#createports
Explore tagged Tumblr posts
Text
Optimizing React Performance: Tips for Faster and More Efficient Web Apps
Introduction
React, the popular JavaScript library for building user interfaces, has become a go-to choice for many developers due to its efficient rendering, component-based architecture, and rich ecosystem. However, as applications grow in complexity, maintaining optimal performance can become a challenge. In this article, we'll explore a range of techniques and best practices to help you optimize the performance of your React applications, ensuring they are fast, responsive, and efficient.
Memoization with React.memo()
One of the most effective ways to improve React performance is through the use of memoization. The React.memo() higher-order component (HOC) can be used to wrap functional components, preventing unnecessary re-renders when the component's props have not changed.import React, { memo } from 'react'; const MyComponent = memo(({ prop1, prop2 }) => { // component implementation });
By wrapping your components with React.memo(), React will skip re-rendering the component if its props have not changed, leading to significant performance improvements, especially for expensive or frequently rendered components.
Leveraging Virtualization with react-virtualized
When dealing with large datasets or long lists, traditional rendering can become slow and inefficient. React-virtualized is a powerful library that implements virtualization, rendering only the visible items in the viewport and dynamically loading more as the user scrolls. This technique can dramatically improve the performance of your application, especially when working with large data sets.import { List } from 'react-virtualized'; <List width={500} height={400} rowCount={1000} rowHeight={50} rowRenderer={({ index, key, style }) => ( <div key={key} style={style}> Row {index} </div> )} />
Code Splitting and Lazy Loading
Code splitting is a technique that allows you to split your application's code into smaller, more manageable chunks. This can be particularly beneficial for large applications, where loading the entire codebase upfront can lead to slow initial load times. By implementing code splitting and lazy loading, you can ensure that only the necessary code is loaded when it's needed, improving the overall performance of your application.import { lazy, Suspense } from 'react'; const MyComponent = lazy(() => import('./MyComponent')); <Suspense fallback={<div>Loading...</div>}> <MyComponent /> </Suspense>
Optimizing Rendering with React.PureComponent and shouldComponentUpdate
In class-based components, you can use React.PureComponent or implement the shouldComponentUpdate lifecycle method to control when a component should re-render. These techniques can help you avoid unnecessary re-renders, leading to improved performance.import React, { PureComponent } from 'react'; class MyComponent extends PureComponent { // component implementation }
Leveraging React.Fragment and Portals
React.Fragment allows you to group multiple elements without adding an extra DOM node, which can improve the efficiency of your component tree. Additionally, React Portals provide a way to render components outside the main component tree, which can be useful for modals, tooltips, and other UI elements that need to be positioned independently.import { Fragment, createPortal } from 'react'; return ( <Fragment> {/* component content */} </Fragment> {createPortal( <div className="modal">Modal content</div>, document.body )} );
Conclusion
Optimizing the performance of your React applications is crucial for providing a smooth and responsive user experience. By implementing techniques like memoization, virtualization, code splitting, and efficient rendering, you can ensure that your web apps are fast, efficient, and scalable. Remember, performance optimization is an ongoing process, and it's essential to continuously monitor and optimize your application as it grows and evolves. In conclusion, optimizing the performance of your React applications is essential for delivering a seamless user experience. By implementing techniques like memoization, virtualization, and efficient rendering, you can ensure your web apps are fast and responsive. For further enhancements or to explore advanced features, consider partnering with a reputable React.js development company or hire React.js developers to take your projects to the next level.
