#the C 'static' keyword
Explore tagged Tumblr posts
Text
I am the world's #1 dynamic programming language hater. Give me static or give me death.
#static scheduling#static typing#static analysis#static storage#the C 'static' keyword#static web pages#i beg of thee all these things#my thoughts#programming#well thunks and closures can be okay#but im going to be eyeing them with suspicion the whole time
12 notes
·
View notes
Note
How DOES the C preprocessor create two generations of completely asinine programmers??
oh man hahah oh maaan. ok, this won't be very approachable.
i don't recall what point i was trying to make with the whole "two generations" part but ill take this opportunity to justifiably hate on the preprocessor, holy fuck the amount of damage it has caused on software is immeasurable, if you ever thought computer programmers were smart people on principle...
the cpp:
there are like forty preprocessor directives, and they all inject a truly mind-boggling amount of vicious design problems and have done so for longer than ive been alive. there really only ever needed to be one: #include , if only to save you the trouble of manually having to copy header files in full & paste them at the top of your code. and christ almighty, we couldn't even get that right. C (c89) has way, waaaay fewer keywords than any other language. theres like 30, and half of those aren't ever used, have no meaning or impact in the 21st century (shit like "register" and "auto"). and C programmers still fail to understand all of them properly, specifically "static" (used in a global context) which marks some symbol as inelligible to be touched externally (e.g. you can't use "extern" to access it). the whole fucking point of static is to make #include'd headers rational, to have a clear seperation between external, intended-to-be-accessed API symbols, and internal, opaque shit. nobody bothers. it's all there, out in the open, if you #include something, you get all of it, and brother, this is only the beginning, you also get all of its preprocessor garbage.
this is where the hell begins:
#if #else
hey, do these look familiar? we already fucking have if/else. do you know what is hard to understand? perfectly minimally written if/else logic, in long functions. do you know what is nearly impossible to understand? poorly written if/else rats nests (which is what you find 99% of the time). do you know what is completely impossible to understand? that same poorly-written procedural if/else rat's nest code that itself is is subject to another higher-order if/else logic.
it's important to remember that the cpp is a glorified search/replace. in all it's terrifying glory it fucking looks to be turing complete, hell, im sure the C++ preprocessor is turing complete, the irony of this shouldn't be lost on you. if you have some long if/else logic you're trying to understand, that itself is is subject to cpp #if/#else, the logical step would be to run the cpp and get the output pure C and work from there, do you know how to do that? you open the gcc or llvm/clang man page, and your tty session's mem usage quadruples. great job idiot. trying figuring out how to do that in the following eight thousand pages. and even if you do, you're going to be running the #includes, and your output "pure C" file (bereft of cpp logic) is going to be like 40k lines. lol.
the worst is yet to come:
#define #ifdef #ifndef (<- WTF) #undef you can define shit. you can define "anything". you can pick a name, whatever, and you can "define it". full stop. "#define foo". or, you can give it a value: "#define foo 1". and of course, you can define it as a function: "#define foo(x) return x". wow. xzibit would be proud. you dog, we heard you wanted to kill yourself, so we put a programming language in your programming language.
the function-defines are pretty lol purely in concept. when you find them in the wild, they will always look something like this:
#define foo(x,y) \ (((x << y)) * (x))
i've seen up to seven parens in a row. why? because since cpp is, again, just a fucking find&replace, you never think about operator precedence and that leads to hilarious antipaterns like the classic
#define min(x,y) a < b ? a : b
which will just stick "a < b ? a: b" ternary statement wherever min(.. is used. just raw text replacement. it never works. you always get bitten by operator precedence.
the absolute worst is just the bare defines:
#define NO_ASN1 #define POSIX_SUPPORTED #define NO_POSIX
etc. etc. how could this be worse? first of all, what the fuck are any of these things. did they exist before? they do now. what are they defined as? probably just "1" internally, but that isn't the point, the philosophy here is the problem. back in reality, in C, you can't just do something like "x = 0;" out of nowhere, because you've never declared x. you've never given it a type. similar, you can't read its value, you'll get a similar compiler error. but cpp macros just suddenly exist, until they suddenly don't. ifdef? ifndef? (if not defined). no matter what, every permutation of these will have a "valid answer" and will run without problem. let me demonstrate how this fucks things up.
do you remember "heartbleed" ? the "big" openssl vulnerability ? probably about a decade ago now. i'm choosing this one specifically, since, for some reason, it was the first in an annoying trend for vulns to be given catchy nicknames, slick websites, logos, cable news coverage, etc. even though it was only a moderate vulnerability in the grand scheme of things...
(holy shit, libssl has had huge numbers of remote root vulns in the past, which is way fucking worse, heartbleed only gave you a random sampling of a tiny bit of internal memory, only after heavy ticking -- and nowadays, god, some of the chinese bluetooth shit would make your eyeballs explode if you saw it; a popular bt RF PHY chip can be hijacked and somehow made to rewrite some uefi ROMs and even, i think, the microcode on some intel chips)
anyways, heartbleed, yeah, so it's a great example since you could blame it two-fold on the cpp. it involved a generic bounds-checking failure, buf underflow, standard shit, but that wasn't due to carelessness (don't get me wrong, libssl is some of the worst code in existence) but because the flawed cpp logic resulted in code that:
A.) was de-facto worthless in definition B.) a combination of code supporting ancient crap. i'm older than most of you, and heartbleed happened early in my undergrad. the related legacy support code in question hadn't been relevant since clinton was in office.
to summarize, it had to do with DTLS heartbeats. DTLS involves handling TLS (or SSLv3, as it was then, in the 90s) only over UDP. that is how old we're talking. and this code was compiled into libssl in the early 2010s -- when TLS had been the standard for a while. TLS (unlike SSLv3 & predecessors) runs over TCP only. having "DTLS heartbeat support in TLS does not make sense by definition. it is like drawing a triangle on a piece of paper whose angles don't add up to 180.
how the fuck did that happen? the preprocessor.
why the fuck was code from last century ending up compiled in? who else but!! the fucking preprocessor. some shit like:
#ifndef TCP_SUPPORT <some crap related to UDP heartbeats> #endif ... #ifndef NO_UDP_ONLY <some TCP specific crap> #endif
the header responsible for defining these macros wasn't included, so the answer to BOTH of these "if not defined" blocks is true! because they were never defined!! do you see?
you don't have to trust my worldview on this. have you ever tried to compile some code that uses autoconf/automake as a build system? do you know what every single person i've spoken to refers to these as? autohell, for automatic hell. autohell lives and dies on cpp macros, and you can see firsthand how well that works. almost all my C code has the following compile process:
"$ make". done. Makefile length: 20 lines.
the worst i've ever deviated was having a configure script (probably 40 lines) that had to be rune before make. what about autohell? jesus, these days most autohell-cursed code does all their shit in a huge meta-wrapper bash script (autogen.sh), but short of that, if you decode the forty fucking page INSTALL doc, you end up with:
$ automake (fails, some shit like "AUTOMAKE_1.13 or higher is required) $ autoconf (fails, some shit like "AUTOMCONF_1.12 or lower is required) $ aclocal (fails, ???) $ libtoolize (doesn't fail, but screws up the tree in a way that not even a `make clean` fixes $ ???????? (pull hair out, google) $ autoreconf -i (the magic word) $ ./configure (takes eighty minutes and generates GBs of intermediaries) $ make (runs in 2 seconds)
in conclusion: roflcopter
⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯⎯ disclaimer | private policy | unsubscribe
159 notes
·
View notes
Text
Re-Learning C# Studies #1 and #2
Tuesday 09 May 2023
Yes starting from the beginning again! I actually started yesterday night but forgot to post about it! So, this is a combined post! Yesterday I made my study plan (I used ChatGPT to make the plan since I'm lazy~) and I started with Day 1 and 2 which focuses on "Basic Syntax and Data Types"~!
