Don't wanna be here? Send us removal request.
Text
static libraries in c
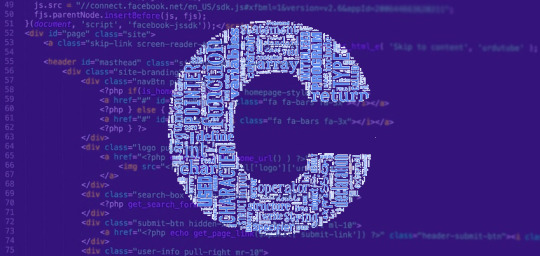
libraries are that part that allows us to have modularized code, and understanding how they work and how we can relate to them can make all the difference.
Why use libraries
reduce development time and increase code quality.
How they work
a library provides common functions that have already been provided by other programmers and this avoids code duplication.
How to create them
To create a library you have to take into account that there are two types of libraries, dynamic and static.
In this example I want to show you how to create a static library.
1. Go to the directory where all your functions are located and your header file "file.h" where you have the prototypes of the functions.
2. now you are going to compile the programs and create a .o file output.
for this we use the following command: gcc -Wall -pedantic -Werror -Wextra -c *.c
has now generated some .o files.
3. The basic tool used to create static libraries is a program called 'ar'.
This program can be used to create static libraries.
To create a static library, we can use a command like this:
ar -rc libholbertonschool.a *.o
this command creates a static library named "libholbertonschool.a" and places copies of all .o files in it.
to verify that the files have been copied successfully we can use the following command:
ar -t libholbertonschool.a
4. After creating or modifying a file, it is necessary to index it. This index is subsequently used by the compiler to speed up the search for symbols within the library, and to make sure that the order of symbols in the library does not matter during compilation.
The command used to create or update the index is called 'ranlib' and is invoked as follows:
ranlib libholbertonschool.a
How to use them
to use the library you just created, all you have to do is include the header file in your program call and call the function to use, but at compile time you will have to submit the library.
by: Alejandro alomia
0 notes
Text
Compilation in C
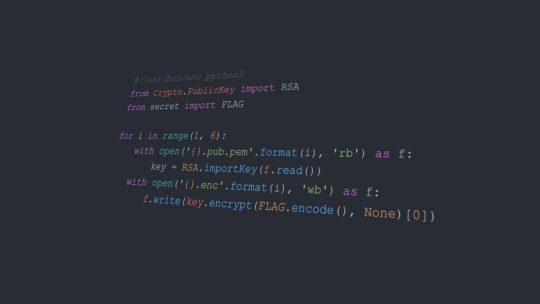
C is a well-known programming language used by many.
But this time I want to talk about how the compilation of a C program works using the gcc compiler.
What is compilation?
Compiling is the process in which we convert a file that we as people understand into a language called machine language, which is the language that computers understand.
Fases de la compilación
1.0 Source code: To compile our program we have to have the source code, the source code is our plain text file, where the instructions to be performed by the computer are written.
In this example I wrote a program that displays a message on the screen.
As it is a program written in C, in this example we will be using the gcc compiler.
To start compiling our program what we must do is the following.
Locate our file or source code
After that we will have to use the following command.
gcc is the compiler.
main.c is the source code.
-o is the output option.
main is the name by which the executable program will be expected after compilation is complete.
the gcc compiler has many other options that control the output type, here are some other options.
-c: Compile or assemble the source files, but do not link. The linking stage simply is not done. The ultimate output is in the form of an object file for each source file.
-S: Stop after the stage of compilation proper; do not assemble. The output is in the form of an assembler code file for each non-assembler input file specified.
-E: Stop after the preprocessing stage; do not run the compiler proper. The output is in the form of preprocessed source code, which is sent to the standard output.
-o file: Place the primary output in file file. This applies to whatever sort of output is being produced, whether it be an executable file, an object file, an assembler file or preprocessed C code.
The steps that follow are steps that are not visible to us but we must understand how they work and what they do.
1.1 Lexical analysis: This is the first phase of the compiler, and it consists of receiving as input the source code of another program to produce an output composed of tokens or symbols, this serves later for another process called syntactic analysis.
1.2 Parsing: At this stage the input text is converted into other structures (commonly trees) that are more useful for further analysis and capture the implicit hierarchy of the input.
1.3 Semantic analysis: In this stage, the source program is reviewed to try to find semantic errors and gather information about the types for the subsequent code generation phase.
1.4 Intermediate code generation: After syntactic and semantic analysis, some compilers provide an explicit intermediate representation of the source program.
1.5 Optimization: This is the part where a code fragment is transformed into another equivalent fragment that executes in a more efficient way, using less computational resources.
1.6 Object code generator: This is the final phase of the compiler, usually consisting of relocatable machine code or assembly code. The memory location for each of the variables to be used in the program is also selected.

1.7 Object code: The file that results after making a compilation is called object code, and this is the file that is used to execute the program.
2 notes
·
View notes