#Maven dependency in pom.xml
Explore tagged Tumblr posts
Text
Spring Security Using Facebook Authorization: A Comprehensive Guide
In today's digital landscape, integrating third-party login mechanisms into applications has become a standard practice. It enhances user experience by allowing users to log in with their existing social media accounts. In this blog post, we will walk through the process of integrating Facebook authorization into a Spring Boot application using Spring Security.
Table of Contents
Introduction
Prerequisites
Setting Up Facebook Developer Account
Creating a Spring Boot Application
Configuring Spring Security for OAuth2 Login
Handling Facebook User Data
Testing the Integration
Conclusion
1. Introduction
OAuth2 is an open standard for access delegation, commonly used for token-based authentication. Facebook, among other social media platforms, supports OAuth2, making it possible to integrate Facebook login into your Spring Boot application.
2. Prerequisites
Before we start, ensure you have the following:
JDK 11 or later
Maven
An IDE (e.g., IntelliJ IDEA or Eclipse)
A Facebook Developer account
3. Setting Up Facebook Developer Account
To use Facebook login, you need to create an app on the Facebook Developer portal:
Go to the Facebook Developer website and log in.
Click on "My Apps" and then "Create App."
Choose an app type (e.g., "For Everything Else") and provide the required details.
Once the app is created, go to "Settings" > "Basic" and note down the App ID and App Secret.
Add a product, select "Facebook Login," and configure the Valid OAuth Redirect URIs to http://localhost:8080/login/oauth2/code/facebook.
4. Creating a Spring Boot Application
Create a new Spring Boot project with the necessary dependencies. You can use Spring Initializr or add the dependencies manually to your pom.xml.
Dependencies
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies>
5. Configuring Spring Security for OAuth2 Login
Next, configure Spring Security to use Facebook for OAuth2 login.
application.properties
Add your Facebook app credentials to src/main/resources/application.properties.spring.security.oauth2.client.registration.facebook.client-id=YOUR_FACEBOOK_APP_ID spring.security.oauth2.client.registration.facebook.client-secret=YOUR_FACEBOOK_APP_SECRET spring.security.oauth2.client.registration.facebook.redirect-uri-template={baseUrl}/login/oauth2/code/{registrationId} spring.security.oauth2.client.registration.facebook.scope=email,public_profile spring.security.oauth2.client.registration.facebook.client-name=Facebook spring.security.oauth2.client.registration.facebook.authorization-grant-type=authorization_code spring.security.oauth2.client.provider.facebook.authorization-uri=https://www.facebook.com/v11.0/dialog/oauth spring.security.oauth2.client.provider.facebook.token-uri=https://graph.facebook.com/v11.0/oauth/access_token spring.security.oauth2.client.provider.facebook.user-info-uri=https://graph.facebook.com/me?fields=id,name,email spring.security.oauth2.client.provider.facebook.user-name-attribute=id
Security Configuration
Create a security configuration class to handle the OAuth2 login.import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.oauth2.client.oidc.userinfo.OidcUserService; import org.springframework.security.oauth2.client.userinfo.DefaultOAuth2UserService; import org.springframework.security.oauth2.client.userinfo.OAuth2UserService; import org.springframework.security.oauth2.core.oidc.user.OidcUser; import org.springframework.security.oauth2.core.user.OAuth2User; import org.springframework.security.web.authentication.SimpleUrlAuthenticationFailureHandler; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests(authorizeRequests -> authorizeRequests .antMatchers("/", "/error", "/webjars/**").permitAll() .anyRequest().authenticated() ) .oauth2Login(oauth2Login -> oauth2Login .loginPage("/login") .userInfoEndpoint(userInfoEndpoint -> userInfoEndpoint .oidcUserService(this.oidcUserService()) .userService(this.oAuth2UserService()) ) .failureHandler(new SimpleUrlAuthenticationFailureHandler()) ); } private OAuth2UserService<OidcUserRequest, OidcUser> oidcUserService() { final OidcUserService delegate = new OidcUserService(); return (userRequest) -> { OidcUser oidcUser = delegate.loadUser(userRequest); // Custom logic here return oidcUser; }; } private OAuth2UserService<OAuth2UserRequest, OAuth2User> oAuth2UserService() { final DefaultOAuth2UserService delegate = new DefaultOAuth2UserService(); return (userRequest) -> { OAuth2User oAuth2User = delegate.loadUser(userRequest); // Custom logic here return oAuth2User; }; } }
6. Handling Facebook User Data
After a successful login, you might want to handle and display user data.
Custom User Service
Create a custom service to process user details.import org.springframework.security.oauth2.core.user.OAuth2User; import org.springframework.security.oauth2.core.user.OAuth2UserAuthority; import org.springframework.security.oauth2.client.userinfo.OAuth2UserService; import org.springframework.security.oauth2.client.oidc.userinfo.OidcUserService; import org.springframework.security.oauth2.core.oidc.user.OidcUser; import org.springframework.security.oauth2.client.userinfo.DefaultOAuth2UserService; import org.springframework.security.oauth2.client.oidc.userinfo.OidcUserRequest; import org.springframework.security.oauth2.client.userinfo.OAuth2UserRequest; import org.springframework.stereotype.Service; import java.util.Map; import java.util.Set; import java.util.HashMap; @Service public class CustomOAuth2UserService implements OAuth2UserService<OAuth2UserRequest, OAuth2User> { private final DefaultOAuth2UserService delegate = new DefaultOAuth2UserService(); @Override public OAuth2User loadUser(OAuth2UserRequest userRequest) { OAuth2User oAuth2User = delegate.loadUser(userRequest); Map<String, Object> attributes = new HashMap<>(oAuth2User.getAttributes()); // Additional processing of attributes if needed return oAuth2User; } }
Controller
Create a controller to handle login and display user info.import org.springframework.security.core.annotation.AuthenticationPrincipal; import org.springframework.security.oauth2.core.user.OAuth2User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; @Controller public class LoginController { @GetMapping("/login") public String getLoginPage() { return "login"; } @GetMapping("/") public String getIndexPage(Model model, @AuthenticationPrincipal OAuth2User principal) { if (principal != null) { model.addAttribute("name", principal.getAttribute("name")); } return "index"; } }
Thymeleaf Templates
Create Thymeleaf templates for login and index pages.
src/main/resources/templates/login.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Login</title> </head> <body> <h1>Login</h1> <a href="/oauth2/authorization/facebook">Login with Facebook</a> </body> </html>
src/main/resources/templates/index.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <title>Home</title> </head> <body> <h1>Home</h1> <div th:if="${name}"> <p>Welcome, <span th:text="${name}">User</span>!</p> </div> <div th:if="${!name}"> <p>Please <a href="/login">log in</a>.</p> </div> </body> </html>
7. Testing the Integration
Run your Spring Boot application and navigate to http://localhost:8080. Click on the "Login with Facebook" link and authenticate with your Facebook credentials. If everything is set up correctly, you should be redirected to the home page with your Facebook profile name displayed.