0 notes
Photo
Grow your #Business 2X Faster with An AGILE, End to End #Ecommerce solution Provider. More https://bit.ly/2KbTQMT
#TestwareInformatics #MagentoEcommerce #CreatePortal #websiteBuilder #Webdesigncompany
#TestwareInformatics#MagentoEcommerce#CreatePortal#websiteBuilder#Webdesigncompany#Business#technology#webdesign#web#software#magento#magento website development#people#public#usef
0 notes
Photo

I T S B R U T A L O U T H E R E 🎵 ___________________________ LOCATION @saadiyatrotana WITH @nowunited THOUGHT Got a broken ego, broken heart (It's brutal out here, it's brutal out here) And God, I don't even know where to start -Lyrics Olívia Rodrigo 💭 it’s over 40 degrees out here 💥 ______________________ CLICK BIO ✖️ All Social Media ✖️ IGTV ✖️See You There✖️ REELS ✖️A few songs ✖️ ______________________ - - - - - - - - - - - - _____________________ #poolside #goldenhour #sunsets #united #nowunited #pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #ig_podiumportraits #pursuitofportrait #feauturepalette #discoverportrait #bravogreatphoto #bevisuallyinspired #moodyports #portraitcentral #shotzdelight #createports #singerslife _________________ (at Saadiyat Rotana Resort & Villas) https://www.instagram.com/p/CQ1m-26tlD6/?utm_medium=tumblr
#poolside#goldenhour#sunsets#united#nowunited#pursuepretty#portrait_shots#girlportrait#portrait_star#portrait_vision#quotestoliveby#collectivetrend#australiangirl#portraitsru#ig_podiumportraits#pursuitofportrait#feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight#createports#savannahclarke#singerslife
13 notes
·
View notes
Text
Gutenberg Components: Modal
Modals are really common in any user interface. It can be used for alerts, to render additional information or provide a way to enter data. In this tutorial, we will learn about the Gutenberg Component Modal and see what options does it provides to us. If you’re interested in reading the source code of this component, head to the repository. A great thing about this component is that you can call it anywhere in your application, but the Modal will still load in the parent node of the DOM, so it won’t be affected by any CSS rules such as overflow:hidden and such. This is done because this component uses the function createPortal(). You can read about this in the React documentation. The Gutenberg component Modal is built using: IsolatedEventContainer – prevents certain events from propagating outside of the container; accepts ModalFrame, ModalFrame – accepts ModalHeader and all children elements, ModalHeader – shows the modal header with a close button. Modal Properties There are several properties which you can pass to the Modal component: title – used in modal header, onRequestClose – a function that is called when the modal should be closed, Source: https://managewp.org/articles/18406/gutenberg-components-modal
from Willie Chiu's Blog https://williechiu40.wordpress.com/2019/02/28/gutenberg-components-modal/
0 notes
Text
Gutenberg Components: Modal
Modals are really common in any user interface. It can be used for alerts, to render additional information or provide a way to enter data. In this tutorial, we will learn about the Gutenberg Component Modal and see what options does it provides to us. If you’re interested in reading the source code of this component, head to the repository. A great thing about this component is that you can call it anywhere in your application, but the Modal will still load in the parent node of the DOM, so it won’t be affected by any CSS rules such as overflow:hidden and such. This is done because this component uses the function createPortal(). You can read about this in the React documentation. The Gutenberg component Modal is built using: IsolatedEventContainer – prevents certain events from propagating outside of the container; accepts ModalFrame, ModalFrame – accepts ModalHeader and all children elements, ModalHeader – shows the modal header with a close button. Modal Properties There are several properties which you can pass to the Modal component: title – used in modal header, onRequestClose – a function that is called when the modal should be closed, from ManageWP.org https://managewp.org/articles/18406/gutenberg-components-modal
0 notes
Text
5 ReactJS Expert Advice https://ift.tt/2IR9eca
5 ReactJS Expert Advice
Learn how to make the most of the most common JavaScript structure on the web.
ReactJS expert advice React has become one of the most common tools in the Web Developer's Library. Get yourself a set of basic techniques that will improve your code output.
