#TypeScript includes a set of built-in types
Explore tagged Tumblr posts
Text

#To help with string manipulation#TypeScript includes a set of built-in types#which can be used in the manipulation of casing in string types. - Uppercase<StringType> converts each character in the string to the uppe#follow @capela.dev for weekly posts on web development with JavaScript. ----- typescript javascript programming developer ts js np
0 notes
Text
For the #PlayStation7 UI framework, we can start with a skeleton of the structure that allows for intuitive navigation, personalization, accessibility features, interactive tutorials, and feedback mechanisms. This foundation will give us a clear and modular layout for managing and expanding each component.
I’ll break down each area and propose a code structure for a scalable and flexible UI system. Since this is for a game console, it would likely be built using a high-performance front-end framework like React or Unity UI Toolkit if we want C# for seamless integration. I’ll draft this in React with TypeScript for scalability and reusability.
1. Base UI Component Structure
The UI can be organized in a hierarchy of components that handle each aspect:
// App.tsx - Main entry point
import React from 'react';
import { NavigationMenu } from './components/NavigationMenu';
import { Personalization } from './components/Personalization';
import { Accessibility } from './components/Accessibility';
import { Tutorials } from './components/Tutorials';
import { Feedback } from './components/Feedback';
export const App: React.FC = () => {
return (
<div className="ps7-ui">
<NavigationMenu />
<Personalization />
<Accessibility />
<Tutorials />
<Feedback />
</div>
);
};
Each component would encapsulate its own logic and state, allowing for modular updates and improved maintainability.
2. Intuitive Navigation
The NavigationMenu component could use React Router to manage routes for easy navigation between games, apps, and settings. Quick access can be achieved using a menu structure that includes hotkeys or icons.
// components/NavigationMenu.tsx
import React from 'react';
import { Link } from 'react-router-dom';
export const NavigationMenu: React.FC = () => {
return (
<nav className="navigation-menu">
<Link to="/home">Home</Link>
<Link to="/games">Games</Link>
<Link to="/apps">Apps</Link>
<Link to="/settings">Settings</Link>
</nav>
);
};
3. Personalization Options
The Personalization component could offer theme and layout options. We can use context for storing and accessing user preferences globally.
// components/Personalization.tsx
import React, { useState, useContext } from 'react';
import { UserContext } from '../context/UserContext';
export const Personalization: React.FC = () => {
const { userPreferences, setUserPreferences } = useContext(UserContext);
const handleThemeChange = (theme: string) => {
setUserPreferences({ ...userPreferences, theme });
};
return (
<div className="personalization">
<h3>Customize Your Experience</h3>
<select onChange={(e) => handleThemeChange(e.target.value)}>
<option value="dark">Dark</option>
<option value="light">Light</option>
</select>
</div>
);
};
4. Accessibility Features
The Accessibility component includes support for voice commands, color blindness options, and mobility support. We can add accessibility settings to UserContext.
// components/Accessibility.tsx
import React, { useContext } from 'react';
import { UserContext } from '../context/UserContext';
export const Accessibility: React.FC = () => {
const { accessibilityOptions, setAccessibilityOptions } = useContext(UserContext);
const toggleVoiceCommands = () => {
setAccessibilityOptions({ ...accessibilityOptions, voiceCommands: !accessibilityOptions.voiceCommands });
};
return (
<div className="accessibility">
<h3>Accessibility Settings</h3>
<label>
Voice Commands
<input type="checkbox" checked={accessibilityOptions.voiceCommands} onChange={toggleVoiceCommands} />
</label>
</div>
);
};
5. Interactive Tutorials
The Tutorials component will guide new users through the setup. You can create individual tutorial steps as components or objects within an array.
// components/Tutorials.tsx
import React, { useState } from 'react';
export const Tutorials: React.FC = () => {
const [currentStep, setCurrentStep] = useState(0);
const tutorialSteps = [
"Welcome to PlayStation 7",
"How to Navigate",
"Personalization Settings",
"Feedback and Support",
];
return (
<div className="tutorials">
<h3>{tutorialSteps[currentStep]}</h3>
<button onClick={() => setCurrentStep((prev) => (prev + 1) % tutorialSteps.length)}>Next</button>
</div>
);
};
6. Feedback Mechanisms
The Feedback component enables users to submit feedback. This could involve a backend integration for storing data and using WebSocket for real-time updates.
// components/Feedback.tsx
import React, { useState } from 'react';
export const Feedback: React.FC = () => {
const [feedback, setFeedback] = useState("");
const handleSubmit = () => {
// Logic to send feedback to the server
console.log("Feedback submitted:", feedback);
};
return (
<div className="feedback">
<h3>Give Us Your Feedback</h3>
<textarea value={feedback} onChange={(e) => setFeedback(e.target.value)} />
<button onClick={handleSubmit}>Submit</button>
</div>
);
};
7. Context for Global State
A UserContext can hold user preferences, accessibility settings, and other globally accessible states, making the app reactive and user-tailored.
// context/UserContext.tsx
import React, { createContext, useState } from 'react';
interface UserPreferences {
theme: string;
layout: string;
}
interface AccessibilityOptions {
voiceCommands: boolean;
colorBlindMode: boolean;
}
interface UserContextProps {
userPreferences: UserPreferences;
setUserPreferences: React.Dispatch<React.SetStateAction<UserPreferences>>;
accessibilityOptions: AccessibilityOptions;
setAccessibilityOptions: React.Dispatch<React.SetStateAction<AccessibilityOptions>>;
}
export const UserContext = createContext<UserContextProps>({} as UserContextProps);
export const UserProvider: React.FC = ({ children }) => {
const [userPreferences, setUserPreferences] = useState<UserPreferences>({ theme: 'dark', layout: 'grid' });
const [accessibilityOptions, setAccessibilityOptions] = useState<AccessibilityOptions>({ voiceCommands: false, colorBlindMode: false });
return (
<UserContext.Provider value={{ userPreferences, setUserPreferences, accessibilityOptions, setAccessibilityOptions }}>
{children}
</UserContext.Provider>
);
};
Wrapping Up
By organizing the code into components and using context, this framework can be expanded for future updates and personalized settings, all without sacrificing modularity. This structure allows each branch of the UI to evolve independently, providing a scalable, interactive, and customizable experience for #PlayStation7 users.
Would you like to explore one of these sections in even more detail, or should I add more code to specific functionalities?
0 notes
Text
10 Best Frameworks for Front-End Development in 2024
The front-end development ecosystem is constantly evolving, and 2024 is no exception. With an increasing focus on user experience, performance, and scalability, choosing the right framework can make all the difference in building efficient, modern web applications. Here are the 10 best frameworks for front-end development in 2024.
1. React
React continues to dominate the front-end space in 2024. Maintained by Facebook, it’s a JavaScript library (often called a framework) that enables developers to build dynamic, responsive user interfaces with reusable components. React’s virtual DOM ensures fast updates, making it ideal for high-performance applications.
Key Features:
Component-based architecture
Virtual DOM for faster rendering
Strong community and ecosystem
Support for React Native for mobile apps
2. Vue.js
Vue.js has gained popularity for its simplicity and flexibility. It’s a progressive framework, meaning you can use as much or as little of it as you need, making it an excellent choice for both small projects and large-scale applications.
Key Features:
Reactive two-way data binding
Focused on ease of integration
Lightweight and flexible
Growing plugin ecosystem
3. Angular
Developed by Google, Angular is a full-fledged framework for building robust web applications. It provides a complete set of tools for developers, including dependency injection, routing, and a powerful CLI (Command Line Interface). Angular’s TypeScript-based architecture ensures strong typing and scalable code.
Key Features:
Two-way data binding and dependency injection
Built-in tools for routing, forms, and HTTP services
TypeScript support for scalable applications
Robust testing capabilities
4. Svelte
Svelte has emerged as a game-changer in front-end development. Unlike other frameworks, Svelte shifts much of the work from the browser to the compile step. This results in faster, more efficient applications. Svelte doesn’t use a virtual DOM, which gives it a unique performance edge.
Key Features:
No virtual DOM for faster performance
Compile-time optimization
Simpler and more intuitive syntax
Minimal boilerplate code
5. Next.js
Next.js, built on top of React, is designed for server-side rendering (SSR) and static site generation (SSG). This makes it an excellent choice for SEO-friendly websites and applications that need fast loading times. In 2024, Next.js remains a top choice for building dynamic, high-performance web apps.
Key Features:
Server-side rendering (SSR) and static site generation (SSG)
Easy integration with React
Built-in API routes
Powerful routing and pre-rendering features
Read More: 10 Best Frameworks for Front-End Development in 2024
#backend frameworks#front end development#wordpress website development#webtracktechnologies#wordpress developer#custom website development services#web designing#front end developer#best web development services in usa
0 notes
Text
Choosing the Right Development framework for Your Mobile App: A Comparative Analysis
The market for mobile app development has been evolving rapidly in terms of meeting the standards and expectations of users. Not to mention the market for mobile app development has recorded a growth at the rate of 14% in terms of CAGR. This has made it imperative for businesses to look for the best framework of mobile applications that can contribute towards developing a stellar website.
