Here's what the web app looks like:
2. Creating a Buddy Project
If you don't have a Buddy account already, now is a good time to create one. Buddy offers two premium tiers and a free tier, all of which are cloud based. The free tier, which gives you 1 GB of RAM and 2 virtual CPUs, will suffice for now.
Once you're logged in to your Buddy account, press the Create new project button to get started.
When prompted to select a Git hosting provider, choose GitHub and give Buddy access to your GitHub repositories.
You should now be able to see all your GitHub repositories on Buddy. Click on the hackathon-starter repository to start creating automations for it.
Note that Buddy automatically recognizes our Node.js app as an Express application. It's because our starter template uses the Express web app framework.
3. Creating a Pipeline
On Buddy, a pipeline is what allows you to orchestrate and run all your tasks. Whenever you need to automate something with Buddy, you either create a new pipeline for it or add it to an existing pipeline.
Click on the Add a new pipeline button to start creating your first pipeline. In the form shown next, give a name to the pipeline and choose On push as the trigger mode. As you may have guessed, choosing this mode means that the pipeline is executed as soon as you push your commits to GitHub.
The next step is to add actions to your pipeline. To help you get started, Buddy intelligently generates a list of actions that are most relevant to your project.
For now, choose the Node.js action, which loads a Docker container that has Node.js installed on it. We'll be using this action to build our web app and run all its tests. So, on the next screen, go ahead and type in the following commands:
npm install npm test
4. Attaching a Service
Our web app uses MongoDB as its database. If it fails to establish a connection to a MongoDB server on startup, it will exit with an error. Therefore, our Docker container on Buddy must have access to a MongoDB server.
Buddy allows you to easily attach a wide variety of databases and other services to its Docker containers. To attach a MongoDB server, switch to the Services tab and select MongoDB. In the form shown next, you'll be able to specify details such as the hostname, port, and MongoDB version you prefer.
Make a note of the details you enter and press the Save this action button.
Next, you must configure the web app to use the URI of Buddy's MongoDB server. To do so, you can either change the value of the MONGODB_URI field in the .env.example file, or you can use an environment variable on Buddy. For now, let's go ahead with the latter option.
So switch to the Variables tab and press the Add a new variable button. In the dialog that pops up, set the Key field to MONGODB_URI and the Value field to a valid MongoDB connection string that's based on the hostname you chose earlier. Then press the Create variable button.
The official documentation has a lot more information about using environment variables in a Buddy pipeline.
5. Running the Pipeline
Our pipeline is already runnable, even though it has only one action. To run it, press the Run pipeline button.
You will now be taken to a screen where you can monitor the progress of the pipeline in real time. Furthermore, you can press any of the Logs buttons (there's one for each action in the pipeline) to take a closer look at the actual output of the commands that are being executed.
You can, of course, also run the pipeline by pushing a commit to your GitHub repository. I suggest you make a few changes to the web app, such as changing its header by modifying the views/partials/header.pug file, and then run the following commands:
git add . git commit -m "changed the header" git push origin master
When the last command has finished, you should be able to see a new execution of the pipeline start automatically.
6. Moving Files
When a build is successful and all the tests have passed, you'd usually want to move your code to production. Buddy has predefined actions that help you securely transfer files to several popular hosting solutions, such as the Google Cloud Platform, DigitalOcean, and Amazon Web Services. Furthermore, if you prefer using your own private server that runs SFTP or FTP, Buddy can directly use those protocols too.
In this tutorial, we'll be using a Google Compute Engine instance, which is nothing but a virtual machine hosted on Google's cloud, as our production server. So switch to the Actions tab of the pipeline and press the + button shown below the Build and test action to add a new action.
On the next screen, scroll down to the Google Cloud Platform section and select the Compute Engine option.
In the form that pops up, you must specify the IP address of your VM. Additionally, to allow Buddy to connect to the VM, you must provide a username and choose an authentication mode.
The easiest authentication mode in my opinion is Buddy's SSH key. When you choose this mode, Buddy will display an RSA public key that you can simply add to your VM's list of authorized keys.
To make sure that the credentials you entered are valid, you can now press the Test action button. If there are no errors, you should see a test log that looks like this:
Next, choose GitHub repository as the source of the files and use the Remote path field to specify the destination directory on the Google Cloud VM. The Browse button lets you browse through the filesystem of the VM and select the right directory.
Finally, press the Add this action button.
7. Using SSH
Once you've copied the code to your production server, you must again build and install all its dependencies there. You must also restart the web app for the code changes to take effect. To perform such varied tasks, you'll need a shell. The SSH action gives you one, so add it as the last action of your pipeline.
In the form that pops up, you must again specify your VM's IP address and login credentials. Then, you can type in the commands you want to run. Here's a quick way to install the dependencies and restart the Node.js server:
pkill -HUP node #stop node server cd my_project npm install #install dependencies export MONGODB_URI= nohup node app.js > /dev/null 2>&1 & #start node server
As shown in the Bash code above, you must reset the MONGODB_URI environment variable. This is to make sure that your production server connects to its own MongoDB instance, instead of Buddy's MongoDB service.
Press the Add this action button again to update the pipeline.
At this point, the pipeline has three actions that run sequentially. It should look like this:
Press the Run pipeline button to start it. If there are no errors, it should take Buddy only a minute or two to build, test, and deploy your Node.js web app to your Google Cloud VM.
Conclusion
Being able to instantly publish new features, bug fixes, and enhancements to your web apps gives you a definite edge over your competition. In this tutorial, you learned how to use Buddy's pipelines, predefined actions, and attachable services to automate and speed up common tasks such as building, testing, and deploying Node.js applications.
There's a lot more the Buddy platform can do. To learn more about it, do refer to its extensive documentation.
by Ashraff Hathibelagal via Envato Tuts+ Code https://ift.tt/33rH96G
1 note
·
View note
Photo
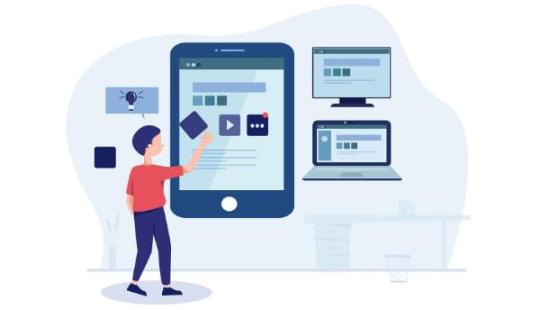
React Native End-to-end Testing and Automation with Detox
Detox is an end-to-end testing and automation framework that runs on a device or a simulator, just like an actual end user.
Software development demands fast responses to user and/or market needs. This fast development cycle can result (sooner or later) in parts of a project being broken, especially when the project grows so large. Developers get overwhelmed with all the technical complexities of the project, and even the business people start to find it hard to keep track of all scenarios the product caters for.
In this scenario, there’s a need for software to keep on top of the project and allow us to deploy with confidence. But why end-to-end testing? Aren’t unit testing and integration testing enough? And why bother with the complexity that comes with end-to-end testing?
First of all, the complexity issue has been tackled by most of the end-to-end frameworks, to the extent that some tools (whether free, paid or limited) allow us to record the test as a user, then replay it and generate the necessary code. Of course, that doesn’t cover the full range of scenarios that you’d be able to address programmatically, but it’s still a very handy feature.
Want to learn React Native from the ground up? This article is an extract from our Premium library. Get an entire collection of React Native books covering fundamentals, projects, tips and tools & more with SitePoint Premium. Join now for just $9/month.
End-to-end Integration and Unit Testing
End-to-end testing versus integration testing versus unit testing: I always find the word “versus” drives people to take camps — as if it’s a war between good and evil. That drives us to take camps instead of learning from each other and understanding the why instead of the how. The examples are countless: Angular versus React, React versus Angular versus Vue, and even more, React versus Angular versus Vue versus Svelte. Each camp trash talks the other.
jQuery made me a better developer by taking advantage of the facade pattern $('') to tame the wild DOM beast and keep my mind on the task at hand. Angular made me a better developer by taking advantage of componentizing the reusable parts into directives that can be composed (v1). React made me a better developer by taking advantage of functional programming, immutability, identity reference comparison, and the level of composability that I don’t find in other frameworks. Vue made me a better developer by taking advantage of reactive programming and the push model. I could go on and on, but I’m just trying to demonstrate the point that we need to concentrate more on the why: why this tool was created in the first place, what problems it solves, and whether there are other ways of solving the same problems.
As You Go Up, You Gain More Confidence
As you go more on the spectrum of simulating the user journey, you have to do more work to simulate the user interaction with the product. But on the other hand, you get the most confidence because you’re testing the real product that the user interacts with. So, you catch all the issues—whether it’s a styling issue that could cause a whole section or a whole interaction process to be invisible or non interactive, a content issue, a UI issue, an API issue, a server issue, or a database issue. You get all of this covered, which gives you the most confidence.
Why Detox?
We discussed the benefit of end-to-end testing to begin with and its value in providing the most confidence when deploying new features or fixing issues. But why Detox in particular? At the time of writing, it’s the most popular library for end-to-end testing in React Native and the one that has the most active community. On top of that, it’s the one React Native recommends in its documentation.
The Detox testing philosophy is “gray-box testing”. Gray-box testing is testing where the framework knows about the internals of the product it’s testing.In other words, it knows it’s in React Native and knows how to start up the application as a child of the Detox process and how to reload it if needed after each test. So each test result is independent of the others.
Prerequisites
macOS High Sierra 10.13 or above
Xcode 10.1 or above
Homebrew:
/usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
Node 8.3.0 or above:
brew update && brew install node
Apple Simulator Utilities: brew tap wix/brew and brew install applesimutils
Detox CLI 10.0.7 or above:
npm install -g detox-cli
See the Result in Action
First, let’s clone a very interesting open-source React Native project for the sake of learning, then add Detox to it:
git clone https://github.com/ahmedam55/movie-swiper-detox-testing.git cd movie-swiper-detox-testing npm install react-native run-ios
Create an account on The Movie DB website to be able to test all the application scenarios. Then add your username and password in .env file with usernamePlaceholder and passwordPlaceholder respectively:
isTesting=true username=usernamePlaceholder password=passwordPlaceholder
After that, you can now run the tests:
detox test
Note that I had to fork this repo from the original one as there were a lot of breaking changes between detox-cli, detox, and the project libraries. Use the following steps as a basis for what to do:
Migrate it completely to latest React Native project.
Update all the libraries to fix issues faced by Detox when testing.
Toggle animations and infinite timers if the environment is testing.
Add the test suite package.
Setup for New Projects
Add Detox to Our Dependencies
Go to your project’s root directory and add Detox:
npm install detox --save-dev
Configure Detox
Open the package.json file and add the following right after the project name config. Be sure to replace movieSwiper in the iOS config with the name of your app. Here we’re telling Detox where to find the binary app and the command to build it. (This is optional. We can always execute react-native run-ios instead.) Also choose which type of simulator: ios.simulator, ios.none, android.emulator, or android.attached. And choose which device to test on:
{ "name": "movie-swiper-detox-testing", // add these: "detox": { "configurations": { "ios.sim.debug": { "binaryPath": "ios/build/movieSwiper/Build/Products/Debug-iphonesimulator/movieSwiper.app", "build": "xcodebuild -project ios/movieSwiper.xcodeproj -scheme movieSwiper -configuration Debug -sdk iphonesimulator -derivedDataPath ios/build", "type": "ios.simulator", "name": "iPhone 7 Plus" } } } }
Here’s a breakdown of what the config above does:
Execute react-native run-ios to create the binary app.
Search for the binary app at the root of the project: find . -name "*.app".
Put the result in the build directory.
Before firing up the test suite, make sure the device name you specified is available (for example, iPhone 7). You can do that from the terminal by executing the following:
xcrun simctl list
Here’s what it looks like:
Now that weve added Detox to our project and told it which simulator to start the application with, we need a test runner to manage the assertions and the reporting—whether it’s on the terminal or otherwise.
Detox supports both Jest and Mocha. We’ll go with Jest, as it has bigger community and bigger feature set. In addition to that, it supports parallel test execution, which could be handy to speed up the end-to-end tests as they grow in number.
Adding Jest to Dev Dependencies
Execute the following to install Jest:
npm install jest jest-cli --save-dev
The post React Native End-to-end Testing and Automation with Detox appeared first on SitePoint.
by Ahmed Mahmoud via SitePoint https://ift.tt/2JTJWxK
1 note
·
View note
Photo
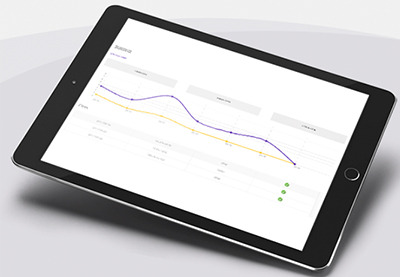
ADning Advertising: Create Adverts For Your WordPress Site
Advertising is one of the main ways that websites generate revenue.
Perhaps you created a blog specifically to drive people towards your website’s Store page, and want to give visitors that extra little push? Maybe you run several websites, and want to promote your biggest-earner across your entire portfolio? Or maybe you don’t have anything to promote, but wouldn’t mind generating some cash by selling advertising space to third parties?
Despite the money-making potential, WordPress doesn’t support advertisements out-of-the-box. Most WordPress themes don’t even have space where you can display ads!
Best WordPress Advertising Plugins of 2019
Creating a successful advertising campaign is a must in today's crowded eCommerce world. Once you have your website and products in place, you must have the...
Daniel Strongin
04 Apr 2019
WordPress Plugins
10 Best WordPress Slider & Carousel Plugins of 2019
Whether you want to show off customer testimonials, your latest blog posts, your best images, or just celebrate the members of your team, there is a...
Nona Blackman
19 Mar 2019
WordPress
8 Best WordPress Booking and Reservation Plugins
In the digital age, users are online 24/7. Everyone prefers to check availability and make appointments, reservations, or bookings online. They want to do...
Lorca Lokassa Sa
12 Apr 2019
WordPress
Best Affiliate WooCommerce Plugins Compared
WooCommerce is an excellent platform for selling digital and physical products. Adding an affiliate system is an excellent way to increase your reach around...
Lorca Lokassa Sa
26 Jun 2019
WordPress
If you dream of turning your website into an additional source of revenue, then you have two options: dig into your theme’s files and modify its code to support adverts,or let a plugin do all the hard work for you!
In this article, I’ll show you how to create a range of adverts and display them across your website, using the popular ADning Advertising plugin. To help ensure your ads generate as many click-throughs as possible, we’ll be using ADning to display customized ads based on factors such as the visitor’s geographical location, and the time of day. Finally, I’ll show how to monetize your website even if you don’t have anything to promote, by selling advertising space to third parties.
Getting Started With the ADning Advertising Plugin
The first step, is installing the ADning Advertising plugin:
If you haven’t already registered, create your free Envato Market account.
Head over to the CodeCanyon website, click the little Sign In button in the upper-right corner, and then enter your Envato account details.
Once you’re logged into your account, head over to the ADning Advertising listing, click Buy Now and then follow the onscreen instructions to complete your purchase.
When prompted, download the ADning plugin.
Unzip the downloaded file; the subsequent folder should contain several folders and files, including angwp.zip, which you’ll need to upload to WordPress.
Adding a Third Party Plugin to WordPress
Once you’ve downloaded the ADning Advertising plugin, you’ll need to upload it to your WordPress account:
Log into WordPress, if you haven’t already.
Select Plugins from WordPress’ left-hand menu, followed by Add New.
Select the Upload Plugin button.
Now scroll to the If you have a plugin in a .zip format section, and select Choose file.
Select your angwp.zip file and click Install Now.
ADning will now be uploaded to your WordPress account. After a few moments, a new ADning item should appear in WordPress’ left-hand menu.
Activating the ADning Advertising Plugin
Next, you’ll need to active ADning, by entering your license key:
Head over to the CodeCanyon website and log into your account.
