Text
§Python - Scalability and Performance Considerations
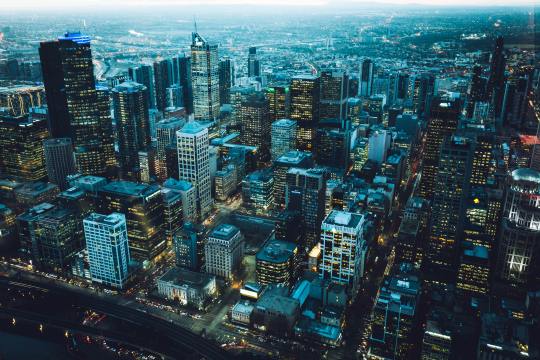
1. Scaling Strategies:
- Definition: Scalability is the ability of a system to handle increased loads. Strategies include vertical scaling (adding more resources to a single server) and horizontal scaling (adding more servers).
- Practical Example: Setting up a load balancer to distribute incoming traffic to multiple servers for horizontal scaling.
```nginx
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
```
2. Caching Techniques:
- Definition: Caching involves storing frequently accessed data to reduce the need to retrieve it from the original source, improving response times.
- Practical Example: Implementing caching in a Python web application using Flask-Caching.
```python
from flask import Flask
from flask_caching import Cache
app = Flask(__name)
cache = Cache(app, config={'CACHE_TYPE': 'simple'})
@app.route('/')
@cache.cached(timeout=60)
def cached_route():
return 'This is a cached response.'
if __name__ == '__main__':
app.run()
```
3. Load Balancing and Clustering:
- Definition: Load balancers distribute incoming requests among multiple servers, while clustering involves a group of servers working together as a single system.
- Practical Example: Implementing load balancing and clustering for a Python application using Nginx and Gunicorn.
```nginx
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
```
4. Profiling and Monitoring:
- Definition: Profiling and monitoring tools are essential for identifying performance bottlenecks and monitoring system health.
- Practical Example: Using Python's `cProfile` module for performance profiling in a web application.
```python
import cProfile
def slow_function():
# Simulate a slow function
for _ in range(1000000):
_ = 1 + 1
if __name__ == '__main__':
profiler = cProfile.Profile()
profiler.enable()
slow_function()
profiler.disable()
profiler.print_stats(sort='cumtime')
```
5. Scaling for High Traffic:
- Definition: Preparing your application to handle high levels of traffic efficiently. This may include optimizing database queries, using content delivery networks (CDNs), and reducing unnecessary load.
- Practical Example: Using a CDN like Amazon CloudFront to serve static content for a web application.
These considerations and practical examples are crucial for ensuring your back-end can efficiently handle increased loads, providing a responsive and reliable user experience as your application scales.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Note
bro i want to work under u
humbled to grant you the knowledge I have acquired but my schedule is pretty busy you can go through my book and courses till then...
Thankyou
God bless you.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Web Servers, Databases, and Server-Side Frameworks
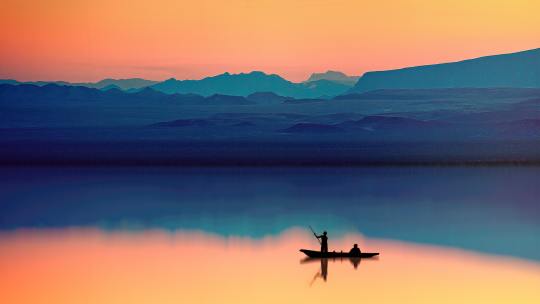
1. Web Servers:
- Definition: Web servers are responsible for handling incoming HTTP requests and serving responses to clients. Popular web servers include Apache, Nginx, and Microsoft Internet Information Services (IIS).
- Practical Example: Using Nginx to serve static files for a Python web application.
```nginx
server {
listen 80;
server_name example.com;
location /static/ {
alias /path/to/static/files;
}
location / {
proxy_pass http://127.0.0.1:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
```
2. Databases:
- Definition: Databases are crucial for storing and managing structured data. There are two main categories: relational databases (e.g., MySQL, PostgreSQL) and NoSQL databases (e.g., MongoDB, Redis).
- Practical Example: Using MySQL as a relational database for a Python web application.
```python
import mysql.connector
connection = mysql.connector.connect(
host="localhost",
user="username",
password="password",
database="mydatabase"
)
```
3. Server-Side Frameworks:
- Definition: Server-side frameworks provide tools and libraries for building web applications efficiently. Python offers several popular frameworks, including Django, Flask, and Pyramid.
- Practical Example: Creating a simple web application using the Flask framework.
```python
from flask import Flask
app = Flask(__name)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
```
4. Web Application Deployment:
- Definition: Deploying a web application involves making it available to users. Tools like Docker and cloud platforms (e.g., AWS, Azure) are essential for deployment.
- Practical Example: Deploying a Python web application using Docker.
```Dockerfile
FROM python:3.8
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
```
5. Load Balancing:
- Definition: Load balancing ensures that incoming traffic is evenly distributed among multiple servers, enhancing performance and fault tolerance.
- Practical Example: Setting up load balancing for a Python web application using Nginx.
```nginx
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
```
These components collectively form the core of the back-end technology stack, and selecting the right combination of web servers, databases, frameworks, and deployment strategies depends on the specific requirements of your project. The practical examples provided demonstrate how these components work together to create a functional web application.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
§ Components of a Typical Back-End Stack
The components of a typical back-end stack in-depth, with practical examples as part of the Back-End Technology Stack.
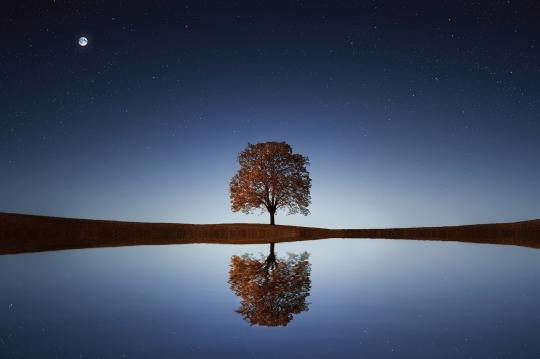
A typical back-end technology stack comprises several key components that work together to handle the server-side functionality of a web application. Let's explore each component with practical examples:
1. Web Server:
- Definition: The web server handles incoming HTTP requests and serves responses.
- Practical Example: In Python, you can use Flask to create a simple web server.
```python
from flask import Flask
app = Flask(__name)
@app.route('/')
def hello():
return "Hello, World!"
if __name__ == '__main__':
app.run()
```
2. Application Server:
- Definition: The application server executes application code, manages application logic, and communicates with the database.
- Practical Example: For a Python application, uWSGI or Gunicorn can serve as application servers.
3. Database:
- Definition: Databases store and manage data. Common options include relational databases like MySQL and PostgreSQL or NoSQL databases like MongoDB.
- Practical Example: Using Python to connect to a PostgreSQL database.
```python
import psycopg2
conn = psycopg2.connect(
database="mydb",
user="myuser",
password="mypassword",
host="localhost",
port="5432"
)
```
4. Programming Language:
- Definition: The choice of programming language can vary, but common languages include Python, Java, Ruby, and PHP.
