#serial1
Explore tagged Tumblr posts
Text
Introduction: Making a rocket flight computer might sound like a daunting task at first, but with the right guidance, it can be a fun and challenging project that will help you learn a lot about electronics and programming. A rocket flight computer is essentially a device that uses sensors, microcontrollers, and software to gather and process data during the flight of a rocket. This data can include altitude, velocity, temperature, and other parameters that can help you ensure a safe and successful rocket launch. In this article, we will guide you through the process of making a rocket flight computer from scratch, including the materials you need, the components you need to assemble, and the programming you need to do. We will also include a FAQs section at the end to answer some common questions about rocket flight computers. Materials: To make a rocket flight computer, you will need the following materials: - Microcontroller board: We recommend using an Arduino or a Raspberry Pi for this project, as they are easy to use and have plenty of online support and tutorials. - Sensors: You will need sensors to measure altitude, temperature, and other parameters. For altitude, you can use a barometric pressure sensor, such as the BMP280 or the BME280. For temperature, you can use a thermistor or a digital temperature sensor, such as the DS18B20. - GPS module: A GPS module will allow you to track the location and speed of your rocket during flight. - SD card module: An SD card module will allow you to store data on an SD card during flight, which you can later analyze on your computer. - Power source: You will need a power source for your flight computer. You can use a battery pack, a power bank, or a USB cable connected to your computer. - Breadboard and jumper wires: You will need a breadboard and jumper wires to connect your components together. - Case: You may want to use a case to protect your flight computer during flight. Components: Once you have gathered all the materials, you will need to assemble them into a flight computer. Here are the steps: 1. Connect the sensors: Connect the sensors to the microcontroller board using jumper wires. For the BMP280 or the BME280 sensor, connect VCC to 3.3V, GND to GND, SDA to A4, and SCL to A5. For the DS18B20 temperature sensor, connect VCC to 5V, GND to GND, and the signal pin to a digital pin on your microcontroller board. 2. Connect the GPS module: Connect the GPS module to the microcontroller board using jumper wires. Connect VCC to 3.3V, GND to GND, RX to a digital pin on your microcontroller board, and TX to another digital pin on your microcontroller board. 3. Connect the SD card module: Connect the SD card module to the microcontroller board using jumper wires. Connect VCC to 5V, GND to GND, MISO to pin 12, MOSI to pin 11, CLK to pin 13, and CS to pin 10. 4. Write the code: Once you have connected all the components, you will need to write the code for your flight computer. You can use the libraries provided by the sensors and GPS modules to read data from them, and you can use the SD card library to write data to the SD card. You will also need to create a loop that reads and processes data from the sensors and GPS module and writes it to the SD card. Here is an example code for an Arduino flight computer (note that you may need to adjust the code for your specific components and sensors): #include #include #include #define BMP_SDA A4 #define BMP_SCL A5 #define GPS_RX 10 #define GPS_TX 11 Adafruit_BMP280 bmp; Adafruit_GPS gps(&Serial1); File dataFile; void setup() Serial.begin(9600); while (!Serial); Serial1.begin(9600); bmp.begin(0x76); SD.begin(10); dataFile = SD.open("data.log", FILE_WRITE); gps.sendCommand(PMTK_SET_NMEA_OUTPUT_RMCONLY); gps.sendCommand(PMTK_SET_NMEA_UPDATE_10HZ); gps.sendCommand(PGCMD_ANTENNA); delay(1000); void loop() float altitude = bmp.readAltitude(1013.25); float temperature = bmp.readTemperature(); char* date = gps.date; char* time = gps.time;