The market growth has been increasingly dynamic which has paved the path for constant innovation. This means that to achieve success in a competitive market scenario b2c e-commerce solutions need to create a mobile framework that is versatile and adaptable.
What do you mean by Mobile Application Development frameworks?
When we are talking about mobile application development frameworks, it is mostly tools that have been pre-built. This includes different libraries and best practices whose objective is to help simplify and standardize the entire application development procedure. Through the help of these frameworks, it becomes possible to develop a strong foundation for developers in terms of creating applications. They also contribute towards providing the essential components required including back and services and elements associated with the user interface.
It is important to note that, in B2C e-commerce, there are different kinds of frameworks available, each having their own specific features and development requirements that will need to be adjusted based on the project. When it comes to B2C e-commerce solutions, developers need to know how to develop strong mobile app frameworks. Additionally, understanding B2B ecommerce application development is important for addressing the unique needs and complexities of B2B transactions.
Comparing Different Mobile App Development frameworks
Each mobile app development ecosystem has its own set of features, which will need to be assessed by developers before choosing to implement it in their online store. The wrong choice of application system in B2C e-commerce will cause you to lose both time and money. Now that we have some clarity about how important mobile application development frameworks are in the B2C e-commerce landscape, let's draw a comparison between the top frameworks that are present in the current market.
1. IONIC
The following application already has over 5 million applications built by them and is considered to be one of the oldest app development platforms available in the market. The many reasons why it is considered to be the best in the market is because of its:
● Strong code portability
● A huge community support
● Prevalence of aesthetic designs
● The presence of predefined UI templates, and,
● Ease of use and documentation.
The following b2c e-commerce solutions make use of the TypeScript framework that can be transformed into plain JavaScript code. Considered to be the most cost-efficient framework in the market, this is perfect for developing applications related to specific tasks that have low to moderate traffic.
2. Xamarin
Another popular application development framework you will find in the market is that of Xamarin. owned by Microsoft, it is considered for its easy integration and manageability across different operating systems of Android, Windows, and IOS. The major reasons why it has risen to popularity in B2C e-commerce are:
● Over 90% code reusability
● Easy to integrate, mostly hardware
● Prevalence of complete development ecosystem
● Presence of several native applications having good performance.
Mostly with the use of a .NET the types language (C#) to manage several mobile platforms. It is considered to be the best choice when using Microsoft Cloud solutions owing to its reliability and high performance.
3. ReactNative
Considered to be the best b2c e-commerce solutions in the market in terms of providing a JavaScript library for every device and platform. One can easily make use of a single codebase across several platforms making it easy to create specific questions of different feature components. considered to be the most used application for both the Android and IOS systems. the reasons why it is considered to be effective is because:
● It provides easy integration of different third-party plug-ins
● Has hybrid applications that can provide you with better performance
● It is a cost-effective alternative.
It is considered to be one of the most popular and robust programming languages present in the current b2c e-commerce. The major advantage associated with this application is that it provides writing modules that can be either in Swift, Java, or even Objective-C.
4. NativeScript
Another popular mobile up development framework is that of NativeScript. it provides the use of JavaScript for front-end development. The user interface of this framework is mostly made in the combination of both XML and CSS. Similarly, the business logic aspect is developed using JavaScript, with its superset in TypeScript. The reasons why it has found popularity in the b2c e-commerce solutions are:
● Provides the ability to manage split custom content including CSS styles
● Enables fast execution
● Compiles itself and runs mostly as a native application.
If you are looking for an application that can provide you with performance, this will be considered to be the best choice for you in the current b2c e-commerce. The best part about this framework is that it allows for complete application development across the web including both Android and IOS solutions. There are very minimal changes required in the user interface so that the need you look and feel of the application remains in that.
5. Kotlin
Developed by JetBrains, Kotlin is another important app development framework that is endorsed by Google for its Android app development. It is also interoperable with Java which makes it more lucrative in the b2c e-commerce. It utilizes Java and provides it with expressive Syntax and concise solutions. The reasons why it has gained popularity are:
● Can we use it with various IDEs
● It is mostly designed for native Android application development
● It is easy to set up with the help of Android Studio and easy to learn for Java developers.
The best part about this framework is that it can be easily interoperated with Java alongside the existing Android code. Moreover, it compiles to bytecode that seemingly provides better performance as compared to Java.
How do we implement these frameworks?
One of the most immediate questions that arises is how to implement the streamworks or gain more knowledge about the frameworks to choose the best for your online store. There are several organizations available providing B2C e-commerce solutions about these applications. One such organization is that of Magento.
You can easily hire Magento developers to help and assist you with selecting the right application development framework. When you hire dedicated Magento developers, they will help you assess your needs and your business goal based on which the app development framework will be selected. The advantages associated with your business to hire dedicated Magento developers are:
● Analyze your business needs
● Provide you with a better understanding of how the system works
● Creating a modern and responsive interface for you to manage
● Providing you with support post-implementation.
Deciding to hire Magento developers, will ensure you make the correct decision in terms of selecting the best framework for your business.
Parting Words
It is quite difficult to choose the best app development framework because not every business out there has the same needs for their websites. It has been evident from the comparison that each framework has its own features and advantages that will need to be considered before deciding on choosing the best out of them. It is the reason why you should consider hiring a Magento Web Development Company to help you make the correct choice when selecting different app frameworks.
Originally Published At - Choosing the Right Development framework for Your Mobile App: A Comparative Analysis
0 notes
Text
Kickstart Your Journey with Angular 18 and ASP.NET 8.0 Free Coupon
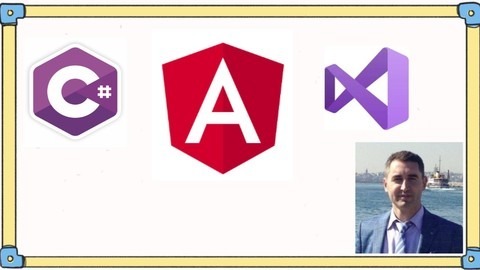
The ever-evolving world of web development demands continuous learning and adaptation. If you're looking to build modern, dynamic web applications, mastering a powerful front-end framework like Angular 18 in tandem with a robust back-end solution like ASP.NET 8.0 is a strategic move. This combination equips you with the tools to create seamless user experiences and high-performing applications.
Web Development Careers: Unveiling the Path to Success
This article serves as your comprehensive guide to kickstarting your journey with Angular 18 and ASP.NET 8.0. We'll delve into these technologies, explore their valuable features, and guide you through the learning process with a special bonus - a free coupon for a comprehensive Udemy course!
Why Angular 18 and ASP.NET 8.0?
Angular 18:
Modern Framework: Built with TypeScript for strong typing and improved developer experience.
Angular 18 New Features: A complete guide for developers - Kellton
Improved Performance: Ivy compiler optimizations for faster build times and smoother app performance.
Enhanced Forms Module: Streamlined form handling and validation for better user interaction.
Strict Mode by Default: Catches potential errors at compile time, promoting cleaner code.
Rich Ecosystem: Extensive library support and a vibrant developer community.
ASP.NET 8.0:
Cross-Platform Development: Build applications for Windows, Linux, and macOS with minimal code changes.
Improved Web API: Enhanced developer experience for creating RESTful APIs.
Enhanced Security: Robust built-in security features to protect your applications.
Cloud-Native Development: Seamless integration with cloud platforms like Azure.
Modern Development Tools: Visual Studio support provides a powerful IDE for development.
Together, Angular 18 and ASP.NET 8.0 offer a compelling combination for building full-fledged web applications.
Learning Path
1. Building a Strong Foundation:
HTML, CSS, and JavaScript: Mastering these fundamentals is crucial for understanding the building blocks of web applications.
TypeScript: Learn this superset of JavaScript for improved code type safety and maintainability.
2. Delving into Angular 18:
Understanding Components: Grasp the core building blocks of Angular applications.
Data Binding and Services: Utilize these techniques to manage data flow efficiently.
Routing and Navigation: Create seamless navigation experiences within your application.
Forms and Validation: Build user-friendly forms with robust validation.
Dependency Injection: Understand this design pattern for cleaner and more maintainable code.
3. Exploring ASP.NET 8.0:
Setting Up the Development Environment: Install the .NET SDK and learn to navigate Visual Studio.
Building Web APIs: Create RESTful APIs using ASP.NET Core MVC for communication between front-end and back-end.
Database Integration: Learn to connect your web APIs to databases for data persistence.
Security Best Practices: Implement authentication and authorization measures to secure your applications.
