#E-currency Exchange Script
Explore tagged Tumblr posts
Text
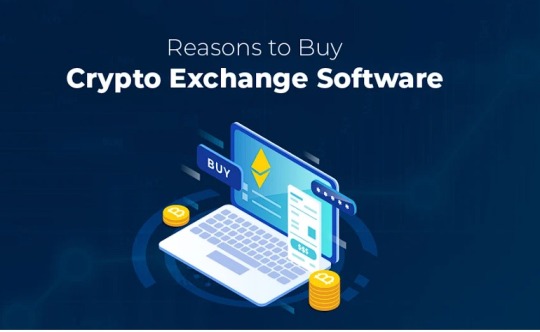
Promotion for the purchase of a script for creating an online exchanger. Script from the company "iEXExchanger iexbase" with a $1000 discount! Discounts apply through a referral program or promotional code. Go to the developer's website: https://exchanger.iexbase.com/?ref=09d4213a-3141-11ee-86fa-a3cd7d0542a9
#iEXExchanger iexbase#iexbase#iEXExchanger#moneytop#premiumexchanger#exchanger script#exchanger cms#Premium Exchanger#E-currency Exchange Script
1 note
·
View note
Text
While the world is heading towards US dollar-pegged stablecoins, Akshay Naheta, a former executive at SoftBank, is pioneering a distinctive stablecoin called DRAM, backed by the United Arab Emirates Dirham. Last month, Paytm entered the crypto arena with its USD-pegged stablecoin PYUSD and got good support within the industry. Naheta, Ditched USD for Dirham? Will it Work? But what made this collaboration go against the market wave? With DRAM Trust, Naheta aims to tap into the burgeoning stablecoin market, which analysts project to expand over 20X, reaching a value of $2.8 trillion in the next five years. DRAM coins are engineered to provide stability, particularly in nations grappling with high inflation, such as Turkey, Egypt, and Pakistan. The initiative primarily targets the unbanked and underbanked populations. “Our main focus is the unbanked and underbanked in these nations. If you want to diversify your risk and be in a currency complementary to the dollar, a big percentage of money can move into this.”In contrast to many stablecoins typically pegged to the US dollar, DRAM sets itself apart by tethering its value to the Dirham. This stablecoin will be accessible on decentralized exchanges like Uniswap, Sushiswap, and Pancakeswap. Furthermore, the team plans to collaborate with centralized exchanges shortly.This innovative project is anticipated to gain substantial traction in the UAE, owing to its significant expatriate community and proximity to regions with high inflation rates. The decision came to light when the US government faced a series of troubles and may be on the verge of shutdown. This event can favor the coin and could trigger instant adoption. Chanelising UAE’s Financial Stability & DTR’s Global PlanAkshay Naheta’s venture underscores the growing influence of the UAE in the global financial landscape. He likens the nation’s role to that of Switzerland, citing its geopolitical neutrality, robust transportation infrastructure, and prominence as a top-tier tourism destination.DTR, headquartered in the Abu Dhabi Global Market, is committed to democratizing finance by developing digital financial technologies. The firm also has plans to introduce a decentralized wallet solution in early 2024, aiming to enhance the accessibility and functionality of digital tokens for a wider audience.!function(f,b,e,v,n,t,s) if(f.fbq)return;n=f.fbq=function()n.callMethod? n.callMethod.apply(n,arguments):n.queue.push(arguments); if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0'; n.queue=[];t=b.createElement(e);t.async=!0; t.src=v;s=b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t,s)(window,document,'script', ' fbq('init', '887971145773722'); fbq('track', 'PageView');
0 notes
Link
Introdução: Se você está interessado em entrar no emocionante mundo das criptomoedas e deseja criar sua própria plataforma de troca de dinheiro e bitcoins, não procure mais! Desde 2017, o Exchangerix tem sido uma escolha confiável para empreendedores que desejam estabelecer sua presença no mercado de trocas de criptomoedas. Com um script PHP robusto e uma base de mais de 2.000 clientes satisfeitos, o Exchangerix oferece uma solução completa para atender às suas necessidades de troca online. Neste artigo, exploraremos os destaques deste software poderoso e como ele pode facilitar o lançamento do seu próprio site de troca.
O Poder do Exchangerix: O Exchangerix ganhou uma reputação sólida ao longo dos anos devido à sua poderosa plataforma de troca. Com um rico script PHP no núcleo, ele oferece uma ampla gama de recursos e funcionalidades para garantir uma experiência de usuário excepcional. Seja você um novato no mundo das criptomoedas ou um especialista experiente, o Exchangerix foi projetado para ser facilmente configurável e personalizável de acordo com as suas necessidades.
0 notes
Text
Why is the adoption of cryptocurrency increasing in the market?
Cryptocurrency, as everyone knows has become a crucial part of the finance industry. The decentralized currency is being admired in the current world for its extremely high values. This currency has influenced the market to a large extent. As a result, an increasing number of businesses have started accepting crypto coins for making payments. The biggest names such as Starbucks, Microsoft, Paypal, and others are offering the option to pay the bills using cryptocurrency. Let’s explore why the cryptocurrency is being accepted largely in today’s world:
Rapid transactions: The transactions done using Cryptocurrencies can be easily and rapidly done throughout the world due to the cross-border functionality. Thus, expanding the reach of a business to an international level has become convenient. Unlike centralized currency transactions, there is no middleman required for processing the transactions, thus the process becomes cheaper and quicker.
Economical transactions: Cryptocurrency uses blockchain technology for transactional purposes, therefore, the overall expense to be spent on the transactions is reduced drastically with the elimination of a third party and other processing charges.
High security: Businesses are privileged hugely with the admirable feature of blockchain technology. The irreversible nature of blockchain technology helps in neglecting fraudulent activities and resolves several issues being faced by e-commerce sites.
Modernized business: Accepting cryptocurrencies in the current scenario is one of the trendiest and most advanced offerings by a modern business. It may attract several individuals and crypto users to your business. In order to establish a business that offers a wide range of payment options, businesses are widely welcoming payments using crypto coins. This is contributing to the popularity of the crypto industry.
Currently, the crypto market is immensely popular, and more and more individuals are investing and adopting cryptocurrencies to stay advanced in today’s time along with enjoying several benefits of the industry. The demand for crypto platforms is rising to a new level in such a scenario. You can also take advantage of the current trends and develop a crypto-based business to avail of huge benefits.
To get any kind of crypto-related services such as crypto exchange development you can get in touch with Zeligz web store’s highly experienced team. Their crypto exchange script has been developed with commendable features and can be customized according to the client’s needs. Their professionals are extremely talented and can offer you the results that will lead you to the path of success.
#crypto hyip script#cryptocurrencies#bitcoin#script#smart contracts#blockchain#cryptocurreny trading#hyip script#crypto exchange script#development#crypto#exchange#scripts
2 notes
·
View notes
Text
What are the legal and regulatory aspects that startups need to consider when using Cryptocurrency Exchange script?
What is Cryptocurrency Exchange Script?
A Cryptocurrency Exchange Script is a pre-built exchange software solution that paves the way for startups to establish their own cryptocurrency exchange, akin to the world's leading trading platforms. This script is designed with all the advanced features and security components necessary for the seamless operation of an exchange platform.
Cryptocurrency Exchange Script Development:
With this script in hand, you can create a feature-rich cryptocurrency trading website in just 7 to 10 days, providing a significant advantage for your business. It offers support for a wide range of reputable cryptocurrencies, including Bitcoin, Cardano, Ripple, Ethereum, Bitcoin Cash, BNB, Matic, Shiba Inu, Dogecoin, and many others.

Key Features of Cryptocurrency Exchange Script:
User Authentication:
Secure user authentication is a fundamental feature of any cryptocurrency exchange script. This encompasses user account creation, two-factor authentication, and password management.
Wallet Management:
The script empowers users to manage their digital currency wallets, enabling them to view their balances and conduct cryptocurrency deposits and withdrawals.