float latitude = gps.latitude; char* latDir = gps.lat; float longitude = gps.longitude; char* lonDir = gps.lon; float speed = gps.speed; float angle = gps.angle; float mag = gps.mag; Serial.print(altitude); Serial.print(","); Serial.print(temperature); Serial.print(","); Serial.print(date); Serial.print(","); Serial.print(time); Serial.print(","); Serial.print(latitude, 6); Serial.print(","); Serial.print(latDir); Serial.print(","); Serial.print(longitude, 6); Serial.print(","); Serial.print(lonDir); Serial.print(","); Serial.print(speed); Serial.print(","); Serial.print(angle); Serial.print(","); Serial.println(mag); dataFile.print(altitude); dataFile.print(","); dataFile.print(temperature); dataFile.print(","); dataFile.print(date); dataFile.print(","); dataFile.print(time); dataFile.print(","); dataFile.print(latitude, 6); dataFile.print(","); dataFile.print(latDir); dataFile.print(","); dataFile.print(longitude, 6); dataFile.print(","); dataFile.print(lonDir); dataFile.print(","); dataFile.print(speed); dataFile.print(","); dataFile.print(angle); dataFile.print(","); dataFile.println(mag); dataFile.flush(); delay(100); 5. Test the flight computer: Once you have written the code, you can upload it to your microcontroller board and test the flight computer. You can do this by connecting the flight computer to your computer using a USB cable, and opening the serial monitor to see the data being read from the sensors and GPS module. You should also test the SD card module to make sure it is writing data correctly. FAQs: 1. What can I do with a rocket flight computer? A rocket flight computer can help you gather data during the flight of a rocket, which can be useful for analyzing and optimizing the rocket's performance. It can also help you ensure a safe and successful rocket launch by detecting any anomalies or errors during flight. 2. Can I use a different microcontroller board for the flight computer? Yes, you can use any microcontroller board that has the required sensors and libraries. However, Arduino and Raspberry Pi are popular choices due to their low cost and ease of use. 3. How do I mount the flight computer on my rocket? You can mount the flight computer inside a protective case or container, and attach it to the rocket using tape, glue, or a mounting bracket. Make sure the flight computer is secure and well-protected during flight. Conclusion: Making a rocket flight computer can be a challenging but rewarding project that will help you learn a lot about electronics, programming, and rocketry. By following the steps outlined in this article, you can create a flight computer that will gather and process data during the flight of a rocket, and help you ensure a safe and successful rocket launch. Remember to always prioritize safety during your rocketry experiments, and have fun exploring the exciting world of rocket science! Images: [Insert images of a rocket flight computer, sensors, microcontroller board, GPS module, SD card module, and breadboard] If you have any questions or comments Please contact us on our contact page or via our Facebook page. #rocket #flight #computer
0 notes
Text
Code the Soundtoys project:
noteSend:
https://makecode.microbit.org/_9i2TfHTsKJ40
noteReciever:
MIDI:
#include <MIDIUSB.h>
#include <MIDIUSB_Defs.h>
#include <frequencyToNote.h>
#include <pitchToFrequency.h>
#include <pitchToNote.h>
//#include <Tone.h>
/*
* MIDIUSB_test.ino
*
* Created: 4/6/2015 10:47:08 AM
* Author: gurbrinder grewal
* Modified by Arduino LLC (2015)
*/
#include "MIDIUSB.h"
/*
* This code includes the MIDIUSB library, which is used for sending and receiving MIDI messages via USB.
*/
// First parameter is the event type (0x09 = note on, 0x08 = note off).
// Second parameter is note-on/note-off, combined with the channel.
// Channel can be anything between 0-15. Typically reported to the user as 1-16.
// Third parameter is the note number (48 = middle C).
// Fourth parameter is the velocity (64 = normal, 127 = fastest).
void noteOn(byte channel, byte pitch, byte velocity) {
midiEventPacket_t noteOn = {0x09, 0x90 | channel, pitch, velocity};
MidiUSB.sendMIDI(noteOn);
Serial.print(" plays note ");
Serial.println(pitch);
}
/*
* This function sends a "note on" MIDI message with the specified channel, pitch, and velocity. The MIDI message is created as a midiEventPacket_t structure and then sent using the MidiUSB.sendMIDI function.
*/
void noteOff(byte channel, byte pitch, byte velocity) {
midiEventPacket_t noteOff = {0x08, 0x80 | channel, pitch, velocity};
MidiUSB.sendMIDI(noteOff);
}
/*
* This function sends a "note off" MIDI message with the specified channel, pitch, and velocity. The MIDI message is created as a midiEventPacket_t structure and then sent using the MidiUSB.sendMIDI function.
*/
float frequencies[127];
int a = 440; // a is 440 hz...
float midiToFreq(int midiNote) {
return (a * pow(2.0, (midiNote - 69) / 12.0));
}
/*
* This code initializes an array of float values called frequencies with 127 elements, and also defines a constant a with a value of 880. The midiToFreq function takes a MIDI note number as an argument and returns the corresponding frequency in Hz using the formula A4 * 2^((midiNote - 69) / 12).