Select your username in the upper-right corner, followed by Downloads.
Find the ADning Advertising item, and select its accompanying Download button.
Choose License certificate & purchase code from the dropdown menu.
Once your license has downloaded, open it and find your Item purchase code. You'll need to copy this information then, in your WordPress account, select ADning from the left-hand menu. Select the Product License tab and paste your purchase code into the Add your license key here field.
Finally, click Activate ADning. After a few moments, you should see a Plugin activated successfully message. Your plugin is now ready to use!
Placing Adverts on Your Website
In this section, we’ll be placing four different styles of adverts on our WordPress website:
A banner ad that’ll run along the top of the page.
An embedded banner that’ll be displayed inside the page’s content.
A popup ad.
An “injected” advert that’ll be displayed on a line specified by you.
To create these ads, I’ll be using a graphic that promotes Envato Elements, but you can use any graphic you want.
Creating Your First ADning Ad
To display an advert, we need to create:
A banner, which is essentially the graphic that we want to use.
A campaign, which sets a start and an end date for your advert.
An Ad Zone, which defines where your banner will appear on the page, and the kind of advert that’ll be displayed to the visitor, such as a popup or a vertical banner.
We then need to link these three components together, and insert the finished advert into our website using either shortcode or a widget.
While this may seem like a lot of setup just to display a single ad, you can re-use these elements multiple times, for example you may decide to run multiple adverts as part of the same campaign, or use the same Ad Zone for all your banners.
Turn Any Image Into a Banner
Let’s start by creating a banner, using a graphic of your choice. Note that depending on the dimensions of your chosen graphic, you may need to play around with the common advertisement settings in order to create an advert that looks good and functions correctly.
Select Banner from WordPress’ left-hand menu and click Add New Banner.
Under Title give this banner a descriptive name; I’m using Elements banner.
In Add a banner link, enter the URL that your banner should link to; I’m using the Elements website.
Now open Target and specify the window where the destination URL should load, for example in a new tab or inside the current advertising frame. If you’re linking to an external website, then you’ll typically load the URL in a new tab or window, because you don't want to encourage visitors to navigate away from your website.
Find the Select one of the common banner sizes section and open its accompanying dropdown. Here, you’ll find some of the most commonly used advertising styles and sizes, such as vertical banner, pop under and full banner. Select the banner style and sizing that best represents the advertisement you want to create, or select Custom and then specify an exact size in pixels. Note that this step may require some trial and error!
Select Click here or Drag file to upload and then choose the graphic that you want to use.
Find the Auto Positioning section and specify where your banner should appear on the page. Since I want to display this banner along the top of the screen, I’m selecting Above content.
Scroll to Alignment and then choose from left, right or center alignment; I’m using Center.
When you’re happy with the information you’ve entered, select Save Banner.
Create Your First Advertising Campaign
Next, we need to create a campaign. This campaign will dictate the date and time when the advertisement will first appear on your website, and when it’ll disappear:
Select Campaigns from WordPress’ left-hand menu, then select Add New Campaign.
Give this campaign a descriptive Title; I’m using Elements campaign.
In the Start Date section, enter the month, day, year and time when this campaign should start. To make it easier to test your advert, you should choose either the current date and time, or backdate the advert so it’ll appear on your website immediately.
Click to expand the End date section, and then choose the date and time when this campaign should end, at which point the associated advert(s) will disappear from your website.
When you’re happy with your changes, select Save Campaign.
Make Space for Your Advert: Creating an AD Zone
Finally, we need to create an AD Zone, which is the region of the page where we’ll display our advert. Once we’ve created an AD Zone, you can re-use that zone for subsequent adverts:
Select AD Zones from WordPress’ left-hand menu and click Add New AD Zone.
Give the AD Zone a descriptive Title; I’m using Elements AD Zone.
Find the Select one of the common banner sizes section, and open its accompanying dropdown. Here, you’ll find some common advertisement sizes, such as vertical banner, pop under and full banner. Select the option that best represents the banner you’re placing within this AD Zone. Alternatively, you can select Custom and then specify the exact dimensions.
Now, open the Linked banners dropdown and select the banner we created in the previous step.
Open the Campaign dropdown and select the campaign.
Scroll to the Alignment section and choose how you want to position this AD Zone onscreen; I’m selecting Center.
Once you’re happy with the information you’ve entered, select Save AD Zone.
Connect Your Components
Now we've created all three components, we just need to link our banner to our campaign and AD Zone:
Select Banners from WordPress’ left-hand menu.
Find the banner that you previously created and click its accompanying Edit link.
Open the Campaigns dropdown and select your campaign.
Open Adzones and select the AD Zone you created in the previous step.
Select Save banner.
Displaying Your Advert
Now we have all three of our components, we’re ready to place the advert on our website using either an ADning widget or shortcode. Let’s explore both options:
1. Use a Widget
One option, is to place a widget on your website and then assign it an appropriate AD Zone and banner:
In WordPress’ left-hand menu, select Appearance > Widgets.
Click to expand the ADning ADS section and select Add widget.
Open the Select a banner dropdown and choose the banner that you want to display.
Open Select an Adzone and then select the AD Zone that we created earlier.
Click Save.
Now, load any WordPress page or post, and your banner should appear at the top of the screen.
Give this banner a click to make sure it links through to your chosen URL in either a new tab, window or within the current advertisement frame, depending on how you’ve configured your banner.
Note that at this point you may need to spend some time tweaking your banner style and sizing, depending on your original graphic, how you chose to position that graphic, and your website’s theme.
2. Copy and Paste the Shortcode
Alternatively, you can display the finished advert using shortcode. I'll use a shortcode to display the advert in a widget.
Select AD Zones from WordPress’ left-hand menu. Then find your AD Zone and click its accompanying Edit link.
Scroll to the Export section; this should contain a block of shortcode. Copy all of this code.
In WordPress’ left-hand menu, select Appearance > Widgets.
Click to expand the ADning ADS section.
Select Add widget.
Paste your shortcode into the Or add the adzone shortcode section.
When you're done, click Save.
Once again, load any page or post that makes up your website, and your banner should appear onscreen.
What Other Ad Styles Can I Use?
ADning Advertising supports a wide range of different advertising styles. In this section we’ll be exploring a few simple ways that you can modify our existing banner to create a completely different style of ad.
Creating Embedded Ads
Let’s start by moving our banner from the top of the page and embedding it within our webpage’s content.
Every time you create an embedded ad, you have the option to repeat this advertisement at regular intervals, for example once every five paragraphs.
If your website contains large amounts of text, then an occasional embedded advert can be more appealing than a solid wall of words—just don’t get carried away! Remember that some visitors may be viewing your website on the smaller screen of a smartphone or tablet, where each advert could potentially take up their entire screen, forcing them to scroll to find the content they’re actually interested in.
To turn our banner into an embedded ad:
Select ADning > Banners from WordPress’ left-hand menu.
Find the banner that you want to edit and select its accompanying Edit link.
Scroll to the Auto Positioning section and select the Inside content thumbnail, which will give you access to a new Custom Placement Settings section.
Specify where your advert should appear, using the After x paragraphs field.
Optionally, you can turn this advert into a repeating advert by clicking the Repeat slider so that it changes from No to Yes. If you select Yes, then your advert will repeat at the interval defined in the After x paragraphs field.
When you’re happy with your changes, click Save Banner.
Custom Classnames: Control Exactly Where You Ad Appears
Would you like more control over where each advert appears? Perhaps you want to position an advert next to a relevant paragraph, or maybe pick-and-choose which advert appears on each webpage?
You can control the page, paragraph, and even the line where your advert appears, by creating one or more custom classnames, and then assigning each classname to an advert. Then, you just need to insert the correct custom classname into your website’s HTML, and the associated advert will appear in that exact spot.
Using the Widget Options Plugin
You can create custom classnames quickly and easily using a dedicated plugin; I’m opting to use the free Widget Options plugin.
You can install this plugin from the WordPress plugin directory. To setup it up, just select Plugins > Add New from WordPress’ left-hand menu.
Search for Widget Options.
When the correct plugin appears, select Install Now.
Once the plugin has been installed, click Activate.
Creating Custom Classnames
Next, we need to create a custom classname. Depending on your setup, you might decide to create a single classname for a specific advert, a different classname for each advert, or a single classname that you’ll use across all of your adverts.
To help keep things simple, we’ll be creating a single classname:
In WordPress’ left-hand menu, select Settings > Widget Options.
Find the Classes & ID section and select Configure settings.
Select the Enable ID Field checkbox.
You can now use the Predefined Classes field to create a list of all the custom classnames that you want to use. Type each classname into the field and then press the + button.
When you’re happy with the information you’ve entered, click Save Settings.
Next, select Appearance > Widgets from WordPress’ left-hand menu.
Click to expand the Adning ADS section.
Select the little cog icon, which opens the Settings tab.
Select the Class & ID tab.
The Class & ID tab should contain a list of all the custom classnames that you can use with ADning; select the classname that you just created and click Save.
Assigning Custom Classnames
Next, we need to assign this custom classname to our advert:
Select ADning > Banners from WordPress’ left-hand menu.
Find our advert, and then select its accompanying Edit link.
Scroll to the Default AD placements section and select Inject before/after class.
In the Custom Placement Settings section, open the Where dropdown and specify whether your advert should be placed Before or After the point where you insert the classname into your HTML. Note that it may not be immediately clear which option will work best for your particular website, but you can always return to this page and modify the Before/After setting later.
In the Element field, type the classname that you want to associate with this advertisement and when you're done you can click Save Banner.
Editing Your Website's HTML
Now, we can place this advert anywhere on our website, by inserting the custom classname into our HTML:
Find the page or post where you want to display your advert, and open that item for editing.
Click to select the block where you want to place your ad; a little floating toolbar should appear.
Select the three-dotted icon, followed by Edit as HTML.
You can now add your custom classname to any of the page or post’s HTML tags. For example, imagine I’d created an elementsAd custom class and wanted to place my advert before the following block:
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis id tincidunt turpis. Pellentesque neque magna, egestas quis orci a, luctus pretium tellus. Donec egestas enim massa, sed pellentesque massa tempus in.</p>
In this scenario, I’d add class="elementsAd" to the opening <p> tag:
<p class="elementsAd">Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis id tincidunt turpis. Pellentesque neque magna, egestas quis orci a, luctus pretium tellus. Donec egestas enim massa, sed pellentesque massa tempus in.</p>
Insert the custom classname in your chosen location, then save your changes and navigate to the page or post in question. Your advert should have appeared, at the point where you inserted the custom classname.
Create a Popup Ad
ADning Advertising also supports popup advertisements, which by default appear every time a visitor navigates to a new page.
To convert our banner ad into a popup:
Select ADning > Banners from WordPress’ left-hand menu.
Find the banner that you want to modify and click its accompanying Edit link.
Scroll to the Auto positioning section, and then select Popup/Sticky.
In the Custom Placement Settings section, use the thumbnails to select where this popup should appear onscreen; I’m using Bottom/Center.
Click Save Banner.
Now, whenever you navigate to a new webpage, a popup advertisement will appear.
Immediately, this poses a problem: no-one wants to be confronted by a popup every single time they navigate to a new page! Conveniently, this leads us onto our next topic.
Control Where Your Ads Appear
If a visitor encounters the exact same ad on every single post and page across your site, then in the best case scenario that advert is quickly going to become invisible to them.
In the worst case scenario, the visitor will get so frustrated that they’ll navigate away from your website, especially if you’re using intrusive adverts such as popups.
Often, you’ll want to set some restrictions about where each advert can appear, and ADning gives you several options:
Select ADning > Banners from WordPress’ left-hand menu.
Find the banner ad that you want to edit, and then click its accompanying Edit link.
From here, you can set the following restrictions.
Ban This Advert From Your Home Page
Your home page is often a visitor’s first impression of your website, and a page full of adverts isn’t exactly a great first impression!
To prevent this advert from appearing on your home page, find the Home page section and click its accompanying Show/Hide button, so that it displays Hide.
Don’t Display This Ad on Smartphones or Tablets
The smaller screen of a smartphone or tablet can make a webpage feel much more ad-heavy than it actually is. Since mobile users typically have a much lower tolerance for ads, you may want to reduce the number of adverts that appear on mobile devices.
You can block this advert from smartphones or tablets, or both, using the Show/Hide toggles in the Device Filters section.
For example, if you wanted to prevent this advert from appearing on smartphones, then you’d find the Mobile section and toggle its accompanying button to read Hide.
Block This Ad From Specific Pages and Posts
You may want to ensure that a few webpages remain completely ad-free, for example if there’s a page where an ad would be inappropriate, or you simply want to give your visitors a break!
You can use ADning to name specific pages and posts where this advert should never appear:
Scroll to the For page section.
Click the Show/Hide button so that it reads Hide.
In the accompanying field, start typing the name of the page or post in question, and then select it from the dropdown menu when it appears.
Display This Ad on the Named Page Only
If you only want to display this advert on a small number of pages and posts, then it may be easier to simply name the webpages where this advert should appear:
In the For page section, click the Show/Hide button so that it reads Show.
Enter the name of the page or post where this advert should appear, and then select it from the dropdown menu.
You can repeat the final step, until you’ve named all the webpages where this ad should make an appearance.
Drive User Engagement With Customized Ads
You can encourage visitors to interact with your ads by delivering customized content. ADning gives you the option to tailor your ads based on the visitor’s geographical location, and the date and time when they’re viewing the advert.
Filter Content by Country
There’s lots of reasons why you might display different adverts, depending on the visitor’s location.
You may be able to encourage visitors to engage with your ads, by targeting their location, for example displaying ads that relate to national holidays such as Canada Day, Chinese New Year or St. Patrick’s Day. Since your website has the potential to reach a global audience, you should also consider that some of your ads may be deemed inappropriate, or even offensive in certain parts of the world.
To display ads based on the visitor’s location:
Select ADning > Banners from WordPress’ left-hand menu.
Find the banner that you want to edit, and then click its accompanying Edit link.
Scroll to the Country filters section.
From here, you can block this advert for specific countries:
Press the Show/Hide button so that it changes to Hide.
In the accompanying text field, enter the country, or countries where your advert shouldn’t be displayed.
Alternatively, if this ad is only relevant for a small number of countries, then it may be easier to simply name these locations:
Toggle the Show/Hide button so that it reads Show.
Specify every country where you want to display this ad.
Display Ads Based on Date and Time
Your visitors may be more likely to interact with an advert at a certain date or time. For example, you may have more success displaying entertainment-focused ads on a Friday afternoon, and job adverts on a Monday morning.
If you’re an organized person, then you could even schedule ads to go live months in advance, for example creating a campaign that’ll kick in at midnight on Black Friday.
To control the date and time when an advert is visible on your website, you’ll need to edit the campaign associated with that ad:
Select ADning > Campaigns from WordPress’ left-hand menu.
Find the campaign that you want to edit, and then click its accompanying Edit button.
Click to expand the Advanced date options.
In this expanded panel, you’ll have access to a range of different date and time settings, including the ability to display this advert on a specific day of the week, during the weekend only, or at a certain time.
How to Sell Ad Space to Third Parties
Just because you don’t have anything to advertise, doesn’t mean you can’t generate money from advertisements! Once you’ve created some advertising space on your website, you can use ADning to sell this space to third parties, who will then run their ads on your website.
If you’ve already setup the popular WooCommerce eCommerce plugin, then you can sell this space and receive payments via your existing WooCommerce checkout.
To take advantage of ADning’s WooCommerce integration, you’ll need to setup the ADning WooCommerce plugin, which is included as part of your ADning license.
To setup ADning WooCommerce, select ADning from WordPress’ left-hand menu.
Select the Add-ons tab. Adning WooCommerce will be listed under Included Add-Ons. Give this plugin a click, and it should be installed automatically.
Now select ADning > General Settings from WordPress’ left-hand menu and choose the Sell tab.