Basic Syntax
Just learnt the complete basics of any programming language; it's syntax. I already know this. I am pretty confident in this. It's understanding things like the keywords such as using, namespace, class and static void!
Data Types
Another sub-topic I already know a lot in is the C# data types! In C# you have to declare the type the data will be before using it - unlike languages like JavaScript, Lua and Python where you can just declare and the program will automatically assign the datatype based on the value. Declaring data types in C# is beneficial because it provides more clarity and specificity in the code. I think it helps catch errors quicker as well~!
That's all I learnt in the last 2 days! Really happy I got to go over the basics again! Here are the resources that I used:
Codecademy - link
Software Testing Help - link
TutorialsTeacher - link
Thanks for reading and happy coding! 🥰👍🏾💗
#codeblr#coding#studyblr#studying#progblr#programming#comp sci#computer science#tech#code#learn to code#csharp#csharp study#dotnet
56 notes
·
View notes
Text
The Future of Web Development: Trends, Techniques, and Tools
Web development is a dynamic field that is continually evolving to meet the demands of an increasingly digital world. With businesses relying more on online presence and user experience becoming a priority, web developers must stay abreast of the latest trends, technologies, and best practices. In this blog, we’ll delve into the current landscape of web development, explore emerging trends and tools, and discuss best practices to ensure successful web projects.
Understanding Web Development
Web development involves the creation and maintenance of websites and web applications. It encompasses a variety of tasks, including front-end development (what users see and interact with) and back-end development (the server-side that powers the application). A successful web project requires a blend of design, programming, and usability skills, with a focus on delivering a seamless user experience.
Key Trends in Web Development
Progressive Web Apps (PWAs): PWAs are web applications that provide a native app-like experience within the browser. They offer benefits like offline access, push notifications, and fast loading times. By leveraging modern web capabilities, PWAs enhance user engagement and can lead to higher conversion rates.
Single Page Applications (SPAs): SPAs load a single HTML page and dynamically update content as users interact with the app. This approach reduces page load times and provides a smoother experience. Frameworks like React, Angular, and Vue.js have made developing SPAs easier, allowing developers to create responsive and efficient applications.
Responsive Web Design: With the increasing use of mobile devices, responsive design has become essential. Websites must adapt to various screen sizes and orientations to ensure a consistent user experience. CSS frameworks like Bootstrap and Foundation help developers create fluid, responsive layouts quickly.
Voice Search Optimization: As voice-activated devices like Amazon Alexa and Google Home gain popularity, optimizing websites for voice search is crucial. This involves focusing on natural language processing and long-tail keywords, as users tend to speak in full sentences rather than typing short phrases.
Artificial Intelligence (AI) and Machine Learning: AI is transforming web development by enabling personalized user experiences and smarter applications. Chatbots, for instance, can provide instant customer support, while AI-driven analytics tools help developers understand user behavior and optimize websites accordingly.
Emerging Technologies in Web Development
JAMstack Architecture: JAMstack (JavaScript, APIs, Markup) is a modern web development architecture that decouples the front end from the back end. This approach enhances performance, security, and scalability by serving static content and fetching dynamic content through APIs.
WebAssembly (Wasm): WebAssembly allows developers to run high-performance code on the web. It opens the door for languages like C, C++, and Rust to be used for web applications, enabling complex computations and graphics rendering that were previously difficult to achieve in a browser.
Serverless Computing: Serverless architecture allows developers to build and run applications without managing server infrastructure. Platforms like AWS Lambda and Azure Functions enable developers to focus on writing code while the cloud provider handles scaling and maintenance, resulting in more efficient workflows.
Static Site Generators (SSGs): SSGs like Gatsby and Next.js allow developers to build fast and secure static websites. By pre-rendering pages at build time, SSGs improve performance and enhance SEO, making them ideal for blogs, portfolios, and documentation sites.
API-First Development: This approach prioritizes building APIs before developing the front end. API-first development ensures that various components of an application can communicate effectively and allows for easier integration with third-party services.
Best Practices for Successful Web Development
Focus on User Experience (UX): Prioritizing user experience is essential for any web project. Conduct user research to understand your audience's needs, create wireframes, and test prototypes to ensure your design is intuitive and engaging.
Emphasize Accessibility: Making your website accessible to all users, including those with disabilities, is a fundamental aspect of web development. Adhere to the Web Content Accessibility Guidelines (WCAG) by using semantic HTML, providing alt text for images, and ensuring keyboard navigation is possible.
Optimize Performance: Website performance significantly impacts user satisfaction and SEO. Optimize images, minify CSS and JavaScript, and leverage browser caching to ensure fast loading times. Tools like Google PageSpeed Insights can help identify areas for improvement.
Implement Security Best Practices: Security is paramount in web development. Use HTTPS to encrypt data, implement secure authentication methods, and validate user input to protect against vulnerabilities. Regularly update dependencies to guard against known exploits.
Stay Current with Technology: The web development landscape is constantly changing. Stay informed about the latest trends, tools, and technologies by participating in online courses, attending webinars, and engaging with the developer community. Continuous learning is crucial to maintaining relevance in this field.
Essential Tools for Web Development
Version Control Systems: Git is an essential tool for managing code changes and collaboration among developers. Platforms like GitHub and GitLab facilitate version control and provide features for issue tracking and code reviews.
Development Frameworks: Frameworks like React, Angular, and Vue.js streamline the development process by providing pre-built components and structures. For back-end development, frameworks like Express.js and Django can speed up the creation of server-side applications.
Content Management Systems (CMS): CMS platforms like WordPress, Joomla, and Drupal enable developers to create and manage websites easily. They offer flexibility and scalability, making it simple to update content without requiring extensive coding knowledge.
Design Tools: Tools like Figma, Sketch, and Adobe XD help designers create user interfaces and prototypes. These tools facilitate collaboration between designers and developers, ensuring that the final product aligns with the initial vision.
Analytics and Monitoring Tools: Google Analytics, Hotjar, and other analytics tools provide insights into user behavior, allowing developers to assess the effectiveness of their websites. Monitoring tools can alert developers to issues such as downtime or performance degradation.