I then multiply the result by 20 to compensate for the motor
*/
void setup() {
Serial1.begin(9600);
delay(5000);
for (int i = 0; i < 127; i++){
frequencies[i] = midiToFreq(i);
//Serial.println(frequencies[i]);
}
pinMode(3,OUTPUT);
digitalWrite(3,HIGH);
}
/*
* This function is called once when the program starts up. It initializes the serial communication at a baud rate of 115200 and waits for 5 seconds using delay(5000). It then loops through all 127 possible MIDI note numbers and calculates their corresponding frequencies using the midiToFreq function, storing the results in the frequencies array and printing them to the serial monitor.
*/
// First parameter is the event type (0x0B = control change).
// Second parameter is the event type, combined with the channel.
// Third parameter is the control number number (0-119).
// Fourth parameter is the control value (0-127).
void controlChange(byte channel, byte control, byte value) {
midiEventPacket_t event = {0x0B, 0xB0 | channel, control, value};
MidiUSB.sendMIDI(event);
}
/*
* This function sends a "control change" MIDI message with the specified channel, control number, and value. The MIDI message is created as a midiEventPacket_t structure and then sent using the MidiUSB.sendMIDI function.
*/
int voiceCount = 0;
/*
* The first line of the loop() function declares a variable called rx of type midiEventPacket_t. This variable is used to store MIDI events as they are read from the USB MIDI input.
The loop then enters a do-while loop, which continues to execute as long as the rx.header value is not equal to 0. This loop is used to continually read MIDI events and process them as they are received.
Inside the if statement, the code checks if the rx.header value is not equal to 0, indicating that a MIDI event has been received. If a MIDI event has been received, the code processes the event as follows:
*/
void loop() {
midiEventPacket_t rx;
do {
if (
rx = MidiUSB.read();
rx.header != 0) {
//Serial.print(rx.byte2);
//Serial.print(" note = ");
float myNote = frequencies[rx.byte2];
//Serial.write("$");
//Serial.println(myNote);
//Serial.print("STATUS = ");
//Serial.println(rx.byte1);
/* * This function sends a "control change" MIDI message with the specified channel, control number, and value. The MIDI message is created as a midiEventPacket_t structure and then sent using the MidiUSB.sendMIDI function. */
if(rx.byte1 == 144){
Serial1.println(rx.byte2);
voiceCount ++;
digitalWrite(3,LOW);
playNote(frequencies[rx.byte2]);
} else if(rx.byte1 = 128){
voiceCount--;
Serial1.println(rx.byte2+127);
if(voiceCount == 0){
noTone(2);
digitalWrite(3,HIGH);
}
}
}
} while (rx.header != 0);
}
/*
* If the MIDI event is a "note on" event, the code increments a counter called voiceCount, which is used to keep track of how many notes are currently being played. The code then calls a function called playNote() to play the note. The playNote() function takes a frequency as an argument and plays that frequency on pin 2 using the tone() function.
If the MIDI event is a "note off" event, the code decrements the voiceCount counter. If voiceCount is zero, this means that there are no notes currently being played, so the code turns off the sound using the noTone() function.