4. Building an Angular 18 and ASP.NET 8.0 Application:
Project Setup: Create separate projects for the Angular front-end and the ASP.NET back-end.
API Integration: Establish communication between the Angular app and the ASP.NET Web API.
Data Fetching and Display: Fetch data from the API endpoints and display it in the Angular application.
User Management: (Optional) Implement user login and registration functionalities through the API.
Resources and Learning Tools:
Udemy Courses (Free Coupon Included!): Explore a comprehensive Udemy course with video lectures, quizzes, and practical exercises. This article includes a special free coupon to unlock this valuable resource! (Details below)
Official Documentation: Both Angular and ASP.NET provide detailed documentation to guide your learning journey.
Online Tutorials and Blogs: Leverage the vast amount of online resources available for Angular and ASP.NET.
Community Forums: Engage with other developers on forums and communities to ask questions and share knowledge.
Free Udemy Course Coupon!
Get a head start on your Angular 18 and ASP.NET 8.0 journey with a free coupon for a Udemy course! This comprehensive course will equip you with the essential skills to build dynamic web applications.
0 notes
Text
The Framework Forge: Choosing the Right Tool for Web Development in 2024
The web development landscape is an ever-shifting terrain. New frameworks emerge, existing ones evolve, and developers are constantly on the lookout for the best tool for the job. But with so many options available, deciding on the "best framework" can feel like navigating a labyrinth. Fear not, intrepid developer! This guide will equip you with the knowledge to conquer the framework forge and select the perfect match for your next web project.
Understanding Frameworks: Why They Matter
Web development frameworks are pre-built libraries that provide a foundation for creating web applications. They offer a structured approach, including pre-written code for common functionalities, reducing development time and effort. Frameworks also enforce best practices, promoting clean, maintainable code. Here's why frameworks matter:
Faster Development: Frameworks come equipped with pre-built components and functionalities, allowing developers to focus on the unique aspects of their projects rather than reinventing the wheel.
Improved Code Quality: Frameworks often enforce coding conventions and best practices, leading to cleaner, more maintainable code. This reduces bugs and improves long-term project health.
Enhanced Security: Many frameworks prioritize security by providing built-in features and functionalities to protect against common web vulnerabilities.
Large Community & Support: Popular frameworks often have vast developer communities providing resources, tutorials, and assistance when needed.
Picking Your Weapon: Top Frameworks for 2024
While there's no single "best framework," some stand out in different areas. Here are some of the most popular contenders in 2024, categorized by their primary focus:
Frontend Frameworks (Client-side):
React (JavaScript): A versatile and powerful library for building dynamic user interfaces. React's component-based architecture promotes code reusability and maintainability. Its virtual DOM (Document Object Model) ensures efficient rendering, making it suitable for complex and data-driven applications. React boasts a massive community and is a popular choice for single-page web applications (SPAs).
Vue.js (JavaScript): Vue.js offers a balance between flexibility and structure. It's known for its ease of learning and adoption, making it a great choice for beginners or projects requiring a rapid development pace. Vue.js provides a good mix of features for building interactive web interfaces, and its progressive nature allows developers to integrate it incrementally into existing projects.
Angular (TypeScript): An opinionated framework from Google, Angular is ideal for large, complex enterprise applications. It enforces a structured development approach with features like dependency injection and routing built-in. Angular's use of TypeScript provides strong typing and code structure, improving development efficiency and reducing errors. However, its rigid structure might feel restrictive for smaller projects or those requiring more flexibility.
Backend Frameworks (Server-side):
Django (Python): This Python-based high-level framework is renowned for its "batteries-included" philosophy. Django provides a comprehensive set of tools for building web applications, including user authentication, database management, and security features. Its focus on developer ergonomics and rapid development makes it a favorite for building complex web applications with tight deadlines.
Express.js (JavaScript): Express is a lightweight and flexible Node.js framework that provides a foundation for building web applications and APIs. Its minimalist approach offers a high degree of customization, allowing developers to tailor the framework to their specific needs. Express is a popular choice for building microservices and RESTful APIs due to its scalability and performance.
Laravel (PHP): Laravel is a feature-rich PHP framework known for its expressive syntax and robust set of tools. It offers a clean MVC (Model-View-Controller) architecture, streamlining development and promoting maintainability. Laravel excels at building complex web applications with a focus on security and performance.
Full-stack Frameworks:
Ruby on Rails (Ruby): Rails is a pioneer in the web development framework world. This Ruby-based framework is known for its convention-over-configuration approach, which promotes rapid development and reduces boilerplate code. Rails offers a strong foundation for building web applications with a focus on developer productivity and clean code.
FAQs
Q. How do I choose the right framework?
Here's a roadmap:
Project Type: Building a social media app? Consider React or Django. Need a data-driven dashboard? Explore Angular or Flask.
Team Skills: If your team is strong in JavaScript, React or Express might be a good fit. For Python lovers, Django or Flask could shine.
Learning Curve: New to frameworks? Explore options like Vue.js or Flask with a gentler learning curve.
Community & Resources: Bigger frameworks often have larger, supportive communities and more learning resources available.
Q. Where can I learn more?
Framework Documentation: Each framework has official documentation, a great place to start.
Online Tutorials: Websites like https://www.w3schools.com/ and https://www.codecademy.com/catalog offer interactive tutorials for many frameworks.
YouTube Channels: Channels like Traversy Media, freeCodeCamp, and The Net Ninja offer a wealth of framework-specific video tutorials.
In conclusion
Remember: There's no single "best framework" for every project. By carefully evaluating your project requirements, team expertise, and the factors mentioned above, you can make an informed decision and select the framework that empowers you to build a successful web application in 2024.
0 notes
Text
Unveiling Your Best Tech Stacks for Web App Development in 2024: A Bangalore Perspective
In the bustling tech hub of Bangalore, where innovation is a way of life, staying ahead of the curve in web app development is paramount. As we step into 2024, the landscape of web development continues to evolve rapidly, propelled by emerging technologies and changing user expectations. Choosing the right tech stack is crucial to thrive in this dynamic environment. Let's delve into the key components of the best tech stacks for web app development in 2024, tailored for the vibrant ecosystem of Bangalore.
Frontend Frameworks:
React.js: Renowned for its flexibility and performance, React.js remains a top choice for building interactive user interfaces. Its component-based architecture facilitates modular development, which is perfect for teams collaborating on complex projects.
Angular: With its robust framework and comprehensive tooling, Angular empowers developers to create scalable web applications with ease. Its TypeScript support ensures enhanced reliability and maintainability, making it a preferred option for enterprise-grade solutions.
Backend Technologies:
Node.js: Leveraging the power of JavaScript, Node.js continues to dominate the backend landscape. Its non-blocking, event-driven architecture enables high concurrency, ideal for real-time applications. Coupled with frameworks like Express.js, Node.js streamlines server-side development, accelerating time-to-market.
Django: Known for its simplicity and scalability, Django remains a stalwart in backend development. Built on Python, it offers a robust set of features, including an ORM layer, authentication mechanisms, and a built-in admin interface. For rapid prototyping and agile development, Django proves to be an invaluable asset.
Database Solutions:
MongoDB: As the demand for flexible, schema-less databases rises, MongoDB emerges as a frontrunner. Its document-oriented model and horizontal scalability make it an excellent choice for handling unstructured data, a common requirement in modern web applications.
PostgreSQL: For mission-critical applications requiring ACID compliance and relational integrity, PostgreSQL stands out as a reliable option. Its extensible architecture and support for advanced features like JSONB data type ensure optimal performance and data integrity.
DevOps Tools:
Docker: Containerization has revolutionized the way web applications are deployed and managed. Docker simplifies the packaging of applications and their dependencies into portable containers, ensuring consistency across different environments. With Kubernetes orchestrating containerized workloads, scalability, and resilience are seamlessly achieved.
Jenkins: Automating the build, test, and deployment pipelines is essential for accelerating the development lifecycle. Jenkins, with its robust plugin ecosystem and CI/CD capabilities, empowers teams to achieve continuous integration and delivery effortlessly.
Cloud Platforms:
AWS: Amazon Web Services continues to lead the cloud computing market with its vast array of services and global infrastructure. From scalable compute instances to serverless computing with AWS Lambda, developers in Bangalore can harness the power of AWS to build and deploy resilient web applications with ease.
Google Cloud Platform: With its focus on data analytics and machine learning, Google Cloud Platform offers unique advantages for AI-driven web applications. Leveraging services like BigQuery and TensorFlow, developers can unlock insights and deliver personalized experiences to users.
In conclusion, crafting the best tech stack for web app development in 2024 involves carefully balancing cutting-edge technologies, scalability, and developer experience. In Bangalore's thriving tech ecosystem, where innovation flourishes at every corner, embracing these trends and harnessing the power of web development services is key to staying ahead in the digital race. By leveraging the right tools and frameworks, developers can bring their visions to life and shape the future of web applications in the years to come.