Click to expand the WooCommerce Settings section. Find the Create Adning Ads WooCommerce Product and give it a click. To start accepting payments via your WooCommerce checkout, select the Activate toggle so that it turns from No to Yes.
Click Save changes.
You should now be able to setup and manage all of your third party ads, via ADning’s Frontend AD Manager:
Navigate to the Sell screen, if you haven’t already (ADning > General Settings > Sell.)
Click to expand the Frontend AD Manager Settings section.
Click the Frontend AD Manager link, and the AD Manager should open in a new tab, ready for you to configure your third party ads.
Conclusion
In this article, I showed you how to setup and use the popular ADning Advertising plugin. If you’ve been following along, then you’ll have created a selection of ads, and then customized these ads in several different ways, including displaying different ads based on the visitor’s location, and the time of day. Finally, I showed how you to start selling advertising space to third parties, using the ADning WooCommerce plugin and AD Manager.
You can learn more about creating, displaying and managing WordPress ads, over at the official ADning website, or by taking a look at the plugin’s documentation.
WordPress Plugins
Best WordPress Advertising Plugins of 2019
Daniel Strongin
WordPress
10 Best WordPress Slider & Carousel Plugins of 2019
Nona Blackman
WordPress
8 Best WordPress Booking and Reservation Plugins
Lorca Lokassa Sa
by Jessica Thornsby via Envato Tuts+ Code https://ift.tt/2WFZWZr
1 note
·
View note
Photo
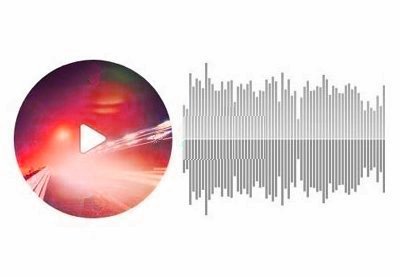
Add Engagement With Interactive Media Plugins for WordPress
Visual engagement is an indispensable part of any website. Without it users won't engage with your content. It also means that you are not reaching any audience or grabbing attention, which in turn means you have no conversions and no revenue.
You need to create visual engagement that leads to satisfying user experiences that in turn create high conversions and high revenue.
But managing the large amount of images, audio, and videos you need to make your website function effective requires a lot of space and organization. You need plugins to save you time.
WordPress media is an umbrella term a large number of plugins including media library management plugins to interactive media plugins like audio, video, flipbooks, galleries, maps, countdown clocks, notifications, timelines, popups, and forms.
In this article I will show you some different types of WordPress media plugins available on CodeCanyon.
15 Best WordPress Audio Player and Video Player Plugins
CodeCanyon has a wide range of best-selling WordPress audio and video players. No matter what your vision for your audio or video content, there is a player...
Jane Baker
30 Nov 2018
WordPress
Top WordPress Audio and Video Plugins of 2019
WordPress audio and video plugins are essential if you are looking to add video and audio players to your website. Discover the best plugins for 2019 that...
Daniel Strongin
29 Apr 2019
WordPress Plugins
How to Add the Sticky HTML5 WordPress Music Player to Your Site
This tutorial will teach you how to quickly create a sticky music player for your WordPress website with continuous playback. This WordPress audio player...
Monty Shokeen
20 Sep 2019
WordPress
7 Best WordPress Video Gallery Plugins
Looking to add a beautiful video gallery or grid to your WordPress site? Figure out what you need, and then check out seven of the best video gallery plugins...
Kyle Sloka-Frey
25 Jan 2019
WordPress Plugins
6 Best Weather WordPress Widgets & Plugins
Websites for restaurants, retreat centers, country clubs, and many other businesses and organizations can benefit from a weather WordPress widget. Take a...
Kyle Sloka-Frey
28 Feb 2019
WordPress
Best Video Background Plugins for WordPress
If you’ve been thinking of adding a video background to your WordPress site, here are the eight best plugins available at CodeCanyon.
Nona Blackman
29 Sep 2017
WordPress
Understanding WordPress Media Plugins
Visuals are the best way to optimize your pages and content. Visual elements are called media. Media includes but is not limited to images, audio, video, animation, and so on.
Countdown clocks, contact forms, timelines, buttons, maps, notifications, icons, avatars, popups are also all media and are crucial to the success of a website.
When it comes to understanding WordPress media you have to start from the Media Library where you manage images, audio, video, and documents. The media library allows you to upload and manage media files, add images to posts, and even do quick edits to your images. You can also create galleries and subfolders.
But Media Library has a limit. If you run a website that requires few images then the built-in WordPress Media Library is enough. However, if you run large websites that require a lot of images, videos, audio, and more then you will need to use a media library management plugin.You will also need to use plugins specific to each different media element, for example gallery plugins for images, audio plugins for audio, video plugins for video and so on.
Types of WordPress Media Plugins
WordPress media plugins help you create, upload, store, manage, and display different kinds of media. In short, provide tools for managing and displaying your media.
The list below is not exhaustive but it shows what is included under the umbrella of WordPress media plugins:
media library management plugins: organizing and processing images, video or other media
interactive media plugins: for creating interactive experiences on your site
image builder plugins: for designing and creating new images using layers and effects
map plugins: for creating and displaying maps
galleries plugins: for displaying images
audio or video plugins: for adding audio or video players to your site
iframe plugins: for embedding other content on your site in an iframe
social media plugins: for embedding content from social media
avatar plugins: for displaying or creating user avatars
icons plugins: make it easy to add icons to your site
button plugins: for creating eye-catching buttons like CTAs (call to actions)
countdown plugins: for showing a countdown to some important event
What Do WordPress Media Management Plugins Do?
Different WordPress plugins work differently and what they do varies. But in general they help you:
categorize items according to theme, size, and more
add, delete, arrange, and sort items in the media library
add titles to media
do batch upload and add many images quickly
provide external storage for your media
manage how users interact with your media for example users can click on images and open them in separate pages.
Why Should You Use WordPress Media plugins?
They extend the functionality of your website back-end so it can handle large volumes of media.
They make your website more attractive to bring in users.
They allow users to easily navigate images and media on your website.
They increase user engagement and as a result conversions and profits.
They improve the SEO ranking of your website on search engines.
Things to Consider When Choosing a Media Plugin
Speed: You want a lightweight plugin that won’t slow down your website.
Features: Think about storage, integration with social networks, email marketing platforms, and payment gateways
Responsiveness and mobile-friendliness: More than 70% of traffic to websites comes from mobile devices. Does your media plugin work on mobile browsers?
Ease of use: You shouldn't require coding knowledge to be able to use Media plugins. They should be easy to use and customize.
Security: Users trust you with their personal and financial data. Choose media plugins that have a great track record when it comes to security.
Regular Updates: Regular updates solve security vulnerabilities. Choose a plugin with a track record of updates and maintenance.
Cost: Compare the prices of other plugins and see what fits your budget.
Ratings and reviews: What other users say is proof of quality of the plugin and the trustworthiness of the developer.
Downloads: The number of downloads is proof of popularity of the plugin among users. It shows they trust the provider.
Support: You may run into some issues. Make sure that the seller offers support. See what other users say about the quality of support from the provider.
WordPress Media Plugins on CodeCanyon
On CodeCanyon, will find many popular and best-selling WordPress media plugins that will make your website engaging to visitors. To help with your selection, I’ve classified them according to the categories below.
Media Library Management Plugins
The Media Library is where you can manage your images, audio, videos, and documents all in one place. The default WordPress Media Library is sufficient to manage a limited number of images. But business, magazine, and large blogging websites that regularly upload large numbers of images need plugins for efficient management of media files.
These top media management plugins will help you manage your media library.
WordPress Real Media Library
FileBird
Media Library Categories Premium
Dropr: Dropbox Plugin
Out-of-the-Box: Dropbox Plugin
Multisite Shared Media
Real Physical Media
Use-your-Drive: Google Drive Plugin
WP Media Manager
Groups File Access
File Manager Plugin
IconPress Pro: Icon Management Plugin
WP Media File Manager
FileBase: Media Library Folders
Leopard: WordPress Offload Media
Pixabay: Import Free Stock Images
WordPress Plugins
Organize Your WordPress Media Library With Folders
Daniel Strongin
WordPress Gallery and Slider Plugins
Gallery plugins have been covered extensively in other Envato Tuts+ posts. The articles below will lead you to an overview of gallery plugins available on CodeCanyon.
Image Builders and Virtual Tour Builders
Image and virtual tour building plugins make it easy to create new visual content for your site by combining other images.
Imagelinks: Interactive Image Builder
iPanorama 360: Interactive Virtual Tour Builder
Vision Interactive: Image Map Builder
WordPress
Best Interactive JavaScript Plugins to Liven Up Your WordPress Site
Lorca Lokassa Sa
WordPress Interactive Map Plugins
These plugins will help you add interactive map features to your website so users can learn geography or find directions for locations where they need to be!
Mapplic: Custom Interactive Map
Responsive Styled Google Map
5sec Google Maps
Interactive World Maps
Super Store Finder
Responsive Google Maps
Image Map Hotspot
Map List Pro
Advanced Google Maps
MapSVG
Interactive US Map
Agile Store Locator
WordPress Store Locator
WP Multi Store Locator Pro
WordPress
Add Google Maps and Social Login to Your WordPress Community With UserPro
Jessica Thornsby
Quiz, Survey, and Poll Plugins for WordPress
Surveys, quizzes, and polls are interactive forms. There are plugins that are specifically built to allow you to create your own engaging surveys, polls, and quizzes—all kinds of content that have been shown to be very popular with website visitors.
Buzzfeed Quiz Builder
Modal Survey: Poll Survey & Quiz
Advisor Quiz
OnionBuzz: Viral Quiz Maker
Quizmaker
Contest Bundle
ARI Stream Quiz
TotalPoll Pro
WordPress
Best WordPress Quiz Plugins of 2019
Monty Shokeen
WordPress
How to Pick a WordPress Form Builder Plugin
Lorca Lokassa Sa
Flipbook and PDF Viewer Plugins
Digital flipbooks look and feel like printed publications. Their pages can be flipped and turned. They’re a perfect way to show reports, presentations, magazines, catalogs, brochures, books, photo essays, and portfolios. They offer a great interactive experience. Try the following plugins and find out for yourself.
WordPress Flipbook
Real3D Flipbook
Diamond Flipbook
Responsive Flipbook Plugin
iPages Flipbook
PDF to Flipbook Extension
dFlip Flipbook
PDF Viewer for WordPress
PDF Light Viewer Pro
Bookshelf for Real3D Flipbook
WordPress
How to Find the Best WordPress Gallery Plugins for Images or Video
Lorca Lokassa Sa
WordPress
Best WordPress Flipbook Plugins Compared
Jane Baker
WordPress Video Plugins
These plugins are essential if you want to add video players to your website.
YouTube WordPress Video Import Plugin
Video Blogster Pro
Elite Video Player
Ultimate Video Player
Video Robot: Ultimate Video Importer
HTML5 Video Player and Full Screen Background
Image and Video Full Screen Background
Video Contest WordPress Plugin
Most Wanted WordPress Plugins Pack
Easy Video Player
WordPress
20 WordPress Video Plugins and Players to Add Engagement
Rachel McCollin
WordPress Audio Plugins
Similarly, these plugins are essential if you want to add audio players to your website.
Native Web Radio Player
Responsive HTML5 Radio Player Pro
ZoomSounds
tPlayer
Radio Player Shoutcast and Icecast
WavePlayer
MP3 Sticky Player
Responsive HTML5 Music Player
Hero Shoutcast and Icecast Radio Player
bzPlayer Pro
WordPress
15 Best WordPress Audio Player and Video Player Plugins
Jane Baker
WordPress Plugins
Top WordPress Audio and Video Plugins of 2019
Daniel Strongin
Social Media Plugins for WordPress
There are many media plugins and each fulfills a specific function like counting likes, creating posters, embedding carousels of content from social media, streaming, and so on.
Arqam: Social Counter Plugin
Easy Social Share Buttons
SocialFans: Responsive Social Counter Plugin
AX Social Stream: Social Board for WordPress
Flow-Flow Social Stream
Broadcast Extension for Flow-Flow Social Stream
Social Stream for WordPress with Carousel
Social Auto Poster
Pinterest Automatic Pin
Comment Slider for FaceBook
Instagram Journal
Grace - Instagram Feed Gallery
AccessPress Social
WordPress Plugins
20 Best Social Plugins for WordPress
Nona Blackman
Visual Timeline Plugins for WordPress
These timeline plugins will help connect events together to visually tell a story that is engaging.
Cool Timeline Pro
Content Timeline
WP Timeline
Everest Timeline
Visual Line
Social SEO FaceBook Responsive Timeline Feed
Avatar and Icon Plugins
Avatars are used for user profile images and creating a connection with the audience. Avatar plugins allow users to upload custom avatar images and showcase additional information about content authors.
Icons are symbols that represent particular information. Icon plugins come with collections of symbols that help you choose the relevant icon for particular information.
These plugins will help you add avatars and icons to your pages.
IconPress Pro: Icon Management Plugin
Iconize
LivIcons Evolution
User Avatars Plugin
SVG Avatars Generator
My Team Showcase
Heroes Assemble: Team Showcase Plugin
A Fancy WordPress Author List
All-in-One Support Button and Call Request
Social Sider
Easy Side Tab Pro
Button Plugins for WordPress
Buttons are the most effective tools to get your users to take action. You can use buttons to redirect users to a desired action like buy now, sign up now, purchase, or to a promotions link. These button plugins will get your users to take action.
Buttons X: Powerful Button Builder
Floatton: Floating Action Button
Easy Social Share Buttons
iframe Plugins
These plugins allow you to display content from external sites and sources on your website, so users don't have to leave your site.
Advanced iFrame Pro
Live Chat Unlimited
Live Chat Complete
Popups and Opt-in Plugins
How to convert your visitors to subscribers is an ongoing question. Popup opt-ins have the highest conversion rate of all opt-ins. But for popups to be effective they have to well designed and used thoughtfully. These popup and opt-in plugins will help you gain more conversions.
ConvertPlus
Slick Popup Pro
WordPress Popups Plugin
Ninja Popups
ConvertPlus Pop Plugin
Layered Popups
Popup Press
Opt-in Panda
Master Popups
Sidetabs Layered Popups
Popping Sidebars and Widgets
WordPress Vimeo YouTube Popup Plugin
Popup and Modals Windows Generator
WordPress Plugins
20+ Best Popup & Opt-In WordPress Plugins
Nona Blackman
WordPress
How to Create an Exit Popup With the Layered Popup Plugin for WordPress
Esther Vaati
Hover Effects Plugins
These plugins allow you to create engaging effects for images on your website. Effects like transitions, transforms, flips, animations and more.
Media Hovers
Hover Effects Pack
Image Hover Effects
Marvelous Hover Effects
Weather Plugins
For event planners and venue managers the weather is part of their planning. Bad weather can mean low attendance. These weather plugins help planning.
Astero
Always Sunny
Simple Weather
WordPress
6 Best Weather WordPress Widgets & Plugins
Kyle Sloka-Frey
Countdown and Timer Plugins
If you have a sale, product launch, or event to sell tickets for, these countdown times plugins will create a sense of urgency for users on your website and increase your conversions.
CountDown Pro
Product Countdown
WooCommerce Coupon Countdown
Countdown Timer
Viavi Countdown
Woo Sale Revolution
Everest Coming Soon
Notification Plugins
Notifications have a greater rate of opt-in than emails. They grab attention by appearing directly on the browser. This results in increased engagement, high rate of return audience, increased conversions and revenues. If you're serious about engaging an audience you already have, these notification plugins will bring users back to your website for more.
Apex Notification Bar
Advanced Floating Content
Warning Old Browser
HashBar Pro
Some Useful Bonus Plugins
Even though they don't fit into any category, these plugins are very useful for your website.
