#testng annotations
Explore tagged Tumblr posts
Text
Unlocking TestNG Annotations: A Beginner's Guide
TestNG is a powerful testing framework for Java that simplifies the process of writing and running tests. One of its key features is the use of annotations, which are special markers in the code that convey information to the TestNG engine. These annotations help in defining the test methods, setting up preconditions, and handling post-execution actions. Let's dive into the essential TestNG annotations and how to unlock their potential.
1. Setting Up Test Class:
To begin using TestNG annotations, create a Java class for your test. Make sure to import the necessary TestNG libraries.
2. @BeforeSuite and @AfterSuite:
Use @BeforeSuite and @AfterSuite annotations to designate methods that should run before and after the entire test suite.
3. @BeforeClass and @AfterClass:
These annotations are used for methods that run before and after all the test methods in a class.
4. @BeforeMethod and @AfterMethod:
Use @BeforeMethod and @AfterMethod to specify methods that run before and after each test method.
5. @Test:
The @Test annotation marks a method as a test method. These methods are the actual tests you want to run.
6. @DataProvider:
If you need to run the same test with different sets of data, use the @DataProvider annotation.
7. @Parameters:
Another way to pass parameters to test methods is by using @Parameters annotation.
8. Assert Statements:
Use TestNG's assert statements for verifying expected outcomes.
9. Running Tests:
To execute your TestNG tests, you can use tools like Maven, Gradle, or run directly from your IDE.
Congratulations! You've unlocked the power of TestNG annotations to create structured and efficient test suites in Java. Experiment with these annotations to enhance your testing capabilities and improve the reliability of your code.
#testng annotations#software testing#software development#sdet#career goals#tech trends#emerging trends
0 notes
Text
Online Selenium Training with Real-Time Projects and Hands-On Practice
Unlock the Power of Selenium: Your Gateway to a High-Demand Tech Career
Imagine automating tedious web application testing, saving your team hours of manual work, and becoming the go-to test automation expert at your company. That’s the power of Selenium — and with H2K Infosys’ Online Selenium Training, you can master this skill with real-time projects and hands-on practice, even if you’re just starting out.
Selenium is the most in-demand automation testing tool used in the software industry today. Its open-source nature, flexibility, and cross-browser compatibility make it the top choice for companies around the world. Whether you're a beginner exploring testing careers or a manual tester transitioning into automation, this is your opportunity to level up.
Why Selenium? The Demand is Sky-High
80% of companies now prefer automated testing for their web applications.
LinkedIn reports over 20,000+ Selenium job openings in the U.S. alone.
Glassdoor data shows the average Selenium automation tester salary in the U.S. ranges from $85K to $120K+ per year.
Selenium is more than just a tool; it’s a career catalyst. Organizations worldwide are investing in Selenium testers who can automate test cases and enhance software quality. But employers want more than theory — they need hands-on skills. That’s where H2K Infosys bridges the gap.
What Makes Our Selenium Training Online Stand Out?
Our Selenium course online is designed for professionals who want job-ready skills and certification-backed confidence. Here’s what you’ll get when you join:
✅ Real-Time Projects
You’ll build practical skills by working on real-world scenarios such as:
Automating login functionality for web apps
Creating Selenium test scripts using TestNG and Java
Developing complete frameworks (Data-Driven, Hybrid, and Page Object Model)
Running test suites on multiple browsers using Selenium Grid
✅ Hands-On Practice Sessions
Theory is only half the battle. You’ll practice with:
Live coding sessions
Automation script writing
Debugging and test execution in IDEs like Eclipse and IntelliJ
Integrating Selenium with Maven, Git, and Jenkins
✅ Instructor-Led Live Classes
Every session is led by certified professionals with years of Selenium software testing experience. Our trainers break down complex concepts into easy-to-understand lessons.
✅ Selenium Certification Training
Our Selenium certification course prepares you for global certification exams with mock tests, quizzes, and interview preparation sessions. Get recognized for your skills and showcase your expertise to employers.
Who Should Take This Selenium Course?
Our Selenium training for beginners and experienced testers alike covers every critical area of automation testing. It’s ideal for:
Manual testers looking to shift to automation
Fresh graduates wanting to break into software testing
QA engineers aiming to upgrade their skills
Developers interested in automating testing processes
No prior coding experience? No problem. We start with the basics and build your confidence step by step.
What You Will Learn in Our Selenium Certification Training
📘 Module 1: Introduction to Automation Testing
What is software testing?
Manual vs. automation testing
Benefits of automation in Agile environments
💻 Module 2: Java for Selenium
Core Java concepts for testers
OOPs basics: Classes, Objects, Inheritance, and more
Collections, Arrays, and Exception Handling
🔧 Module 3: Selenium WebDriver
Browser automation with Selenium WebDriver
Finding elements using locators (ID, Name, XPath, CSS)
Handling alerts, frames, pop-ups, and drop-downs
🧪 Module 4: TestNG Framework
Setting up TestNG with Eclipse
Annotations and test execution order
Parallel testing and test reports
🧰 Module 5: Selenium Frameworks
Data-Driven Framework using Apache POI
Page Object Model for reusable code
Hybrid Frameworks and real-time scenarios
🛠 Module 6: Tools Integration
Maven for project management
Jenkins for Continuous Integration (CI)
Git for version control
🧾 Module 7: Real-Time Project Implementation
End-to-end project: Test e-commerce application functionality
Build a Selenium automation suite
Debug, execute, and analyze test results
Real-World Applications: Why Hands-On Practice Matters
Most Selenium testers struggle because they’ve only watched videos or read theory. But actual job roles demand script writing, debugging, and automation problem-solving.
At H2K Infosys, you’ll build your own Selenium automation testing projects from scratch. You’ll:
Write code to automate login and checkout processes
Handle browser compatibility issues
Integrate scripts with real-time CI/CD pipelines
This level of practice sets you apart in job interviews and on the job.
Career Benefits of Selenium Certification Course
Wondering how a Selenium certification training can boost your career?
1. Stand Out to Recruiters
Certified professionals with project experience are preferred over candidates without credentials or practice.
2. Land High-Paying Roles
Roles like Selenium Automation Tester, QA Analyst, Test Engineer, and SDET are in high demand.
3. Global Opportunities
Selenium skills are in demand in the U.S., Canada, UK, India, and Australia. Remote opportunities are booming too.
4. Future-Proof Your Career
As manual testing fades out, automation is the future. Selenium keeps you relevant and marketable.
FAQs About Online Selenium Training
Q1: Is this Selenium course suitable for complete beginners? Yes! We start with the basics and offer full guidance, making it perfect for beginners.
Q2: Do I need to know Java before joining? No, Java is covered from scratch in the course to help you understand Selenium better.
Q3: Will I get a certificate after course completion? Absolutely. You'll receive a course completion certificate and get trained for industry-recognized Selenium certifications.
Q4: Is this training live or self-paced? Our training is live instructor-led, allowing for interaction, Q&A, and guidance on real-time projects.
Key Takeaways
Selenium is a must-have skill in today’s software testing job market.
Hands-on experience and real-time project work are crucial to mastering Selenium.
H2K Infosys provides live training, expert guidance, and certification support.
This Selenium training online suits both beginners and experienced testers.
Career opportunities and salaries are excellent for certified Selenium professionals.
Final Thoughts
Automation is no longer optional. It’s a must. If you’re serious about building a strong tech career, investing in Selenium certification training is one of the smartest decisions you can make.
Ready to upgrade your skills and career? Enroll now in our Online Selenium Training at H2K Infosys and get hands-on experience with real-time projects. Your future in automation starts today.
#Selenium Training#Selenium Training online#Selenium certification#Selenium certification training#Selenium certification course#Selenium course#Selenium course online#Selenium course training#Selenium training for beginners#Online Selenium training#selenium automation testing#selenium software testing
0 notes
Text
Regression Testing Tools: Ensuring Software Stability
Regression testing ensures that new code changes do not break existing functionality, making it a critical part of the software development lifecycle. As software evolves, frequent updates and feature additions can introduce unintended defects. To mitigate this risk, developers and testers rely on regression testing tools to automate and streamline the testing process.
What is Regression Testing?
Regression testing is a software testing practice that verifies whether recent changes in code have affected existing features and functionality. It helps maintain software stability by re-executing previously successful test cases after modifications, such as bug fixes, new features, or code refactoring. This ensures that no new defects are introduced and that the software continues to function as expected.
Importance of Regression Testing Tools
Manually performing regression testing is time-consuming and error-prone, which is why automated regression testing tools play a crucial role in maintaining software quality. These tools help accelerate the testing process, improve test coverage, and reduce human errors. They integrate with CI/CD pipelines to ensure continuous testing, making it easier to catch issues early in the development cycle.