0 notes
Text
Tech Trends: Exploring the Leading Web Development Technologies
In the rapidly evolving landscape of web development, staying up-to-date with the latest technologies is essential for developers and businesses alike. As technology continues to advance, new tools, frameworks, and libraries emerge, offering innovative solutions and opportunities for building modern web applications. In this article, we'll delve into the leading web development technologies that are shaping the future of web development, exploring their features, benefits, and potential applications in today's digital landscape.
1. React.js:
React.js continues to be a dominant force in the world of web development, known for its component-based architecture and efficient rendering capabilities. Developed by Facebook, React.js simplifies the process of building interactive user interfaces by breaking down complex UIs into reusable components. Its virtual DOM implementation ensures optimal performance and seamless updates, making it ideal for building dynamic single-page applications (SPAs) and progressive web apps (PWAs). With a vast ecosystem of libraries, tools, and community support, React.js remains a top choice for developers seeking to build modern and scalable web applications.
2. Vue.js:
Vue.js has rapidly gained popularity among developers due to its simplicity, versatility, and progressive adoption curve. With its intuitive syntax and lightweight nature, Vue.js offers a highly approachable framework for building interactive web interfaces. Its reactive data binding and component-based architecture enable developers to create modular and scalable applications with ease. Vue.js is particularly well-suited for rapid prototyping, small to medium-sized projects, and integrating with existing web applications. As Vue.js continues to mature and expand its ecosystem, it remains a formidable contender in the web development landscape.
3. Angular:
Angular, developed and maintained by Google, is a robust framework for building complex and feature-rich web applications. Angular's comprehensive feature set, including two-way data binding, dependency injection, and TypeScript support, makes it a powerful choice for enterprise-scale projects. With its strong ecosystem, extensive documentation, and built-in tooling, Angular provides developers with everything they need to develop scalable and maintainable applications. Despite its steep learning curve, Angular's stability, performance, and support for large-scale applications make it a preferred choice for businesses seeking enterprise-grade solutions.
4. Next.js:
Next.js has gained significant traction as a leading framework for server-side rendering (SSR) and static site generation (SSG) in React applications. Next.js simplifies the process of building performant and SEO-friendly web applications by enabling developers to pre-render pages at build time or on the server. Its built-in support for routing, code splitting, and automatic optimization streamlines the development workflow and enhances the user experience. Next.js is particularly well-suited for building content-heavy websites, e-commerce platforms, and blogs, offering developers a powerful toolkit for building modern web applications with ease.
5. GraphQL:
GraphQL has emerged as a game-changer in the world of web development, offering a more efficient and flexible alternative to traditional REST APIs. Developed by Facebook, GraphQL enables clients to request precisely the data they need, minimizing over-fetching and under-fetching of data. Its type system and introspection capabilities empower developers to define clear schemas and explore data relationships effectively. GraphQL's popularity continues to grow, driven by its ability to streamline data fetching and enable seamless integration with various frontend frameworks and backends.
Conclusion:
As we explore the leading web development technologies, it's clear that the landscape is rich with choices for developers seeking to build modern, scalable, and performant web applications. Whether you opt for React.js, Vue.js, Angular, Next.js, GraphQL, or a combination of these technologies, the key is to select the right tools and frameworks that align with your project requirements, team expertise, and long-term goals. By staying abreast of the latest trends and advancements in web development, developers can leverage the power of these technologies to create exceptional web experiences that delight users and drive business success in the digital age.
0 notes
Text
Angular Training
Angular Traning in Hyderabad
Angular training is a specialized program designed to teach individuals how to develop web applications using the Angular framework. Angular is a popular front-end framework maintained by Google that's widely used for creating dynamic and interactive user interfaces.
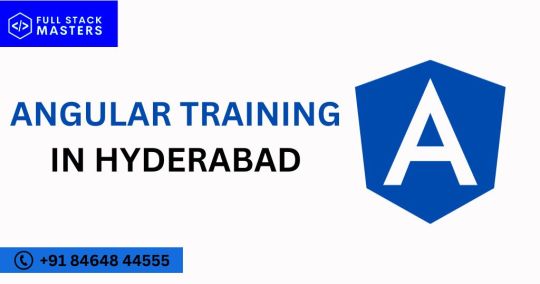
overview of Angular training:
1. Core Concepts: Angular training starts with the core concepts of the framework, introducing you to key concepts like components, modules, templates, and data binding.
2. TypeScript: Angular is built with TypeScript, a statically typed superset of JavaScript. Training often includes a solid understanding of TypeScript, as it's integral to Angular development.
3. Component-Based Architecture: Angular is based on a component-based architecture. Training covers how to design and build components, which are the building blocks of Angular applications.
4. Templates and Directives: You'll learn how to create dynamic views using templates and how to use directives like *ngIf, *ngFor, and custom directives to manipulate the Document Object Model (DOM).
5. Dependency Injection: Understanding dependency injection is crucial for managing and sharing services and data across an Angular application.
6. Routing: Angular training often includes the use of the Angular Router to create single-page applications with multiple views and navigation.
7. Forms: You'll explore the creation of reactive forms and template-driven forms for handling user input and validation.
8. HTTP and APIs: Training covers how to make HTTP requests and work with APIs to fetch and send data to a server.
9. State Management: Advanced Angular training might delve into state management solutions like NgRx for managing the application's state.
10. Testing: You'll learn how to write unit tests for your Angular components and services using tools like Jasmine and Karma.
11. Project Work: Angular training typically involves hands-on projects to reinforce your skills and give you real-world experience in building Angular applications.
12. Best Practices: You'll be introduced to best practices and coding standards to write clean, maintainable, and performant Angular code.
Angular training is essential for web developers who want to create modern, robust, and interactive web applications. It equips you with the skills and knowledge needed to harness the power of Angular and become proficient in front-end web development.
Learning Angular through training offers several benefits that can enhance your skills and career prospects in web development. Here are some of the key advantages of learning Angular:
Career Opportunities: Angular is a widely used front-end framework, and demand for Angular developers is high. Learning Angular can open up numerous job opportunities in web development.
Versatility: Angular is suitable for building a wide range of applications, from single-page applications (SPAs) to complex web platforms. It provides a versatile set of tools for creating interactive and dynamic user interfaces.
Structured Development: Angular enforces a structured and organized development approach, which can lead to cleaner, more maintainable code. This is especially beneficial for large-scale projects.
Component-Based Architecture: Angular's component-based architecture encourages the creation of reusable and modular components, making it easier to manage and scale applications.
TypeScript Proficiency: Learning Angular involves using TypeScript, a statically typed superset of JavaScript. Proficiency in TypeScript can improve code quality and help catch errors at compile time.
Strong Community and Ecosystem: Angular has a large and active developer community. You'll have access to a wealth of resources, libraries, and third-party tools to enhance your development workflow.
Built-In Features: Angular comes with many built-in features, such as routing, dependency injection, and state management (e.g., NgRx). This reduces the need for external libraries and simplifies application development.
Scalability: Angular is well-suited for large and complex applications, offering tools for code splitting, lazy loading, and other performance optimizations.
SEO-Friendly: Angular can be configured to be search engine optimization (SEO) friendly, making it easier for search engines to crawl and index your content.
Cross-Platform Development: Angular can be used for developing web applications as well as mobile applications using frameworks like Ionic and NativeScript.
Internationalization (i18n) Support: Angular provides support for internationalization and localization, allowing you to create applications for a global audience.
Up-to-Date Skills: Learning Angular keeps your skills relevant in the fast-paced field of web development, as the framework is continually updated and improved.
Employability: Angular proficiency enhances your employability and can lead to higher-paying job opportunities in the web development industry.
Continuous Learning: Web development is an ever-evolving field. Angular training encourages continuous learning and staying up-to-date with the latest web development trends and best practices.
In summary, learning Angular through training provides you with valuable skills and expertise that are in high demand in the web development industry. It offers a structured and powerful approach to building web applications, making it a worthwhile investment in your career. https://fullstackmasters.in/
0 notes
Text
Review And Compare Popular Frontend And Backend Development Frameworks
Web Development Frameworks: Review and compare popular frontend and backend development frameworks, such as React, Angular, Vue.js, Django, and Ruby on Rails.
Web development frameworks are the building blocks of modern web applications, empowering developers to create robust and feature-rich websites and web applications more efficiently. In this article, we will review and compare popular frontend and backend development frameworks, including React, Angular, Vue.js, Django, and Ruby on Rails, to help you make an informed decision when selecting the right tool for your web development projects.
Frontend Development Frameworks
1. React
Strengths:
Component-Based: React is known for its component-based architecture, making it easy to build and manage complex user interfaces.