Trading Engine:
The heart of the cryptocurrency exchange, the trading engine, facilitates the buying and selling of digital currencies. It includes advanced features such as order books, trading charts, and order matching algorithms.
Payment Gateway Integration:
Seamlessly integrate payment gateways, allowing users to deposit funds into their accounts and make withdrawals. Popular payment methods encompass credit cards, bank transfers, and e-wallets.
KYC/AML Procedures:
To combat fraud and illegal activities, many countries require Know Your Customer (KYC) and Anti-Money Laundering (AML) procedures. The script comes equipped with built-in procedures to meet these requirements.
Multilingual Support:
To attract users from across the globe, the cryptocurrency exchange script supports multiple languages, ensuring inclusivity.
Customer Support:
A robust customer support system is essential, including features like a help desk, live chat, and a support ticket system to assist users efficiently.
Security Features:
Cryptocurrency exchange scripts are fortified with robust security features to shield against cyberattacks, data breaches, and other security threats.
Customizability:
A quality cryptocurrency exchange script allows businesses to customize the platform to align with their unique needs, including branding, color schemes, and additional features.
These are some of the core features you can expect from a typical cryptocurrency exchange script. It's worth noting that different software solutions may offer additional or different features to meet specific business requirements.
Why Choose Hivelance for Your Cryptocurrency Exchange Script Development?
Hivelance stands as the foremost provider of cryptocurrency exchange software services, offering a competitive edge. With extensive experience in blockchain solutions, including cryptocurrency exchange script development, our team comprises experienced developers, designers, and project managers to guide you through the development process, ensuring the success of your exchange. We provide a range of benefits, including expertise, customization, security, and unwavering support, for businesses seeking to develop their cryptocurrency exchange script.
Grab Up To 73% Off on Black Friday Deals for all our services like Cryptocurrency Exchange Script Development Services. Launch your Cryptocurrency Exchange Platform easily!
#cryptocurrency exchange script#cryptocurrency exchange software#Cryptocurrency Trading Script#Bitcoin Exchange Script#Bitcoin Trading Script#Bitcoin Exchange Website Script
1 note
·
View note
Text
PROJECT RIPRO
Social and economic project
Common words about the project
At the top of the page is the moto of our platform, which is being made for good purposes of making first crypto currency with payments to every registrated user of our site, with the possibility of using this money directly. If somebody stats to analyze the ICOs he can see that many of them lack possibility to use their coins like usual money. It is almost always about investments but not about using them as payment. In the opinion of our team, this problem is the main reason why crypto currencies aren’t used widely. Our platform will try to fix it.
Purpose of our project
So, let us try to describe our project. It will be a site, which will have its own currency called Krist. 1 Krist will consist of Quants. This currency will have many differences from other crypto currencies; one of them is an annual inflation of 4%. In case of emergency, it can be changed. If 20% of active users will vote for an additional inflation the site will do so, but overall annual inflation will be not more than 4%. It means that, for example, if we print 5% this year we will be obligated to print 3% next year. Inflation is needed to encourage people not only to invest but also to spend our cryptocurrency.
Inflation will be made by neomining. New coins will be issued not using the technology of blockchain but using the servers that will support our site. Like decentralized hosting. The crypto currency will be distributed according to the power of the server of the miner. However, not all the inflation will go directly to miners (only 30%). Other coins in the same shares will be distributed to people and to the administration. Also 10% from the income of the administration will go to the users. All payments will be distributed every hour.
Business also can be conducted on our platform. To have access to that you will need to click on a special button in the account menu. No ID or other personal information will be needed. Even login and password can be generated by the system when first registration should be done. That also provides some anonymity to our accounts.
The economics of our platform
Now it is the time to speak about practice. This part is called economics by our team. It will consist of:
· Stock market
· Crypto currency market
· Freelance market
· Content service
· E-market
· Crowdfunding service
· Exchange market
· Casino
· Private banks
For every service different conditions apply. For example, to get a loan from a private bank you will need to provide a copy of your ID, make a photo with the original, open a copy of your ID on your mobile device and make a selfie from other device. Also you have to make a video where you will have to say your name and wrote it on a paper. It is done like that because of security reasons.
Stock market
On this service, the requirements are dependent on the level of usage of the service. There are some levels:
1. The base. The possibility only to sell and buy stocks, using the average market price. You will need a usual account.
2. Basic level. The possibility to sell and buy stocks and to define their price. Business account is required.
3. Middle level. The previous possibilities plus betting on average price for any time. Business account is required and trade experience of 72000 basic hour payments. It is the last level, which is given automatically.
4. Maximum level. The possibility of betting, trading and making your own stock market. Business account is required, trade experience of 72 million basic hour payments, service fee also have to be payed. There can be some exemptions.
Stocks can be issued by every business accounts, even without token movements. No level requires full identification. Service fee on every transaction on stock market is 2%.
Crypto currency market
On this service is almost the same situation, but there are some difference. There is not possibility of buying and selling crypto currencies. Only betting on the future price. There are two levels. The requirements are the same as third and fourth level, but for the first level you will not need any betting experience. Service commission is 2%.
Every user can issue his own crypto currency and bet or trade it, also he can buy something if the seller agrees to accept it.
Freelance market
In this part of the resource, it is possible to offer a service or to make a request. Every diversion will be a different page, where you can find offers. Clicking to a special button, you can move to the pages with requests. For shopping you need usual account but for selling you need business account. Services will be available on this diversions:
v Design:
o Logotypes
o Banners and icons
o Business cards
o Web and mobile designs
o Design in social webs
o Graphic design
o Ready chaebols and paintings
o Art and illustrations
o Vector graphic
o Photoshop
o 3D graphic
o Advertising
o Package design
o Style
o Presentations and infographics
o Interior and exterior
v IT
o Layout
o Site settings
o Making a site
o Bots and scripts
o Domain and hosting
o Usability and testing
o Mobile apps
o Programs for PC
o Games
o Computer and IT help
o Server administration
o Making technical documentation
v Texts and translation
o Articles
o Business texts
o Literature
o Resume and vacancies
o Filling with context
o Text typing
o Text correction
o Translation
o Post for social networks
v SEO and traffic
o Audits and consultations
o SEO optimization
o Semantic core
o Links
o Traffic
v Advertising and PR
o Advertisement
o Context advertisement
o Social webs and SMM
o Bulletin desks
o E-mail marketing
o Data bases
o Client bases
o Statistics and analytics
v Audio and video
o Audio recording
o Music and songs
o Audio correction
o Videos
o Introduction and animation
o Filming and montage
v Business and life
o Consulting
o Personal adviser
o Project management
o Accountancy and taxes
o Juridical help
o Site selling
o Telephone sells
o HR
o Repairs
o Building
v Other
Service fee is 1%
Content service
It will consist of:
· Photo bank
· Video bank
· Wikipedia analogue
· Video hosting
· Social web
· Library
· Game hosting
Photo bank and video bank are the same as freelance market but without diversions. Business account is required for entering. In addition, documents can be required. Five percent service fee.
Wikipedia analogue. In our opinion, working with tons of information without rewarding is not right. Every account can right or edit any articles. Reward will be distributed according to the percentage of all views. If somebody edits the article than he gets the profits. Video hosting is about the same.
Social web. It will be very simple. There will be a messenger, user pages, blog pages, and societies. Also there will be a feature with premium societies. These societies will provide special content and they will have subscriptions. On those subscriptions 5% fee will be imposed.
Library and game hosting will be very similar. There will have a possibility to publish your own game or book for every type of account. Reward will be distributed according to number of readings (full book) or games.
For every type of service except photo and video banks can be different subscriptions for each of them. Any user can purchase common monthly subscription for all the services (with premium societies). A 5% fee will be imposed on each subscription. After all income to the administration from content service will be less than 0.1% all subscriptions will be free. Common monthly subscription before cancelation will be exact 20% from the monthly basic payments.