- Practical Example: Backend code in Python can be written using web frameworks like Django or Flask.
5. Framework:
- Definition: Frameworks provide tools and libraries for building web applications efficiently.
- Practical Example: Using Django, a Python web framework, to create a simple view.
```python
from django.http import HttpResponse
def hello(request):
return HttpResponse("Hello, World!")
```
6. Cache:
- Definition: Caching systems like Redis or Memcached improve performance by storing frequently accessed data in memory.
- Practical Example: Using Redis to cache query results in a Python application.
7. Message Queue:
- Definition: Message queues like RabbitMQ or Apache Kafka are used for handling asynchronous tasks and decoupling components.
- Practical Example: Using Celery with RabbitMQ to process background tasks in a Python application.
8. Security:
- Definition: Security components include tools for user authentication, authorization, and data encryption.
- Practical Example: Implementing user authentication in a Python application using a library like Django's authentication system.
9. Middleware:
- Definition: Middleware components handle tasks like logging, request processing, and security.
- Practical Example: Creating custom middleware in a Python web application to log requests.
10. APIs:
- Definition: APIs facilitate communication with external services and applications.
- Practical Example: Creating a RESTful API in a Python application using a library like Flask-RESTful.
11. Containerization:
- Definition: Containerization tools like Docker are used to package and deploy applications and their dependencies.
- Practical Example: Dockerizing a Python web application for easier deployment and scalability.
12. Load Balancer:
- Definition: Load balancers distribute incoming traffic across multiple servers to ensure high availability and load distribution.
- Practical Example: Configuring an Nginx load balancer for a Python application.
These components collectively form the backbone of a robust back-end technology stack, and their selection depends on the specific requirements of your web application. Each component plays a crucial role in ensuring the performance, security, and functionality of your back-end system.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Collaboration Between Back-End and Front-End...
Let's continue with the code examples from the previous explanation. To run the front-end and back-end code locally, you'll need to follow these steps:
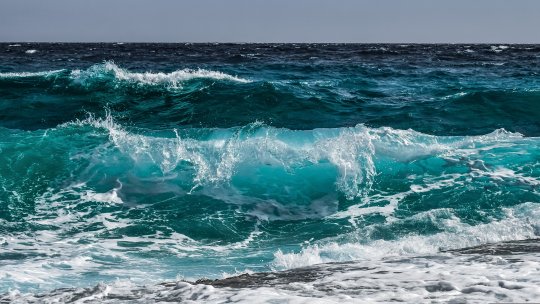
Front-End (HTML, CSS, JavaScript):
1. Create an HTML file (e.g., `index.html`) and add the following code to it:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>User Registration</title>
</head>
<body>
<h1>User Registration</h1>
<form id="registrationForm">
<label for="firstName">First Name:</label>
<input type="text" id="firstName" name="firstName" required><br>
<label for="lastName">Last Name:</label>
<input type="text" id="lastName" name="lastName" required><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required><br>
<button type="submit">Register</button>
</form>
<script>
document.getElementById("registrationForm").addEventListener("submit", function (event) {
event.preventDefault();
const formData = new FormData(event.target);
const userData = {};
formData.forEach((value, key) => {
userData[key] = value;
});
// Send the user data to the back end for processing
fetch("/api/register", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
})
.then(response => response.json())
.then(data => {
alert(data.message);
})
.catch(error => {
console.error("Error:", error);
});
});
</script>
</body>
</html>
```
Back-End (Python with Flask):
2. Create a Python file (e.g., `app.py`) and add the following code to it:
```python
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route("/api/register", methods=["POST"])
def register_user():
user_data = request.get_json()
# Perform user registration logic (e.g., validate data, save to a database)
# For simplicity, let's just return a success message
response_data = {"message": "User registered successfully"}
return jsonify(response_data)
if __name__ == "__main__":
app.run(debug=True)
```
3. Install Flask if you haven't already by running `pip install Flask` in your terminal.
4. Run the Flask app by executing `python app.py` in your terminal.
Now, when you open `index.html` in your web browser and submit the registration form, it will send a POST request to the local Flask server running on your machine, simulating a front-end and back-end interaction. The back end will respond with a success message.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shreejitjadhav#dinvstr#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
I'll provide you with a simple runnable example for both front-end and back-end development.
Front-End Development (HTML, CSS, JavaScript):
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Front-End Example</title>
<style>
/* CSS for styling */
body {
font-family: Arial, sans-serif;
}
button {
padding: 10px 20px;
background-color: #007bff;
color: #fff;
border: none;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Welcome to Our Website</h1>
<p>This is a sample front-end page.</p>
<button id="clickMeButton">Click Me</button>
<script>
// JavaScript code for front-end interactivity
document.getElementById("clickMeButton").addEventListener("click", function () {
alert("Button clicked!");
});
</script>
</body>
</html>
```
2. Save this file as `index.html` and open it in a web browser. You'll see a webpage with a button. When you click the button, an alert will pop up.
Back-End Development (Node.js):
1. Install Node.js if you haven't already (https://nodejs.org/).
2. Create a JavaScript file (server.js):
```javascript
const http = require("http");
const server = http.createServer((req, res) => {
res.writeHead(200, { "Content-Type": "text/plain" });
res.end("Hello, Back-End!\n");
});
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
```
3. Open your terminal/command prompt, navigate to the directory containing `server.js`, and run the following command to start the server:
```
node server.js
```
4. The server will start on port 3000 (you can change the port if needed). Access it by opening a web browser and entering `http://localhost:3000` in the address bar. You should see "Hello, Back-End!" displayed in the browser.
These examples demonstrate a simple front-end webpage with a button and a Node.js back-end server that responds to requests. You can use these as starting points for more complex front-end and back-end development projects.
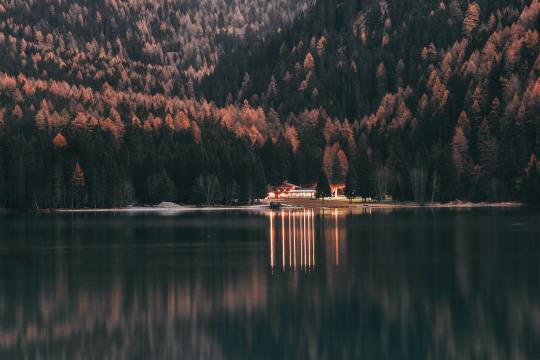
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Front-End Development Explained:
Front-End Development Explained:
What is Front-End Development?
Front-end development, also known as client-side development, focuses on creating the visual and interactive elements of a website or web application that users directly interact with. It involves building the user interface (UI) and ensuring that the web application is visually appealing, responsive, and easy to navigate.
Key Responsibilities of Front-End Developers:
1. Creating Web Layouts: Front-end developers design and create the layout of web pages using HTML (Hypertext Markup Language). HTML defines the structure and content of the web page, including headings, paragraphs, images, links, and forms.
2. Styling with CSS: Front-end developers use CSS (Cascading Style Sheets) to apply styles, such as colors, fonts, spacing, and animations, to HTML elements. This ensures a visually appealing and consistent design across the website.