*/
void playNote(int note){
int voice = findVoice(note);
noteOn(0, note, 127); // send MIDI note on message with voice index
tone(2,note);
}
// Voice allocation code:
int voices[4] = {-1, -1, -1, -1};
int findVoice(int midiNote){
bool foundVoice = false;
for(int i = 0; i<4; i++){
if(voices[i] == -1){
voices[i] = midiNote;
foundVoice = true;
return i;
}
}
if(!foundVoice){
int randomlySelectedVoice = random(0,3);
voices[randomlySelectedVoice] = midiNote;
return randomlySelectedVoice;
}
}
int releaseVoice(int midiNote){
for(int i = 0; i<4; i++){
if(voices[i] == midiNote){
voices[i] = -1;
return i;
}
}
}
0 notes
Photo

I didn’t know I needed a #harleydavidson for #christmas but I kinda do now #serial1 #moshcty #notmyphoto #electricbike https://www.instagram.com/p/CHsiN0GDTZO/?igshid=1pp2gdcquofqx
0 notes
Photo

Working on a new project with 2x2cycles for the #livewire let’s see if we can pull it off! I would like to mount a #serial1 @serial1cycles onto my @harleydavidson #livewire . If we can pull this off is anyone interested In a unit for their bikes? To see my regress visit + www.facebook.com/hdlivewire [] Visit/join and like the fastest growing most in fluential #harleydavidson #livewire #electricmotorcycles group and page where enthusiasts, owners and riders share experiences, tips and tricks about this #electricmotorcycle [] [] #linkinbio for easy post/page/group access [] [] #findyourfreedom #rideon #livewire #ridethefuture #ChangeHowYouMove [] [] [] !!!YouTube !!! [] Join us to see Videos with content (how to, demos, ride alongs in stunning 360 degrees and much more!) related to the #livewire, in our new YouTube channel for all things #livewire! Visit/subscribe and like the videos. [ ] Visit https://tinyurl.com/ybwchbr2 + + https://tinyurl.com/ybwchbr2 (at Los Angeles, California) https://www.instagram.com/p/CHq8-vZBUMT/?igshid=a9gr07r5s6n1
#livewire#serial1#harleydavidson#electricmotorcycles#electricmotorcycle#linkinbio#findyourfreedom#rideon#ridethefuture#changehowyoumove
0 notes
Video
youtube
Introducing Serial 1 eBicycles, Powered By Harley-Davidson
Tendo o nome de "Serial Number One", o apelido para a primeira motocicleta da Harley-Davidson, a Serial 1 Cycle Company combina a capacidade de desenvolvimento de produto de classe mundial da Harley-Davidson e a liderança em propulsão elétrica de duas rodas com a agilidade e inovação de marca dedicada exclusivamente ao produto e cliente eBicycle.
#HD#HarleyDavidson#SerialNumberOne#eBicycle#Serial1CycleCompany#Eletrificada#Mobilidade#BicicletaEletrica#Serial1#Serial1ebicycles#novidade#lançamento
0 notes
Photo
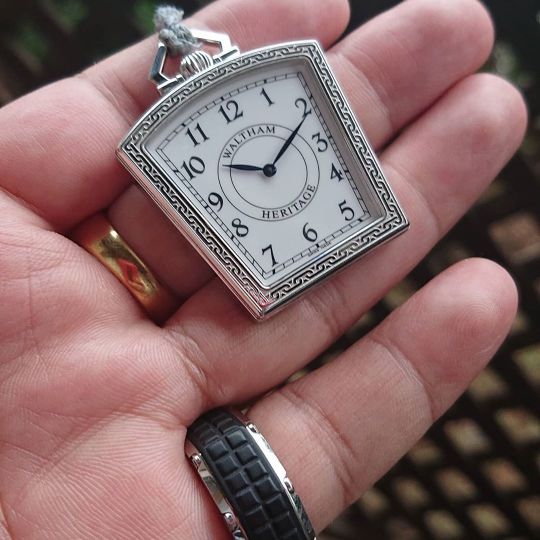
#WALTHAM #WALTHAMHERITAGE #HERITAGE #WALTHAMWATCH #HP43 #ETA7001 #POCKETWATCH #K18 #750 #WG #VERYRARE #SERIALNUMBER1 #SERIAL1 #NUMBER1 #LIMITED100 #INSTAWATCH #CHRONO24 #JAPAN #TOKYO #ウォルサム #懐中時計 #100本限定 #シリアル1 #レア #ヴィンテージ #時計 #東京 #大宮 #限定 #時計好きと繋がりたい (Saitama-shi, Saitama, Japan) https://www.instagram.com/p/B-vW1SfHDvP/?igshid=m1avb9boih3x
#waltham#walthamheritage#heritage#walthamwatch#hp43#eta7001#pocketwatch#k18#750#wg#veryrare#serialnumber1#serial1#number1#limited100#instawatch#chrono24#japan#tokyo#ウォルサム#懐中時計#100本限定#シリアル1#レア#ヴィンテージ#時計#東京#大宮#限定#時計好きと繋がりたい
0 notes
Photo

-Момент, который сломал наши сердца...