Conclusion
Web development is a rapidly evolving field that requires a blend of creativity, technical skills, and a user-centric approach. By understanding the latest trends and technologies, adhering to best practices, and leveraging essential tools, developers can create engaging and effective web experiences. As we look to the future, those who embrace innovation and prioritize user experience will be best positioned for success in the competitive world of web development. Whether you are a seasoned developer or just starting, staying informed and adaptable is key to thriving in this dynamic landscape.
more about details :- https://fabvancesolutions.com/
#fabvancesolutions#digitalagency#digitalmarketingservices#graphic design#startup#ecommerce#branding#marketing#digitalstrategy#googleimagesmarketing
2 notes
·
View notes
Text
i think i a m about to go insane
why would i use the static keyword for a function when i can just declare it in the .c file for a .h file and just like... not declare it in the .h file???????? Like, no other translation unit outside of that .c is going to be able to access it. Is it like to be explicit???????? waht the fuck????????
2 notes
·
View notes
Text
What are the important topics in the C++ language?
C++ Introduction
C++ Basics
Features
Installation
cout, cin, end
Variable
Data types
Keywords
Operators
Identifiers
Expression
3. Control statement
If-else and it's type
Switch
Loop
Entry control
Exit control
Break statement
Continue statement
Goto statement
Comment statement
4. Function
Call by values and call by reference
Recursion
Storage classes
5. Array
Simple array
Multidimensional array
6. Pointer basic
Pointers
Refrences
Memory management
7. Object and Class
Object oriented programme introduction and benifites and concept
Object class
Constructor
Destructor
Static
Structure
Enumeration
Friend function
Math function
8. Inheritance
9. Polymorphism
10. Abstraction
11. Namespace
12. Strings
13. Exception
Exception handling
Try/catch
User defined
14. Templates
15. Signal handling
16. File and Stream
File and stream
getline()
17. int to string
18. STL (Standard Template Library)
STL components
Vector
Deque
List
Set
Stack
Queue
Priority queue
Map
Multimap
Betset
Algorithm
And many more
19. Iterators and it's concept
20. After above can go through to C++11 and C++17
2 notes
·
View notes
Text
Mastering Data Science Using Python
Data Science is not just a buzzword; it's the backbone of modern decision-making and innovation. If you're looking to step into this exciting field, Data Science using Python is a fantastic place to start. Python, with its simplicity and vast libraries, has become the go-to programming language for aspiring data scientists. Let’s explore everything you need to know to get started with Data Science using Python and take your skills to the next level.
What is Data Science?
In simple terms, Data Science is all about extracting meaningful insights from data. These insights help businesses make smarter decisions, predict trends, and even shape new innovations. Data Science involves various stages, including:
Data Collection
Data Cleaning
Data Analysis
Data Visualization
Machine Learning
Why Choose Python for Data Science?
Python is the heart of Data Science for several compelling reasons:
Ease of Learning: Python’s syntax is intuitive and beginner-friendly, making it ideal for those new to programming.
Versatile Libraries: Libraries like Pandas, NumPy, Matplotlib, and Scikit-learn make Python a powerhouse for data manipulation, analysis, and machine learning.
Community Support: With a vast and active community, you’ll always find solutions to challenges you face.
Integration: Python integrates seamlessly with other technologies, enabling smooth workflows.
Getting Started with Data Science Using Python
1. Set Up Your Python Environment
To begin, install Python on your system. Use tools like Anaconda, which comes preloaded with essential libraries for Data Science.
Once installed, launch Jupyter Notebook, an interactive environment for coding and visualizing data.
2. Learn the Basics of Python
Before diving into Data Science, get comfortable with Python basics:
Variables and Data Types
Control Structures (loops and conditionals)
Functions and Modules
File Handling
You can explore free resources or take a Python for Beginners course to grasp these fundamentals.
3. Libraries Essential for Data Science
Python’s true power lies in its libraries. Here are the must-know ones:
a) NumPy
NumPy is your go-to for numerical computations. It handles large datasets and supports multi-dimensional arrays.
Common Use Cases: Mathematical operations, linear algebra, random sampling.
Keywords to Highlight: NumPy for Data Science, NumPy Arrays, Data Manipulation in Python.
b) Pandas
Pandas simplifies working with structured data like tables. It’s perfect for data manipulation and analysis.
Key Features: DataFrames, filtering, and merging datasets.
Top Keywords: Pandas for Beginners, DataFrame Operations, Pandas Tutorial.
c) Matplotlib and Seaborn
For data visualization, Matplotlib and Seaborn are unbeatable.
Matplotlib: For creating static, animated, or interactive visualizations.
Seaborn: For aesthetically pleasing statistical plots.
Keywords to Use: Data Visualization with Python, Seaborn vs. Matplotlib, Python Graphs.
d) Scikit-learn
Scikit-learn is the go-to library for machine learning, offering tools for classification, regression, and clustering.
Steps to Implement Data Science Projects
Step 1: Data Collection
You can collect data from sources like web APIs, web scraping, or public datasets available on platforms like Kaggle.
Step 2: Data Cleaning
Raw data is often messy. Use Python to clean and preprocess it.
Remove duplicates and missing values using Pandas.
Normalize or scale data for analysis.
Step 3: Exploratory Data Analysis (EDA)
EDA involves understanding the dataset and finding patterns.
Use Pandas for descriptive statistics.
Visualize data using Matplotlib or Seaborn.
Step 4: Build Machine Learning Models
With Scikit-learn, you can train machine learning models to make predictions. Start with simple algorithms like:
Linear Regression
Logistic Regression
Decision Trees
Step 5: Data Visualization
Communicating results is critical in Data Science. Create impactful visuals that tell a story.
Use Case: Visualizing sales trends over time.
Best Practices for Data Science Using Python
1. Document Your Code
Always write comments and document your work to ensure your code is understandable.
2. Practice Regularly
Consistent practice on platforms like Kaggle or HackerRank helps sharpen your skills.
3. Stay Updated
Follow Python communities and blogs to stay updated on the latest tools and trends.
Top Resources to Learn Data Science Using Python
1. Online Courses
Platforms like Udemy, Coursera, and edX offer excellent Data Science courses.
Recommended Course: "Data Science with Python - Beginner to Pro" on Udemy.
2. Books
Books like "Python for Data Analysis" by Wes McKinney are excellent resources.
Keywords: Best Books for Data Science, Python Analysis Books, Data Science Guides.
3. Practice Platforms
Kaggle for hands-on projects.
HackerRank for Python coding challenges.
Career Opportunities in Data Science
Data Science offers lucrative career options, including roles like:
Data Analyst
Machine Learning Engineer
Business Intelligence Analyst
Data Scientist
How to Stand Out in Data Science
1. Build a Portfolio
Showcase projects on platforms like GitHub to demonstrate your skills.
2. Earn Certifications
Certifications like Google Data Analytics Professional Certificate or IBM Data Science Professional Certificate add credibility to your resume.
Conclusion
Learning Data Science using Python can open doors to exciting opportunities and career growth. Python's simplicity and powerful libraries make it an ideal choice for beginners and professionals alike. With consistent effort and the right resources, you can master this skill and stand out in the competitive field of Data Science.