Key Features to Look for in Regression Testing Tools
Selecting the right regression testing tool depends on factors such as automation capabilities, integration with CI/CD pipelines, and ease of use. Some essential features to consider include:
Automated test execution – Reduces manual effort and increases efficiency.
Cross-browser and cross-platform support – Ensures compatibility across different environments.
Integration with CI/CD tools – Enables seamless testing within DevOps workflows.
Comprehensive reporting – Provides detailed insights into test results and failures.
Support for scripting and codeless automation – Caters to both technical and non-technical users.
Top Regression Testing Tools
Selenium
A popular open-source tool for web application testing that supports multiple programming languages. It enables browser automation and allows for cross-browser testing.
JUnit
A Java-based testing framework widely used for unit and regression testing. It provides assertions, test runners, and annotations for structured test execution.
TestNG
Inspired by JUnit, TestNG offers additional functionalities such as parallel testing, data-driven testing, and configurable test executions.
Cypress
A modern front-end testing framework designed for fast and reliable browser testing. It provides real-time reloading and debugging features, making it popular among developers.
Playwright
An advanced browser automation tool that supports testing in multiple environments, including Chromium, Firefox, and WebKit. It enables automated UI testing with robust debugging options.
Katalon Studio
A comprehensive automation testing tool that supports web, mobile, API, and desktop testing. It offers both scripting and codeless automation features.
Appium
An open-source tool specifically designed for mobile app testing. It supports testing across iOS and Android platforms using a single codebase.
IBM Rational Functional Tester (RFT)
An enterprise-grade tool with advanced automation features for functional and regression testing. It supports multiple scripting languages and integrates with IBM’s development tools.
Keploy
An AI-driven open-source testing tool that automatically generates test cases and stubs/mocks for integration and unit testing. It enables teams to achieve 90% test coverage in minutes, making it a powerful choice for regression testing in modern software development.
How to Choose the Right Regression Testing Tool
The best regression testing tool depends on your project requirements, tech stack, and team expertise. Consider the following when making a decision:
Project Scope – Choose a tool that aligns with your application type (web, mobile, API).
Ease of Use – Look for tools that suit your team's technical skills.
Integration Support – Ensure the tool works seamlessly with your CI/CD pipelines and version control systems.
Scalability – Opt for a tool that can handle growing test cases as your project expands.
Community and Support – Open-source tools like Keploy and Selenium have strong community support, while enterprise tools offer dedicated assistance.
Best Practices for Effective Regression Testing
To maximize the effectiveness of regression testing, follow these best practices:
Prioritize Test Cases – Focus on high-risk areas and critical functionalities.
Maintain Test Scripts – Keep test cases updated as the application evolves.
Use Automation Strategically – Automate repetitive and time-consuming tests while keeping some exploratory testing manual.
Integrate with CI/CD Pipelines – Run regression tests automatically with every code change to catch issues early.
Leverage AI and Machine Learning – Tools like Keploy enhance test generation and execution efficiency.
Conclusion Regression testing tools help teams maintain software reliability by ensuring that new changes do not introduce unexpected defects. By leveraging automation, integrating with CI/CD workflows, and following best practices, development teams can enhance software quality and accelerate the release process. Choosing the right tool, whether open-source options like Selenium and Keploy or enterprise solutions like IBM RFT, depends on project needs and long-term goals. Investing in a robust regression testing strategy ultimately leads to more stable software and a better user experience.
0 notes
Text
Do you want to know what every Java developer should know? We have shortlisted some of the highly recommended concepts and components of Java language for beginners and senior programmers. These things to learn in Java may help you get the best Java developer job you deserve. Java technology has evolved and has become really huge in the last decade. There are just too many things and its almost impossible for one person to master all of them. Your knowledge about Java technology will depend completely on what you working on. In any technology say Java or some other language, it is more important and valuable to know the language fundamentals thoroughly (OOP concepts, interfaces, class, objects, threading, etc.) rather than specific frameworks or syntax. It's always easy to quickly learn new technologies when you master the fundamentals. Are you are a beginner? Looking for some help and guidance on how to get started on this language, our exclusive article on How to Learn Java and Java libraries to know is a must read for you before getting started on Java. I just updated this article in November 2016 and things are more relevant to recent Java trends. Java Interview Preparation Tips Part 0: Things You Must Know For a Java Interview Part 1: Core Java Interview Questions Part 2: JDBC Interview Questions Part 3: Collections Framework Interview Questions Part 4: Threading Interview Questions Part 5: Serialization Interview Questions Part 6: Classpath Related Questions Part 7: Java Architect Scalability Questions Java developers knowledge expectation changes based on the profile. In this post I have divided it into 3 profiles: College Graduate, Experienced Java Developer, Experienced Java Web Developer. 7 Things a College graduate must know to get a Java developer job If you are a college graduate with no job experience then as a Java developer you need to understand the following basic things. How Java Virtual Machine works? e.g. (Platform Independence, Garbage Collection, class files, etc) What are the Object-Oriented Programming Concepts Implemented in Java? Multi-threading Java Collection framework Good understanding of data types and few of "java.lang" classes like String, Math, System, etc. java.io stream concepts. Understand the concept of Swing/AWT event-based programming. Do not spend a lot of time on this but understand the best practices. Servlets and JSP concepts. 9 Things an experienced Java Developer must know to thrive If you are an experienced professional then as a Java developer you may also need to understand the following basic things in addition to the ones listed above. Understand design patterns and its usage in Java Improvements in language from major version changes (Lambda, NIO, Generics, Annotations, Enums, ...). Coding Conventions. Build tool (Ant) or Project Management Tool (Maven). Version control System like GIT/SVN/Perforce/Clearcase. Apache Commons Libraries & few other common open source libraries. Continuous Integration Tools e.g. Jenkins and Hudson. Unit testing - e.g. JUnit and TestNG Unit testing Mocking libraries like Mockito Fundamental understanding of JSON and XML Understand Business layer frameworks - like Spring Understanding dependency injection (e.g. Spring, Google Guice, and Plain Java Dependency injection) 4 Things a Java Web Developer (JEE) Developer must know If you are an experienced professional working on Web-based development then as a JEE developer you also need to understand the following basic things in addition to the ones (7+9) listed above. Understanding of MVC Frameworks - Open source web frameworks like - Spring MVC, Struts, Vaadin, etc. Understanding of Microservice based framework like Spring Boot. Important Note: A lot of Front End UI development is now shifted to JavaScript frameworks. Therefore do not focus on Java-based frameworks that focus on the user interface (e.g. JSF or related frameworks).
Instead, learn JavaScript related frameworks like Angular.js or Backbone.js Fundamental understanding of Web Services and REST based service development. Good understanding of Web/Application server like Tomcat, Glassfish, WebLogic, WebSphere, Jetty, etc. Unix environment - A working knowledge of Unix environment can be beneficial as most of the Java servers are hosted on Unix based environment in production. Looking at the list of things it really feels difficult for a person to know each and everything in depth. As I already said it is more important and valuable to know the language fundamentals thoroughly and rest can be learned quickly when required. Can you think of something which is not part of this post? Please don't forget to share it with me in the comments section & I will try to include it in the list. Article Updates Article updated in April 2019 - Updated Introduction section, fixed minor text, and updated answers. Updated on November 2016 - Made changes as per latest technology trends and stacks.
0 notes
Text
Top Automation Testing Frameworks for Efficient Software Development
Whether you are a seasoned developer or a newcomer, the need for tools that allow software developers to make their software high quality delivered quickly is a necessity. Automation Testing has proven to be a game changer, allowing the developers and QA teams to maintain the quality of the software, shorten time-to-market, and optimize repetitive testing processes. However, the main factor behind successful Automation Testing is selecting the appropriate test automation framework.
In this blog, we will discuss some of the most popular Test Automation frameworks, their features that make them unique and how they enhance QA process that helps in efficient Software Development. GhostQA can certainly help you in delivering seamless and efficient Automation Testing strategies if you're looking for a professional approach in implementing the ideal framework.
What is a Test Automation Framework?
A Test Automation framework means a collection of guidelines, tools, and practices that are used to automate the testing process in a simple and standardized way. This application serves as a guiding framework for scripting automation in a homogenous way which can be easily maintainable and scalable.
By adopting the right framework, the team can leverage the benefits of Automation Testing, such as increased speed, better coverage, and below human error.
Why Choosing the Right Framework Matters
The Test Automation framework is the single most important decision that will affect the effectiveness and reliability of testing efforts. Here’s why choosing the appropriate framework is important:
Ensure all technology stacks are compatible with your tech.
Makes test scripts easier to write and maintain.
Improves team collaboration and productivity.