Reviewer WordPress Plugin
Yellow Pencil Visual CSS3 Editor
Cool Timeline Pro
Translator Revolution
XL WooCommerce Sales Triggers
WooCommerce Availability Scheduler
Conclusion
I hope the WordPress media plugins highlighted in this article will help you in your journey build a dynamic WordPress website for your business.
If you want to improve your skills building WordPress sites, check out our free WordPress tutorials.
Also the following articles will help you learn more about the WordPress media plugins available on CodeCanyon.
WordPress
15 Best WordPress Audio Player and Video Player Plugins
Jane Baker
WordPress
How to Add the Sticky HTML5 WordPress Music Player to Your Site
Monty Shokeen
WordPress
How to Use the WordPress Responsive YouTube Playlist Video Player Plugin
Sajal Soni
WordPress Plugins
7 Best WordPress Video Gallery Plugins
Kyle Sloka-Frey
WordPress
20 WordPress Video Plugins and Players to Add Engagement
Rachel McCollin
WordPress Plugins
How to Create an Interactive Slider With the LayerSlider Plugin for WordPress
Daniel Strongin
WordPress Plugins
20 Best Social Plugins for WordPress
Nona Blackman
WordPress
Automatically Create a Multilingual WordPress Site With a Translator Plugin
Esther Vaati
WordPress
Best Video Background Plugins for WordPress
Nona Blackman
WordPress
6 Best Weather WordPress Widgets & Plugins
Kyle Sloka-Frey
WordPress
20 WordPress Video Plugins and Players to Add Engagement
Rachel McCollin
WordPress Plugins
20+ Best Popup & Opt-In WordPress Plugins
Nona Blackman
by Lorca Lokassa Sa via Envato Tuts+ Code https://ift.tt/2q7nF8u
1 note
·
View note
Photo
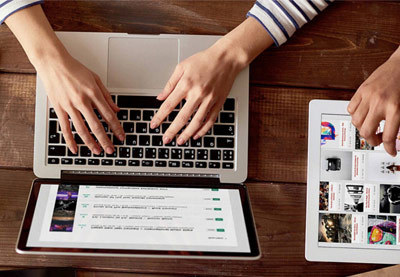
Show an Event Calendar With a Free WordPress Calendar Plugin
Many businesses need to share event calendars with customers. For example, a restaurant can use an event calendar to advertise special musical events, or holiday menus. Similarly, schools can use event calendars on their websites to let students and teachers know about any upcoming activities in school related to sports, exams or other events.
Event calendar plugins are helpful for individuals as well if they want to keep track of different things they are supposed to do. For example, you could use an event calendar plugins to mark important meetings and events like birthdays of friends and family.
Choose the Best WordPress Event Calendar Plugins
Looking for the best WordPress event calendar plugins? This list has all the top event calendar plugins you need to consider.
Nona Blackman
28 Feb 2019
WordPress Plugins
Insert a Calendar Into WordPress With the Pro Event Calendar Plugin
Displaying a calendar on your website is a must for many business owners. The Pro Event Calendar plugin makes it easy to add an event calendar to your posts...
Daniel Strongin
24 Sep 2019
WordPress Plugins
20 Best WordPress Calendar Plugins and Widgets
Whether you need an event calendar plugin, a booking system with payments, or a Google Calendar widget, this list of plugins will have something to improve...
Esther Vaati
06 Sep 2019
WordPress
Guide to WordPress Event Calendar and Booking Plugins
WordPress calendar plugins let users make bookings, schedule events, pay for appointments, and more. If your website does any of these things, you need a...
Lorca Lokassa Sa
21 Sep 2019
WordPress
In this tutorial, you will learn how to use the free My Calendar WordPress event management plugin in order to create events. The plugin has a lot of amazing features that we will use in the tutorial.
What We'll Be Building
Our focus in this tutorial will be on creating a calendar that allows a company to mark all the events that they manage like parties, weddings, art exhibits etc. They will be able to see all the future and past events.
We will make the events color coded so that they are easy to identify. Users will be able to click on any specific event category and only see matched events in the calendar. Clicking on any event will show a pop-up with all the basic information about the event like its timing and location.
We will begin by installing the plugin. Then, we will change some settings to modify the input and output. After that, we will create some event categories, add some event locations and finally add the events to our calendar.
Also, there is a good chance that you will want to make some changes to the appearance of the event calendar so that it blends in with the rest of your website. Therefore, we will add some of our own CSS rules in the last step to make some cosmetic changes in the calendar.
Creating an Event Calendar
Setting Things Up
The first step towards creating our event calendar would be installing the My Calendar plugin. Once you have installed and activated the plugin, go My Calendar > Settings in the admin dashboard. You will see a bunch of tabs there.
Click on General and then specify the ID for the page where you want the event calendar to appear. In our case, it is 118. This step is optional and the plugin will automatically create an event calendar page for you when you publish your first event. However, it is good know know how to show the event calendar on a specific page.
Whenever you create a new event with this plugin, you will have to fill out some details about the events. This could include many things like event location, short description etc Not all of these input fields are activated by default. Therefore, you should now head to the Input tab and check the fields that you want to appear, as in the image below.
You have probably noticed that there are a lot of buttons and dropdown menus on the event calendar page besides the calendar itself. The order in which these buttons are displayed is controlled by settings in the Output tab. You can drag the elements up and down to display them in a specific order. Just drag an element below the Hide row in order to prevent it from displaying.
It is possible to control what information is shown to users in the pop-up once they click on an event by toggling some checkboxes under Single Event Details.
Creating Event Categories
Now that we have set everything up, it is time to create actual content for our events calendar.
We will begin by adding some categories that will be used to mark different events. This plugin gives users the option to only see events from specific categories. Events can be filtered this way once they have been assigned different categories.
To add a new category to the calendar, simply go to My Calendar > Manage Categories and then specify a name and label color for the category. You can also choose to display an icon before the category by selecting one from the Category Icon dropdown.
Once you have added all the details for a category, click on the Add Category button to add the category to the event calendar.
Adding Event Locations
Any events that you organize will take place somewhere. The plugin does a great job when it comes to specifying locations of different events.
You can add a new location to the calendar plugin by going to My Calendar > Add New Location. The next page will contain a lot of input fields that ask for different details of the location. This includes the usual things like street address, city, postal code and country etc. You don't have to fill in all these values. Some of these details can be skipped.
Make sure that you have entered a name for the location at the top. This will help you easily identify these locations in the dropdown menu when creating events.
In this example, we will add a location for an art exhibit that is taking place in Kentucky, USA. The address is fictional but it will give you an idea about filling out your own details.
The plugin will give users a link to Google Maps with the address that you specified. This makes it easier for people to figure out exactly where the event is happening. Any locations that you add to the plugin can be edited by clicking on My Calendar > Manage Locations.
Adding Events
We can now start adding events to our events calendar. To add an event, simply go to My Calendar > Add New Event.
Now, fill out the title and description of the event. The title is displayed within the dates on the event calendar. The description will be displayed on the event description page when users click on the Read More link in the pop-up. Set the event category to Exhibit from the dropdown menu.
You can now specify a date and time for the event. There is a good chance that at least a few events that you add to the calendar will be recurring events. In this case, it would be very time consuming to add all the repeated events to the calendar one by one.
To make things easier for you, the My Calendar plugin gives you the option to specify the repetition pattern for an event. This means that you can specify how many times an event will repeat and the frequency with which it will repeat.
At the beginning of the tutorial, we checked the box to enable a short description input field for events. This allows us to provide a short summary of the event which will be displayed in the pop-up whenever a user clicks on the event in the calendar.
You can specify a location for the event at the bottom of the Add New Event page. Simply choose Art Exhibit (Kentucky) for this example. After that, click on the Publish button at the top or bottom of the page.
Change the Styling of the Event Calendar
After following all the steps in this tutorial, your event calendar will look like the image below. If its styling is different from the following image, simply go to My Calendar > Style Editor and then pick the twentyeighteen.css file from the dropdown in the sidebar as the primary stylesheet for the calendar. There are a bunch of other themes that you can apply to the calendar. Just pick one that you like the most. Some of them like inherit.css are meant to provide the minimum possible styling so that most of the style rules from your theme are automatically applied to the calendar.
The style editor page shows all the CSS rules from the selected file that are applied to the calendar. You can make all kinds of changes here and they will be reflected on the event calendar page.
For now, we will simply adjust the spacing and fonts that are applied to different elements like the table caption, header and category legend at the bottom. We will also add some of our own style rules to adjust the appearance of the calendar to our liking.
Here are all the rules that we add to our event calendar. Simply place them at the bottom of the style editor and the changes will be reflected on the event calendar page once you click on Save Changes button at the bottom.
.mc-main .my-calendar-header .no-icon, .mc-main .mc_bottomnav .no-icon { display: inline-block; /* width: 12px; */ width: 15px; height: 15px; vertical-align: middle; margin-right: .25em; border-radius: 12px; position: relative; top: -2px; border: 1px solid #fff; } .mc-main .my-calendar-header span, .mc-main .my-calendar-header a, .mc-main .mc_bottomnav span, .mc-main .mc_bottomnav a, .mc-main .my-calendar-header select, .mc-main .my-calendar-header input { color: #313233; color: var(--primary-dark); /* border: 1px solid #efefef; border: 1px solid var(--highlight-light); */ border-radius: 5px; padding: 4px 6px; font-size: 14px; /* font-family: Arial; */ background: #fff; background: var(--secondary-light); } .mc-main .my-calendar-header input:hover, .mc-main .my-calendar-header input:focus, .mc-main .my-calendar-header a:hover, .mc-main .mc_bottomnav a:hover, .mc-main .my-calendar-header a:focus, .mc-main .mc_bottomnav a:focus { background: black; } div.mc-print { margin-top: 2rem; } .mc_bottomnav.my-calendar-footer { margin-top: 5rem; } .mc-main button.close { top: 5px; left: 5px; } .mc-main .calendar-event .details, .mc-main .calendar-events { border: 5px solid #ececec; box-shadow: 0 0 20px #999; } .mc-main .mc-time .day { border-radius: 0; } .mc-main .mc-time .month { border-radius: 0; border-right: 1px solid #bbb; } .mc-main .my-calendar-header span, .mc-main .my-calendar-header a, .mc-main .mc_bottomnav span, .mc-main .mc_bottomnav a, .mc-main .my-calendar-header select, .mc-main .my-calendar-header input { color: #313233; color: var(--primary-dark); border-radius: 0px; padding: 4px 10px; font-size: 14px; background: #fff; background: var(--secondary-light); border-bottom: 1px solid black; } .mc-main th abbr, .mc-main .event-time abbr { border-bottom: none; text-decoration: none; font-family: 'Passion One'; font-weight: 400; font-size: 2.5rem; line-height: 1; } .mc-main caption, .mc-main.list .my-calendar-month, .mc-main .heading { font-size: 2.5rem; color: #666; color: var(--highlight-dark); text-align: right; margin: 0; font-family: 'Passion One'; text-transform: uppercase; } .mc-main .category-key li.current a { border: 1px solid #969696; background: #dadada; }
Now, go to the event calendar page and click on one of the events that you added. This should open a nice pop-up that shows all the basic details of the event like its timing, location and a short description.
Final Thoughts
In this tutorial, we learned how to use the My Calendar plugin in order to create a basic event calendar for our website. The plugin makes it really easy to create and add events along with a simple description.
However, there are still a couple of things missing from the plugin like widgets and a more advanced description page that provides extra features like payment options and the ability for users to add reviews about an event. The plugin is also a bit hard to style. If you want to use a more advanced event calendar plugin on your website, you should take a look at the WordPress event calendar plugins on CodeCanyon.
WordPress Plugins
Choose the Best WordPress Event Calendar Plugins
Nona Blackman
WordPress Plugins
Insert a Calendar Into WordPress With the Pro Event Calendar Plugin
Daniel Strongin
WordPress
20 Best WordPress Calendar Plugins and Widgets
Esther Vaati
WordPress
Guide to WordPress Event Calendar and Booking Plugins
Lorca Lokassa Sa
WordPress
8 Best WordPress Booking and Reservation Plugins
Lorca Lokassa Sa
WordPress
How to Create a Google Calendar Plugin for WordPress
Ashraff Hathibelagal
by Monty Shokeen via Envato Tuts+ Code https://ift.tt/2C23cok
1 note
·
View note
Photo
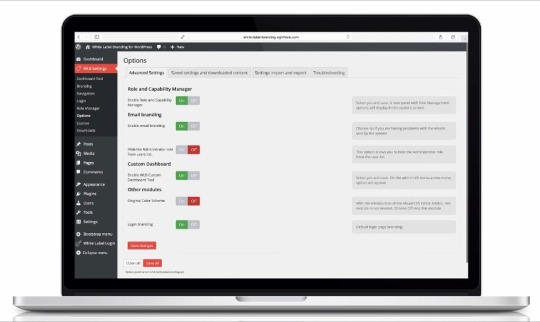
10 Best White Label Branding Plugins for WordPress
What is White Label Branding?
Your local supermarket chain has its own supermarket brand products it offers to its customers. These supermarket brand products are cheaper compared to the same product sold under a fancy brand name. Does this mean that the supermarket manufactures its own products?
Not at all. The products are manufactured by third parties contracted by the supermarket chain to produce products under the supermarket brand name. The supermarket chain gives an impression that it has manufactured the products.
This is called white label branding. It is a worldwide phenomena. And it is not limited to supermarket chains. All industries and sectors practice it.
Think of big electronic manufacturers and retailers of your favorite desktops, laptops, mobile phones and so on. They too put their brands, logos, and serial numbers on white label products.
White label branding is not restricted to physical products. It is widely practiced in digital products too.
In this article II will explain how white label branding works in WordPress then I will explore some white label branding plugins for WordPress that are available on Code Canyon.
WordPress sites can have a cookie cutter appearance recognizable by many. The content management side especially. You can customize the appearance of the back-end to reflect your clients’ brands by using white label branding plugins.
How Does White Label Branding Work for WordPress?
Here is how white label branding works for digital products: you buy white label digital products and services, put your brand name on them, and resell them as your own.
But white label branding for WordPress works differently.
How White Label Branding Works for WordPress Developers
As a developer you have many clients. This means you have to create their websites—to reflect their unique brands. Coding a new WordPress website from scratch every time you have a new project is costly both in time and money.
Turnkey WordPress websites offer a simple solution. You can use the same website template—both front-end and back-end—for different clients. You only need to customize the website to reflect specific identity of each client.
This involves adding the brand name, logo, and specific graphics and content that identify and differentiate each clients. It means removing standard logo, graphics and information that comes with the WordPress and personalizing the website with clients’ content.
Most of the time what customers see when they visit a website is the front-end. This is the public image that represents the company.
But there is more to a website than the front-end. There is the back-end with the dashboard that customers don’t get to see.
People who run the website use the back-end every day. They want to manage their own content, inventory, images and so on. They do this using the dashboard. Every time they log in to the back-end they want to see their brand reflected and not the cookie cutter WordPress logo and messages.
Using White Label Branding Can Help Your Reputation as a Developer
Imagine every time clients log into the back-end they are greeted by the same standard WordPress dashboard message and WordPress logo. They wonder why am I not greeted by my own brand name? My own logo? My own messaging?
This is where using turnkey websites can reflect badly on you to your customers and ruin your reputation. If don’t do it right your clients will end up feeling you took them for their money and gave them a standard template you use with every client.
Full customization of both the front-end and the back-end of the WordPress website is important because it is a total reflection of the client’s business not only for their customers who only see the front-end but also for the business owners and employees who have to work with back-end tools. This kind of user experience and satisfaction reflects well on you as a developer.
How White Label Branding is Done
White label branding can be done manually or by using plugins.
The manual process involves making changes to your WordPress theme's PHP code by inserting snippets of code to perform the following tasks:
replacing WordPress logos with the client’s logo
replacing standard login images with client’s image
replacing the header
customizing the admin menu
replacing footer text like Powered by WordPress with your own wording
If you have no coding knowledge or little coding experience then manually white labeling is overwhelming. Same applies to the experienced developer pressed for time.