3. Adding Interactivity with JavaScript: JavaScript is used to make web pages interactive. Front-end developers write JavaScript code to create features like image sliders, form validation, and dynamic content loading.
4. Responsive Design: Front-end developers ensure that websites are responsive, meaning they adapt to different screen sizes (e.g., desktop, tablet, mobile) for a seamless user experience.
Practical Example:
Let's say you're building an e-commerce website. As a front-end developer, you would design and implement the product listing page. This involves creating HTML structure for product cards, styling them with CSS to make them visually appealing, and using JavaScript to enable features like filtering products by category.
In summary, front-end development is crucial for creating the user interface and ensuring a positive user experience on websites and web applications. It involves HTML, CSS, and JavaScript to design, style, and add interactivity to web pages. Front-end developers play a key role in making a website visually appealing and user-friendly.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
Here are some popular combinations of back-end and front-end technologies:
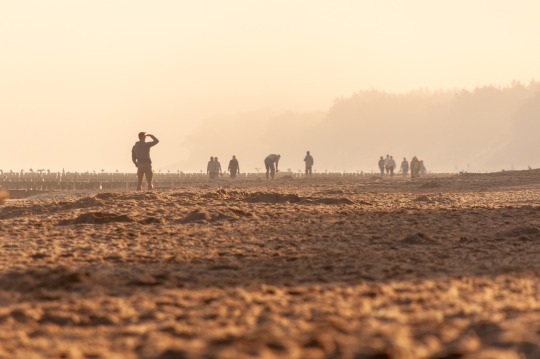
Front-End: HTML/CSS/JavaScript
Back-End: Node.js/Express.js: A JavaScript runtime environment that allows you to build server-side applications using JavaScript.
Front-End: HTML/CSS/JavaScript
Back-End: Ruby on Rails: A Ruby-based web framework known for its simplicity and convention over configuration.
Front-End: HTML/CSS/JavaScript
Back-End: Python/Django: A Python web framework that promotes rapid development and clean, pragmatic design.
Front-End: HTML/CSS/JavaScript
Back-End: PHP/Laravel: PHP is a popular server-side scripting language, and Laravel is a PHP web application framework.
Front-End: HTML/CSS/JavaScript
Back-End: Java/Spring: A Java-based framework known for its robustness and scalability.
Front-End: HTML/CSS/JavaScript
Back-End: ASP.NET (C#): A framework developed by Microsoft for building web applications using C#.
Front-End: HTML/CSS/JavaScript
Back-End: Flask (Python): A lightweight Python web framework that is often used for smaller web applications and APIs.
Front-End: HTML/CSS/JavaScript
Back-End: Express.js (Node.js) and MongoDB: A combination of a JavaScript-based back-end with a NoSQL database like MongoDB.
Front-End: HTML/CSS/JavaScript
Back-End: Ruby on Rails and PostgreSQL: Ruby on Rails paired with the PostgreSQL database.
Front-End: HTML/CSS/JavaScript
Back-End: PHP and MySQL: A classic combination for building dynamic web applications.
#fullstackdeveloper#developer#whatisfullstackdeveloper#fullstackdeveloperjobs#fullstackwebdeveloper#softwaredeveloper#dontbecomeafullstackdeveloper#shouldyoubecomeafullstackdeveloper?#aideveloper#howtobecomeasoftwaredeveloper#whyyoushouldntbecomeafulltackdeveloper#dataanalystvswebdeveloper#webdeveloper#developerjobs#howtobeaideveloper#fullstackdeveloperjob#willaireplacewebdevelopers
0 notes
Text
§ Key Responsibilities of Back-End Developers
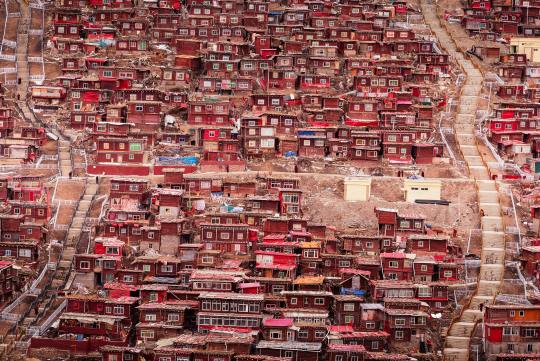
Key Responsibilities of Back-End Developers
Back-end developers play a crucial role in web development, focusing on the server-side of applications. Here's an in-depth look at their key responsibilities, along with practical examples:
Data Management:
Responsibility: Back-end developers are responsible for storing, retrieving, and managing data efficiently. This includes working with databases.
Practical Example: In an e-commerce website, back-end developers manage the database containing product details, user information, and order history. They create queries to retrieve and update this data.
Server-Side Logic:
Responsibility: Back-end developers implement the core logic and functionality of web applications. This includes handling user requests, processing data, and executing algorithms.
Practical Example: In a social media platform, back-end developers code the logic for handling user interactions, such as posting updates, liking posts, and managing user connections.
Security and Authentication:
Responsibility: Ensuring the security of user data and authentication processes is crucial. Back-end developers implement security measures to protect against unauthorized access and data breaches.
Practical Example: Back-end developers use encryption techniques to secure user passwords in the database. They also develop authentication systems that verify user credentials during login.
API Development:
Responsibility: Back-end developers often create APIs (Application Programming Interfaces) that enable communication between different parts of an application or with external systems.
Practical Example: A back-end developer working on a weather app might create an API that fetches real-time weather data from an external service and delivers it to the front end.
Performance Optimization:
Responsibility: Back-end developers optimize the performance of web applications by improving server response times, minimizing resource usage, and scaling for high traffic.
Practical Example: In an e-commerce platform, back-end developers use caching mechanisms to store frequently accessed product data, reducing the load on the server and improving page load times.
Database Design:
Responsibility: Designing efficient database schemas that align with the application's requirements is crucial. Back-end developers handle database design and normalization.
Practical Example: When building a blog platform, back-end developers design the database to store articles, comments, and user profiles in a structured manner for easy retrieval.
Middleware Development:
Responsibility: Middleware components are essential for request processing. Back-end developers create middleware to handle tasks like authentication, logging, and error handling.
Practical Example: Middleware might be used to log each incoming request, check if the user is authenticated, and route the request to the appropriate controller.
0 notes
Text
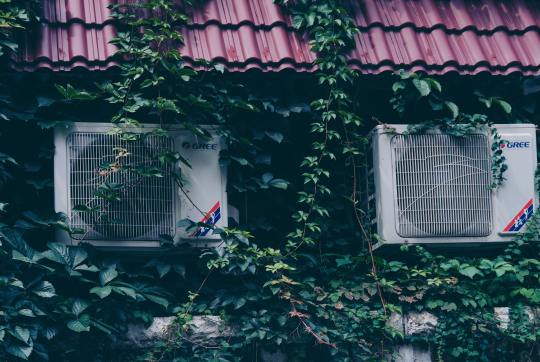
Overview of Back-End vs. Front-End
Overview of Back-End vs. Front-End Development
Back-end and front-end development are two distinct but interconnected components of web development. Understanding their differences and roles is fundamental for anyone entering the field. Here's an in-depth explanation with practical examples:
Back-End Development:
Definition: Back-end development focuses on the server-side of web applications. It deals with managing data, logic, and server operations.