Increases scalability for future project needs.
Decreases the overall test cost, without compromising on quality.
Top Automation Testing Frameworks
Let us look at some of the most used and efficient Automation Testing frameworks:
1. Selenium
Overview: Selenium is an open-source framework most commonly used to perform testing on web applications. It works with various browsers, platforms, and programming languages.
Features:
Functional on multiple browsers and multiple platforms.
Strong community support and regular iterations.
Best For: Web application functional and regression testing.
2. Appium
Overview: Appium is a widely used open-source framework for testing mobile applications. It is compatible with Android, iOS, and Windows apps.
Features:
Works with native, hybrid and mobile web apps.
It works with the same APIs across multiple platforms.
Enables scripts to be reused on multiple platforms.
Best For: The testing of apps for mobile devices.
3. TestNG
Overview: TestNG is a framework for the Java programming language inspired from JUnit. This supports a variety of different test setups and annotations.
Features:
Enables parallel execution to speed things up.
Various flexible options for test configuration
Provides extensive reporting that you can tailor to your specifications.
Best For: Integration, functional and unit testing.
4. Cypress
Overview: Cypress is an end-to-end testing framework for modern web applications.
Features:
Test execution with on-the-fly reloading.
Waits for commands and DOM updates implicitly.
Debugging tools for developers built into the platform.
Best For: UI Testing and end-to-end testing for web-based applications.
5. JUnit
Overview: JUnit is another popular framework for Java applications mainly focused on unit testing.
Features:
Makes test-driven development (TDD) easier.
Rich support for assertions and annotations.
Best for small and focused tests.
Best For: When writing unit tests for Java-based applications.
6. Katalon Studio
Overview: Katalon Studio is an end-to-end testing solution providing web, API, mobile, and desktop testing capabilities.
Features:
Built-in templates and intuitive interface
Favors manual as well as through automation testing.
Best For: Teams looking for a user-friendly, all-in-one solution.
7. Robot Framework
Overview: Robot Framework is a generic open-source test automation framework that uses a keyword-driven approach.
Features:
Easily readable test cases.
You can extend with libraries and tools.
Great for the less technical members of your team.
Best For: Acceptance test and RPA (Robotic process automation).
How Automation Testing Benefits Software Development
There are several advantages of adopting Automation Testing frameworks:
Faster Testing Cycles: Automated tests run faster than manual tests leading to a decreased testing time.
Improved Accuracy: Reduced human error leads to more accurate results
Reusability of Tests: The frameworks help in reusing the test scripts on different projects.
Increased Test Coverage: It allows the testing of huge datasets and numerous scenarios.
Cost Efficiency: Despite the initial investment, it saves a lot of time and resources in the long run.
Challenges in Automation Testing
Although Automation Testing comes with lots of advantages, there are also some challenges:
High Initial Costs: Setting up a framework will require time and resources.
Complex Tool Integration: Deciding to use the right tools and ensuring compatibility can be a struggle.
Skill Gaps: Team members might require training in order to effectively use advanced frameworks.
Maintenance Effort: Whenever the application changes, it is imperative to update the test scripts.
GhostQA: Your Trusted Partner for Automation Testing
GhostQA focuses on helping businesses with effective Automation Testing solutions. So, if you want help regarding selecting a Test Automation framework, or want us to implement solid strategies, GhostQA is your choice.
Why Choose GhostQA?
Deep knowledge of frameworks (Selenium, Appium, Cypress, etc.).
Custom solutions designed around your specific project requirements.
Proven approaches for overcoming automation dilemmas.
Professional service to guarantee that your testing workflow is smooth and trustworthy.
Best Practices for Using Automation Frameworks
Select the Right Framework: Make sure it suits your project requirements and team experience.
Plan Test Cases Strategically: Prioritize high-value and repeated tasks to be automated.
Incorporate Regular Maintenance: Refresh the scripts based on modifications in the application and the environment.
Use a Hybrid Approach: Integrate both manual and automated testing for coverage
Leverage Reporting Tools: Take advantage of detailed reports to monitor progress and find opportunities for growth.
Conclusion
It is obligatory to select the right Automation Testing framework for the best software development. Testing frameworks such as Selenium, Appium, and Katalon Studio have a variety of features that help fast-track testing tasks and improve product quality.
Joining forces with GhostQA gives you a road of expertise and solutions right for you making sure your Test Automation steps are easy and prosperous.
Start using the appropriate Automation Testing framework now to reduce your development cycles, enhance test accuracy, and build superior software. Get in touch with GhostQA to see how we can revolutionize your testing methodologies.
#quality assurance#automated testing#test automation#software testing#performance testing#automation testing#functional testing#regression testing#load testing
0 notes
Text
Selenium WebDriver with Java & TestNG Testing Framework
Introduction to Selenium WebDriver, Java, and TestNG
What is Selenium WebDriver?
Selenium WebDriver is a widely used open-source automation testing tool for web applications. It allows testers to execute tests directly on browsers and supports multiple programming languages like Java, Python, and C#.
Why Use Java for Selenium?
Java is the most popular language for Selenium due to its robust libraries, extensive community support, and compatibility with various tools like TestNG and Maven.
What is TestNG Framework?
TestNG (Test Next Generation) is a testing framework inspired by JUnit but offers advanced features like annotations, data-driven testing, and parallel execution, making it an ideal companion for Selenium.
Setting Up Selenium WebDriver with Java
Prerequisites for Installation
Java Installation
Ensure Java Development Kit (JDK) is installed on your system. Use the command java -version to confirm the installation.
Eclipse IDE Setup
Download and install Eclipse IDE for Java Developers. It provides a user-friendly environment for writing Selenium scripts.
Configuring Selenium WebDriver
Downloading Selenium JAR Files
Visit the Selenium website and download the WebDriver Java Client Library.
Adding JAR Files to Eclipse
Import the downloaded JAR files into your Eclipse project by navigating to Project > Build Path > Add External JARs.
Introduction to TestNG Framework
Why TestNG for Selenium?
TestNG simplifies test case management with features like grouping, prioritization, and result reporting.
Installing TestNG in Eclipse
TestNG Plugin Installation
Install the TestNG plugin via Eclipse Marketplace.
Verifying Installation
After installation, you should see the TestNG option in the Eclipse toolbar.
Writing Your First Selenium Test Script
Creating a Java Project in Eclipse
Start by creating a new Java project and adding Selenium and TestNG libraries to it.
Writing a Basic Selenium Script
Launching a Browser
Use WebDriver commands to open a browser, e.g., WebDriver driver = new ChromeDriver();.
Navigating to a Web Page
Navigate to a URL using the driver.get("URL"); method.
Locating Web Elements
Use locators like ID, Name, or XPath to interact with elements.
Integrating TestNG with Selenium
Writing TestNG Annotations
Annotations like @Test, @BeforeTest, and @AfterTest help structure your test cases.
Executing Test Cases with TestNG
@Test Annotation Explained
Mark methods as test cases with the @Test annotation.
Generating TestNG Reports
After execution, TestNG generates a detailed HTML report showing test results.
Advanced Features of Selenium with TestNG
Parameterization in TestNG
Using DataProvider Annotation
DataProvider allows you to pass multiple sets of data to a test case.
Passing Parameters via XML
Define test parameters in the TestNG XML file for dynamic execution.
Parallel Test Execution
Running Tests in Parallel Browsers
Configure the TestNG XML file to execute tests simultaneously on different browsers.
Handling Web Elements in Selenium
Working with Forms
Input Fields and Buttons
Automate form filling and button clicks using WebDriver commands.
Managing Dropdowns and Checkboxes
Use Select class for dropdowns and isSelected() for checkboxes.
Handling Alerts and Popups
Switch to alerts with driver.switchTo().alert(); for handling popups.
Best Practices for Selenium Testing
Designing Modular Test Scripts
Break down test scripts into reusable modules for better maintainability.
Implementing Page Object Model (POM)
Organize your code by creating separate classes for each page in your application.
Handling Synchronization Issues
Use implicit and explicit waits to handle delays in element loading.
Debugging and Troubleshooting Selenium Scripts
Common Errors in Selenium Testing
ElementNotVisibleException
Occurs when attempting to interact with hidden elements.
NoSuchElementException
Triggered when the WebDriver cannot locate an element.
Debugging Tools in Eclipse
Use breakpoints and the debugging perspective in Eclipse to identify issues.
Conclusion
Mastering Selenium WebDriver with Java and TestNG opens doors to efficient and robust automation testing. By understanding the basics, leveraging TestNG’s features, and adhering to best practices, you can build a powerful testing suite.
FAQs
Can I use Selenium with other programming languages?
Yes, Selenium supports multiple languages like Python, C#, Ruby, and JavaScript.