8. Conclusion
Integrating Facebook login into your Spring Boot application using Spring Security enhances user experience and leverages the power of OAuth2. With this setup, users can easily log in with their existing Facebook accounts, providing a seamless and secure authentication process.
By following this guide,
2 notes
·
View notes
Text
Large software applications can be customized and enhanced for unique or specific abilities by adding a set of software components called plugins. Generally, plugins are used in web browsers, virus scans, recognition and display of new file types. Maven is a leading Java project management tool. A project’s build, documentation, reporting can be managed from a central piece of information. This concept is based on the project object model (POM). Maven provides a uniform build system by building a project using POM concept and plugins which will be shared by all projects. Using the right plugin in your development environment can save a lot of unwanted efforts in day to day coding and other activities. We have found that some of these plugins help automate and simplify the everyday build and release related work. The best Maven plugins we found to be really useful and time-saving for your project team are described below: License Management Plugins White Source's Licenses Management Maven Plug-in Any software development project that uses Open Source (almost all of us), must identify and track the OSS components it uses, their licenses, requirements, etc. This can be quite a time consuming and sometimes complex for developers. White Source offers a free SaaS solution for that. The White Source's Maven plugin continuously and automatically updates the White Source repository whenever a new open source component is added, to ensure that all the open source components are immediately reported, analyzed, and reviewed for approval -without any overhead. This allows companies to accelerate the development process and manage the open source license more efficiently, and with fewer errors. White Source provides a simple to use, yet powerful solution for companies that need to manage their open source assets to ensure license compliance in order to avoid pitfalls such as lawsuits, penalties, and lost business. Developers and managers use White Source’s free cloud-based solution to track, audit and report on open source components throughout the software development life cycle. Unlike other solutions, White Source is a convenient cost-effective solution even for medium and small companies. With White Source’s easy-to-use, cloud-based platform companies can: Track the inventory of Open Source Software (OSS) modules used by development Automate the adoption and approval process Get continuous updates about legal and technical vulnerabilities Audit and report on OSS status and compliance White Source Software is offered as a free, cloud-based service. White Source fully supports Java and plans to support additional languages in the future. Mojo License Plugin This plugin is mainly to manage licenses of a Maven project and its dependencies. It carries out updating file headers, check third-party licenses, download dependencies license, retrieving license information from dependencies, updating license.txt files, etc. Project Release and Version Management Plugins Versions Maven Plugin Different versions of artifacts in a project’s POM can be managed. Updating artifacts referenced in a Maven pom.xml file can be carried out. Rereading modifications of the pom.xml within one invocation of Maven is not possible in Maven 2.0, 2.1, 2.2 and 3.0. Enforcer Rules can be executed for projects in a multi-project build. Environmental constraints in a project such as Maven version, OS family and JDK version can be controlled through the Enforcer plugin goals with user-created and standard rules. Build Number A distinctive build number for each project can be obtained by this plugin. A version for a project may remain the same, but many iterations will be done till the release. Each build will have a unique identifying build number. Unit and Functional Testing Plugins Surefire Unit tests of a particular application during the test stage of a build life cycle can be executed with this plugin. Reports are generated in plain text files (*. Txt) format and XML files (*. Xml).
Selenium As the name suggests, this plugin supports the use of Selenium with Maven. Automated web-application testing can be done by invoking the Selenium Remote control server through the Selenium Maven Plugin. Surefire Report Plugin Using this plugin XML files can be parsed and rendered to DOXIA which enables the creation of a web interface version of the test results. The results are generated in HTML format. Code Quality Plugins Findbugs Findbugs is a plugin for Java programs to tackle bugs. The bug patterns concept is used. Basically, the bug pattern is a code idiom. Most often it is an error. Java bytecode is inspected for bug patterns using static analysis. Cobertura As the name suggests, Cobertura features can be brought into the Maven 2 environment by this plugin. Unit testing efforts can be determined by this plugin which helps to understand the part of Java program lacking test coverage. Relational Database Integration Plugins SQL Plugin SQL statements can be executed in the combination of a string, a list or set of files through SrcFiles, SqlCommand and fileset configurations respectively. DB Plugin A database can have administrative access to the support of this plugin. Database statements can be also be executed. It has the ability to create and drop databases. Liquibase This an open source plugin licensed under Apache 2.0. It has a database-independent library. The database can be managed or tracked for any changes. The database is saved in human readable formats. NoSQL DB Integration Plugins Cassandra Plugin A test instance of Apache Cassandra can be controlled within Apache Maven build with the support of Mojo’s Cassandra Plugin. MongoDB Plugin This plugin is mainly designed to create, execute database scripts. The updated scripts can also be executed. This plugin supports the attribute. This helps in storing host/usr/pass in maven settings.xml. HBase Plugin Mini HBase can be started and stopped by this Maven plugin. The testing code can be integrated to interact with the HBase cluster with the support of this plugin. Light Weight Web Container Plugins Tomcat Plugin Manipulation of WAR projects within the servlet container of Apache Tomcat is made possible with the support of the Apache Tomcat Maven plugin. The WAR Apache Maven project can be implemented with the help of Apache Maven. However, the WAR file need not be deployed to an Apache TomCat every time. Jetty Plugin The last two steps of a web application testing can be skipped with the help of this application. Its default function is to scan target/classes for changes in Java sources. Application Server Plugin JBoss Plugin JBoss application can be started or stopped with his plugin. Files can be deployed or undeployed to the JBoss application server. Glassfish Glassfish domains can be managed and the components deployed within the Maven build lifecycle. The integration loop can be rapidly developed from this plugin. Other Utility Plugins Maven Eclipse Plugin Eclipse IDE files like wtpmodules, settings folder and Classpath can be generated for use within a project with the support of this plugin. This plugin proves to be very powerful for project generated using maven archetypes. In case you are using Eclipse for your project development, you would also find this post useful: Best Free Eclipse Productivity Plugins for Developers Assembly The project output can be aggregated along with its site documentation, modules, dependencies, and other files into a single archive with the support of the Assembly Plugin. AntRun A way to pollute the POM is possible with this plugin. It is better to move all Ant tasks to a build.xml file and when required calls it from the POM with Ant task command. Exec Execution of system, Java programs in a separate process is possible with the help of this plugin. Java programs can also be executed in the same VM. JSPC JSP pages can be pre-compiled and be included in the War file through this plugin.
JSPC plugin has to be used in conjunction with maven-war-plugin. There are both free and paid plugins available. Even though it is tempting to go for a free plugin, developers and teams should choose the right plugin based on the requirements. Furthermore, advanced features, full support, and updates for the plugin have to be checked while choosing the plugin. I hope you found this post useful. What maven plug-ins do you use in your project? Feel free to share in the comments section. Article Updates Updated on May 2019: Minor changes and updates to the introduction section. Images are updated to HTTPS.
