#newstring
Explore tagged Tumblr posts
Text
scuffed nanaya dub current workflow below for the few freaks who find that stuff interesting/for my own memorization ↓↓↓
Translation
I made a spreadsheet with every voice file and their line listed (transcribed via soundboard playing them into Google translate or using a romaji to hiragana converter), and have been taking those lines and running them through different translation sites. Google Translate is one of them as mentioned before but, DeepL has especially been used in the process. Along with that, I've found myself opening Jisho to double check translations when unclear.
Though, as I don't fully know what moves these correspond to until in-game, they do result in some awkward 'two-parter' moves.
Direction
This has been the easiest part as I'm just able to listen to the tone of the original line and reference it for my own delivery. With Nanaya, I've tried to give him that somewhat deep voice like heard in his japanese counterpart, though didn't quite follow through for all lines (which I'll try to go through and fix). Though, it's a little weird going into that range, despite it being right in my natural tone.
Recording + Effect Chain
I use a SM57 routed into a Scarlett which I don't seem to remember the model name for. I'm recording into Audacity with the Scarlett dial at 3 o'clock, then I run my audio through a macro that does as follows: Loudness Normalization (Perceived Loudness, -19 LUFs), Noise Reduction (depends on your setup).
The audio files once all recorded are all imported into FL Studio and ran through a channel with the Fruity Multiband Compressor preset 'Vocal' with minor adjustments to the gain dials (especially the high to emulate the crunch). I'd probably turn the channel up to where it's in-between it's max & default position.
Exporting & Naming
This part is the one that sucked the most. Be sure to make sure all the FL tracks are the same name as the project, which can be done easily by double clicking on that bar inbetween the track name and audio, and right clicking on any track, clicking on "Auto name."
From there, export all playlist tracks into a folder. Now, here's the issue that made me avoid using FL for this type of stuff.
ALL THE FILES HAVE THE PROJECT NAME AT THE START!!!
Chillax, go open Powershell and put in as follows:
"C:\...." is the directory where your exported audio is, cd stands for change directory. This is so Powershell is only looking there. The end string is long so here's it in copyable text.
Get-ChildItem *.* | Rename-Item -NewName {$_.Name -replace "StringtoReplace", "newString"}
In this case, the Stringtoreplace is "nanaya voice - ", and the newString is "".
Importing
First thing I learnt when I started this was that "0005.p" in Melty's directory is where audio files are stored, and that if you exported it's contents using ghmr93 (found on the Mizuumi wiki page for MBAACC, in the links section called "File Extractor") and moved replaced the original "0005.p" with the newly extracted "se" folder, the game would run it's audio from the se folder.
Simply replace the specific character audio in battle_se with your new audio under the same file names.
And that's just about all for this post, as it's 1:42am as I'm writing this part of the text. Oh and I accidentally deleted the Tohno win quotes ...and will have to rerecord them, though I did plan to just straight up redo them all anyways, so.
9 notes
·
View notes
Photo

#sitstrings #newstring #guitar https://www.instagram.com/p/CKQeAgDFSk2/?igshid=1we8ksj77h0vq
0 notes
Video
instagram
Sequence shortened 🏂 I also sang some songs today, thank you to everyone who supported me out there on my first proper busk. Found some really delicious CBD things at the vegan market and I'm looking forward to sharing them all with you in the next few days
(at Quedam Shopping Centre, Yeovil)
https://www.instagram.com/p/BuhD7rGFVnr/?utm_source=ig_tumblr_share&igshid=1e569wd1oohz
#stringchange#newstring#brokenstring#brokeastring#brokenstrings#busking#buskers#buskerslife#buskinglife#busk#singitout#veganmarket#yeovil#somerset#cbdmovement#cbdhelps#veganstoner#vegansdoitbetter#cbdisthefuture#veganisthefuture#hempisthefuture#cbdismedicine#busker#singingformoney#liveformusic#musicforlife#liveforlivemusic#fortheloveofmusic#passionformusic#musicforthepeople
0 notes
Video
instagram
#busybusy #guitarintonation #newstrings #roughcut... #musiclife #musician #guitar #bass #musicproducer #music4life #evaderknives https://www.instagram.com/p/CTQakqVjV7q/?utm_medium=tumblr
#busybusy#guitarintonation#newstrings#roughcut#musiclife#musician#guitar#bass#musicproducer#music4life#evaderknives
1 note
·
View note
Video
🎶Bad Liar - Imagine Dragons Recently replaced my nylon strings with titanium strings and definitely still getting used to how they feel, but mannn I love the sound of fresh strings ✨ • • • • • • • #badliar #imaginedragons #ukulele #ukulelecover #luna #lunauke #lunaukulele #lunahightide #lunahightideconcertkoa #badliarcover #cover #vocals #fun #love #life #newstrings #origins #beautiful #home #adventure #imaginedragonscover #keychange #titaniumstrings https://www.instagram.com/p/B33hKxiJ5k6/?igshid=1d7fs4nn739hq
#badliar#imaginedragons#ukulele#ukulelecover#luna#lunauke#lunaukulele#lunahightide#lunahightideconcertkoa#badliarcover#cover#vocals#fun#love#life#newstrings#origins#beautiful#home#adventure#imaginedragonscover#keychange#titaniumstrings
6 notes
·
View notes
Photo
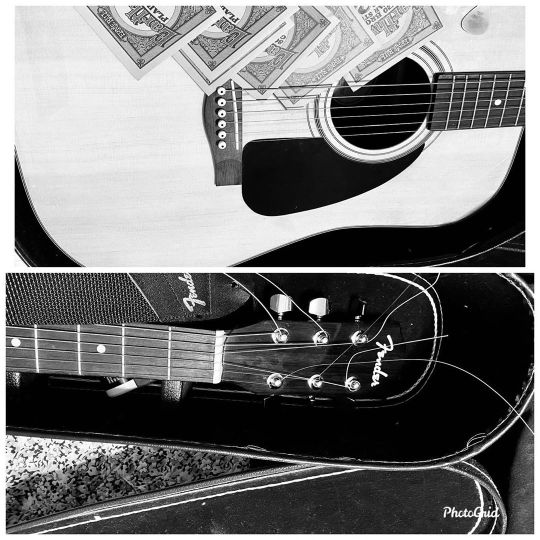
So this happened on a #rainyday day and good songs inspired me today. #newstrings #ernieball #fender #fenderguitars #guitar #acousticguitar #30afest #imstilllearning #freefalling #tompetty #sundayvibes (at Secret Spot) https://www.instagram.com/p/B7zMpD5jyvX/?igshid=s64nztj89ngh
#rainyday#newstrings#ernieball#fender#fenderguitars#guitar#acousticguitar#30afest#imstilllearning#freefalling#tompetty#sundayvibes
1 note
·
View note
Photo

