#willaireplacewebdevelopers
Explore tagged Tumblr posts
Text
§Python - Scalability and Performance Considerations
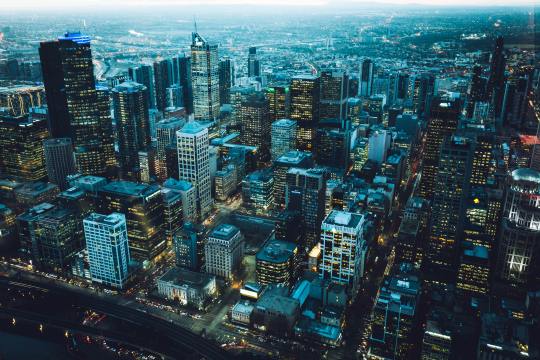
1. Scaling Strategies:
- Definition: Scalability is the ability of a system to handle increased loads. Strategies include vertical scaling (adding more resources to a single server) and horizontal scaling (adding more servers).
- Practical Example: Setting up a load balancer to distribute incoming traffic to multiple servers for horizontal scaling.
```nginx
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
```
2. Caching Techniques:
- Definition: Caching involves storing frequently accessed data to reduce the need to retrieve it from the original source, improving response times.
- Practical Example: Implementing caching in a Python web application using Flask-Caching.
```python
from flask import Flask
from flask_caching import Cache
app = Flask(__name)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/')
@cache.cached(timeout=60)
def cached_route():
return 'This is a cached response.'
if __name__ == '__main__':
app.run()
```
3. Load Balancing and Clustering:
- Definition: Load balancers distribute incoming requests among multiple servers, while clustering involves a group of servers working together as a single system.
- Practical Example: Implementing load balancing and clustering for a Python application using Nginx and Gunicorn.
```nginx
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
```
4. Profiling and Monitoring:
- Definition: Profiling and monitoring tools are essential for identifying performance bottlenecks and monitoring system health.
- Practical Example: Using Python's `cProfile` module for performance profiling in a web application.
```python
import cProfile
def slow_function():
# Simulate a slow function
for _ in range(1000000):
_ = 1 + 1
if __name__ == '__main__':
profiler = cProfile.Profile()
profiler.enable()
slow_function()
profiler.disable()
profiler.print_stats(sort='cumtime')
```
5. Scaling for High Traffic:
- Definition: Preparing your application to handle high levels of traffic efficiently. This may include optimizing database queries, using content delivery networks (CDNs), and reducing unnecessary load.
- Practical Example: Using a CDN like Amazon CloudFront to serve static content for a web application.
These considerations and practical examples are crucial for ensuring your back-end can efficiently handle increased loads, providing a responsive and reliable user experience as your application scales.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Note
bro i want to work under u
humbled to grant you the knowledge I have acquired but my schedule is pretty busy you can go through my book and courses till then...
Thankyou
God bless you.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Web Servers, Databases, and Server-Side Frameworks
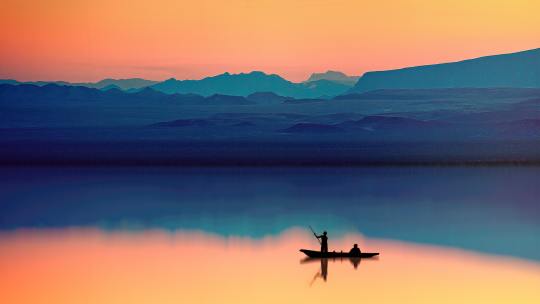
1. Web Servers:
- Definition: Web servers are responsible for handling incoming HTTP requests and serving responses to clients. Popular web servers include Apache, Nginx, and Microsoft Internet Information Services (IIS).
- Practical Example: Using Nginx to serve static files for a Python web application.