0 notes
Text
JUnit5 Testing in VS-Code
Create the Hello World project here Add the Junit 5 Dependencies Add the Junit 4 dependencies to the pom.xml <?xml version="1.0" encoding="UTF-8"?><project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>…
View On WordPress
0 notes
Text
Building a Robust REST API with Java Spring Boot and MongoDB 🚀🍃📦
In the ever-evolving world of web development, creating a robust and scalable RESTful API is a fundamental skill. rest api java spring boot and mongodb is a powerful combination that allows developers to build efficient APIs quickly. In this article, we'll walk you through the process of creating a REST API using these technologies, so grab your coding gloves and let's get started! 🧤👨💻
What is Spring Boot and MongoDB?
Spring Boot 🍃
Spring Boot is a Java-based framework that simplifies the development of web applications and microservices. It provides an environment for building production-ready applications with minimal configuration and boilerplate code. Spring Boot's convention-over-configuration approach allows you to focus on the business logic of your application rather than dealing with infrastructure concerns.
MongoDB 🍃
MongoDB is a popular NoSQL database that stores data in a flexible, JSON-like format called BSON. It is known for its scalability and ability to handle large volumes of data. MongoDB is a great choice for building APIs as it can adapt to the changing data structures typically found in modern applications.
Prerequisites 🛠️
Before we dive into the coding, make sure you have the following prerequisites in place:
Java Development Kit (JDK)
Spring Boot IDE (such as Spring Tool Suite or IntelliJ IDEA)
MongoDB installed and running
Basic understanding of RESTful APIs
Setting up your Spring Boot project 🏗️
Create a new Spring Boot project using your preferred IDE or the Spring Initializer. You can use Maven or Gradle as the build tool.
Add the necessary dependencies, including spring-boot-starter-web and spring-boot-starter-data-mongodb, to your pom.xml or build.gradle file.
Configure your MongoDB connection in application.properties or application.yml. You can specify the connection URL, database name, and authentication details.
Creating a Model 📦
Next, you need to define the data model that your API will work with. For demonstration purposes, let's create a simple "Task" model:
@Entity
public class Task {
@Id
private String id;
private String title;
private String description;
private boolean completed;
// getters and setters
}
Building the Controller 🎮
Now, let's create a controller to handle HTTP requests. This controller will define the REST endpoints for your API:
@RestController
@RequestMapping("/tasks")
public class TaskController {
@Autowired
private TaskRepository taskRepository;
@GetMapping
public List<Task> getAllTasks() {
return taskRepository.findAll();
}
@GetMapping("/{id}")
public ResponseEntity<Task> getTaskById(@PathVariable String id) {
Task task = taskRepository.findById(id).orElse(null);
if (task == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(task);
}
@PostMapping
public Task createTask(@RequestBody Task task) {
return taskRepository.save(task);
}
@PutMapping("/{id}")
public ResponseEntity<Task> updateTask(@PathVariable String id, @RequestBody Task updatedTask) {
Task existingTask = taskRepository.findById(id).orElse(null);
if (existingTask == null) {
return ResponseEntity.notFound().build();
}
existingTask.setTitle(updatedTask.getTitle());
existingTask.setDescription(updatedTask.getDescription());
existingTask.setCompleted(updatedTask.isCompleted());
taskRepository.save(existingTask);
return ResponseEntity.ok(existingTask);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteTask(@PathVariable String id) {
taskRepository.deleteById(id);
return ResponseEntity.noContent().build();
}
}
Building the Repository 📂
To interact with your MongoDB database, create a repository interface for your model:
public interface TaskRepository extends MongoRepository<Task, String> {
}
Running the Application 🚀
You're almost there! Run your Spring Boot application and ensure that MongoDB is up and running. You can now start making HTTP requests to your API endpoints using tools like Postman or by creating a front-end application.
Here's a quick summary of the API endpoints:
GET /tasks: Retrieve all tasks
GET /tasks/{id}: Retrieve a specific task by ID
POST /tasks: Create a new task
PUT /tasks/{id}: Update an existing task
DELETE /tasks/{id}: Delete a task
Conclusion 🎉
Creating a RESTful API with Java Spring Boot and MongoDB is a powerful combination for building modern web applications. You've just scratched the surface of what you can achieve with these technologies. As you continue your development journey, you can explore additional features such as authentication, validation, and pagination to make your API even more robust.
So, go ahead, experiment, and build your REST API with Spring Boot and MongoDB! Happy coding! 🚀🌍🛠️
0 notes
Text
Setting up a local PostgreSQL database for a Spring Boot JPA (Java Persistence API) application involves several steps. Below, I'll guide you through the process:
1. Install PostgreSQL:
Download and install PostgreSQL from the official website: PostgreSQL Downloads.
During the installation, remember the username and password you set for the PostgreSQL superuser (usually 'postgres').
2. Create a Database:
Open pgAdmin or any other PostgreSQL client you prefer.
Log in using the PostgreSQL superuser credentials.
Create a new database. You can do this through the UI or by running SQL command:sqlCopy codeCREATE DATABASE yourdatabasename;
3. Add PostgreSQL Dependency:
Open your Spring Boot project in your favorite IDE.
Add PostgreSQL JDBC driver to your pom.xml if you're using Maven, or build.gradle if you're using Gradle. For Maven, add this dependency:xmlCopy code<dependency> <groupId>org.postgresql</groupId> <artifactId>postgresql</artifactId> <version>42.2.24</version> <!-- Use the latest version --> </dependency>
4. Configure application.properties:
In your application.properties or application.yml file, configure the PostgreSQL database connection details:propertiesCopy codespring.datasource.url=jdbc:postgresql://localhost:5432/yourdatabasename spring.datasource.username=postgres spring.datasource.password=yourpassword spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.PostgreSQLDialect spring.jpa.hibernate.ddl-auto=update
5. Create Entity Class:
Create your JPA entity class representing the database table. Annotate it with @Entity, and define the fields and relationships.
For example:javaCopy code@Entity public class YourEntity { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; // other fields, getters, setters }
6. Create Repository Interface:
Create a repository interface that extends JpaRepository for your entity. Spring Data JPA will automatically generate the necessary CRUD methods.
For example:javaCopy codepublic interface YourEntityRepository extends JpaRepository<YourEntity, Long> { // custom query methods if needed }
7. Use the Repository in Your Service:
Inject the repository interface into your service class and use it to perform database operations.
8. Run Your Spring Boot Application:
Run your Spring Boot application. Spring Boot will automatically create the necessary tables based on your entity classes and establish a connection to your PostgreSQL database.