E-market
A marketplace with the possibility to pay in crypto currencies. There will be ranging by regions, countries, cities and even districts. Anybody can add their division and get 1% income from all sells in that place. This action will have to be confirmed by the administration. Everything can be done by usual account. 5% service fee.
Crowdfunding platform
Every account can raise funds. There will be a list of ongoing projects with brief description in 3 sentences. Clicking on this description you can enter the main page with full description and invest button. You can donate any sum. Every withdraw fee is 1%. However, if you had not confirmed your real ID before withdraw a 10% security fee will be imposed. There will be three types of projects:
1. Personal goals
2. Charity
3. Business
Crypto exchange market
The interface will be almost the same as freelance market. You can demand some currency or you can offer. Everybody can participate. In addition, any account can set their own exchange rate and exchange fee. However, on every transaction 2% service fee will be imposed. On the main page, there will be some statistics like average exchange rate of the previous day.
Casinos
Every account can participate. There will be many different services with average wining coefficient of 96%. In addition, many another interesting features:
· It will be possible to make your own casino when you will meet the requirements of the administration. On all profits 10% transaction fee will be imposed.
· You can sell your game code on special market. 10% service fee.
Private Banks
There will be an administration bank. It will give loans to private banks. Only those banks will loan money to users. Private bank can sell ID and loan of the person who is not paying his bills for 6 months. For both obligation 5% fee will be imposed. Only business account can create his or her private bank after meeting administration requirements.
Education
This part consist of two services – personal education and mass education. Personal education is basic type accessible by every account. Any price can be charged. There will be a special service on the site for that. Where you can either request a teacher or offer a course. In addition, you can teach on the same platform. However, there is limitation of one pupil at a time.
Mass education will be divided on these types:
· School
· College
· University
On all types of mass education there will be fixed price of 0.5 of monthly basic income. Usual account can only create a school. There will be an opportunity to sell and teach a course to big crowd of people. In addition, there will be an automatic payment system. College is a union of schools teaching many subjects in one course. Universities will enroll students of this type of education without any exams. Also college will have possibility to issue a certificate and register the pupil in a special system where his paper can be checked. Universities are almost the same as colleges. However, here many teachers are discussing one subject. It is the final step of education in our platform.
Creation of colleges and universities has to be organized with the administration. On every transaction 1% fee will be imposed. But if the income from education sector will be less than 0.1% there will not be any fee and mass education will be free.
Referral system
There is possible to enroll other people and profit from that. A user can use special link. When people register under this link, the first person receives 1% from their income. If you want to escape this, you need to pay a special sum to the person from who you are referral. Sometimes the administration can help you to do that.
The start of the project
It will be two stages of this event – pre-ICO and ICO. Total 1 trillion coins will be sold. 90% of that will go to the investors from pre-ICO. It will be a small list of them. Mostly they will be some famous entrepreneurs or big cryptocurrency markets. Only 10% will be offered to the public. It will be a special site, where you can try out the crypto currency payments. For the first type of investors, a special unique code will be offered to access their accounts. They will have a special account. The coins will be distributed according to the percentage of bitcoin investments. Unfortunately, we will only accept bitcoins.
Conclusion
Thank you for your attention! We think you saw some advantages of our project. Our team is thinking that the basic income is the best proposal of this project. It enables to access knowledge with paying the teachers for theirs work and not only the teachers.
If you want to participate - contact me in the chat.
#it#crypto bitcoin#cryptocoin#new technology#finance#cryptocurreny trading#cryptocurrency#universal basic income#opportunity#new currency#social good#money#extra money#moneymaker#monetizze#earncrypto#earnbitcoin#earn money#earnmore#investor#investing101#investment#investingtips#investing#new way of earning money#education#skills#skillbuilding#new skills
2 notes
·
View notes
Text
During a discussion about cryptocurrency regulations, Congressman Warren Davidson recently expressed concerns about the actions of Gary Gensler, the Chairman of the U.S. Securities and Exchange Commission (SEC). Davidson, a House Financial Services Committee member, highlighted the need for transparency and accountability within the SEC.During the conversation with Thinking Crypto, Davidson also discussed possibly issuing a subpoena to Gary Gensler to obtain necessary documents and communications related to the SEC’s activities. He said that patience with Gensler’s leadership had worn thin, and a subpoena might be necessary to ensure transparency and cooperation.Davidson believed that the SEC’s actions and lack of clear regulations have hindered the cryptocurrency industry’s growth. He cited fraud cases and the need for structural changes within the SEC as significant concerns.The discussion also touched on the SEC’s previous stance on Ethereum, where a speech by former SEC official Bill Hinman was considered guidance by the industry. However, recent revelations have raised questions about the ethics and transparency of that decision.He hopes that the recent court criticisms of the SEC’s actions in the Ripple vs. SEC case will make the SEC change its approach to regulating cryptocurrencies. He also believes that the SEC’s current method of using the Howey test as its primary guideline for determining if something is a security is too vague and that there should be clear laws specifically for digital assets. Davidson also said that having real contracts is crucial when the SEC claims something is an investment contract.Regarding Central Bank Digital Currencies (CBDCs), Davidson argued against their implementation, suggesting that they could be used as tools for government control and coercion. He emphasized the importance of sound and strong money and expressed skepticism about the need for a U.S. CBDC.!function(f,b,e,v,n,t,s) if(f.fbq)return;n=f.fbq=function()n.callMethod? n.callMethod.apply(n,arguments):n.queue.push(arguments); if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0'; n.queue=[];t=b.createElement(e);t.async=!0; t.src=v;s=b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t,s)(window,document,'script', ' fbq('init', '887971145773722'); fbq('track', 'PageView');
0 notes
Text
firewhisky on ice, sunset and vine
you’ve ruined my life by not being mine
Chapter 4 --- previous chapter --- next chapter
Harry Potter fics Masterlist
Acceptable. A fucking A in Herbology class, all thanks to the idiot Death Eater on a secret mission that refused to proofread his essay on Niffler’s Fancy. What the hell was Niffler’s Fancy?
Blaise was livid, murderous, on a path to righteous vengeance.
It was the last round of examination of November, meaning that in less than a month their first section of the year would wrap up. Grades were already decided then, and he could not, for all the Work and Effort Salazar Slytherin had put into building the Chamber of Secrets, have anything lower than Outstanding. He’d allow himself a single Exceeds Expectations in Herbology, but never an Acceptable. That didn’t ‘threw a wrench in his plans’, as Pansy had mockingly said that morning; it utterly ruined his future career and he would not, for the life of him, let a stupid plant destroy everything he had worked hard for.
In the past, he had always managed fine in the class, even with some difficulties: who was he to understand whether the green leaves were ripe enough for a change of pots and why should he care, after all. If it was up to him, the pots would be charmed to automatically know those kinds of things, yet Professor Sprout refused his suggestion. Actually docked Slytherin of 5 points, which he then got back in Transfiguration.
In the past, he could count on a best friend who was as competitive as he was, to help him focus and study something he truly hated, that read through his essays and corrected the very few mistakes and that let him sometimes borrow his own work. It wasn’t cheating, it was collaboration. A currency that was well used in the Slytherin common room. It wasn’t as if Draco didn’t receive his share: au contraire, he rarely did Transfiguration on his own, always aided by Blaise, who, in turn, shared his own work.
That was a fool-proof way to succeed.
But of course Draco Fucking Malfoy had to mess up yet another thing and utterly wreak Blaise’s carefully thought plans.
He had to find a solution, as soon as possible. He had to get at least O on the next essay on the effects of Lumos Solem on the Devil’s Snare, otherwise he could easily kiss goodbye to his nearly perfect grades. He could easily ace the charm part of his composition, for obvious reasons, and probably would’ve managed to get an E rather easily, but he simply couldn’t allow the opportunity to slip.
He had to get an O, no matter the cost.
Which was why Blaise Zabini, renowned Sixth Year Slytherin, Pureblood, Heartthrob, Genius and overall Perfect in Every Way, remained seated on his chair in the greenhouse they currently used for their studies, glaring at his roll of parchment that had failed him once again and checking with the corner of his eyes the quickly emptying room. To anyone, he looked as if he was just packing up slowly, with a bored expression on his face.