0 notes
Text
Amazon Marketing Strategy 2024: Trends, Tips & Examples
Introduction to Amazon Marketing
With over 300 million active customers, Amazon has fast become the marketplace where businesses come alive. Right at the outset of the new year 2024, an integrated Amazon marketing Strategy is more crucial than ever. Any business looking to sell and increase its visibility and even scale up a successful business on the platform will make all the difference in the world by learning the latest trends, tools, and strategies.

Why Amazon is Crucial for Your Business in 2024
Continued dominance has made Amazon an e-commerce giant. The platform accounted for nearly 40% of all eCommerce sales in the United States during 2023. As more users opt for their product purchases online, the influence of Amazon will continue to increase. Businesses need to integrate features that advanced marketing tools on Amazon DSP, sponsored products, and AI-driven features will make available for them to stay afloat and thrive through 2024.
Top Amazon Marketing Trends for 2024
A. Rise of AI-Driven Advertising
Now, with the advent of AI, the manner in which marketing on Amazon is approached has changed. Campaigns can now be optimized using real-time data about customer behaviour to predict how a customer will behave. This kind of predictive algorithm will allow for more users to be targeted with highly personalized ads, thereby increasing conversions.
B. Voice Search Optimization
With increasing applications of voice-activated assistants like Amazon Alexa, optimization for voice search becomes a prime requirement. With consumers using more long-tail keywords and natural language queries to search for products, it offers you an opportunity to capture that traffic if you fine-tune your product listings appropriately.
C. Increased Focus on Amazon DSP
Amazon DSP has become very important. This can run display advertisements on Amazon-owned sites and third parties. It is a highly targeted approach that lets you reach better with more engagement, making it one of the must-have tools for 2024.
D. Video Ads on Amazon
Video marketing is more on the rise than ever, and so too is Amazon. Video ads now occupy a more important place in showing up within search results, product pages, and related product listings. Video content is far more engaging and helps communicate your brand message so much better than static images.
E. Amazon Live for Real-Time Engagement
Amazon Live turns out to be a game-changer for brands that want to target the customer at a real-time level. Live streaming experiences enable brands to present products and answer questions in real-time while driving real-time conversions.
Amazon Marketing Strategies to Implement
A. Optimize Product Listings for 2024
As more and more sellers join, more need there is for optimizing product listings. Quality images and descriptions are important, but so is keyword optimization. Another space is the certain guarantee that all of your listings are mobile-friendly because mobile shopping is having such an impact on performance.
B. Mastering Amazon SEO
Thus, Amazon SEO must rank better on your search results. Ensure that you optimize the titles, bullet points, and product descriptions using suitable keywords. Be sure to use backend keywords, which may help increase your chances of search visibility. Therefore, the more optimized the listing is, the more a customer will view it.
C. Utilizing Sponsored Products and Brands
Sponsored Products and Sponsored Brands enable you to drive even more sales through promoted listings, whereas Sponsored Brands show a portfolio of products along with your customizable headline, which brings big traffic and conversions.
D. Leveraging Customer Reviews for Social Proof
Social proof is, to date, one of the best sales drivers on Amazon. Get happy customers to write reviews; they increase the worthiness of your product. Respond to both positive and negative reviews as that shows you respect customers’ opinions, and this goes a long way in building brand loyalty.
E. Competitor Analysis and Pricing Strategies
Competitor analysis keeps you ahead. It tracks competitor’s prices, promotions, and product descriptions. Dynamically adjust your prices based on the activities of your competitors to win the Buy Box, selling more and getting more visibility.

Real-World Examples of Winning Amazon Marketing Campaigns
A. Case Study 1: Driving Sales with Amazon DSP
A skincare brand used DSP on Amazon to target high-intent shoppers with display ads across Amazon and third-party sites. This resulted in 20% more sales and improved customer retention.
B. Case Study 2: Boosting Brand Visibility with Video Ads
A fitness equipment company utilized the video ads feature of Amazon to bring people closer to the features and benefits of the items. With engaging content with videos, the visibility went up by 35% while the conversions improved by 25%.
C. Case Study 3: Optimizing for Voice Search and Long-Tail Keywords
An electronics company optimized its product listings with long-tail keywords that suit voice searches. This led to a 30% hike in organic traffic and better conversion rates for the customers because they could easily find the products using voice search queries.
Tips for a Successful Amazon Marketing Strategy in 2024
Stay Updated on Algorithm Changes: Amazon updates its A9 algorithm frequently and impacts product ranking. Keep yourself updated and change your listings accordingly.
Invest in AI Tools: AI tools can help provide insights and automate processes to optimize ad spending and increase ROI.
Leverage Amazon DSP: Reaches a greater audience through highly targeted ads.
Emphasize Customer Experience: Fast shipping, reliable customer support, and positive reviews all will increase the level of customer satisfaction.
Focus on Mobile Optimization: More and more customers have begun shopping on mobile so product listings and ads should be mobile-friendly.
How CoTask IT Solutions Can Help You Succeed
CoTask IT Solutions offers full Amazon marketing services aimed to make you successful on the platform. Product listing optimization, advanced campaign management, and many more, you are going to see a customized strategy with CoTask IT Solutions that will be able to maintain your visibility and increase sales on Amazon. We use cutting-edge tools and proven strategies to ensure your business is ahead of the curve in 2024.
Conclusion
Amazon remains one of the primary channels for business growth, filled with many marketing tools and untapped opportunities to connect with more consumers. Keeping up with innovation- with AI-powered advertising, and voice search optimization, Amazon DSP will help businesses optimize strategy and pave the road to success. With the correct tactics, Amazon can be a fantastic driver of sales and brand awareness.
Contact us today for a free consultation and start driving sales and boosting brand awareness!
Original Source: https://cotasks.com/amazon-marketing-strategy-2024-trends-tips-examples/
0 notes
Photo
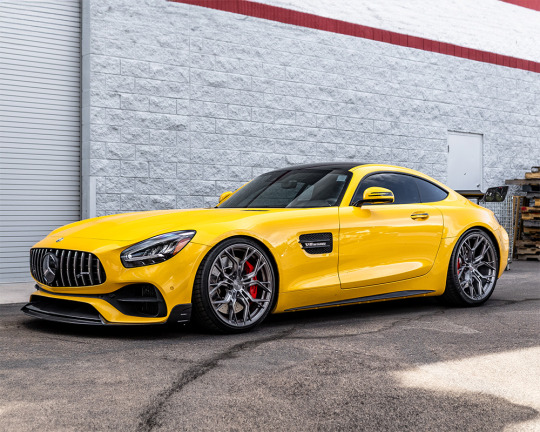
New Post has been published on https://www.vividracing.com/blog/mercedes-amg-gt-c-yellow-on-tinted-brushed-vr-forged-d05-wheels/
Mercedes AMG GT-C Yellow on Tinted Brushed VR Forged D05 Wheels
The Mercedes AMG GT is the German version of the Dodge Viper. Its long hood hiding the 4.0L Bi-Turbo V8 engine that produces nearly 600 horsepower makes this an underrated sports coupe IMO. These cars are super capable of crushing at the track. The AMG GT-R did the Nurburgring in 7 minutes and 10 seconds whereas the AMG GT Black Series crushed times at 6 minutes 43 seconds. Having been in production since 2016, the AMG has gone through some changes and is available in different variants. Like all wheels, going with a lighter weight forged monoblock wheel will help improve acceleration as you save 3x in static weight over stock wheels. But of course, upgrading wheels dramatically improves the look of the car.