Responsibilities:
Handling data storage and retrieval.
Processing and managing user requests.
Ensuring security and authentication.
Implementing - logic and algorithms.
Practical Example: Consider a user registration process. Back-end developers manage the server-side operations involved in securely storing user data, performing authentication, and handling database queries.
Front-End Development:
Definition: Front-end development is concerned with the user interface (UI) and user experience (UX) of web applications. It deals with what users see and interact with directly.
Responsibilities:
Designing and creating the user interface.
Ensuring responsive design for various devices.
Implementing client-side scripting (e.g., JavaScript) for interactivity.
Communicating with the back end via APIs.
Practical Example: In a social media application, front-end developers design and build the user profile page, including the layout, buttons, and real-time updates when a user receives new messages or notifications.
Interconnection:
Back-end and front-end development are tightly interconnected. They work together to deliver a seamless user experience.
Example: When a user submits a login form on the front end, the front-end code sends the request to the back end for authentication. If the credentials are correct, the back end responds, and the front end navigates the user to their dashboard.
Technologies:
Back-End Technologies: Python, Node.js, Ruby on Rails, and more.
Front-End Technologies: HTML, CSS, JavaScript, React, Angular, etc.
Full-Stack Development:
Some developers specialize in both front-end and back-end development, becoming full-stack developers. They can handle the entire web development process.
1 note
·
View note
Text
CHEAT SHEET TO
FULL STACK SOFTWARE DEVELOPMENT (IN 21 WEEKS)
Below is a more extended schedule to cover all the topics we've listed for approximately 2-3 months. This schedule assumes spending a few days on each major topic and sub-topic for a comprehensive understanding. Adjust the pace based on your comfort and learning progress:
Week 1-2: Introduction to Programming – Python
- Programming Structure and Basic Principles
- Programming Constructs - Loops, Functions, Arrays, etc.
Week 3: Git and Version Control
- Git Basics
- Collaborative Git Workflow
Week 4: HTML and CSS
- HTML Basics
- CSS Styling and Layout
Week 5: Object-Oriented Programming - Python
- Object-Oriented Paradigms
- Exception Handling, Collections
Week 6: Data Structures - Linear Data Structures
- Arrays, Strings, Stacks, Queues
- Linked Lists
Week 7: Data Structures - Binary Trees and Tree Traversals
- Binary Trees and Binary Search Trees
- Tree Traversal Algorithms
Week 8: Algorithms - Basic Algorithms and Analysis
- Recursion
- Searching and Sorting Algorithms
- Algorithm Analysis
Week 9: Algorithms - Advanced Algorithms and Evaluation
- Greedy Algorithms
- Graph Algorithms
- Dynamic Programming
- Hashing
Week 10: Database Design & Systems
- Data Models
- SQL Queries
- Database Normalization
- JDBC
Week 11-12: Server-Side Development & Frameworks
- Spring MVC Architecture
- Backend Development with Spring Boot
- ORM & Hibernate
- REST APIs
Week 13: Front End Development - HTML & CSS (Review)
- HTML & CSS Interaction
- Advanced CSS Techniques
Week 14-15: Front-End Development - JavaScript
- JavaScript Fundamentals
- DOM Manipulation
- JSON, AJAX, Event Handling
Week 16: JavaScript Frameworks - React
- Introduction to React
- React Router
- Building Components and SPAs
Week 17: Linux Essentials
- Introduction to Linux OS
- File Structure
- Basic Shell Scripting
Week 18: Cloud Foundations & Containers
- Cloud Service Models and Deployment Models
- Virtual Machines vs. Containers
- Introduction to Containers (Docker)
Week 19-20: AWS Core and Advanced Services
- AWS Organization & IAM
- Compute, Storage, Network
- Database Services (RDS, DynamoDB)
- PaaS - Elastic BeanStalk, CaaS - Elastic Container Service
- Monitoring & Logging - AWS CloudWatch, CloudTrail
- Notifications - SNS, SES, Billing & Account Management
Week 21: DevOps on AWS
- Continuous Integration and Continuous Deployment
- Deployment Pipeline (e.g., AWS CodePipeline, CodeCommit, CodeBuild, CodeDeploy)
- Infrastructure as Code (Terraform, CloudFormation)
Please adjust the schedule based on your individual learning pace and availability. Additionally, feel free to spend more time on topics that particularly interest you or align with your career goals. Practical projects and hands-on exercises will greatly enhance your understanding of these topics.
0 notes
Text
COURSE INTRODUCTION: MASTERING FULL STACK SOFTWARE DEVELOPMENT
Welcome to the "Mastering Full Stack Software Development" course! This comprehensive program is designed to take you on a journey from the fundamentals of programming to advanced topics in web development, data structures, algorithms, and cloud computing. Whether you're a beginner looking to start a career in software development or an experienced developer aiming to broaden your skill set, this course offers something valuable.
COURSE OVERVIEW:
In today's digital age, software development is at the heart of innovation and technological advancement. As a full-stack developer, you will have the capability to work on both the front end and back end of web applications, understand the intricacies of data management, and leverage the power of cloud computing to deploy scalable solutions. This course is carefully structured to provide you with a well-rounded education in various domains of software development.
WHAT YOU'LL LEARN:
- Programming Foundations: We kick off the course with an introduction to programming in Java, covering essential programming constructs, data types, and basic principles that form the foundation of software development.
- Version Control and Collaboration: Next, we delve into Git and version control, a critical skill for team-based development, and explore collaborative Git workflows to manage code efficiently.
- Web Development: We guide you through HTML and CSS to build the front end of web applications, giving you the ability to create visually appealing and responsive user interfaces.
- Object-Oriented Programming: You'll gain expertise in Java's object-oriented paradigm, learn about exception handling, and understand collections to efficiently manage data.
- Data Structures and Algorithms: Explore data structures like arrays, linked lists, and trees, and master fundamental algorithms, ensuring efficient problem-solving and code optimization.
- Advanced Algorithms: Dive deeper into advanced algorithms, including greedy algorithms, graph algorithms, dynamic programming, and hashing.
- Database Design: Understand how to design, create, and query relational databases using SQL, and explore database normalization principles.
- Server-Side Development: Learn about server-side development using Spring MVC, Spring Boot, Hibernate, and REST APIs.
- Front End Development: Discover the world of JavaScript, DOM manipulation, JSON, and AJAX, and harness the power of React to build interactive web applications.
- Linux Essentials: Get acquainted with the Linux operating system, its file structure, and basic shell scripting for efficient system management.
- Cloud Computing: Learn the foundations of cloud computing, delve into containers with Docker, and explore Amazon Web Services (AWS) core and advanced services.
- DevOps on AWS: Gain insights into continuous integration, deployment pipelines, and infrastructure as code to streamline software development and deployment processes.
YOUR JOURNEY STARTS HERE:
This course is designed to be both challenging and rewarding. As you progress through the weeks, you'll not only gain theoretical knowledge but also apply it through practical projects and hands-on exercises. Remember that the best way to learn is by doing, so be prepared to roll up your sleeves and code!