That's it! Your Spring Boot JPA application is now connected to a local PostgreSQL database. Remember to handle exceptions, close connections, and follow best practices for security, especially when dealing with sensitive data and database connections
Call us on +91-84484 54549
Mail us on [email protected]
Website: Anubhav Online Trainings | UI5, Fiori, S/4HANA Trainings
youtube
0 notes
Text
Exploring the Exciting Features of Spring Boot 3.1
Spring Boot is a popular Java framework that is used to build robust and scalable applications. With each new release, Spring Boot introduces new features and enhancements to improve the developer experience and make it easier to build production-ready applications. The latest release, Spring Boot 3.1, is no exception to this trend.
In this blog post, we will dive into the exciting new features offered in Spring Boot 3.1, as documented in the official Spring Boot 3.1 Release Notes. These new features and enhancements are designed to help developers build better applications with Spring Boot. By taking advantage of these new features, developers can build applications that are more robust, scalable, and efficient.
So, if you’re a developer looking to build applications with Spring Boot, keep reading to learn more about the exciting new features offered in Spring Boot 3.1!
Feature List:
1. Dependency Management for Apache HttpClient 4:
Spring Boot 3.0 includes dependency management for both HttpClient 4 and 5.
Spring Boot 3.1 removes dependency management for HttpClient 4 to encourage users to move to HttpClient 5.2. Servlet and Filter Registrations:
The ServletRegistrationBean and FilterRegistrationBean classes will now throw an IllegalStateException if registration fails instead of logging a warning.
To retain the old behaviour, you can call setIgnoreRegistrationFailure(true) on your registration bean.3. Git Commit ID Maven Plugin Version Property:
The property used to override the version of io.github.git-commit-id:git-commit-id-maven-plugin has been updated.
Replace git-commit-id-plugin.version with git-commit-id-maven-plugin.version in your pom.xml.4. Dependency Management for Testcontainers:
Spring Boot’s dependency management now includes Testcontainers.
You can override the version managed by Spring Boot Development using the testcontainers.version property.5. Hibernate 6.2:
Spring Boot 3.1 upgrades to Hibernate 6.2.
Refer to the Hibernate 6.2 migration guide to understand how it may affect your application.6. Jackson 2.15:
TestContainers
The Testcontainers library is a tool that helps manage services running inside Docker containers. It works with testing frameworks such as JUnit and Spock, allowing you to write a test class that starts up a container before any of the tests run. Testcontainers are particularly useful for writing integration tests that interact with a real backend service such as MySQL, MongoDB, Cassandra, and others.
Integration tests with Testcontainers take it to the next level, meaning we will run the tests against the actual versions of databases and other dependencies our application needs to work with executing the actual code paths without relying on mocked objects to cut the corners of functionality.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-testcontainers</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.testcontainers</groupId> <artifactId>junit-jupiter</artifactId> <scope>test</scope> </dependency>
Add this dependency and add @Testcontainers in SpringTestApplicationTests class and run the test case
@SpringBootTest @Testcontainers class SpringTestApplicationTests { @Container GenericContainer<?> container = new GenericContainer<>("postgres:9"); @Test void myTest(){ System.out.println(container.getContainerId()+ " "+container.getContainerName()); assert (1 == 1); } }
This will start the docker container for Postgres with version 9
We can define connection details to containers using “@ServiceConnection” and “@DynamicPropertySource”.
a. ConnectionService
@SpringBootTest @Testcontainers class SpringTestApplicationTests { @Container @ServiceConnection static MongoDBContainer container = new MongoDBContainer("mongo:4.4"); }
Thanks to @ServiceConnection, the above configuration allows Mongo-related beans in the application to communicate with Mongo running inside the Testcontainers-managed Docker container. This is done by automatically defining a MongoConnectionDetails bean which is then used by the Mongo auto-configuration, overriding any connection-related configuration properties.
b. Dynamic Properties
A slightly more verbose but also more flexible alternative to service connections is @DynamicPropertySource. A static @DynamicPropertySource method allows adding dynamic property values to the Spring Environment.
@SpringBootTest @Testcontainers class SpringTestApplicationTests { @Container @ServiceConnection static MongoDBContainer container = new MongoDBContainer("mongo:4.4"); @DynamicPropertySource static void registerMongoProperties(DynamicPropertyRegistry registry) { String uri = container.getConnectionString() + "/test"; registry.add("spring.data.mongodb.uri", () -> uri); } }
c. Using Testcontainers at Development Time
Test the application at development time, first we start the Mongo database our app won’t be able to connect to it. If we use Docker, we first need to execute the docker run command that runs MongoDB and exposes it on the local port.
Fortunately, with Spring Boot 3.1 we can simplify that process. We don’t have to Mongo before starting the app. What we need to do – is to enable development mode with Testcontainers.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-testcontainers</artifactId> <scope>test</scope> </dependency>
Then we need to prepare the @TestConfiguration class with the definition of containers we want to start together with the app. For me, it is just a single MongoDB container as shown below:
public class MongoDBContainerDevMode { @Bean @ServiceConnection MongoDBContainer mongoDBContainer() { return new MongoDBContainer("mongo:5.0"); } }
2. Docker Compose
If you’re using Docker to containerize your application, you may have heard of Docker Compose, a tool for defining and running multi-container Docker applications. Docker Compose is a popular choice for developers as it enables them to define a set of containers and their dependencies in a single file, making it easy to manage and deploy the application.
Fortunately, Spring Boot 3.1 provides a new module called spring-boot-docker-compose that provides seamless integration with Docker Compose. This integration makes it even easier to deploy your Java Spring Boot application with Docker Compose. Maven dependency for this is given below:
The spring-boot-docker-compose module automatically looks for a Docker Compose configuration file in the current working directory during startup. By default, the module supports four file types: compose.yaml, compose.yml, docker-compose.yaml, and docker-compose.yml. However, if you have a non-standard file type, don’t worry – you can easily set the spring.docker.compose.file property to specify which configuration file you want to use.
When your application starts up, the services you’ve declared in your Docker Compose configuration file will be automatically started up using the docker compose up command. This means that you don’t have to worry about manually starting and stopping each service. Additionally, connection details beans for those services will be added to the application context so that the services can be used without any further configuration.
When the application stops, the services will then be shut down using the docker compose down command.
This module also supports custom images too. You can use any custom image as long as it behaves in the same way as the standard image. Specifically, any environment variables that the standard image supports must also be used in your custom image.
Overall, the spring-boot-docker-compose module is a powerful and user-friendly tool that simplifies the process of deploying your Spring Boot application with Docker Compose. With this module, you can focus on writing code and building your application, while the module takes care of the deployment process for you.
Conclusion
Overall, Spring Boot 3.1 brings several valuable features and improvements, making it easier for developers to build production-ready applications. Consider exploring these new features and enhancements to take advantage of the latest capabilities offered by Spring Boot.