In actuality, he was waiting. Waiting for Neville Longbottom to stop being a perfect assistant and leave the room so he could corner the Gryffindor and make his offer. Did he really have to fucking rearrange all the plants on the west side of the room and to colour coordinate the entire glove section right at the moment?
Blaise was desperate, that much was true, but he had his limits: if the bloody plant-head wasn’t done in the next two seconds, he’d accept his fate. Or so he told himself, until said boy moved to grab his seat to fix his bag, springing Blaise to hasten his own process and quickly leave the room before the other boy.
Once he was out of the door, he checked the corridor. While he wasn’t doing inherently illegal per se, he was still one of the best and most prominent Slytherins, and he definitely couldn’t be seen border-lining begging for help from Schlongbottom of all the people. Even Granger might’ve been a better choice at this point, and only because she was the best at everything.
Taking a deep breath, he rehearsed once more his offer in his head, conscious that he had to sound convincing and stern, while also seeming approaching and focused. He had calculated everything: the words, the pace, the stance.
“Excuse me?” came a deep voice from behind him, startling him out of his mind. He had spaced out in the moment of need and was blocking the door to the greenhouse, with a very timidly looking Longbottom staring sheepishly at him.
“How in the actual fuck is he managing to be hot and cute at the same time?” Blaise’s mind took shortcut, shifting its gears into a totally different direction than the one meant at the beginning.
He was speechless. His great offer forgotten, he was looking up at the dorky Gryffindor with what he hoped was a puzzled expression and not a starstruck one. It had become his Achille’s Heel: during their Transfiguration classes, Blaise had found his mind wander towards the other boy, whenever Professor McGonagall wasn’t talking; in the Great Hall, he would turn around and see him with his group of Gryffindors and he’d be rendered baffled by his bright laugh, or, in several occasions when he didn’t have full control over his brain, he’d actually look for Longbottom, whether by scanning over the crowds to see his head or by being in places where he might be as well, even if those were more on the ‘accidental encounter’ side. He had once remained stuck in the library, looking for a book, cause he had caught a glimpse of the Gryffindor studying with a muggle pencil on his bottom lip. Needless to say, he didn’t do many productive and public things that day.
Suddenly, one of his mother’s rules made him remember who he was and what his mission was: ‘Rule number sixteen: do not, under any circumstances, act foolishly around the person you like.’ And so he tried not to.
“Longbottom” he began with a cold and distant voice, trying not to seem nervous but slowly boiling inside, “I would like to make you an offer.”
“Zabini” the other boy said, instantly frying Blaise’s brain as he fixed his bag on his shoulder and moved to lean against the doorframe, “[ic1] what makes you think I would even consider accepting?” That was very much not part of the plan. He wasn’t prepared for Longbottom to talk back with such confidence and all his blood rushed downwards, leaving his brain and making him forget his façade. He was once more dumbly staring, mouth slightly agape as he tried to recompose himself as quickly as possible. He cleared his throat once, to mask his discomfort, before proudly announcing: “It would be extremely beneficial for both of us.”
Once again, bloody Longbottom did something that wasn’t scripted in Blaise’s plan: he rose up a questioning eyebrow[ic2] , looking him up and down and studying him silently for a few heartbeats. It was a furnace under his robes and he was positive he might combust any moment. Longbottom didn’t flirt with anyone, for crying out loud, so Blaise didn’t have a single way to tell if he was being mistaken in his assumption or not! He also was not aware of the other boy’s sexuality, therefore the territory was not only risky in terms of rejection but also in terms of safety. “Rule number four: don’t put yourself in dangerous positions.”
Eventually, the Gryffindor spoke again, sounding interested but casual at the same time: “Well, if that’s the case, do tell, why me?” he asked with a sly smirk on his face, sight that sent another rush of blood down Blaise’s pants. It clearly had to be meant to be an innuendo. Had to.
Yet Blaise choose to play on the safe side, just that once, because he still was not sure about anything and he desperately needed all the help he could get. “Also, tutoring each other means we’ll work really close and who knows what’s gonna happen in time. Keep it in your pants, Zabini, and finish what you started!”
He bit the inside of his cheek and nervously glanced around the empty corridor, before turning once more towards that freaking tall and slightly ripped plant-head and said: “It pains me to admit it, but you’re the best at Herbology in this gods forsaken school and Salazar help me, if I don’t pass this class with at least an E I’ll burn the ministry to the ground.”
Longbottom seemed to be taken aback by that: either Blaise’s honesty shocked him or he had indeed seen other paths those first sentences lead to. Not too bad, they’d have the time to explore those after the Devil’s Snare essay. Which he had to ace flawlessly, he reminded himself, trying not to get distracted by the hand the Gryffindor had brought behind his neck to scratch it.
“Why not directly the school?” he asked suddenly.
“We have another year to attend here and the ministry is a shitty place” came the easy answer, truthful and honest. Hogwarts was not a bad place and the Ministry could stand a renovation, both in terms of building and furniture, and as organization as well. Especially with the new developments, that place was now filled up with vicious rats. “Gotta agree on that” Longbottom admitted, undoubtedly having his own ghosts regarding the place after his and his friends’ little escapade to the Department of Mysteries. “But you said it’d be mutually beneficial? I can’t see how” he continued, a curious gleam in his eyes sparkling.
That was a topic Blaise had practiced over and over, and he was comfortable with it: “It’s really easy. I noticed you are, for a lack of a better word, a little lacklustre when it comes to Transfiguration and I’d thought I’d offer my services in exchange for your help with those stupid plants.” He did derail off track at the end, mainly because the shame of having an A still burnt him and also due to the fact that plans were, indeed, rather stupid. Longbottom moved quickly into a defensive stance, “Plants are not stupid. Think of how many you use daily, sounds stupid to you?” he asked with a sudden aggressiveness on his tone that Blaise had never heard from him and couldn’t particularly say he minded. “You haven’t really talked much with him outside of immediate necessity. Stop thinking with your dick!”
He quickly tried to return on his original path, claiming: “We have different priorities, I love Transfiguration and you like pretty green leaves.”
“They’re not just green!” Longbottom muttered in a quiet voice, sounding entirely too adorable for Blaise’s brain to handle. Coughing and hoping his cheeks weren’t reddening, he tried to regain his composure after having turned in a very metaphorical mush at the scene in front of him.
“You can think about my offer, but I’d like to know before next week” he said, waving a dismissal hand and moving to walk away towards the staircases for his next class. He was almost near the library when he heard Longbottom talk, “We have a Transfiguration revision on Friday, don’t we?” Turning, Blaise nodded slightly at the approaching boy. “That would be correct, Longbottom.” “Well then, Zabini,” he said, either accidentally or purposefully dropping his voice an octave and utterly destroying any futile attempt of Blaise’s to focus on anything afterwards, “I guess I’ll see you tomorrow after History of Magic in the empty classroom two doors after the Charms corridor.” Blaise was rooted on the spot as the Gryffindor adjusted once more his bag and slowly walked away from him.
Almost as in an afterthought, he tilted his head backwards and stated pointedly: “Wouldn’t want anyone seeing me study with a snake. Is the feeling mutual?” He finished his sentence with what Blaise assumed was a wink, yet with only half a face showing it was impossible to tell.
He remained there, uselessly dumbfounded even after the other boy had left, for Merlin knew how long, trying to remember how to function.
Blaise was so incredibly screwed and briefly wondered if he had made a mistake.
BONUS
Neville: “Ginny I did as you suggested and appeared confident and shit and I felt so powerful and does that make me gay?”
Ginny: “No, Nev, we agreed you like both boys and girls.”
Neville: “Yeah but I like Blaise”
Ginny: “A SLYTHERIN? IN THIS ECONOMY?”
Luna “It’s more likely than you’d think”
Ginny: “Not now Luna. What you’re gonna do?”