This 2019 Mercedes AMG GT-C features a set of our VR Forged D05 wheels. The front wheels are a 20×9.5 +50mm weighing 11.1kg and the rear are 21×11.5 +45mm weighing 12.6kg. For tires we went with a 245/35/20 and 325/25/21. The wheels have been finished in a dark tinted brush and clear.
If you want to get a custom set of VR Forged D05 wheels, shop here! https://www.vividracing.com/index.php?keywords=vr+forged+d05
0 notes
Text
Which topic are taught under c++ course
A C++ course typically covers a range of topics designed to introduce students to the fundamentals of programming, as well as more advanced concepts specific to C++. Here's a breakdown of common topics:
1. Introduction to Programming Concepts
Basic Programming Concepts: Variables, data types, operators, expressions.
Input/Output: Using cin, cout, file handling.
Control Structures: Conditional statements (if, else, switch), loops (for, while, do-while).
2. C++ Syntax and Structure
Functions: Definition, declaration, parameters, return types, recursion.
Scope and Lifetime: Local and global variables, static variables.
Preprocessor Directives: #include, #define, macros.
3. Data Structures
Arrays: Single and multi-dimensional arrays, array manipulation.
Strings: C-style strings, string handling functions, the std::string class.
Pointers: Pointer basics, pointer arithmetic, pointers to functions, pointers and arrays.
Dynamic Memory Allocation: new and delete operators, dynamic arrays.
4. Object-Oriented Programming (OOP)
Classes and Objects: Definition, instantiation, access specifiers, member functions.
Constructors and Destructors: Initialization of objects, object cleanup.
Inheritance: Base and derived classes, single and multiple inheritance, access control.
Polymorphism: Function overloading, operator overloading, virtual functions, abstract classes, and interfaces.
Encapsulation: Use of private and public members, getter and setter functions.
Friend Functions and Classes: Use and purpose of friend keyword.
5. Advanced Topics
Templates: Function templates, class templates, template specialization.
Standard Template Library (STL): Vectors, lists, maps, sets, iterators, algorithms.
Exception Handling: try, catch, throw, custom exceptions.
File Handling: Reading from and writing to files using fstream, handling binary files.
Namespaces: Creating and using namespaces, the std namespace.
6. Memory Management
Dynamic Allocation: Managing memory with new and delete.
Smart Pointers: std::unique_ptr, std::shared_ptr, std::weak_ptr.
Memory Leaks and Debugging: Identifying and preventing memory leaks.
7. Algorithms and Problem Solving
Searching and Sorting Algorithms: Linear search, binary search, bubble sort, selection sort, insertion sort.
Recursion: Concepts and use-cases of recursive algorithms.
Data Structures: Linked lists, stacks, queues, trees, and graphs.
8. Multithreading and Concurrency
Thread Basics: Creating and managing threads.
Synchronization: Mutexes, locks, condition variables.
Concurrency Issues: Deadlocks, race conditions.
9. Best Practices and Coding Standards
Code Style: Naming conventions, commenting, formatting.
Optimization Techniques: Efficient coding practices, understanding compiler optimizations.
Design Patterns: Common patterns like Singleton, Factory, Observer.
10. Project Work and Applications
Building Applications: Developing simple to complex applications using C++.
Debugging and Testing: Using debugging tools, writing test cases.
Version Control: Introduction to version control systems like Git.
This comprehensive set of topics equips students with the skills needed to develop efficient, robust, and scalable software using C++. Depending on the course level, additional advanced topics like metaprogramming, networking, or game development might also be covered.
C++ course in chennai
Web designing course in chennai
full stack course in chennai
0 notes
Text
Unleashing the Power of Your Audience: Leveraging User-Generated Content (UGC) to Boost Engagement on Your Affordable Tasmania Website
In today's digital landscape, where attention spans are shorter than ever, standing out from the crowd can be a daunting task for small and medium-sized businesses (SMBs) in Australia. Generic websites simply won't cut it anymore. You need a website that fosters engagement, builds trust, and ultimately drives conversions. This is where User-Generated Content (UGC) comes in.
Affordable web design in Tasmania can be a springboard for leveraging UGC, transforming your website into a dynamic platform that breathes life into your brand. Here's how:
The Allure of User-Generated Content
UGC refers to content created by your customers, fans, or followers. It can take various forms, including:
Reviews and Testimonials: Positive reviews and testimonials from satisfied customers are powerful trust signals. Potential customers in Tasmania are more likely to do business with you if they see others vouching for your products or services.
Social Media Posts: Encourage your audience on platforms like Facebook and Instagram to share photos or videos using your products or services with a specific hashtag. This not only creates a sense of community but also provides valuable visual content for your website.
Blog Comments and Forum Discussions: Foster an interactive environment by encouraging comments on your blog posts or discussions on forums. This allows your audience to share their experiences and insights, adding depth and authenticity to your website.
Customer-Created Content: Run contests or challenges that encourage users to create content related to your brand. This could be anything from recipe videos using your product to travel photos showcasing your travel agency's destinations in Tasmania.
Affordable web design in Tasmania can incorporate dedicated sections on your website specifically designed to showcase UGC. This creates a dynamic and constantly evolving space that keeps visitors engaged.
The Benefits of UGC for Tasmanian Businesses
Integrating UGC into your affordable web design in Tasmania strategy offers a plethora of benefits:
Enhanced Credibility: Genuine customer reviews and experiences hold more weight than self-promotional content. UGC builds trust and establishes your brand as authentic and reliable.
Increased Engagement: UGC fosters a sense of community and encourages user interaction. It goes beyond static content, creating a dynamic platform where users feel like they're part of something bigger.
Cost-Effective Marketing: UGC is essentially free marketing created by your loyal customers. It allows you to leverage the power of word-of-mouth recommendations at no additional cost.
Fresh Content Stream: UGC keeps your website content fresh and dynamic. Engaging user-created photos, videos, and testimonials eliminate the need to constantly generate new content yourself.
Improved SEO: UGC often involves user-generated keywords and local references, which can be beneficial for your website's search engine ranking, especially for searches specific to Tasmania.
Affordable web design in Tasmania can be built with features that make it easy for users to submit content, seamlessly integrating UGC into your website's overall strategy.
Strategies for Implementing UGC on Your Tasmanian Website
Here are some key strategies to leverage UGC effectively on your affordable web design in Tasmania :
Make it Easy to Contribute: Create clear and easy-to-follow guidelines for users to submit content. Integrate buttons or forms on your website that allow seamless sharing of photos, videos, or reviews.