9 notes
·
View notes
Video
youtube
Pierwszy rower elektryczny Harley-Davidson | Kupowałbym.pl
1 note
·
View note
Text
Follow imperialcycles_sj on Instagram
.
.
.
#electricbikes #imperialcycles #fatbikeclub #moped #ebike #bobber #motorizedbicycle #motoredbicycles #ratrodbikes #emoped #bratstyle #emotorbike #electricbike #emoto #industrialdesign #serial1 #custommade #bicimoto #bicimotos #ev #ebikes #emopeds #electricbicycle #motorizedbicycles #motorizedbike #motorizedbikes #emobility #motoredbicycles #ebikes #ftw #forevertwowheels

12 notes
·
View notes
Text
8チャンネルMIDI-CV/Gateインターフェイスを作ってみた
Macからモジュラーシンセサイザーを操作したかったのでMIDI-CV/Gateインターフェイスを作ったという話です。コスト的には6000円程度、ただし作業時間はそれなりに長い。

モジュラーシンセサイザーはCVやGateと言われる信号で操作するので、コンピュータやシーケンサーなどの外部機器とモジュラーシンセサイザーを組み合わせる場合、何らかの形でCV信号やGate信号を外部機器から出力させなければなりません。そして、このCV信号やGate信号はたくさんの数が使えれば使えるほどいろいろなことができるようになるのですが、(お値段・流通の両方で)入手しやすいものだと1組程度のCV・Gate信号しか扱えないことが多いです。
CV・Gate信号のうち、特に扱いが面倒なのがCV信号で、こちらは完全なアナログ信号です。そのためコンピュータからCV信号を出したいという場合、D/Aコンバータ(デジタル・アナログ)コンバータという部品が必要になります。たまたま秋月電子のWebサイトを見ていたところ、Pmod DA4(今は秋月電子での取り扱いは終了)という、12ビットのD/Aコンバータ8つが1素子に組み込まれたものを見つけたので早速購入してチェックしてみたわけですが、こちらは出力電圧が0〜2.5Vとなっており、CVとして利用するには若干電圧レンジが狭いという問題がありました。
一方でこのIC(AD5628)自体について調べると、関連製品として出力電圧が0〜5Vのものもあり、さらに分解能が16ビットのものもラインアップに存在しています。ということでそちら(AD5668-2)を購入してArduinoマイコンと出力バッファを接続し、さらにArduinoからGate信号も出力できるようにした回路を作ったのがこちらになります(なお注釈にある通りこの回路には複数の不具合があります)。
そしてそれを元に作った基板がこちらとなります。

最終的な完成形はこちら。


ArduinoはArduino NANO Everyを使用しています。なぜArduino NANO Everyかというと、マイコン部分を将来的にピン互換がありより高性能なNuculeo STM32F303K8へ置き換えることを考えていたからです。とはいえArduino NANO Everyもそれなりにパワーがあるマイコンなので、普通にMIDI信号を扱うだけなら問題になることはあまりないかと思われます。
また、基板の枚数が増えるとそれだけ基板制作コストがかかるので、ここでは1枚に収めることを至上命題としました。そのため表面実装部品を多用しています。さらにAD5668-2はそれなりにお高いICなので(現時点での価格は2,272円)、失敗した時に簡単に取り外して再利用できるよう別基板(変換基板)上に実装しています。
今回の基板でもっとも大変だったのは、チップFETの実装でした。これはGate信号を安定して取り出すと共に、誤ってそこに電圧を入力してしまってもマイコンが壊れないようにするためのバッファ回路で使用しているのですが、約2×2.5mmのサイズに片面3本、計6本の端子が収まっているという高密度な部品になっており、さらにこれを4つも使っているということで、半田付けが非常に大変でした。
あとはCVの出力バッファで使っているOPアンプの電源を+-逆に設計していたり(このOPアンプは対称なピン配置だったので逆の向きに取り付けるだけで��んとかなった)、AD5668にバイパスコンデンサをつけていなかったり、MIDI信号が入ってきた時だけ光るような意図でダイオードをつけたのに結局ほぼ常時ダイオードが光っていたり(この回路だとMIDI信号が入った瞬間のみOFFになるため消灯が感知できない)、微妙に不具合があったのですが無理やりなんとか組み立てました。次のバージョンを作ることがあったら改善したいですね……。
パネルは東急ハンズで1.5mm厚のアルミ板をカットしてもらい、自宅の卓上ボール盤で穴あけ加工をしました。レールにマウントするための長穴を開けるのが地味に大変でした。最大の原因は長穴の幅(3.2mm)よりも小さいエンドミル(直径2mm)で無理やり加工しようとしたことだと思います。横着せず3.2mmのものを調達しておこうと思いました。

さて、マイコンを使った機材で面倒なのが、マイコンのプログラミングです。と言ってもArduinoではArduino MIDI Libraryという便利なライブラリがあるのでMIDI関連の実装は(MIDIについての知識があれば)そんなに難しくはありません。
また、AD5668についてもAD5668-Libraryというものが公開されていたのでこれを使えば楽勝だろうと思っていたのですが、地味にこちらが動かない。
デジタル回路的には適切に接続されているのは確認済みなので、これが昔習ったデジタル回路がアナログ的要因でまともに動かなくなるやつか……と途方に暮れていたわけですが、AD5668のデータシートをよく見たところ、このAD5668-Libraryでは初期化後のピン状態がデータシート通りにはなっていないことに気付きそこを修正したところ無事に動き出しました。
なお、Arduino NANO Everyはハードウェアシリアルポートが「Serial」と「Serial1」の2つあり、前者はUSBに、後者が基板上の端子(D0とD1)に繋がっています。そのためArduino MIDI Libraryの初期化時にSerial1を使うよう指定しなければならない点に注意が必要です。
あと、せっかくArduino上のUSBシリアルポートが利用できるということで、USBシリアルポート経由でもMIDI信号を流し込めるようにしています。これを使うと、USB-MIDIインターフェイスなどを別途用意しなくても、USB接続のみでMax/MSPのserialオブジェクト経由でMIDI信号を送信できます。ただし基板上の16ピンコネクタから電源を供給している場合、同時にArduinoのUSBポートから電源供給をすると基板上の電圧レギュレータが死にます。そのため、USBの+5Vを断線させたケーブルでの接続が必要になるかと思われます(未確認)。
8 notes
·
View notes
Text