Neville: “Idk but he told me he’d help me study so I’m not gonna waste the opportunity, I’ll flirt when there are no books around us cause otherwise I’ll end up with a Troll in Transfiguration”
Luna: “A Troll in Transfiguration is always better than a Troll in the Dungeons.”
#bleville#blaise zabini#neville longbottom#neville x blaise#blaise is a dumb bottom around neville#harry potter#hp#harry potter and the halfblood prince#hphbp#pomona sprite#herbology#herbology classroom#fanfiction#ao3#ao3 link#my favourite half italian wizard#pining#innuendos#mutual#jkrowling#funny
2 notes
·
View notes
Text
A little-known Guide for the Renaissance Merchant
Fifty-two discoveries from the BiblioPhilly project, No. 23/52
Giorgio di Lorenzo Chiarini, Libro che tracta di marcantie et usanze di paesi, Tuscany (Florence?), 1481, scribe: Lodovicho Bertini, Philadelphia, Temple University Libraries, Special Collections Research Center, (SPC) MSS BH 007 COCH, fol. 9r
The cache of Medieval and Renaissance manuscripts housed at Temple University Libraries’ Special Collections Research Center includes an overlooked source for our understanding of Renaissance economics: a rare manuscript copy of the commercial manual in Italian known as the Libro che tracta di marcantie et usanze di paesi or Book concerning the Trade and Customs of Various Places (MSS BH 007 COCH). This finely written manuscript represents a genre of text essential to the Renaissance merchant. In addition to learning the elements of mathematics and geometry (represented in items like the University of Pennsylvania’s LJS 27 and LJS 488), those who traded in the interconnected Mediterranean world of the fifteenth century needed to be well-informed about the types of goods available in a large number of cities, as well as the units of measure and coinage used, their denominations, and their exchange rates with major domestic currencies. Thus, the manuscript at Temple contains well-organized information for converting weights, measures, and money across Western Europe, North Africa, the Middle East, and the Caucasus, with major sections devoted to the trading capitals of Florence, Venice, and Genoa.
Briefly described in the Supplement to De Ricci’s Census,1 and again in an issue of the hard-to-find Temple University Library Bulletin in 1954, the manuscript seems to have escaped the notice of economic historians. The authors of the Supplement understood that the book was related to the much earlier merchant’s manual, Francesco Balducci Pegolotti’s La pratica della mercatura,2 which was written around 1340. But they also noted in passing that the Temple manuscript is actually a complete copy of a later text whose original composition is attributed to the Florentine merchant Giorgio di Lorenzo Chiarini: the Libro di mercatantie et usanze de’ paesi, or Book of Trade and Customs of Countries. Only three other manuscript copies of this text were known to Franco Borlandi, who produced a critical edition of the text in 1936.3 The earliest of these, dated to 1458, is now in Florence, as is another dated 1483 (Biblioteca Nazionale Centrale, cod. Pal. Panciatichiano 72 and cod. Magliabechiano, XXIX, 203, respectively). A third, probably close in date to 1480, is Paris, Bibliothèque nationale de France, ms. it. 911. A comparison between these manuscripts and MSS BH 007 COCH shows marked similarities, though the Temple University manuscript is the only one to have originally included an introductory miniature.
Giorgio di Lorenzo Chiarini, El libro di mercatantie et usanze de’ paesi, Paris, BnF, ms. it. 911, fol. 1r and ibid., Biblioteca Nazionale Centrale, cod. Magliabechiano, XXIX, 203, fol 1r (photo from Borlandi 1936)
Editions of the book were printed in 1481, around 1497, and 1498, while the widespread diffusion of the book’s contents was further assured by its verbatim inclusion within the two earliest editions of Renaissance Italy’s most popular text on mathematics for merchants, Luca Pacioli’s Summa de arithmetica, geometria, proportioni et proportionalità (Venice: Paganinus de Paganinis, 1494; Toscolano: Paganinus de Paganinis, 1523). However, the printed versions of the book abandon the tabular layout of the manuscripts and compress much of the information into running text, rendering it less useful as a reference tool. It seems clear, then, that our manuscript is not based on the first edition of Chiarini’s work, produced the same year as the manuscript.
The 1481 edition of Chiarini’s text (left), and its position in the 1494 edition of Pacioli (right)
Though our manuscript’s introductory miniature on folio 9r has been excised, and the coat-of-arms below it obliterated, the book helpfully contains a colophon on folio 100r stating that it was copied by a certain Lodovicho Bertini on an unspecified day in July 1481. A coat-of-arms of the Bertini family is discernable on a terracotta monument by della Robbia in the church of San Jacopo in Gallicano, north of Lucca 4, and it is not impossible that the same arms were originally present in the manuscript’s escutcheon. On folio 100r of the manuscript there is also an ownership inscription from the following century written by a certain “Matteo di Lorenzo du Matteo di Francesco,” stating that the book was purchased on 25 March 1591 in Florence, by which time it would already have been seen as an antique. Given the changes over time in commercial conventions and currencies, the usefulness of such a treatise would have diminished quickly, especially during the Age of Discovery. Between its possession by this owner (who may have had it fitted with its current binding) and its presence in the collection of the vellomaniac Sir Thomas Phillipps (1792–1872) as his ms. 6994, the book’s whereabouts are unknown.
(SPC) MSS BH 007 COCH, fol. 100r (final page of manuscript, with colophon by Lodovicho Bertini and ownership inscription of Matteo di Lorenzo di Matteo di Francesco)
Though facts about the copyist Bertini’s life are scarce, his work as a scribe is documented elsewhere. A year before completing this book, for example, he is known to have copied another manuscript known alternately as the Pratica di mercatura or Alphabeto di tutti e costumi, cambi, monete, pesi…. This manuscript is now in Pisa, Biblioteca universitaria, ms. 539, and as far as we can tell based on a list of the manuscript’s contents and a partial transcription, it is virtually identical with the present manuscript, despite never having been identified correctly as a copy of Chiarini’s Libro di mercatantie et usanze de’ paesi.5 Knowing the proper of the source of the text, and understanding that it was not an original work by Bertini, we can start to question why he undertook to make two copies of the same preexisting manual. Was one intended for a friend or relative, or for sale? Or did Bertini plan to keep both copies? A comparison of the reproduction and transcription found in Luciano Lenzi’s article from 2003 on the Pisa codex6 with a detail of folio 92v from our manuscript is instructive:
As is evident from this comparison, our copy is written in a more formal script, with a more refined layout. It may have been a keep-at-home copy of the book complete with heraldry and introductory miniature, while the copy now in Pisa may have been intended as a more utilitarian vademecum to accompany Bertini’s travels.
from WordPress http://bibliophilly.pacscl.org/a-little-known-guide-for-renaissance-merchants/
11 notes
·
View notes
Text
Nike Explores Blockchain for Supply Chain Data Collection
The Chain Integration Pilot (CHIP) of the Auburn University RFID Lab in Alabama has published a proof-of-concept whitepaper that seeks to demonstrate the efficiency savings blockchain technology can unlock across the contemporary supply chain.
The proof-of-concept was designed to ingest, encode, distribute, and store serialized data from multiple points throughout the supply chain on Hyperledger Fabric.
The pilot collected live data from brands Nike, PVH Corp., and Herman Kay, and major United States retailers Kohl’s and Macy’s.
CHIP was launched in 2018 and claims to be the first supply chain project to integrate the information pulled from RFID tags onto a blockchain network.
Blockchain as a supply chain data solution
The project saw data pertaining to 223,036 goods uploaded to a distributed ledger. Only 1% of data entries were uploaded by stores, with 87% of data coming from distribution centers, and the remaining 12% originating from a point of encoding.
As such, CHIP determined that blockchain is a functional solution to issues of serialized data exchange within the supply chain. The report concludes that the participating companies were “able to record transactions containing serialized data in a common language and share that data with their appropriate trade partners.”