What are the limitations of Selenium WebDriver?
Selenium cannot test non-web applications, handle captchas, or manage dynamic page loads efficiently without additional tools.
How does TestNG differ from JUnit?
TestNG offers more advanced features, including parallel testing, better test configuration, and detailed reporting.
Is Selenium WebDriver suitable for mobile testing?
Not directly, but tools like Appium extend Selenium for mobile application testing.
How do I manage dependencies in a large Selenium project?
Use build tools like Maven or Gradle to manage dependencies efficiently.
0 notes
Text
What Skills Are Required To Use Selenium Effectively?
Introduction
Selenium is a widely used open-source tool for web browser automation and testing web applications. It provides testers and developers with a flexible and efficient platform to ensure the quality and functionality of web-based software. However, to leverage Selenium effectively, individuals need a robust set of skills. This article explores the essential technical and soft skills that empower professionals to excel with Selenium.
Understanding The Basics Of Web Technologies
Before diving into Selenium, it's crucial to have a solid grasp of fundamental web technologies. Embark on the journey to unlock the full potential of automation testing with Selenium Training in Chennai at Infycle Technologies, the leading destination for mastering automation tools. These include:
HTML, CSS, and JavaScript: Since Selenium interacts with web elements like buttons, forms, and links, understanding how these elements are structured and styled is essential.
DOM (Document Object Model): Familiarity with the DOM enables testers to locate and interact efficiently with elements on a webpage.
HTTP Protocols: Knowing how web browsers communicate with servers helps understand page load issues and debug network-related test failures.
Proficiency In Programming Languages
Selenium supports multiple programming languages, including Python, Java, C#, Ruby, & JavaScript. To use Selenium effectively, one must:
Choose a programming language based on project requirements and team expertise.
Master the selected language's syntax, logic, and object-oriented programming (OOP) concepts.
Understand how to write clean, maintainable, and modular code.
For example, Python is often favoured for its simplicity, while Java is widely used in enterprise environments.
Knowledge Of Selenium Frameworks
Familiarity with Selenium frameworks enhances test automation. Key frameworks include:
TestNG and JUnit (for Java): These testing frameworks allow for better organization, annotation, and test execution.
Pytest (for Python): A robust framework that simplifies test case management and reporting.
Page Object Model (POM): A design pattern used to create object repositories for web elements, improving test script readability and reusability.
XPath And CSS Selectors Mastery
Locating elements is a core functionality in Selenium. XPath and CSS selectors are powerful tools for identifying elements on a webpage. Skills in:
Writing efficient and precise XPath expressions.
Utilizing CSS selectors for faster and cleaner element identification.
Handling dynamic elements with relative XPath or custom attributes.
Version Control Systems (VCS)
Selenium scripts are often part of larger development projects. Using version control systems like Git ensures collaboration and proper version management. A Selenium tester should:
Understand Git commands for committing, branching, merging, and reverting changes.
Use platforms like GitHub or GitLab for code sharing and version tracking.
Familiarity With Continuous Integration/Continuous Deployment (CI/CD) Tools
In modern software development, CI/CD tools integrate Selenium scripts into automated pipelines. Popular CI/CD tools include:
Jenkins: For scheduling and managing automated test runs.
CircleCI and Travis CI: For seamless integration of test scripts with deployment processes.
Understanding how to configure these tools and integrate Selenium tests into the pipeline is invaluable.
Debugging And Problem-Solving Skills
Test automation often involves troubleshooting. Effective debugging skills include:
Identifying and resolving broken locators, synchronization problems, and environment-specific errors.
Using browser developer tools to inspect web elements and network activity.
Logging errors and analyzing stack traces for quick fixes.
Soft Skills: Communication And Team Collaboration
Technical expertise is only part of the equation. Selenium testers often work in collaborative environments where soft skills matter. These include:
Clear Communication: Explaining test results and automation coverage to non-technical stakeholders.
Team Collaboration: Working closely with developers, product managers, and QA teams to align testing objectives with business goals.
Adaptability: Adjusting to new tools, frameworks, and methodologies as projects evolve.
Experience With Testing Methodologies
A strong foundation in testing methodologies enhances the effectiveness of Selenium testing. Key methodologies include:
Manual Testing Fundamentals: Understanding the principles of manual testing provides insights into writing meaningful automated tests.
Agile and DevOps Practices: Familiarity with iterative development models ensures test automation aligns with rapid development cycles.
Test Strategies: Creating test plans, prioritizing cases, and managing test environments.
Database And API Knowledge
Web applications often interact with databases and APIs. Enhance your journey toward a successful career in software development with Infycle Technologies, the Best Software Training Institute in Chennai. Selenium testers should:
Understand basic SQL queries to verify back-end data.
Use API testing tools like Postman or REST-assured for end-to-end testing scenarios.
Cloud Testing Platforms
Cloud-based platforms like BrowserStack and Sauce Labs allow for cross-browser and cross-platform testing. Skills required include:
Configuring tests to run on different browser and OS combinations.
Using platform-specific features for debugging and reporting.
Keeping Up With Updates And Trends
Selenium evolves continuously, and staying updated is vital. This includes:
Learning new features introduced in Selenium WebDriver.
Understanding emerging technologies like Selenium Grid 4 for distributed testing.
Exploring alternatives or complementary tools like Cypress and Playwright.
Conclusion
Mastering Selenium requires technical expertise, problem-solving capabilities, and collaborative skills. By building a strong foundation in programming, web technologies, and testing frameworks while staying adaptable to new trends, professionals can harness the full potential of Selenium for robust test automation. Whether you are a beginner or an experienced tester, developing these skills will ensure that you remain competitive in the ever-evolving field of software testing.
0 notes
Text
Step-by-Step Guide to Learning Java for Selenium Testing
Java is one of the most widely-used programming languages for Selenium because it’s versatile, well-documented, and offers a large community for support. If you want to advance your career at the Selenium Course in Pune, you need to take a systematic approach and join up for a course that best suits your interests and will greatly expand your learning path. Here’s a step-by-step guide to help you learn Java effectively and set a solid foundation for your Selenium testing journey.
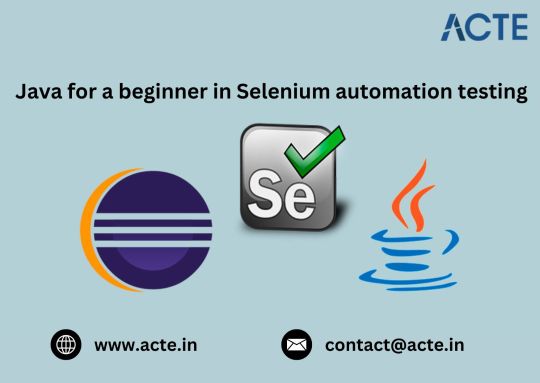
Step 1: Understand Why Java is Important
Before diving in, it’s good to know why you’re learning Java in the first place. Java helps you:
Write test scripts in Selenium.
Use powerful libraries for browser automation.
Handle complex scenarios in testing, like working with APIs or databases.
By understanding its relevance, you’ll stay motivated as you learn. For those looking to excel in Selenium, Selenium Online Course is highly suggested. Look for classes that align with your preferred programming language and learning approach.
Step 2: Start with Basic Java Concepts
Java may seem overwhelming at first, but breaking it down into manageable topics makes it easier. Here are the key concepts to focus on:
Syntax and Structure: Learn how Java programs are written.
Keywords like class, public, and static
How to write main methods (the entry point of any Java program)
Variables and Data Types: Understand how to store and manage data.
Types like int, String, and boolean
Declaring and initializing variables
Control Flow Statements: Learn how to add logic to your programs.
If-else conditions
Loops like for, while, and do-while
Object-Oriented Programming (OOP): This is essential for working with Selenium.
Concepts like classes, objects, inheritance, and polymorphism
How to create and use methods
Collections and Arrays: Learn to work with lists of data.
Arrays
Collections like ArrayList and HashMap
Spend time practicing these basics. Write small programs to reinforce what you’re learning.
Step 3: Use Online Resources and Practice Platforms
Several free and paid resources can help you learn Java:
Video Tutorials: YouTube has great beginner-friendly tutorials.
Interactive Coding Platforms: Try Codecademy, HackerRank, or LeetCode for hands-on practice.
Books: Consider beginner-friendly books like Head First Java.
Documentation: Oracle’s official Java documentation is a reliable resource for reference.
Step 4: Learn Java with Selenium in Mind
Once you’re comfortable with the basics, focus on the Java features you’ll use in Selenium automation testing:
File Handling: Learn to read and write data to files (useful for handling test data).
Exception Handling: Understand how to manage errors and unexpected conditions.