Community and Ecosystem: It has a vast and active community, offering a wide range of libraries, components, and tools.
Virtual DOM: The virtual DOM system enhances performance by minimizing unnecessary updates to the actual DOM.
Weaknesses:
Learning Curve: Beginners may find React’s concepts, such as JSX and component lifecycle, challenging to grasp initially.
Boilerplate Code: React requires a lot of setup and configuration to get started, which can be daunting for newcomers.
2. Angular
Strengths:
Full-Fledged Framework: Angular is a complete framework, offering everything you need for building web applications, including a built-in router and state management.
Two-Way Data Binding: It provides robust two-way data binding, which simplifies handling user input and state changes.
TypeScript Support: Angular’s use of TypeScript enforces strong typing and enhances code quality.
Weaknesses:
Complexity: The extensive set of features and concepts in Angular can lead to a steep learning curve.
Performance: Angular applications can be relatively heavy, affecting load times, especially for smaller projects.
3. Vue.js
Strengths:
Progressive Framework: Vue.js is designed to be incrementally adoptable, allowing developers to use as much or as little of the framework as needed.
Simple Syntax: Vue’s simple and intuitive syntax is beginner-friendly and quick to learn.
Performance: Vue.js is known for its lightweight nature and exceptional performance.
Weaknesses:
Smaller Ecosystem: While Vue’s ecosystem is growing, it is not as extensive as React or Angular.
Less Backing: Vue.js has a smaller company behind it compared to Facebook (React) and Google (Angular), which can affect long-term support and development.
Backend Development Frameworks
1. Django
Strengths:
Batteries Included: Django comes with a wide range of built-in features, including an ORM, authentication, and an admin panel.
High Productivity: Django’s “Don’t Repeat Yourself” (DRY) philosophy and robust documentation contribute to high development productivity.
Security: Django has strong built-in security features, making it a reliable choice for applications handling sensitive data.
Weaknesses:
Opinionated: Django’s opinionated nature can be restrictive for developers who prefer more flexibility in their stack.
Learning Curve: Django has a learning curve for beginners, especially when dealing with its ORM and project structure.
2. Ruby on Rails
Strengths:
Convention Over Configuration: Ruby on Rails embraces convention over configuration, which accelerates development by reducing decision-making.
Active Community: Rails has a strong and active developer community, contributing to a wealth of resources and libraries.
Mature: Ruby on Rails has been around for over a decade and is a reliable choice for web application development.
Weaknesses:
Performance: Ruby on Rails may not be the best choice for high-performance or resource-intensive applications.
Strict Conventions: While conventions can be helpful, they can also limit flexibility for those who want more control over their project’s architecture.
Choosing the Right Framework
The choice between React, Angular, Vue.js, Django, and Ruby on Rails depends on various factors, including your project’s requirements, your team’s familiarity, and your personal preferences:
React, Angular, or Vue.js: If you’re working on frontend development, consider your team’s experience and the project’s size and complexity. React offers a vast ecosystem, Angular provides a complete framework, and Vue.js is lightweight and beginner-friendly.
Django or Ruby on Rails: For backend development, consider your project’s requirements and your team’s expertise. Django is known for security and versatility, while Ruby on Rails offers rapid development with convention over configuration.
In conclusion, the best framework for your project will depend on a variety of factors, so carefully evaluate your specific needs and the strengths and weaknesses of each framework before making a decision. Regardless of your choice, these frameworks are valuable tools for building high-quality web applications efficiently.
Source:
#kushitworld#india#saharanpur#itcompany#digitalmarketing#seo#seo services#webdevelopment#websitedesigning
0 notes
Text
Create React App and TypeScript: How-To
Introduction
Linkdddddfsdf In the fast-evolving landscape of web development, staying up-to-date with the latest tools and technologies is crucial. One such advancement that has gained immense popularity is Create-React App with TypeScript. In this article, we'll explore how this powerful combination can streamline your web app development process, making it more efficient and maintainable. What is Create-React App with TypeScript? Before delving into the benefits and practical aspects, let's start with the basics. Create-React App (CRA) is a tool that helps you set up a new React project with zero configuration. It simplifies the setup process and provides a solid foundation for building React applications.
Getting Started with Create-React App TypeScript
Installing Create-React App with TypeScript To get started with Create-React App (CRA) using TypeScript, you'll need to follow a few straightforward steps. This installation process ensures you have all the necessary tools to begin building modern web applications. Prerequisites Before installing Create-React App with TypeScript, make sure you have the following prerequisites in place: - Node.js: Ensure you have Node.js installed on your machine. You can download it from the official website: Node.js Downloads. - npm (Node Package Manager): npm is usually included with Node.js, so after installing Node.js, you should have npm as well. You can verify this by running npm -v in your terminal. Step 1: Create a New React App To start, open your terminal and navigate to the directory where you want to create your new React app. Once you're in the desired directory, run the following command: npx create-react-app my-react-app --template typescript Replace my-react-app with the name you want to give to your application. This command creates a new React app with TypeScript configuration using Create-React App. Step 2: Navigate to Your App's Directory After the installation process is complete, navigate to your app's directory using the cd command. For example: cd my-react-app Step 3: Start the Development Server Now that you're inside your app's directory, you can start the development server by running the following command: npm start This command will compile your TypeScript code and launch a development server. Your Create-React App with TypeScript is now up and running, and you can access it in your web browser at http://localhost:3000. Step 4: Start Coding You're all set! You can now start building your modern web application using React and TypeScript. You'll find your project's source code in the src directory, and you can make changes to the code using your favorite code editor. Remember to refer to the official documentation for Create-React App and TypeScript for more detailed information and advanced configurations. That's it! You've successfully installed Create-React App with TypeScript and are ready to embark on your web development journey. Enjoy coding! Creating Your First React Application Once you have Create-React App TypeScript installed, it's time to create your first React application. We'll guide you through the process and explain the structure of a basic CRA project.
Advantages of Using Create-React App with TypeScript
Type Safety and Code Predictability TypeScript, a statically typed superset of JavaScript, enhances code quality by providing type safety. We'll show you how TypeScript catches errors at compile time, reducing bugs and improving code predictability. Improved Developer Experience Developers love efficiency. Discover how Create-React App and TypeScript work together to offer a superior developer experience with features like hot reloading, a built-in development server, and more. Scalability and Maintainability As your project grows, so does the complexity. Learn how TypeScript helps in scaling your React application while keeping it maintainable through the use of interfaces and strong typing. Enhanced Tooling and IDE Support Effective tooling can significantly boost your productivity. We'll highlight the excellent tooling and IDE support available for Create-React App with TypeScript, making your coding experience smoother.
Real-World Applications
Building a To-Do List Application Let's put theory into practice. We'll guide you through building a simple To-Do List application using Create-React App with TypeScript. You'll see firsthand how easy it is to get started with this powerful combination. Integrating Third-Party Libraries In the world of web development, you often need to leverage third-party libraries. We'll demonstrate how to seamlessly integrate popular libraries into your Create-React App TypeScript project.
Challenges and Best Practices
Handling API Requests When dealing with APIs, proper data handling is crucial. We'll discuss best practices and common challenges when making API requests in your React application. Testing and Debugging Quality assurance is essential for any web app. Learn how to effectively test and debug your Create-React App TypeScript project to ensure a robust final product.
Conclusion
In conclusion, Create-React App with TypeScript is a game-changer in the world of web development. It offers an efficient and maintainable way to build modern web applications. By harnessing the power of TypeScript and the simplicity of Create-React App, you can create robust and scalable projects with ease. If you're ready to embark on your journey of web development excellence, give Create-React App with TypeScript a try. Your future self will thank you for the decision.
FAQs
- Is TypeScript difficult to learn for beginners? - TypeScript may seem intimidating at first, but it offers great benefits. Learning resources and community support are abundant, making it accessible for beginners. - Can I use Create-React App TypeScript for large-scale projects? - Absolutely! Create-React App TypeScript is designed to scale with your projects, ensuring maintainability and efficiency even for complex applications. - What are the key advantages of using TypeScript in React development? - TypeScript provides type safety, improved developer tooling, and enhanced scalability, all of which contribute to a better development experience. - Are there any performance considerations when using Create-React App TypeScript? - While TypeScript adds type checking, the impact on runtime performance is minimal, and the benefits in terms of code quality outweigh any minor overhead. - Where can I find more resources to learn about Create-React App with TypeScript? - You can find tutorials, documentation, and community discussions online to help you master this powerful combination for web development. Read the full article
0 notes
Text
"Mastering Angular: A Comprehensive Guide to Building Stunning User Interfaces"
"Mastering Angular: A Comprehensive Guide to Building Stunning User Interfaces"
A complete, free-to-use JavaScript framework called Angular is used to create dynamic web apps. In order to build feature-rich single-page applications (SPAs) and progressive web apps (PWAs), developers can use the Angular framework, which Google developed and maintains. Whether Angular is worth learning depends on your specific goals, interests, and the type of web development projects you plan to work on.