Feel free to adjust the pace of your learning to suit your needs and interests. Dive deeper into topics that resonate with you, and don't hesitate to ask questions and seek clarification. The world of software development is vast and ever-evolving, and this course aims to equip you with the skills and knowledge you need to thrive in this dynamic field.
Let's embark on this exciting journey together, and by the end of the course, you'll have the confidence and expertise to tackle real-world software development challenges and pursue a successful career in the field.
Are you ready to become a master of full-stack software development?
Let's get started!?
0 notes
Text
Week 1-2: Introduction to Programming – Python
- Programming Structure and Basic Principles
- Programming Constructs - Loops, Functions, Arrays, etc.
This book serves as a comprehensive guide to prepare you for Python programming interviews. With clear explanations, practical examples, and hands-on exercises, you'll be well-equipped to excel in technical interviews and apply your Python programming skills effectively.
Title: Python Programming Essentials: Interview Prep Guide
Author: SHREEJIT SANJAY JADHAV
Username: @dinvstr
Introduction:
Welcome to "Python Programming Essentials: Interview Prep Guide." This concise book is designed to help you revise and reinforce your knowledge of Python programming, making you well-prepared for software development interviews. Whether you're a beginner or an experienced developer, this guide will serve as a valuable resource to brush up on Python's fundamentals.
Week 1: Programming Structure and Basic Principles
Chapter 1: Introduction to Python
- An overview of Python programming.
- Python's popularity and use cases.
- Setting up your Python development environment.
Chapter 2: Programming Basics
- Understanding Python syntax.
- Variables and data types.
- Basic input and output.
Chapter 3: Control Flow
- Conditional statements (if, else, elif).
- Looping with for and while.
- Using break and continue.
Chapter 4: Functions
- Defining and calling functions.
- Function parameters and return values.
- Scope and lifetime of variables.
Week 2: Programming Constructs - Loops, Functions, Arrays, etc.
Chapter 5: Lists and Arrays
- Creating and manipulating lists.
- List comprehensions.
- Working with multidimensional arrays.
Chapter 6: Strings and Text Processing
- String operations and methods.
- Formatting strings.
- Regular expressions in Python.
Chapter 7: Dictionaries and Sets
- Understanding dictionaries and sets.
- Dictionary methods.
- Use cases and examples.
Chapter 8: Exception Handling
- Handling exceptions using try and except.
- Raising exceptions.
- Custom exception classes.
Chapter 9: Modules and Libraries
- Importing and using modules.
- Creating your own modules.
- Exploring Python's standard library.
Conclusion:
In this book, we've covered Python's core concepts over two weeks of study, divided into easy-to-follow chapters. Each chapter includes practical examples and exercises to reinforce your learning. By the end of this guide, you'll have a solid understanding of Python's programming structure, basic principles, and important constructs.
Use this book as a tool for self-study, practice, and revision before your software development interviews. Remember to write code, work on projects, and explore more advanced topics to further enhance your Python skills. Good luck with your interviews and your future as a Python developer!
Chapter 1: Introduction to Python
Topic Overview:
In this chapter, we will explore the fundamentals of Python programming. Python is a versatile and widely-used programming language known for its simplicity and readability. It has a broad range of applications, from web development to data analysis and machine learning. Before diving into Python's syntax and features, let's start with an overview, understand its popularity, and set up our development environment.
1. An Overview of Python Programming:
Python is a high-level, interpreted, and general-purpose programming language. It was created by Guido van Rossum and first released in 1991. Python is known for its clean and readable syntax, which makes it an excellent choice for both beginners and experienced developers. Here are some key features:
- Readability: Python's syntax emphasizes code readability, which reduces the cost of program maintenance and makes it easier to understand.
- Versatility: Python is a versatile language used in various domains, including web development, data science, artificial intelligence, scientific computing, and more.
- Interpreted Language: Python is an interpreted language, meaning you don't need to compile your code before running it. This makes development faster and more interactive.
- Large Standard Library: Python has a vast standard library that provides modules and functions for a wide range of tasks, reducing the need for external libraries.
2. Python's Popularity and Use Cases:
Python's popularity has grown steadily over the years, and it's now one of the most widely-used programming languages. Here are some reasons for its popularity:
- Web Development: Python is used for web development with frameworks like Django and Flask.
- Data Science: Python is a go-to language for data analysis, data visualization, and machine learning, thanks to libraries like NumPy, pandas, Matplotlib, and scikit-learn.
- Automation: Python is excellent for automating repetitive tasks and scripting.
- Scientific Computing: Scientists and researchers use Python for scientific computing and simulations.
3. Setting Up Your Python Development Environment:
Before you start coding in Python, you need to set up your development environment. Here are the basic steps:
- Install Python: Download and install Python from the official website ( https://www.python.org/ ).
- Choose an IDE or Text Editor: You can use IDEs like PyCharm or VSCode or text editors like Sublime Text or Atom.
- Write Your First Python Program: Create a simple "Hello, World!" program to test your environment:
```python
print("Hello, World!")
```
- Run Your Program: Save the file with a `.py` extension ( e.g., `hello.py` ) and run it from the terminal:
```bash
python hello.py
```
Conclusion:
In this chapter, we've introduced Python as a versatile and popular programming language. We've discussed its key features, use cases, and how to set up a Python development environment. As you prepare for your interview, remember to practice writing Python code, explore libraries relevant to your field of interest, and build small projects to solidify your knowledge. Python's simplicity and power make it an exciting language to learn and work with. Good luck with your Python journey!.
Chapter 2: Programming Basics
Topic Overview:
In this chapter, we will delve into the fundamental building blocks of Python programming. Understanding Python syntax, variables, data types, and input/output operations is crucial as they form the foundation for more advanced programming concepts. Let's explore these basics with examples.
1. Understanding Python Syntax:
Python's syntax is known for its simplicity and readability. Here are some essential syntax rules and examples:
- Indentation: Python uses indentation to define code blocks, such as loops and functions. Indentation should be consistent (usually four spaces) to avoid syntax errors.
Example:
```python
for i in range(5):
print(i) # Correct indentation
```
- Comments: Use the `#` symbol to add comments to your code. Comments are ignored by the interpreter.
Example:
```python
# This is a comment
```
2. Variables and Data Types:
In Python, variables are used to store data. Python is dynamically typed, which means you don't need to specify the data type of a variable explicitly. Here are some common data types:
- Integer ( `int` ): Represents whole numbers.
Example:
```python
age = 25
```
- Float ( `float` ): Represents floating-point numbers (decimals).
Example:
```python
price = 19.99
```
- String ( `str` ): Represents text. Enclose strings in single or double quotes.
Example:
```python
name = "Alice"
```
- Boolean ( `bool` ): Represents True or False values.
Example:
```python
is_valid = True
```
3. Basic Input and Output:
To interact with the user and display results, Python provides input and output functions.
- Input ( `input()` ): Reads user input as a string.
Example:
```python
name = input("Enter your name: ")
```
- Output ( `print()` ): Displays information to the console.
Example:
```python
print("Hello, World!")