New string day! #bassist #bassplayer #laklandbass #drstrings #ernieball #rocknroll #crispstrings #newstrings https://www.instagram.com/p/B4iFGPpA5J8/?igshid=ubwoy55qw73d
1 note
·
View note
Photo

Time to try out some new strings #fender #daddario #nyxl #telecaster #fendertelecaster #tele #newstrings #music #singersongwriter #guitarplayer #guitarist #guitaristsofinstagram #musician #homestudio #recording #newmusic https://www.instagram.com/p/B4QYcJIJKD7/?igshid=xx2rqstxxlua
#fender#daddario#nyxl#telecaster#fendertelecaster#tele#newstrings#music#singersongwriter#guitarplayer#guitarist#guitaristsofinstagram#musician#homestudio#recording#newmusic
1 note
·
View note
Video
New original called “Empty Promises” #originalmusic #musicnow #lyricslater #musiciansofinstagram #guitaristofinstagram #guitarist #epiphone #wildkat #epiphonewildkat #electricguitar #newstrings #riffs (at Providence, Rhode Island) https://www.instagram.com/p/B0hWlHJg-f2/?igshid=9ibuqvku0t7h
#originalmusic#musicnow#lyricslater#musiciansofinstagram#guitaristofinstagram#guitarist#epiphone#wildkat#epiphonewildkat#electricguitar#newstrings#riffs
1 note
·
View note
Photo

New strings! #music #guitar #fender #newstrings #newsounds #spring https://www.instagram.com/p/BwaHkxXhzHY/?igshid=1tc22hu25bntn
3 notes
·
View notes
Photo

Put new strings on my acoustic, I love these!! 🎶🎸 #fender345ce #fenderguitar #fenderguitars #newstrings #acousticguitarist #acousticstrings (at Waco, Texas) https://www.instagram.com/p/CitfU2WM6h7/?igshid=NGJjMDIxMWI=
0 notes
Photo

I love having #newstrings feels like fresh clean line-dried and ironed bedding #luthier (at Saint Leonards, East Sussex, United Kingdom) https://www.instagram.com/p/Ciss-nCo79Y/?igshid=NGJjMDIxMWI=
0 notes
Photo

Also just back in - the Troublemaker Tele, now sporting .10’s! #fender #fenderguitars #troublemakertele #troublemakertelecaster #newstrings #heaviergauge #icebluemetallic #paralleluniverse #telecaster #telecasterdeluxe
#fender#fenderguitars#troublemakertele#troublemakertelecaster#newstrings#heaviergauge#icebluemetallic#paralleluniverse#telecaster#telecasterdeluxe
4 notes
·
View notes
Photo

Saturday’s are for sound holes and new strings... #itsaboutdamntime #newstrings #takamine #takamineguitars #sonostrings #acousticguitar #guitar #guitarplayer #saturdayvibes #saturday #guitarmaintenance (at Guilford, Indiana)
#saturday#guitar#takamineguitars#sonostrings#takamine#saturdayvibes#newstrings#guitarmaintenance#itsaboutdamntime#guitarplayer#acousticguitar
1 note
·
View note
Photo

These stallions are freshly laced up, cleaned up, and ready to boogie! #newstrings #tagteam https://www.instagram.com/p/CRl1ZwFHZZ1/?utm_medium=tumblr
0 notes
Video
instagram
Testing out the new strings! Or course the one time I decide to change my strings I can't find my winder....ha! #fender #stratocaster #newstrings #springcleaning #wishiknewwheremystringwinderwas #clapton https://www.instagram.com/p/BwaPYJshQyV/?igshid=90ccbuhb6sxq
2 notes
·
View notes