```nginx
server {
listen 80;
server_name example.com;
location /static/ {
alias /path/to/static/files;
}
location / {
proxy_pass http://127.0.0.1:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
```
2. Databases:
- Definition: Databases are crucial for storing and managing structured data. There are two main categories: relational databases (e.g., MySQL, PostgreSQL) and NoSQL databases (e.g., MongoDB, Redis).
- Practical Example: Using MySQL as a relational database for a Python web application.
```python
import mysql.connector
connection = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="mydatabase"
)
```
3. Server-Side Frameworks:
- Definition: Server-side frameworks provide tools and libraries for building web applications efficiently. Python offers several popular frameworks, including Django, Flask, and Pyramid.
- Practical Example: Creating a simple web application using the Flask framework.
```python
from flask import Flask
app = Flask(__name)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
```
4. Web Application Deployment:
- Definition: Deploying a web application involves making it available to users. Tools like Docker and cloud platforms (e.g., AWS, Azure) are essential for deployment.
- Practical Example: Deploying a Python web application using Docker.
```Dockerfile
FROM python:3.8
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
```
5. Load Balancing:
- Definition: Load balancing ensures that incoming traffic is evenly distributed among multiple servers, enhancing performance and fault tolerance.
- Practical Example: Setting up load balancing for a Python web application using Nginx.
```nginx
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
```
These components collectively form the core of the back-end technology stack, and selecting the right combination of web servers, databases, frameworks, and deployment strategies depends on the specific requirements of your project. The practical examples provided demonstrate how these components work together to create a functional web application.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
§ Components of a Typical Back-End Stack
The components of a typical back-end stack in-depth, with practical examples as part of the Back-End Technology Stack.
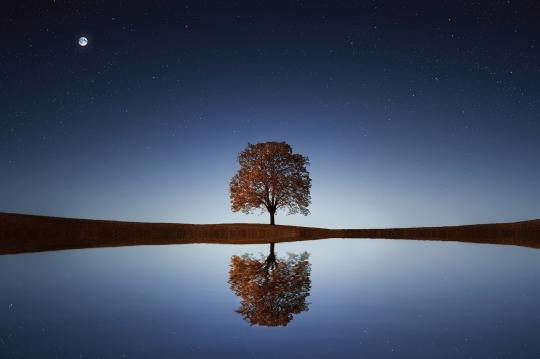
A typical back-end technology stack comprises several key components that work together to handle the server-side functionality of a web application. Let's explore each component with practical examples:
1. Web Server:
- Definition: The web server handles incoming HTTP requests and serves responses.
- Practical Example: In Python, you can use Flask to create a simple web server.
```python
from flask import Flask
app = Flask(__name)
@app.route('/')
def hello():
return "Hello, World!"
if __name__ == '__main__':
app.run()
```
2. Application Server:
- Definition: The application server executes application code, manages application logic, and communicates with the database.
- Practical Example: For a Python application, uWSGI or Gunicorn can serve as application servers.
3. Database:
- Definition: Databases store and manage data. Common options include relational databases like MySQL and PostgreSQL or NoSQL databases like MongoDB.
- Practical Example: Using Python to connect to a PostgreSQL database.
```python
import psycopg2
conn = psycopg2.connect(
database="mydb",
user="myuser",
password="mypassword",
host="localhost",
port="5432"
)
```
4. Programming Language:
- Definition: The choice of programming language can vary, but common languages include Python, Java, Ruby, and PHP.
- Practical Example: Backend code in Python can be written using web frameworks like Django or Flask.
5. Framework:
- Definition: Frameworks provide tools and libraries for building web applications efficiently.
- Practical Example: Using Django, a Python web framework, to create a simple view.
```python
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, World!")
```
6. Cache:
- Definition: Caching systems like Redis or Memcached improve performance by storing frequently accessed data in memory.
- Practical Example: Using Redis to cache query results in a Python application.
7. Message Queue:
- Definition: Message queues like RabbitMQ or Apache Kafka are used for handling asynchronous tasks and decoupling components.
- Practical Example: Using Celery with RabbitMQ to process background tasks in a Python application.