```
You can format output using placeholders:
Example:
```python
name = "Alice"
age = 30
print("Name: {}, Age: {}".format(name, age))
print(name,age)
print(name,"with age:", age)
```
Conclusion:
In this chapter, we've covered essential programming basics in Python, including syntax, variables, data types, and basic input/output operations. These fundamentals are the building blocks of Python programming and will serve as a strong foundation for more advanced topics. As you prepare for your interview, practice writing code, experiment with different data types, and use input/output functions to create interactive programs. Python's simplicity and versatility make it an excellent choice for both beginners and experienced developers. Good luck with your Python journey!
Chapter 3: Control Flow
Topic Overview:
Control flow structures are essential for directing the execution of your Python programs. In this chapter, we'll explore conditional statements like `if`, `else`, and `elif`, as well as looping constructs using `for` and `while` loops. We'll also learn how to control loop execution with `break` and `continue`. Let's dive into these concepts with examples.
1. Conditional Statements ( `if`, `else`, `elif` ):
Conditional statements allow your program to make decisions and execute different code blocks based on certain conditions. Here's how they work:
- `if` Statement: It evaluates a condition and executes a block of code if the condition is true.
Example:
```python
age = 20
if age >= 18:
print("You are an adult.")
```
- `else` Statement: It executes a block of code when the `if` condition is false.
Example:
```python
age = 15
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
```
- `elif` Statement: Used to check multiple conditions sequentially.
Example:
```python
grade = 85
if grade >= 90:
print("A")
elif grade >= 80:
print("B")
elif grade >= 70:
print("C")
else:
print("D")
```
2. Looping with `for` and `while` Loops:
Loops are used to execute a block of code repeatedly.
- `for` Loop: Iterates over a sequence (e.g., list, tuple, string).
Example:
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
```
- `while` Loop: Repeats a block of code as long as a condition is true.
Example:
```python
count = 0
while count < 5:
print(count)
count += 1
```
3. Using `break` and `continue`:
- `break` Statement: Exits the loop prematurely, even if the loop condition is still true.
Example:
```python
for num in range(10):
if num == 5:
break
print(num)
```
- `continue` Statement: Skips the current iteration and continues to the next iteration.
Example:
```python
for num in range(5):
if num == 2:
continue
print(num)
```
Conclusion:
In this chapter, we've covered control flow structures in Python, including conditional statements (`if`, `else`, `elif`), looping constructs (`for` and `while` loops), and how to control loop execution with `break` and `continue`. These concepts are essential for creating dynamic and flexible programs. As you prepare for your interview, practice writing code that uses these control flow structures to solve various problems. Understanding how to make decisions and iterate through data is a key skill for any Python developer. Good luck with your interview preparation!
Chapter 4: Functions
Topic Overview:
Functions are a fundamental concept in Python. They allow you to encapsulate and reuse blocks of code, making your programs more organized and efficient. In this chapter, we'll explore how to define and call functions, work with function parameters and return values, and understand the scope and lifetime of variables within functions.
1. Defining and Calling Functions:
- Defining a Function: To define a function, use the `def` keyword followed by the function name and parentheses.
Example:
```python
def greet():
print("Hello, World!")
```
- Calling a Function: To execute a function, use its name followed by parentheses.
Example:
```python
greet() # Call the greet function
```
2. Function Parameters and Return Values:
- Function Parameters: You can pass data to a function through parameters. Parameters are defined inside the parentheses when defining a function.
Example:
```python
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Call the greet function with a parameter
```
- Return Values: Functions can return values using the `return` statement. The returned value can be assigned to a variable.
Example:
```python
def add(x, y):
return x + y
result = add(3, 4) # Call the add function and store the result
```
3. Scope and Lifetime of Variables:
- Scope: Variables defined inside a function have local scope, meaning they are only accessible within that function.
Example:
```python
def my_function():
x = 10 # Local variable
print(x)
my_function()
# print(x) # This would result in an error because x is not accessible here
```
- Lifetime: Variables have a lifetime that corresponds to their scope. They are created when the function is called and destroyed when the function exits.
Example:
```python
def my_function():
y = 5
print(y)
my_function() # Variable y is created and printed
# print(y) # This would result in an error because y is destroyed after the function call
```
Conclusion:
In this chapter, we've covered the essentials of functions in Python. You've learned how to define and call functions, pass data through parameters, and return values. Additionally, we discussed the scope and lifetime of variables within functions. As you prepare for your interview, practice creating functions to solve various tasks and understand how to manage variables within functions. Functions are a powerful tool for building modular and maintainable code. Good luck with your interview preparation!
Chapter 5: Lists and Arrays
Topic Overview:
Lists and arrays are essential data structures in Python, used to store and manipulate collections of data. In this chapter, we'll explore how to create and manipulate lists, utilize list comprehensions for efficient data processing, and work with multidimensional arrays.
1. Creating and Manipulating Lists:
- Creating Lists: Lists in Python are created by enclosing elements within square brackets `[]`.
Example:
```python
fruits = ["apple", "banana", "cherry"]
```
- Accessing Elements: Elements in a list are accessed using their index, starting from 0.
Example:
```python
first_fruit = fruits[0] # Access the first element
```
- Modifying Lists: Lists are mutable, meaning you can change their elements.
Example:
```python
fruits[1] = "grape" # Modify the second element
```
- List Methods: Python provides various methods for manipulating lists, such as `append()`, `pop()`, `remove()`, and more.
Example:
```python
fruits.append("orange") # Add an element to the end
fruits.pop(0) # Remove the first element
```
2. List Comprehensions:
- List Comprehension: It's a concise way to create lists based on existing lists.
Example:
```python
numbers = [1, 2, 3, 4, 5]
squares = [x ** 2 for x in numbers] # Create a list of squares
```
3. Working with Multidimensional Arrays:
- Multidimensional Lists: Lists can contain other lists, creating multidimensional data structures.
Example:
```python
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
```
- Accessing Elements: Accessing elements in a multidimensional list involves specifying both row and column indices.
Example:
```python
element = matrix[1][2] # Access the element in the second row, third column
```
Conclusion:
In this chapter, we've explored the power of lists and arrays in Python. You've learned how to create and manipulate lists, utilize list comprehensions for concise data transformations, and work with multidimensional arrays for more complex data structures. As you prepare for your interview, practice solving problems that involve lists and arrays, as they are fundamental to many programming tasks. Understanding these data structures will enhance your ability to work with data efficiently in Python. Good luck with your interview preparation!
Chapter 6: Strings and Text Processing
Topic Overview:
Strings are a fundamental data type in Python used for representing text and characters. In this chapter, we will explore various aspects of strings, including common string operations and methods, formatting strings for display, and using regular expressions for advanced text processing.
1. String Operations and Methods:
- String Creation: Strings can be created using single or double quotes.
Example:
```python
text = "Hello, World!"
```
- String Concatenation: You can combine strings using the `+` operator.
Example:
```python
greeting = "Hello, "
name = "Alice"
message = greeting + name
```
- String Methods: Python provides a variety of built-in string methods for tasks like splitting, joining, searching, and modifying strings.