01. Higher-order components
Often, the components share functions with each other, such as registration requests or the network. Can be difficult to maintain, because the number using this logic is increasing. Developers are encouraged to strip from public law and include it when necessary. In the traditional JavaScript application, the concept of the top-ranking function is one way to approach it. In short, these are jobs that take on other functions like arguments and unfair behavior on them. Examples of such methods are routes such as map and filter. Higher Order Components (HOC) - This is the Reactant method to achieve the same thing. These components give behavior to the transferred component.
const Logger = WrappedComponent => ( class Logger extends Component { componentDidMount() { console.log(‘mounted’); } render() { return <WrappedComponent {...this.props} /> } } ); export default Logger(MyComponent);
Export by default Logger (MyComponent); In this example, here the function returns a new wrapped component that displays the original text that was sent with any details. HOCs are regular components and can do everything in their power, for example, to transfer their own requirements and connect to life cycle retrieval. Then the function inserts the parent when exporting. Using HOC, it simplifies maintenance and testing of the most commonly used code fragments. When certain functions are required, it is easy to see, safe, knowing that they will get as expected.
02. Container and presentational components
It is always important to keep the problems separate when developing different parts of the application. If possible, keep data sampling away from view. React uses a similar concept when it comes to components and presentation components.
class ProductsContainer extends Component { [...] componentDidMount() { fetchProducts() .then(products => this.setState({products})); } render() { return <Products products={this.state.products} />; } }
Here, the products are extracted by an external method inside the container, stored inside its storage warehouse, and then transported as reinforcements to the product display component that it displays. The container component does not know how to display the information, and only how to retrieve and configure the information is known. Similarly, the rendering component does not know the data source. The function of any component can be changed without affecting the other. There may be another section of the application - or a completely separate application - that can use the same presentation component. Because it works exclusively on requirements, each part of the app will have to create a container around itself. This approach also simplifies unit testing. In container tests, you only need to worry about the status to be created, while the information element can be moved to static details to achieve the expected result.
03. Error boundaries
No matter how well the project is integrated, the bugs still find a way. This can happen in very specific circumstances, it is difficult to diagnose and leave the application in an unstable state - an absolute nightmare for users and developers. React v16 was released in September 2017. As part of this update, any unhandled errors now unload the entire application. To counter this, the concept of error limits was introduced. When an error occurs in the component, the bubble will appear through the parent until you reach the root of the application, where it will be disabled, or find the component that will handle the error. The idea of the limits of error is a common element that cares about mistakes for their children.
componentDidCatch(error, info) { this.setState({ error: error.message }); } render() { return this.state.error ? <ErrorDisplay/> : this.props.children; }
If you connect to a new life cycle, the error may be
04. Portals
There are times when the component must escape from the parent, somewhere in the DOM. Conditional frames, for example, refer to the top level of the page to avoid problems with z-index and positioning. The portals are also part of version 16, which allows React to display components in a completely separate DOM nodes from the rest of the application. The content will remain in the React structure, but will be displayed elsewhere. This means that any event that is triggered inside the gate will flow through the original in the reactor, not in the container element of the gate itself.
ReactDOM.createPortal( this.props.children, document.getElementById(‘modal’), );
When configuring the selected component, the portal can be restored by the display function. When the content is displayed, it can be wrapped in this component, then displayed in another item.
05. CSS with styled-components
An application design with reusable components can cause problems with conflicting category names. Conventions such as BEM help alleviate this problem, but aim to treat symptoms rather than problems. Components can implement their own styles. This means they have a way to adjust the visual effects "on the fly" without having to use built-in patterns or chapter keys. One of these solutions is a minimalist component that uses JavaScript to its advantage. As the name suggests, instead of creating classNames, it creates completely new ready components. The system uses template tags with ES2015 tags that can accept plain CSS and apply them to the desired element.
const Button = styled.button` font-size: 2rem; background: ${props => props.primary ? ‘#3CB4CB’ : ‘white’}; `;
With the placeholder, the style can be dynamically changed. In this example, the background of the button changes depending on whether the button is moved to Basic Support or not. You can use any expression to calculate the desired style. The created component can be used the same way as any other item, and any items will be moved. Custom components can also be created the same way, instead of using the (ComponentName) style.