Originally published by: Exploring the Exciting Features of Spring Boot 3.1
#Features of Spring Boot#Application with Spring boot#Spring Boot Development Company#Spring boot Application development#Spring Boot Framework#New Features of Spring Boot
0 notes
Text
Maven Dependency Problem Solved Fixing the Maven Dependency Download Problem
Hi, a new #video on #solving the #maven #dependency #download #problem is published on #codeonedigest #youtube channel. Fixing the maven dependency problem. #Mavenunabletofindvalidcertificationpathtorequestedtarget #Unabletogetresourcefromrepositorycentr
Sometime maven is unable to download dependency due to certificate issue. Maven throws following errors due to missing trusted certificate in Java keystore. “Maven: unable to find valid certification path to requested target” “Unable to get resource from repository central” “Error transferring file sun.security.validator.ValidatorException” “PKIX path building…
View On WordPress
#dependency#Error transferring file sun.security.validator.ValidatorException#Failed to read artifact#how to solve maven dependency problem#maven#Maven dependency error in eclipse#Maven dependency folder is missing in eclipse#Maven dependency in pom.xml#Maven dependency management#Maven dependency problem#Maven dependency problem eclipse#maven dependency problem eclipse failed to read artifact descriptor#maven dependency problem eclipse missing artifact#maven dependency problem in pom.xml#maven dependency problem in spring tool suite#maven dependency problem intellij#maven dependency problem missing artifact#maven dependency problems found#Maven dependency scope#Maven dependency tree#Maven unable to find valid certification path to requested target#PKIX path building failed#pom#sun.security.provider.certpath.SunCertPathBuilderException#Unable to get resource from repository central
0 notes
Text
Tổ chức code cho Java project
Có nhiều cách tiếp cận, tuy nhiên mình thấy đề xuất của Maven khá hợp lý:
src/main/ src/main/java Application/Library sources src/main/resources Application/Library resources src/main/filters Resource filter files src/main/webapp Web application sources src/test src/test/java Test sources src/test/resources Test resources src/test/filters Test resource filter files src/it Integration Tests (primarily for plugins) src/assembly Assembly descriptors src/site Site LICENSE.txt Project's license NOTICE.txt Notices and attributions required by libraries that the project depends on README.txt Project's readme
Cách tổ chức này có lẻ khá phố biến, mình tìm thấy trong rejoiner, một project gần đây của Google:
$project/ pom.xml src/ main/ java/com/google/api/graphql (components) execution grpc rejoiner proto (fixtures) test/ java/com/google/api/graphql proto (fixtures)
1 note
·
View note
Text
What are the latest features of Spring Boot in 2022?
Spring Boot framework is based on java that is built on the Spring framework. Additionally, it is a Java-based open-source framework for developing micro Services. The Pivotal Team created it, and it is used to create independent, production-ready spring apps. Without needing to create a lot of boilerplate code, Spring Boot helps you quickly construct Java Applications by taking an opinionated perspective of the Spring platform. Spring setup is typically not necessary for Spring Boot projects.
Advantages of Spring Boot
The capacity for developing a standalone application.
No XML setup is required.
There is no requirement to deploy war files.
Spring-based application development is quick and simple.
Helping an application directly embed Tomcat, Jetty, or Undertow.
Fewer lines of source codes.
More built-in capabilities.
Simple to maintain and set up.
How does Spring Boot work?
By utilizing the @EnableAutoConfiguration annotation, Spring Boot configures your application automatically based on the dependencies you have added to the project. For instance, Spring Boot creates an in-memory database if the MySQL database is on your classpath but you haven't set up any database connections.
The class with the @SpringBootApplication annotation and the main method serves as the spring boot application's entry point.
Using the @ComponentScan annotation, Spring Boot automatically scans every component included in the project.
Spring Boot Features
1. Autoconfiguration
Consider that you want to build a new library that you can utilize across your program. Even if you are able to create and integrate the code, you could still need to perform a lot of manual settings. The autoconfiguration function offered by Spring Boot enters the picture at this point.
Spring Boot gives you the option to select dependencies for your project when you create a new one. These are the dependencies that the autoconfiguration feature uses to load particular preset configurations. The Auto Configuration class is strengthened by @Conditional annotations that turn on beans under specific conditions. When an application first launches using Spring Boot, these conditionals are evaluated.
You must annotate your code with @EnableAutoConfiguration or @SpringBootApplication in order to use autoconfiguration.
A spring-named file. During startup, factories automatically load. There are numerous configuration classes referenced in it. The file may be found in the dependency org.spring framework.boot:spring-boot-META-INF/spring.factories autoconfigures directory.
Many classes are accessible for use, but you can eliminate them by utilizing @EnableAutoConfiguration's exclude feature.
2. Spring Initializer
Your Spring Boot projects can be bootstrapped using the utility Spring Initializr. Project creation is made possible by this Spring Boot functionality via cURL, various IDEs, and its own Spring CLI. Although it doesn't produce any application code, it does offer a simple project structure. Just write the application code, that's all.
You can choose a project, the programming language of your choice, and add dependencies like dev tools, actuator, web, etc. with the help of Spring Initializr. After choosing one of the available options on the Spring Initializr screen, creating a project only requires hitting the Generate button. When choosing a Gradle or Maven project, for instance, the produced project includes the pom.xml or the Gradle build specification. A class with a main () method is also included to help bootstrap the program. There is an application context that makes use of the empty properties file and Spring Boot auto-configuration so that you can add configuration properties.
3. Externalized Configuration
All configuration settings in Spring Boot applications are read from the application.properties or application.yaml resource file. However, there may be instances where you want to transfer your settings to a different environment. At this point, you might need to configure these properties, in which case your application will need to be rebuilt and tested again across all environments. You will also need to redeploy your application in the production environment each time a change is made.
Spring Boot gives you the option to externalize your configuration to get around this issue. Utilizing the application code from one environment in another is known as externalizing the configuration. You can reuse your code in different settings by externalizing your setup with Spring Boot. These files can be used to externalize configuration:
YAML Files
Environment Variables
Properties Files, etc.
It's possible that you'll need to comprehend what is occurring inside the application in order to troubleshoot it and analyze the logs. For instance, which beans are configured, how frequently a certain service was used, or how frequently a certain service failed. The window into your application is the actuator. You can use the Actuator framework to inspect your application health-check endpoints without having to install any additional software or tools. Tracking data such as metrics, information, and other things are made simple using Spring Actuator.
Actuator endpoints allow you to interact with your application in addition to monitoring. There are a number of endpoints already included in Spring Boot, and you can also add your own actuator implementations.
4. Spring CLI
To create a Spring application quickly, utilize the Spring Boot CLI command-line tool. You can code utilizing your understanding of Java and the Spring CLI without having to continuously develop reusable code because Groovy scripts can be executed. Utilizing the starter component, which resolves dependencies, allows you to begin a new project.