Moderate Wisely: While moderation is essential, avoid being overly restrictive. Focus on filtering out spam or offensive content while allowing genuine user voices to shine through.
Show Appreciation: Publicly acknowledge and thank users who contribute content. This demonstrates your appreciation and encourages continued participation. You can even consider offering small incentives like contests or discounts for valuable UGC contributions.
Promote UGC Across Channels: Don't limit UGC to your website. Share user-generated content on social media platforms, email campaigns, or even print materials. Leverage the power of these voices to expand your reach.
Affordable web design in Tasmania can be complemented with social media integration tools, making it easy to share UGC across different platforms.
Examples of UGC Implementation for Tasmanian Businesses
Here are some specific examples of how Tasmanian businesses can leverage UGC:
Restaurants: Encourage diners to share photos of their meals on Instagram with a branded hashtag.
Accommodation Providers: Showcase guest testimonials and photos of their Tasmanian adventures on your website.
Adventure Tour Companies: Feature user-generated videos of exhilarating activities on your website to inspire potential customers.
Retail Stores: Display customer reviews of products alongside product descriptions to build trust and highlight features.
By thinking creatively and tailoring your approach to your specific industry and target audience, you
Affordable Web Design in Tasmania: Building a UGC-Friendly Foundation
Affordable web design in Tasmania plays a crucial role in successfully implementing a UGC strategy. Here's what to consider:
User-Friendly Interface: A website with a clean, intuitive interface encourages user interaction. Make it easy for visitors to navigate, find relevant information, and understand how to submit UGC.
Visually Appealing Design: An aesthetically pleasing website is more likely to capture attention and encourage users to linger. Consider incorporating UGC seamlessly into your overall design to create a cohesive and engaging experience.
Mobile Responsiveness: With the majority of web browsing happening on mobile devices, ensure your affordable web design in Tasmania is fully responsive and displays flawlessly on all screen sizes. This allows users to easily submit and consume UGC on the go.
Social Media Integration: Integrate social media buttons or feeds into your website. This allows users to share UGC directly from your website to their social media networks, further amplifying its reach.
Content Management System (CMS): Many affordable web design in Tasmania solutions utilize user-friendly CMS platforms like WordPress. These platforms offer functionalities to easily manage UGC submissions, categorize content, and integrate it seamlessly into your website.
By prioritizing these aspects during affordable web design in Tasmania, you create a foundation that fosters user engagement and facilitates a smooth UGC integration process.
Addressing Potential Challenges with UGC
While UGC offers numerous benefits, it's important to be aware of potential challenges:
Maintaining Quality Control: While moderation is crucial, it shouldn't stifle user voices. Develop a clear moderation policy that balances quality control with preserving user authenticity.
Encouraging Participation: Not all users will readily contribute content. Run contests, offer incentives, or simply make it easy and rewarding to participate to encourage user-generated content creation.
Negative Reviews: Negative reviews are inevitable. Address negative feedback professionally and promptly, demonstrating your commitment to customer satisfaction.
By proactively addressing these challenges, you can effectively leverage UGC and turn it into a powerful tool for boosting website engagement and brand reputation.
FAQs on Leveraging UGC for Tasmanian Businesses
Q: How do I ensure user-generated content aligns with my brand image?
A: Develop clear guidelines for UGC submissions. Outline desired content types, preferred tone of voice, and any specific requirements you have to ensure alignment with your brand image.
Q: What are some legal considerations with UGC?
A: It's important to obtain permission from users before featuring their content on your website. Consider having a user-generated content disclaimer on your website outlining your terms and conditions for using UGC submissions.
Q: How much time and effort does it take to manage UGC?
A: The time commitment for UGC management depends on the volume of submissions and your moderation strategy. However, with a well-defined process and the right tools, managing UGC can be a relatively streamlined process.
Conclusion:
Affordable web design in Tasmania can be the springboard for a dynamic and engaging website fueled by user-generated content. By embracing UGC, you tap into the power of your audience, fostering trust, boosting engagement, and ultimately driving conversions. Remember, UGC is a conversation, not a monologue. Encourage user interaction, show appreciation for contributions, and watch your Tasmanian website transform into a thriving community hub.
0 notes
Note
forgive me for the questions & any possible inconsistencies or malformations:
how do we compile our symbolic high/low level code into machine language, and how do we find the most efficient method of conversion from symbolic to machine code?
is it possible to include things from asm in C source code?
why do any of the abstractions in C mean anything? how does the compiler translate them?
would it be possible to write and compile a libc in C on a machine which didn't have a precompiled libc? i think the answer is no, so how much peripheral stuff would you need to pull it off?
are these things i could have figured out by just reading the one compiler book with the dragon on the cover?
thanks and apologies.
these are uhh. these are really good questions. like if you're asking these then i think you know way more than you think you do
how do we compile our symbolic high/low level code into machine language?
we do it the natural (only?) way, using parsers, although a bit more technically involved than just a parser. all programming languages are comprised of just keywords (e.g. int, static, enum, if, etc) and operators(+, -, *, %), those are the only reserved terms. everything between them is an identifier (name of variable, struct, etc), so at some level we can just search for the reserved terms and match them, and match whatever is between them as identifiers (var names or whatever). with this, we can tokenize the raw input put it in the context of a defined grammar.
lex is the name of the program that does this. you have simple, defined keywords, and defined operators, and you group them together a few categories (e.g. int, float, long might all fall under the same "variable qualifier" category"). this is tokenization.
those tokens are given to another program, yacc, which deals with it in the context of some context-free defined grammar you've also provided it. this would be a very strict and unambiguous definition of what C code is, defining things like "qualifiers like int and float always proceed an identifier, the addition (+) operator needs two operands, but the ++ (increment by 1) operator only needs one". yacc processes these tokens in the context of the defined grammar, and since those tokens are defined in broad categories, you can start to converge on a method of generating machine code for them.
how do we find the most efficient method of conversion from symbolic to machine code?
ehehehe. ill save this one for later
is it possible to include things from asm in C source code?
yes:
assembly (which translates directly 1-to-1 to machine code) is the only thing a CPU understands, so there has to be a link between the lowest level programming language and assembly at some point. it works about as you'd expect. that asm() block just gets plopped into the ELF right as it appears in the code. there are some features that allow passing C variables in/out of asm() blocks.
why do any of the abstractions in C mean anything? how does the compiler translate them?
hoping above answers this
would it be possible to write and compile a libc in C on a machine which didn't have a precompiled libc? i think the answer is no, so how much peripheral stuff would you need to pull it off?
yes, and in fact, the litmus test that divides "goofy idea some excited kid thought up and posted all over hacker news" and "real programming language" is whether or not that language is bootstrapped, which is whether or not its compiler is written in "itself". e.g. gcc & clang are both C compilers, and they are written in C. bootstrapping is the process of doing this initially. kind of a chicken-and-egg problem, but you just use external things, other languages, or if it is 1970s, direct assembly itself, to create an initial compiler for that language, and the write a compiler for that language in that language, feed it to the intermediate compiler, and bam.
its really hard to do all of this. really hard. lol
are these things i could have figured out by just reading the one compiler book with the dragon on the cover?
idk which one that is
finally...
how do we find the most efficient method of conversion from symbolic to machine code?
so this is kind of an area of debate lol. starting from the bottom, there are some very simple lines of code that directly map to machine code, e.g
a = b + c;
this would just translate to e.g. sparc
add %L1,%L2,%L3 !
if statements would map to branch instructions, etc, etc. pretty straightforward. but there are higher-order optimizations that modern compilers will do, for example, the compiler might see that you write to a variable but never read from it again, and realize since that memory is never read again, you may as well not even bother writing it in the first place, since it won't matter. and then choose to just not include the now-deemed-unnecessary instructions that store whatever values to memory even though you explicitly wrote it in the source code. some of the times this is fine and yields slightly faster code, but other times it results in the buffer you used for your RSA private key not being bzero'd out and me reading it while wearing a balaclava.