Introduction: Making a rocket flight computer might sound like a daunting task at first, but with the right guidance, it can be a fun and challenging project that will help you learn a lot about electronics and programming. A rocket flight computer is essentially a device that uses sensors, microcontrollers, and software to gather and process data during the flight of a rocket. This data can include altitude, velocity, temperature, and other parameters that can help you ensure a safe and successful rocket launch. In this article, we will guide you through the process of making a rocket flight computer from scratch, including the materials you need, the components you need to assemble, and the programming you need to do. We will also include a FAQs section at the end to answer some common questions about rocket flight computers. Materials: To make a rocket flight computer, you will need the following materials: - Microcontroller board: We recommend using an Arduino or a Raspberry Pi for this project, as they are easy to use and have plenty of online support and tutorials. - Sensors: You will need sensors to measure altitude, temperature, and other parameters. For altitude, you can use a barometric pressure sensor, such as the BMP280 or the BME280. For temperature, you can use a thermistor or a digital temperature sensor, such as the DS18B20. - GPS module: A GPS module will allow you to track the location and speed of your rocket during flight. - SD card module: An SD card module will allow you to store data on an SD card during flight, which you can later analyze on your computer. - Power source: You will need a power source for your flight computer. You can use a battery pack, a power bank, or a USB cable connected to your computer. - Breadboard and jumper wires: You will need a breadboard and jumper wires to connect your components together. - Case: You may want to use a case to protect your flight computer during flight. Components: Once you have gathered all the materials, you will need to assemble them into a flight computer. Here are the steps: 1. Connect the sensors: Connect the sensors to the microcontroller board using jumper wires. For the BMP280 or the BME280 sensor, connect VCC to 3.3V, GND to GND, SDA to A4, and SCL to A5. For the DS18B20 temperature sensor, connect VCC to 5V, GND to GND, and the signal pin to a digital pin on your microcontroller board. 2. Connect the GPS module: Connect the GPS module to the microcontroller board using jumper wires. Connect VCC to 3.3V, GND to GND, RX to a digital pin on your microcontroller board, and TX to another digital pin on your microcontroller board. 3. Connect the SD card module: Connect the SD card module to the microcontroller board using jumper wires. Connect VCC to 5V, GND to GND, MISO to pin 12, MOSI to pin 11, CLK to pin 13, and CS to pin 10. 4. Write the code: Once you have connected all the components, you will need to write the code for your flight computer. You can use the libraries provided by the sensors and GPS modules to read data from them, and you can use the SD card library to write data to the SD card. You will also need to create a loop that reads and processes data from the sensors and GPS module, and writes it to the SD card. Here is an example code for an Arduino flight computer (note that you may need to adjust the code for your specific components and sensors): #include #include #include #define BMP_SDA A4 #define BMP_SCL A5 #define GPS_RX 10 #define GPS_TX 11 Adafruit_BMP280 bmp; Adafruit_GPS gps(&Serial1); File dataFile; void setup() Serial.begin(9600); while (!Serial); Serial1.begin(9600); bmp.begin(0x76); SD.begin(10); dataFile = SD.open("data.log", FILE_WRITE); gps.sendCommand(PMTK_SET_NMEA_OUTPUT_RMCONLY); gps.sendCommand(PMTK_SET_NMEA_UPDATE_10HZ); gps.sendCommand(PGCMD_ANTENNA); delay(1000); void loop() float altitude = bmp.readAltitude(1013.25); float temperature = bmp.readTemperature(); char* date = gps.date;