Multithreading: While not essential at first, it’s useful for parallel testing.
Annotations: Used frequently in TestNG (a testing framework for Selenium).
Step 5: Start Writing Selenium Scripts
As you gain confidence in Java, begin integrating it with Selenium:
Set Up Your Environment: Install Java, Selenium WebDriver, and an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse.
Learn Selenium Basics: Write scripts to open a browser, click buttons, and fill out forms.
Use Java for Advanced Selenium Features:
Dynamic locators
Data-driven testing with Excel
Handling alerts, frames, and windows
Step 6: Practice, Practice, Practice
The key to mastering Java for Selenium is consistent practice:
Work on real-world projects.
Solve problems on coding platforms.
Explore sample Selenium projects on GitHub.
Step 7: Join Communities and Seek Help
Join Java and Selenium communities to connect with others:
Forums: Stack Overflow, Reddit’s r/selenium
Groups: LinkedIn groups and Discord servers for testers
Meetups: Attend webinars or local testing meetups
Being part of a community ensures you’re never stuck for too long and exposes you to new techniques.
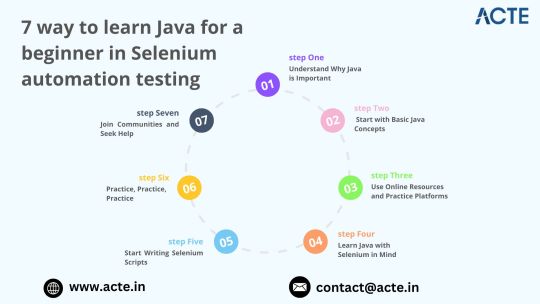
Learning Java for Selenium automation testing might feel challenging at first, but with consistent effort and the right resources, you’ll get there. Focus on the basics, keep practicing, and gradually dive into more complex topics. Remember, every expert was once a beginner—and you’re on the right path!
0 notes
Text
Unlock Your Career Potential with Selenium Automation Testing in Mohali
In the rapidly evolving world of software development, automation testing has become a critical component of ensuring product quality and efficiency. Among the various tools available, Selenium stands out as one of the most popular and effective frameworks for web application testing. If you’re looking to enhance your skills in automation testing, Mohali is home to some of the best training institutes, including Mohali Career Point (MCP).
Why Choose Selenium for Automation Testing?
Selenium is an open-source automation testing tool widely used in the IT industry. Its advantages include:
Cost-Effective: Being open-source, Selenium has no licensing fees, making it an economical choice for organizations of all sizes.
Versatile and Efficient: Selenium supports multiple programming languages, including Java, Python, and Ruby. It also works seamlessly across various operating systems and web browsers like Chrome, Firefox, and Safari.
Robust Community Support: With a large and active community, developers can easily find resources, plugins, and tools to extend Selenium’s functionality.
Flexibility: Selenium can be integrated with other testing frameworks like TestNG and JUnit, providing greater flexibility in test case management.
Comprehensive Selenium Training at MCP in Mohali
At Mohali Career Point, we offer a structured Selenium training program that caters to beginners and advanced users alike. Our course is divided into six comprehensive modules, covering everything from the basics to advanced automation concepts.
Course Modules Overview
Selenium Introduction: Understand what Selenium is, its components, and how it compares to other tools like QTP.
Selenium IDE and RC: Learn how to install and use Selenium IDE for creating and running test cases.
Core Java Introduction and OOPs Concepts: Gain foundational knowledge in Java programming, which is essential for using Selenium effectively.
Selenium WebDriver and Selenium Grid: Dive deep into WebDriver features, browser navigation, element identification, and cross-browser testing.
TestNG and Testing Frameworks: Explore TestNG for managing your tests efficiently, including annotations, data providers, and report generation.
Database Testing — Java Data Base Connectivity: Learn how to connect your test cases to databases and execute queries using JDBC.
Real-Time Project Training
What sets our training apart is the emphasis on practical experience. Our live project training module is designed to give you hands-on experience, allowing you to apply what you’ve learned in a real-world setting. This practical knowledge is invaluable and can significantly enhance your employability.
Why Choose MCP for Selenium Training in Mohali?
Expert Trainers: Our trainers are seasoned professionals with extensive experience in automation testing. They provide in-depth knowledge and practical insights to help you excel.
Tailored Learning: We cater to individual learning paces, ensuring that every student fully grasps the concepts before moving forward.
Career Support: MCP is committed to your success. We offer career counseling and job placement assistance to help you secure high-paying positions in the IT industry.
Diverse Course Offerings: In addition to Selenium, MCP offers various courses in advanced Java, Python, and database management, allowing you to broaden your skill set.
Join MCP Today!
If you’re looking to kickstart a successful career in automation testing, look no further than Mohali Career Point. Our comprehensive Selenium training program will equip you with the knowledge and skills needed to thrive in the competitive IT landscape.
Contact Us Today!
📧 Email: [email protected] 📞 Phone: +91 7696 2050 51 / +91 7906 6891 91 📍 Address: SC-130 Top Floor, Phase 7, Mohali, 160059
Embrace the future of software testing. Choose MCP for your Selenium training in Mohali and take the first step towards a rewarding career in automation testing!
0 notes
Text
Reliance Infrastructure Announces Major Expansion Plans

Reliance Infrastructure, part of the Anil Ambani-led Reliance Group, has unveiled its plans for a major expansion in key sectors, including power, roads, and urban infrastructure. The company aims to strengthen its position in the growing Indian infrastructure market by investing in sustainable energy projects, upgrading transportation networks, and enhancing urban development. With these strategic moves, Reliance Infrastructure is set to capitalize on the rising demand for infrastructure services across the country, positioning itself as a leader in the sector.
Read more about-
Selenium Webdriver with Java & TestNG Testing Framework: A Complete Guide

In today’s fast-paced digital world, automated testing has become a critical component in ensuring software quality and speed of development. Whether you’re a beginner or an experienced developer, understanding how to use Selenium Webdriver with Java & TestNG Testing Framework can greatly enhance your software testing capabilities. This combination not only helps you automate testing for web applications but also makes the testing process more efficient and less prone to human error.
In this blog, we will dive into the details of Selenium Webdriver, why it is paired with Java, and how using TestNG as a testing framework creates a powerful testing toolset for web automation.
What is Selenium Webdriver?
Selenium Webdriver is an open-source tool designed for automating web application testing. Its primary function is to enable you to simulate user actions on a browser, from clicking buttons and filling out forms to navigating through pages. It supports multiple browsers like Chrome, Firefox, and Safari, making it a versatile tool for cross-browser testing.
Using Selenium Webdriver with Java offers a unique advantage because Java is widely adopted, easy to learn, and highly scalable. The pairing of these two tools allows developers and testers to write concise and reusable test scripts that are maintainable across different environments.
Why Choose Selenium with Java?
Selenium Webdriver can be used with multiple programming languages like Python, C#, Ruby, and Java. But Selenium Webdriver with Java is particularly popular for a few reasons:
Extensive Libraries: Java has a rich set of libraries that simplify coding and interaction with browsers.
Large Community Support: Since Java is one of the most widely used programming languages, there’s ample community support to help troubleshoot any issues you encounter.
Integration with Tools: Java integrates easily with tools like Maven, Jenkins, and other CI/CD pipelines, making it perfect for test automation in agile environments.
Understanding TestNG: The Power of Test Organization
TestNG is a testing framework inspired by JUnit and NUnit but with more powerful features. It allows you to organize your test cases effectively, manage the execution of those tests, and generate detailed reports on the results.
Combining Selenium Webdriver with Java & TestNG Testing Framework provides a robust environment where you can easily manage and scale your test cases. Some key benefits of using TestNG are:
Annotations: TestNG uses annotations like @Test, @BeforeTest, and @AfterTest to manage the execution flow of tests.
Parallel Execution: You can run tests in parallel, which reduces the overall test execution time.
Grouping: It allows you to group your test cases and execute specific groups, depending on the testing scenario.
Detailed Reports: TestNG automatically generates detailed reports after the test execution, highlighting passed, failed, and skipped tests.
Setting Up Selenium Webdriver with Java & TestNG
Step 1: Download and Install Java
Before you begin, you’ll need to install the Java Development Kit (JDK). Ensure you have the correct version installed and set up the Java environment variables.
Step 2: Install Selenium Webdriver
Next, download Selenium Webdriver from the official Selenium website. Selenium provides a Java Client Library that you’ll need to add to your project.
Step 3: Install TestNG
TestNG is a third-party library, and you can add it to your project through Maven or manually download the JAR files. If you're using Maven, simply add the TestNG dependency to your pom.xml file.