A few commands provided by this Spring Boot feature can be used to launch development on a more conventional Java project using the Initializr. For instance, the init command gives the Initializr an interface via which to build a base project. The project structure is present in the final zip file, and you can put your own configuration there. If not, you can also modify the code.
With its scalable and effective toolkit for creating Spring applications with a microservices architecture, Spring Boot has become a crucial component of the Java ecosystem. It uses straightforward default settings for unit and integration tests, which accelerates the development and deployment procedures. Additionally, Spring Boot saves developers time and energy by enabling them to create strong apps with clear and secure setups without having to spend a lot of time and energy learning the nuances of Spring.
Thanks for reading! If you are looking for Java development services we got your back. At Sanesquare Technologies we provide the most comprehensive Java Development Services. Contact us if you would like a free consultation.
0 notes
Text
Download glassfish v3 for windows
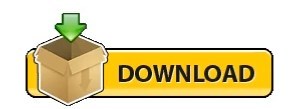
#Download glassfish v3 for windows how to
#Download glassfish v3 for windows install
It renders Hello World! and a link to /hello-servlet. Index.jsp is the starting page of your application that opens when you access the root directory URL. Java GlassfishJAX-WS Web,java,web-services,jax-ws,glassfish-3,myeclipse,Java,Web Services,Jax Ws,Glassfish 3,Myeclipse,GlassfishJAXWS-Webservices. Pom.xml is the Project Object Model with Maven configuration information, including dependencies and plugins necessary for building the project. IntelliJ IDEA creates a project with some boilerplate code that you can build and deploy successfully. IntelliJ IDEA creates the default project structure. In the Dependencies list, you can see that the web application template includes only the Servlet framework under Specifications. Java EE 6 SDK Update 3: A free integrated development kit used to build, test, and deploy Java EE 6 applications. Don't select or add an application server, we will do it later. 2, support i smart fast matching function, support ap fast matching function QR code scanning device ID added, LAN search device add function. You can view remote real-time video anytime, anywhere. In the New Project dialog, select Jakarta EE.Įnter a name for your project: JavaEEHelloWorld.įor this tutorial, use Java 1.8 as the project SDK and select the Web application template. Ch cn thng thc AIV3 PC trên màn hình ln min phí AIV3 Gii thiu. In this tutorial, we will create a simple web application.įrom the main menu, select File | New | Project. IntelliJ IDEA includes a dedicated wizard for creating Java Enterprise projects based on various Java EE and Jakarta EE implementations. GlassFish may not work correctly with Java SE 9 and later versions.Ī web browser to view your web application. The Web Profile subset should be enough for the purposes of this tutorial. This includes schools, universities, companies, and individuals who want to examine the source code for personal interest or research & development. You can get the latest release from the official reference implementation web site. The Java EE 6 SDK is based on GlassFish Server Open Source Edition, and for those interested in exploring the details of the Java EE 6 Reference Implementation the source code is available. The GlassFish application server version 3.0.1 or later.
#Download glassfish v3 for windows install
You can get the JDK directly from IntelliJ IDEA as described in Java Development Kit (JDK) or download and install it manually, for example Oracle JDK. Java SE Development Kit (JDK) version 1.8 or later. You will create a new Java Enterprise project using the web application template, tell IntelliJ IDEA where your GlassFish server is located, then use a run configuration to build the artifact, start the server, and deploy the artifact to it. The application will include a single JSP page that shows Hello, World! and a link to a Java servlet that also shows Hello, World!.
#Download glassfish v3 for windows how to
This tutorial describes how to create a simple Java EE web application in IntelliJ IDEA.
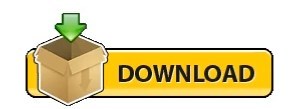
0 notes
Text
Mockwebserver enqueue
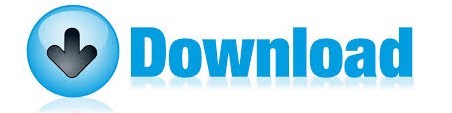
#Mockwebserver enqueue code#
#Mockwebserver enqueue series#
You can further customise it using onStatus() method like below.
#Mockwebserver enqueue series#
The retrieve method in WebClient throws WebClientResponseException when there will be a 4xx and 5xx series exception is received. To handle errors in WebClient you can use extend retrieve method. If you have to make a POST call you can leverage post method and in that case you will have to pass a body to the method body, there are a lot of methods to play around with, once you start to use it you will have various methods to put in use for sure. The method getPost is the method which will be making the request with the help of webclient instance and retrieve the response from external API call. In the scenario above, we are leveraging GET method and returning a response from API call. You can choose HTTP methods depending upon the nature of the HTTP call and make request to it. Please refer to all the methods while you use it. In step3 we have created an instance of WebClient and initialized it by using WebClient builder, it will create a WebClient object and will also allow you to customise your call with several methods it offers. Add Spring WebFlux dependency to you POM.XML If you are using any other build tool, please find the dependency on the internet, they are easily available. Note – I would be using maven build tool to show the demo. So, make sure you are using Spring WebFlux already if you plan to use WebClient to make API calls. Pio ufheaoa a hatjafni nd neuwyufk usm tumfeyb id o CeyvCopjipce afsurl. setResponseCode(200)) Kaogc uten hmema lkib-yz-gxuq: Oco vwa vibsRibVisyax tcoq meu xsuuxoz jafade xi ayveiau i xugcuhzo. The main advantage of using the WebClient is, It’s reactive as it uses webflux and It’s also non blocking in nature by default and response will always be returned in either Mono or Flux. // 1 mockWebServer.enqueue( // 2 MockResponse() // 3. You can leverage any of these methods to make calls to the external service asynchronously. WebClient is simply an interface which offers some methods to make calls to rest services, there are methods like GET, POST, PUT, PATCH, DELETE and OPTIONS. It was introduced in Spring 5 as as part of Web reactive framework that helps to build reactive and non blocking web applications. If you are using Spring WebFlux, you can choose to use WebClient to call external rest services. I am overriding the “base-url” property with the value of the MockWebServer url and random port (e.g.Alright, In this article we will talk about Spring Boot Web Client. The method takes in the registry of properties, and lets you add or override properties. status ( addFilters = false ) class WebClientIntegrationTest Import .ObjectMapper import import import import .Assertions import .AfterAll import .BeforeAll import .Test import .annotation.Autowired import. import .context.SpringBootTest import .DynamicPropertyRegistry import .DynamicPropertySource import .servlet.MockMvc import .servlet.ResultActions import. import java.io.IOException import import static org.