34 notes
·
View notes
Text
what does the static keyword do in C++??
i’m a mechanical engineering student who’s terrified of programming ask me anything about compsci and i’ll answer it wrong
852 notes
·
View notes
Text
Wixpatriots: Revolutionizing Your Online Presence
Welcome to the digital era, "Patriot SEO Agency" where online visibility can make or break a business. In this landscape, Search Engine Optimization (SEO) plays a pivotal role in ensuring your brand stands out amidst the digital noise. Today, let's delve into the world of Wixpatriots, a game-changing SEO agency that goes beyond the ordinary to catapult your business to new heights.
II. Wixpatriots: A Brief Overview
A. Introduction to Wixpatriots
Wixpatriots isn't just another SEO agency; it's a digital partner committed to your success. Founded on the principles of innovation and client satisfaction, Wixpatriots brings a fresh perspective to the SEO game.
B. Unique Approach to SEO
What sets Wixpatriots apart is its unique approach to SEO. It's not just about ranking high on search engines; it's about crafting a digital strategy that aligns with your business goals.
C. Company Values and Mission
Patriot SEO Agency operates on a foundation of integrity, transparency, and excellence. The mission is simple: to empower businesses through strategic SEO solutions tailored to their unique needs.
III. The Power of Effective SEO
A. Enhancing Online Visibility
Wixpatriots understands the importance of being seen in the vast digital landscape. Through meticulous SEO strategies, they ensure your brand gets the attention it deserves.
B. Boosting Website Traffic
It's not just about getting clicks; it's about attracting the right audience. Wixpatriots employs strategies that drive organic traffic, increasing the chances of converting visitors into customers.
C. Improving User Experience
SEO isn't just about keywords; it's about enhancing the overall user experience. Wixpatriots optimizes your website to ensure it's not just search engine-friendly but user-friendly too.
IV. Wixpatriots Services
A. Detailed SEO Analysis
Before crafting a strategy, Patriot SEO Agency conducts a thorough analysis of your current online presence, identifying strengths, weaknesses, and opportunities.
B. On-Page Optimization
From keyword placement to content structure, Wixpatriots fine-tunes every aspect of your website for optimal performance on search engines.
C. Off-Page Strategies
Wixpatriots doesn't stop at your website. They leverage off-page strategies to build your online authority and credibility.
D. Tailored Solutions for Clients
One size doesn't fit all. Wixpatriots understands that each business is unique, and so are its SEO needs. They offer customized solutions that align with your specific goals.
V. The Wixpatriots Difference
A. Customized SEO Plans
Wixpatriots doesn't believe in generic solutions. They tailor their SEO plans to suit your business objectives, ensuring maximum impact.
B. Proven Track Record
Results matter, and Wixpatriots has a proven track record of delivering tangible results for clients across various industries.
C. Client Success Stories
The success of Wixpatriots is mirrored in the success stories of its clients. Real businesses, real results.
VI. The SEO Landscape: Trends and Changes
A. Ever-Evolving SEO Algorithms
SEO isn't static. Patriot SEO Agency stays abreast of the latest algorithm changes, adapting strategies to ensure sustained success.
B. Staying Ahead of the Curve
In the dynamic world of SEO, staying ahead is crucial. Wixpatriots embraces innovation, staying ahead of industry trends.
C. Adapting Strategies for Optimal Results
Change is the only constant. Wixpatriots isn't afraid to adapt its strategies to ensure optimal results for your business.
VII. How Wixpatriots Tackles SEO Challenges
A. Analyzing Industry-Specific Challenges
Every industry comes with its unique challenges. Wixpatriots conducts in-depth research to understand and tackle industry-specific SEO hurdles.
B. Crafting Effective Solutions
Identifying challenges is one thing; crafting effective solutions is another. Wixpatriots doesn't just diagnose; they remedy.
C. Continuous Improvement Mindset
SEO is an ongoing process. Wixpatriots adopts a continuous improvement mindset, ensuring your digital strategy evolves with the changing landscape.
VIII. Wixpatriots Client-Centric Approach
A. Understanding Client Goals
Your success is Wixpatriots' success. They take the time to understand your business goals, aligning their strategies with your vision.
B. Building Long-Term Partnerships
Wixpatriots doesn't aim for quick wins. They're in it for the long haul, building lasting partnerships with their clients.
C. Transparent Communication
No hidden agendas. Wixpatriots believes in transparent communication, keeping you informed and involved throughout the SEO journey.
IX. Case Studies: Wixpatriots Impact
A. Successful SEO Campaigns
Explore real-life examples of how Wixpatriots' SEO campaigns have propelled businesses to the top of search engine rankings.
B. Measurable Results
Numbers don't lie. Wixpatriots provides measurable results, showcasing the tangible impact of their SEO strategies.
C. Client Testimonials
Hear directly from clients who have experienced the Wixpatriots difference. Their testimonials speak volumes about the efficacy of Wixpatriots' services.
X. Why Choose Wixpatriots
A. Expertise and Experience
Backed by a team of seasoned professionals, Wixpatriots brings a wealth of expertise and experience to the table.
B. Tailored Solutions
No cookie-cutter approaches. Wixpatriots crafts tailored solutions that align with your business objectives.
C. Transparent Pricing
No hidden fees or surprises. Wixpatriots believes in transparent pricing, ensuring you get value for your investment.
XI. Wixpatriots Blog: Insights and Tips
A. Regularly Updated Content
Stay informed with the latest in SEO through Wixpatriots' regularly updated blog, offering insights and tips for businesses navigating the digital landscape.
B. SEO Tips and Tricks
Unlock the secrets of effective SEO with Wixpatriots' expert tips and tricks shared on their blog.
C. Industry News and Trends
Be ahead of the curve by staying updated on industry news and trends curated by Wixpatriots' knowledgeable team.
XII. Building a Strong Online Presence with Wixpatriots
A. Steps to Enhance Online Visibility
Wixpatriots provides actionable steps to enhance your online visibility, making your brand more discoverable.
