#react usememo
Explore tagged Tumblr posts
Text
REACT: Tips and Tricks
[et_pb_section fb_built=”1″ _builder_version=”4.27.4″ _module_preset=”default” global_colors_info=”{}”][et_pb_row _builder_version=”4.27.4″ _module_preset=”default” global_colors_info=”{}”][et_pb_column type=”4_4″ _builder_version=”4.27.4″ _module_preset=”default” global_colors_info=”{}”][et_pb_text _builder_version=”4.27.4″ _module_preset=”default” global_colors_info=”{}”] Introduction React has…
#content management systems#cyber-security#cybersecurity#e-commerce#Featured Snippets#JAMstack#React best practices#React coding techniques#React debugging#React development#React hooks#React lazy loading#React memoization#React optimization#React performance#React security#React state management#React testing#React tips#React useMemo#React useRef#web development
0 notes
Text
Memoization related bugs in React always feel unfair to me on some level, like the code is mocking me. :P "I am going to do exactly what you told me to do, but just because, I am also going to do it 639472926294 times and crash your browser with an infinite loop. Hope this helps!"
(For new React devs following me, do yourself a favor and get familiar with `useMemo` and `useCallback` now. You won't need them often but the times when you do are often a really frustrating and baffling bug. :P )
3 notes
·
View notes
Text
Flexpeak - Front e Back - Opções
Módulo 1 - Revisão de JavaScript e Fundamentos do Backend: • Revisão de JavaScript: Fundamentos • Variáveis e Tipos de Dados (let, const, var) • Estruturas de Controle (if, switch, for, while) • Funções (function, arrow functions, callbacks) • Manipulação de Arrays e Objetos (map, filter, reduce) • Introdução a Promises e Async/Await • Revisão de JavaScript: Programação Assíncrona e Módulos • Promises e Async/Await na prática Módulo 2 – Controle de Versão com Git / GitHub • O que é controle de versão e por que usá-lo? • Diferença entre Git (local) e GitHub (remoto) • Instalação e configuração inicial (git config) • Repositório e inicialização (git init) • Staging e commits (git add, git commit) • Histórico de commits (git log) • Atualização do repositório (git pull, git push) • Clonagem de repositório (git clone) • Criando um repositório no GitHub e conectando ao repositório local • Adicionando e confirmando mudanças (git commit -m "mensagem") • Enviando código para o repositório remoto (git push origin main) • O que são commits semânticos e por que usá-los? • Estrutura de um commit semântico: • Tipos comuns de commits semânticos(feat, fix, docs, style, refactor, test, chore) • Criando e alternando entre branches (git branch, git checkout -b) • Trabalhando com múltiplos branches • Fazendo merges entre branches (git merge) • Resolução de conflitos • Criando um Pull Request no GitHub Módulo 3 – Desenvolvimento Backend com NodeJS • O que é o Node.js e por que usá-lo? • Módulos do Node.js (require, import/export) • Uso do npm e package.json • Ambiente e Configuração com dotenv • Criando um servidor com Express.js • Uso de Middleware e Rotas • Testando endpoints com Insomnia • O que é um ORM e por que usar Sequelize? • Configuração do Sequelize (sequelize-cli) • Criando conexões com MySQL • Criando Models, Migrations e Seeds • Operações CRUD (findAll, findByPk, create, update, destroy) • Validações no Sequelize • Estruturando Controllers e Services • Introdução à autenticação com JWT • Implementação de Login e Registro • Middleware de autenticação • Proteção de rotas • Upload de arquivos com multer • Validação de arquivos enviados • Tratamento de erros com express-async-errors Módulo 4 - Desenvolvimento Frontend com React.js • O que é React.js e como funciona? • Criando um projeto com Vite ou Create React App • Estruturação do Projeto: Organização de pastas e arquivos, convenções e padrões • Criando Componentes: Componentes reutilizáveis, estruturação de layouts e boas práticas • JSX e Componentes Funcionais • Props e Estado (useState) • Comunicação pai → filho e filho → pai • Uso de useEffect para chamadas de API • Manipulação de formulários com useState • Context API para Gerenciamento de Estado • Configuração do react-router-dom • Rotas Dinâmicas e Parâmetros • Consumo de API com fetch e axios • Exibindo dados da API Node.js no frontend • Autenticação no frontend com JWT • Armazenamento de tokens (localStorage, sessionStorage) • Hooks avançados: useContext, useReducer, useMemo • Implementação de logout e proteção de rotas
Módulo 5 - Implantação na AWS • O que é AWS e como ela pode ser usada? • Criando uma instância EC2 e configurando ambiente • Instalando Node.js, MySQL na AWS • Configuração de ambiente e variáveis no servidor • Deploy da API Node.js na AWS • Deploy do Frontend React na AWS • Configuração de permissões e CORS • Conectando o frontend ao backend na AWS • Otimização e dicas de performance
Matricular-se
0 notes
Text
React Performance Optimization Tips for Faster Apps
If you're looking to build high-performance React applications and want to enhance your development skills, consider enrolling in the best React training institute in Hyderabad . Efficient app performance is crucial to delivering seamless user experiences, and React offers numerous techniques to achieve that.
1. Use React.memo for Component Optimization
React.memo is a higher-order component that helps prevent unnecessary re-renders by memoizing the result. It is especially useful for functional components that rely on props.
2. Lazy Loading and Code Splitting
Implement lazy loading using React.lazy() and Suspense to load components only when needed. Code splitting reduces the initial load time, improving overall application speed.
3. Optimize State Management
Efficient state management using tools like Redux, Context API, or Zustand can significantly reduce unnecessary re-renders. Keeping the state minimal and organized enhances app responsiveness.
4. Virtualize Large Lists
For rendering extensive lists or tables, use libraries like react-window or react-virtualized to render only visible items, reducing memory consumption and improving speed.
5. Avoid Inline Functions and Object Creations
Inline functions and objects can cause frequent re-renders. Instead, define functions and objects outside the component or use useCallback and useMemo for memoization.
6. Optimize Images and Assets
Compress and use modern image formats like WebP. Leverage lazy loading for images and ensure correct asset management to reduce load times.
Conclusion
Mastering these performance optimization tips can significantly enhance your React applications. To gain practical, hands-on experience, consider training with Monopoly IT Solutions, the leading institute for React training in Hyderabad. Take the first step toward building faster and more efficient applications today.
0 notes
Text
Master React Hooks: Best Practices for useEffect, useCallback, useMemo
1. Introduction React Hooks revolutionized front-end development by allowing functional components to manage state and side effects without the need for class components. Among the various hooks, useEffect, useCallback, and useMemo are particularly powerful for handling side effects, memoizing functions, and optimizing rendering performance. This tutorial will guide you through mastering these…
0 notes
Text
What is React.js? A Complete Guide for 2025

What is React.js?
What is React js? A Complete Guide for 2025 dives deep into React.js, the groundbreaking JavaScript library that has transformed the way developers build dynamic and interactive user interfaces. As one of the most powerful and widely adopted tools in modern web development, React, originally created by Facebook (now Meta), continues to dominate the industry with its efficient, component-based architecture and unparalleled flexibility. Whether you're a beginner or an experienced developer, this guide will equip you with everything you need to master React.js in 2025 and beyond.
Reasons for Popularity:
Fast and efficient: Utilizes Virtual DOM for performance and optimization
Component-based: Allows for code reusability and organization
Huge ecosystem: There are many libraries and tools out there that enhance the development experience
It is backed by Meta: All the time updates and new features are added
History and Evolution of React.js
React.js was originally launched in 2013 by Facebook. Over the years, it has passed through several major versions:
2015: React 0.14 was introduced with functional components.
2016: React 15 was designed to improve performance, and so was the implementation of the Fiber architecture.
2017: React 16 brought in Hooks and Error Boundaries.
2020: React 17 emphasized easier upgrades and gradual adoption.
2022+: React 18 introduced concurrent rendering with automatic batching.
Why to Learn React.js by 2025?
Performance and Speed
With fast UI updates made possible by the React Virtual DOM and concurrent rendering, React will be an indispensable framework for modern applications.
Large Community Support
React has a vast user base consisting of millions of developers, and thus, finding resources, tutorials, and support is easier than before.
Flexibility and Scalability
Applications of any size can be developed using React, whether they are small projects or enterprise-level applications.
Core Features of React.js
Virtual DOM
In React, unlike in the normal DOM, only those parts of the UI that were actually changed would be updated, thereby giving faster performance.
Component-Based Architecture
In React, an application is made up of building blocks called components, which are reusable, thereby minimizing redundancy.
JSX (JavaScript XML)
JSX enables developers to write markup directly inside the code.
One-Way Data Binding
This aids in keeping tighter control over the data flow, which is beneficial for easier debugging.
Setting Up React.js in 2025
Install Node.js and npm.
Create a React app using:
npx create-react-app my-app
cd my-app
npm start
Use Vite for faster builds:
npm create vite@latest my-app --template react
Building a Basic React.js Application
Creating Components
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
Using State with Hooks
import { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<button onClick={() => setCount(count + 1)}>
Count: {count}
</button>
);
}
React.js Best Practices for 2025
Functional components are preferred over class components.
Keep components small and reusable.
Render optimization through memoization (React.memo, useMemo).
Follow proper folder structures.
Error boundaries should be implemented.
Learning React.js at the TCCI Computer Coaching Institute
TCCI offers a comprehensive course on the training of React.js:
React from the beginning to advanced
Projects work from real life
Personal mentorship
Getting certified after completion
Conclusion
React.js will dominate frontend development in 2025. No matter whether you're a fresher or have many years of experience in programming, learning React opens doors to innumerable opportunities. Join TCCI Computer Coaching Institute to master React.js practicing real-time!
Location: Ahmedabad, Gujarat
Call now on +91 9825618292
Get information from https://tccicomputercoaching.wordpress.com/
FAQ
What is React.js mainly used for?
React.js is used for building fast, interactive user interfaces for web applications.
How long does it take to learn React.js?
Depending on prior experience, it can take 2-3 months to become proficient.
Is React.js still relevant in 2025?
Yes, React remains one of the most in-demand frontend technologies.
What are the prerequisites for learning React.js?
Basic knowledge of HTML, CSS, and JavaScript is recommended.
Can I use React.js for mobile development?
Yes! React Native allows you to build mobile apps using React.js.
#ReactJS#WebDevelopment#LearnReact#ReactTutorial#FrontendDevelopment#JavaScript#Coding#TechGuide#Programming#ReactJS2025
0 notes
Text
The Ultimate Guide to a React JS Course
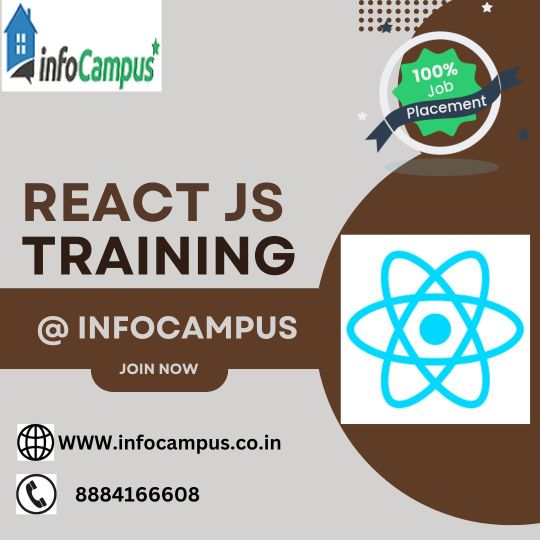
With the growing demand for dynamic web applications, React.js has become one of the most sought-after JavaScript libraries for building user interfaces. Whether you are a beginner or an experienced developer looking to expand your skill set, enrolling in a React.js course can be a game-changer. This guide will provide a comprehensive overview of what a React.js course entails and why learning React can elevate your career.
What is React.js?
React.js is an open-source JavaScript library developed by Facebook for building interactive and high-performance user interfaces, especially for single-page applications (SPAs). It allows developers to create reusable UI components, manage application state efficiently, and ensure a seamless user experience.
Why Learn React.js?
React.js is widely used in the industry due to its numerous advantages:
Component-Based Architecture – React follows a modular approach, making code more reusable and maintainable.
Virtual DOM – React updates only the necessary parts of the UI, resulting in improved performance and faster rendering.
Strong Community Support – React has extensive documentation, third-party libraries, and active developer support.
Demand in the Job Market – React is a popular skill among employers, opening doors to high-paying job opportunities.
Flexibility – React can be used for web applications, mobile applications (React Native), and even desktop applications.
Key Topics Covered in a React.js Course
A React.js course typically covers the following essential concepts:
Fundamentals of React.js
Introduction to React.js and its ecosystem
Setting up a development environment
Understanding JSX (JavaScript XML)
Components, Props, and State Management
Handling Events and Lifecycle Methods
Event Handling in React
Understanding Functional and Class Components
React Component Lifecycle Methods
State Management in React
Using React Hooks (useState, useEffect, useContext)
State lifting and prop drilling
Introduction to Redux for global state management
Context API for managing state without Redux
React Routing and Navigation
React Router for navigation
Dynamic routing and nested routes
Protected routes and authentication
Working with APIs and Asynchronous Operations
Fetching data with Fetch API and Axios
Handling Promises and Async/Await
Managing API responses and error handling
Advanced React Concepts
Performance optimization with React.memo and useMemo
Lazy loading and code splitting
Higher-Order Components (HOC) and Render Props
Testing in React
Introduction to Jest and React Testing Library
Writing unit and integration tests
Debugging and handling errors
Deployment and Best Practices
Building and optimizing production-ready React applications
Hosting React applications using Netlify, Vercel, and Firebase
Continuous Integration/Continuous Deployment (CI/CD) workflows
Who Should Enroll in a React.js Course?
A React.js course is suitable for:
Beginners in Web Development – Those looking to start their journey in frontend development.
Frontend Developers – Developers familiar with JavaScript who want to specialize in React.
Backend Developers – Those who wish to learn frontend technologies to become Full Stack Developers.
Entrepreneurs & Freelancers – Individuals looking to build dynamic web applications efficiently.
Students & Career Changers – Those seeking a career in web development with in-demand skills.
How to Choose the Right React.js Course?
Take into account the following elements when choosing a React.js course:
Course Curriculum – Ensure it covers both fundamentals and advanced topics.
Hands-on Projects – Look for a course that includes real-world projects and practical applications.
Instructor Expertise – Check if the instructor has industry experience and strong teaching skills.
Certification & Job Support – Some courses offer certification and career assistance, which can be beneficial.
Flexibility – Online, part-time, or full-time options should fit your schedule.
Career Opportunities After Learning React.js
After completing a React.js course, you can explore various job roles, such as:
React Developer – Specializing in building React applications.
Frontend Developer – Working with UI/UX and JavaScript frameworks.
Full Stack Developer - This role combines backend technologies like Node.js with React.J.S.
Software Engineer – Developing scalable web applications.
Freelance Developer – Building applications for clients or personal projects.
Conclusion
A React.js course is an excellent investment for anyone looking to master modern web development. By learning React, you gain the ability to build fast, scalable, and maintainable applications that are widely used across industries. Whether you are a beginner or an experienced developer, React skills can help you land high-paying jobs and grow your career.
Transform your career with React Training in Marathahalli, offered by Infocampus. This comprehensive course is designed to equip you with the essential skills needed to excel in the fast-paced world of web development. Master the powerful React framework and learn how to build dynamic, high-performance applications that stand out. For more details Call: 8884166608 or 9740557058
Visit: https://infocampus.co.in/reactjs-training-in-marathahalli-bangalore.html
0 notes
Text
Most Common React JS Web Development Mistakes & How to Fix Them
React JS has become a popular choice for web development due to its flexibility, performance, and component-based architecture. However, many developers, especially beginners, often make mistakes that can impact the efficiency and maintainability of their applications. In this blog, we will explore some of the most common React JS web development mistakes and how to fix them.
1. Not Using Functional Components and Hooks
The Mistake:
Many developers still rely on class components when functional components combined with Hooks offer a more efficient and readable approach.
How to Fix:
Use functional components and Hooks like useState and useEffect instead of class-based components. For example:// Instead of this class Example extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return <button onClick={() => this.setState({ count: this.state.count + 1 })}>Click {this.state.count}</button>; } }// Use this import { useState } from 'react'; function Example() { const [count, setCount] = useState(0); return <button onClick={() => setCount(count + 1)}>Click {count}</button>; }
2. Ignoring Key Props in Lists
The Mistake:
When rendering lists, developers often forget to include the key prop, which can lead to inefficient rendering and UI bugs.
How to Fix:
Ensure that each list item has a unique key:{items.map(item => ( <div key={item.id}>{item.name}</div> ))}
3. Overusing useEffect for Side Effects
The Mistake:
Many developers misuse useEffect, leading to unnecessary re-renders and performance issues.
How to Fix:
Only use useEffect when necessary, and optimize dependencies to prevent infinite loops:useEffect(() => { fetchData(); }, []); // Runs only once
4. Not Optimizing Performance with useMemo and useCallback
The Mistake:
Failing to memoize functions and values results in unnecessary re-renders.
How to Fix:
Use useMemo and useCallback to optimize expensive calculations and function references:const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]); const memoizedCallback = useCallback(() => doSomething(a), [a]);
5. Modifying State Directly
The Mistake:
Updating state directly instead of using setState or useState can lead to unpredictable behavior.
How to Fix:
Always use state setters:const [data, setData] = useState([]); setData([...data, newItem]);
6. Not Using PropTypes or TypeScript
The Mistake:
Not validating props can lead to runtime errors and unexpected behavior.
How to Fix:
Use PropTypes or TypeScript for type checking:import PropTypes from 'prop-types'; function Component({ name }) { return <h1>{name}</h1>; } Component.propTypes = { name: PropTypes.string.isRequired, };
Conclusion
By avoiding these common mistakes in React JS for web development, you can build more efficient, maintainable, and scalable applications. Whether you’re a beginner or an experienced developer, following best practices will help you create a better user experience while optimizing your React applications.
1 note
·
View note
Text
#coding#devlog#html#programming#python#software engineering#unity#indiedev#artificial intelligence#marketing
0 notes
Text
Enhancing Web Performance with Advanced React: Skills Every Developer Needs
React has revolutionized front-end development, enabling developers to build dynamic, responsive interfaces. However, as applications grow, so do their performance demands. Advanced React training provides developers with the skills and tools to optimize their applications, ensuring efficiency and responsiveness, even under heavy loads. Knowing which essential performance-enhancing techniques in React that every developer should master can help them deliver a seamless user experience.
Optimizing Component Rendering with React.memo
To boost performance, controlling component re-rendering is crucial. By default, React re-renders a component whenever its parent component updates. React’s React.memo function helps manage this by memoizing a component’s output, causing it to re-render only when props change. This technique is especially useful in applications with nested components or frequent updates, as it cuts down on unnecessary renders, easing the browser’s workload. Mastering React.memo helps developers handle large datasets and complex UI interactions more smoothly.
Leveraging Lazy Loading for Efficient Resource Management
Applications with numerous components and resources often load slowly, affecting user experience. Lazy loading with React.lazy defers the loading of components until needed, improving initial load time by prioritizing essential interface parts. Used with React’s Suspense, lazy loading enhances responsiveness in data-heavy applications by managing resources effectively. Learning to implement lazy loading allows developers to create faster-loading applications that only fetch what’s necessary in real-time, improving performance.
Minimizing State-Related Re-Renders with useCallback and useMemo
State changes in React can lead to re-renders that may slow down applications, particularly when multiple interactive components are present. The useCallback and useMemo hooks control how functions and computed values are reused across renders, optimizing performance. useCallback memoizes functions to avoid re-creation, and useMemo memoizes computed values to prevent redundant calculations. These hooks are vital for managing resource-intensive functions, helping keep applications efficient and responsive.
Reducing Load with Code Splitting
Code splitting allows developers to break application code into smaller bundles, loading only essential parts upfront to enhance performance. This technique, often implemented with Webpack, delays loading non-critical code, improving initial load times. For applications with large codebases or media-rich content, code splitting conserves resources and enhances user experience. Advanced React training covers code-splitting strategies that enable developers to build scalable applications without sacrificing performance.
Monitoring and Profiling with React DevTools Profiler
Effective performance optimization requires real-time insights. The React DevTools Profiler lets developers track component render times, pinpointing slow re-renders and potential bottlenecks. By diagnosing issues based on render patterns, the Profiler helps developers make targeted improvements. This tool is essential for isolating and troubleshooting performance issues that can be challenging to identify, ensuring optimized performance at every stage of development.
Building High-Performance React Applications for Seamless Experience
Optimizing React applications goes beyond basic React skills. Learning and understanding techniques like React.memo, lazy loading, state management hooks, code splitting, and profiling with React DevTools equips developers to build efficient, high-performance applications. Web Age Solutions’ advanced React training empowers developers with these skills, helping them create scalable applications that meet the demands of today’s user expectations.
For more information visit: https://www.webagesolutions.com/courses/react-training
0 notes
Text
What's New in React.js: Key Features and Updates in React 19 (October 2024)
React.js Updates (October 2024)
1. React 19 Release
The long-awaited React 19 has launched with several significant updates designed to improve both developer experience and application performance:
· Enhanced integration with web components
· Simplified document metadata management
· Optimized background asset loading
These changes promise to make React applications faster, more efficient, and easier to manage for developers.
2. Improved Server Components
React 19 continues to improve its server component architecture, allowing components to be rendered on the server before reaching the client. This results in:
· Faster load times
· Better SEO, as pages are more optimized for search engines
By moving heavy lifting to the server, React 19 ensures smoother user interactions and better search engine indexing.
3. New Document Head Management
React 19 introduces the <DocumentHead> component, streamlining the process of managing SEO-related tags such as titles and meta descriptions. This update makes it easier for developers to:
· Maintain consistent branding across pages
· Improve SEO practices without repeating the same code
This is a key improvement for developers focused on SEO optimization.
4. Background Asset Loading
With React 19, asset loading has been optimized to load images and other resources in the background while users are interacting with the current page. This feature:
· Reduces waiting times
· Improves the overall user experience, especially during page transitions
By preloading resources, React ensures smoother and faster interactions on the web.
5. Enhanced Hooks
The update to hooks in React 19 provides developers with more control over when and how components update. This means:
· Cleaner, more efficient code without relying on some previously necessary hooks like useMemo
· Better performance and simpler management of application state
These enhancements make the codebase easier to maintain and optimize.
At the React Conf 2024, several sessions highlighted these advancements and demonstrated how React 19’s new features can be used to build faster, more efficient apps. Experts shared their insights on server components, hooks, and other major updates that are shaping the future of web development.
In Summary:
React 19 brings a host of improvements that make web development more efficient and user-friendly:
· Faster load times
· Better SEO
· More control over code with enhanced hooks and streamlined workflows
With these new features, React continues to solidify its position as one of the leading frameworks for modern web development.
This version excludes external references, focusing solely on the key highlights of React 19 and its impact on web development.
Stay Connected with NetBots
Follow NetBots on social media to learn more:
Facebook
Instagram
Pinterest
X (Twitter)
LinkedIn
#software company#information tecnology#digital marketing#web development#designing#netbotstech#netbots#skardu
0 notes
Text
Top 10 React JS Interview Questions for 2025
As the demand for skilled React developers continues to rise, it's essential to prepare for interviews effectively. As you prepare for interviews in 2025, it's vital to stay updated with key concepts and best practices. This blog covers the essential React JS interview questions and answers, perfect for those looking to enhance their skills through React JS training.
1. What are the main features of React?
React is known for its component-based architecture, virtual DOM for optimized rendering, one-way data binding, and hooks for managing state and side effects. These features make it a powerful choice for building dynamic web applications.
2. Explain the concept of Virtual DOM.
The Virtual DOM is a lightweight copy of the actual DOM. React updates the Virtual DOM first and then efficiently syncs the changes to the real DOM. This process minimizes direct manipulations, resulting in faster updates and improved performance.
3. What are hooks in React?
Hooks are functions that let you use state and other React features without writing a class. Key hooks include `useState`, `useEffect`, and `useContext`, which facilitate managing state and side effects in functional components.
4. How do you manage state in React?
State in React can be managed using the `useState` hook for local component state or by using libraries like Redux or Context API for global state management. Understanding when to lift state up is crucial for effective state management.
5. Can you explain the lifecycle methods of a React component?
Lifecycle methods allow you to hook into specific points in a component's life. Key methods include `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount`. With hooks, you can replicate these lifecycle methods using `useEffect`.
6. What is the purpose of keys in React?
Keys help React identify which items have changed, are added, or are removed. Using unique keys improves performance during re-renders and helps maintain component state across updates.
7. How does React handle forms?
React handles forms using controlled components, where form data is handled by the component's state. You can use the `onChange` event to update the state as users interact with the form elements.
8. Explain the difference between state and props (properties).
State is a component's local data storage, while props are used to pass data from parent to child components. Props are immutable within the child component, while state can be modified.
9. What are higher-order components (HOCs)?
HOCs are functions that take a component and return a new component, adding additional functionality. They are commonly used for code reuse, such as implementing authentication or logging.
10. Why is React considered efficient?
React's efficiency comes from its virtual DOM and reconciliation process, which minimizes the number of direct updates to the real DOM. This leads to better performance, especially in applications with dynamic content.
11. What is the Context API in React?
The Context API is a feature in React that allows you to share state across the entire application without having to pass props down manually at every level. It provides a way to create global variables that can be accessed by any component, making it ideal for theming, user authentication, or managing application settings. To use it, you create a Context object, wrap your component tree in a `Provider`, and consume the context with `useContext` or a `Consumer` component.
12. How can you optimize performance in a React application?
-Performance optimization in React can be achieved through several techniques, including:
-Code Splitting: Use dynamic imports to split your code into smaller chunks, which can be loaded on demand.
-Memoization: Utilize `React.memo` for components and `useMemo` or `useCallback` for functions to prevent unnecessary re-renders.
-Pure Components: Use `PureComponent` or `shouldComponentUpdate` to prevent re-rendering when props or state haven’t changed.
-Lazy Loading: Implement lazy loading for images and components to improve initial load time.
-Efficient State Management: Avoid lifting state unnecessarily and prefer local state management when feasible.
Being well-prepared for your interview can make a significant difference in landing your desired job in React development. To further enhance your skills, consider joining the best React JS training in Trivandrum. They offer comprehensive courses that cover everything from the basics to advanced concepts.
0 notes
Text
The Importance of DOM Manipulation: Strategies and Best Practices for Businesses

DOM (Document Object Model) manipulation is the foundation of interactive web development, allowing developers to dynamically update, modify, or structure HTML documents. Whether you're building a dynamic single-page application (SPA) or creating a user-friendly e-commerce platform, efficient DOM manipulation is crucial for delivering responsive, real-time experiences.
This guide delves into the importance of DOM manipulation, key strategies businesses should adopt, and best practices that maximize performance, maintainability, and user experience. Alongside actionable tips and case studies, we’ll also explore data-driven insights to demonstrate the effectiveness of these strategies.
Why DOM Manipulation Matters
DOM manipulation involves programmatically changing the structure, content, or style of web pages. It’s central to creating interactive and engaging user experiences in modern web development. From simple tasks like dynamically updating form input values to complex operations such as rendering large datasets in real-time, DOM manipulation enables developers to:
Enhance User Experience: By enabling live updates without requiring page reloads, DOM manipulation creates smooth, uninterrupted interactions.
Improve Performance: Efficient manipulation reduces the need for unnecessary data transfers and re-renders, resulting in better application performance.
Enable Complex Functionality: DOM manipulation is essential for dynamic applications such as e-commerce sites, SPAs, and dashboards that need to respond instantly to user input.
Key Strategies for Effective DOM Manipulation
1. Understand and Optimize the DOM Tree
The DOM is structured as a tree of nodes, where every HTML element is represented as an object. Understanding this structure is critical for optimizing DOM manipulation. Each change you make to the DOM affects the tree and can lead to performance bottlenecks if not handled properly.
Best Practices:
Limit DOM Access: Frequent access to the DOM can be expensive. Retrieve DOM elements once and store them in variables for reuse.
Batch DOM Updates: Instead of making multiple small updates, group changes together and apply them all at once to minimize reflows and repaints.
Minimize Layout Thrashing: When you modify an element’s style or geometry, it forces the browser to recalculate layout and re-render the page. Reducing unnecessary style recalculations can prevent "layout thrashing."
Actionable Tip:
Use documentFragment for batch updates. Instead of inserting individual elements into the DOM one by one, use document.createDocumentFragment() to collect multiple elements and append them all at once, reducing reflows and repaints.
javascript
Copy code
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const newDiv = document.createElement("div");
newDiv.textContent = `Item ${i}`;
fragment.appendChild(newDiv);
}
document.getElementById("container").appendChild(fragment);
2. Leverage Virtual DOM for Performance-Heavy Applications
One of the most effective strategies for DOM manipulation is using a Virtual DOM—a lightweight representation of the actual DOM that libraries like React or Vue use to optimize rendering. By comparing the new state of the virtual DOM to the previous one, these libraries only update the parts of the real DOM that have changed, improving performance.
Best Practices:
Choose the Right Framework: For large-scale applications, adopting frameworks like React, Vue, or Svelte can offload the complexity of DOM updates. The virtual DOM diffing algorithm ensures that only the necessary parts of the page are re-rendered, preventing excessive updates.
Avoid Unnecessary Component Re-renders: In frameworks like React, prevent unnecessary re-renders by using React.memo or useMemo to cache the results of expensive operations.
Case Study:
A retail company optimized its e-commerce platform by switching from vanilla JavaScript DOM manipulation to React's virtual DOM. They saw a 40% reduction in page load times and a 25% increase in conversions due to improved responsiveness during peak shopping times.
3. Efficient Event Delegation
In a dynamic environment, where elements are created or removed frequently, attaching event listeners to individual elements can become inefficient and cluttered. Instead, event delegation allows you to handle events at a higher level in the DOM hierarchy by using event bubbling.
Best Practices:
Delegate Events to Parent Elements: Attach a single event listener to a parent container to manage events for multiple child elements. This reduces the number of listeners and improves performance.
Limit Event Listener Attachments: Avoid attaching multiple listeners to individual elements, especially for large datasets. Attach listeners at the parent level and use event.target to determine the clicked element.
Actionable Tip:
javascript
Copy code
document.getElementById("listContainer").addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
console.log("List item clicked: ", event.target.textContent);
}
});
4. Avoid Frequent DOM Reflows
Reflows occur when the browser recalculates the layout of a webpage due to changes in the DOM, such as adding new elements or altering the size or position of existing ones. Frequent reflows are costly in terms of performance.
Best Practices:
Use CSS Classes: Instead of modifying styles inline for each element, toggle CSS classes to apply multiple style changes at once.
Minimize Synchronous Layout Queries: Layout queries like offsetWidth, offsetHeight, or getComputedStyle can trigger reflows. Cache these values if needed repeatedly.
Actionable Tip:
If you need to update styles, batch them together in a single operation rather than applying individual changes to each element.
javascript
Copy code
const element = document.getElementById("myElement");
element.style.width = "200px";
element.style.height = "100px";
element.style.backgroundColor = "blue";
5. Asynchronous DOM Manipulation
DOM manipulation in JavaScript can block the main thread, causing performance issues if operations are too time-consuming. Asynchronous techniques like requestAnimationFrame and web workers can help mitigate this problem.
Best Practices:
Use requestAnimationFrame for Animations: When performing animations, use requestAnimationFrame to synchronize updates with the browser's refresh rate, resulting in smoother visual updates.
Offload Heavy Tasks to Web Workers: Use web workers to offload intensive tasks (like parsing large JSON objects) from the main thread, freeing up resources for DOM rendering.
Case Study:
An online learning platform reduced input lag in their dashboard by using requestAnimationFrame for DOM updates and web workers for parsing large student datasets. This resulted in a 35% improvement in performance during peak user hours.
Best Practices for DOM Manipulation in Business Applications
1. Optimize for Mobile Performance
With mobile traffic surpassing desktop, it's crucial to optimize DOM manipulation for mobile devices. Mobile browsers have limited resources, so excessive DOM manipulation can severely impact performance.
Best Practice:
Minimize DOM Size: Avoid generating large, deeply nested DOM structures. Mobile devices struggle with rendering and reflowing large DOM trees, so keep the DOM lean.
2. Debounce Input Events
For performance-sensitive applications like search boxes or form inputs, frequent DOM updates on every keystroke can cause lag.
Best Practice:
Debounce Event Handlers: Use debouncing to limit how frequently event handlers are called, particularly for input events like key presses.
javascript
Copy code
function debounce(func, delay) {
let timeoutId;
return function(...args) {
if (timeoutId) clearTimeout(timeoutId);
timeoutId = setTimeout(() => func(...args), delay);
};
}
document.getElementById("search").addEventListener("input", debounce(handleSearch, 300));
3. Lazy Load DOM Elements
Loading all elements at once can impact performance, especially on long pages or applications with dynamic content. Lazy loading allows you to defer the creation of DOM elements until they are needed, reducing initial load time.
Best Practice:
Implement Lazy Loading: Use techniques such as lazy loading for images, videos, and long lists. For dynamic content, only load elements when they enter the viewport using Intersection Observer.
Data-Driven Insights
Performance Impact: According to Google’s web performance research, sites that reduce DOM manipulation and optimize reflows see a 15-20% reduction in page load times and a corresponding 10-30% improvement in user engagement.
Conversion Rates: A case study by Walmart demonstrated that reducing page load time by one second led to a 2% increase in conversions. Optimized DOM manipulation contributed to this improvement by streamlining interactive elements.
User Satisfaction: Research from Akamai found that 53% of mobile users abandon sites that take more than three seconds to load. Efficient DOM manipulation plays a critical role in keeping load times fast, even as web apps become more complex.
Conclusion: Mastering DOM Manipulation for Business Success
DOM manipulation is a cornerstone of modern web development, and mastering it is essential for businesses aiming to deliver fast, dynamic, and engaging user experiences. By understanding the structure of the DOM, leveraging virtual DOM techniques, and optimizing event handling, businesses can boost performance and user satisfaction. From reducing layout thrashing to implementing efficient event delegation, the strategies outlined in this guide help businesses create scalable and maintainable applications. When applied thoughtfully, these best practices not only improve performance but also enhance collaboration among development teams, leading to better long-term outcomes.
0 notes
Text
Exploring React Hooks: A Practical Guide to Functional Components
Introduction
React Hooks revolutionized the way developers write React components by introducing a more functional approach to state management and side effects. In this comprehensive guide, we will explore React Hooks in depth, covering their benefits, use cases, and practical examples to help you harness the power of functional components in your React applications.
What are React Hooks?
React Hooks are functions that enable you to use state and other React features in functional components. They allow you to reuse stateful logic across components without changing the component hierarchy. Some key React Hooks include useState, useEffect, useContext, and useReducer, each serving a specific purpose in managing component state and side effects.
Benefits of React Hooks
Simplified Logic: Hooks enable you to encapsulate and reuse complex logic in functional components, leading to cleaner and more maintainable code.
Improved Readability: By removing the need for class components and lifecycle methods, Hooks make code easier to read and understand.
Better Performance: Functional components with Hooks can optimize re-renders and improve performance compared to class components.
Code Reusability: Hooks promote code reusability by allowing you to extract and share logic across multiple components.
Practical Examples of React Hooks
useState: Manage component state in functional components.
const [count, setCount] = useState(0);
useEffect: Perform side effects in functional components.
useEffect(() => { // side effect code }, [dependency]);
useContext: Access context values in functional components.
const value = useContext(MyContext);
useReducer: Manage more complex state logic in functional components.
const [state, dispatch] = useReducer(reducer, initialState);
Best Practices for Using React Hooks
Keep Hooks at the Top Level: Ensure that Hooks are called at the top level of your functional components, not inside loops, conditions, or nested functions.
Follow Naming Conventions: Prefix custom Hooks with "use" to distinguish them from regular functions.
Separate Concerns: Split logic into separate custom Hooks for better organization and reusability.
Optimize Performance: Use the useMemo and useCallback Hooks to optimize performance by memoizing values and functions.
Conclusion
React Hooks have transformed the way developers approach state management and side effects in React applications, offering a more functional and concise alternative to class components. By mastering React Hooks like useState, useEffect, useContext, and useReducer, you can enhance the efficiency, readability, and maintainability of your codebase. Whether you're a seasoned React developer or just starting out, exploring React Hooks is a valuable journey towards building more robust and scalable functional components in your projects. For advanced implementations or assistance with React development, consider partnering with a reputable React.js development company or hire React.js developers to elevate your applications to the next level.
0 notes
Text
How Do React Hooks Use the Push Method?
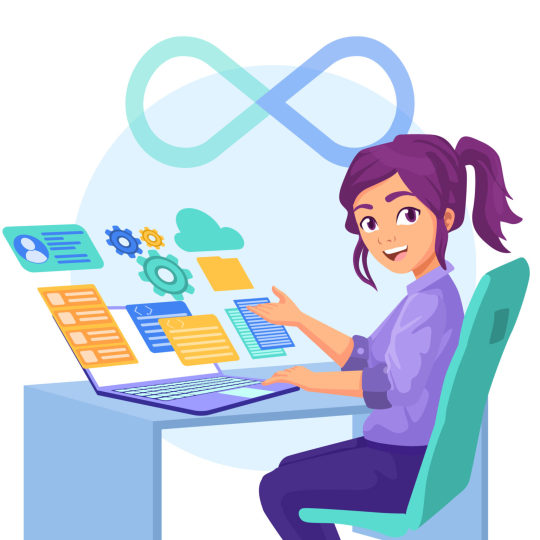
The push () method accepts an array and returns a new length after adding one or more members to the end of the array. The push method typically aids in adding the value to the array. It is a practical way to assign one or more key-value pairs to the object using bracket notation.
Using the push method makes assigning the value simple. These choices work well for adding the item to the array’s end. React hooks enable minimal code that may be used to quickly and easily create comparable features. These are good choices for using the lifecycle method in combination with the React state, even without using the writing class.If you need to integrate the Push method in your React apps then you need to hire React experts who will simply integrate this functionality in your app.
What are React Hooks?
React Hooks are a type of function that lets users connect function components to react state and lifecycle elements. UseState, useEffect, useReducer, useRef, use Callback, useContext, and useMemo are just a few of the built-in hooks that React offers. We can also design your own custom hooks.
As of React version 16.8, hooks for React are accessible. Before the introduction of hooks, only the class component—not the functional component—was able to preserve state. After the introduction of hooks, the functional component’s state can also be maintained.
Using all of React’s features, you may develop fully functional components by implementing the hooks concept. Unlike classes, this enables us to make everything simpler.
Advantages of React Hooks
Improving the Component Tree’s Readability
As it makes it possible to read context information outside of JSX, the “useContext” hook has been an invaluable tool for significantly increasing the readability of JSX. Although “useContext” makes it even cleaner, the static “contextType” assign in class components made this possible in the past.
Not only is the code easier to read, but using the React dev tools to debug makes it much simpler to read the component tree. This is really beneficial for components that were previously using many nested contexts.
Incorporating Adverse Effects
It was possible to add event listeners in “componentDidMount” and remove them later in “componentWillUnmount” for class components, which divided the setup and removal of side effects across several lifecycle methods. Any component with several side effects may result in code that is harder to read and has related functionality distributed over a number of disjointed lifecycle methods.
However, the “useEffect” handles side effect creation and breakdown, which fixes this issue. In order to accomplish this, it lets the effect function return a function that splits down the effect.
Acceptable and Reusable Logic
Undoubtedly, custom hooks are an excellent way to share functionality between different components. A custom hook is basically a function that, like a regular hook, can be called within a functional component and utilizes one or more React hooks.
Must You Include The Push Method?
The call() or apply()objects, which have a distinctive resemblance to arrays, are usually utilized in conjunction with the Push method. The push approach, in particular, depends on the length of the property, which makes it simple to choose which feature is best for beginning to insert values to a certain extent.
The index is set to 0 when the length property is not translated to a numerical value. In particular, it covers the potential for length with the nonexistent. In the process, length cases will be produced.
As strings are native, array-like objects are not appropriate choices for use in applications. These strings offer an appropriate solution to perfection and are unchangeable. Object parameters that resemble arrays are a good method for quickly figuring out the whole plan of action for excellence.
Hiring React js developers is a quick and hassle-free choice if you want to add the push function to your React hooks. React useState() hooks are required to update the array; they do not include the push() method for the Array object. In this process, the spread operator is a very practical choice.
push(element0)
push(element0, element1)
push(element0, element1, /* ... ,*/ elementN)
How Can I Use UseState() To Create An Array State?
The new length property of the object is included in the return value, which makes it easier to generate the Array state using useState(). To enable the array state variable, you must use the useState() hook.
import React from "react";
const { useState } = React;
const [myArray, setMyArray] = useState([]);
The variable containing the state array and the special technique for quickly updating each property are returned by the useState() hook. Without the useState() method, updating the array is typically very challenging.
It is quite convenient to add the new element to the State Array. It would be quite easy to add a new member to the array when this state is present.
myArray.push(1)
It’s a great option to use the best method return from useState while utilizing React to update the array with ease. Even with the new array that is formed by merging the old array with the new members in the JavaScript Spread operator, the update method, or setMyArray(), is useful for modifying the state. Using the useState update function makes it easy to create the new array from the old array.
setMyArray(oldArray => [...oldArray, newElement]);
It is convenient to utilize the first technique, and the function is enabled with the old array as the first parameter. Better access to older arrays containing state objects is also provided.
onChange = value => checked => {
this.setState({ checked }, () => {
this.setState(prevState => {
Object.assign(prevState.permission, { [value]: this.state.checked });
});
});
};
<CheckboxGroup
options={options}
value={checked}
onChange={this.onChange(this.props.label)}
/>
Adding The Array In React State Using Hooks
The.concat() function is useful for producing the speedy updating even without any problem, however the.push() function does not work well with updating the state in React app development. The spread operator makes it simple to enable JavaScript array state. In the React state, there are numerous methods for quickly adding an item to the array.
Pushing the item into the array is not a convenient choice because it is not quite as convenient to change the state directly. Using React Hooks to update state is made simple by the array’s React state. It’d be far more practical to store JavaScript objects with the array in React state.
1. The push() method adds new elements to the array’s end.
2. The push() method modifies the array’s length.
3. The push() function returns a new length.
For instance, useState is a hook that is enabled with functional components that make it simple to add additional functionality for the local state. React seems like a good choice for maintaining state in between renders.
The command useState([]) would automatically initialize the state to contain an empty array because these are known as the useState Hooks. The array is shown and is a suitable parameter for passing useState().
import React, { useState } from "react"
import ReactDOM from "react-dom"
function App() {
// React Hooks declarations
const [searches, setSearches] = useState([])
const [query, setQuery] = useState("")
const handleClick = () => {
// Save search term state to React Hooks
}
// ...
Pass a callback to the function that creates a new array in the state setter.
To add the new element to the end of the state array, we can pass in a callback that produces a new array. This would update the state array.
import React, { useState } from "react";
export default function App() {
const [arr, setArr] = useState(["Demo"]);
return (
<div classname="App">
<button onclick="{()" ==""> setArr((oldArray) => [...oldArray, "Demo"])}>
Click Here
</button>
<div>
{arr.map((a, i) => (
<p key="{i}">{a}</p>
))}
</div>
</div>
);
}
When using React Hooks, why does.Push() not work?
These are known as the reducers, and they are typically enabled by using the setSearches() major method. For updating the passed value’s current state, it is a handy alternative. TypeError, the search engine, is used to update these features with a number from an array. As the array’s method and state are enabled by .push, the state of [] replaces for the Map React state, and .length equals 1.
JavaScript does not apply code 1.map() when the.map() functioned.
The setSearches state setter or reducer function from React Hooks is a very practical way to update the state without altering the previous one. The rescue operation is taking place at Array. prototype.Concat() is a function that is used in push().
Reason.Concat() creates a new array in order to update the state. The old array would remain fully intact automatically. They would inevitably offer a higher return on the modified array.
setSearches([query].concat(searches)) // prepend to React State
To get the combined arrays quickly, you can use a JavaScript spread operator. In the React state, they are used to add the item to the array.
[...searches, query] to add a new entry to the array's end
To prepend an item to the front of the array, use [query,...searches].
// Save search term state to React Hooks with spread operator and wrapper function
// Using .concat(), no wrapper function (not recommended)
setSearches(searches.concat(query))
// Using .concat(), wrapper function (recommended)
setSearches(searches => searches.concat(query))
// Spread operator, no wrapper function (not recommended)
setSearches([...searches, query])
// Spread operator, wrapper function (recommended)
setSearches(searches => [...searches, query])
Implement the State Setter Function
It is possible to give a callback into the state setter function that useState returns, which takes the old value of a state and produces a new one.
We only need to return a duplicate of the array in order to add the member we want to push to the end.
import { useState } from "react";
export default function App() {
const [arr, setArr] = useState([0]);
return (
<div><button> setArr((arr) => [...arr, arr[arr.length - 1] + 1])} > push </button> {JSON.stringify(arr)}</div>
); }
Conclusion
React component state arrays can be updated with new items at the end by sending a callback to the state setter function, which receives the old array value and returns the new and latest array value. As we saw above, we can modify the state of an array in React by using the Push function, specifically the useState hook. Incorporating new elements to JavaScript arrays is appropriate when using these methods. For integrating the React applications in the Push Method, you need to hire React Expert from a reputable React app development company in the USA.
Need More Information? Contact Us Today.
The React Company : Your Partner in React Excellence.
0 notes
Text
Title: Exploring Hooks: How to Supercharge Your React Components with Achieversit
Introduction: Since its introduction in React 16.8, hooks have revolutionized the way developers write React components, offering a more concise and intuitive way to manage state and side effects. In this guide, Achieversit takes you on a journey to explore the power of hooks and how they can supercharge your React components. Whether you're a beginner or an experienced React developer, mastering hooks is essential for building modern and efficient React applications.
Understanding Hooks: Achieversit starts by introducing the concept of hooks in React.js, explaining how they allow you to use state and other React features without writing class components. We'll explore the useState and useEffect hooks, discussing their syntax, usage, and common use cases.
Leveraging useState: The useState hook enables functional components to manage local state, eliminating the need for class components. Achieversit demonstrates how to use useState to initialize and update state variables, handle user input, and manage complex state logic within your React components.
Embracing useEffect: The useEffect hook provides a way to perform side effects in functional components, such as fetching data, subscribing to events, or updating the DOM. Achieversit dives deep into useEffect, exploring its various use cases, dependencies, cleanup mechanisms, and best practices for managing side effects in React components.
Custom Hooks: Custom hooks allow you to extract and reuse logic across multiple components, promoting code reusability and maintainability. Achieversit showcases how to create custom hooks to encapsulate complex logic, abstract common patterns, and share stateful logic between components effectively.
useContext Hook: The useContext hook provides a convenient way to consume context within functional components, enabling you to access global state or configuration settings without prop drilling. Achieversit demonstrates how to use useContext to access context values and update context state within your React components.
useRef Hook: The useRef hook allows you to create mutable references to DOM elements or values that persist across re-renders, without causing a component to re-render. Achieversit explores the useRef hook, showcasing its use cases for accessing DOM elements, managing focus, implementing timers, and optimizing performance in React components.
useMemo and useCallback Hooks: The useMemo and useCallback hooks are used to optimize performance by memoizing expensive computations and preventing unnecessary re-renders. Achieversit explains how to use useMemo and useCallback to optimize function components, improve rendering performance, and avoid unnecessary computations in React applications.
Conclusion: By mastering hooks, Achieversit empowers developers to write cleaner, more concise, and more maintainable React components. Whether you're building simple UI components or complex application logic, understanding how to leverage hooks effectively is essential for unlocking the full potential of React.js and building modern, high-performance web applications.
0 notes