Of course, you don’t have to do all that manually. There are plugins you can use to do white labeling.
What Do White Label Branding Plugins Do?
They give you the ability to control and transform the appearance the back-end.
They help you customize the back-end of your WordPress without coding.
They come with extensive documentation that helps you do the customization by yourself.
You can completely transform the look of the dashboard, customize the appearance of the admin menu, logo, header, the width of the logo, the footer logo, and the login form, as well as replace standard WordPress messages with customized messages that reflect clients’ brands.
White Label Branding Plugins on CodeCanyon
On CodeCanyon you will find white label branding plugins that will help you customize your clients’ WordPress backend to reflect their brands.
1. White Label Branding for Multisite
White Label Branding for Multisite lets you control the branding of the main site and all sub-sites in a network of websites powered by WordPress Multisite.
It also allows you to:
give each sub-site the ability to do their own branding and customize their menus for the editor role
customize the menus and logos on each sub-site in your WordPress Multisite Network
replace the WordPress logo from the log-in screen and dashboard with your own identity or even your client’s
add custom dashboard meta box viewable only to editors or all users with your own welcome message or help
User bruceakinson says:
Amazing features, worked perfectly for what I needed it to do.
2. White Label Branding for WordPress (Single Site)
With the White Label Branding plugin you have the ultimate tool for customizing the WordPress admin and login screen.
It allows you to take full control over branding in the WordPress admin by:
customizing your login screen, menus and logos
changing the color scheme of the entire admin setion
creating your own advanced login templates
creating new user roles and assign capabilities
Not only that but, you can decide who has access to what features by hiding elements for other administrators.
You can even create a fake administrator account! This is useful if you want to give your clients “admin” access, but still limit what they have access to. The real administrator will also be hidden from the users list. This way a client with the “fake” administrator account will never know that they don’t have full access to all features.
Usery kops says
The ultimate white label tool - fantastic.
3. White Label Login for WordPress
White Label Login makes it easy to customize the default WordPress login.
It provides the following UI styles for login:
slide login
push login
modal login
In addition you can:
customize e-mails for registration and reset password
customize login and logout redirects by user role
insert dynamic login and logout links in the WordPress menu
insert dynamic buttons in your content with shortcodes
rewrite rules for wp-admin.php and wp-login.php
create social login support for logins using Facebook, Twitter, Google+, Linkedin and Microsoft Live
Also included in its list of dynamic features is a visual CSS editor for customizing colors and fonts for each screen. The plugin supports 600+ Google Fonts.
User by naftas says:
Very flexible plugin. Gives very good possibilities for the website developer for fine tuning of all login features.
4. WPAlter
WPAlter completely changes the style of the WordPress admin panel to your desired color theme.
Using this plugin in you can:
remove WordPress texts and logo
create custom login themes and add a custom logo for login and admin pages
hide, rename and re-arrange admin menu items.
hide admin menu items based on user roles
You can also white label emails.
WPAlter is tested for compatibility with popular plugins: Visual Composer, WP Super cache, WP Total cache, Contact form 7, WooCommerce.
User WPBlueLabel has this to say:
Great plugin. Allows tons of customisation that really enhances the WordPress Dashboard experience. Thanks a lot.
5. Material Admin
This multisite-compatible white label branding WordPress theme comes with:
100 elegant themes and gives you an option to create your own custom theme
20+ custom dashboard widgets for site and visitor statistics
LTR and RTL modes so can be used for any language
In terms of white label branding of the WordPress back-end you can easily:
rearrange menu and submenu items
change menu icons
enable or disable menu and submenu items
It also comes with a fully customizable login screen.
User thesourcep says.
Works awesome straight out of the box. Great default layouts and options and support is second to none. Awesome job—thank you!
6. Legacy Admin
Legacy Admin is an advanced, feature-rich white label WordPress admin theme.
It comes with the following:
20 elegant themes fully customizable
fully customizable beautiful login screen theme
admin menu management
Here is what Legacy Admin allows you to do in terms of white label branding:
customize admin top bar (all links and CSS Styles)
customize footer
customize login section
customize admin look and feel to represent your branding needs
customize the admin menu
add a custom logo and favicon for the admin panel
It is translation-compatible with RTL and LTR modes that can be used to support any language.
Finally, Legacy Admin is also multisite-compatible so it’s easy to install and ready to use on a multisite network.
Check out the demo and see why user Spac3Rat says:
Absolutely amazing. It transforms the WordPress admin drastically, making it pop. Love it.
7. Slate Pro
Say goodbye to the run-of-the-mill WordPress. With Slate Pro:
you can reimagine WordPress with a clean and simplified design
change or remove all WordPress branding
custom brand clients’ admins with custom colors and a custom login screen
Your clients don’t even have to know that you’re using WordPress! Also Slate Pro is multisite-compatible so you can control the look of all sub-sites.
User ATingle says:
An A1 plugin, oozes class—very, very impressed. Major props to the designers.
8. WPShapere
WPShapere is a very popular back-end customization plugin on CodeCanyon. It’s a very light plugin, easy to install, and easy to customize.
Here is what you can do with this powerful plugin:
remove the WordPress logo from the admin bar
upload your own logo to login page and on admin bar
customize login page design
customize the admin theme with 16 in-built themes to kick start your project
remove the WordPress default dashboard widgets
add your own content widgets and RSS widgets
customize admin bar links
add your custom logo, text content or links on the footer
create user access restrictions
User ChazzLayne says:
The perfect back-end customization suite, it's the missing piece that WordPress should have given developers from the start!
9. Ultra Admin
Ultra Admin is a combination theme and white label branding plugin that helps you design a WordPress site for your clients with your own company branding.
It comes with 30 built in theme templates. You can customize the admin menu, top bar, buttons, content boxes, typography, forms, text and background colors, logo and so on.
White Label Branding features will help you transform the back-end by:
rearranging menu and submenu items
changing menu icons
controlling top bar links
customizing footer
setting plugin access permissions
customing the login page
User Eight7Teen says
This is by far the most full-featured WP admin white label plugin available. It is incredibly well built and the developer seems eager to solve any issues you may run into. Definitely recommend!
Bonus
Here's one more plugin that isn't specifically a white label branding plugin, but could be useful for any white label branding project.
Menu By User Role
Menu by User Role gives you complete control over the menus in your WordPress powered website. You have the ability to:
create public menu
create a menu for logged-in users
create a separate menu for each user role defined in your website
In addition you can create a custom navigation menu, which can be used instead of thw default menu. In order to use this feature it must be registered in the theme’s functions.php file.
You can easily add pages, posts, categories and custom links to the menu. And you can even create multi-level menus with just a few clicks and organize them by drag and drop.
This plugin can be used together with White Label Branding for WordPress.
User deezeeweb says
Works really well - with every theme and user plugin I've used.
Conclusion
These are some white label branding plugins that caught my eye. You can find more plugins on CodeCanyon.
WordPress
Top WordPress Security Tips for Admins
Reginald Dawson
WordPress
Persisted WordPress Admin Notices: Part 1
David Gwyer
WordPress
10 Best WordPress Slider & Carousel Plugins of 2019
Nona Blackman
WordPress
Best Affiliate WooCommerce Plugins Compared
Lorca Lokassa Sa
WooCommerce
20 Best Shipping & Pricing WooCommerce Plugins
Nona Blackman
by Lorca Lokassa Sa via Envato Tuts+ Code https://ift.tt/2BWoyU8
1 note
·
View note
Photo
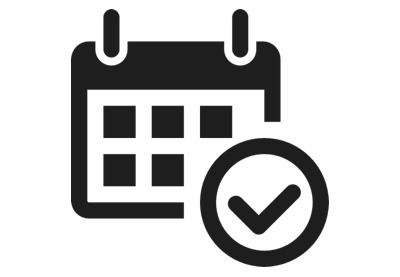
How to Create an Appointment Booking Calendar With a WordPress Plugin
Many businesses require their customers to book appointments. Whether you are a yoga instructor, barber, or a counselor, you will need to book appointments to ensure that all your customers are served in an orderly and timely manner. Posting a phone number to call to book appointments on your website is an absolute must. However, this should not be the only way for your customers to book an appointment.
In today’s internet-driven world, customers are now used to having the option to not only call your business to book an appointment but are used to being able to book an appointment at their convenience twenty-four hours a day, seven days a week. This makes having a way to book appointments from your website a necessity. Without a way for you to book appointments from your website, you will lose out on new and returning customers.
8 Best WordPress Booking and Reservation Plugins
In the digital age, users are online 24/7. Everyone prefers to check availability and make appointments, reservations, or bookings online. They want to do...
Lorca Lokassa Sa
12 Apr 2019
WordPress
20 Best WordPress Calendar Plugins and Widgets
Whether you need an event calendar plugin, a booking system with payments, or a Google Calendar widget, this list of plugins will have something to improve...
Esther Vaati
06 Sep 2019
WordPress
Choose the Best WordPress Event Calendar Plugins
Looking for the best WordPress event calendar plugins? This list has all the top event calendar plugins you need to consider.
Nona Blackman
28 Feb 2019
WordPress Plugins
Get Started With a Free Booking Calendar Plugin in WordPress
I'm going to show you how to use the Easy Appointments plugin for WordPress to create an appointment booking system. We’ll start by exploring the basic...
Sajal Soni
18 Mar 2019
WordPress
How to Add a Calendar to Your WordPress Site With the Events Schedule Plugin
Build a schedule for your website, complete with Google Maps integration, call to action buttons, and a complete automated booking and ticketing system for...
Jessica Thornsby
14 Mar 2019
WordPress
Best Free WordPress Calendar Plugins
In this post, we will review some of the most popular free WordPress calendar plugins that you can start using in your projects.
Monty Shokeen
18 Jun 2019
WordPress
Thankfully, WordPress has a collection of booking plugins that you can easily integrate into your site. However, many of these booking plugins do not have all of the functions that you need to make it customizable to your business. The top-selling WordPress plugin, Booked, is a fully-featured appointment booking plugin that can be tailored to your specific business and help you grow your customer base. In this article, I am going to go over how you can use this plugin to create a booking system on your WordPress website.
What We Will Be Building
We will be building a booking system for our massage business. This business runs every Monday through Friday from 9 a.m. to 3 p.m. We will create a calendar that will allow our customers to sign up for one of the three types of massages that we offer during our business hours. Here is what the booking calendar that our customers will use to complete their massage bookings.
Creating a Booking Calendar
After you have installed the Booked plugin, select Appointments > Calendars from the WordPress admin menu. From here, we will create a massage booking calendar. Under the name text field, type Massage Bookings and click the Add New Custom Calendar button. This will create a custom calendar.
Next, we will begin adjusting the settings of the calendar. So head on over to Settings in the appointments plugin on the WordPress dashboard. We will first begin editing our calendar to fit our massage business by adjusting the settings under the general tab.
Under the Booking Options section, we would like to make sure we know the name of the person booking an appointment with us, so we will select the Require “Name” Only option.
Scrolling down, the general settings, we will come to the Time Slot Intervals section. This will allow us to choose how long each massage appointment will last. Each massage appointment will last one hour, so we will select the Every 1 hour option from the drop-down menu.
Now we will change the Cancellation Buffer. We want to make sure that appointments can’t be canceled too close to the appointment time, so we can charge customers if they don’t cancel early enough. We will select 2 hours in the drop-down menu here so customers can’t cancel their appointment within two hours of the appointment.
Next, we go to Display Options and check the Hide weekends in the calendar options as our business won’t be operating on the weekends, as there is no need to show weekends on the calendar.
Now that we are done with the general settings, we need to adjust the time slots available on the calendar. Click the time slots tab and you will be brought to the editing page. Under editing time slots, choose the Massage Bookings calendar so we edit our custom calendar.
We are running our business Monday through Friday from 9 am to 3 pm, so will need to reflect this in the time slots. Under Monday, click the Add Timeslots button. Next, click the Bulk button to allow us to create multi-time slots. Select 9 am in the Start Time dropdown menu, 3 pm in the End Time menu, and 1 space available in the last dropdown menu. Since there is only one massage practitioner, we will only allow one appointment to be booked.
Massage Types
We will be offering three types of massages for our business, a normal, deep tissue, and hot stone massage. We want to know what massage our customers will be signing up for when they book their appointments. To gather this information we are going to set up our own custom fields in the booking. Head on over the Custom Fields tab on top of the booked settings menu. Make sure to choose the right calendar to edit by selecting the Massage Bookings calendar from the drop-down menu.
Next, click on the + radio buttons button. From there, check the required field box to make sure that the customer lets us know what type of massage he wants to receive before their booking will be accepted. In the Enter a label text field, type in "Type of massage you would like to receive.” Next, we add in two more fields using the + radio button button and type in our three types of massages into these option fields. Here is what the custom field should look like.
Adding the Calendar to Our Website
Now that we have created the booking calendar, it is now time to add it to our website. Select the Shortcodes tab in the plugins setting and scroll down to the Display a Custom Calendar section and copy this shortcode to your clipboard. From there, create a new page and paste this shortcode into the text field. Now preview the page and the booking calendar that you just created will be ready to use!
To view all of the appointments that were made you can head on over to your WordPress dashboard and select Appointments > Appointments from the admin menu. You will then be taken to another calendar where you can click on each day and view the appointments booked and plan your business accordingly.
Feel free to view the video below to watch the calendar creation process in action.
Getting the Most Out of the Booked Plugin
In this article, we only covered a few of the features available on this complete booking WordPress plugin. As mentioned, this plugin allows you to fully customize the booking experience. Below are some other features that you can use to further customize the booking experience for your customers.
Pending Appointments
Depending on your business, you may need to review a booked appointment before you can accept this booking. With this plugin, you can easily set up manual approving of appointments in the General settings tab. In the New Appointment Default section, choose set as pending. Any custom information that you need to receive from your customer in order to review, you can add in the custom fields tab as you can create checkboxes, text fields, and much more.
Custom Emails
Through the various stages of the booking process, emails will be sent out to your customers. When an appointment is confirmed, cancelled, or approved, an email from your WordPress platform will send a custom email to the customer. In the plugin's settings, you can customize these emails to say anything you would like. Including anything that will make your customers more comfortable and excited about your business would be best here.
Editing Appointments
One important feature that must be mentioned is the ability to edit appointments. This will be incredibly useful when you need to change the date of the appointment. If you go into the Appointments tab in the WordPress dashboard, you will be brought to a calendar of all your appointments. From there, click on the specific date that you want to change the date or time in and select the appointment that you want to change. An edit appointment pop up will appear where you can update the time and date and notify your customer about this change.
Conclusion
When running a business that requires the booking of appointments, it is necessary to not only have a phone number for your customers to call and book an appointment but to also have a way your customers to make these bookings on your website.
By using the WordPress plugin Booked, you can easily add a fully customizable booking calendar to your website that fits your business needs. To give this plugin a try, head over to CodeCanyon and check out the Booked WordPress plugin. And while you're here, check out some of the other great WordPress plugins available from CodeCanyon.
WordPress
8 Best WordPress Booking and Reservation Plugins
Lorca Lokassa Sa
WordPress
20 Best WordPress Calendar Plugins and Widgets
Esther Vaati
WordPress Plugins
Choose the Best WordPress Event Calendar Plugins
Nona Blackman
WordPress
Get Started With a Free Booking Calendar Plugin in WordPress
Sajal Soni
WordPress
How to Add a Calendar to Your WordPress Site With the Events Schedule Plugin
Jessica Thornsby
WordPress
Best Free WordPress Calendar Plugins
Monty Shokeen
by Daniel Strongin via Envato Tuts+ Code https://ift.tt/34erLKC
1 note
·
View note
Photo
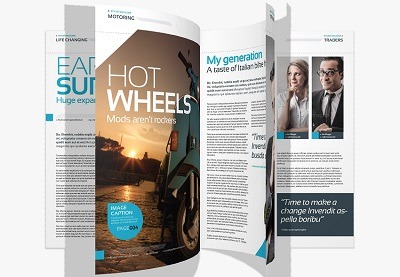
How to Create a Travel Gallery Flipbook With the Real3D WordPress Plugin
Whether you are running a blog, business, or any type of website, you will need to attract and keep your audience's attention to be successful. A major part of attracting and keeping users' attention is to display eye-catching photos on your website.