from Blogger https://ift.tt/2KsLbpP via IFTTT
0 notes
Photo

🎄 . . . . . . . . . . #bsas #argentina #loves_latino #loves_americas #instagramers #instagrames #igersbsas #igersargentina #ig_argentina #ig_all_americas #argentina360 #agameoftones #ig_buenosaires #argentina_ig #ig_cameras_united #watchthisinstagood #igs_america #igshotz #ig_sharepoint #hallazgosemanal #portrait #argentina_estrella #visualoflife #vivirenbuenosaires #govyrl #createports #loves_buenosaires #loves_argentina_
#ig_all_americas#portrait#vivirenbuenosaires#argentina360#createports#igersargentina#igersbsas#argentina#agameoftones#loves_argentina_#ig_argentina#ig_sharepoint#hallazgosemanal#govyrl#ig_buenosaires#argentina_estrella#ig_cameras_united#igs_america#loves_latino#argentina_ig#watchthisinstagood#igshotz#instagramers#bsas#visualoflife#loves_buenosaires#loves_americas#instagrames
0 notes
Photo

T H E S U N ___________________________ LOCATION Abu Dhabi WITH @nowunited THOUGHT Whatever makes you feel the sun from the inside out Chase that ! ______________________ CLICK BIO ✖️ All Social Media ✖️ IGTV ✖️See You There✖️ REELS ✖️A few songs ✖️ ______________________ - - - - - - - - - - - - _____________________ #poolside #goldenhour #sunsets #united #nowunited #pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #ig_podiumportraits #pursuitofportrait #feauturepalette #discoverportrait #bravogreatphoto #bevisuallyinspired #moodyports #portraitcentral #shotzdelight #createports #singerslife _________________ (at Saadiyat Beach Club) https://www.instagram.com/p/CQHT4DAtu52/?utm_medium=tumblr
#poolside#goldenhour#sunsets#united#nowunited#pursuepretty#portrait_shots#girlportrait#portrait_star#portrait_vision#quotestoliveby#collectivetrend#australiangirl#portraitsru#ig_podiumportraits#pursuitofportrait#feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight#createports#savannahclarke#singerslife
4 notes
·
View notes
Photo

P O O L D A Z E ___________________________ LOCATION WITH @nowunited THOUGHT Sometimes sitting and doing nothing is the best something you can do. -Karen Salmansohn ______________________ CLICK BIO ✖️ All Social Media ✖️ IGTV ✖️See You There✖️ REELS ✖️A few songs ✖️ ______________________ - - - - - - - - - - - - _____________________ #poolside #goldenhour #sunsets #united #nowunited #pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #ig_podiumportraits #pursuitofportrait #feauturepalette #discoverportrait #bravogreatphoto #bevisuallyinspired #moodyports #portraitcentral #shotzdelight #createports #singerslife _________________ (at Saadiyat Beach Club) https://www.instagram.com/p/CQGmjQcN8Da/?utm_medium=tumblr
#poolside#goldenhour#sunsets#united#nowunited#pursuepretty#portrait_shots#girlportrait#portrait_star#portrait_vision#quotestoliveby#collectivetrend#australiangirl#portraitsru#ig_podiumportraits#pursuitofportrait#feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight#createports#savannahclarke#singerslife
5 notes
·
View notes
Photo

P E A C E ______________________ PHOTO FujiFilm THOUGHTS A simple smile. That’s the start of opening your heart and being compassionate to others. - Dalai Lama ____________________ CLICK BIO ✖️All social media - Facebook Spotify Soundcloud ... 🔝🔝🔝🔝🔝🔝🔝🔝 ______________________ - - - - - - - - - - - - - ______________________ #pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #skivvy#simplicity#actressinthemaking#kings_shots#ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #actresslife#clickbio _______________________ (at Sydney, Australia) https://www.instagram.com/p/CAqzp1GHKiK/?igshid=1i56hfyu8x0tt