B. Crafting Compelling Content
Content is king. Wixpatriots guides you on crafting compelling content that resonates with your audience and search engines.
C. Leveraging Social Media
Go beyond your website. Wixpatriots explores the role of social media in boosting your online presence.
XIII. Conclusion
In a world dominated by digital interactions, having a robust online presence is non-negotiable. "Wix Patriots" emerges as a beacon of excellence in the SEO landscape, offering tailored solutions, proven results, and a client-centric approach. Elevate your digital game with Wixpatriots, where success isn't just a destination; it's a journey.
XIV. Frequently Asked Questions
A. How Long Does It Take to See SEO Results?
Patience is key in the world of SEO. While it varies, Wixpatriots aims for tangible results within the first few months of implementing their strategies.
B. Can Wixpatriots Handle Small Businesses and Large Enterprises?
Absolutely. Wixpatriots caters to businesses of all sizes, tailoring their SEO strategies to meet the unique needs of each client.
C. Are the SEO Strategies Employed by Wixpatriots Ethical?
Wixpatriots takes pride in ethical SEO practices, ensuring your online success is built on a solid foundation of integrity.
D. What Sets Wixpatriots Apart from Other SEO Agencies?
Wixpatriots stands out with its client-centric approach, transparent communication, and a track record of delivering measurable results.
E. How Does Wixpatriots Keep Up With Changing SEO Algorithms?
Adaptability is at the core of Wixpatriots' strategy. The team stays updated on industry trends, ensuring their SEO strategies evolve with changing algorithms.
0 notes
Text
Top 10 Hardest Programming Languages In 2024
Learning to code and program can be a challenging yet rewarding process. With hundreds of programming languages in existence, some are considered more difficult than others. The learning curve varies greatly between languages, with some having complex syntax and concepts that take considerable time and effort to master. Even experienced coders can find certain languages tricky. In this blog post, I'll explore 10 of the most notoriously difficult programming languages for beginners and professionals alike. Evaluating complexity, nuanced semantics, steep learning curves, and convoluted frameworks, here are the top 10 hardest programming languages to learn and use effectively.
1. C++
Known for its complexity, low-level memory management, and convoluted syntax, C++ is considered one of the most difficult languages. Developers must manage memory allocation and deallocation, pointer arithmetic, and navigate complex syntactic features. While very powerful, C++ takes time to learn properly.
2. Haskell
Haskell's highly mathematical nature and advanced type system mark it as a challenge for most. The functional programming paradigm requires a different mindset. Concepts like lazy evaluation and type classes make Haskell particularly hard to master.
3. Lisp
Lisp's use of prefix notation for expressions and heavy reliance on recursion gives it a steep learning curve. The unique syntax and coding style require time to get comfortable with. As a highly dynamic language, runtime errors can also be challenging.
4. Rust
Rust's strict compile-time checks around memory safety and concurrency make coding error-prone. The borrower checker further complicates matters. Developing Rust programs requires learning new paradigms around ownership and lifetimes.
5. Objective-C
With a complex object-oriented framework built on top of C, Objective-C is difficult. The language relies heavily on protocols, delegates, categories, and extensions, which have subtle distinctions. Just getting familiar with the API takes time.
6. Scala
Scala blends object-oriented and functional programming in a strongly typed language. It has advanced type systems featuring type inference and abstract types. The multitude of programming paradigms makes Scala challenging to learn.
7. Perl
Known for its messy and inconsistent syntax, Perl has over 100,000 individual language constructs, including shortened keywords and symbols. Programming Perl requires dealing with nuances and edge cases in the language.
8. Assembly
Any low-level assembly language will prove difficult with manual memory management and little abstraction from the hardware. Coding in assembly requires understanding computer architecture fundamentals and machine code instructions. The learning curve is quite steep.
9. Ada
Originally designed for safety-critical embedded systems, Ada is a complex statically typed language with strong data typing. Some find its verbosity and rigid requirements like exception handling make it particularly challenging to learn.
10. Forth
A stack-based programming language, Forth employs a highly uncommon programming style using reverse Polish notation. The unique syntax and paradigm mean developers have to "unlearn" other languages to grasp Forth.
Conclusion
Learning any programming language requires time, effort, and practice. But some have earned reputations as being particularly tricky. Languages like C++, Haskell, Lisp, and Scala are always known for their complexity and steep learning curves. Others like Objective-C, Perl, and Forth employ convoluted and unconventional syntax. Low-level languages like assembly and Rust are challenging due to their strict requirements and close ties to hardware. While no language is impossible to learn, it's worth being aware of the obstacles and difficulties certain languages present. Even experienced programmers can find some languages require more effort to achieve proficiency. But with passion and practice, you can master even the most notoriously difficult programming languages.
0 notes
Text
Polymorphism in C++ is a powerful concept that allows objects to be treated as instances of their parent class as well as an instance of their child classes. Polymorphism is derived from the Greek words "poly" which means many and "morph" which means forms. In C++ programming, there are mainly two types of polymorphism: compile-time polymorphism and runtime polymorphism.

Compile-time polymorphism, also known as static polymorphism or early binding, is achieved using function overloading and operator overloading. Function overloading allows different functions to have the same name but different parameters. This means that the compiler can decide which function to call based on the parameters passed to it.
For example, in C++, we can have multiple functions with the same name but different parameters like:
c
int add(int a, int b);
float add(float a, float b);
Similarly, operator overloading allows us to define the behavior of operators for user-defined data types. For example, we can overload the '+' operator for a custom class to perform addition based on our requirements.
Compile-time polymorphism provides performance benefits as the function calls are resolved at compile time.
On the other hand, runtime polymorphism, also known as dynamic polymorphism or late binding, is achieved using inheritance and virtual functions. Inheritance allows a class to inherit properties and behaviors from another class. By using inheritance, we can create a parent class and multiple child classes, each with its own unique behavior.
Virtual functions are functions in the base class that are overridden by the derived class. They allow the function to be resolved at runtime, based on the actual object being referred to. This means that the correct function is called based on the type of object at runtime. The use of the virtual keyword in C++ indicates that the function is virtual and will be resolved dynamically.
class Shape
{
public:
virtual void draw()
{
cout << "Drawing shape" << endl;
}
};
class Circle : public Shape {
public:
void draw() override
{
cout << "Drawing circle" << endl;
}
};
class Square : public Shape
{
public:
void draw() override
{
cout << "Drawing square" << endl;
}
};
In the above example, the draw() function is declared as virtual in the base class Shape and overridden in the derived classes Circle and Square. When we call the draw() function on objects of type Shape, the actual function called will depend on the type of object.
TCCI provides the best training in C++ programming through different learning methods/media is located in Bopal Ahmedabad and ISCON Ambli Road in Ahmedabad.
For More Information:
Call us @ +91 9825618292
Visit us @ http://tccicomputercoaching.com
#computer class in bopal Ahmedabad#computer class in ISCON Ambli Ahmedabad#computer coaching institute in bopal Ahmedabad#computer coaching institute in ISCON Ambli Ahmedabad#computer course in bopal Ahmedabad
0 notes