While displaying these images in a gallery is the usual way to present them, there are other creative and interactive ways to not only show these images but other text and documents. One creative way to display your website's content is with flipbooks.
Flipbooks give your audience a fun and interactive way to view your website's content by allowing them to feel like they have a book in front of them. Flipbooks also help you display your content in an organized way that is not possible with the use of a gallery or other ways of displaying your content.
Best WordPress Flipbook Plugins Compared
Displaying your content as a flipbook is an effective way to share content, and there are a wide range of WordPress flipbook plugins available on the market...
Jane Baker
28 Jan 2019
WordPress
17 Best WordPress Gallery Plugins
Tame chaos and transform your content using one of the best WordPress gallery plugins available on CodeCanyon. Read on to find out about these WordPress...
Jane Baker
01 Feb 2019
WordPress
10 Best WordPress Gallery Plugins of 2019
There are thousands of WordPress gallery plugins available on the market today, so choosing one that works for you can sometimes be confusing, time...
Lorca Lokassa Sa
27 May 2019
WordPress Plugins
Best Free WordPress Gallery Plugins
With so many plugins out there, it can be a time-consuming process to find the right one for your needs. In this tutorial, I'll help you figure out the best...
Monty Shokeen
13 Aug 2019
WordPress
In this post, we'll be working with the number one best selling WordPress flipbook plugin on CodeCanyon: Real3D FlipBook. This plugin will help you create a stunning-looking flipbook within minutes and help keep your audience interested in what your website has to offer.
In this article, I am going to show you just how simple it is to create a 3D flipbook with the Real3D FlipBook plugin to grab and keep the attention of your audience.
What We Will Be Building
The flipbook that we will be creating is going to be for our travel blog. In one of our posts in the blog, we are going to add a 3D flipbook created by the Real3D FlipBook plugin to show off the photos that were taken on our last trip to Europe.
We visited four different countries in Europe and are going to show off our top four photos for each country in the flipbook to show our travel blog audience. To make this flipbook visually appealing and easy to navigate we are going to change around the styling of the flipbook, how the actual 3D book flipping functions, add a table of contents, and enable a few other features that will enhance the viewer's experience.
Creating the Flipbook
To create this travel flipbook, you'll need to have the Real 3D Flipbook plugin installed. Select Real 3D Flipbook from the WordPress admin sidebar and click Flipbooks.
This will open up the Flipbook creation page where we will then click the Add New button next to the title, Flipbooks, on the top of your screen. We will label our new flipbook European Travel Flipbook.
Now it is time to start creating the pages in the flipbook. There are two options to choose from here. We can create our flipbook pages from a PDF or from JPEG images. Seeing as we took these photos and have them in the JPEG format, we will click the Select Images button below JPG Flipbook which will bring up the WordPress media library. From there we are going to select our sixteen images. We are going to have four images for each of the four countries that we visited. Once we have selected our images, click the Send to Flipbook button at the bottom right-hand corner of the media library.
Now that we have all the images that we would like included in our flipbook, we need to create a table of contents so our viewers will know what country each image is from. On the top of the flipbook dashboard, click the Table of Contents tab.
This will bring up the table of contents editor where we can add in as many titles and page numbers as we need. Each grid represents a line of the table of contents. Again, we want to let our audience know where each country's photos begin and end. The first four photos are from Italy, so we will type in Italy in the first field and then the page that it starts on in the next field which is 1. Next, click the Add Item button to add another table of contents line and we will repeat this process for the other three countries. Here is what our final table of contents will look like.
The next step for creating our flipbook is to adjust the general settings. Click the General tab at the top of the Real3D FlipBook dashboard. From here we are going to adjust two different settings, the view mode and the page flip sound. For the view mode, click the webgl setting from the drop-down menu. This will make sure that the flipbook that is displayed contains realistic 3D page-flipping with lights and shadows to create a realistic effect. Moving on to the page flip sound setting, we will simply click the enabled setting to allow the page sound to play. Adjusting these settings ensures that we create as realistic as a flipbook as we possibly can to keep our viewers interested in viewing the flipbook. Here is what our flipbook looks like now.
Our flipbook is really starting to come together, but the styling of the entire flipbook is rather bland and we would like it to be more pleasing to the eye, so we are now going to adjust the user interface in the UI tab on Real3D FlipBook dashboard. Once you click this tab, you will be directed to the UI menu where will change the background color and side navigation bars to really make the flipbook pop out of the screen.
First, click on the Flipbook Background accordion and then click on color. We will choose the default blue color at the bottom right of the color selector. Next, click on the Side Navigation Buttons accordion menu to expand out the menu. To highlight the navigation button, we will change the background color to be red which will contrast nicely with the blue background color.
To make the navigation buttons more appealing to click we are going to change the radius so the button will be a circle instead of a square. We will type 30 into the radius field. The last styling change on the button is going to be the size. Again, we want this navigation button to invite the user to keep navigating, so we will bump up the size to 48. Finally, click the update button at the bottom right-hand corner of the plugin dashboard to save all of the updates that we just made.
The last setting we are going to change is in the Menu Buttons tab. Click on the Menu Buttons tab and head down to the Download PDF accordion menu and click it. We would like our travel blog audience to be able to download this flipbook so they can share it with their friends and view it offline. To allow this, we are going to click Enabled from the drop-down menu next to the Enabled menu item at the top of the Download PDF accordion menu.
There you have it, we have just created a stunning looking flipbook for our European travels to be displayed on our travel blog. You can see this entire flipbook creation process in the video below.
Getting the Most Out of the Plugin
In this article, we looked at how you can use the flipbook plugin to create a virtual book of travel photos. The Real3D FlipBook plugin has countless more uses though. Here are a few ideas for the different types of flipbooks that you can create with the plugin.
Catalogs
The Real3D FlipBook plugin is great for catalogs. They allow you to create a realistic catalog in the digital world that can be downloaded by your customers and potential customers. Having an online catalog is a must for today's internet-driven businesses and adding your products to a catalog created by Real3D Flipbook will give you complete control over how you want to present your products.
Books or Magazines
A book or magazine can easily be created with this plugin. The average attention span of your online audience is continually going down, so creating an eye-catching and interesting book or magazine is necessary. The Real 3D FlipBook gives you complete control over the customization of your book or magazine and makes it much more visually appealing to read your material as opposed to just reading a static looking PDF.
Other Customizations
The Real3D Flipbook plugin is completely customizable. In this article, we only had a chance to go over a few of the features offered by the plugin. Below I have listed three notable functions that can help you get the most out of this plugin.
Bookmarks
If you are creating a catalog, book, magazine, or any flipbook with a large number of pages, chances are your reader won’t finish viewing your flipbook in one sitting. Enabling the bookmark feature in the Menu Buttons tab on the plugin dashboard will allow your viewers to bookmark the exact page they were on so they can come back at another point in time and continue reading.
Camera Angle
When the WebGL display of the flipbook is enabled to show off the book's 3D capabilities, you will be able to edit the angle at which the flipbook is viewed. By changing the camera angle, you can create the illusion that the reader is actually reading a flipbook that is right in front of them. The options to adjust for this are the camera pan angle and the camera tilt angle under the WebGL tab. Adjust these settings to taste to create a realistic reading perspective for your flipbook.
Search
By default, the search function on the flipbook is turned off. If your flipbook contains a lot of information, then having this search function turned on is a must so your reader can find exactly what they are looking for. Enable this function under the Menu Buttons tab.
Conclusion
When displaying any type of content to your website's audience, you need to able to attract and maintain their attention. The use of galleries and other gallery-like displays can present your images to your audience, but don't quite have the appeal of flipbooks.
By using the Real3D FlipBook plugin you can easily create a visually appealing flipbook that gives your audience a fresh new experience when visiting your website.
To give this plugin a try, head over to CodeCanyon and check out the Real3D FlipBook plugin. And while you're here, check out some of the other great WordPress plugins available from CodeCanyon.
WordPress
Best WordPress Flipbook Plugins Compared
Jane Baker
WordPress
17 Best WordPress Gallery Plugins
Jane Baker
WordPress Plugins
10 Best WordPress Gallery Plugins of 2019
Lorca Lokassa Sa
WordPress
Best Free WordPress Gallery Plugins
Monty Shokeen
by Daniel Strongin via Envato Tuts+ Code https://ift.tt/32Vpi7P
1 note
·
View note
Photo
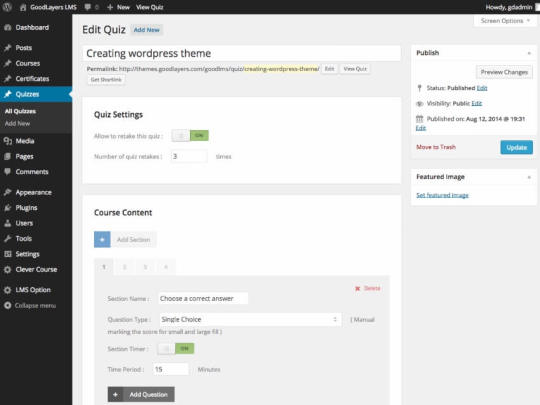
The Best Learning Management System Plugins for WordPress
Technology has completely transformed how we learn and how training is delivered. Physical presence in a physical location is no longer necessary. Technology has not done away with the instructor or the student though. Instead, it has made how and where we access and interact with learning material easy, dynamic, and flexible.
In fact, it has made instructor-to-student and student-to-student interaction more dynamic. Students learn from each other because there is greater collaboration between students.
Training is now commonly delivered and administered through learning management systems. Lessons are created and uploaded on these systems and can be accessed anytime, anyplace. Learning can happen by from desktops or mobile devices, from websites and apps.
In this article we will explore WordPress learning management systems (LMS) plugins.
Let’s get started.
Why is eLearning Popular?
The future of learning has been with us for a long time now. Learning accessible from anywhere anytime, at your own pace, using wide variety of devices from desktops to tablets to mobile phones. Learning delivered by systems that host large directories of courses. These large directories of e-courses utilize learning management systems.
As a result, there is an explosion in learning. Online learning platforms have responded to users educational needs and preferences for learning anywhere anytime by using responsive websites and apps. This makes sense since over 70% of users go online using mobile devices. And 90% of users spend their time in apps.
Many companies have also embraced online training for their staff. Some deliver their training to an externally-managed platforms that their staff can access. They don’t get involved in running the platform. Other companies have their own self-hosted learning management systems where they create, manage, and deliver training content.
The upside of this is saving on the logistical costs of planning location-based training or seminars for hundreds of employees.
Online learning platforms are very popular and profitable. If you want to get in the game so you too can host courses, or if you’re a company thinking of hosting your own training, or a developer tasked with implementing learning management infrastructures for your clients, then WordPress is your ally.
The fact that WordPress is a content management system makes it a perfect foundation on which to build learning management systems. It has a powerful and stable base, and its functionality can be extended a thousand fold through use of plugins.
But building learning management systems from scratch is extremely costly. This should not dash your hopes of managing your own self-hosted learning management system.
Here is where learning management systems (LMS) plugins for WordPress come in. There are developers who have specialized in catering specifically to this niche.
What is a Learning Management Systems Plugin?
A learning management systems plugin is a cloud-based software that delivers online learning. It allow instructors to create, manage, track, and deliver learning content or e-courses.
WordPress, which is already a comprehensive CMS, is the perfect platform for creating and hosting your Learning Management System. To offer online courses you just need to download and install an LMS plugin. This will provide functionality to several classes of user:
Administrators can manage different learners, teachers, and take care of other administrative tasks from the LMS dashboard.
Teachers can access tools for creating courses, quizzes, grading, and uploading.
Students can select what they want to learn from the directory and immerse themselves in learning.
Components of Learning Management Systems
An LMS is made up of many components enable different users to achieve their objectives.
Automated Administrative and Communication Tools
Communication is key to any successful venture. Automating communication takes a load off administrators’ shoulders. Communication to teachers and students can include notification about accounts and payments, email reminders, weekly course summaries, real-time onscreen progress indicators for each and so on.
Administrative tools include tools for registering teachers and calculating teachers commissions,
Diverse Course Options
An LMS should combine different learning methods. For example, learning content can delivered via audio, videos, powerpoint, multimedia, written text, games, social learning, and more.
Course Content Creation Tools
An LMS comes with built-in authoring tools that allow for easy creation of course content.
Engagement
Chats, forums, and other kinds of online community software tools integrated into the LMS offer opportunities for students to engage with their peers. They are not limited by location. They can collaborate on assignments and projects.
Assessment and Testing tools
Test to see how students are learning and retaining material in the courses through quizzes and exams.
Instant Feedback
Teachers can give learners feedback to show them where they need to improve.
Grading and Scoring
Allows yracking student progress and performance over the duration of the course.
Certification
An LMS should come with the ability create certificates for students that complete a course.
Reporting
Gives you access to data like number of students taking a particular course, individual reports for each student, and how much time students spend on each lesson and quiz.
Feedback
The only way to improve is through user feedback. An LMS system should offer tools for gathering feedback and suggestions from students and learning what they think about the quality of the courses and the system itself.
Reviews
An LMS system should offer students tools to review and rate their learning experience for courses. This will be helpful for others who want to take the course.
Things to Consider When Choosing a Learning Management System
Ease of Use
A user-focused LMS system should be intuitive and simple to navigate and customize.
Compatibility With Mobile Devices
80% of users go online using their mobile devices and prefer to use them for everything including learning. LMS should have companion apps in iOS and Android.
Monetization
A good LMS should offer different payment models like:
one time payment
subscriptions for regular access to courses
course bundles
coupon codes
discounts
Integration
A WordPress LMS system should integrate with software you already use like CRM software, accounting software, and more.
In addition it should be able to integrate with:
payment gateways like Paypal, Stripe, or 2Checkout
email marketing gateways like MailChimp
eCommerce plugins like WooCommerce, or Easy Digital Downloads
Multi-Language Support
If you want to offer online courses to a wider international audience your LMS plugin should offer translation support files.
Regular Updates
When bugs are left unattended they make the system vulnerable to attacks. Regular updates mean the system is reliable and secure.
Security
Users want to know that their personal and financial data is safe.
Reviews
Consider seriously what other users have to say. Users trust the word other users. They have used the system and seen how it works and what its strengths and weaknesses are.
LMS plugins available on CodeCanyon
CodeCanyon has some of the most feature-packed and lowest cost LMS plugins on the market. Here are a few of the very best.
1. Good LMS
Good LMS has many great features for creating and selling online and onsite courses.
It has an easy-to-use interface that makes creating courses a breeze. Teachers can offer quizzes, including timed quizes, and give students the option to take quizzes again.
Students can earn badges as they progress and they get a certificate at the end of each course. They will also have the ability to review and rate the course.
Payment
pay with Paypal, Stripe, Paymill or Authorized.net
admin can also provide other methods such as bank transfer
You can try this plugin for free today with the live preview.
2. Ultimate Learning Pro
Ultimate Learning Pro is one of the most comprehensive LMS plugins on CodeCanyon.
It makes it easy to create and sell courses to millions of students. Teachers can register to teach courses and you can approve them. You can have multiple teachers for one course.
It has an easy-to-navigate interface where important information needing your urgent attention is arranged in tabs.
You can offer lesson previews, set lesson durations, and set up lessons drip content so you can release lessons at regular intervals. Students can take notes, receive badges, gain points, see their grades.
You can create quizzes, give hint to questions, set quiz duration and passing grade. Also questions can be multiple choice, essay, or fill in the blanks.