Step 4: Writing Your First Test
Once everything is set up, you can create your first test. Below is a simple example of how to use Selenium Webdriver with Java & TestNG Testing Framework.
java
Copy code
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class SeleniumTest {
WebDriver driver;
@BeforeTest
public void setup() {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
driver = new ChromeDriver();
}
@Test
public void openGoogle() {
driver.get("https://www.google.com");
System.out.println("Google page opened");
}
@AfterTest
public void teardown() {
driver.quit();
}
}
In this example, the @BeforeTest annotation ensures that the ChromeDriver is set up before running the test. The @Test method opens Google and prints a confirmation message, while the @AfterTest method closes the browser once the test is complete.
Key Advantages of Using Selenium Webdriver with Java & TestNG
Cross-browser Testing: Selenium Webdriver allows you to test on multiple browsers, ensuring your web application performs consistently across platforms.
Scalability: The combination of Java and TestNG allows you to create modular, scalable tests that can be executed in parallel.
CI/CD Integration: Using TestNG with Selenium Webdriver ensures smooth integration into CI/CD pipelines, enabling continuous testing.
Extensive Documentation: Both Selenium and Java have extensive documentation, making it easier for developers to find solutions and get started quickly.
Free AI Tools for Testing Automation
In the modern age of technology, you can also take advantage of free AI tools that assist in test automation. Many AI-powered testing platforms help reduce the time it takes to write test cases by suggesting test scripts, predicting potential failure points, and automating repetitive tasks. Tools like Mabl and Testim use AI to enhance traditional testing strategies, giving developers an edge when managing complex test suites. You can integrate these tools with Selenium Webdriver with Java & TestNG Testing Framework to further streamline your automation testing process.
Common Challenges and Solutions
Synchronization Issues: Sometimes, the web elements may take time to load, and Selenium might not wait long enough before interacting with them. The solution is to use WebDriverWait for explicit waits. Example: java Copy code WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("element_id")));
Handling Multiple Windows: While testing, you might encounter scenarios where a new window or tab opens. Selenium allows you to switch between multiple windows using the switchTo() method. Example: java Copy code String mainWindow = driver.getWindowHandle();
for (String handle : driver.getWindowHandles()) {
driver.switchTo().window(handle);
}
Dynamic Elements: Some web elements have dynamic IDs or names that change every time the page is reloaded. In such cases, it’s best to use CSS selectors or XPath. Example: java Copy code WebElement dynamicElement = driver.findElement(By.xpath("//input[contains(@id, 'dynamic')]"));
Conclusion
Mastering Selenium Webdriver with Java & TestNG Testing Framework is essential for anyone looking to excel in the field of automated web testing. The combination of these tools allows you to create efficient, reusable, and scalable test scripts that ensure your web applications are bug-free and performant. Whether you’re testing a small-scale project or managing a large automation suite, the flexibility and power of these tools make them invaluable.
By integrating AI tools into your automation pipeline, you can take your testing strategies to the next level, reducing manual intervention and making the testing process faster and more reliable.
In summary, leveraging Selenium Webdriver with Java along with the robust features of TestNG sets you up for success in the automation world. If you’re looking to enhance your testing knowledge or explore more advanced concepts, start building your test suites today! Happy testing!
0 notes
Text
The Top Selenium Frameworks for Effective Automated Testing
Selenium is a leading tool for web application automation, but to fully leverage its capabilities, it's essential to pair it with a suitable testing framework. This guide delves into some of the best Selenium frameworks, each offering unique features and benefits to help streamline your testing process.
Why a Testing Framework Matters
A robust testing framework is the backbone of any automated testing strategy. Embracing Selenium's capabilities becomes even more accessible and impactful with Selenium Training in Pune. It provides structure for writing, managing, and executing test cases, enhancing productivity, improving test coverage, and ensuring reliable results. Here, we explore several top-tier frameworks that integrate seamlessly with Selenium.
TestNG: Versatile and Robust
Overview
Inspired by JUnit and NUnit, TestNG is designed to handle various test categories, including unit, functional, end-to-end, and integration tests. Its powerful features cater to complex testing needs.
Key Features
Parallel Execution: TestNG allows for parallel test runs, significantly reducing the time needed to execute extensive test suites.
Comprehensive Reports: It generates detailed, customizable reports that provide insights into test executions.
Annotations: Offers a rich set of annotations like @Test, @BeforeMethod, and @AfterMethod, aiding in efficient test management.
Data-Driven Testing: Facilitates data-driven tests with the @DataProvider annotation, enabling the same test to run with different data sets.
Benefits
TestNG's flexibility and comprehensive feature set make it ideal for complex scenarios. Its ability to run parallel tests and generate detailed reports saves time and enhances test analysis.
JUnit: Simplicity and Effectiveness
Overview
JUnit is a widely used testing framework for Java applications, renowned for its simplicity and ease of use.
Key Features
User-Friendly: JUnit’s straightforward approach simplifies writing and executing tests.
Annotations: Provides essential annotations like @Test, @Before, and @After, aiding in test case management.
Integration: Seamlessly integrates with various development tools and CI/CD pipelines. To unlock the full potential of Selenium and master the art of web automation, consider enrolling in the Top Selenium Online Training.
Benefits
JUnit's simplicity makes it perfect for small to medium-sized projects. Its ease of integration and straightforward testing approach can speed up development and ensure quality.
Cucumber: BDD for Better Collaboration
Overview
Cucumber facilitates Behavior Driven Development (BDD), allowing test cases to be written in plain English using the Gherkin language, making them accessible to both technical and non-technical stakeholders.
Key Features
Plain Language Syntax: Uses Gherkin to make test cases readable by anyone on the project, including business analysts and non-technical team members.
BDD Focus: Encourages collaboration between developers, QA, and business stakeholders, ensuring comprehensive application testing.
Multi-Language Support: Supports various programming languages, including Java, Ruby, and Kotlin.
Benefits
Cucumber’s BDD approach ensures clear communication among all stakeholders, fostering better collaboration and reducing misunderstandings. Its versatility and plain language syntax make it easy to adopt.
Robot Framework: Simplifying Testing with Keywords
Overview
Robot Framework is a generic, open-source automation framework using keyword-driven testing. Known for its easy-to-read syntax and extensive library support, it's highly accessible.
Key Features
Keyword-Driven: Uses keywords to define test cases, simplifying the process of writing and reading tests.
Library Support: Supports numerous libraries, including SeleniumLibrary, for using Selenium WebDriver.
Language-Independent: Its modular architecture supports various tools and libraries.
Benefits
Robot Framework’s keyword-driven approach makes test case creation straightforward, accessible to testers with varying technical expertise. Its extensive library support and modular architecture enhance its versatility.
Conclusion
Choosing the right framework for Selenium testing hinges on your specific project requirements and team capabilities. TestNG offers flexibility and detailed reporting for complex scenarios. JUnit’s simplicity and ease of use are ideal for smaller projects. Cucumber’s BDD approach promotes collaboration between technical and non-technical team members. Robot Framework’s keyword-driven testing simplifies test creation, making it accessible and versatile.
Understanding the strengths and unique features of each framework will help you select the one that best fits your needs, ensuring efficient and effective Selenium testing. Whether you're handling a small project or a large, intricate system, there’s a Selenium framework tailored to help you achieve your testing goals.
1 note
·
View note
Text
Selenium: Key Points to Learn
Selenium is a powerful tool for automating web applications for testing purposes, but it can also be used for web scraping and automating repetitive web-based tasks. For those keen to excel in Selenium, enrolling in a Selenium course in Pune can be highly advantageous. Such a program provides a unique opportunity to acquire comprehensive knowledge and practical skills crucial for mastering Selenium. To effectively learn and use Selenium, here are the key points to focus on:
1. Understanding Selenium and Its Components
Selenium WebDriver: The core component that drives the browser.
Selenium IDE: A browser extension for record-and-playback of interactions.
Selenium Grid: A tool to run tests on different machines and browsers in parallel.
2. Setting Up the Environment
Install WebDriver: Download the WebDriver for the browser you intend to automate (e.g., ChromeDriver for Google Chrome).
Configure IDE: If using an Integrated Development Environment (IDE) like Eclipse, IntelliJ, or VSCode, ensure it's set up with necessary plugins.
3. Programming Languages
Language Support: Selenium supports multiple programming languages including Java, Python, C#, Ruby, and JavaScript.
Learning Basics: Have a good grasp of the basics of the programming language you'll use with Selenium.
4. Basic Selenium Commands
Navigation: Learn how to navigate to URLs and interact with browser history.
Locators: Master different locators (ID, Name, Class Name, Tag Name, CSS Selector, XPath) to find elements on a web page.
Actions: Perform actions like click, sendKeys (for typing), and others like drag and drop.