char* time = gps.time; float latitude = gps.latitude; char* latDir = gps.lat; float longitude = gps.longitude; char* lonDir = gps.lon; float speed = gps.speed; float angle = gps.angle; float mag = gps.mag; Serial.print(altitude); Serial.print(","); Serial.print(temperature); Serial.print(","); Serial.print(date); Serial.print(","); Serial.print(time); Serial.print(","); Serial.print(latitude, 6); Serial.print(","); Serial.print(latDir); Serial.print(","); Serial.print(longitude, 6); Serial.print(","); Serial.print(lonDir); Serial.print(","); Serial.print(speed); Serial.print(","); Serial.print(angle); Serial.print(","); Serial.println(mag); dataFile.print(altitude); dataFile.print(","); dataFile.print(temperature); dataFile.print(","); dataFile.print(date); dataFile.print(","); dataFile.print(time); dataFile.print(","); dataFile.print(latitude, 6); dataFile.print(","); dataFile.print(latDir); dataFile.print(","); dataFile.print(longitude, 6); dataFile.print(","); dataFile.print(lonDir); dataFile.print(","); dataFile.print(speed); dataFile.print(","); dataFile.print(angle); dataFile.print(","); dataFile.println(mag); dataFile.flush(); delay(100); 5. Test the flight computer: Once you have written the code, you can upload it to your microcontroller board and test the flight computer. You can do this by connecting the flight computer to your computer using a USB cable, and opening the serial monitor to see the data being read from the sensors and GPS module. You should also test the SD card module to make sure it is writing data correctly. FAQs: 1. What can I do with a rocket flight computer? A rocket flight computer can help you gather data during the flight of a rocket, which can be useful for analyzing and optimizing the rocket's performance. It can also help you ensure a safe and successful rocket launch by detecting any anomalies or errors during flight. 2. Can I use a different microcontroller board for the flight computer? Yes, you can use any microcontroller board that has the required sensors and libraries. However, Arduino and Raspberry Pi are popular choices due to their low cost and ease of use. 3. How do I mount the flight computer on my rocket? You can mount the flight computer inside a protective case or container, and attach it to the rocket using tape, glue, or a mounting bracket. Make sure the flight computer is secure and well-protected during flight. Conclusion: Making a rocket flight computer can be a challenging but rewarding project that will help you learn a lot about electronics, programming, and rocketry. By following the steps outlined in this article, you can create a flight computer that will gather and process data during the flight of a rocket, and help you ensure a safe and successful rocket launch. Remember to always prioritize safety during your rocketry experiments, and have fun exploring the exciting world of rocket science! Images: [Insert images of a rocket flight computer, sensors, microcontroller board, GPS module, SD card module, and breadboard] If you have any questions or comments Please contact us on our contact page or via our Facebook page. #rocket #flight #computer
0 notes
Text
Source code for Youtube Tutorial on Teensy/Arduino Development
// Source Code for Serial BT connection Youtube video
// Video can be found here: https://youtu.be/0wYoakgjckg