In addition to accepting multiple currencies, it supports many payment options including PayPal, Stripe, 2CheckOut. It can also be integrated with platforms like WooCommerce and Easy Digital Downloads.
Checkout the live preview and see why daedubu says this about Ultimate Learning Pro:
This is an amazing plugin! Easy and powerful :) The best plugin for e-learning on my site. Thanks for all, especially to your fantastic support team!
3. WPLMS Student App
WPLMS Student app is a mobile app developed for the WPLMS Learning management system so students can learn anywhere and anytime.
To use this app you must have the WPLMS Learning Management System WP Education Theme. This is great because it allows for a consistent look between the website and the app you create. And most importantly, you can easily sync data between apps and websites.
Some best features of this app include:
Students can register directly in the app and their account is created in the site.
Students can browse courses in the app's directory and subscribe to the courses.
Students can enroll themselves in free courses or pay via a WooCommerce checkout system which supports multiple payment gateways.
Students can keep track of their courses through the app or your website.
Students courses are loaded and available in offline mode.
User dashboards with course and quiz graphs, and a quiz results section.
Quizes with multiple choice questions, multiple correct choices, fill in the blanks, dropdown selection, and text answers.
And so much more.
Check out the free live preview of this LMS and see why this is the perfect app for you.
Other Top LMS Plugins
4. LearnDash
LearnDash is one of the top learning management systems on the market. Its list of users include Fortune 500 companies, universities, training organizations, entrepreneurs, WordPress developers and designers, and more.
It has with all kinds of tools you need to build a thriving business by selling courses.
Its powerful course builder where you can create multilayered courses and even reuse the lessons. It takes into accounts different learning styles so you can engage learners by using all kinds of media from video, audio, images, Google docs. You can also break up courses into sections, lessons, topics, quizzes.
Features on the Admin side include: front-end registration, detailed reporting on student progress, creating user groups and allowing others to manage them, email notifications to learners, ability to approve, comment, and award points for assignments.
You can sell courses by offering one time price, subscriptions, memberships, and course licensing.
5. Sensei LMS
Sensei LMS is made by the same company that brought you WordPress and WooCommerce. And the basic edition is free, so you can get started using it today! However, to sell your courses you'll need to purchase a paid extension from the WooCommerce site.
This LMS is very simple to use. No coding skills are required and it works with your preferred WordPress theme.
Some of this easy-to-use LMS plugin's interesting features include:
Learner management and analysis allows you to view reports on learning progress, set up two way communication between teacher and learner, and set up notifications.
Protect your downloadable files and videos by making them available only to specific users.
Teachers have the ability to create a question bank for quizzes
Easily add shortcodes to any content areas of your page.
Ability to create multilingual courses when combined with WPML.
And more...
Sensei also has very extensive Documentation.
6. WP CourseWare
WP Courseware is a powerful plugin that allows you to create and sell online courses.
Features that come with this plugin include:
easy-to-use drag-and-drop course builder
drip content so you schedule your courses to be released at particular intervals
powerful quiz creation functionality with great options for creating quizzes
It comes with built-in shopping cart support. You can sell courses as a one time purchase or monthly membership subscription, or schedule installment payments.
It integrates smoothly with:
eCommerce plugins like EasyDigitalDownloads and WooCommerce
payment gateways like PayPal and Stripe
membership plugins like MemberPress, Paid Membership Pro and others
most WordPress themes and plugins
In addition, it has great documentation resources to get you started.
Conclusion
While these among the LMS plugins that caught my eye, there are many out there in the market today.
The following articles will help with ideas about how you can expand your online course platform.
WordPress Plugins
Choose the Best WordPress Membership Plugin
Nona Blackman
WordPress Plugins
7 Best WordPress Community Plugins for 2019
Lorca Lokassa Sa
WordPress
Monetize Your Content: Create a Membership WordPress Site With UMP
Jessica Thornsby
WordPress
Best Affiliate WooCommerce Plugins Compared
Lorca Lokassa Sa
by Lorca Lokassa Sa via Envato Tuts+ Code https://ift.tt/36glb8c
1 note
·
View note
Photo
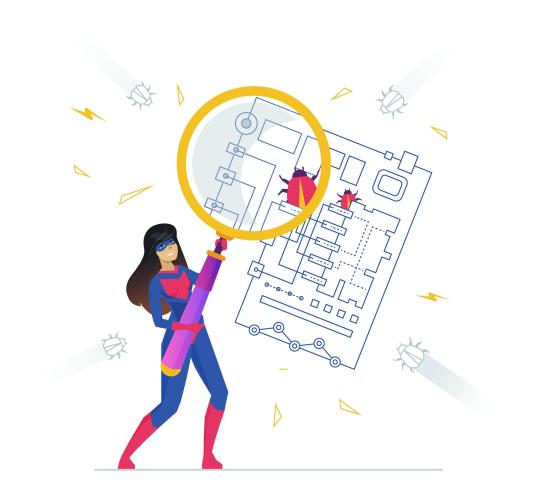
6 Tools for Debugging React Native
Debugging is an essential part of software development. It’s through debugging that we know what’s wrong and what’s right, what works and what doesn’t. Debugging provides the opportunity to assess our code and fix problems before they’re pushed to production.
In the React Native world, debugging may be done in different ways and with different tools, since React Native is composed of different environments (iOS and Android), which means there’s an assortment of problems and a variety of tools needed for debugging.
Thanks to the large number of contributors to the React Native ecosystem, many debugging tools are available. In this brief guide, we’ll explore the most commonly used of them, starting with the Developer Menu.
Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it. — Brian W. Kernighan
The Developer Menu
The in-app developer menu is your first gate for debugging React Native, it has many options which we can use to do different things. Let’s break down each option.
Reload: reloads the app
Debug JS Remotely: opens a channel to a JavaScript debugger
Enable Live Reload: makes the app reload automatically on clicking Save
Enable Hot Reloading: watches for changes accrued in a changed file
Toggle Inspector: toggles an inspector interface, which allows us to inspect any UI element on the screen and its properties, and presents and interface that has other tabs like networking, which shows us the HTTP calls, and a tab for performance.
The post 6 Tools for Debugging React Native appeared first on SitePoint.
by Said Hayani via SitePoint https://ift.tt/32UuicR
1 note
·
View note
Photo
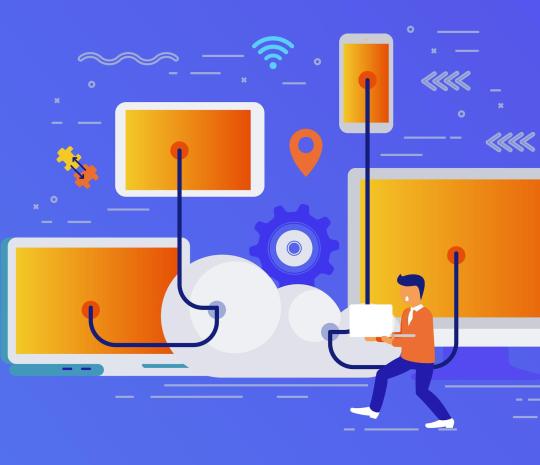
Why Your Agency Should Offer Managed Cloud Hosting to Clients
This article was created in partnership with Cloudways. Thank you for supporting the partners who make SitePoint possible.
When it comes to end-to-end services, digital agencies offer an impressive range. From requirement analysis to post-deployment maintenance, these agencies do everything to make sure that their clients are able to fully leverage their projects for maximum business efficiency.
In this backdrop, many agencies (particularly those that deal with web-based projects) also offer hosting as part of their services to their customers. While small and up-and-coming digital agencies might not have hosting on their service brochure, mid-tier and top-shelf agencies see hosting as an integral service offering to their clients.
Setting up Hosting for Customers
For a web-based project, web hosting is an essential component that determines the success (and failure) of the project. Since the agency has developed the project, many clients trust the agency-managed hosting for their project.
High-performance applications (online stores and CRM in particular) demand a hosting solution that’s able to keep pace with the high request volume and a large number of concurrent connections. Clients with these projects can’t compromise on the post-deployment performance of the applications. As such, agencies prefer an in-house hosting setup that caters to the specific requirements of the projects.
Agencies Benefit From In-house Hosting
Before going into what benefits agencies get from an in-house hosting setup, it’s important to understand the major requirements of high-performance projects. Without going too much into the details, in-house hosting solutions are set up to make sure that custom-built projects continue to perform on the following parameters:
the number of visitors per hour/day/month
the number of simultaneous visitors
the maximum number of connections allowed
the number of simultaneous requests/orders
the size and complexity of the products catalog (number of products, product categories, attributes)
the content requirements and traffic on content assets such as blogs
the volume of search queries on the site
the size and connections on the database
With in-house hosting solutions, agencies (and their clients) get a whole range of benefits such as those outlined below.
Custom Hardware and Software
Hardware requirements for custom, high-performance projects generally include three components: CPU, RAM and Disk Space. Since each project has custom requirements that are often not available in off-the-shelf hosting solutions available in the market, agencies opt for setting up in-house hardware platforms for their customers.
Custom hardware setups usually cost more than the conventional, commercially available hosting hardware architecture. The cost of setting up and maintaining the hosting architecture is usually the responsibility of the dev agency, which usually bills the client for these services.
Another related (and in my opinion, more important) requirement of these projects is a custom environment that comprises an OS layer and a facilitation layer made of servers and caches. A custom environment allows agencies to build their projects without worrying about conflicts with the OS and server software required to execute the codebase. Thanks to in-house hosting, digital agencies can completely customize the OS and server layer to the project specifications.
End-to-End Management of Project Hosting
Project requirements change and clients often revise their requirements and scope. These changes also impact the hosting requirements and specifications. Since the hosting process is being managed in-house, the agency can take proactive actions to improve hosting setup specifications and ensure continued performance for the application.
Passive Income Stream
In almost all cases, agency-managed hosting solutions are built and maintained on the client’s dollars. The agency proposes hosting setup specifications and sets it up once the client pays for it. Once the setup is active, the client pays for the maintenance and upkeep of the hosting solution. This is a passive income channel that is often an important supplement to agency revenues.
Challenges In-agency Managed Hosting
Despite the benefits, managing an in-house hosting setup can prove to be a drag on the agency operations. In particular, agency-managed hosting causes the following challenges for the business processes.
Hosting Architecture Requires Continuous Attention
Since this is an in-house managed hosting solution, it’s obvious that the agency is responsible for keeping both the hardware and software layers operational. While the hardware layer (the physical server machines and the networking equipment) have a lower ratio of failure, it’s important to note that the software components of the hosting solution require detailed attention and upkeep.
Both hardware and software vendors regularly release patches that fix bugs and enhance product functionality. In many cases, these patches are mission-critical and essential for the continued performance of the project’s hosting. In in-house managed hosting, this is the responsibility of a dedicated team that performs no other function.
The Constant Need for Security
Web servers are the prime target of cybercriminals because of the wealth of information and user data on them. The problem with server security is that it's a full-time function that requires specialists on the team. The same goes for clients’ applications (CMSs such as WordPress are especially vulnerable) that could potentially open up security loopholes in the server and application security. Not many agencies can afford a dedicated infosec expert on the payroll. Thus, there's always the danger that clients’ applications can get hacked because the agency-managed hosting is unable to maintain the required security standards.
Sysadmins Prove to be an Overhead
Sysadmins are among the highest-paid professions in the ICT industry, and rightly so! They manage entire data centers and handle all aspects of hosting servers from provisioning to maintenance. The problem with sysadmins is the high recruitment and operational costs of these professionals. Thus, hiring a sysadmin to manage in-house hosting is a serious decision that's out of the budget of many dev agencies.
Deviation from the Core Business
Digital agencies are in the business of building applications and custom projects that create value for their clients. An in-house hosting solution requires competence that lies outside the normal scope of the dev agencies. In addition, managing hosting solutions require expenses that eat away into profits without generating enough revenue to justify their inclusion in business offerings.
Shared Hosting is a False Start
The good news is that many agencies are aware of the issues with in-house, agency-managed hosting and have come to realize that this is not the ideal solution for managing clients’ hosting focused expectations.
However, since the clients’ requirements continue to grow and the need for hosting solutions for custom-developed apps is on the rise, a number of agencies have turned to shared hosting as an alternative to agency managed in-house hosting solutions.
When opting for shared hosting solutions, agencies try to reduce the cost of hosting solutions while providing a comparable hosting solution to the clients.
Before going into the description of why shared hosting solutions are in fact counterproductive for dev agencies, it's important to understand how shared hosting solutions work.
Shared Hosting in a Nutshell
As the name implies, shared hosting is a solution where several websites/applications are hosted on a single physical server. This means that the physical resources (CPU, RAM, Disk space and bandwidth (in some cases) get shared among the websites hosted on the server.
While this is not a bad solution per se, it's not the right ft for high-performance applications. These applications have a minimum server resource requirements that often exceed the allocated “quota” allocated by the shared hosting server.
Many digital agencies try to integrate shared hosting solutions in their customer-focused services by eliminating sysadmins from the equation and asking the developers to manage the hosting servers for the clients.
The post Why Your Agency Should Offer Managed Cloud Hosting to Clients appeared first on SitePoint.
by SitePoint Sponsors via SitePoint https://ift.tt/2BOyKOj
1 note
·
View note
Photo
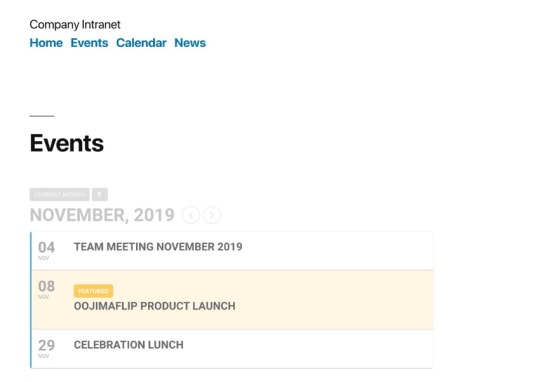
Add a Calendar to Your Company Intranet With a WordPress Plugin
What You'll Be Creating
WordPress is a great system for building a company intranet.
You may already be using WordPress for your public website, but it also handles intranets really well. From sharing news, to social interaction and collaboration, WordPress lets you add functionality to your company intranet that helps you communicate more effectively as a team and share resources.
One resource you might want to share via your intranet is a calendar. You can use this not only to book meetings, but also to provide details of events that will affect the whole team and discuss an event in advance.
Guide to WordPress Event Calendar and Booking Plugins
WordPress calendar plugins let users make bookings, schedule events, pay for appointments, and more. If your website does any of these things, you need a...
Lorca Lokassa Sa
21 Sep 2019
WordPress
How to Use the WordPress Event Calendar Plugin
For certain kinds of business, having a calendar on your WordPress website is an absolute necessity. Whether you need to share information about opening and...
Daniel Strongin
15 Jul 2019
WordPress Plugins
Choose the Best WordPress Event Calendar Plugins
Looking for the best WordPress event calendar plugins? This list has all the top event calendar plugins you need to consider.
Nona Blackman
28 Feb 2019
WordPress Plugins
How to Add a Calendar to Your WordPress Site With the Events Schedule Plugin
Build a schedule for your website, complete with Google Maps integration, call to action buttons, and a complete automated booking and ticketing system for...
Jessica Thornsby
14 Mar 2019
WordPress
In this tutorial, I'll show you how you can use the EventON plugin to add a calendar to your company intranet. We'll create a calendar system that is more than just a link to a Google Calendar (although it can link to that): it'll be a hub for sharing company events and planning for them too.
Why Add a Calendar Plugin to Your Company Intranet?
A company intranet is a great place to share information and resources between team members. It's where they can find out about company news, look up information about products and services, check data about products and orders, and more.
What you add to your intranet will depend on your business and the needs of your team. But one invaluable resource in any intranet will always be an events calendar.
You could go down the simple route and simply embed a Google Calendar in one of the pages of your intranet. But using a dedicated events plugin like EventON lets you add much more detail to each event.