Here are some factors to consider when deciding if learning Angular is the right choice for you:
1. Project Requirements:
·If you are working on or planning to work on projects that require building complex single-page applications (SPAs), progressive web apps (PWAs), or enterprise-level applications, Angular can be a valuable tool. Angular's comprehensive framework and extensive toolset are well-suited for such scenarios.
2. TypeScript Familiarity:
Angular is built with TypeScript, so if you're already familiar with TypeScript or interested in learning it, Angular can be a logical choice. TypeScript offers strong typing, better code quality, and excellent tooling support.
3. Enterprise Development:
· Angular is a popular choice for large-scale enterprise applications due to its modularity, dependency injection, and strong support for testing. If you're looking to work in an enterprise development environment, learning Angular could be advantageous.
4.Community and Job Opportunities:
Angular has a substantial and active community, which can be beneficial for finding resources, getting help, and staying up-to-date with best practices. It also has a presence in the job market, and many companies seek Angular developers.
5. Personal Preference:
Your personal development preferences matter. Some developers prefer Angular's opinionated structure and built-in solutions for various tasks, while others may lean towards more lightweight or flexible frameworks.
6. Learning Curve:
Angular has a steeper learning curve compared to some other frameworks like React or Vue.js. However, if you are committed to learning and are willing to invest time in mastering it, the knowledge can be valuable.
7. Alternative Frameworks:
Consider other popular front-end frameworks like React and Vue.js. These frameworks have their own strengths and are well-suited for different types of projects. Exploring multiple frameworks can give you a broader skill set.
8. Project Diversity:
Depending on your career goals, it can be beneficial to have knowledge in multiple frameworks and technologies. Learning Angular along with other frameworks can make you a more versatile developer.
Here are some essential details about Angular: 1. Complete Framework: Angular is a full-featured framework that offers a variety of tools, libraries, and best practices for developing sophisticated online applications. It offers solutions for numerous application development issues, including routing, state management, form handling, and others. 2. Component-Based Architecture: Angular has a component-based architecture, akin to React, in which the user interface (UI) of the application is broken up into reusable components. By encapsulating their own logic, templates, and styles, these components encourage the modularity and reuse of code.
3.TypeScript: TypeScript, a statically typed superset of JavaScript, is used to create Angular. TypeScript enables greater code maintainability, compile-time error detection, and good tooling for code navigation and refactoring.
4. Two-Way Data Binding: Angular provides two-way data binding, which ensures that updates to the user interface automatically affect the application's data model and vice versa. Data synchronization between components and the UI is made easier as a result.
• The dependency injection system in Angular is quite effective at managing the dependencies and services that components need. This encourages the testability and reuse of code.
2. Navigation and Routing:
• A strong routing system is built into Angular, allowing programmers to specify routes and manage navigation inside their applications. Building SPAs with many views and intuitive navigation is impossible without it.
3. Integration of RxJS:
• RxJS (Reactive Extensions for JavaScript), which Angular effortlessly interacts with, enables developers to efficiently manage asynchronous actions and data streams. Real-time data handling and HTTP requests benefit particularly from this.
4. Form handling: Angular offers thorough support for generating forms, including reactive forms (model-driven forms) and template-driven forms. This makes handling user input and validation simpler.
5. Strong CLI: The Angular CLI makes project setup, code creation, testing, and deployment chores simpler. It simplifies the development process and promotes project-wide consistency.
6. Cross-Platform Development: Angular can be used for more than only online applications. It may be used to create desktop applications using Electron as well as cross-platform mobile applications for smartphones using technologies like Ionic or NativeScript.
Large Developer Community and Ecosystem: Angular has a sizable and vibrant developer community, which has produced a sizable ecosystem of libraries, extensions, and third-party integrations. Access to information and solutions for varied development needs is ensured through this community assistance.
7. Enterprise-Ready: Angular's broad toolkit, strong testing support, and scalability capabilities make it an excellent choice for enterprise-level applications. It is a popular option for creating extensive and intricate software systems.
Angular is a thorough, opinionated framework that provides a wide selection of tools and capabilities for developing contemporary web apps. TypeScript's component-based architecture 1. Cross-Platform Development: Angular can be used for more than only online applications. It may be used to create desktop applications using Electron as well as cross-platform mobile applications for smartphones using technologies like Ionic or NativeScript.
Large Developer Community and Ecosystem: Angular has a sizable and vibrant developer community, which has produced a sizable ecosystem of libraries, extensions, and third-party integrations. Access to information and solutions for varied development needs is ensured through this community assistance.
8. Enterprise-Ready: Angular's broad toolkit, strong testing support, and scalability capabilities make it an excellent choice for enterprise-level applications. It is a popular option for creating extensive and intricate software systems.
Angular is a thorough, opinionated framework that provides a wide selection of tools and capabilities for developing contemporary web apps.
In summary, Angular is worth learning if you have a clear understanding of your project requirements, career goals, and personal preferences. It is a powerful and well-established framework with a strong community and job market presence, making it a valuable skill for many developers. However, it's essential to evaluate whether Angular aligns with your specific needs and interests in web development.
I strongly advise getting in touch with ACTE Institution because they provide certifications and opportunities for job placement if you want to learn more about Angular. You can study more effectively with the aid of knowledgeable tutors. These services are available both offline and online. Take things slow, and if you're interested, think about signing up for a course.
1 note
·
View note
Text
What are custom visuals in Power BI?
Custom visuals in Power BI refer to visualizations that are not natively available in the standard set of visualizations provided by Power BI. These visuals are created by the Power BI community, third-party developers, or by users themselves using the Power BI Developer Tools. Custom visuals expand the range of visualizations that can be used in Power BI reports and dashboards, offering unique and specialized ways to present and analyze data.
The Power BI marketplace, also known as AppSource, provides a platform for users to discover and download custom visuals created by the community and third-party developers. These visuals cover a wide range of functionalities and design styles, allowing users to find specific visualizations that suit their data analysis requirements. The marketplace offers custom visuals for various purposes, including charts, maps, tables, gauges, timelines, and more.
To use a custom visual in Power BI, users need to download the visual from the marketplace and import it into their Power BI report. Once imported, the custom visual appears in the "Visualizations" pane, where users can drag and drop it onto their report canvas and configure it using the associated settings and properties. Custom visuals can be fully interactive, allowing users to drill down, filter, and slice data just like the built-in visuals provided by Power BI.
Custom visuals in Power BI provide several benefits. They enhance the visual capabilities of Power BI, enabling users to create unique and engaging reports that cater to their specific needs. Custom visuals also allow users to leverage advanced chart types, interactive features, and specialized visualizations that may not be available in the standard set of visualizations. This flexibility empowers users to communicate data insights effectively and present information in a visually compelling manner. By obtaining Power BI Course, you can advance your career in Power BI. With this course, you can demonstrate your expertise in Power BI Desktop, Architecture, DAX, Service, Mobile Apps, Reports, many more fundamental concepts, and many more critical concepts among others.
Furthermore, custom visuals encourage collaboration and knowledge sharing within the Power BI community. Developers and users can share their custom visuals through the marketplace, allowing others to benefit from their creations. This promotes the exchange of innovative ideas, best practices, and creative solutions, fostering a vibrant ecosystem around Power BI.
Power BI also provides a set of developer tools, such as the Power BI Visuals SDK, which enables users to build their own custom visuals from scratch. These tools include development frameworks, libraries, and APIs that developers can use to create and customize visuals with their desired functionalities and design. The Power BI Developer Tools support various programming languages, such as TypeScript and JavaScript, allowing developers to leverage their existing skills and knowledge.
In summary, custom visuals in Power BI expand the range of available visualizations beyond the standard set provided by Power BI. They are created by the community, third-party developers, or users themselves using the Power BI Developer Tools. Custom visuals enhance the visual capabilities of Power BI, allowing users to create unique and specialized visualizations for their reports and dashboards. They provide advanced chart types, interactive features, and specialized designs that cater to specific data analysis requirements.
The Power BI marketplace facilitates the discovery and sharing of custom visuals, fostering collaboration and knowledge exchange within the Power BI community. Additionally, the Power BI Developer Tools enable users to build their own custom visuals, promoting innovation and customization in data visualization.
0 notes
Text
Senior Front-end Engineer, Apps - Remote
Company: Parity As stewards of the Polkadot and Substrate ecosystem, Parity is laying the foundation for a better web which respects the freedom and data of individuals and empowers developers to create better services through decentralised technology. The internet is too important to billions of people for it to be at the mercy of a few powerful companies. Like Polkadot, Parity was built on a foundation of being decentralised and open, which trickles down to how we work. We’re a distributed organisation and have been from the beginning. Being distributed isn’t just a way of doing business—it’s a mentality that is at the core of our culture. We have a flat structure that pushes power to the edges and empowers our people to take ownership of their role, authority coupled with responsibilities.