The paper identifies “a tremendous amount of error and inefficiency in currency supply systems,” estimating that the elimination of counterfeiting and shrinkage in the supply chain could unlock $181 worth of business opportunities.
Traditional supply chain tracking technologies deemed “antiquated”
By contrast, the paper argues that previously existing networks for exchanging are built for “antiquated internet technologies,” and are not suitable to handle the massive volumes of serialized data that are generated throughout the contemporary supply chain.’
The team points to the absence of “an effective, industry-wide solution for exchanging serialized data between business partners,” despite the introduction of serialized data such as RFID tags and QR codes over a decade ago.
Further, the report argues that previous attempts to integrate infrastructure to collect information on masse’ across the supply chain have been “constrained by the industry-wide ineptitude for sharing serialized data.”
window.fbAsyncInit = function () { FB.init({ appId: '1922752334671725', xfbml: true, version: 'v2.9' }); FB.AppEvents.logPageView(); }; (function (d, s, id) { var js, fjs = d.getElementsByTagName(s)[0]; if (d.getElementById(id)) { return; } js = d.createElement(s); js.id = id; js.src = "http://connect.facebook.net/en_US/sdk.js"; js.defer = true; fjs.parentNode.insertBefore(js, fjs); }(document, 'script', 'facebook-jssdk')); !function (f, b, e, v, n, t, s) { if (f.fbq) return; n = f.fbq = function () { n.callMethod ? n.callMethod.apply(n, arguments) : n.queue.push(arguments) }; if (!f._fbq) f._fbq = n; n.push = n; n.loaded = !0; n.version = '2.0'; n.queue = []; t = b.createElement(e); t.defer = !0; t.src = v; s = b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t, s) }(window, document, 'script', 'https://connect.facebook.net/en_US/fbevents.js'); fbq('init', '1922752334671725'); fbq('track', 'PageView'); Source link
The post Nike Explores Blockchain for Supply Chain Data Collection appeared first on CoinRetreat.
from CoinRetreat https://ift.tt/2xhmQ0v
1 note
·
View note
Text
Nike Explores Blockchain for Supply Chain Data Collection
The Chain Integration Pilot (CHIP) of the Auburn University RFID Lab in Alabama has published a proof-of-concept whitepaper that seeks to demonstrate the efficiency savings blockchain technology can unlock across the contemporary supply chain.
The proof-of-concept was designed to ingest, encode, distribute, and store serialized data from multiple points throughout the supply chain on Hyperledger Fabric.
The pilot collected live data from brands Nike, PVH Corp., and Herman Kay, and major United States retailers Kohl’s and Macy’s.
CHIP was launched in 2018 and claims to be the first supply chain project to integrate the information pulled from RFID tags onto a blockchain network.
Blockchain as a supply chain data solution
The project saw data pertaining to 223,036 goods uploaded to a distributed ledger. Only 1% of data entries were uploaded by stores, with 87% of data coming from distribution centers, and the remaining 12% originating from a point of encoding.
As such, CHIP determined that blockchain is a functional solution to issues of serialized data exchange within the supply chain. The report concludes that the participating companies were “able to record transactions containing serialized data in a common language and share that data with their appropriate trade partners.”
The paper identifies “a tremendous amount of error and inefficiency in currency supply systems,” estimating that the elimination of counterfeiting and shrinkage in the supply chain could unlock $181 worth of business opportunities.
Traditional supply chain tracking technologies deemed “antiquated”
By contrast, the paper argues that previously existing networks for exchanging are built for “antiquated internet technologies,” and are not suitable to handle the massive volumes of serialized data that are generated throughout the contemporary supply chain.’
The team points to the absence of “an effective, industry-wide solution for exchanging serialized data between business partners,” despite the introduction of serialized data such as RFID tags and QR codes over a decade ago.
Further, the report argues that previous attempts to integrate infrastructure to collect information on masse’ across the supply chain have been “constrained by the industry-wide ineptitude for sharing serialized data.”
window.fbAsyncInit = function () { FB.init({ appId: '1922752334671725', xfbml: true, version: 'v2.9' }); FB.AppEvents.logPageView(); }; (function (d, s, id) { var js, fjs = d.getElementsByTagName(s)[0]; if (d.getElementById(id)) { return; } js = d.createElement(s); js.id = id; js.src = "http://connect.facebook.net/en_US/sdk.js"; js.defer = true; fjs.parentNode.insertBefore(js, fjs); }(document, 'script', 'facebook-jssdk')); !function (f, b, e, v, n, t, s) { if (f.fbq) return; n = f.fbq = function () { n.callMethod ? n.callMethod.apply(n, arguments) : n.queue.push(arguments) }; if (!f._fbq) f._fbq = n; n.push = n; n.loaded = !0; n.version = '2.0'; n.queue = []; t = b.createElement(e); t.defer = !0; t.src = v; s = b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t, s) }(window, document, 'script', 'https://connect.facebook.net/en_US/fbevents.js'); fbq('init', '1922752334671725'); fbq('track', 'PageView'); Source link
The post Nike Explores Blockchain for Supply Chain Data Collection appeared first on Coin First.
from Coin First https://ift.tt/3cCL0Tm
1 note
·
View note
Text
Week 6 Lectures
Never would I have thought that I would receive a lecture via a skype call... however this course continues to surprise me. Notes for this week’s lectures as per usual :) This week notes will be in their purest form: brain dribble.
Morning Lecture
WEP:
Needed a 64 bit key, but how do we get users to generate a 64 bit key. Was a lot to ask users to generate. Designers decided to generate the last 24 bits by themselves, using an IV.
Seed was different for each packet, 40 bit key that was shared and everyone used, and then the 24 bit value that was generated.
To decrypt, you get the 24 bit thing sent in the clear - (IV) and combine with the secret to get the 64 bit
Danger when someone transmits the same data under the same key - data is replicated in the same frame
Collision for IV - square root of 2^24 = 2^12 ~= 4000
Relatively small amount of packets needed to be sent before collision
Mixing data and control (key characteristic of attacks):
WEP attack - carrying out the normal function, can be abused by users to gain more control
I.e. Richard smuggling expensive express envelopes by hiding them in a satchel, posting them to himself
If there is a potential ambiguity in the channel and you are able to control how that is resolved - you gain control of the channel
Buffer Overflow:
Computer rapidly switches between jobs - “context switching” rather than concurrencyModern cpu’s use the notion of interruption Stack keeps track of what is being used
Latest process - top of the stack. When it’s finished, the information about the process gets thrown out, stack pointer gets moved down
After process have been re-awakened, need information about what the process is currently doing. This is stored in the disk, because RAM is expensive
Stack is also used to store local data about the program - much faster
Running program data is in the stack, as well as other frozen processes
Stack is stored backwards -> grows down
If can persuade the buffer you are writing to is bigger than it is - then you can be writing to other memory of the person that is asleep
Pointer to the next instruction about to be executed -> control
Contains other information
Write to the return address, overwrite the current thing
Proof of work:
Bitcoin - can’t counterfeit easily (work ratio)
No matter how good something is, every 18 months your attacker gains 1 bit of work due to Moore’s law i.e. lose one bit of security
Number of transistors per square inch on integrated circuits had doubled every year since the integrated circuit was invented.
Disk encryption:
Thread model - attacker has physical access to the disk, assume full control of the hard drive
Generate random key, encrypted version of the key stored in the disk
Evening Lecture:
Web Seminar
HTTP:
Application layer protocol used to send messages between browsers and web servers. HTTP requests go from the browser to the server.
Databases / SQL - browser sending an HTTP get request from the server with the username and password as data
Server queries database with “SELECT password FROM users WHERE match”
HTTP Cookies/Sessions
An HTTP cookie is a small piece of data sent in a response from a web server and stored on the user’s computer by the browser
A session cookies is a unique ID generated by the server and sent to the user when they first connect or login
Browser sends it with all HTTP requests
XSS - cross-site scripting is an attack in which an attacker injects data, such as a malicious program
Reflected XSS - occurs when user input is immediately returned by a web application.