5. Advanced Interactions
Waits: Implement implicit and explicit waits to handle dynamic web content.
Frames and Windows: Handle frames, windows, and alerts effectively.
Keyboard and Mouse Events: Use Actions class for complex user interactions like double-click, right-click, and hover.
6. Page Object Model (POM)
Design Pattern: Use POM to create an object repository for web elements, enhancing test maintenance and reducing code duplication.
Implementation: Structure your project to include page classes and test classes.
7. Test Framework Integration
JUnit/TestNG: Integrate Selenium with testing frameworks like JUnit or TestNG for better test structure, reporting, and annotations.
Assertions: Use assertions to validate test outcomes.
8. Handling Web Elements
Dynamic Elements: Learn strategies to interact with elements that change dynamically.
Dropdowns, Checkboxes, and Radio Buttons: Work with common form elements effectively.
9. Error Handling and Debugging
Exception Handling: Implement try-catch blocks to handle exceptions.
Logs and Reports: Utilize logging frameworks and create detailed test reports. Enrolling in a top-rated Selenium course online can unleash the full power of Selenium, offering individuals a deeper understanding of its intricacies.
10. Selenium Grid
Parallel Execution: Set up Selenium Grid to run tests across multiple environments simultaneously.
Configuration: Understand hub and node configuration and how to set up and tear down the Grid.
11. Best Practices
Clean Code: Write readable, maintainable, and reusable code.
Modular Approach: Break tests into smaller, manageable units.
Continuous Integration: Integrate Selenium tests with CI/CD pipelines using tools like Jenkins.
12. Community and Resources
Documentation: Regularly consult the official Selenium documentation.
Community Forums: Engage with the Selenium community through forums, Stack Overflow, and GitHub.
Tutorials and Courses: Leverage online tutorials, courses, and webinars for continuous learning.
Conclusion
Learning Selenium is a journey that involves understanding its core components, setting up the environment, mastering basic and advanced commands, implementing design patterns like POM, integrating with test frameworks, and following best practices. By focusing on these key areas, you can become proficient in automating web testing and other web-related tasks using Selenium. Happy testing!
0 notes
Text
A Guide to Unit Testing Tools: Choosing the Best for Your Project
Unit testing is a critical practice in software development that ensures individual components of code function as expected. Choosing the right unit testing tool can significantly impact test efficiency, maintainability, and overall software quality. In this article, we will explore different unit testing tools, their features, and how they enhance software development workflows.
What is Unit Testing?
Unit testing involves testing individual functions, methods, or components in isolation to verify their correctness. It is the foundation of a robust testing strategy and helps catch bugs early in the development cycle. By testing each unit separately, developers can ensure that every function performs as intended before integrating it into the larger system.
Why Use Unit Testing Tools?
Manually writing and executing tests is inefficient, making unit testing tools essential for automating test execution, assertions, and reporting. These tools improve test coverage, detect regressions, and streamline debugging, allowing teams to release high-quality software with confidence.
Key Features of an Effective Unit Testing Tool
Automation Capabilities: Enables efficient test execution with minimal manual effort.
Mocking and Stubbing Support: Allows isolation of dependencies for accurate testing.
Integration with CI/CD Pipelines: Ensures continuous testing during development.
Code Coverage Analysis: Helps measure test effectiveness and identify untested code.
Top Unit Testing Tools for Different Programming Languages
Unit Testing Tools for Java
JUnit: The most widely used Java testing framework, offering annotations and assertions for writing clean test cases.
TestNG: An advanced testing framework with better support for parallel execution and dependency testing.
Unit Testing Tools for JavaScript
Jest: A zero-config, fast testing framework developed by Facebook, ideal for React and Node.js applications.
Mocha & Chai: A flexible testing framework (Mocha) paired with an assertion library (Chai) for writing structured test cases.
Unit Testing Tools for Python
PyTest: A powerful and simple-to-use testing framework with built-in fixtures and parameterization.
unittest: Python’s standard library for unit testing, offering structured test discovery and execution.
Unit Testing Tools for C#
NUnit: A feature-rich framework similar to JUnit, widely used in .NET applications.
xUnit.net: A modern testing framework focused on extensibility and test performance.
Unit Testing Tools for C++
Google Test (GTest): A robust testing framework offering rich assertion macros and test parameterization.
Boost.Test: A flexible and scalable framework designed for C++ unit testing.
Unit Testing Tools for Go
Go Testing Framework: Built into the Go standard library, providing minimal yet effective unit testing support.
Testify: An extended testing toolkit for Go with powerful assertions and mocking.
How to Choose the Right Unit Testing Tool?
Project Requirements: Choose a tool that aligns with your tech stack and test complexity.
Ease of Use: A tool should have simple syntax and good documentation.
Community Support: Strong community backing ensures continuous updates and issue resolution.
Integration with Other Tools: The tool should integrate seamlessly with CI/CD pipelines, mocking frameworks, and coverage tools.
Best Practices for Unit Testing
Write Clear and Isolated Tests: Each test should validate a single functionality.
Use Mocks and Stubs Wisely: Avoid dependencies to ensure test reliability.
Automate and Run Tests Frequently: Continuous testing prevents regressions.
Measure Code Coverage: Ensure critical parts of the codebase are tested.
Enhancing Unit Testing with Keploy
Keploy is an AI-powered test case generator that simplifies unit testing by automatically generating test cases and mocks. It helps developers achieve higher test coverage without writing test cases manually, reducing the testing burden and improving software quality. Keploy ensures efficient test execution, making it an invaluable tool for modern software development.
Conclusion Unit testing tools play a crucial role in modern software development by ensuring code correctness and stability. By selecting the right tool and following best practices, teams can streamline testing, prevent bugs, and build reliable applications. Whether using JUnit, Jest, PyTest, NUnit, or Keploy, leveraging automation can make unit testing more effective and efficient.
0 notes
Text
Unleash Your Selenium Skills: Mastering Advanced Automation Techniques for Training

Selenium is a game-changer in the world of software testing, empowering testers to automate website testing effortlessly. However, truly mastering Selenium requires delving into its advanced capabilities beyond the basics. This guide, tailored for those undergoing Selenium training, explores advanced automation techniques to enhance your proficiency in Selenium.
Understanding Selenium: Selenium is a powerful tool used for automating web browser interactions. It comprises various components, with Selenium WebDriver being the core for browser automation. Essentially, Selenium enables testers to mimic user actions on websites programmatically.
Advanced Automation Techniques:
Streamline with Page Object Model (POM): Adopting the Page Object Model facilitates organizing test code into reusable components, enhancing maintainability and readability. Each component corresponds to a web page, encapsulating its elements and interactions.
Enhance Testing with Data-Driven Approaches: Data-driven testing empowers testers to execute test cases with multiple datasets, validating application behavior under diverse scenarios. By integrating external data sources, such as Excel sheets or databases, testers can parameterize test data and execute tests efficiently.
Optimize Testing with TestNG: TestNG integration enriches Selenium testing capabilities, offering features like parallel execution, parameterization, and comprehensive reporting. Leveraging TestNG annotations enables effective test management and execution control.
Ensure Compatibility with Cross-Browser Testing: Cross-browser testing is essential to ensure consistent performance across different web browsers. Selenium's compatibility with various browsers, coupled with cloud-based testing platforms, facilitates comprehensive cross-browser testing.
Precision with Advanced Locators: Mastering advanced locator strategies enhances the reliability and efficiency of test scripts. Techniques such as dynamic XPath and CSS selector optimization enable precise element identification, ensuring robust test execution.
Adapt to Dynamic Elements: Selenium provides mechanisms to handle dynamic elements encountered during testing. Techniques like implicit and explicit waits, along with dynamic element identification strategies, ensure stable test execution in dynamic application environments.
Conclusion: Elevating your Selenium skills goes beyond the basics. By embracing advanced automation techniques like the Page Object Model, data-driven testing, TestNG integration, cross-browser testing, advanced locators, and dynamic element handling, you can become proficient in automating complex web application tests. Continuous learning and practice are key to mastering Selenium, especially for those undergoing Selenium training.
Get Software services offering online selenium training and QA certifications. We have certified professionals in IT testing experience in various industries. For more details reach out to us.
Contact:
Canada : +1 905 275 6446
987 King Street East, Suite 103 Hamilton, Ontario L8M 1C6, Canada.
0 notes
Text
Mastering Selenium: A Comprehensive Resource for Effective Web Automation Testing
In the wide spectrum of web development, prime importance is accorded to quality assurance as it guarantees the smooth functioning of applications across various platforms and browsers. Selenium steps into this scene as a significant player with its suite of tools designed for automating web browsers. For professionals in QA and development, becoming proficient in Selenium equates to excelling in maintaining sturdy web applications. Selenium offers a diverse and complete set of tools, particularly useful for tasks like UI testing and regression, although it requires mindful study to be approached correctly. To transition from a Selenium beginner to a maestro, you can consider enrolling in a Selenium Course in Bangalore which provides an in-depth comprehension of crucial Selenium features and practices for a range of web automation tasks.