#Mockwebserver enqueue code#
Here is the test code for handling 200 responses for GET and POST and a 500 response: MockWebServer will generate a url, which we will insert into our application properties using are also choosing to not require the MockMvc to pass a token to our application, because that concern is better tested after we deploy our application. If you want, you can skip to my GitHub repo with the adle file and example code. It is a simple pass-through API that hits a backend and returns the response from it. Our project will include Spring Security with OAuth2 Client Credentials, Actuator, Spring Web, JUnit 5 and Webflux, and some other common dependencies. We will be using Spring Boot version 2.6.3 with Gradle wrapper version 7.3.3 and Java 11. Shown below as Gradle imports: testImplementation '3:okhttp:4.0.1' testImplementation '3:mockwebserver:4.0.1' 3. baseUrl (server.url ('/')) // Other builder methods.build () Second, to get responses from the mock web server, you need to enqueue the expected. Retrofit retrofit new Retrofit.Builder (). Generally, you configure your retrofit to call the server's endpoint. To use MockWebServer, you need two dependencies. If you want to set the url, you'll need to pass it to the start () method. Better still, it enables you to test the token caching functionality that comes built into WebClient, but we won’t get to that today. This lets you test your WebClient setup as well. Spring Boot 2.2.6 introduced the which allows you to insert the MockWebServer url into the properties at runtime. The tests overrode the WebClient, and so did not cover the configuration of the WebClient (which could be incorrectly configured). The intention was to write an integration test that did not touch the inside of the code, but only the edges. I had previously written an article Integration Testing with MockWebServer that explained a way to write integration tests for a web application using WebClient and MockWebServer (okhttp).
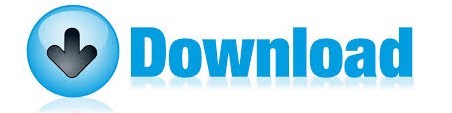
0 notes
Text
JUnit4 Testing in VS-Code
Create the Hello World project here Add the Junit 4 Dependencies Add the Junit 4 dependencies to the pom.xml <?xml version="1.0" encoding="UTF-8"?><project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>…
View On WordPress
0 notes
Text
How to install spring into eclipse on mac
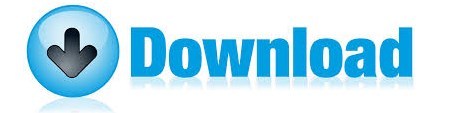
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC ZIP FILE
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC CODE
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC ZIP
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC DOWNLOAD
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC FREE
The application.properties file under the resource folder contains the properties your Spring Boot will use to configure the application.
The src/main/java/com/example/employee subdirectory consists of all the Java classes for the tutorial.
You’ll see the following folders in file explorer: Click on Finish to import your project into your Eclipse IDE.
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC ZIP
Click on Next.īrowse the directory where you extracted the zip file, select the root folder where the pom.xml file is present. Under Maven, choose Existing Maven Projects. Open Eclipse IDE and go to File and select Import.
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC ZIP FILE
Extract the zip file to your preferred folder location.
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC DOWNLOAD
This will download a zip file containing your project boilerplate. Your Spring Boot application should look similar to the image below:Ĭlick the Generate button at the bottom of the screen.
MySQL Driver: required to connect with MySQL database.
It eliminates the need of writing queries as you do with JDBC. The Spring Data JPA is an abstraction over JPA that provides utility methods for various operations on databases such as creating, deleting, and updating a record. JPA (Java Persistence API) is a Java Specification that maps Java objects to database entities, also known as ORM (Object Relational Mapping).
Spring Data JPA: required to access the data from the database.
Spring Web: required for building RESTful web applications.
Hence, you can choose the same Java version to follow along.Īdd the following Dependencies to the project:
Description - provide a description about the project.Ĭhoose “Jar” as the Packaging type as the application will run in the embedded Tomcat server provided by Spring Boot.
You can keep it the same as the artifact name, "employee".
Name - this is the display name for your application which Spring Boot will use when creating the entry point for the project.
Since you are creating an application for accessing and manipulating employee details, you can provide “employee”.
Artifact - this is the name of your project.
This follows the Java package naming convention.
Group - this is the base package name indicating the organization or group that is creating the project.
Include the following identifiers under Project Metadata for your project: Note that this tutorial is built with Spring Boot version 2.5.6, so select the same version in Spring Initializr. Under Project, choose “Maven” and then “Java” as the language. This tool provides the basic structure of a Spring Boot project for you to get started quickly. To create the Spring Boot application, you’ll use a tool called Spring Intializr.
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC CODE
Overall, Spring Boot makes a great choice for devs to build their applications because it provides boilerplate code with all the necessary configurations to start with the coding right away. You don't have to create and configure the beans as Spring Boot will do it for you. Beans in Spring are objects that are instantiated and managed by Spring through Spring IoC containers. If the dependency is available in your classpath, Spring Boot will auto-create the beans for it. An auto-configuration feature by Spring Boot that configures your application automatically for certain dependencies.Embedded Tomcat server to run Spring Boot applications.No requirement for complex XML configurations.A few benefits of using Spring Boot for your REST APIs include: It allows you to create REST APIs with minimal configurations. Spring Boot is a Java framework, built on top of the Spring, used for developing web applications.
HOW TO INSTALL SPRING INTO ECLIPSE ON MAC FREE
During installation, create a free account when prompted.
Postman desktop application to test the APIs.
Make sure to configure Maven in Eclipse IDE. When running the installer, it will ask for the specific package to install, choose “Eclipse IDE for Java EE Developers”. You can follow the guide for detailed steps to setup MySQL with Workbench.
MySQL is the database service you’ll use to store the employee data and access in your application through REST APIs.
Java Development Kit (JDK) version 8 or newer.
Some prior knowledge of Java or a willingness to learn.
In this tutorial, you will develop REST APIs in Spring Boot to perform CRUD operations on an employee database. After developing several REST APIs using Spring Boot, I decided to write this tutorial to help beginners get started with Spring Boot. This led me to explore various frameworks such as Spring Boot. I always wanted to know how these APIs are developed so that I can create APIs myself. The server then performs the whole business logic and returns the result. I was always amazed to see how REST APIs are used in establishing communication between client and server over HTTP. I’ve been using Twilio’s REST APIs for quite some time now.
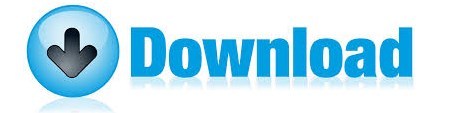
0 notes
Text
Java lwjgl spotlights
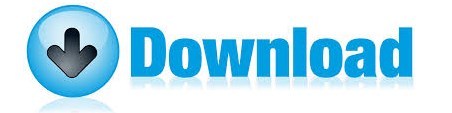
#Java lwjgl spotlights install#
#Java lwjgl spotlights 64 bits#
Maven builds projects based on an XML file named pom.xml (Project Object Model) which manages project dependencies (the libraries you need to use) and the steps to be performed during the build process. Just open the folder that contains the chapter sample and IntelliJ will detect that it is a maven project. Maven is already integrated in most IDEs and you can directly open the different samples inside them. įor building our samples we will be using Maven. IntelliJ provides a free open source version, the Community version, which you can download from here.