8. Security:
- Definition: Security components include tools for user authentication, authorization, and data encryption.
- Practical Example: Implementing user authentication in a Python application using a library like Django's authentication system.
9. Middleware:
- Definition: Middleware components handle tasks like logging, request processing, and security.
- Practical Example: Creating custom middleware in a Python web application to log requests.
10. APIs:
- Definition: APIs facilitate communication with external services and applications.
- Practical Example: Creating a RESTful API in a Python application using a library like Flask-RESTful.
11. Containerization:
- Definition: Containerization tools like Docker are used to package and deploy applications and their dependencies.
- Practical Example: Dockerizing a Python web application for easier deployment and scalability.
12. Load Balancer:
- Definition: Load balancers distribute incoming traffic across multiple servers to ensure high availability and load distribution.
- Practical Example: Configuring an Nginx load balancer for a Python application.
These components collectively form the backbone of a robust back-end technology stack, and their selection depends on the specific requirements of your web application. Each component plays a crucial role in ensuring the performance, security, and functionality of your back-end system.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Collaboration Between Back-End and Front-End...
Let's continue with the code examples from the previous explanation. To run the front-end and back-end code locally, you'll need to follow these steps:
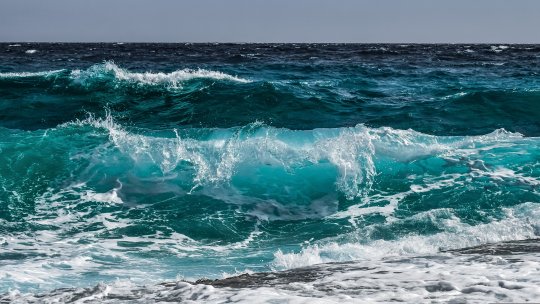
Front-End (HTML, CSS, JavaScript):
1. Create an HTML file (e.g., `index.html`) and add the following code to it:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>User Registration</title>
</head>
<body>
<h1>User Registration</h1>
<form id="registrationForm">
<label for="firstName">First Name:</label>
<input type="text" id="firstName" name="firstName" required><br>
<label for="lastName">Last Name:</label>
<input type="text" id="lastName" name="lastName" required><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required><br>
<button type="submit">Register</button>
</form>
<script>
document.getElementById("registrationForm").addEventListener("submit", function (event) {
event.preventDefault();
const formData = new FormData(event.target);
const userData = {};
formData.forEach((value, key) => {
userData[key] = value;
});
// Send the user data to the back end for processing
fetch("/api/register", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
})
.then(response => response.json())
.then(data => {
alert(data.message);
})
.catch(error => {
console.error("Error:", error);
});
});
</script>
</body>
</html>
```
Back-End (Python with Flask):
2. Create a Python file (e.g., `app.py`) and add the following code to it:
```python
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route("/api/register", methods=["POST"])
def register_user():
user_data = request.get_json()
# Perform user registration logic (e.g., validate data, save to a database)
# For simplicity, let's just return a success message
response_data = {"message": "User registered successfully"}
return jsonify(response_data)
if __name__ == "__main__":
app.run(debug=True)
```
3. Install Flask if you haven't already by running `pip install Flask` in your terminal.
4. Run the Flask app by executing `python app.py` in your terminal.
Now, when you open `index.html` in your web browser and submit the registration form, it will send a POST request to the local Flask server running on your machine, simulating a front-end and back-end interaction. The back end will respond with a success message.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
I'll provide you with a simple runnable example for both front-end and back-end development.
Front-End Development (HTML, CSS, JavaScript):
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Front-End Example</title>
<style>
/* CSS for styling */
body {
font-family: Arial, sans-serif;
}
button {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Welcome to Our Website</h1>
<p>This is a sample front-end page.</p>
<button id="clickMeButton">Click Me</button>
<script>
// JavaScript code for front-end interactivity
document.getElementById("clickMeButton").addEventListener("click", function () {
alert("Button clicked!");
});
</script>
</body>
</html>
```
2. Save this file as `index.html` and open it in a web browser. You'll see a webpage with a button. When you click the button, an alert will pop up.