You can include images, file uploads, details of the organizer and the venue, and more. You can set some events as featured so they're clearly more important, and you can enable comments so that team members can discuss details of the event right inside your intranet.
The EventON plugin also lets team members link events to their own calendar on Google or elsewhere, so they can save details of events for themselves.
Let's take a look at how it works.
Installing and Configuring the EventON PLugin
Firstly, you'll need to purchase and download the EventON plugin from CodeCanyon.
Once you've done that, you'l need to configure your event settings. Go to myEventON > Settings.
There are a number of configurable settings. Some of the most useful are below:
General Calendar Settings: Enable or disable specific settings, including advanced settings. If you leave all this at the default settings, you won't go far wrong.
Google Maps API: add a Google Maps API key to display Google Maps on event pages. This will help team members find events which are out of the office.
Time Settings: Configure the way time and date are displayed in the calendar.
Appearance: Configure the colors and styles used in your calendar.
EventCard: configure the way individual events are displayed.
Creating an Event
The next step is to create your first event.
Go to Events > Add Event to see the event editing page.
The editing screen includes a main editing pane where you can add information about the event, plus sections for date, time, location and more. You can also add event organizers.
Event organizers and locations are saved separately so that you can reuse them for future events.
Work through the screen, providing all the information about your event until it contains everything you need.
On the right hand side of the screen there are metaboxes where you can provide more information about your event. These include:
event categories
event color
event image
Repeating and Duplicate Events
As well as creating events, you can also duplicate them to create new ones. This is useful if you have a second event which is very similar to an earlier event, such as team meetings.
If the event will be exactly the same every time it runs, you don't need to duplicate it: instead you can set it as a repeating event.
You can find this below the event time and date settings and above the location settings. Set the event to repeat weekly, monthly or at another interval and multiple events will automatically be created for you.
Displaying Events
Each event will have its own event page and will be added to the main Events page which the plugin will automatically create when you activate it. Make sure you add this to your navigation menu.
This page will display a list of upcoming events by month.
Click on any event and you'll be able to see full details. What is displayed will depend on the way you configured the event when setting it up.
You can change the settings for your events so that when they are clicked on, an event page is opened instead. This is useful if an event has a lot of information.
To do this, go to the event editing page and scroll down to the User Interaction for event click section.
The option on the right will open a new page if a user clicks on the event.
Then when a user clicks on the event in the main Events page, they will be taken to a full page for that event with more information.
Adding Extra Features
The EventON plugin is designed to be a simple, minimal plugin that will display information about events with little effort or fuss.
But if you need extra functionality, you can install addons for the plugin. These include:
RSVPs: invite team members to events and they can RSVP.
RSS feed: make a list of event available to RSS readers.
Events map: put all the events in a month on one Google map.
Full cal: display events in a grid style calendar instead of a list.
Weekly view: display events in a weekly view.
These are some of the add-ons that might be useful for a company intranet: it isn't an exhaustive list.
Summary
A company intranet is a great resource for sharing information between company staff and team members. By adding an events calendar, you can plan events and meetings, share resources relating to them, and discuss the agenda ahead of time.
The EventOn plugin will help you add a clean events calendar to your intranet with multiple options for customization and addons if you need them.
WordPress
Guide to WordPress Event Calendar and Booking Plugins
Lorca Lokassa Sa
WordPress Plugins
How to Use the WordPress Event Calendar Plugin
Daniel Strongin
WordPress Plugins
Choose the Best WordPress Event Calendar Plugins
Nona Blackman
WordPress
How to Add a Calendar to Your WordPress Site With the Events Schedule Plugin
Jessica Thornsby
by Rachel McCollin via Envato Tuts+ Code https://ift.tt/3487YMO
1 note
·
View note
Photo
7 CSS Units You Might Not Know About
Learn CSS: The Complete Guide
We've built a complete guide to help you learn CSS, whether you're just getting started with the basics or you want to explore more advanced CSS.
New CSS Techniques
It’s easy to get stuck working with the CSS techniques we know well, but doing so puts us at a disadvantage when new problems surface.
As the web continues to grow, the demand for new solutions will also continue to grow. Therefore, as web designers and front end developers, we have no choice but to know our toolset, and know it well.
That means knowing even the specialty tools - the ones that aren’t used as often, but when they are needed, are exactly the right tool for the job.
Today, I'm going to introduce you to some CSS tools you might not have known about before. These tools are each units of measurement, like pixels or ems, but it’s quite possible that you’ve never heard of them! Let’s dive in.
rem
We’ll start with something that’s similar to something you are probably already familiar with. The em unit is defined as the current font-size. So, for instance, if you set a font size on the body element, the em value of any child element within the body will be equal to that font size.
<body> <div class="test">Test</div> </body>
body { font-size: 14px; } div { font-size: 1.2em; // calculated at 14px * 1.2, or 16.8px }
Here, we’ve said that the div will have a font-size of 1.2em. That’s 1.2 times whatever the font-size it has inherited, in this case 14px. The result is 16.8px.
However, what happens when you cascade em-defined font sizes inside each other? In the following snippet we apply exactly the same CSS as above. Each div inherits its font-size from its parent, giving us gradually increasing font-sizes.
<body> <div> Test <!-- 14 * 1.2 = 16.8px --> <div> Test <!-- 16.8 * 1.2 = 20.16px --> <div> Test <!-- 20.16 * 1.2 = 24.192px --> </div> </div> </div> </body>
While this may be desired in some cases, often you might want to simply rely on a single metric to scale against. In this case, you should use rem. The “r” in rem stands for “root”; this is equal to the font-size set at the root element; in most cases that being the html element.
html { font-size: 14px; } div { font-size: 1.2rem; }
In all three of the nested divs in the previous example, the font would evaluate to 16.8px.
Good for Grids
Rems aren’t only useful for font sizing. For example, you could base an entire grid system or UI style library on the root HTML font-size using rem, and utilize scaling of em in specific places. This would give you more predictable font sizing and scaling.
.container { width: 70rem; // 70 * 14px = 980px }
Conceptually, the idea behind a strategy like this is to allow your interface to scale with the size of your content. However, it may not necessarily make the most sense for every case.
Can I use it?
Feature: rem (root em) units on caniuse.com
vh and vw
Responsive web design techniques rely heavily on percentage rules. However, CSS percentage isn’t always the best solution for every problem. CSS width is relative to the nearest containing parent element. What if you wanted to use the width or height of the viewport instead of the width of the parent element? That’s exactly what the vh and vw units provide.
The vh element is equal to 1/100 of the height of the viewport. For example, if the browser’s height is 900px, 1vh would evaluate to 9px. Similarly, if the viewport width is 750px, 1vw would evaluate to 7.5px.
There are seemingly endless uses for these rules. For example, a very simple way of doing full-height or near full-height slides can be achieved with a single line of CSS:
.slide { height: 100vh; }
Imagine you wanted a headline that was set to fill the width of the screen. To accomplish this, you would set a font-size in vw. That size will scale with the browser’s width.
Can I use it?
Feature: Viewport units: vw, vh on caniuse.com
vmin and vmax
While vh and vm are always related to the viewport height and width, respectively, vmin and vmax are related to the maximum or minimum of those widths and heights, depending on which is smaller and larger. For example, if the browser was set to 1100px wide and the 700px tall, 1vmin would be 7px and 1vmax would be 11px. However, if the width was set to 800px and the height set to 1080px, vmin would be equal to 8px while vmax would be set to 10.8px.
So, when might you use these values?
Imagine you need an element that is always visible on screen. Using a height and width set to a vmin value below 100 would enable this. For example, a square element that always touches at least two sides of the screen might be defined like this:
.box { height: 100vmin; width: 100vmin; }
If you needed a square box that always covers the visible viewport (touching all four sides of the screen at all times), use the same rules except with vmax.
.box { height: 100vmax; width: 100vmax; }
Combinations of these rules provide a very flexible way of utilizing the size of your viewport in new and exciting ways.
Can I use it?
Feature: Viewport units: vmin, vmax on caniuse.com
ex and ch
The units ex and ch, similar to em and rem, rely on the current font and font size. However, unlike em and rem, these units also rely on the font-family, as they are determined based on font-specific measures.
The ch unit, or the character unit is defined as being the “advanced measure” of the width of the zero character, 0. Some very interesting discussion about what this means can be found on Eric Meyers's blog, but the basic concept is that, given a monospace font, a box with a width of N character units, such as width: 40ch;, can always contain a string with 40 characters in that particular font. While conventional uses of this particular rule relate to laying out braille, the possibilities for creativity here certainly extend beyond these simple applications.
The ex unit is defined as the “x-height of the current font OR one-half of one em”. Thex-height of a given font is the height of the lower-case x of that font. Often times, this is about at the middle mark of the font.
x-height; the height of the lower case x (read more about The Anatomy of Web Typography)
There are many uses for this kind of unit, most of them being for typographic micro-adjustments. For example, the sup element, which stands for superscript, can be pushed up in the line using position relative and a bottom value of 1ex. Similarly, you can pull a subscript element down. The browser defaults for these utilize superscript- and subscript-specific vertical-align rules, but if you wanted more granular control, you could handle the type more explicitly like this:
sup { position: relative; bottom: 1ex; } sub { position: relative; bottom: -1ex; }
Can I Use it?
The ex unit has been around since CSS1, though you won’t find such solid support for the ch unit. For specifics on support, check out CSS units and values on quirksmode.org.
Conclusion
Keeping an eye on the continued development and expansion of CSS is incredibly important so that you know all of the tools in your toolset. Perhaps you will encounter a particular problem that requires an unexpected solution utilizing one of these more obscure measurement units. Take time to read over new specifications. Sign up for news updates from great resources like cssweekly. And don’t forget, sign up now for weekly updates, courses, free tutorials and resources like this one from Web Design on Tuts+!
Further Reading
More CSS unit goodness.
Taking the “Erm..” Out of Ems
Taking Ems Even Further
Caniuse Viewport units
by Jonathan Cutrell via Envato Tuts+ Code https://ift.tt/2JnKfjV
1 note
·
View note
Photo
How to Create File Upload Forms on Your WordPress Site
Forms are an easy way to collect information from website visitors, and file uploads allow users to add even more useful or important information. Some of the data which you can collect from file upload forms include:
user-submitted images and videos
content in the form of blog posts
resume files
In this post, I'll show you how to create a resume upload form for a WordPress website. Users will be able to upload resume files in PDF format. By the end of this tutorial, we should have something like this.
I'll also show you how to add these uploaded files to Dropbox.
20 Best WordPress Login Forms on CodeCanyon
Sometimes you need to modify your WordPress login form to make it more user-friendly or maybe add some features. No matter your reason, here are 20 best...
Eric Dye
09 Apr 2018
WordPress
Best WordPress Form Builder Plugins for 2019
If you own a WordPress site, forms are indispensable for creating a satisfying user experience and increasing your conversion rates. You need forms for...
Lorca Lokassa Sa
01 Apr 2019
WordPress Plugins
Create a Drag-and-Drop Contact Form With the FormCraft 3 WordPress Plugin
Whether you are running an online store, marketplace, or a blog on your WordPress website, you'll need a contact form. In this article, I am going to show...
Daniel Strongin
28 Aug 2019
WordPress Plugins
Creating WordPress Forms That Get Filled In
The forms on your site are useless unless people actually complete them. Find out how to create great forms that encourage people to click submit.
Rachel McCollin
26 Jun 2019
WordPress
Drag and Drop File Uploader Add-on for Contact Form 7
The Drop Uploader add-on for Contact Form 7 is a powerful plugin that allows you to add an upload area of any format to a form. You can also add several uploading areas to one form. It also allows you to copy these uploaded files to your preferred server or to Dropbox, which provides another backup for your data.
Other features include:
Javascript (front-end) file validation
ability to restrict specific file extensions
unlimited file upload ensures you can upload files of any size
ability to drag and drop or browse during upload
styling customization including colors, browse buttons and icons
receive uploaded files as links, mail attachments or both
receive attachments as zip files
store files in Dropbox
delete old files at a specific time
The plugin is translation ready and supports English, Spanish, French, Italian, German, Russian and Ukraine
Create Your Resume Upload Form
To get started creating an upload form, first purchase and download the Drop Uploader for CF7 plugin. You can find your installable WordPress files in the download section of your account.
Once you download the WordPress files, log in to your WordPress site, and install the plugin. Go to Plugins > Add New and upload the WordPress zip file you got from CodeCanyon. After uploading, click Install Now, wait a few seconds, and then click Activate. You can now start using the plugin.
Configurations
Go to Settings > CF7 Drop Uploader Settings and customize the Drop Uploader Style and other options such as layout and file storage.
File Storage
CF7 Drop Uploader offers three ways of storing files:
Attachment: if you enable this option, all files will be archived in to zip files.
Link: this option allows you to store uploaded files as links. It also allows you to delete the files at a specified time.
Dropbox: this option allows you to integrate and add your files to Dropbox. All you need is the Dropbox token, which you can obtain from your Dropbox account. You can also generate shareable links and link them to files or folder.
Create Your First File Upload Form
Install Contact Form 7 from the official WordPress plugins directory. Once done, you can now start creating your forms. Click Contact > Add New in your WordPress Dashboard menu. Contact Form 7 comes pre-configured with a ready to use template as shown below
Click on Drop Uploader, and you should see a popup like the one below.
Mark the field type as a required field, set the Files count limit, and input Accepted file types as PDF format. Select the HTML Link checkbox if you wish to send links in HTML. Once you are done, click on Insert Tag, and all the changes are applied to the form. Rearrange the fields as you would want them to appear in your form. You can also add a message by clicking on the Drop Uploader Message tab.
The form template also contains additional fields such as checkboxes, date, and radio buttons, which you can use to make any form.
Next, go to the Mail tab and add the uploader shortcode—in my case [dropuploader-313]—to the message body and save the changes.
You can also receive the uploaded files as mail attachments by pasting the shortcode id of the uploader to the File Attachments section.
Embed Your Resume Upload Form in a Page
The final step is to embed the upload form to a WordPress page. To add the upload form, click the Add shortcode option and paste the shortcode of the contact form.
Receive Uploaded Files in Dropbox
In this section, we’ll cover how you to integrate Dropbox with your contact forms and send copies to Dropbox.
The first thing is to head to Dropbox developers and log in to your Dropbox account. Click on Create apps, select the Dropbox API option, choose the type of access you need for the API, and create a name for your app. Finally, click the Create app button. You will be redirected to the page which contains all the app’s information. Scroll to the OAuth 2 section and click on the Generate token button.
Once the token has been generated, copy and paste it to the Dropbox token section on your WordPress site.
To ensure your files will be stored in Dropbox, edit the form by enabling receiving files option. Go to the Drop Uploader tab and activate the Dropbox setting.
Save your form settings. In addition to receiving files as links in the message body, you will also receive files via Dropbox. To confirm if your file submissions have been saved to your Dropbox account, simply login to your Dropbox account and check under Apps.
Conclusion
This post has covered everything you need to get started on creating upload forms and storing your information. CF7 Drop Uploader will cater to every need, whether its for big or small files. If you are looking for a way to quickly create upload forms that automatically sends your file uploads to your Dropbox, this is an easy way to manage files and ensure safekeeping for your files. Take advantage of this awesome plugin and easily create file uploads.
WordPress
20 Best WordPress Login Forms on CodeCanyon
Eric Dye
WordPress Plugins
Best WordPress Form Builder Plugins for 2019
Lorca Lokassa Sa
WordPress
Creating WordPress Forms That Get Filled In
Rachel McCollin
WordPress
Use a Drag-and-Drop Form Builder for WordPress
Ashraff Hathibelagal
WordPress
How to Pick a WordPress Form Builder Plugin
Lorca Lokassa Sa
by Esther Vaati via Envato Tuts+ Code https://ift.tt/2WgZ5hl
1 note
·
View note