Stored - you enter data which is stored within the application and then returned later
xss.game.appstop.com
SQL injection is a code injection technique in which malicious SQL statements are inserted into an entry field for execution.
Goal behind an SQL query is to gain access
‘ or 1 == 1 --
Blind SQL injections are identical to normal SQL Injection except that when an attacker attempts to exploit an application rather than getting a useful error message
Cross Site Request Forgery:
Attack on an authenticated user i,.e. Already logged in
When you log in to a website it sends you a cookie to your browser to keep you logged in.
Bank attack:
If attacker knows the format of the bank request, they can hide a transfer request inside an img using html
Cross Site Request Forgery Defences:
Primary mitigation is with tokens
Generate a suitably random token, store value server-side
Sent token to user, expect this token back as part of any user requests
In a GET request, this token will be appended to the URL
If a website has XSS vulnerabilities, CSRF mitigations are pointless
Crypto Seminar
Payment Process: Current versus Bitcoin:
Current payment systems require third-party intermediaries that often charge high processing fees
Machine-to-machine payment using the Bitcoin protocol allows for direct payment between individuals, as well as support micropayments -> reduce transaction costs
Crypto:
Built using cryptographic principles i.e. blockchain and hashing
Difficult to fake transactions - too many bits so it isn’t worth
Blockchain:
Method of storing data
A chain of chronologically linked blocks where each block is linked to the previous block
Blocks are unique - no two blocks will have the same hash
Data:
Consists of hundreds of transactions
Put around 2000 transactions in one block
Hashes:
Block’s hash summarises the data into a combination of letters and numbers
SHA-256 hashing algorithm
If a transaction in the block is changed, the hash is changed
This is important because each block has the hash of the previous block -> need to check against all previous blocks
Tamper evident
When a transaction is mine, it isn’t immediately added but placed in a transaction pool
The miner gathers enough to form a block - called a candidate block
Hash the block header along with a nonce
When we hash we hope the block hash value is below a certain target value
The nonce is a random number brute forced by miners to try and create the correct hash
When nonce is found, it is broadcast and the block is added to the existing chain
Proof of Stake:
Growth of mining pools could eventually lead back to a centralised system
PoW mining uses excessive amounts of electricity
PoS algorithm attributes mining power to proportion of total bitcoins held, rather than computing power
Rewards are transaction fees rather than new cryptocurrency
Types of crypto currencies:
Bitcoin
Uses the SHA-256 algorithm - very processor intensive and complex requires lots of dedicated hardware
Litecoin
More accessible for normal uses to mine on their CPUs as the algorithm used is less CPU intensive, but more memory intensive
Facebook Libra
Centralised architecture - libra will be managed by the Libra Association, having more control over the blockchain
There is no ‘mining” - to set up a node on Libra, need $10000
Privacy:
Blockchain doesn’t have a strong concept of identity (public, private) key pairing
Doesn’t exempt transaction from tracing
Two main ways:
Relations between address - inferring identity
Interactions between nodes and users
Monero:
Unlinkability -> stealth addresses with view keys
Transaction mixing -> ring signatures
Concealing transaction amounts -> RingCt signatures
Historical flaws:
51% attack:
Double-spend
Purpose might also be to discredit a crypto instead of money
Credibility decided on the majority
Off-springs created one’s solution for a hash is not added into their own spin-off
Motive might be to discredit the cryptocurrency
Past Attacks:
Usually happened on small networks
Verge 51% attack, on April 2018
Groups of hackers found two main flaws in the system:
Bug which lowered the hashing difficulty for a hashing algo (Scrypt)
Verge allowed 5 different hashing algorithms, and only the difficulty for Scrypt is lowered
Hacked 3 times over 2 weeks
Cryptocurrency exchanges:
Mt.Gox - bitcoin exchange that was launched in 2010. Handled over 70% of all Bitcoin transactions in 2013
Previous owner retained admin level user account when MtGox was sold in 2011
Attacker logged in to the account
Assigned himself a large number of BTC which he sold on the exchange
Price dropped immediately
Obtained private keys of MtGox clients
Created selling orders on these accounts and bought the BTC he stole
SQL Injection vulnerability was found
MtGox user database began circulating online and included:
Plain text email addresses
Usernames
MD5 Hashed passwords, with some unsalted
Future of Cryptocurrency:
Adoption
Overcoming resistance from:
People
Established finance institutes (eg banks)
Governments (they don’t like that you don’t pay tax by concurrency)
Ease of use
Volatility
Threats
Blockchain its laek
Quantum computers
To the sft that utilises cryptocurrency
Cryptocurrency wallet/exchange/
Strong private keys
Symmetric Ciphers
Two sorts of ciphers, symmetric and asymmetric -> regards the keys
If you know the key
For a symmetric: you can decrypt and encrypt
For an asymmetric: you have separate private and public keys to decrypt and encrypt (RSA)
Earthquakes:
How would I cope, how would my business cope? -> ‘gobag’
Home Study - read up about the “block modes” - only need to learn/understand ECB, CBC, CTR
Authentication:
Identifying for who? Computer/human?
Facebook. Police, baggage screening
Authentication and identification - what is the difference?
What decisions?
Computerised authentication system -> needs to make a decision about whether it is you or not
Factors:
Something that you have
Something that you know - i.e a password. Easy way of doing authentication
How do you know that you share the same secret?
Something that you are - Unfakeable
Two factor authentication:
Something that you have AND something that you know i.e. and password
All of these things seem different, but ultimately they are all just things that you know, and are all secrets
Something that you are can be replicated
Serious problem -> authenticating bombs, missiles etc
Biometrics - not real authentication, collecting another shared secret from a person, and can be bypassed
1 note
·
View note
Text
[ad_1] Memecoins’ security and dependability as a medium of exchange remain a topic of debate in the cryptosphere. Memecoins are said to be safe and decentralized, but some are concerned about their volatility and lack of oversight. Memecoin proponents claim that because they are decentralized, users have more control over their money and are protected from fraud and theft. Furthermore, memecoins leverage blockchain technology, ensuring transactions are securely recorded on a decentralized ledger, making them resistant to hacking and manipulation. Governments and central banks that implement measures to maintain stability, control inflation, and guarantee the integrity of the financial system support traditional currencies. Memecoins, on the other hand, are more vulnerable to increased volatility and market manipulation due to their decentralized structure and lack of governmental oversight. Memecoins’ volatility is a source of worry because of their propensity for sudden, erratic price changes. As a result, using memecoins as a trustworthy medium of exchange or store of value may present difficulties. Nonetheless, these risks can be reduced with appropriate regulations in place, improving the security and dependability of memecoins as a medium of exchange. Finding the ideal mix between regulation and innovation is essential, though. Overregulation may hinder creativity and obstruct the growth of the memecoin ecosystem. Memecoins must be made safe and reliable over the long term to be a practical form of currency; hence, it is crucial to find a legal structure that addresses concerns while fostering innovation. Related: PEPE vs. DOGE: How the memecoins performed their first time hitting a $1B market cap window.fbAsyncInit = function () FB.init( appId: '1922752334671725', xfbml: true, version: 'v2.9' ); FB.AppEvents.logPageView(); ; (function (d, s, id) var js, fjs = d.getElementsByTagName(s)[0]; if (d.getElementById(id)) return; js = d.createElement(s); js.id = id; js.src = " js.defer = true; fjs.parentNode.insertBefore(js, fjs); (document, 'script', 'facebook-jssdk')); !function (f, b, e, v, n, t, s) if (f.fbq) return; n = f.fbq = function () n.callMethod ? n.callMethod.apply(n, arguments) : n.queue.push(arguments) ; if (!f._fbq) f._fbq = n; n.push = n; n.loaded = !0; n.version = '2.0'; n.queue = []; t = b.createElement(e); t.defer = !0; t.src = v; s = b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t, s) (window, document, 'script', ' fbq('init', '1922752334671725'); fbq('track', 'PageView'); [ad_2] Source
0 notes