#pursuepretty#portrait_shots#girlportrait#portrait_star#portrait_vision#quotestoliveby#collectivetrend#australiangirl#portraitsru#skivvy#simplicity#actressinthemaking#kings_shots#ig_podiumportraits#pursuitofportrait#feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight#createports#savannahclarke#singerslife#actresslife#clickbio
14 notes
·
View notes
Video
instagram
W H A T D O Y O U C H O O S E ? ______________________ PHOTO FujiFilm THOUGHTS We are who we choose to be - Green Goblin ____________________ CLICK BIO ✖️All social media - Facebook Spotify Soundcloud ... 🔝🔝🔝🔝🔝🔝🔝🔝 ______________________ - - - - - - - - - - - - - ______________________ #pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #skivvy #simplicity#choices#kings_shots#ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #actresslife#clickbio _______________________ (at Sydney, Australia) https://www.instagram.com/p/CAqehBfnR2q/?igshid=1wfgavh3i35z9
#pursuepretty#portrait_shots#girlportrait#portrait_star#portrait_vision#quotestoliveby#collectivetrend#australiangirl#portraitsru#skivvy#simplicity#choices#kings_shots#ig_podiumportraits#pursuitofportrait#feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight#createports#savannahclarke#singerslife#actresslife#clickbio
8 notes
·
View notes
Photo

A L L T H E R E _____________________ PHOTO IPhone LOCATION Sydney Australia THOUGHT Wherever you are Be all there -Jim Elliot ______________________ CLICK BIO ✖️All social media ✖️ IGTV ✖️See you there ✖️ ______________________ - - - - - - - - - _____________________ #makeuplooks #purplevibes #everydayproject #wednesdayvibes #portraitphotography #portrait_mood #teenchoice #australiangirl #australianmade #blueeyes #purpkeeyeshadow #glammakeup #strengthquotes #savannahclarke #kdportraitgallery#aussieactress#australiangirl##collectivesoul #pursuitofportrait #discoverportrait#bravogreatphoto#moodyports#portraitcentral#shotzdelight #createports #singersongwriter #singerslife#musiclover#discoverportraits #portraits_mf _____________________ (at Sydney, Australia) https://www.instagram.com/p/CAYxlcDHgN2/?igshid=1n3wpd6kpa61k
#makeuplooks#purplevibes#everydayproject#wednesdayvibes#portraitphotography#portrait_mood#teenchoice#australiangirl#australianmade#blueeyes#purpkeeyeshadow#glammakeup#strengthquotes#savannahclarke#kdportraitgallery#aussieactress#collectivesoul#pursuitofportrait#discoverportrait#bravogreatphoto#moodyports#portraitcentral#shotzdelight#createports#singersongwriter#singerslife#musiclover#discoverportraits#portraits_mf
6 notes
·
View notes
Photo
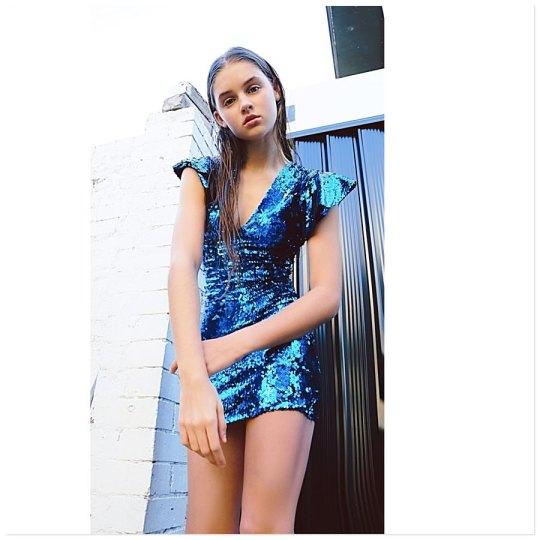
I N F I N I T Y __________________________________________ PHOTO FujiFilm STYLE @worshiphernow THOUGHTS We have our own language you and me. One that doesn’t have a word for goodbye - j m storm ________________________________________ CLICK BIO ✖️All social media - Facebook Spotify Soundcloud … __________________________________________ - - - - - - - - - - - - - __________________________________________ #pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #glitterdress#simplicity#actressinthemaking#kings_shots#ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #actresslife#clickbio ___________________________________________ (at Sydney, Australia) https://www.instagram.com/p/B4xsXGFno9z/?igshid=1bsmlthj6fbhd