About the role: The role is about building user facing applications and reusable UI components that help millions of people interact with the Polkadot Network and move forward the Web3 vision. This requires thinking about UI from the standpoint of the protocol developer, the frontend developer and the end user. The resulting UI components should empower front-end developers to craft chain-backed experiences which delight end users. You will make use of cutting edge technologies built in-house at Parity (for instance Capi), helping to set a standard in the developer community building on Substrate. About the team: All our projects are open source and most of our communication happens on GitHub. Our team is based across multiple timezones, so we tend to favour asynchronous communication over meetings and ceremonies. Familiarity with open source workflows on GitHub is highly appreciated. We are highly autonomous so we look for someone who is driven by passion and can deliver without precise step-by-step instructions coming from above. Requirements: - Due to the nature of our work we can only consider applicants with production experience on complex TypeScript projects. - Advanced expertise with TypeScript and React is a must, but we value production experience with other frontend frameworks as long as they make use of a type system. - Expert knowledge of patterns that help with effective state management is highly appreciated - Strong problem solving and debugging skills - We look for excellent communicators, who actively participate in planning and driving new features. - Experience building Web3 apps is a plus, but not a must. You just have to be passionate about frontend tech. Tasks: - Collaborate with designers, other developers and the open source community to determine optimal routes to best-meet the needs of users - Write clean, easy-to-understand, idiomatic front-end TypeScript - Relentlessly drive for more intuitive and useful UI and approaches to UI, across the org
About working for us: For everyone who joins us: - Competitive remuneration packages, including tokens (where legally possible), based on iterative market research - Remote-first, global working environment with flexible hours - Collaborative, fast-paced, and self-initiating culture, designed to mimic an open source workflow - Energising and collaborative team and company retreats all over the world - Opportunity to learn more about Web3 while on the job, with access to some of the brightest minds in this space; we have plenty of educational initiatives such as internal sessions, all-hands, AMAs, hackathons, etc. - Teammates who are genuinely excited about their job, impact, and Parity’s mission - Opportunity to relocate to Germany or Portugal For those joining us as employees in Germany, Portugal, or the U.K.: - 28 paid vacation days per year - Work laptop (macOS or Linux-based) and equipment to enable you to work successfully - £2,500 yearly learning and development budget for conferences or courses of your choice Not a perfect match to our requirements? We're still excited to receive your application and hear how you think you can help us achieve our mission. APPLY ON THE COMPANY WEBSITE To get free remote job alerts, please join our telegram channel “Global Job Alerts” or follow us on Twitter for latest job updates. Disclaimer: - This job opening is available on the respective company website as of 5thJuly 2023. The job openings may get expired by the time you check the post. - Candidates are requested to study and verify all the job details before applying and contact the respective company representative in case they have any queries. - The owner of this site has provided all the available information regarding the location of the job i.e. work from anywhere, work from home, fully remote, remote, etc. However, if you would like to have any clarification regarding the location of the job or have any further queries or doubts; please contact the respective company representative. Viewers are advised to do full requisite enquiries regarding job location before applying for each job. - Authentic companies never ask for payments for any job-related processes. Please carry out financial transactions (if any) at your own risk. - All the information and logos are taken from the respective company website. Read the full article
0 notes
Text
Web Development Frameworks

Introduction
Web development frameworks play a crucial role in simplifying and accelerating the process of building robust, scalable, and interactive web applications. These frameworks provide developers with a set of tools, libraries, and predefined structures that facilitate efficient coding and enhance productivity. In this article, we will explore some popular web development frameworks and their key features, enabling developers to make informed choices based on their project requirements.
1. Angular

Angular, developed and maintained by Google, is a powerful front-end framework for building dynamic and feature-rich single-page applications (SPAs). It follows the Model-View-Controller (MVC) architectural pattern and provides a comprehensive toolkit for building complex user interfaces. Key features of Angular include:
Two-way data binding for seamless synchronization between data models and views.
Dependency injection for modular and reusable code.
Component-based architecture for building reusable UI components.
Routing capabilities for managing application navigation.
Support for TypeScript, a statically-typed superset of JavaScript.
2. React

React, developed by Facebook, is a popular JavaScript library for building user interfaces. It is widely used for creating interactive and responsive web applications. React follows a component-based approach, where UIs are divided into reusable components. Key features of React include:
Virtual DOM (Document Object Model) for efficient rendering and performance optimization.
JSX (JavaScript XML) syntax for writing component templates.
Component lifecycle methods for handling various stages of a component’s lifecycle.
Unidirectional data flow for predictable state management.
Huge ecosystem of libraries and tools for enhanced development.
3. Vue.js

Vue.js is a progressive JavaScript framework that is gaining popularity due to its simplicity and flexibility. It is designed to be incrementally adoptable, allowing developers to gradually integrate it into existing projects. Key features of Vue.js include:
Easy learning curve and straightforward syntax.
Reactive and declarative rendering for efficient data binding.
Component-based architecture for building reusable UI components.
Vue Router for managing application routing.
Vuex for state management in larger applications.
4. Django

Django is a high-level Python web framework known for its robustness, security, and scalability. It follows the Model-View-Template (MVT) architectural pattern and emphasizes the principle of “Don’t Repeat Yourself” (DRY). Key features of Django include:
Built-in admin interface for effortless administration of database models.
Object-Relational Mapping (ORM) for database interactions.
URL routing and view management for handling HTTP requests.
Template engine for separating HTML code from business logic.
Authentication and authorization mechanisms for building secure applications.
5. Ruby on Rails

Ruby on Rails, commonly known as Rails, is a popular full-stack web development framework written in Ruby. It follows the convention-over-configuration principle, allowing developers to focus on writing application logic rather than boilerplate code. Key features of Ruby on Rails include:
ActiveRecord, an ORM for database interactions.
Convention-based naming and directory structure for rapid development.
ActionPack for handling HTTP requests and generating responses.
Integrated testing framework for easy testing of applications.
Support for building RESTful APIs.
Conclusion
Web development frameworks provide developers with the necessary tools and structures to streamline the development process and create efficient, scalable web applications. Angular, React, Vue.js, Django, and Ruby on Rails are just a few examples of the wide range of frameworks available. Each framework has its own strengths and suitability for different project requirements. By understanding the key features and characteristics of these frameworks, developers can make informed decisions to build robust and successful web applications.
FAQs (Frequently Asked Questions)
FAQ 1: What is the difference between a web development framework and a library?
A web development framework provides a complete set of tools, libraries, and predefined structures for building web applications. It often includes features like routing, data binding, and template rendering. On the other hand, a library is a collection of prewritten code that developers can use to perform specific tasks, such as manipulating DOM elements or making HTTP requests. While both frameworks and libraries can simplify development, frameworks typically provide a more comprehensive solution for building entire applications.
FAQ 2: How do I choose the right web development framework for my project?
Choosing the right web development framework depends on various factors, including the complexity of your project, your familiarity with a particular programming language, and the specific requirements of your application. Consider factors such as community support, documentation, learning curve, performance, and the availability of plugins or extensions. It’s recommended to conduct research, explore tutorials, and try out different frameworks to assess their suitability for your project.
FAQ 3: Can I use multiple web development frameworks together?
In some cases, it is possible to use multiple web development frameworks together, depending on the requirements of your project. However, it can introduce complexity and potential conflicts between different frameworks. It’s important to carefully evaluate the compatibility and integration possibilities before combining multiple frameworks. In some cases, it might be more efficient to choose a single framework that provides the necessary features for your project.
FAQ 4: Are web development frameworks only for front-end development?
While web development frameworks are commonly associated with front-end development, there are also frameworks available for back-end development. Frameworks like Django and Ruby on Rails are examples of full-stack web development frameworks that provide tools and libraries for both front-end and back-end development. These frameworks typically offer features for database interactions, server-side rendering, and handling HTTP requests.
FAQ 5: Can I build a web application without using a web development framework?
Yes, it is possible to build a web application without using a web development framework. However, using a framework can significantly simplify the development process, enhance productivity, and provide standardized practices. Frameworks handle common tasks, such as routing, data management, and template rendering, which can save developers time and effort. Additionally, frameworks often have active communities and extensive documentation, which can be beneficial for troubleshooting and learning best practices.
0 notes
Text
Top 10 Angular-Built Websites And Applications
Angular is a popular front-end web application framework that is extensively used for designing single-page web applications. This framework was developed by Google. It has been a leader among the most popular frameworks on the web since the moment it came into being in 2009 and continues to be of great significance. Its first version was AngularJS, also known as Angular 1. For many years, this version was widely used, and several well-known apps were created using AngularJS. However, once Angular v.2 was released, it lost the "JS" ending and the framework is now just known as Angular.