Example:
```python
text = "Python is fun"
words = text.split() # Split the string into words
```
2. Formatting Strings:
- String Formatting: Python offers several ways to format strings, including using f-strings, the `.format()` method, and the `%` operator.
Example (f-string):
```python
name = "Alice"
age = 30
message = f"My name is {name} and I am {age} years old."
```
3. Regular Expressions in Python:
- Regular Expressions (Regex): Regex is a powerful tool for pattern matching and text manipulation. Python's `re` module enables you to work with regular expressions.
Example:
```python
import re
text = "Email me at [email protected] or [email protected]"
pattern = r'\S+@\S+' # Match email addresses
matches = re.findall(pattern, text)
print(matches) # ['[email protected]', '[email protected]']
```
Conclusion:
In this chapter, we've covered the essentials of working with strings in Python. You've learned about string creation, concatenation, and common string methods. Additionally, we explored different string formatting techniques for creating well-structured output. Lastly, we introduced regular expressions as a powerful tool for advanced text processing tasks.
As you prepare for your interview, practice using strings and regular expressions to solve text-related problems. These skills are valuable for working with data, parsing information, and ensuring data quality. Mastery of string manipulation is a fundamental skill for any Python developer. Good luck with your interview preparation!
Chapter 7: Dictionaries and Sets
Topic Overview:
Dictionaries and sets are important data structures in Python. In this chapter, we will explore the concepts of dictionaries and sets, understand their methods and use cases, and provide examples to solidify your understanding.
1. Understanding Dictionaries and Sets:
- Dictionaries: A dictionary is an unordered collection of key-value pairs. Each key is unique and maps to a specific value.
Example:
```python
student = {"name": "Alice", "age": 25, "grade": "A"}
```
- Sets: A set is an unordered collection of unique elements. It is useful for tasks that require uniqueness.
Example:
```python
unique_numbers = {1, 2, 3, 4, 5}
```
2. Dictionary Methods:
- Accessing Values: You can access dictionary values using keys.
Example:
```python
name = student["name"] # Access the value associated with the "name" key
```
- Adding and Modifying: You can add new key-value pairs or modify existing ones.
Example:
```python
student["age"] = 26 # Modify the age value
student["city"] = "New York" # Add a new key-value pair
```
- Dictionary Methods: Python provides various methods like `keys()`, `values()`, and `items()` for working with dictionaries.
Example:
```python
keys = student.keys() # Get a list of keys
values = student.values() # Get a list of values
```
3. Use Cases and Examples:
- Use Case 1
- Frequency Count: Dictionaries can be used to count the frequency of elements in a list.
Example:
```python
alphabets = ["apple", "banana", "apple", "cherry"]
word_count = {}
for word in alphabets:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
```
- Use Case 2
- Set Operations: Sets are useful for operations like union, intersection, and difference.
Example:
```python
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union = set1.union(set2) # Union of sets
intersection = set1.intersection(set2) # Intersection of sets
```
Conclusion:
In this chapter, we've explored the concepts of dictionaries and sets in Python. You've learned how dictionaries store key-value pairs and sets store unique elements. Additionally, we covered dictionary methods for accessing, adding, and modifying elements, as well as use cases for these data structures.
As you prepare for your interview, practice working with dictionaries and sets to solve real-world problems. Understanding when and how to use these data structures can greatly enhance your Python programming skills. Good luck with your interview preparation!
Chapter 8: Exception Handling
Topic Overview:
Exception handling is a crucial aspect of writing robust and reliable Python code. In this chapter, we will explore how to handle exceptions using `try` and `except` blocks, how to raise exceptions, and how to create custom exception classes to handle specific error conditions.
1. Handling Exceptions Using `try` and `except`:
- Basic Exception Handling: Exceptions are errors that occur during program execution. You can catch and handle exceptions using `try` and `except` blocks.
Example:
```python
try:
result = 10 / 0 # Attempt to divide by zero
except ZeroDivisionError as e:
print(f"Error: {e}")
```
- Handling Multiple Exceptions: You can handle different types of exceptions in separate `except` blocks.
Example:
```python
try:
value = int("abc") # Attempt to convert a non-integer string to int
except ValueError as e:
print(f"ValueError: {e}")
except Exception as e:
print(f"An error occurred: {e}")
```
2. Raising Exceptions:
- Raising Exceptions: You can explicitly raise exceptions using the `raise` statement.
Example:
```python
age = -5
if age < 0:
raise ValueError("Age cannot be negative")
```
3. Custom Exception Classes:
- Creating Custom Exceptions: You can create custom exception classes by subclassing the built-in `Exception` class. This allows you to handle application-specific error conditions.
Example:
```python
class CustomError(Exception):
def __init__(self, message):
super().__init__(message)
try:
raise CustomError("This is a custom exception")
except CustomError as e:
print(f"Custom Error: {e}")
```
Conclusion:
In this chapter, we've explored exception handling in Python. You've learned how to use `try` and `except` blocks to handle exceptions gracefully, how to raise exceptions to indicate errors explicitly, and how to create custom exception classes for specialized error handling.
Exception handling is crucial for writing reliable and fault-tolerant code. As you prepare for your interview, practice handling different types of exceptions and consider scenarios where custom exceptions might be useful in your applications. Mastering exception handling will help you write more robust Python programs. Good luck with your interview preparation!
Chapter 9: Modules and Libraries
Topic Overview:
In this chapter, we will delve into the world of modules and libraries in Python. You'll learn how to import and use existing modules, create your own modules, and explore Python's extensive standard library, which provides a wealth of pre-built functionality.
1. Importing and Using Modules:
- Importing Modules: Python allows you to import external modules to extend the functionality of your code. You can import modules using the `import` statement.
Example:
```python
import math
result = math.sqrt(25) # Using the sqrt function from the math module
```
- Alias for Modules: You can use aliases to simplify module names.
Example:
```python
import numpy as np
result = np.array([1, 2, 3])
```
2. Creating Your Own Modules:
- Creating Modules: You can create your own Python modules by saving a Python file with a `.py` extension. Functions, classes, and variables defined in the module can be accessed in other Python scripts.
Example: `my_module.py`
```python
def greet(name):
return f"Hello, {name}!"
```
Using the module in another script:
```python
import my_module
message = my_module.greet("Alice")
```
3. Exploring Python's Standard Library:
- Standard Library: Python comes with a rich standard library that provides a wide range of modules for various purposes. These modules are readily available for use in your programs.
Example:
```python
import datetime
today = datetime.date.today()
```
- Common Standard Library Modules: Some common standard library modules include `os`, `sys`, `datetime`, `json`, and `random`. These modules offer functions and classes for file operations, system interactions, date and time handling, data serialization, and random number generation, among others.
Conclusion:
In this chapter, you've learned about the importance of modules and libraries in Python. Modules extend Python's capabilities, and you can import them to access pre-built functions and classes. Additionally, you can create your own modules to encapsulate reusable code.
Python's standard library is a treasure trove of modules that cover a wide range of tasks, making it easier to develop robust and feature-rich applications. As you prepare for your interview, explore Python's standard library and practice importing and using modules to enhance your Python programming skills. Good luck with your interview preparation!