// Include for LED libary #include <FastLED.h>
// Define Constants #define NUM_LEDS 6 #define DATA_PIN 17
// our led strip refrence CRGB leds[NUM_LEDS];
void setup() { // If you need a delay for power or some other thing delay(10);
// Setup LEDs using FastLED libary FastLED.addLeds<NEOPIXEL, DATA_PIN>(leds, NUM_LEDS);
// Set color to yellow while setting up things leds[0] = CRGB::Yellow; FastLED.show();
// Setup Serial ports Serial1.begin(9600); //rx1 and tx1 = pins 0 and 1 on Teensy for BT Serial.begin(9600); // Default Serial over USB
// why not delay(200);
// Setup done, set color to green leds[0] = CRGB::Green; FastLED.show();
} // end setup
void loop() { char inChar; //if(Serial1.available() > 0) // use for BT //if(Serial.available() > 0) // use for USB if(Serial1.available() || Serial.available()) { //inChar = (char)Serial1.read(); // will not be -1 -- BT //inChar = (char)Serial.read(); // will not be -1 -- USB
inChar = (char)Serial1.available()?Serial1.read():Serial.read();
// Set base colors leds[0] = CRGB::Green; // take out with leap leds[1] = CRGB::Black; leds[2] = CRGB::Black; leds[3] = CRGB::Black; leds[4] = CRGB::Black; leds[5] = CRGB::Black; switch(inChar) { // No Hand case 'N': leds[0] = CRGB::Red;
break;
// Right Hand Sceen case 'R': leds[0] = CRGB::Green; break;
// Right Hand Sideways case 'S': leds[0] = CRGB::Blue; break;
// 0 Fingers case '0': case '`': leds[1] = CRGB::Black; leds[2] = CRGB::Black; leds[3] = CRGB::Black; leds[4] = CRGB::Black; leds[5] = CRGB::Black; break;
// one finger case '1': leds[1] = CRGB::Green;
break;
case '2': leds[1] = CRGB::Green; leds[2] = CRGB::Green;
break;
case '3': leds[1] = CRGB::Green; leds[2] = CRGB::Green; leds[3] = CRGB::Green;
break;
case '4': leds[1] = CRGB::Green; leds[2] = CRGB::Green; leds[3] = CRGB::Green; leds[4] = CRGB::Green;
break;
case '5': leds[1] = CRGB::Green; leds[2] = CRGB::Green; leds[3] = CRGB::Green; leds[4] = CRGB::Green; leds[5] = CRGB::Green; break;
// used if some other input is recived. AKA: unprogrammed key above. default: leds[0] = CRGB::Red; break; } // end switch
// Apply Lights FastLED.show();
// Will write to both serial ports if available if(Serial.availableForWrite()) { Serial.println(inChar); // USB } if(Serial1.availableForWrite()) { Serial1.println(inChar); // BT }
}// end if
} // end loop
1 note
·
View note
Photo

Serial 1 - Harley-Davidson kündigt den Einstieg in den Markt der E-Bikes an. #serial1 #serial1harleydavidson #harleysite #harleydavidson #elektrofahrraeder #ebikes #harleyebikes https://www.instagram.com/p/CG5FdP9hV0E/?igshid=w7nxgwp8pg8z
1 note
·
View note
Text
0 notes
Text
Serial1's new e-bikes run on Google Cloud
Serial1’s new e-bikes run on Google Cloud
Bicycles are no longer just two wheels with handlebars. With the arrival of electric models, they have become hyper-connected vehicles powered by the cloud! The manufacturer Serial1 has thus unveiled an agreement to integrate its new models with Google Cloud. The second-generation CTY e-bikes made by Serial1 are cloud-powered. More precisely, by the cloud of Google Cloud! The manufacturer and…

View On WordPress
0 notes
Text

ESP32 – Programming Three Serial Ports (UARTs) Using the Arduino IDE
When working with the Arduino IDE, you access the serial ports through the Serial class (Serial, Serial1, Serial2). However, Serial1 and Serial2 will not work with the ESP32, and there is a good reason for that.
ESP32 – Programming Three Serial Ports (UARTs) Using the Arduino IDE - Copperhill (copperhilltech.com)
0 notes