Angular is used to create a lot of websites and applications because of its outstanding functionality, simplicity, and potential. Websites built with Angular are user-friendly, feature-rich, and responsive. Angular apps and websites have established a strong reputation and have been used by tech giants like Microsoft, Gmail, and Samsung. With time, Angular has continued to reach new heights, grabbing big fish in the industry like Gmail, PayPal, and Upwork. So, what exactly is Angular? Let’s take a look!
What is Angular?
Unless you are already familiar with it, Angular is an open-source framework for web application development. Misko Hevery and Adam Abrons found Angular in 2009. It is based on TypeScript, a statically-typed superset of JavaScript, and provides a set of powerful features to design web applications. Web solutions built in Angular are scalable, dynamic, and responsive. Angular is a crucial component of the popular frontend development combo the ‘JavaScript Big 3’ – a combination of the frameworks React, Angular, and Vue.
It has features like MVC architecture, an extensible template language, bidirectional data flow, and HTML templates that make it easier and more convenient for web developers to create top-notch AngularJS websites. Angular also includes a powerful set of tools for managing application state, routing, form validation, etc. Moreover, it provides seamless integration with other popular libraries and frameworks, such as RxJS, Bootstrap, and Material Design.
Angular comes with a component-based architecture. An Angular application has a tree of components. Here, each component is a fundamental building block of the user interface. Components can be reused across different parts of an application and can communicate with each other using services.
Some of the key benefits of partnering with an AngularJS development company for web development include faster development time, better code organization and maintainability, improved performance, and an improved end-user experience. Angular is widely used by developers and companies around the world and has a large and active community of contributors and users.
Best Examples of Angular Applications and Websites
1. Gmail
One of the top Angular application examples is Gmail. What’s a better way to prove Angular’s potential than by handling the world’s No. 1 email service? If you take a good look at the Gmail service, you will find out how powerful Angular is. Gmail has been using Angular since 2009. Gmail has more than 1.5 billion daily users. And, Angular helps it to effortlessly handle the heavy traffic generated by such a huge user base. As such, this single-page app runs speedily, flawlessly, and without crashes.
Take a look at the key features of Gmail! It offers functionalities like a simple and intuitive interface, data rendering on the front end, and offline access to cached data. Angular manages every action within a single HTML page, whether you're reading an email, writing a new message, or switching tabs. When new emails and messages come in, it provides data on the front end at once. Instantaneous initial loading takes no more than a few seconds, after which you can start working with emails and tabs right away. Even if you go offline, you can still get access to some of the features.
Gmail's innovative feature ‘live Hangouts chats’ is simple and yet, powerful. You can add Hangouts to your Angular apps. The other notable features include mail drafting with in-built syntax and grammar, predictive messaging, and spell-check.
2. PayPal
I am sure that most individuals, even the ones without technical backgrounds, know about this multinational payment company. This angular application acts as an electronic replacement for conventional paper methods like cheques and money orders and supports rapid online money transfers. The company operates as an online payment processor and more than 305 million people have active accounts on PayPal's platform. The app performs flawlessly and can handle substantial traffic.
Because PayPal is used for financial transactions, it requires an instant, real-time data transfer with high-tech security features. Thanks to Angular, the app caters to these requirements successfully. Using the Angular tool checkout.js, PayPal users enjoy a seamless checkout experience. Customers can make purchases and complete the entire transaction without ever leaving the website.
3. Forbes
Forbes is a popular American business magazine that publishes articles on business, industry, making investments, and topics associated with marketing. Related topics like technology, communications, science, politics, and law are covered by Forbes as well. The Forbes website was developed using Angular 9+ and various cutting-edge technologies, including Core-JS, BackboneJS, LightJS, and others. It is one of the most visited websites in the world with more than 74 million monthly users in the US.
Many Forbes readers belong to the business world or are celebrities. Hence, the website is expected to work smoothly and take minimal time to load accurate information from dependable sources. Thanks to Angular, Forbes offers an uninterrupted and enriching UX. Angular’s code reusability feature, makes the Forbes website performant on any operating system, browser, or device. Besides, the website boasts of features such as self-updating pages, an attractive design to attract attention, and simplicity in use.
4. Upwork
Upwork is one of the most popular Angular apps available. This service makes it possible for companies to locate freelancers, agencies, and independent professionals for any kind of project from any corner of the world. When the COVID-19 pandemic broke out three years ago, there was a recession that damaged the infrastructure of many industries. During such moments of crisis, Upwork helped many companies and freelancers by connecting them.
Using Upwork, businesses can find a freelancer who satisfies their specific requirements. At the same time, the platform helps freelancers to find out employers and projects that suit their requirements. Freelancers can search for jobs by applying filters like project type, project length, etc. So this "world’s work marketplace" has millions of users on its website daily, which is operated smoothly by Angular. Upwork uses Angular as part of its technology stack to offer a responsive single-page interface. Thanks to Angular, Upwork has features like mobile app calling, payment getaways, intuitive architecture, and private security and verification mechanisms. Like many other Angular-written websites, Upwork exhibits excellent webpage performance.
5. Deutsche Bank
Another great example of an AngularJS website is Deutsche Bank, a German investment and financial services company that uses AngularJS. Angular was used to create the developer portal's front page. Deutsche Bank’s Application Programming Interface can be accessed through the developer’s portal. This API serves as a point of access for the numerous international organizations looking to integrate the transaction systems of Deutsche Bank into their web software solutions.
6. Weather.com
This website, as its name implies, provides weather forecasts. It was bought by IBM in 2015. When examining Angular websites, weather.com is one to keep an eye out for. There is no better way to find out how powerful Angular is than to analyze how weather.com works.
In addition to providing accurate hourly and daily weather forecasts, the website also provides daily headlines, live broadcasts, factoids, air quality tracking, and entertainment material. The best example of how Angular functions is Weather.com, which displays real-time broadcasting on websites or apps with numerous geographical locations. Angular makes it simpler to process data on websites with a large number of users and frequent changes. Not only does it show high-definition aerial shots of places, but also provides alerts on severe weather conditions and weather-related disasters.
7. Delta Airlines
Among many AngularJS web application development models, one such website is Delta. One of the most competitive American Airlines, Delta Air Lines provides flight tickets to 300+ locations in 60 different countries. They, therefore, have a large number of users on their website at once, thanks to this level of service.
Angular 9+ was used by Delta to create a robust and quick website. Delta has deployed the framework on its homepage, and that decision has been a game changer for them.
8. Guardian
The Guardian is a UK-based newspaper that was started in 1821. It’s not surprising that The Guardian has developed into a dependable source of news and information throughout the world, given that it first provided news offline and later had a sizable online presence.
It is available online and has been developed with AngularJS. It provides reliable news from all over the world and reaches several thousand people every day, be it about finance, football, etc. So due to the endless number of people spending time on their websites and generating traffic, they used AngularJS to create a highly readable and accessible web app.
9. Wikiwand
Wikiwand founder Lior Grossman once said: "It did not make rational sense to us that what is now the fifth most widely visited website in the globe, which is used by nearly one-half of the world's population, has an interface that has not been up-to-date in more than a decade." We realized that Wikipedia’s UI was cluttered, tricky to read (large segments of tiny text), impossible to navigate, and not user-friendly. So, their team leveraged Angular app development to create Wikiwand, a software wrapper meant for the articles of Wikipedia.
From children to adults, we all use Wikipedia as our source of information for so many things. Even though we all love Wikipedia, we will have to admit its interface is outdated, difficult to read and navigate, full of distracting stuff, etc. This is where Wikiwand uses its magic; it provides a modern touch to Wikipedia pages with the use of Angular technology. It has enhanced the readability as well as navigation. Now, you get advanced navigation features on both the web and mobile apps which ultimately results in a better user experience.
10. Google
Google uses a lot of technologies to improve its services. Given that Google created Angular in the first place, it makes sense that Google would use it. That’s why it is one of the best examples of an Angular application. Because Angular is so effective for single web pages, Google uses it in many of its products.
Google Play Store
Google Voice application
Google Open Source
Google Play Book
The Google.org website
Google Arts and Culture
Wrap Up:
This concludes our list of the top 10 Angular websites and Angular applications, and well-known companies that best demonstrate Angular's potential. Anything that well-known and large companies use must be of the highest caliber. The Angular webpages and web applications that are listed in this article are all widely recognized globally. If there is one thing you should take away from this, it should be that Angular is a flexible framework that can improve the usability and interactivity of your web applications.
Now that you've gotten some helpful recommendations on the kinds of projects for which this common framework is suitable, you know when it's most important to focus on building Angular webpages and which Angular tools to use to get the best results. Therefore, it is important to seek technical assistance from a specialized Angular development agency before beginning any project.
0 notes