Practice Questions with Solutions:
1. Programming Structure and Basic Principles:
a. What are the basic building blocks of a Python program? Explain them briefly.
The basic building blocks of a Python program are:
- Statements: These are individual lines of code that perform specific actions or operations.
- Variables: Variables are used to store data values. They can hold different data types, such as integers, floats, strings, or custom objects.
- Data Types: Python has various data types, including int (integer), float (floating-point number), str (string), list (ordered collection), tuple (immutable collection), dict (dictionary), and more.
- Operators: Operators are used to perform operations on variables and values. Examples include + (addition), - (subtraction), * (multiplication), / (division), and % (modulus).
- Control Structures: These include conditional statements (if, elif, else) and loops (for, while) that allow you to control the flow of your program.
b. Write a Python program to calculate the factorial of a number using a recursive function.
Here's a Python program to calculate the factorial of a number using a recursive function:
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
# Input from the user
num = int(input("Enter a number: "))
if num < 0:
print("Factorial is not defined for negative numbers.")
elif num == 0:
print("Factorial of 0 is 1")
else:
print(f"Factorial of {num} is {factorial(num)}")
```
c. Explain the concept of variables, data types, and type conversion in Python with examples.
- Variables: Variables are used to store data values. In Python, you don't need to declare a variable's type explicitly. Example:
```python
x = 5 # Integer
name = "Alice" # String
pi = 3.1415 # Float
```
- Data Types: Python has several data types, such as int, float, str, list, tuple, dict, and bool. Each data type has specific characteristics and operations associated with it.
- Type Conversion: Type conversion allows you to change a variable's data type. For example:
```python
num_str = "42"
num_int = int(num_str) # Convert string to integer
```
d. How do you comment your code in Python? Provide examples of single-line and multi-line comments.
- Single-line comments: You can use the `#` symbol to write single-line comments.
Example:
```python
# This is a single-line comment
```
- Multi-line comments: Python doesn't have a built-in syntax for multi-line comments, but you can use triple-quotes (single or double) as a workaround.
Example:
```python
'''
This is a
multi-line comment
using triple-quotes.
'''
```
e. Write a Python program to find the largest element in a list.
Here's a Python program to find the largest element in a list:
```python
def find_largest_element(lst):
if len(lst) == 0:
return None # Return None for an empty list
else:
largest = lst[0]
for num in lst:
if num > largest:
largest = num
return largest
# Example list
numbers = [12, 45, 67, 23, 89, 56]
largest_num = find_largest_element(numbers)
if largest_num is not None:
print(f"The largest element in the list is {largest_num}")
else:
print("The list is empty.")
```
This program defines a function `find_largest_element` that iterates through the list to find the largest element. It also handles the case of an empty list.
2. Programming Constructs - Loops, Functions, Arrays, etc.:
a. Write a Python function to check if a given number is prime or not.
Here's a Python function to check if a number is prime or not:
```python
def is_prime(number):
if number <= 1:
return False
elif number <= 3:
return True
elif number % 2 == 0 or number % 3 == 0:
return False
i = 5
while i * i <= number:
if number % i == 0 or number % (i + 2) == 0:
return False
i += 6
return True
# Example usage:
num = int(input("Enter a number: "))
if is_prime(num):
print(f"{num} is a prime number.")
else:
print(f"{num} is not a prime number.")
```
b. What is the difference between a `for` loop and a `while` loop in Python? Provide an example of each.
- `for` loop: A `for` loop is used for iterating over a sequence (e.g., a list, tuple, string) or other iterable objects. It executes a block of code a specified number of times.
Example:
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
```
- `while` loop: A `while` loop is used to repeatedly execute a block of code as long as a specified condition is true.
Example:
```python
i = 0
while i < 5:
print(i)
i += 1
```
c. Create a Python list of integers and write code to calculate the sum of all the even numbers in the list.
Here's a Python code to calculate the sum of all the even numbers in a list:
```python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_sum = sum(x for x in numbers if x % 2 == 0)
print("Sum of even numbers:", even_sum)
```
d. Define a Python function that accepts a list of numbers and returns the list sorted in ascending order.
Here's a Python function that sorts a list of numbers in ascending order:
```python
def sort_list_ascending(input_list):
return sorted(input_list)
# Example usage:
numbers = [9, 3, 7, 1, 5]
sorted_numbers = sort_list_ascending(numbers)
print("Sorted list in ascending order:", sorted_numbers)
```
e. Explain the concept of slicing in Python lists and demonstrate it with examples.
Slicing in Python allows you to extract a portion of a list (or other iterable) by specifying a start and stop index. The syntax for slicing is `start:stop:step`, where `start` is the index where the slice begins, `stop` is the index where the slice ends (exclusive), and `step` is the interval between elements.
Example:
```python
fruits = ["apple", "banana", "cherry", "date", "fig", "grape"]
# Slice from index 1 to 4 (exclusive), with a step of 2
result = fruits[1:4:2]
print(result) # Output: ['banana', 'date']
```
In this example, we sliced the `fruits` list to extract elements from index 1 to 4 (exclusive) with a step of 2, resulting in `['banana', 'date']`. Slicing is a powerful way to work with subsets of lists in Python.
Suggested Resources for Continued Learning and Practice:
1. Online Courses:d
- Coursera: "Python for Everybody" by University of Michigan.
- edX: "Introduction to Python: Absolute Beginner" by Microsoft.
- Codecademy: Python Programming Courses.
2. Books:
- "Python Crash Course" by Eric Matthes.
- "Automate the Boring Stuff with Python" by Al Sweigart.
- "Learn Python the Hard Way" by Zed Shaw.
3. Websites and Platforms:
- LeetCode: Offers Python coding challenges and competitions.
- HackerRank: Provides Python programming exercises and challenges.
- Project Euler: Features mathematical and computational problems to solve using Python.
4. Documentation and Tutorials:
- Python Official Documentation: A comprehensive resource for Python.
- W3Schools Python Tutorial: Interactive tutorials for Python beginners.
- GeeksforGeeks: Python Programming Language.
5. YouTube Channels:
- Corey Schafer's Python Tutorials.
- Sentdex's Python Programming Tutorials.
6. Practice Projects:
- Work on small Python projects to apply your knowledge.
- Build a web scraper, create a simple game, or develop a personal blog using Python.
7. Coding Practice Platforms:
Resources for Practicing Programming Constructs:
LeetCode: LeetCode offers coding challenges in Python and other languages. It's a great platform to practice programming constructs like loops, functions, and arrays. Visit LeetCode ( https://leetcode.com/ ).
HackerRank: HackerRank provides coding challenges and competitions in Python. It covers various topics, including algorithms, data structures, and more. Explore it at HackerRank ( https://www.hackerrank.com/ ).
Project Euler: Project Euler offers a collection of challenging mathematical and computational problems. Solving these problems in Python can sharpen your programming skills. You can find it at Project Euler ( https://projecteuler.net/ ).
Remember to practice regularly, review your code, and seek help from forums or mentors when needed. As you work through these resources and practice questions, you'll strengthen your Python programming skills and be well-prepared for interviews. Good luck with your preparation!
0 notes