Text
[ad_1] MUMBAI: The rupee gained 24 paise to 81.73 against the US dollar in early trade on Monday amid a positive trend in domestic equities. Forex traders said foreign fund inflows also supported the local unit. At the interbank foreign exchange, the domestic unit opened at 81.85 against the dollar, then climbed to 81.73, registering a rise of 24 paise over its previous close. On Friday, the rupee closed at 81.97 against the US currency. The forex traders said the rupee is likely to trade with a positive bias on improved global risk sentiments and fresh foreign fund inflows. Moreover, weak crude oil prices and muted dollar index may also support the domestic currency, they added. Meanwhile, the dollar index, which gauges the greenback's strength against a basket of six currencies, fell 0.09 per cent to 104.43. In the domestic equity market, the 30-share BSE Sensex rose 564.81 points or 0.94 per cent to 60,373.78 points. The broader NSE Nifty advanced 166.95 points or 0.95 per cent to 17,761.30 points. Foreign Institutional Investors (FIIs) were net buyers in the capital market on Friday as they purchased shares worth Rs 246.24 crore, according to exchange data.!(function(f, b, e, v, n, t, s) window.TimesApps = window.TimesApps )( window, document, 'script', 'https://connect.facebook.net/en_US/fbevents.js', );if(typeof window !== 'undefined') ; const TimesApps = window; TimesApps.loadScriptsOnceAdsReady = () => var scripts = [ 'https://www.googletagmanager.com/gtag/js?id=AW-877820074', 'https://www.googletagmanager.com/gtag/js?id=AW-658129294', 'https://timesofindia.indiatimes.com/grxpushnotification_js/minify-1,version-2.cms', 'https://connect.facebook.net/en_US/sdk.js#version=v10.0&xfbml=true', 'https://timesofindia.indiatimes.com/locateservice_js/minify-1,version-14.cms' ]; scripts.forEach(function(url) url.indexOf('locateservice_js') !== -1 )) script.src = url; else if (url.indexOf('colombia_v2')== -1 && url.indexOf('sdkloader')== -1 && url.indexOf('tvid.in/sdk')== -1 && url.indexOf('connect.facebook.net') == -1) script.src = url; script.async = true; script.defer = true; document.body.appendChild(script); ); [ad_2] Source link
0 notes
Text
Brian Armstrong, the CEO and founder of Coinbase, a pioneering public cryptocurrency exchange in the U.S., recently shared his insights on the future trajectory of cryptocurrency. Having weathered the storms of U.S. regulation and watched the digital currency space evolve, Armstrong’s take on what lies ahead is both fascinating and important for us crypto enthusiasts.From the Internet to CryptoDrawing a parallel between the internet’s early days and the current state of crypto, Armstrong reflected on how initial skepticism and distrust of new technology often transition to widespread acceptance. The internet, once perceived as an odd, alternative platform, has now overshadowed traditional media forms.Similarly, while early crypto enthusiasts might have been seen as outsiders or high-risk takers, the field is gradually pulling in a more diverse crowd. It’s not just about the technology anymore but its broader applications, from the art world to remittances and beyond.Battles With The RegulatorsArmstrong is no stranger to regulatory skirmishes, especially with the Securities and Exchange Commission (SEC). But with recent legal victories suggesting a favorable tide for crypto, there’s optimism in the air. During the All In Summit, Armstrong touched on the vital theme of crypto regulation and his vision for the industry’s path forward.Though advocating for innovation, Armstrong doesn’t shy away from the necessity of regulatory frameworks. Drawing from traditional financial industry practices, he believes in establishing essential rules that promote both safety and innovation. While centralized crypto entities should follow strict guidelines, decentralized platforms, he feels, should be treated more similarly to software entities.While Armstrong extensively discussed the current state of cryptocurrency, he dropped hints about what the future holds. Predicting the next wave of crypto popularity and adoption, Armstrong suggests that a range of emerging use cases are paving the way for another significant bull run in the market during the first half of 2024. His reason is as the crypto industry matures and evolves, these triggers will catalyze a surge, bringing in a new wave of investors, innovations, and opportunities.[embed]https://www.youtube.com/watch?v=Bqeq8cm-JP0[/embed]!function(f,b,e,v,n,t,s) if(f.fbq)return;n=f.fbq=function()n.callMethod? n.callMethod.apply(n,arguments):n.queue.push(arguments); if(!f._fbq)f._fbq=n;n.push=n;n.loaded=!0;n.version='2.0'; n.queue=[];t=b.createElement(e);t.async=!0; t.src=v;s=b.getElementsByTagName(e)[0]; s.parentNode.insertBefore(t,s)(window,document,'script', ' fbq('init', '887971145773722'); fbq('track', 'PageView');
0 notes
Text
Create Your Own Crypto Exchange Using Advanced Trading Features
What is Cryptocurrency Exchange script?
Cryptocurrency Exchanges Script is a ready-made exchange software. With this script, a startup can successfully construct a cryptocurrency exchange similar to any of the world's leading trading platforms. This cryptocurrency exchange script includes all of the advanced features and security elements required to establish an exchange platform.
You may create a feature-rich cryptocurrency trading website with this script within 7 to 10 days, which is a significant advantage. All trustworthy cryptos, such as bitcoin, Cardano, Ripple, Ethereum, bitcoin cash, BNB, Matic, Shiba Inu, Dogecoin, and others, are supported by the premium crypto exchange script for trading.

White Label Cryptocurrency Exchange script:
A white label cryptocurrency exchange script is a pre-built software solution that can be used to create a cryptocurrency exchange platform. "White label" means that the software is not branded and can be customized to meet the specific needs of a particular business or organization.
Using a white label cryptocurrency exchange script can save time and money compared to building an exchange platform from scratch. The script typically includes all of the necessary features for a basic exchange, such as order books, trading charts, and wallet management. It may also include additional features, such as compliance tools, KYC/AML procedures, and customer support.
Features of Cryptocurrency Exchange Script:
User Authentication: Secure user authentication is a critical feature of any cryptocurrency exchange script. This includes user account creation, two-factor authentication, and password management.
Wallet Management: A cryptocurrency exchange script should allow users to manage their digital currency wallets, view their balance, and deposit or withdraw cryptocurrency.
Trading Engine: The trading engine is the heart of the cryptocurrency exchange, allowing users to buy and sell digital currencies. The trading engine should have advanced features such as order books, trading charts, and order matching algorithms.
Payment Gateway Integration: Payment gateway integration allows users to deposit funds into their account and make withdrawals. Popular payment gateways used in cryptocurrency exchanges include credit cards, bank transfers, and e-wallets.
KYC/AML Procedures: Many countries require Know Your Customer (KYC) and Anti-Money Laundering (AML) procedures to prevent fraud and illegal activities. A cryptocurrency exchange script should have built-in procedures to meet these requirements.
Multilingual Support: Cryptocurrency exchange scripts should support multiple languages to attract global users.
Customer Support: A cryptocurrency exchange script should have an efficient customer support system, including a help desk, live chat, and a support ticket system.
Security Features: Cryptocurrency exchange scripts should be built with robust security features to protect against cyber attacks, data breaches, and other security threats.
Customizability: A good cryptocurrency exchange script should allow businesses to customize the platform to suit their needs, including branding, color schemes, and features.
These are some of the key features that can be expected from a typical cryptocurrency exchange script. However, it's important to note that different software solutions may offer additional or different features, depending on the specific needs of the business or organization using it.
why hivelance for develop your cryptocurrency exchange script?
Hivelance is the top provider of cryptocurrency exchange software services, giving you the advantage over the competition. We had extensive experience in developing blockchain solutions, including cryptocurrency exchange script development. Our team includes experienced developers, designers, and project managers who can help guide you through the development process and ensure that your exchange is successful. We offer a range of benefits for businesses looking to develop their own cryptocurrency exchange script. From expertise and customization to security and support.
1 note
·
View note