#pursuepretty#portrait_shots#girlportrait#portrait_star#portrait_vision#quotestoliveby#collectivetrend#australiangirl#portraitsru#glitterdress#simplicity#actressinthemaking#kings_shots#ig_podiumportraits#pursuitofportrait#feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight#createports#savannahclarke#singerslife#actresslife#clickbio
32 notes
·
View notes
Text

L I F E
_________________________________________
PHOTO FujiFilm
THOUGHTS
We meet no ordinary people in our life
- CS Lewis
______________________________________
CLICK BIO ✖️All Social Media ✖️
_______________________________________
-
-
-
-
-
-
______________________
#pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #greentops#simplicity#actressinthemaking#kings_shots#ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #greentop#savannahclarke #singerslife #actresslife#clickbio
__________________________________________
https://www.instagram.com/p/B6EGW2FnSuG/?hl=en
https://mobile.twitter.com/hashtag/collectivetrend?src=hashtag_click
https://compiled.social/SAVANNAH
17 notes
·
View notes
Photo

M E A N T T O B E _______________________ PHOTO FujiFilm LOCATION Sydney Australia THOUGHT You may not end up Where you thought you were going But you will always end up where you are meant to be. __________________________ IGTV📺see you there📺 CLICK BIO ✖️New Song- Wake Up ✖️ ______________________ - - - - - - - - - - - - - - ______________________ #portrait_mood #pinkmood #candypink#portrait_ig #pursuitofportraits #portrait_shots #collectivetrend #kings_portraits #everydayproject #kdportraitgallery#aussieactress#australiangirl#australiansinfilm #collectivesoul #pursuitofportrait #discoverportrait#bravogreatphoto#moodyports#portraitcentral#shotzdelight #createports #australianactor#actor_jg #singersongwriter #singerslife#musiclovers#actorslife#discoverportraits #portraits_mf #savannahclarke _____________________ (at Sydney, Australia) https://www.instagram.com/p/B-xdRfoHq2K/?igshid=dplrbdt4gmyb
#portrait_mood#pinkmood#candypink#portrait_ig#pursuitofportraits#portrait_shots#collectivetrend#kings_portraits#everydayproject#kdportraitgallery#aussieactress#australiangirl#australiansinfilm#collectivesoul#pursuitofportrait#discoverportrait#bravogreatphoto#moodyports#portraitcentral#shotzdelight#createports#australianactor#actor_jg#singersongwriter#singerslife#musiclovers#actorslife#discoverportraits#portraits_mf#savannahclarke
5 notes
·
View notes
Text


T R U S T
_________________________________________
PHOTO IPhone X
STYLE
@worshiphernow
THOUGHTS
Sometimes you don’t know why
You just have to trust the feeling.
-Kristen Butler
________________________________________
CLICK BIO ✖️All social media ✖️
________________________________________
-
-
-
-
-
-
-
-
-
-
-
-
-
_______________________________________
#pursuepretty #portrait_shots #girlportrait #portrait_star #portrait_vision #quotestoliveby #collectivetrend #australiangirl #portraitsru #glitterdress#simplicity#actressinthemaking#kings_shots#ig_podiumportraits #pursuitofportrait #feauturepalette#discoverportrait#bravogreatphoto#bevisuallyinspired#moodyports#portraitcentral#shotzdelight #createports #savannahclarke #singerslife #actresslife#clickbio
_________________________________________
_
instagram
9 notes
·
View notes