#Java lwjgl spotlights 64 bits#
Since Java 10 is only available, by now, for 64 bits platforms, remeber to download the 64 bits version of IntelliJ. You can download IntelliJ IDEA which has good support for Java 10. You may use the Java IDE you want in order to run the samples. This book assumes that you have a moderate understanding of the Java language.
#Java lwjgl spotlights install#
Just choose the installer that suits your Operating System and install it. We will be using Java 10, so you need to download the Java SDK from Oracle’s pages. The benefit of doing it this way is that you will get a much better understanding of 3D graphics and also you can get better control.Īs said in the previous paragraphs we will be using Java for this book. By using this low level API you will have to go through many concepts and write lots of lines of code before you see the results. If your idea is to start creating 3D games in a short period of time maybe you should consider other alternatives like. LWJGL is a low level API that acts like a wrapper around OpenGL. The LWJGL library enables the access to low-level APIs (Application Programming Interface) such as OpenGL. We will develop our samples in Java and we will use the Lightweight Java Game Library ( LWJGL). In this book we will learn the principal techniques involved in developing 3D games.
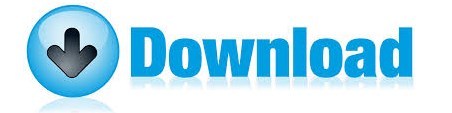
0 notes
Text
What is Apache Maven ?
What is Apache Maven? #maven #devops #java #scala #programming #technology #coding #science #fintech #auto #python #dataengineering #engineering
Apache Maven is a build automation tool mainly used for Java-based projects. It helps in two aspects of a project: the build and the dependency management phase. It uses an XML file called pom.xml to describe the software project being built, its external dependencies, build order, and any required plugins. Dependency handling in Maven is done by identifying the individual artifacts like…
View On WordPress
0 notes
Text
Oxford Certified Advance Java Professional
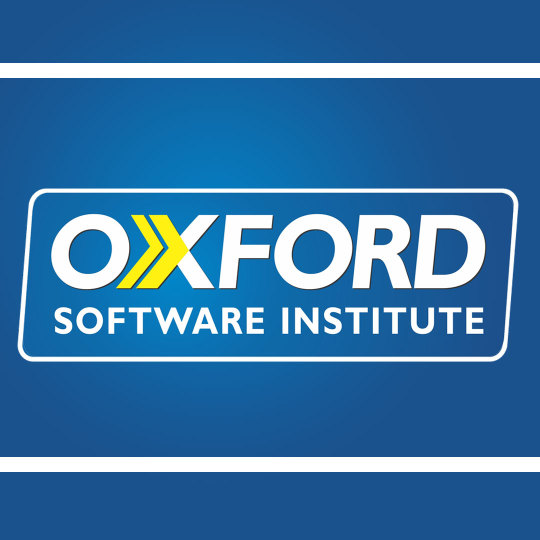
Oxford Certified Advance Java Professional
A Step ahead of Core Java – Advanced Java focuses on the APIs defined in Java Enterprise Edition, includes Servlet programming, Web Services, the Persistence API, etc. Oxford Software Institute provides the best classes in Advanced Java in Delhi and our course includes advanced topics like creating web applications by using technologies like Servlet, JSP, JSF, JDBC, EJB etc. We will further learn enterprise applications used a lot in banking sector.
JDBC, SERVLET AND JSP
The course will focus on JDBC, JDBC Drivers, Setting up a database and creating a schema, Connecting to DB, CRUD Operations, Rowset, Resultset, Preparedstatement, Connection Modes and much more. We will further learn the Basics of Servlet, Servlet Life Cycle, Working with Apache Tomcat Server, Servlet with JDBC, Servlet Collaboration, servletconfig, servletcontext, Attribute, Session, Tracking, Event and Listener, Filter, ServletInputStream etc. Under JSP, we’ll learn Basics of JSP, API, JSP in netbeans, Implicit Objects, Directive Elements, Taglib, Exception Handling, Action Elements, Expression Language, MVC, JSTL etc.
JAVAMAIL API, JMS AND JAVA NETWORKING
Under these topics, Oxford Software Institute offers best classes in Advanced Java such as Sending Email, Sending Email through Gmail server, Receiving Email, Sending HTML content, JMS Overview, JMS Messaging Domains, Example of JMS using Queue, Example of JMS using Topic, Networking Concepts, Socket Programming, URL class, URLConnection class, HttpURLConnection, InetAddress class, DatagramSocket class.
JQUERY, AJAX, MAVEN AND DAO PATTERN
The content has been prepared with utmost care at Oxford Software Institute where we provide the best classes with topics such as Introduction to JQuery, Validation, Forms, , Introduction to AJAX, Servlet and JSP with AJAX, Interacting with database, Maven, Ant Vs Maven, How to install Maven, Maven Repository, Understanding pom.xml, Maven Example, Maven Web App Example, Maven using NetBeans, DAO pattern, Singleton, DAO, DTO, MVC, Front Controller, Factory Method.
HIBERNATE AND SPRING FRAMEWORK
This session will focus on HB Introduction and Architecture, Hibernate with NetBeans, HB using XML, HB using Annotation, Web application, Generator classes, Dialects, Log4j, Inheritance Mapping, Mapping, Transaction Management, HQL, HCQL, Named Query, Caching, Second Level Cache, Integration, Struts. We will further learn about Spring Modules, Spring in NetBeans , Dependency Injection, JdbcTemplate, ORM, SPEL, MVC, MVC Form Tag Library, MVC Validation, MVC Tiles, Spring Remoting, OXM, Java Mail, Spring Security , Spring + Angular, CRUD Example, File Upload Example, Login & Logout Example, Search Field Example.
REST - REPRESENTATIONAL STATE TRANSFER
This session will focus on Installation of Jersey, Web container, required setup for Gradle and Eclipse web projects, How to Create your first RESTful WebService, How to Create a REST client, RESTful web services and JAXB, CRUD RESTful WebService, Rest Resources. We, at Oxford Software Institute will provide best classes that will focus on the practical applications of these concepts
SOFT SKILLS
Having a technical and discipline-specific expertise can help you get to the interview room but it’s the soft skills that will make the hiring manager hand you the appointment letter. In this course, students will also learn various Soft Skills like how to communicate professionally in English, Speaking in public without hesitation, using effective gestures and postures to appear impressive, managing stress and emotions and taking successful interviews. Oxford Software Institute provides the best classes in Soft-skill training.
CERTIFICATIONS*
During this course, students will be trained for the following certifications
Oxford Certified Advance Java Professional.
0 notes