Plunging into Web Technologies
To master Selenium automation, a thorough understanding of web technologies is fundamental. HTML lays the structure, CSS embellishes it, and JavaScript instills dynamics into a stagnant webpage. Mastery over these three is crucial for seamlessly pinpointing and altering web elements with Selenium. This knowledge shapes both, your perception of web applications and your ability to programmatically control them.
The Language of Selenium – Choose What You Prefer
Selenium's beauty lies in its multilingual capabilities; it converses in the language you find easiest. Be it the object-oriented style of Java, Python’s clear syntax, C#'s sturdiness, or JavaScript's widespread application, you pick your preference. Familiarity with language semantics, syntax, control structures, and exception handling equips you with the tools to script your test cases effectively.
Selenium WebDriver: Navigating Your Test Suite
The Selenium WebDriver goes beyond being a mere tool; it acts as the command center of your automated testing environment. Although setting it up is easy, learning to exploit its full potential demands time and perseverance. It involves understanding how web browsers respond to your instructions, how the WebDriver interacts with browser engines, and how you can execute tasks ranging from simple clicks to more intricate parallel executions.
Locating Strategies: Directing WebDriver
The complex web of elements necessitates a sharp sense of navigation. A diverse set of tools is at your disposal���IDs, classes, names, XPath expressions, and CSS selectors. Each locator caters to a distinct need, and the key to a consistent test is selecting the appropriate one. Striking a balance between accuracy and efficiency is crucial for writing locators resilient to minor UI alterations.
Capitalizing on Test Frameworks: Structuring Your Tests
Frameworks like JUnit, TestNG, and others not only organize test execution but provide context. Assertions validate the expected behavior of our applications, annotations designate the roles of various methods, while reports provide a detailed account of the tests’ successes and failures. The adoption of a framework isn't solely about utilizing tools; it's about crafting a quality assurance narrative for your application.
Controlling the Flight of Time: Managing Synchronization
Races, including web testing, can spring unexpected winners, highlighting race conditions. Synchronization isn’t merely about pausing; it's about orchestrating harmonious interactions. Whether it is using explicit waits for specific conditions or implicit waits that set a baseline patience for the WebDriver, executing scripts smoothly without unnecessary delays requires fine balancing.
The Page Object Model: Organizing Test Blueprints
Envision navigating a labyrinth as intricate as a webpage. The Page Object Model doesn’t merely delineate a route; it generates a comprehensive labyrinth map. By encompassing page elements and interactions within classes, the POM ensures test maintainability and scalability. Further, it separates the test logic from the UI specifics, transforming maintenance from a feared overhaul into a scripted dance.
Test Automation Philosophies: Standardizing Reliable Coding
Scripting test codes mirrors an art form, calling for clean, reusable, and modular code. It requires meaningful naming conventions reflecting their actions and error handling that communicates effectively. The objective isn't limited to automation but extends to establishing an ecosystem where each test script functions like a well-positioned cog in a well-engineered machine, comprehensible, and lasting.
The CI Connection: Maintaining Constant Quality
Incorporating Selenium into CI pipelines using Jenkins or Azure DevOps signifies a cultural shift rather than automation. It embraces a practice where every commit is scrutinized, preventing any regression or bug from going unnoticed. It's about early identification of issues in the development process, reinforcing confidence in deployment.
Beyond Automation: The Art of Exploratory Testing
While Selenium excels in functional testing, exploratory testing thrives in the realm of creativity. Here, human intuition and ingeniousness come into play, discerning nuanced use-cases and user experience issues that algorithms struggle to identify. Automation and exploratory testing function in unison, enriching and informing each other.
Lifelong Learning: Achieving Mastery in Selenium
Becoming a Selenium maestro isn't an overnight feat; it unveils through constant learning and practice. It extends beyond tool familiarity to a deep understanding of its relationship with software development practices, design patterns, and human-computer interaction. It establishes a symbiotic relationship where each advancement in web technology opens a new window for testing, and in return, Selenium guides these technologies towards maturity.
To acquire extensive expertise in using Selenium WebDriver for diverse web automation tasks, contemplate enrolling in Selenium Online Training, which provides community support and job placement assistance.
Conclusion
Emerging as a Selenium expert symbolizes a journey—a fusion of technological comprehension, programming skills, tool mastery, and adherence to best practices. It's about laying foundations, structuring, and incessant innovation to pace with web application development. With concentration, diligence, and a zest for exploration, the ultimate aim of gaining proficiency in Selenium automation testing will stand not just as a testament to your abilities, but a beacon of quality in the software development community.
Take the next step to become a Selenium master, harness power, and shape web application quality into its epitome. And most importantly, remember that just like any craft, it is the commitment to refining the details that breeds excellence.
0 notes
Text
Selenium Webdriver with Java & TestNG Testing Framework
Introduction
Automated testing is crucial for ensuring the quality and reliability of software applications. Selenium WebDriver, combined with the TestNG testing framework, provides a powerful solution for automating web application testing. Together, they offer a comprehensive toolset for developers and testers to create efficient and reliable test scripts.
Understanding Selenium WebDriver
Selenium WebDriver is a popular open-source tool for automating web browsers. It allows developers to interact with web elements and perform actions like clicking buttons, entering text, and navigating pages. Selenium's ability to support multiple browsers and programming languages makes it a versatile choice for web automation.
Key features of Selenium WebDriver include:
Cross-browser compatibility
Support for multiple programming languages
Ability to execute tests on remote machines
Integration with various testing frameworks
Setting Up Selenium WebDriver with Java
To get started with Selenium WebDriver in Java, you need to set up your development environment. Here’s what you need:
Java Development Kit (JDK) installed
Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA
Selenium WebDriver library
Installing and Configuring Selenium in Java:
Install Java and an IDE: Download and install JDK and your preferred IDE.
Download Selenium WebDriver: Add the Selenium WebDriver library to your project. This can be done by adding the Selenium JAR files to your build path.
Set up WebDriver Executables: Download the browser-specific WebDriver executables (e.g., ChromeDriver for Chrome) and set the system properties in your test scripts.
Introduction to TestNG Framework
TestNG is a testing framework inspired by JUnit and NUnit but introduces new functionalities that make it more powerful and easier to use. TestNG provides a range of features that enhance the testing process, such as annotations, parameterization, and support for parallel test execution.
Benefits of using TestNG include:
Annotations for better test organization
Support for data-driven testing
Flexible test configuration
Detailed reporting and logging
Integrating Selenium with TestNG
Integrating Selenium WebDriver with TestNG is straightforward and offers several advantages. By using TestNG, you can organize your test cases into test suites, run them in parallel, and generate comprehensive reports.
To integrate Selenium with TestNG:
Add TestNG to Your Project: Install TestNG in your IDE and add it to your project’s build path.
Write Test Cases: Use TestNG annotations to define test methods, before/after test setup, and teardown.
Run Tests with TestNG: Use the TestNG runner to execute your test cases and view the results.
Debugging and Reporting in TestNG
Debugging test failures is an essential part of the testing process. TestNG provides detailed reports that include the status of each test, the time taken, and any errors encountered. Use these reports to identify and fix issues quickly.
Best Practices for Writing Selenium Tests
To ensure your Selenium tests are efficient and maintainable, follow these best practices:
Use meaningful test names and comments.
Avoid hard-coding values; use variables or configuration files.
Implement a robust test data management strategy.
Regularly update and refactor test scripts.
Managing Test Suites in TestNG
TestNG allows you to group multiple test cases into test suites. This is done using XML configuration files that specify the test classes and methods to run. Managing test suites helps organize tests and improve test execution control.
Continuous Integration with Selenium and TestNG
Integrating Selenium and TestNG with continuous integration (CI) tools like Jenkins enables automated test execution as part of the development pipeline. Set up CI/CD pipelines to automatically build, test, and deploy your applications, ensuring high quality and reliability.
Challenges and Limitations in Selenium Testing
Selenium testing can present challenges, such as handling dynamic web elements, managing test data, and maintaining tests. Solutions include using explicit waits, implementing page object models, and continuously updating tests.
Conclusion
Selenium WebDriver and TestNG together provide a powerful solution for automated web testing. They offer flexibility, scalability, and a wide range of features to enhance the testing process. Embracing these tools enables developers and testers to create robust, efficient, and reliable automated tests.
0 notes