Back-End Development (Node.js):
1. Install Node.js if you haven't already (https://nodejs.org/).
2. Create a JavaScript file (server.js):
```javascript
const http = require("http");
const server = http.createServer((req, res) => {
res.writeHead(200, { "Content-Type": "text/plain" });
res.end("Hello, Back-End!\n");
});
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
```
3. Open your terminal/command prompt, navigate to the directory containing `server.js`, and run the following command to start the server:
```
node server.js
```
4. The server will start on port 3000 (you can change the port if needed). Access it by opening a web browser and entering `http://localhost:3000` in the address bar. You should see "Hello, Back-End!" displayed in the browser.
These examples demonstrate a simple front-end webpage with a button and a Node.js back-end server that responds to requests. You can use these as starting points for more complex front-end and back-end development projects.
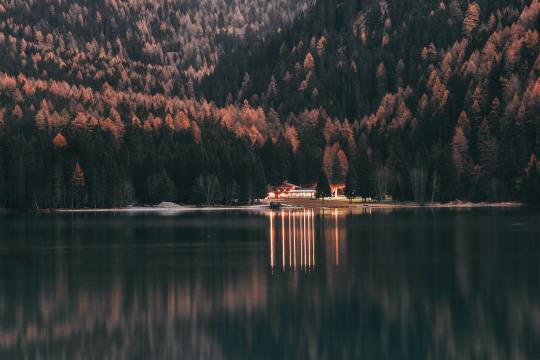
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Here are some popular combinations of back-end and front-end technologies:
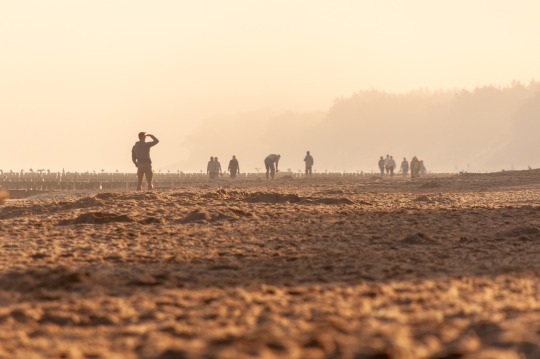
Front-End: HTML/CSS/JavaScript
Back-End: Node.js/Express.js: A JavaScript runtime environment that allows you to build server-side applications using JavaScript.
Front-End: HTML/CSS/JavaScript
Back-End: Ruby on Rails: A Ruby-based web framework known for its simplicity and convention over configuration.
Front-End: HTML/CSS/JavaScript
Back-End: Python/Django: A Python web framework that promotes rapid development and clean, pragmatic design.
Front-End: HTML/CSS/JavaScript
Back-End: PHP/Laravel: PHP is a popular server-side scripting language, and Laravel is a PHP web application framework.
Front-End: HTML/CSS/JavaScript
Back-End: Java/Spring: A Java-based framework known for its robustness and scalability.
Front-End: HTML/CSS/JavaScript
Back-End: ASP.NET (C#): A framework developed by Microsoft for building web applications using C#.
Front-End: HTML/CSS/JavaScript
Back-End: Flask (Python): A lightweight Python web framework that is often used for smaller web applications and APIs.
Front-End: HTML/CSS/JavaScript
Back-End: Express.js (Node.js) and MongoDB: A combination of a JavaScript-based back-end with a NoSQL database like MongoDB.
Front-End: HTML/CSS/JavaScript
Back-End: Ruby on Rails and PostgreSQL: Ruby on Rails paired with the PostgreSQL database.
Front-End: HTML/CSS/JavaScript
Back-End: PHP and MySQL: A classic combination for building dynamic web applications.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes